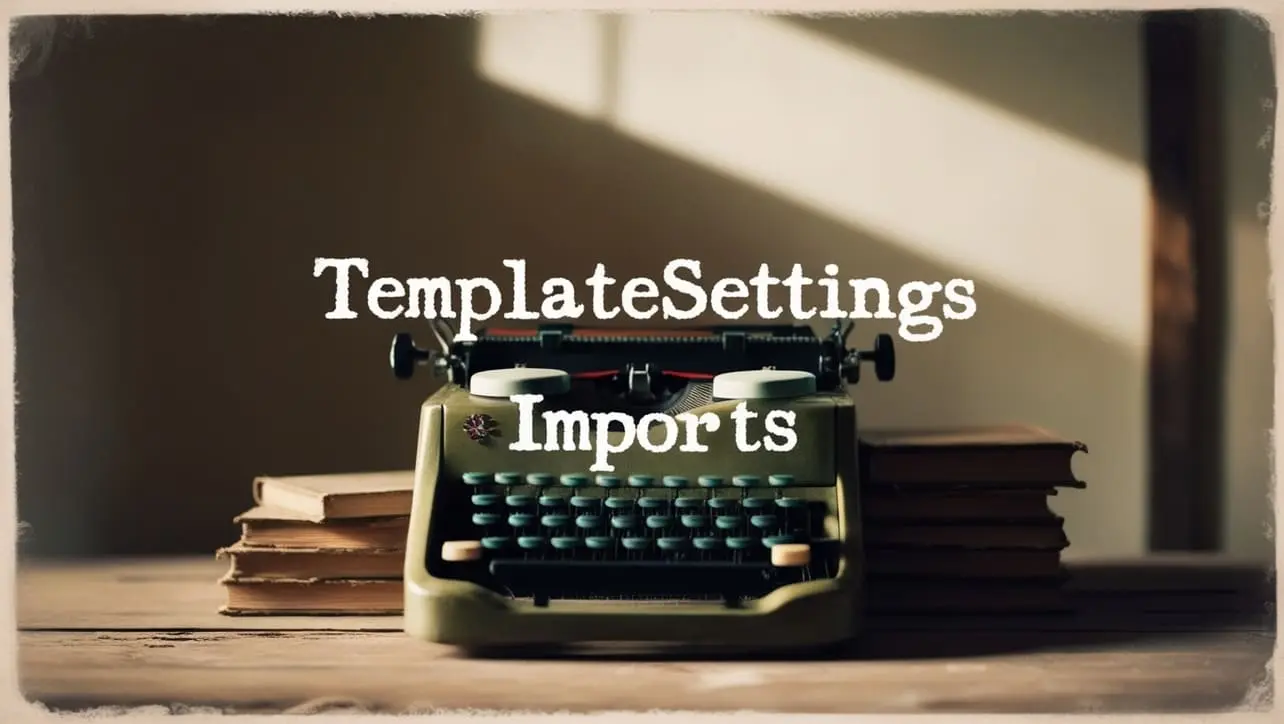
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.pickBy() Object Method
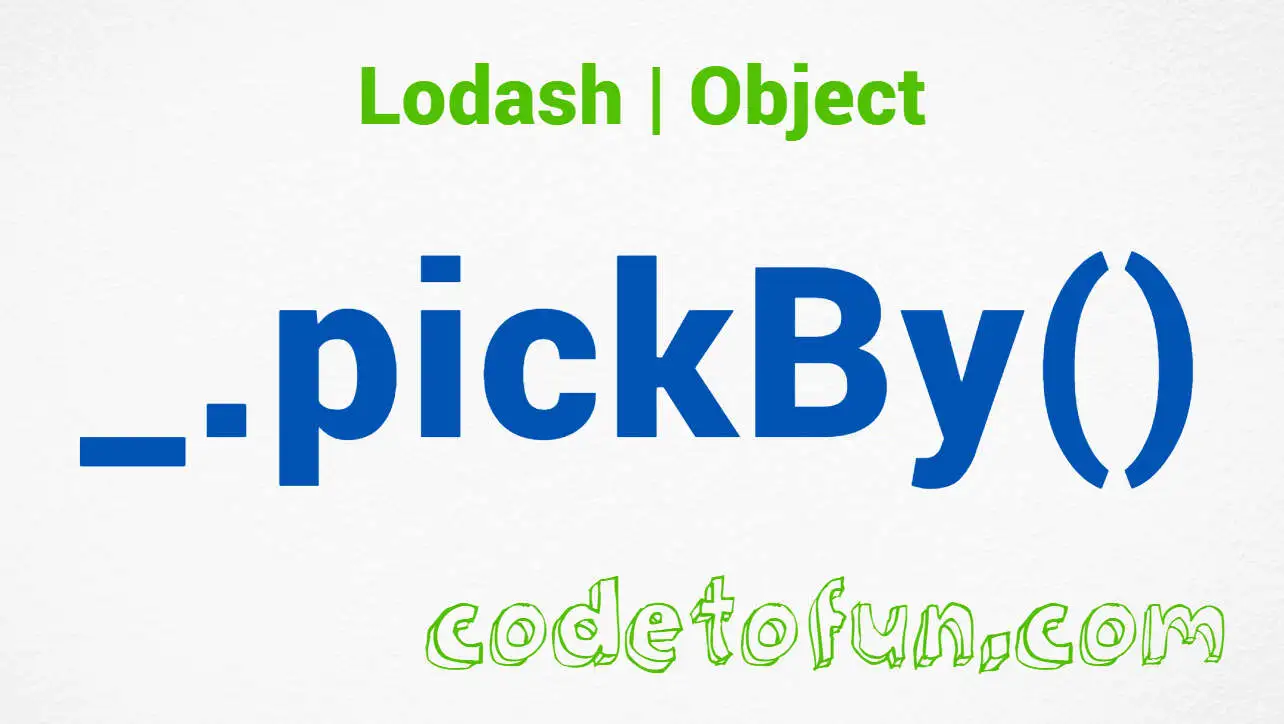
Photo Credit to CodeToFun
🙋 Introduction
When working with JavaScript objects, selectively extracting properties based on specific criteria is a common requirement. Lodash, a popular utility library, offers a variety of functions to streamline object manipulation tasks. Among these functions is _.pickBy()
, a powerful method that allows you to filter object properties based on a provided predicate function.
This method enhances code readability and reduces boilerplate, making it invaluable for developers dealing with complex data structures.
🧠 Understanding _.pickBy() Method
The _.pickBy()
method in Lodash is designed to extract object properties that satisfy a given predicate function. This enables developers to selectively choose properties based on their values or other criteria, offering flexibility and control over object manipulation.
💡 Syntax
The syntax for the _.pickBy()
method is straightforward:
_.pickBy(object, [predicate])
- object: The object to process.
- predicate (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.pickBy()
method:
const _ = require('lodash');
const user = {
name: 'Alice',
age: 30,
isAdmin: true,
isActive: false,
};
const activeUser = _.pickBy(user, value => value === true);
console.log(activeUser);
// Output: { isAdmin: true }
In this example, the user object is processed by _.pickBy()
to extract properties with truthy values, resulting in a new object containing only active properties.
🏆 Best Practices
When working with the _.pickBy()
method, consider the following best practices:
Define Clear Predicates:
Ensure that the predicate function used with
_.pickBy()
clearly defines the criteria for selecting properties. This enhances code readability and makes the intent of property selection explicit.example.jsCopiedconst sportsEquipment = { ball: 'football', racket: 'tennis', skates: null, }; const validEquipment = _.pickBy(sportsEquipment, value => value !== null && value !== undefined); console.log(validEquipment); // Output: { ball: 'football', racket: 'tennis' }
Handle Complex Predicates:
Handle complex predicate logic with ease by leveraging the flexibility of arrow functions or custom predicate functions. This allows you to address specific edge cases and tailor property selection according to your requirements.
