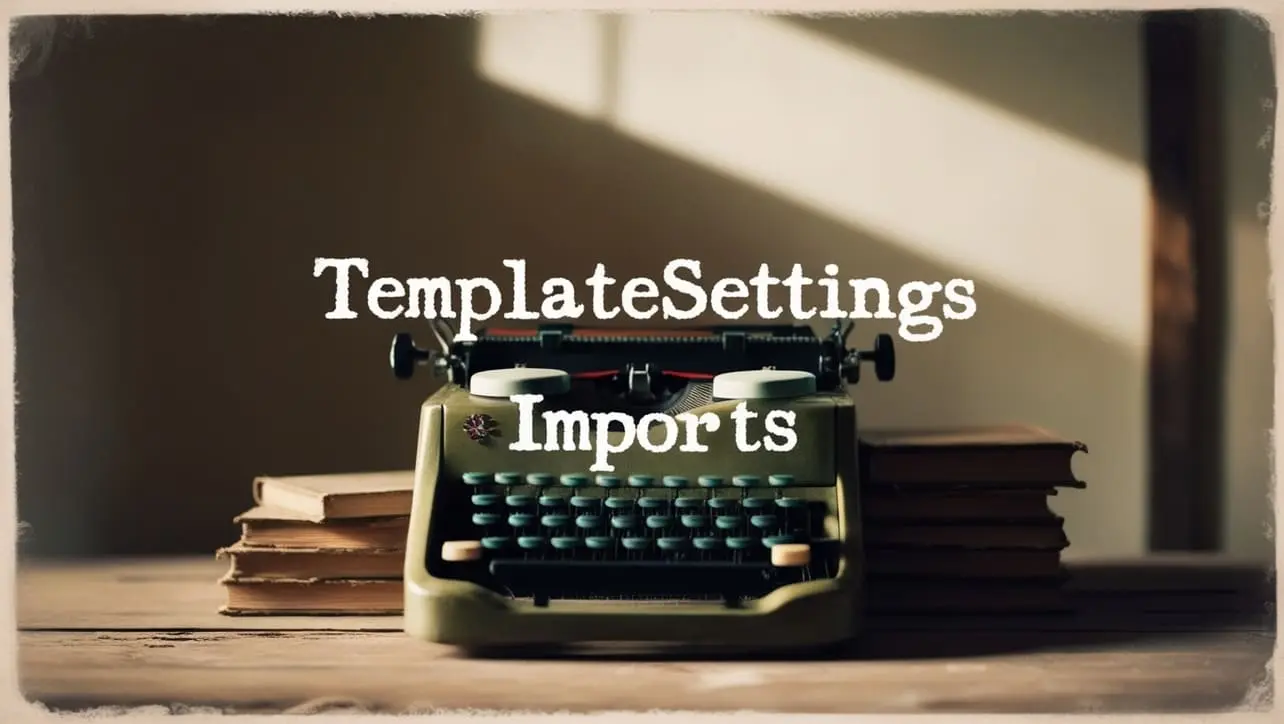
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.mapValues() Object Method
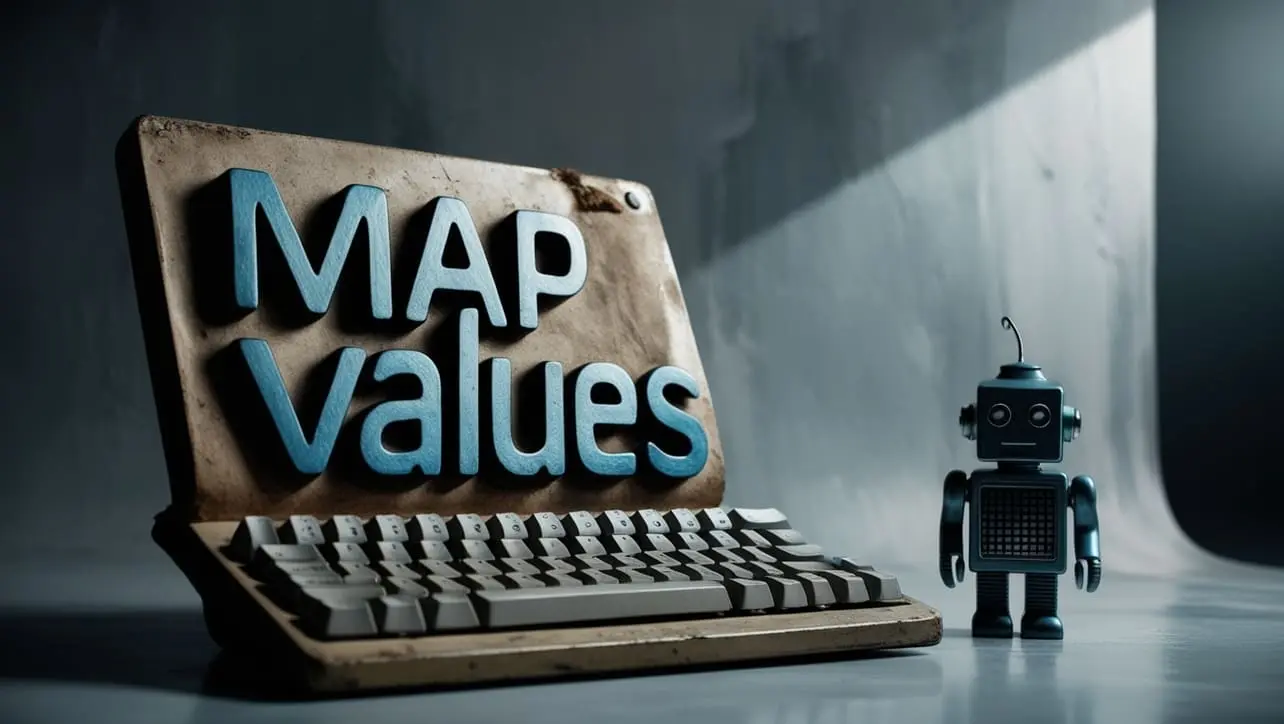
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, efficient manipulation of objects is essential for creating robust applications. Lodash, a popular utility library, provides a plethora of functions to streamline object manipulation tasks. Among these functions is the _.mapValues()
method, which offers a powerful way to transform the values of an object while preserving its structure.
This method is invaluable for developers seeking to enhance code readability and maintainability when working with complex data structures.
🧠 Understanding _.mapValues() Method
The _.mapValues()
method in Lodash enables developers to iterate over the values of an object and apply a transformation function to each value. This allows for the creation of a new object with the same keys but modified values, providing flexibility in data processing and transformation.
💡 Syntax
The syntax for the _.mapValues()
method is straightforward:
_.mapValues(object, [iteratee])
- object: The object to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.mapValues()
method:
const _ = require('lodash');
const originalObject = {
a: 1,
b: 2,
c: 3
};
const transformedObject = _.mapValues(originalObject, value => value * 2);
console.log(transformedObject);
// Output: { a: 2, b: 4, c: 6 }
In this example, the originalObject is processed by _.mapValues()
, doubling each value to create a new object with modified values.
🏆 Best Practices
When working with the _.mapValues()
method, consider the following best practices:
Understand Object Structure:
Before applying
_.mapValues()
, ensure a clear understanding of the structure and contents of the input object. This helps in defining appropriate transformation logic and handling edge cases effectively.example.jsCopiedconst employeeDetails = { emp1: { name: 'John', age: 30 }, emp2: { name: 'Alice', age: 25 }, emp3: { name: 'Bob', age: 35 } }; const updatedEmployeeDetails = _.mapValues(employeeDetails, employee => { return { ...employee, isSenior: employee.age > 30 }; }); console.log(updatedEmployeeDetails);
Handle Undefined Values:
Be prepared to handle undefined values returned by the iteratee function. Implement fallback logic or error handling to address scenarios where values may not be transformed as expected.
