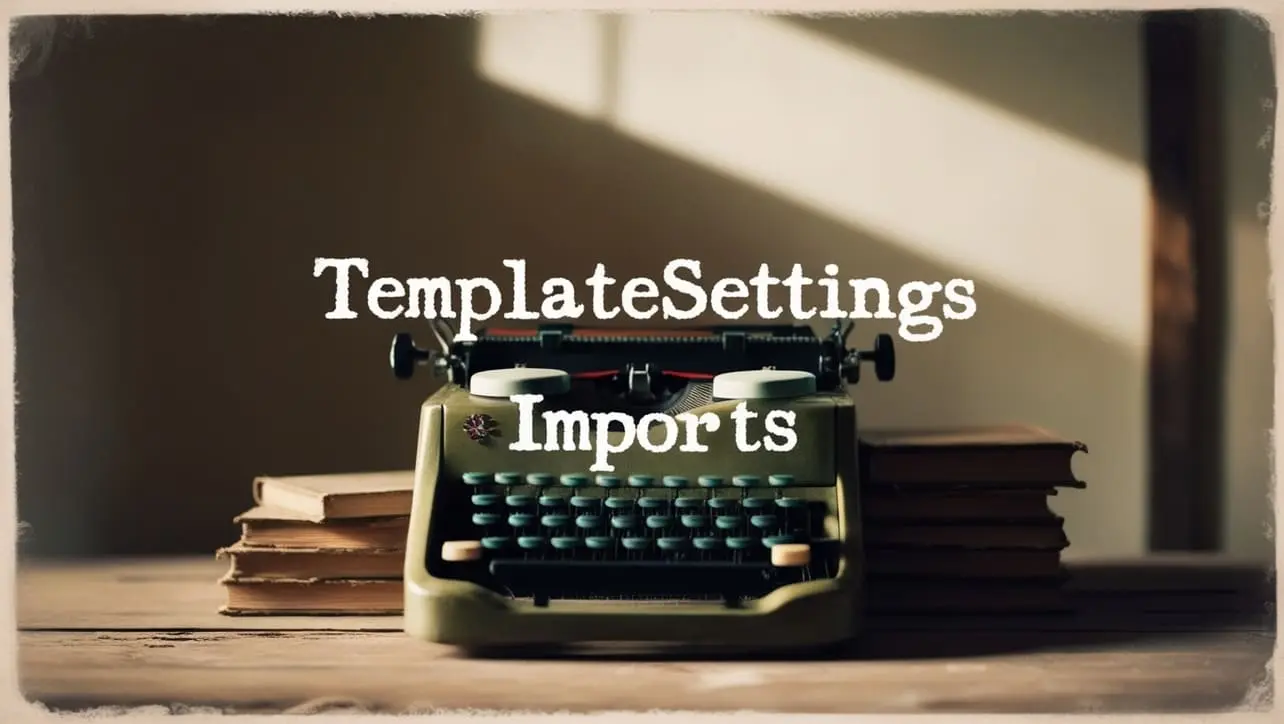
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.keys() Object Method
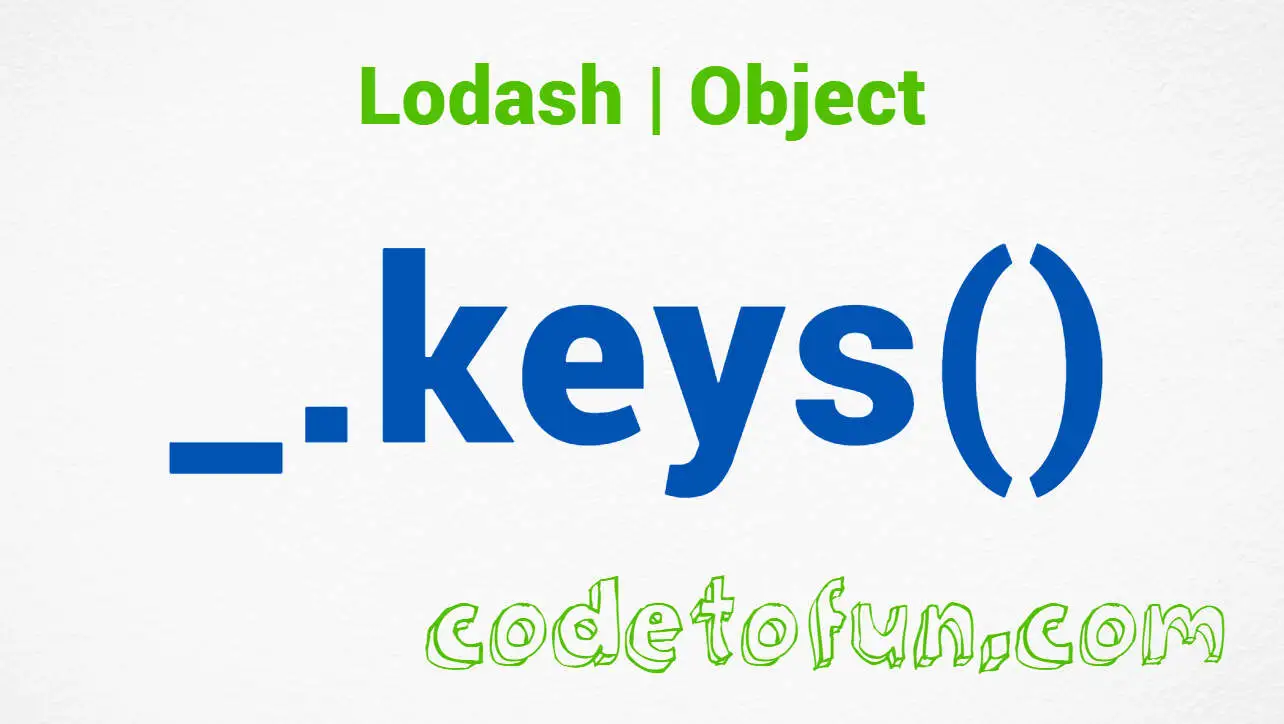
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, efficient manipulation of objects is essential. Lodash, a popular utility library, offers a multitude of functions to streamline object operations. Among these functions is the _.keys()
method, which provides a convenient way to extract the keys of an object.
Understanding how to leverage _.keys()
can greatly enhance your ability to work with objects in JavaScript.
🧠 Understanding _.keys() Method
The _.keys()
method in Lodash retrieves all the keys of an object and returns them in an array. This allows developers to easily access and iterate over the keys of an object, enabling various manipulation and analysis tasks.
💡 Syntax
The syntax for the _.keys()
method is straightforward:
_.keys(object)
- object: The object whose keys are to be retrieved.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.keys()
method:
const _ = require('lodash');
const sampleObject = { a: 1, b: 2, c: 3 };
const keysArray = _.keys(sampleObject);
console.log(keysArray);
// Output: ['a', 'b', 'c']
In this example, the keys of the sampleObject are extracted using _.keys()
, resulting in an array containing the keys.