example.jsCopiedconst inventory = { apple: { quantity: 5, price: 2 }, banana: { quantity: 10, price: 1 }, orange: { quantity: 0, price: 3 }, }; const inStockItems = _.pickBy(inventory, item => item.quantity > 0); console.log(inStockItems); // Output: { apple: { quantity: 5, price: 2 }, banana: { quantity: 10, price: 1 } }
Performance Considerations:
Consider the performance implications when working with large objects or complex predicate functions. Optimize your code to ensure efficient property selection and processing, especially in performance-sensitive applications.
example.jsCopiedconst largeObject = /* ...fetch data from API or elsewhere... */; const selectedProperties = _.pickBy(largeObject, (value, key) => /* ...custom predicate logic... */); console.log(selectedProperties);
📚 Use Cases
Selective Data Extraction:
_.pickBy()
is particularly useful when you need to selectively extract data from objects based on specific criteria. This allows you to focus on relevant information and discard unnecessary properties, streamlining data processing tasks.example.jsCopiedconst userPreferences = { darkMode: true, notifications: false, fontSize: 'medium', theme: 'light', }; const activePreferences = _.pickBy(userPreferences, value => value === true); console.log(activePreferences); // Output: { darkMode: true }
Conditional Rendering:
In user interface development,
_.pickBy()
can be utilized to conditionally render components or features based on user preferences or other dynamic criteria. This enables dynamic UI customization and enhances user experience.example.jsCopiedconst featureFlags = { enableExperimentalFeature: false, showBetaBanner: true, displayNewUI: false, }; const enabledFeatures = _.pickBy(featureFlags, value => value === true); console.log(enabledFeatures); // Output: { showBetaBanner: true }
Data Transformation:
When transforming data structures,
_.pickBy()
can be employed to extract and transform specific properties, facilitating data normalization or aggregation tasks.example.jsCopiedconst rawUserData = /* ...fetch data from API or elsewhere... */ ; const transformedUserData = _.pickBy(rawUserData, (value, key) => { // Apply transformation logic based on property key or value return /* ...transformed value or condition... */; }); console.log(transformedUserData);
🎉 Conclusion
The _.pickBy()
method in Lodash offers a versatile solution for selectively extracting object properties based on specific criteria. Whether you're filtering data, customizing user interfaces, or transforming objects, this method provides a powerful tool for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.pickBy()
method in your Lodash projects.
👨💻 Join our Community:
Author
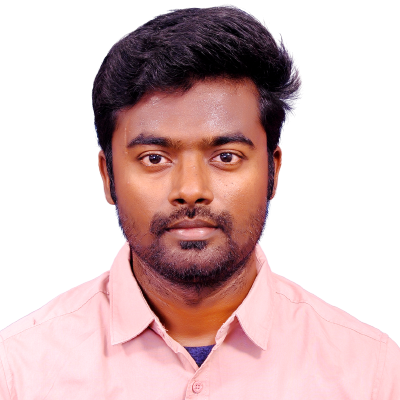
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
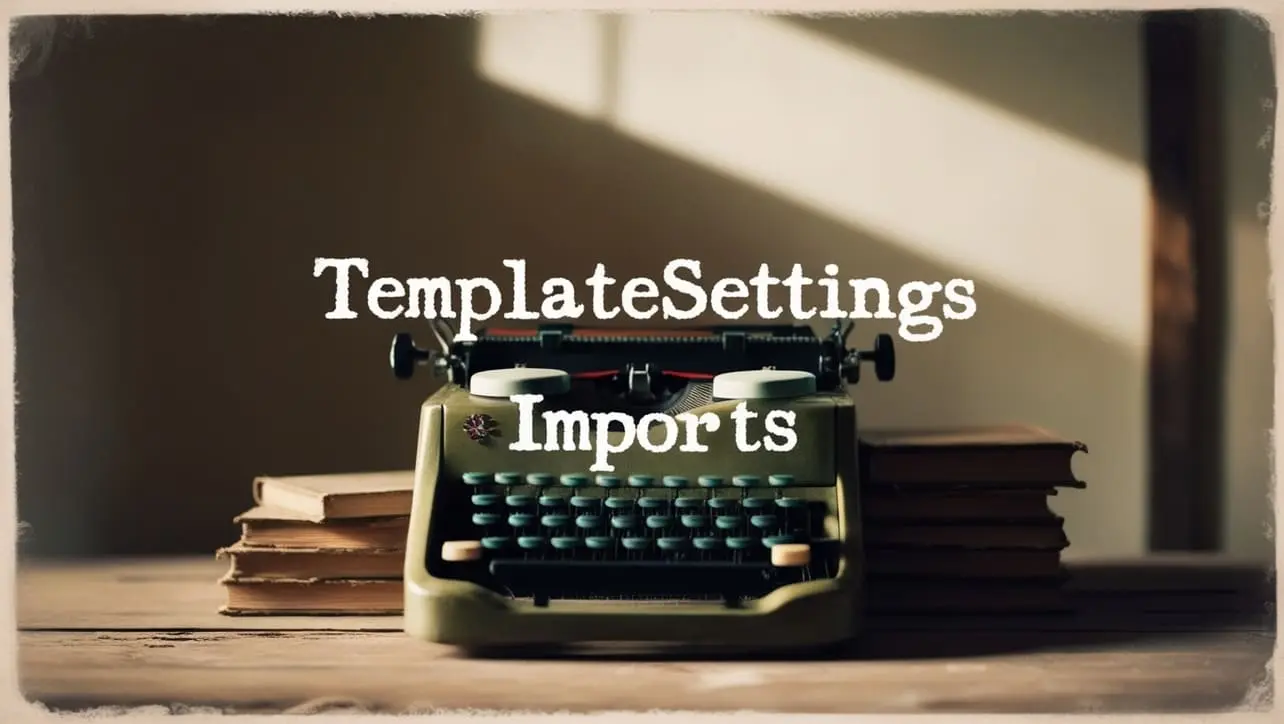