example.jsCopiedconst originalObject = { a: 1, b: 2, c: 3 }; const transformedObject = _.mapValues(originalObject, value => { if(value % 2 === 0) { return value * 2; } else { // Fallback logic for odd values return value; } }); console.log(transformedObject);
Leverage Iteratee Functionality:
Utilize the iteratee parameter to define custom transformation logic based on specific requirements. This allows for tailored processing of object values, enhancing code flexibility and maintainability.
example.jsCopiedconst originalObject = { a: 'apple', b: 'banana', c: 'cherry' }; const transformedObject = _.mapValues(originalObject, value => value.toUpperCase()); console.log(transformedObject); // Output: { a: 'APPLE', b: 'BANANA', c: 'CHERRY' }
📚 Use Cases
Data Transformation:
_.mapValues()
is invaluable for transforming data structures, such as converting data formats or applying calculations to values, while preserving the original object's structure.example.jsCopiedconst temperatureReadings = { day1: 25, day2: 28, day3: 22 }; const convertedTemperatureReadings = _.mapValues(temperatureReadings, temp => (temp - 32) * (5 / 9)); console.log(convertedTemperatureReadings);
Object Normalization:
In scenarios where object normalization is required,
_.mapValues()
can be used to standardize data representations or enforce specific conventions across object values.example.jsCopiedconst mixedCaseKeys = { Name: 'John', Age: 30, Occupation: 'Developer' }; const