🏆 Best Practices
When working with the _.keys()
method, consider the following best practices:
Object Validity Check:
Before using
_.keys()
, ensure that the input is a valid object to prevent unexpected errors.example.jsCopiedconst invalidObject = 'not an object'; const keysOfInvalidObject = _.keys(invalidObject); console.log(keysOfInvalidObject); // Output: []
Iterating Over Object Keys:
Use
_.keys()
in conjunction with iteration methods to perform operations on object keys efficiently.example.jsCopiedconst user = { name: 'John', age: 30, email: 'john@example.com' }; _.keys(user).forEach(key => { console.log(`${key}: ${user[key]}`); });
Object Manipulation:
Utilize
_.keys()
to manipulate object properties dynamically, such as copying or filtering specific keys.example.jsCopiedconst sourceObject = { a: 1, b: 2, c: 3 }; const filteredKeys = ['a', 'c']; const filteredObject = _.pick(sourceObject, filteredKeys); console.log(filteredObject); // Output: { a: 1, c: 3 }
📚 Use Cases
Object Property Enumeration:
_.keys()
facilitates the enumeration of object properties, enabling comprehensive analysis and manipulation.example.jsCopiedconst car = { make: 'Toyota', model: 'Camry', year: 2020 }; const carProperties = _.keys(car); console.log(carProperties); // Output: ['make', 'model', 'year']
Object Property Validation:
When validating or sanitizing object properties,
_.keys()
can be instrumental in extracting and examining property names.example.jsCopiedconst formData = { username: 'john_doe', password: 'password123', email: 'john@example.com' }; const requiredFields = ['username', 'password', 'email']; const missingFields = _.difference(requiredFields, _.keys(formData)); console.log(missingFields); // Output: []
