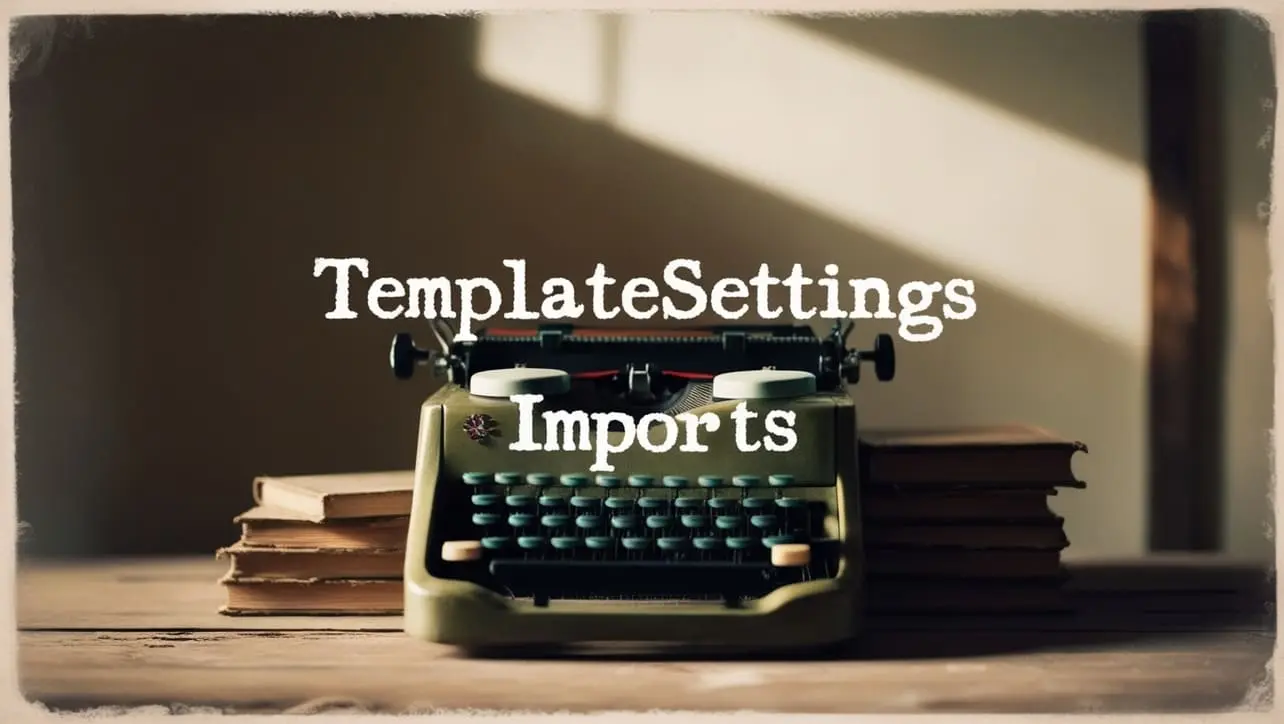
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.invert() Object Method
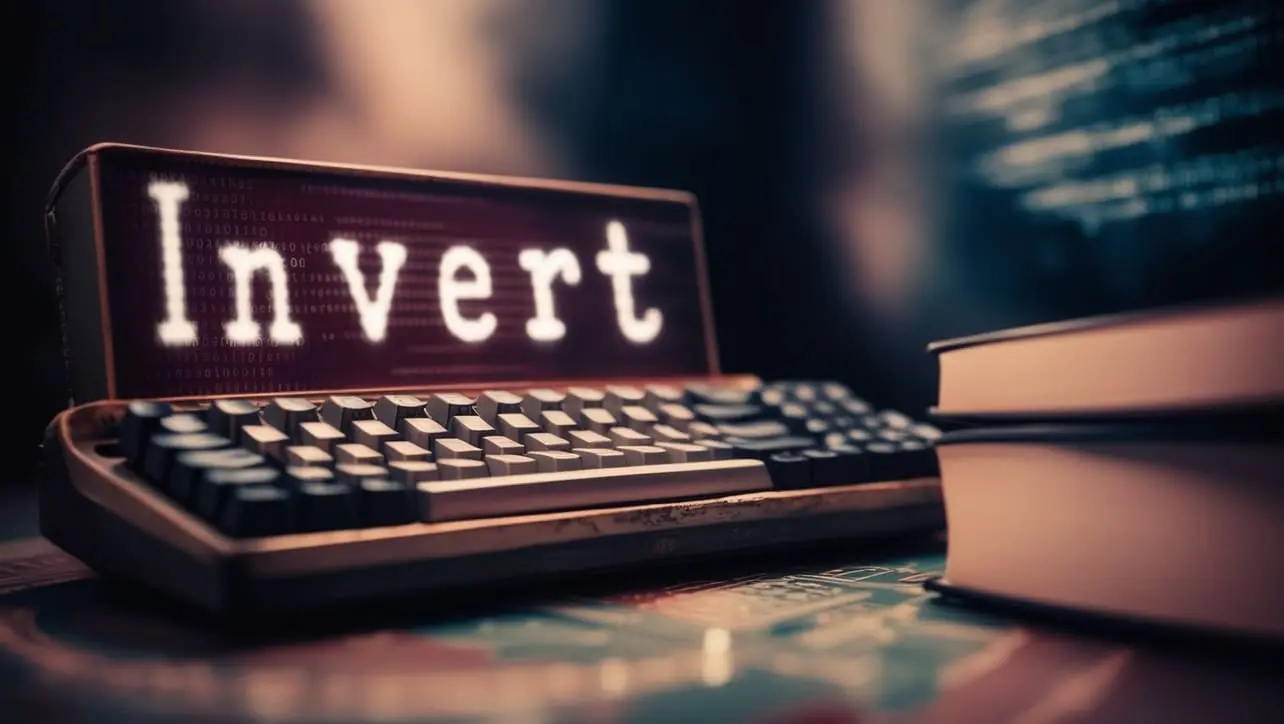
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, manipulating objects is a common task. Lodash, a comprehensive utility library, provides a wealth of functions to simplify object operations. Among these functions is the _.invert()
method, a powerful tool for inverting the keys and values of an object.
This method enhances the versatility of object handling, offering a convenient way to switch between keys and values.
🧠 Understanding _.invert() Method
The _.invert()
method in Lodash transforms an object by swapping its keys and values. This can be particularly useful when dealing with dictionaries or scenarios where you need to perform lookups based on values rather than keys.
💡 Syntax
The syntax for the _.invert()
method is straightforward:
_.invert(object)
- object: The object to invert.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.invert()
method:
const _ = require('lodash');
const originalObject = { apple: 'red', banana: 'yellow', grape: 'purple' };
const invertedObject = _.invert(originalObject);
console.log(invertedObject);
// Output: { red: 'apple', yellow: 'banana', purple: 'grape' }
In this example, the originalObject is inverted using _.invert()
, resulting in a new object where the keys and values are swapped.
🏆 Best Practices
When working with the _.invert()
method, consider the following best practices:
Handling Duplicate Values:
Be aware that if your original object has duplicate values, using
_.invert()
may lead to unintended consequences. The method will use the last occurrence of a value as the key in the inverted object, potentially overwriting previous keys.example.jsCopiedconst objectWithDuplicates = { A: 1, B: 2, C: 1 }; const invertedObjectWithDuplicates = _.invert(objectWithDuplicates); console.log(invertedObjectWithDuplicates); // Output: { '1': 'C', '2': 'B' }