normalizedObject = _.mapValues(mixedCaseKeys, value => typeof value === 'string' ? value.toLowerCase() : value); console.log(normalizedObject);
Data Validation and Sanitization:
_.mapValues()
can also aid in data validation and sanitization by applying validation rules or sanitization functions to object values.example.jsCopiedconst userInput = { username: ' john_doe ', email: ' john@example.com ', age: '30' }; const sanitizedUserInput = _.mapValues(userInput, value => value.trim()); console.log(sanitizedUserInput);
🎉 Conclusion
The _.mapValues()
method in Lodash offers a versatile solution for transforming object values with ease and precision. Whether you're performing data transformations, normalizing object structures, or validating user input, this method empowers developers to streamline object manipulation tasks in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.mapValues()
method in your Lodash projects.
👨💻 Join our Community:
Author
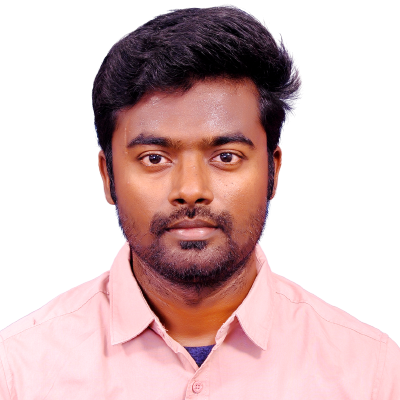