Object Property Transformation:
_.keys()
can facilitate the transformation of object properties, allowing for dynamic restructuring or manipulation.example.jsCopiedconst originalObject = { a: 1, b: 2, c: 3 }; const transformedObject = {}; _.keys(originalObject).forEach(key => { transformedObject[key.toUpperCase()] = originalObject[key]; }); console.log(transformedObject); // Output: { A: 1, B: 2, C: 3 }
🎉 Conclusion
The _.keys()
method in Lodash simplifies object manipulation by providing a straightforward mechanism to extract keys from objects. Whether you're iterating over object properties, validating inputs, or transforming data, _.keys()
offers a versatile solution to streamline your JavaScript development workflow.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.keys()
method in your Lodash projects.
👨💻 Join our Community:
Author
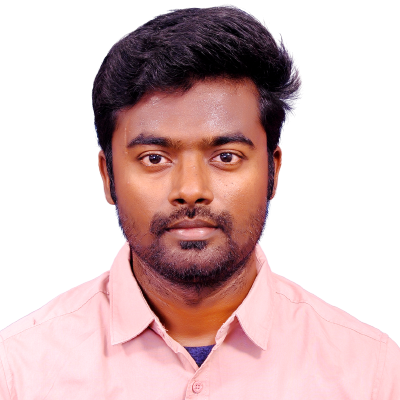
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
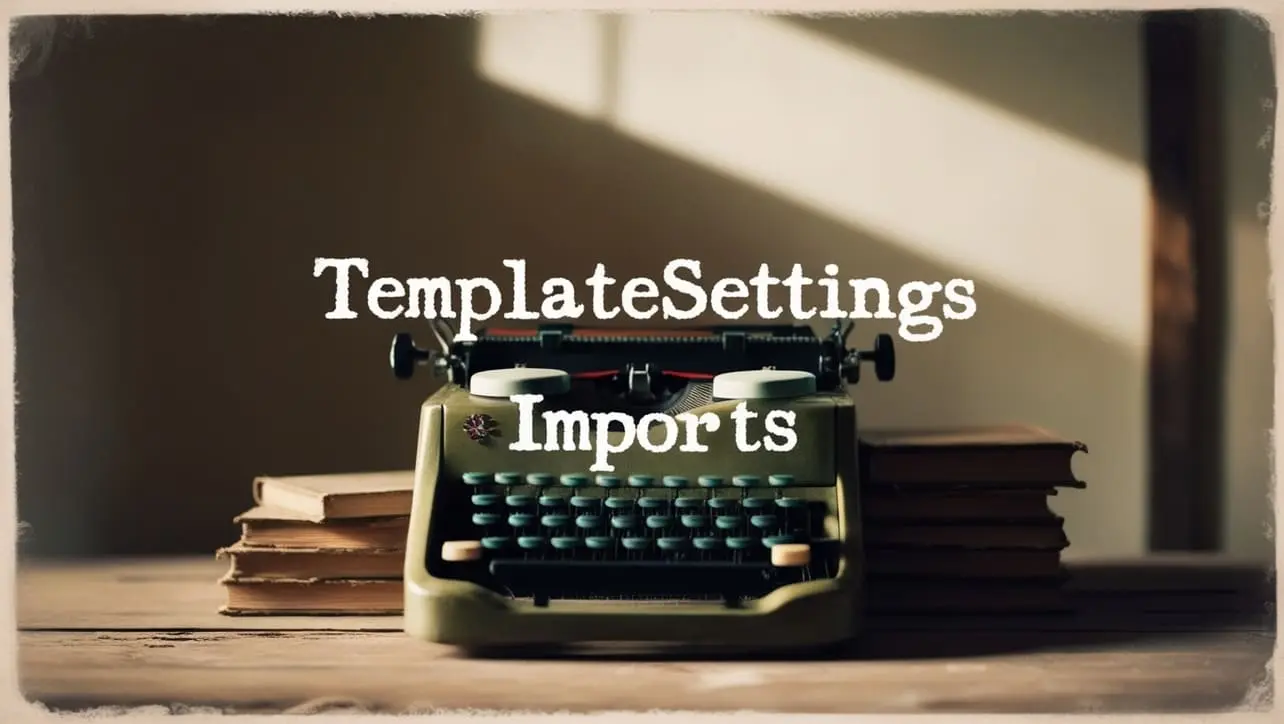
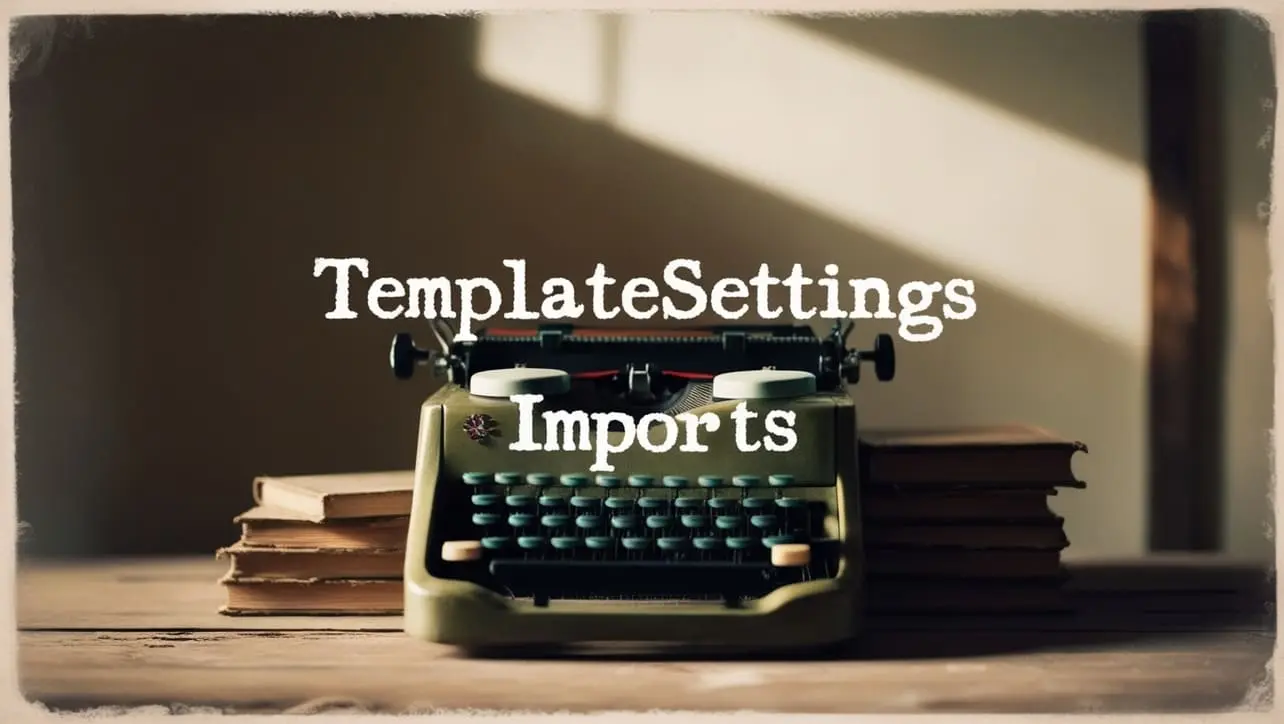
