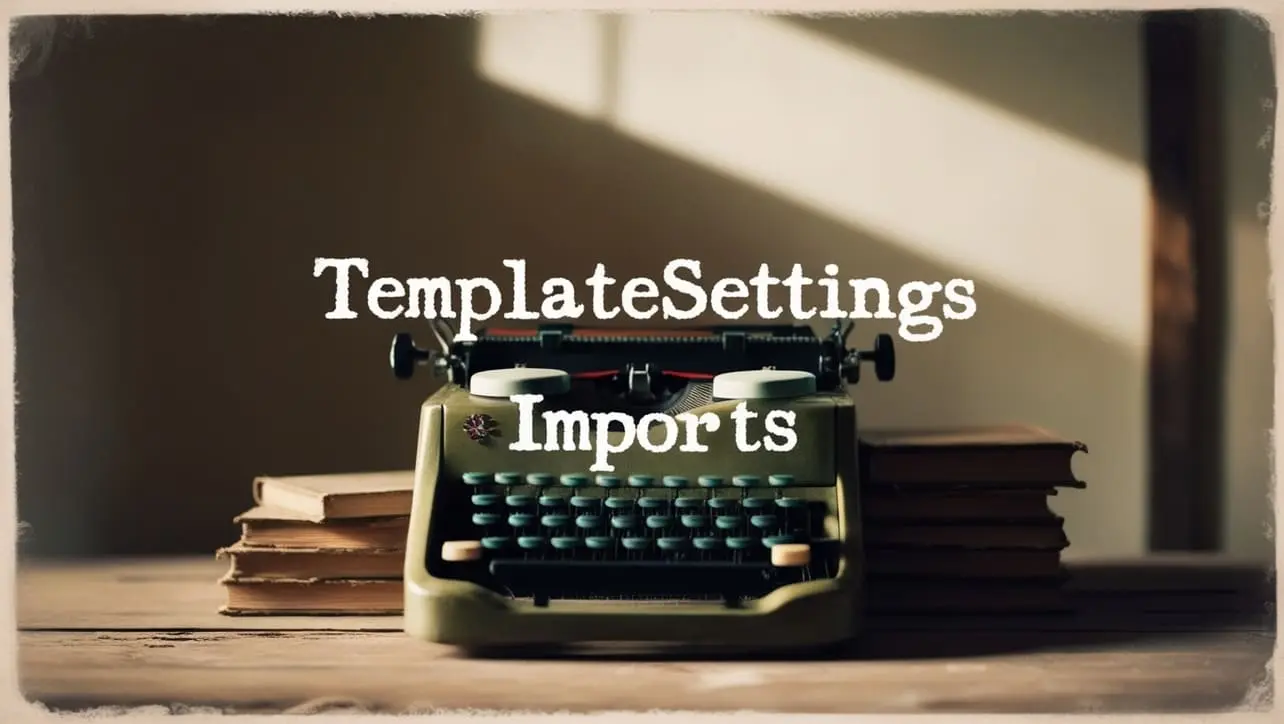
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.omitBy() Object Method
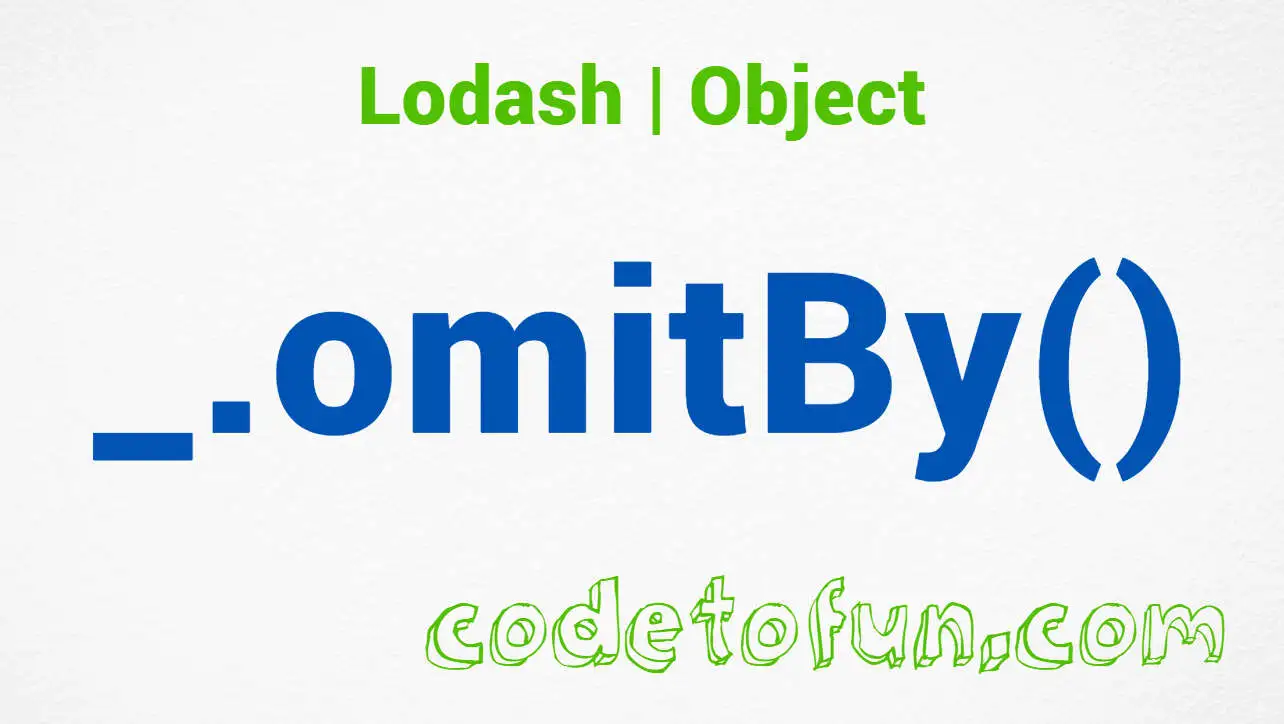
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, efficiently managing objects is essential for maintaining clean and concise code. Lodash, a comprehensive utility library, offers a plethora of functions to simplify object manipulation. Among these functions lies the _.omitBy()
method, a powerful tool for selectively omitting properties from an object based on specified conditions.
This method enhances code clarity and flexibility, making it invaluable for developers working with complex data structures.
🧠 Understanding _.omitBy() Method
The _.omitBy()
method in Lodash enables developers to create a new object by omitting properties that meet certain criteria. By providing a predicate function, developers can specify conditions under which properties should be omitted, offering granular control over object transformation.
💡 Syntax
The syntax for the _.omitBy()
method is straightforward:
_.omitBy(object, predicate)
- object: The object to process.
- predicate: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.omitBy()
method:
const _ = require('lodash');
const user = {
id: 1,
username: 'john_doe',
email: 'john@example.com',
isAdmin: true,
};
const sanitizedUser = _.omitBy(user, (value, key) => key === 'isAdmin');
console.log(sanitizedUser);
// Output: { id: 1, username: 'john_doe', email: 'john@example.com' }
In this example, the user object is processed by _.omitBy()
to create a new object sanitizedUser without the property isAdmin.
🏆 Best Practices
When working with the _.omitBy()
method, consider the following best practices:
Define Clear Predicate Functions:
Ensure that predicate functions used with
_.omitBy()
are concise and clearly define the conditions for property omission. This promotes code readability and maintainability.example.jsCopiedconst product = { id: 1, name: 'Laptop', price: 999, isAvailable: true, }; const sanitizedProduct = _.omitBy(product, (value, key) => key.startsWith('is')); console.log(sanitizedProduct); // Output: { id: 1, name: 'Laptop', price: 999 }
Consider Performance Implications:
Be mindful of performance when working with large objects or complex predicate functions. Keep predicate functions efficient to avoid unnecessary processing overhead.