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
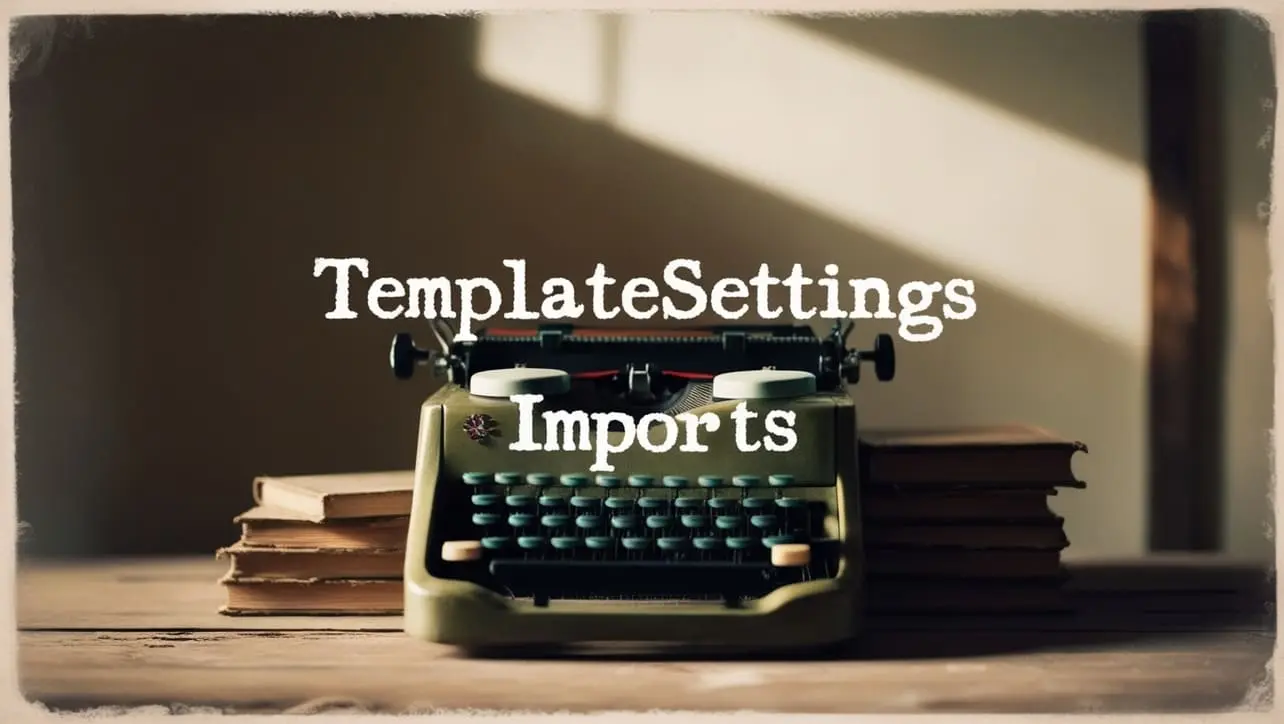
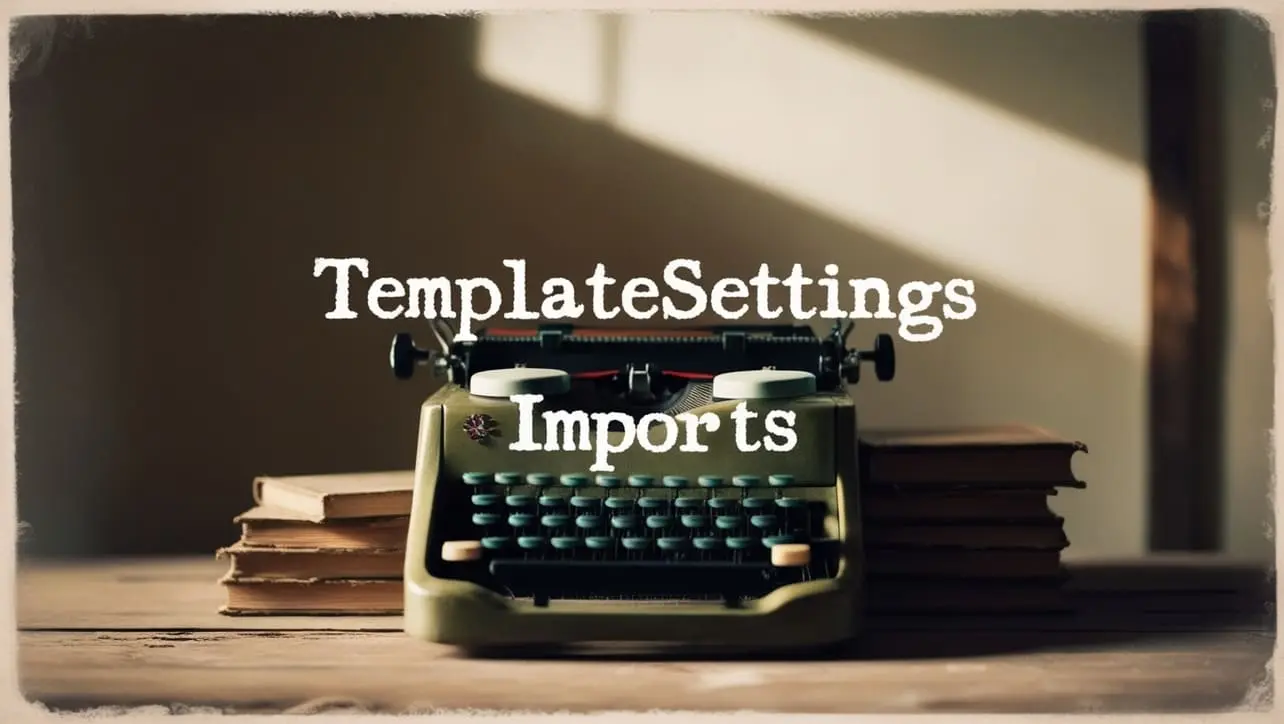
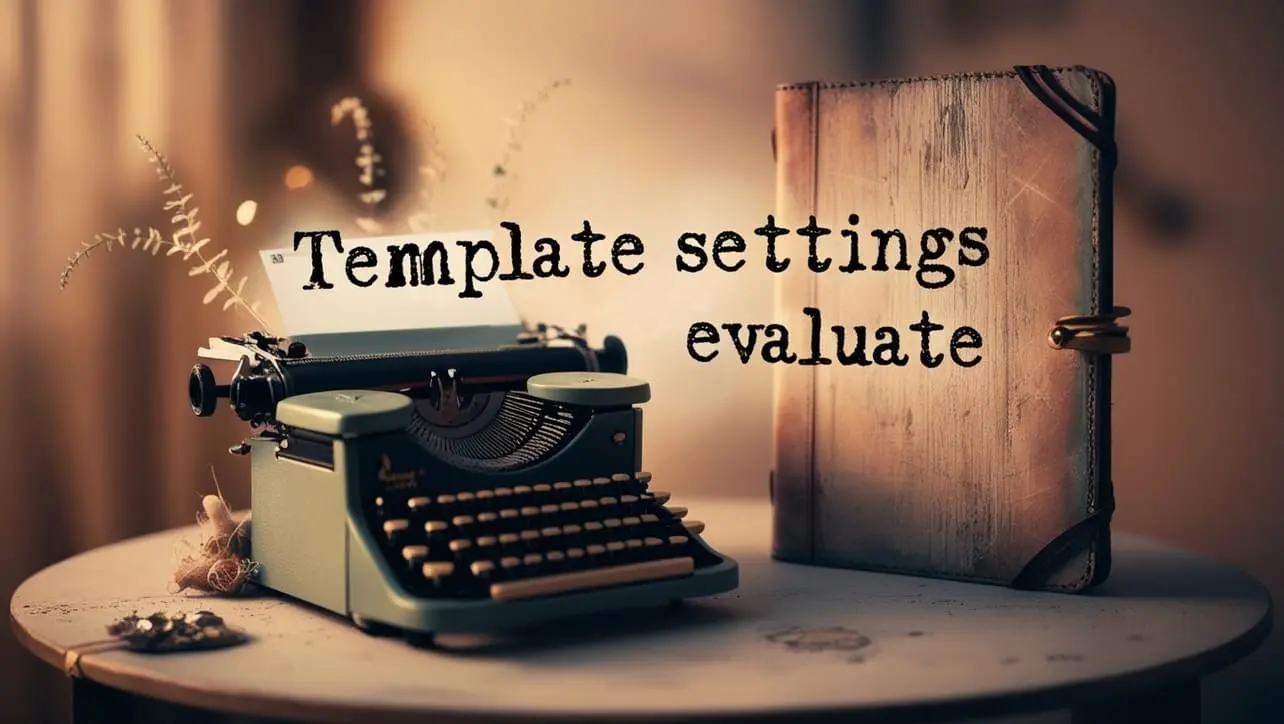
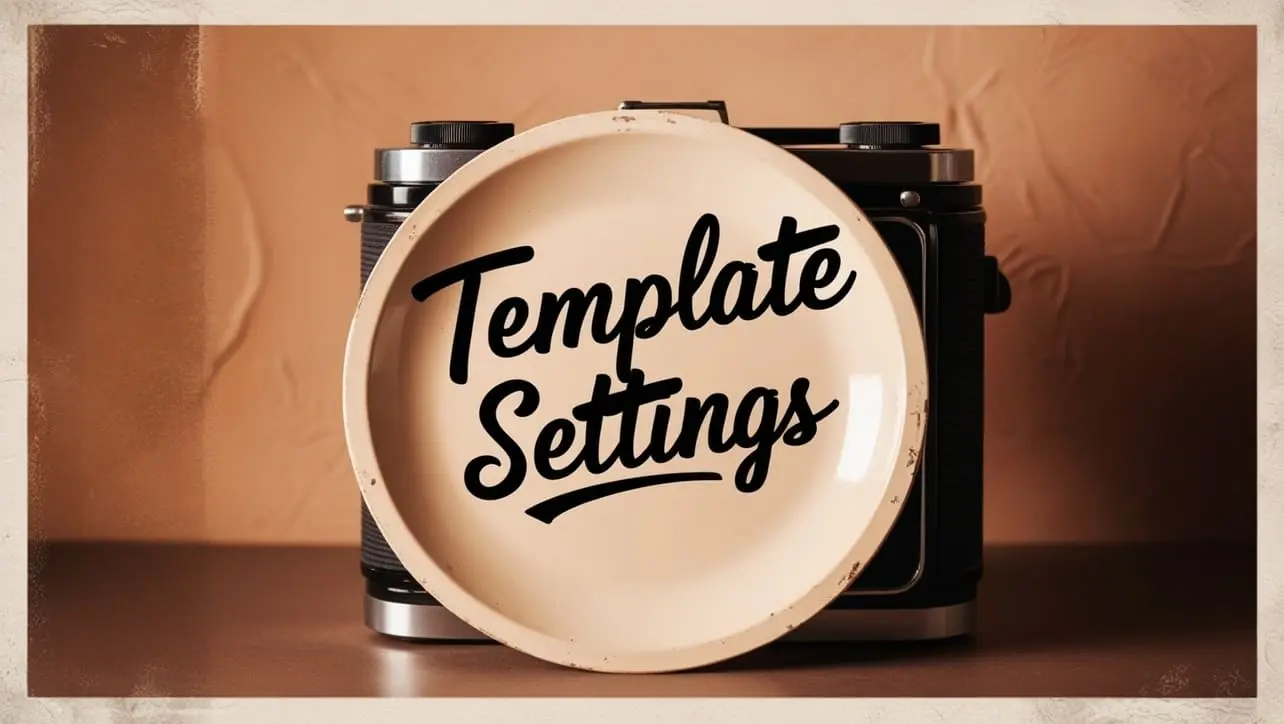
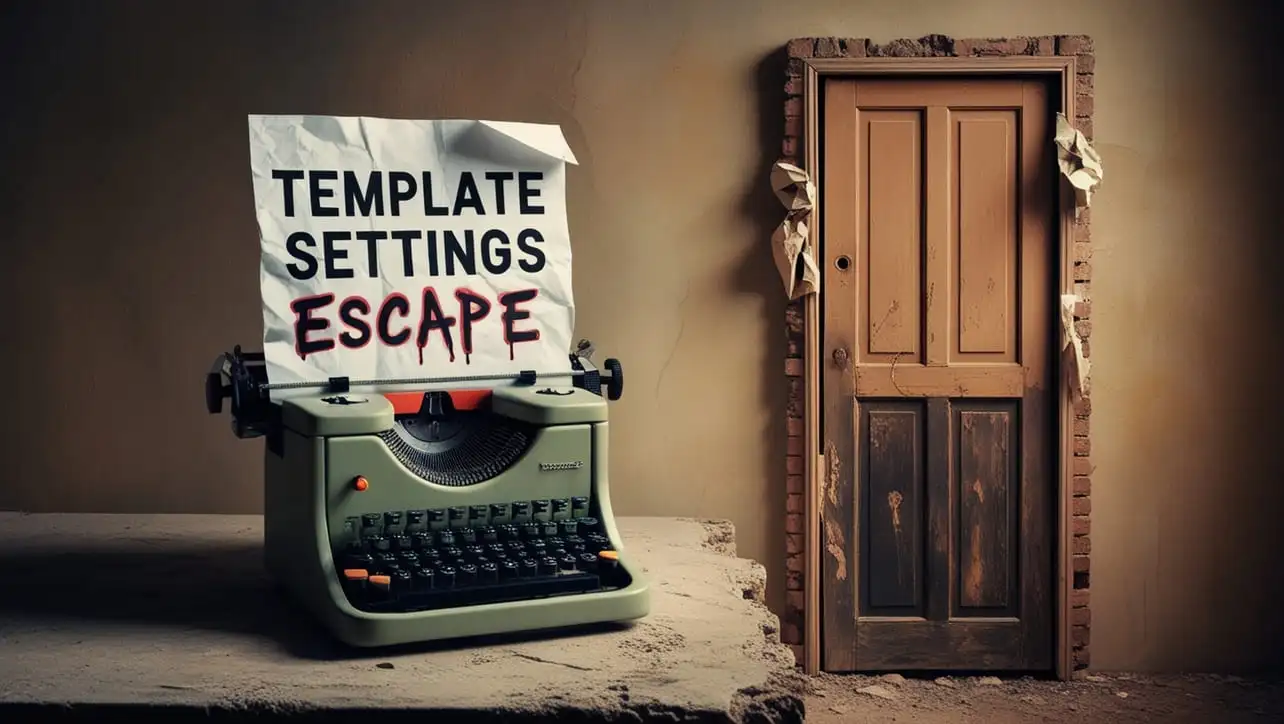
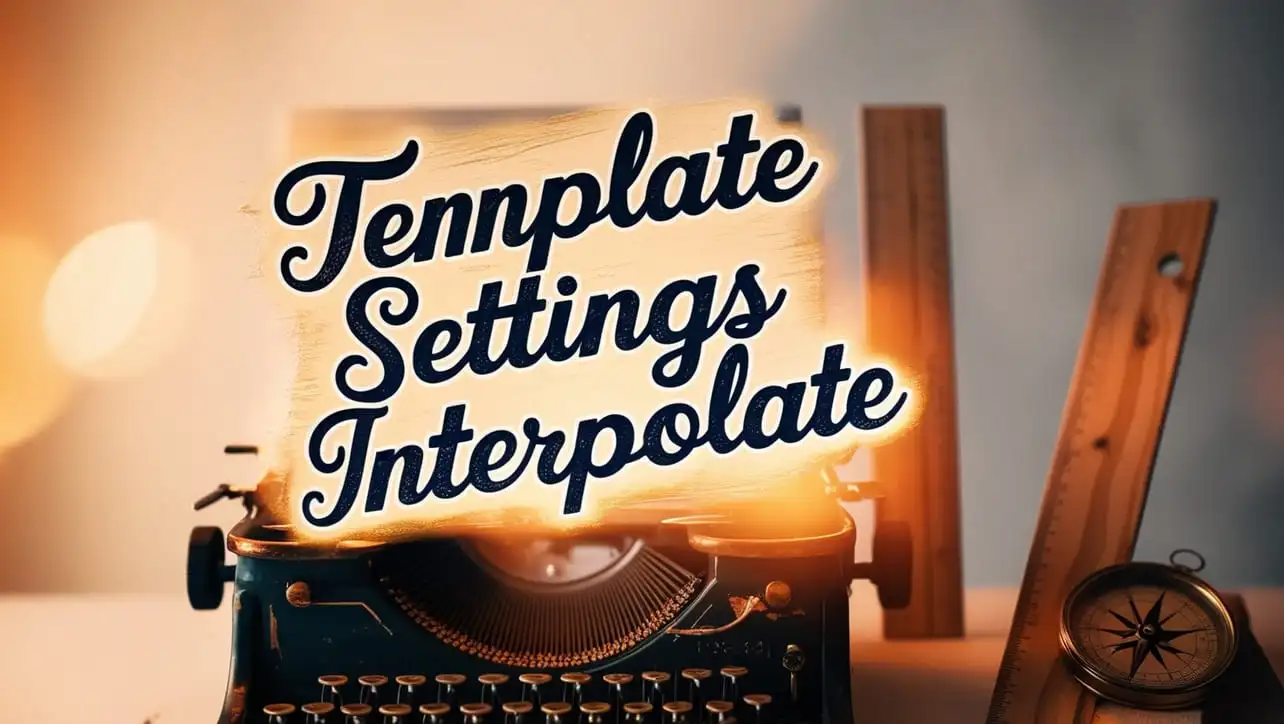
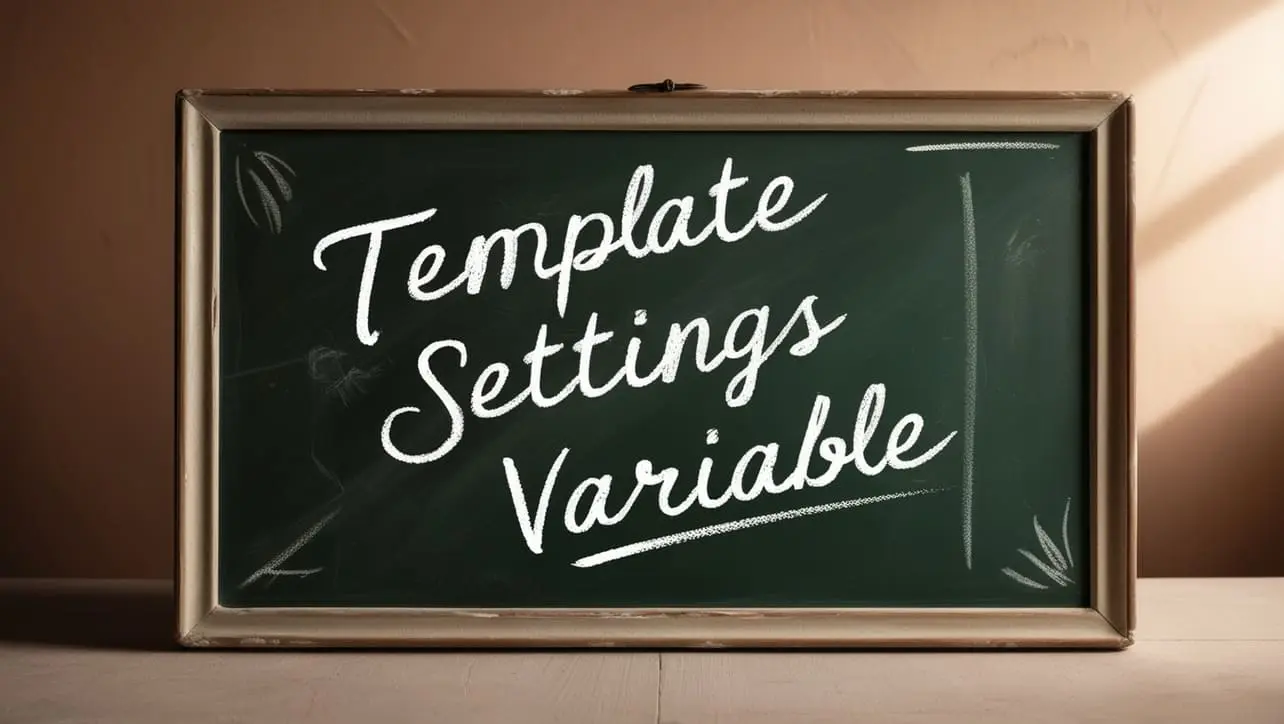
If you have any doubts regarding this article (Lodash _.mapValues() Object Method), please comment here. I will help you immediately.