Lodash _.templateSettings.imports Property
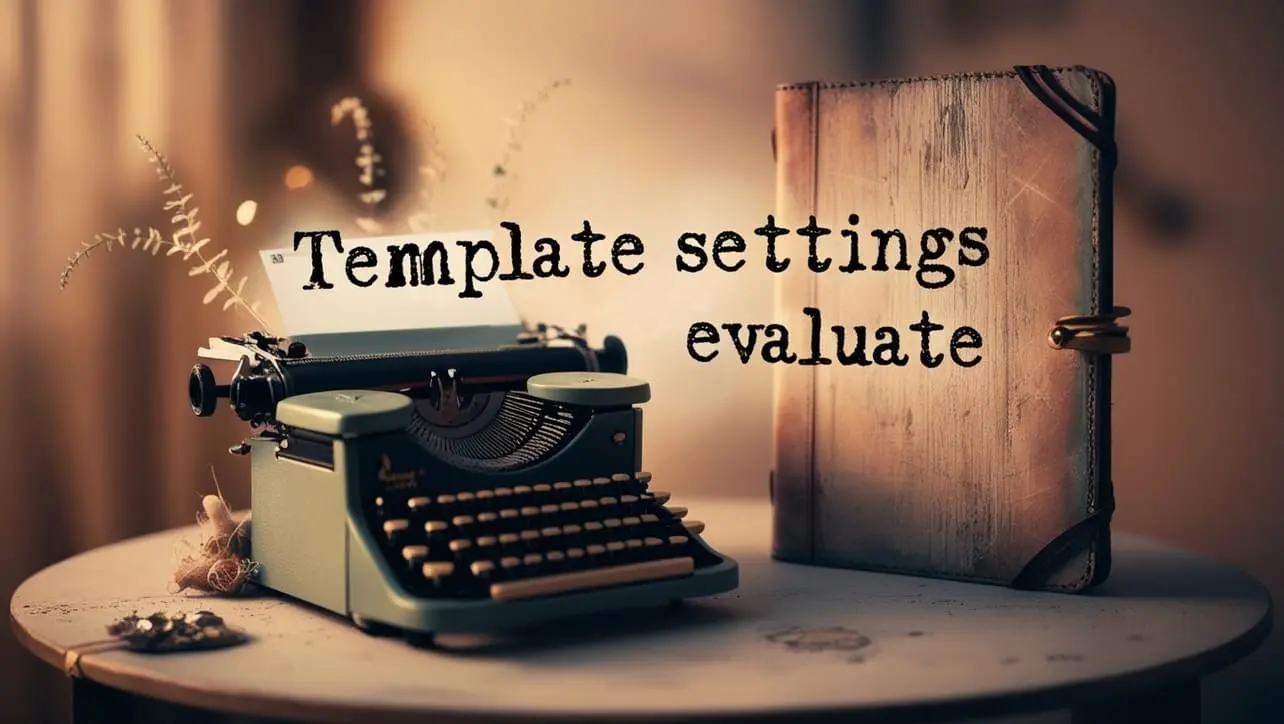
Lodash _.templateSettings.evaluate Property
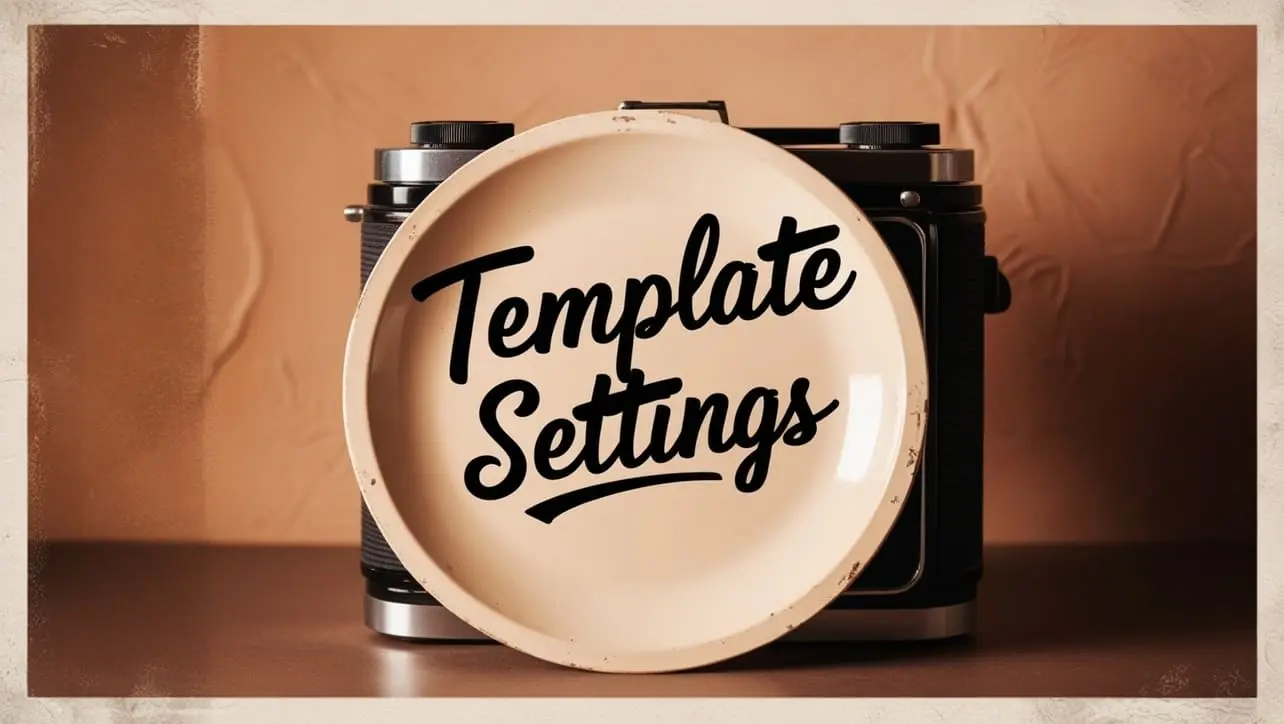
Lodash _.templateSettings Property
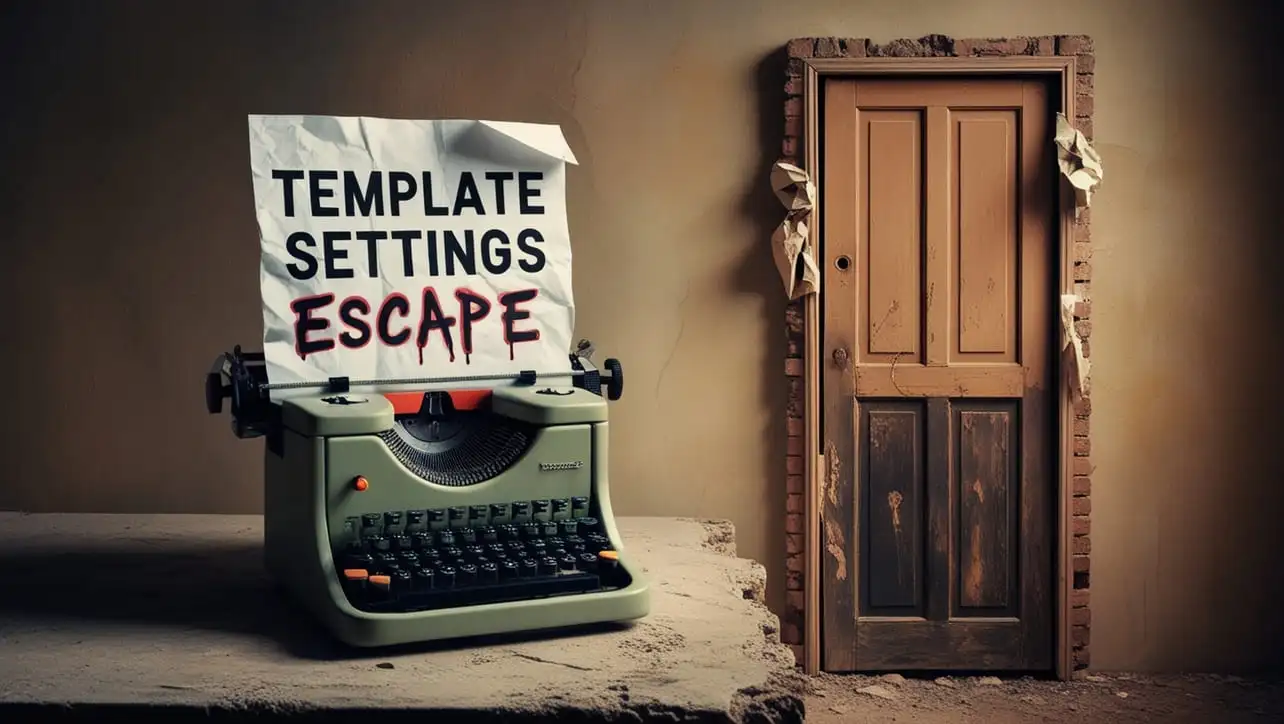
Lodash _.templateSettings.escape Property
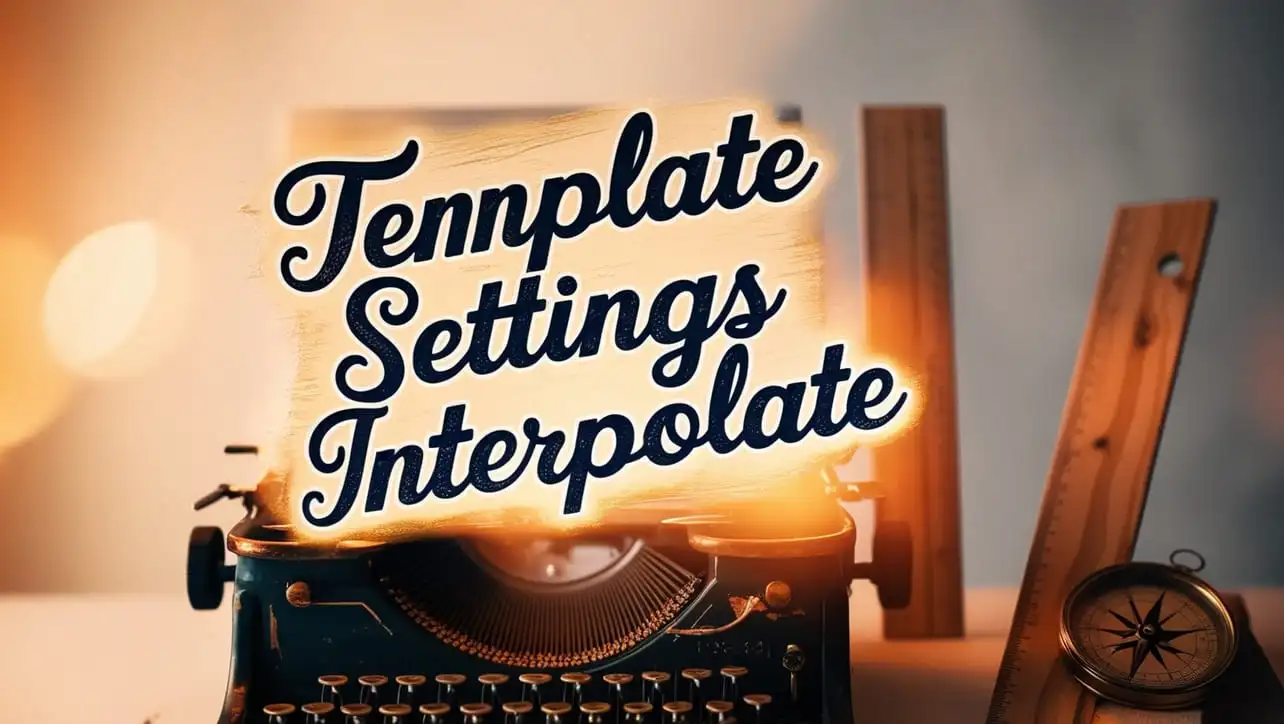
Lodash _.templateSettings.interpolate Property
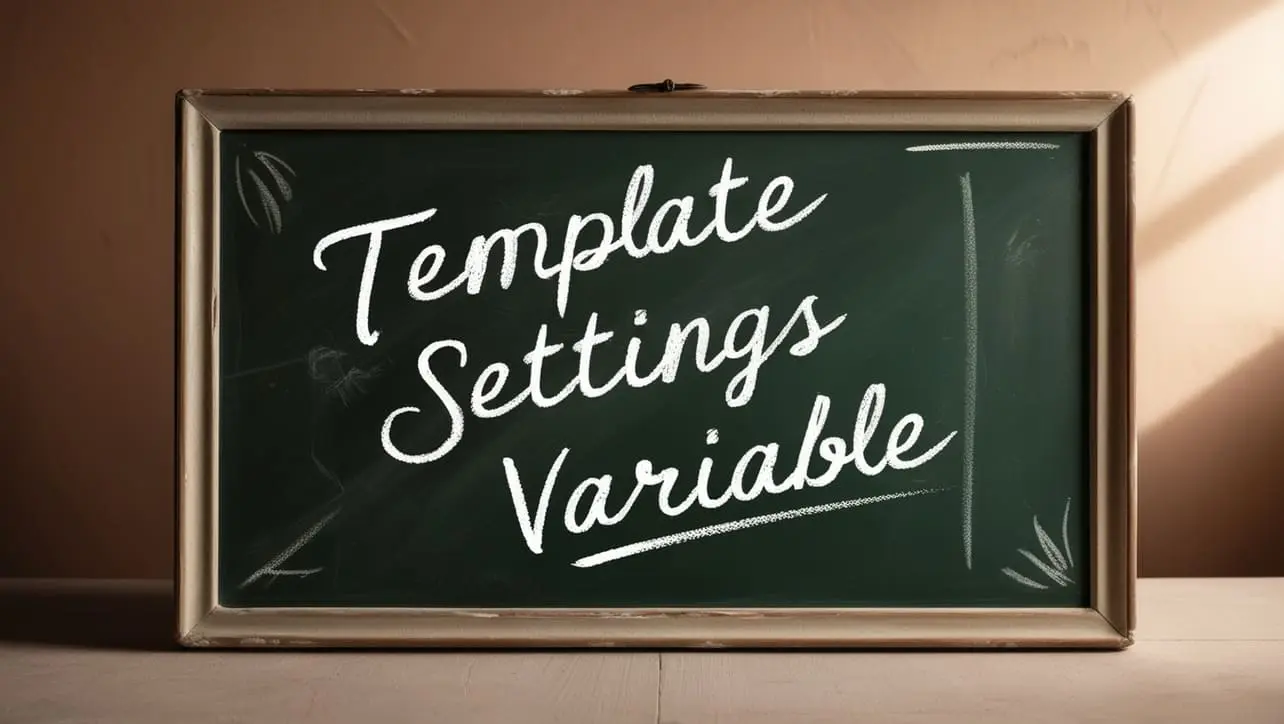
If you have any doubts regarding this article (Lodash _.keys() Object Method), please comment here. I will help you immediately.