Validate Object Structure:
Before applying
_.invert()
, ensure that your object contains simple key-value pairs. The method is designed for such structures and may not behave as expected with nested or complex objects.example.jsCopiedconst complexObject = { user: { name: 'John', age: 30 }, role: 'admin' }; const invertedComplexObject = _.invert(complexObject); console.log(invertedComplexObject); // Output: { '[object Object]': 'role' }
Consider Use Cases:
Evaluate whether inverting keys and values is the most appropriate solution for your use case. While powerful,
_.invert()
may not be suitable for every scenario, so choose it based on your specific needs.example.jsCopiedconst numericalObject = { 1: 'one', 2: 'two', 3: 'three' }; const invertedNumericalObject = _.invert(numericalObject); console.log(invertedNumericalObject); // Output: { one: '1', two: '2', three: '3' }
📚 Use Cases
Creating Lookup Tables:
_.invert()
is particularly useful when you need to create a lookup table for quick value-based lookups. By inverting the object, you can easily map values to their corresponding keys.example.jsCopiedconst colorCodes = { red: '#FF0000', green: '#00FF00', blue: '#0000FF' }; const invertedColorCodes = _.invert(colorCodes); console.log(invertedColorCodes['#00FF00']); // Output: 'green'
Handling Enums or Constants:
In scenarios where you have enums or constant values,
_.invert()
can be employed to switch between symbolic names and actual values.example.jsCopiedconst actionTypes = { LOGIN: 'user/login', LOGOUT: 'user/logout', PROFILE: 'user/profile' }; const invertedActionTypes = _.invert(actionTypes); console.log(invertedActionTypes['user/profile']); // Output: 'PROFILE'
Removing Redundant Data:
By inverting an object, you can identify and eliminate redundant data, especially when dealing with one-to-one mappings.