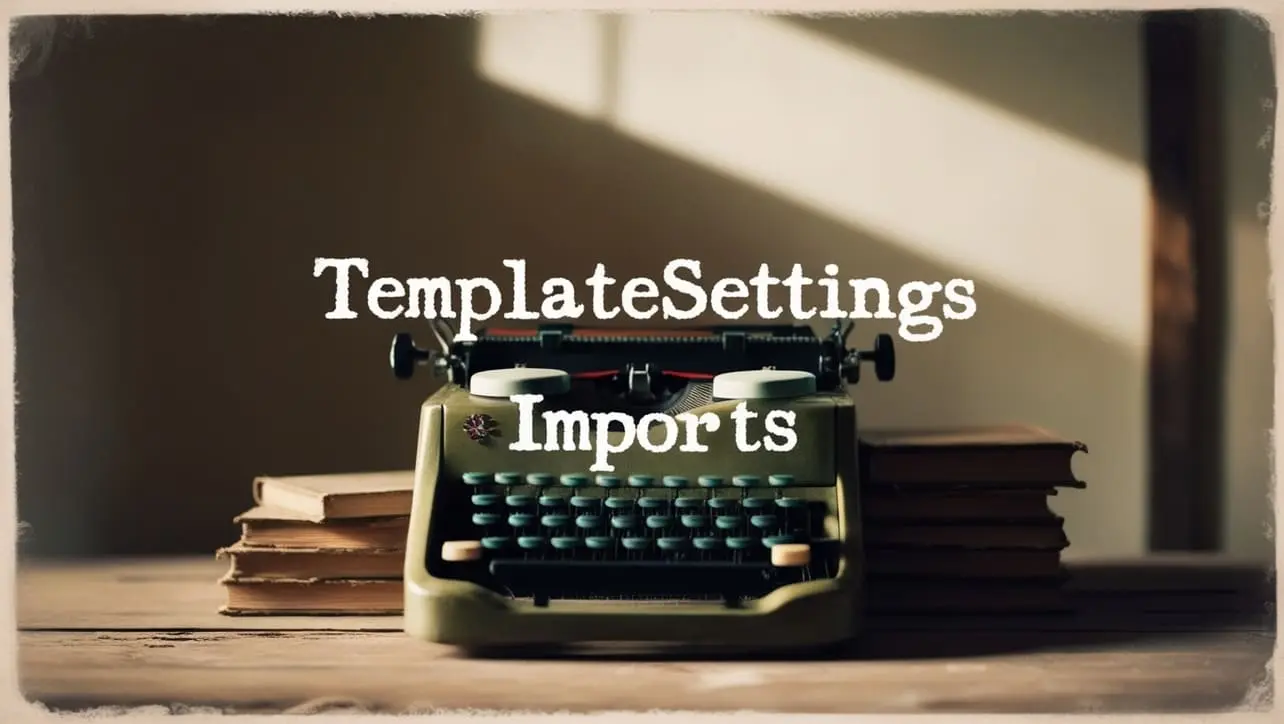
Lodash _.templateSettings.imports Property
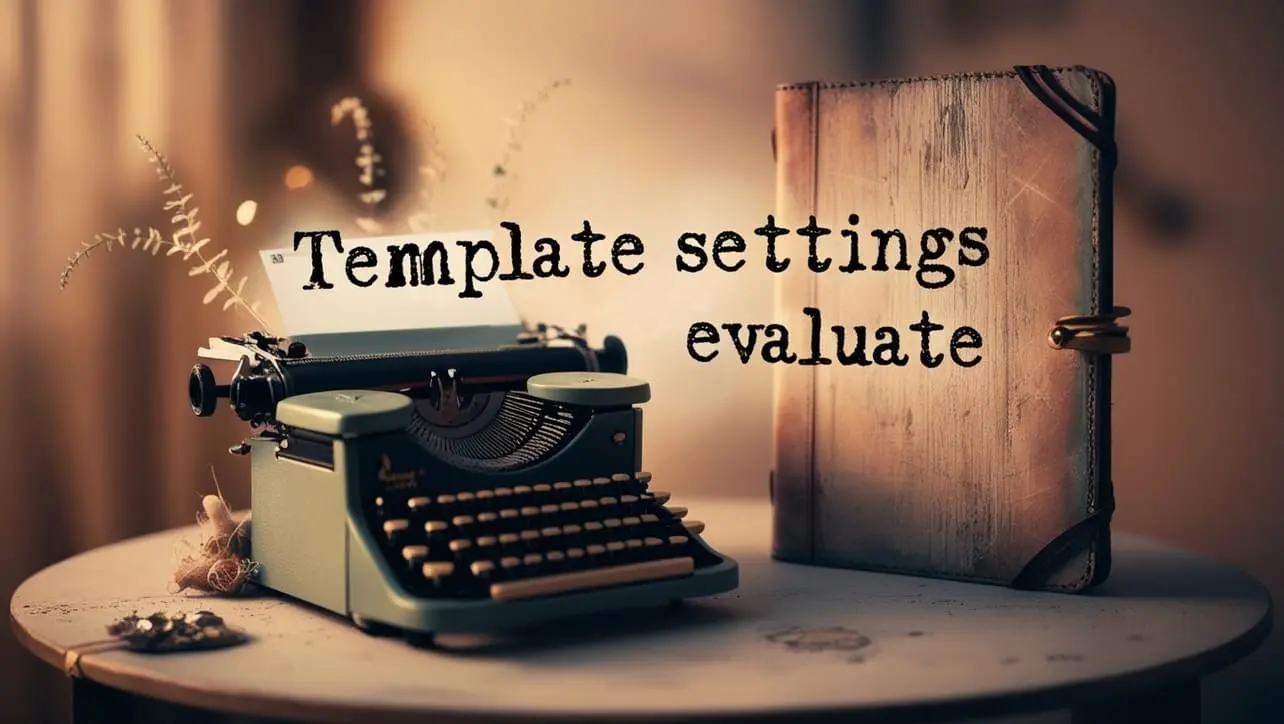
Lodash _.templateSettings.evaluate Property
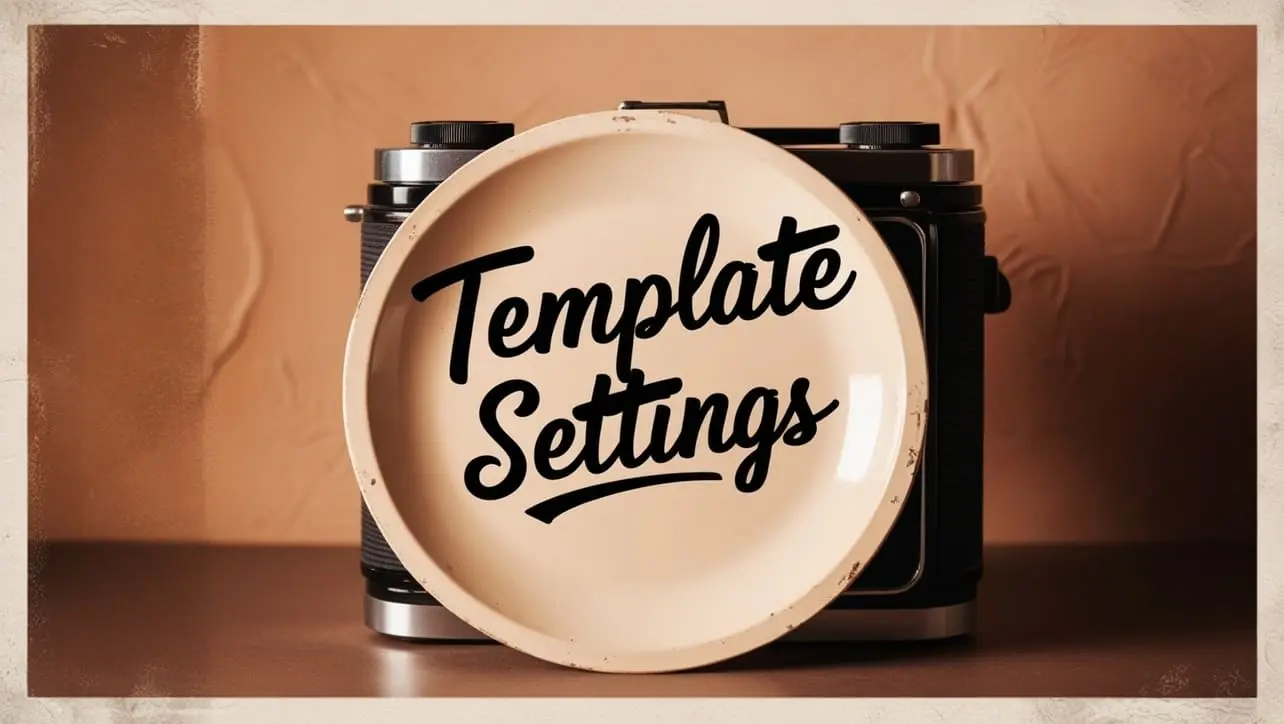
Lodash _.templateSettings Property
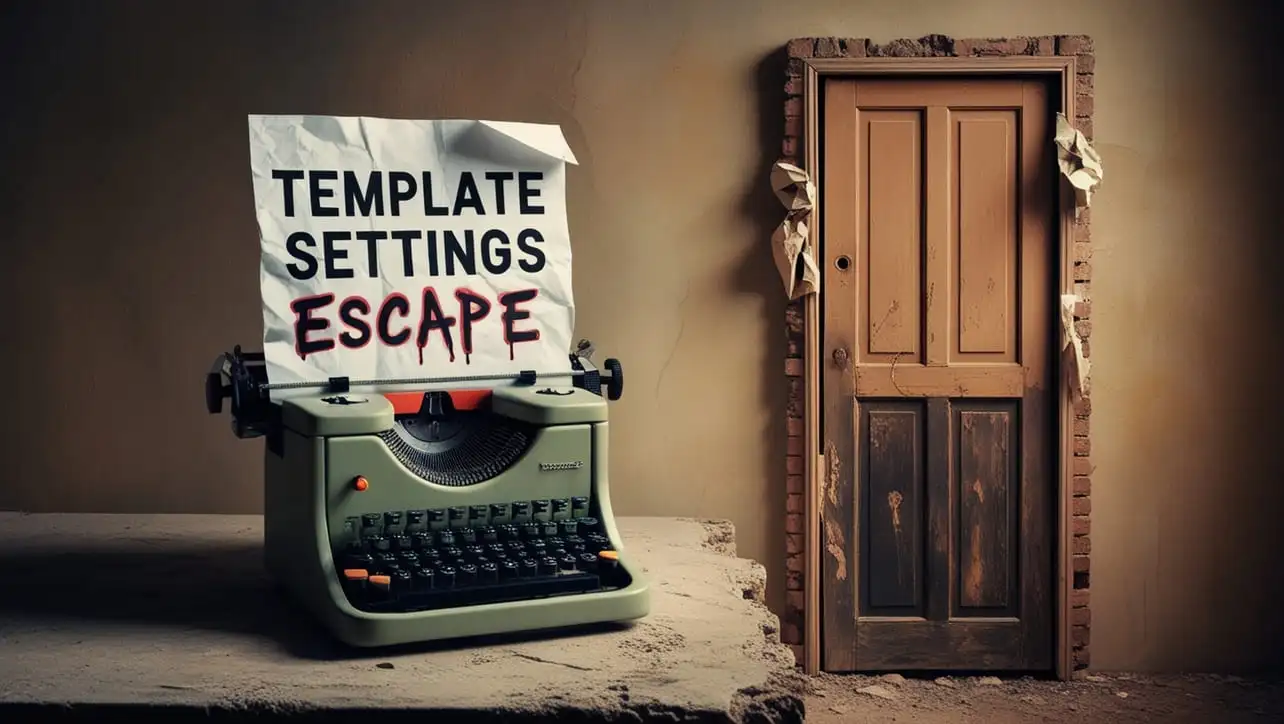
Lodash _.templateSettings.escape Property
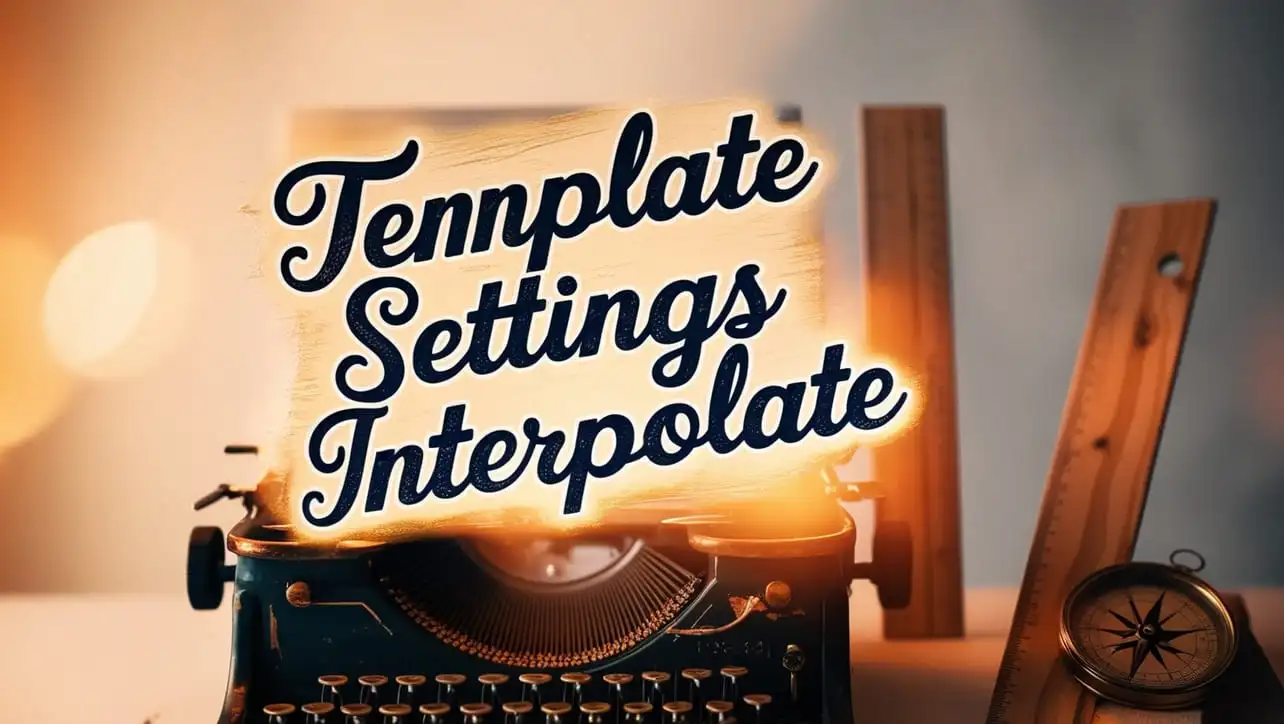
Lodash _.templateSettings.interpolate Property
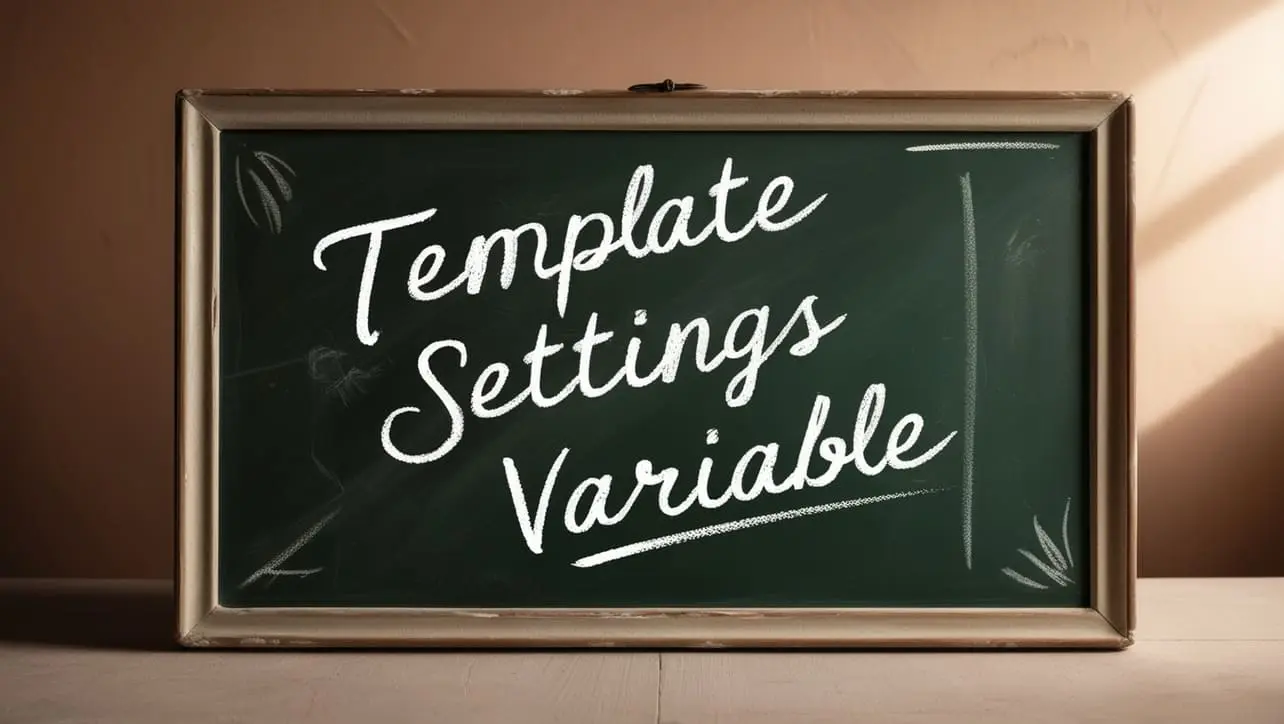
If you have any doubts regarding this article (Lodash _.pickBy() Object Method), please comment here. I will help you immediately.