example.jsCopiedconst largeObject = /* ...large object data... */; const sanitizedObject = _.omitBy(largeObject, (value, key) => /* ...complex predicate function... */); console.log(sanitizedObject);
Test Edge Cases:
Test
_.omitBy()
with various edge cases to ensure robustness and handle unexpected scenarios effectively.example.jsCopiedconst edgeCaseObject = { a: null, b: undefined, c: 0, d: '', }; const sanitizedEdgeCaseObject = _.omitBy(edgeCaseObject, value => !value); console.log(sanitizedEdgeCaseObject); // Output: { c: 0, d: '' }
📚 Use Cases
Data Sanitization:
_.omitBy()
is useful for sanitizing sensitive data by selectively omitting certain properties from an object before transmitting or storing it.example.jsCopiedconst sensitiveUserData = /* ...fetch sensitive user data... */; const sanitizedUserData = _.omitBy(sensitiveUserData, (value, key) => key.startsWith('password')); console.log(sanitizedUserData);
API Response Filtering:
When building APIs,
_.omitBy()
can be used to filter out unnecessary or sensitive information from response objects before sending them to clients.example.jsCopiedapp.get('/user/:id', (req, res) => { const userId = req.params.id; const userData = /* ...fetch user data... */ ; const sanitizedUserData = _.omitBy(userData, (value, key) => key.startsWith('password')); res.json(sanitizedUserData); });
Dynamic Object Manipulation:
In scenarios where object properties need to be dynamically manipulated based on runtime conditions,
_.omitBy()
offers a flexible solution.example.jsCopiedconst dynamicObject = /* ...generate object dynamically... */; const sanitizedObject = _.omitBy(dynamicObject, (value, key) => /* ...condition based on runtime data... */); console.log(sanitizedObject);
🎉 Conclusion
The _.omitBy()
method in Lodash empowers developers to selectively omit properties from objects based on specified conditions, enhancing code flexibility and maintainability. Whether you're sanitizing data, filtering API responses, or dynamically manipulating objects, _.omitBy()
provides a versatile tool for object transformation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.omitBy()
method in your Lodash projects.
👨💻 Join our Community:
Author
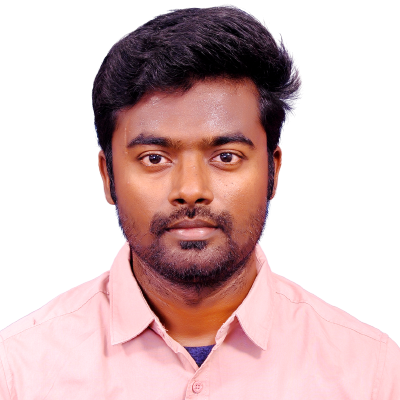
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
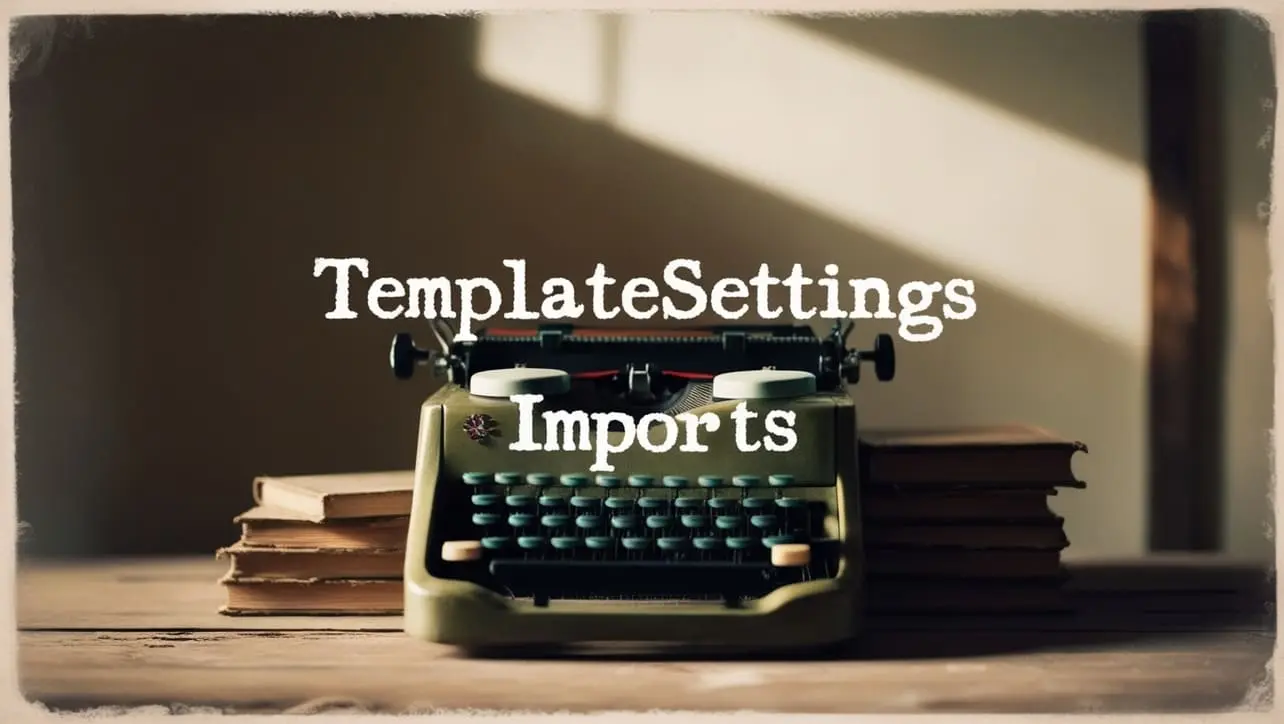
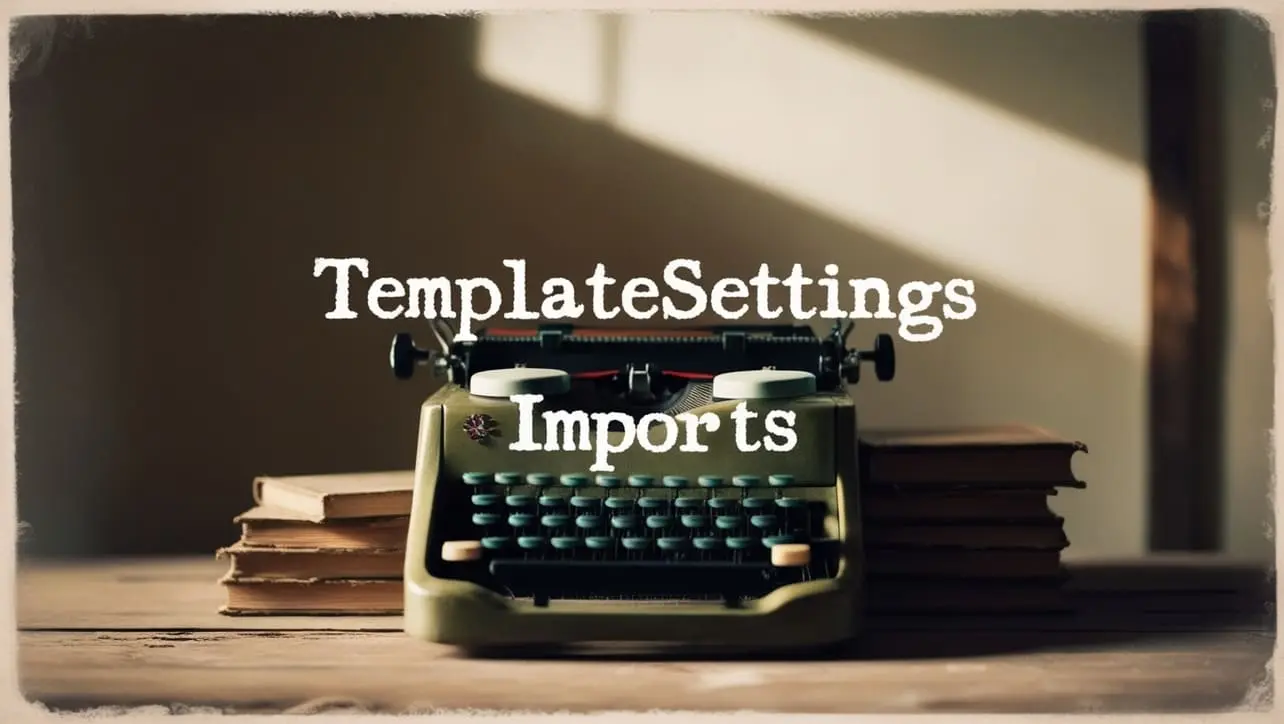
Lodash _.templateSettings.imports Property
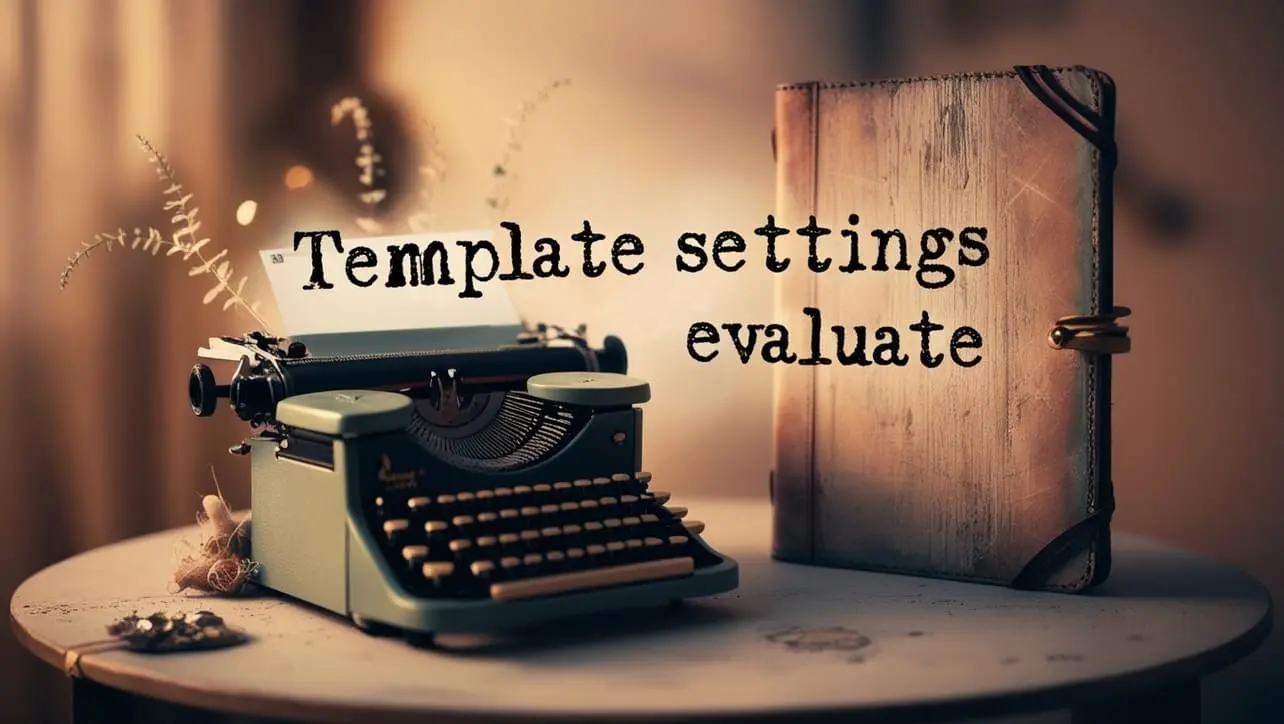
Lodash _.templateSettings.evaluate Property
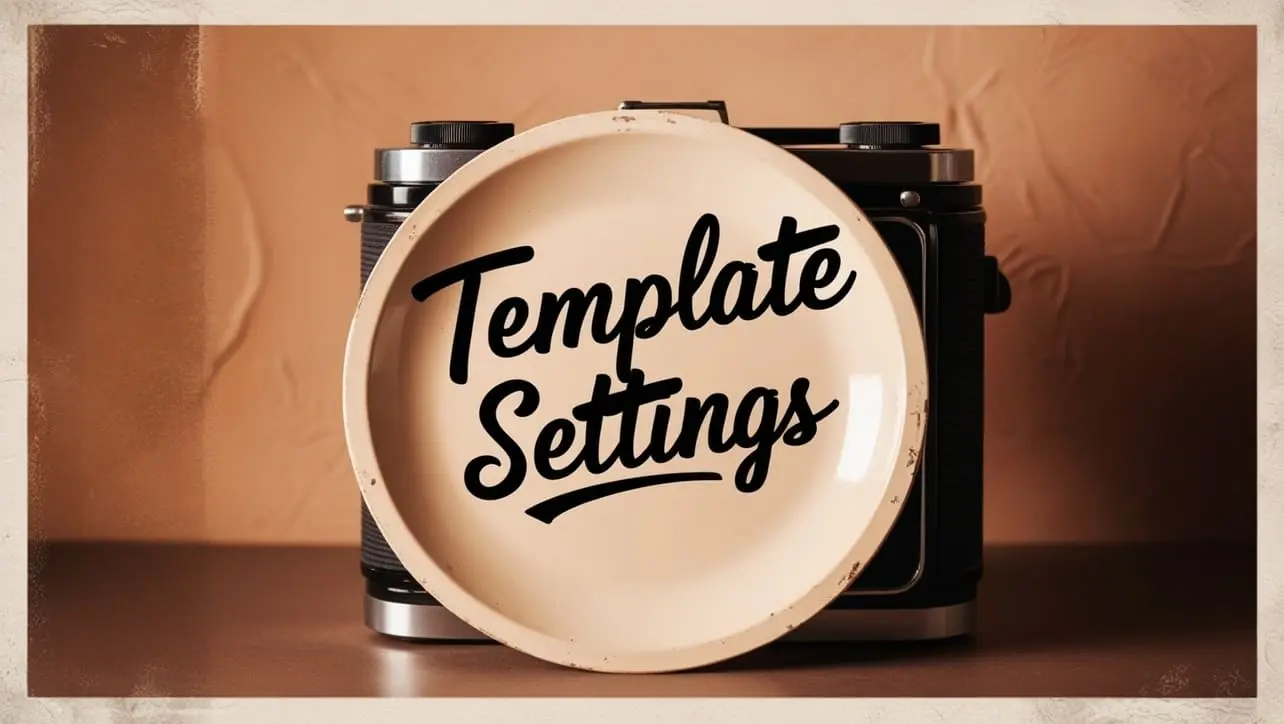
Lodash _.templateSettings Property
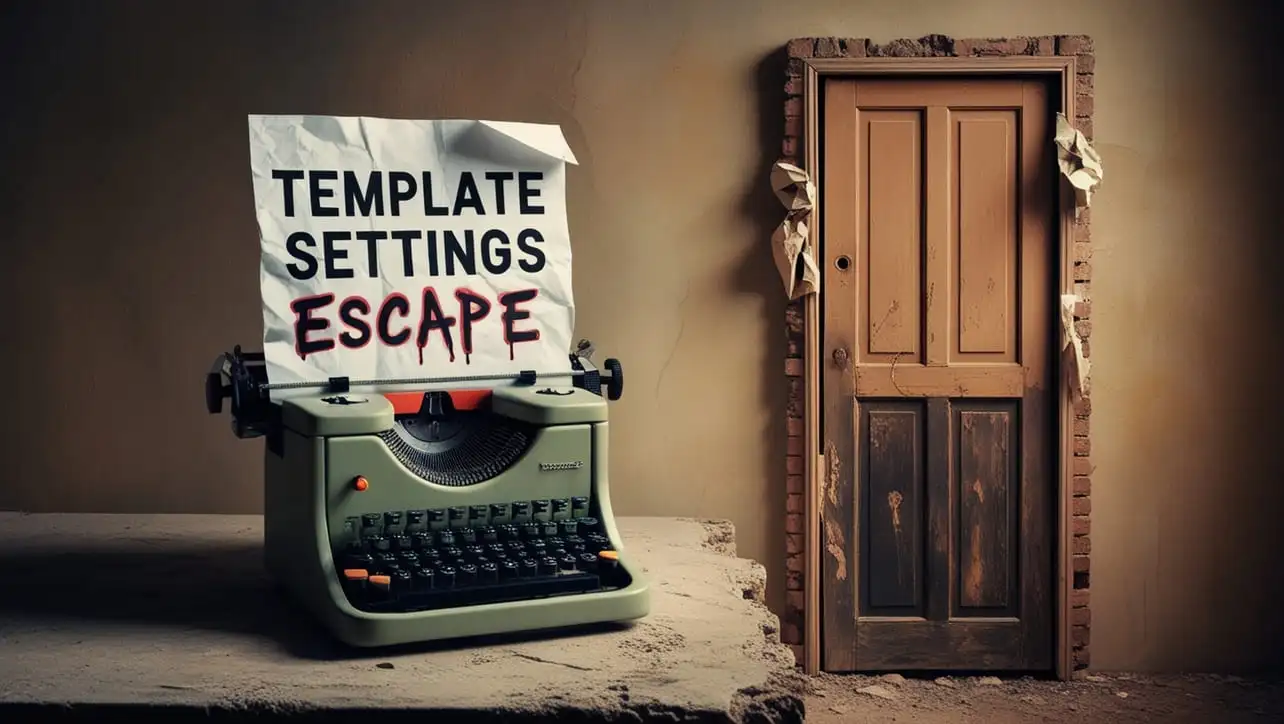
Lodash _.templateSettings.escape Property
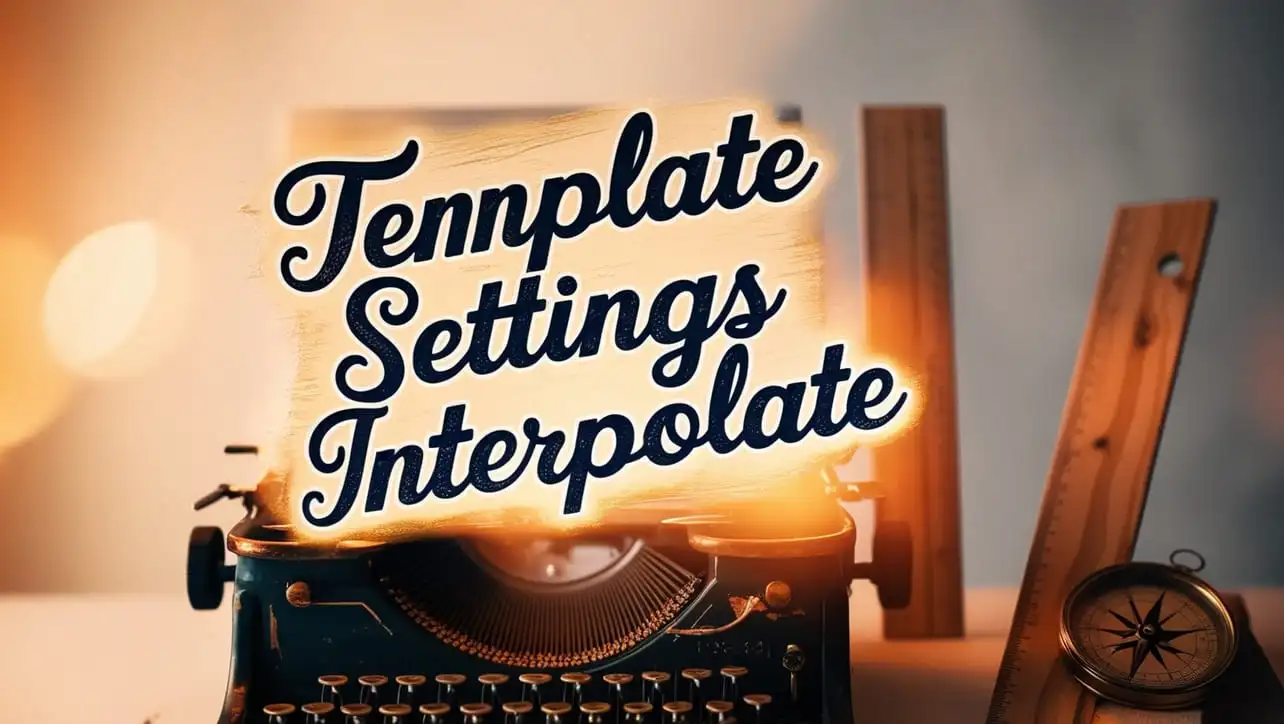
Lodash _.templateSettings.interpolate Property
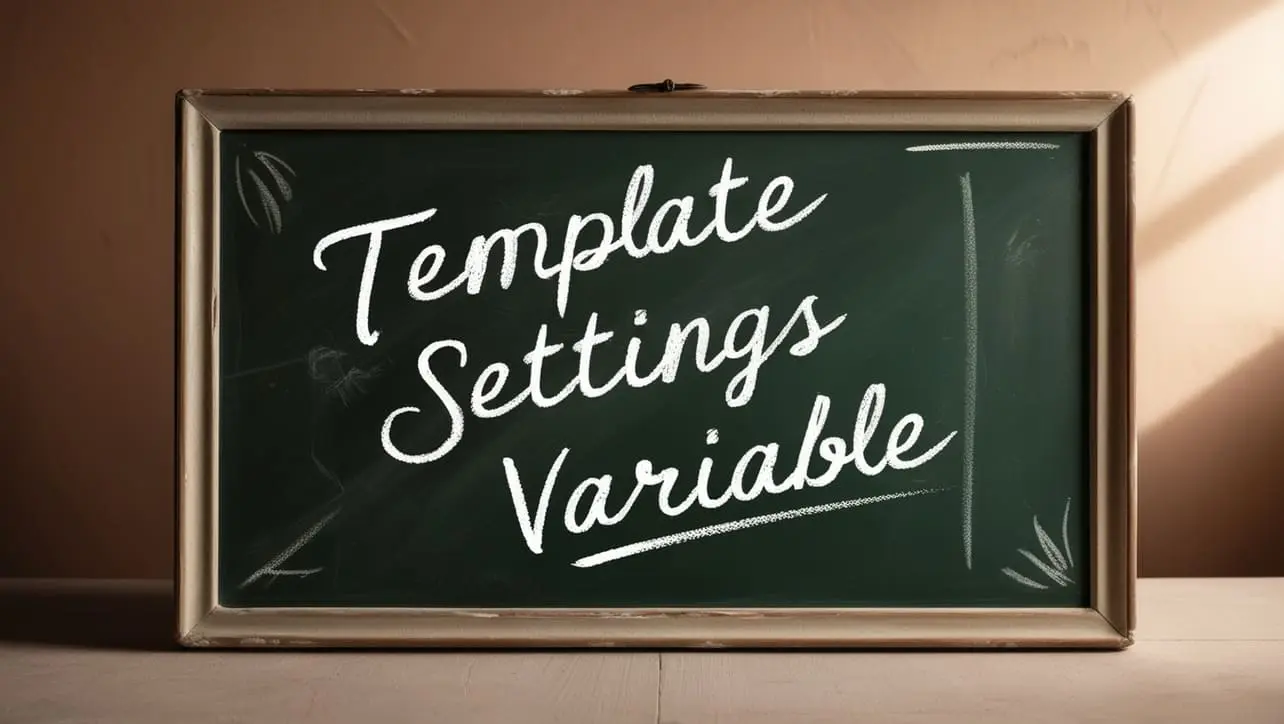
If you have any doubts regarding this article (Lodash _.omitBy() Object Method), please comment here. I will help you immediately.