example.jsCopiedconst synonyms = { hot: 'warm', cold: 'chilly', happy: 'joyful' }; const invertedSynonyms = _.invert(synonyms); console.log(invertedSynonyms); // Output: { warm: 'hot', chilly: 'cold', joyful: 'happy' }
🎉 Conclusion
The _.invert()
method in Lodash provides a convenient and efficient way to invert the keys and values of an object. Whether you're creating lookup tables, handling enums, or simplifying data structures, this method offers a versatile solution for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.invert()
method in your Lodash projects.
👨💻 Join our Community:
Author
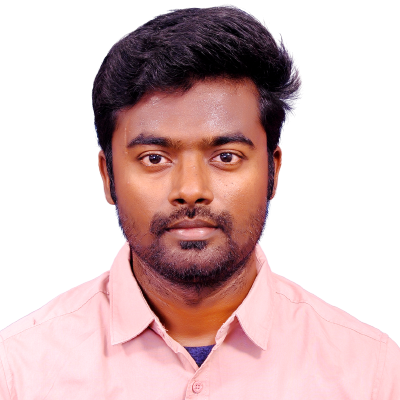
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
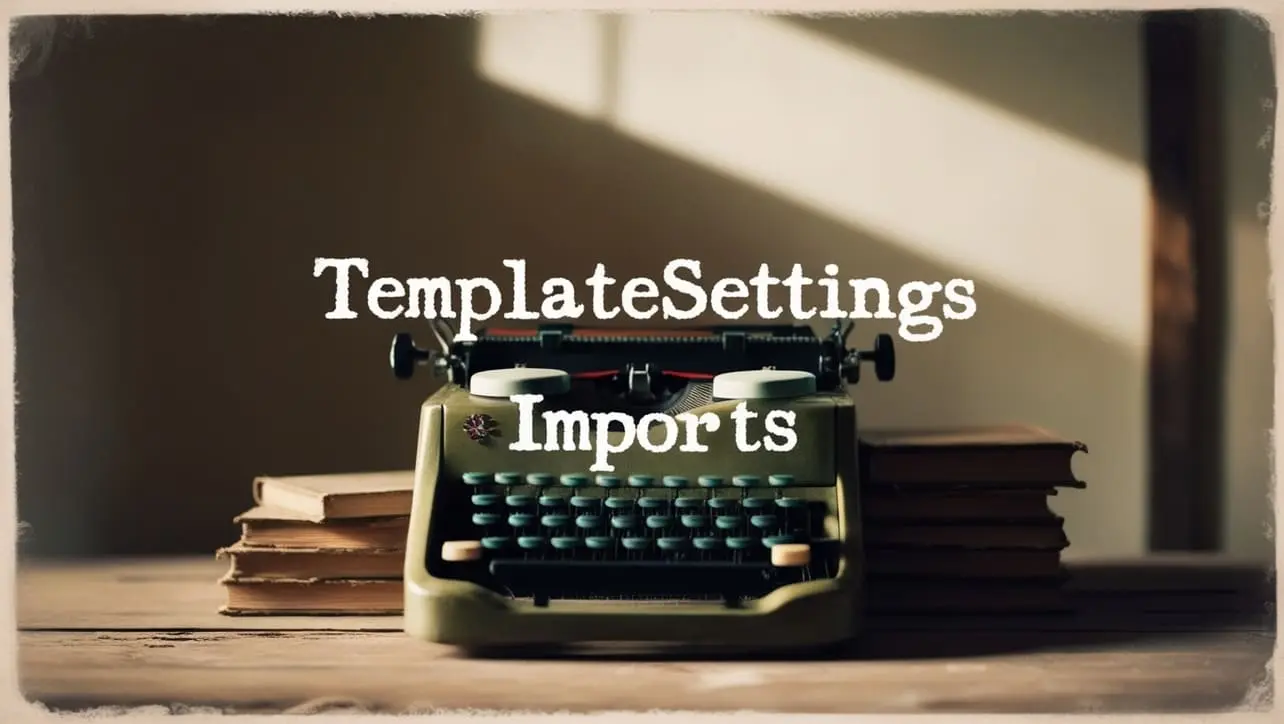
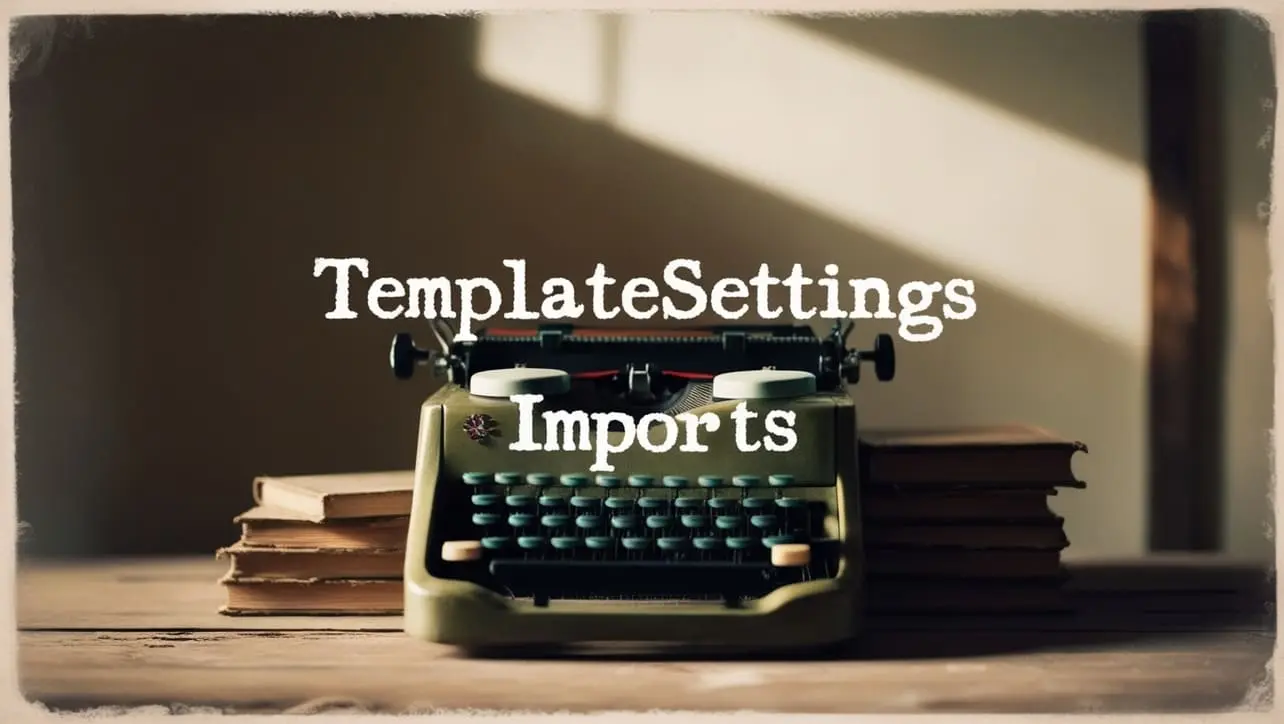
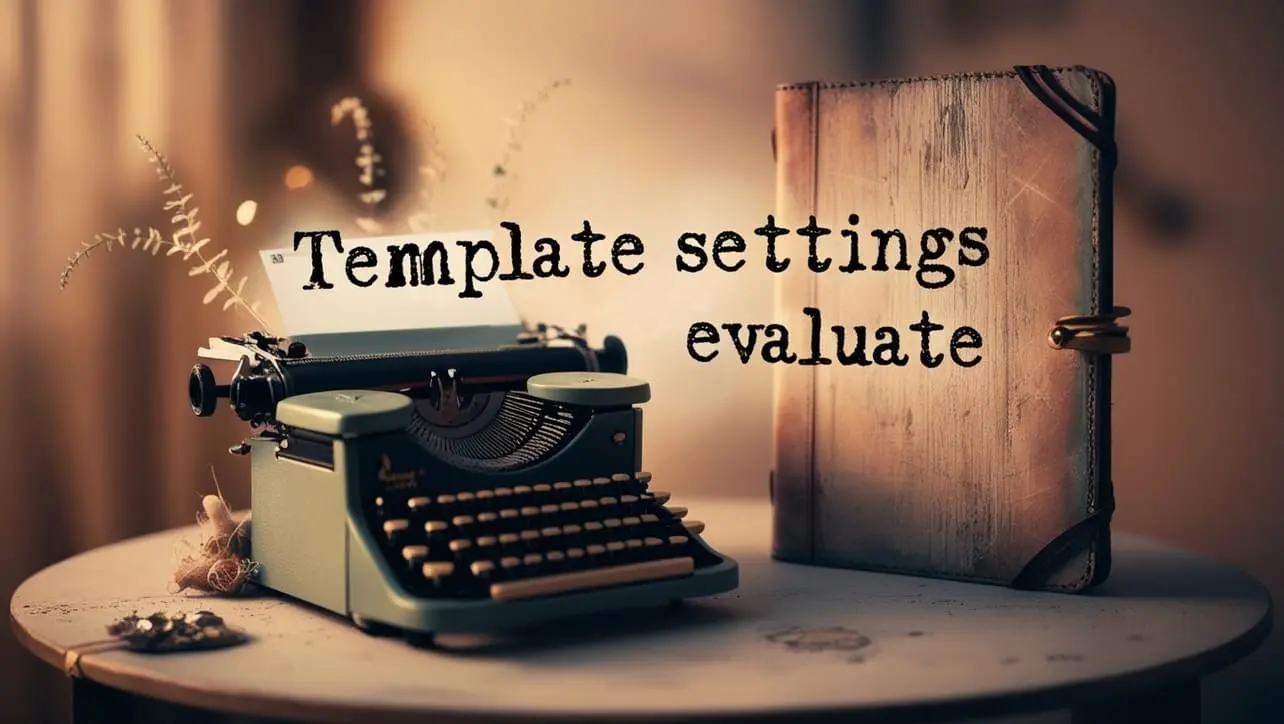
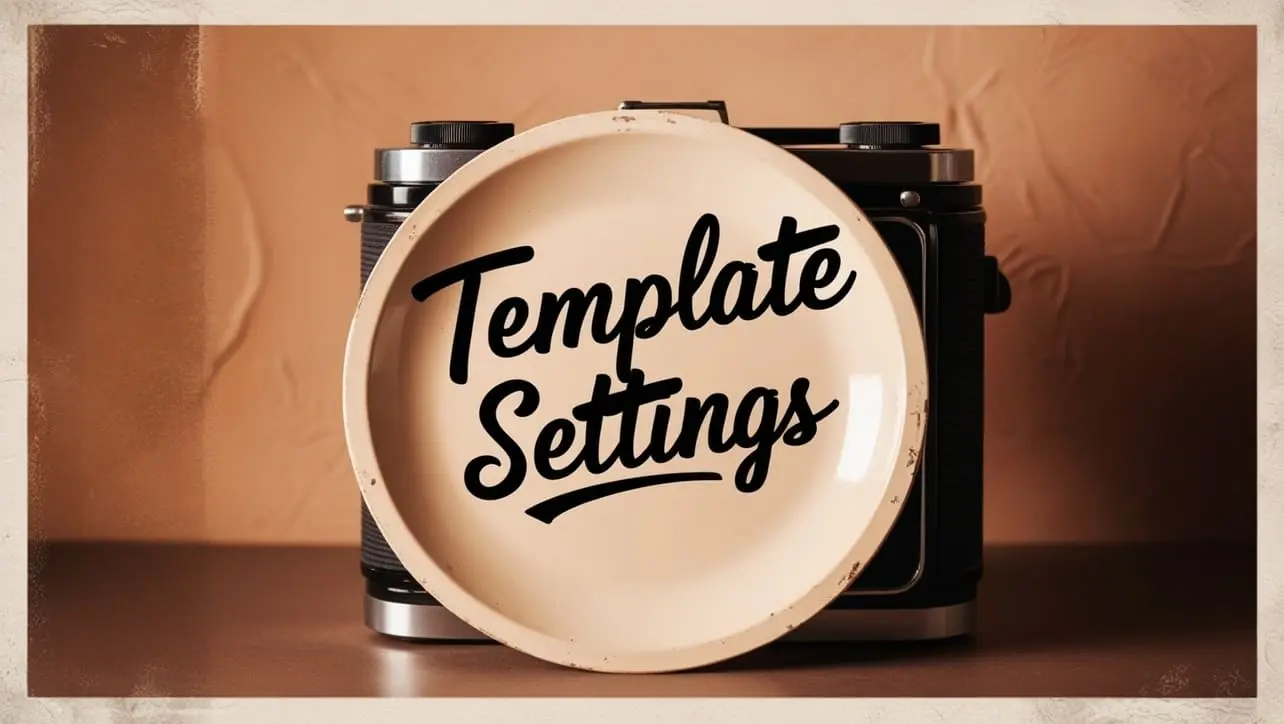
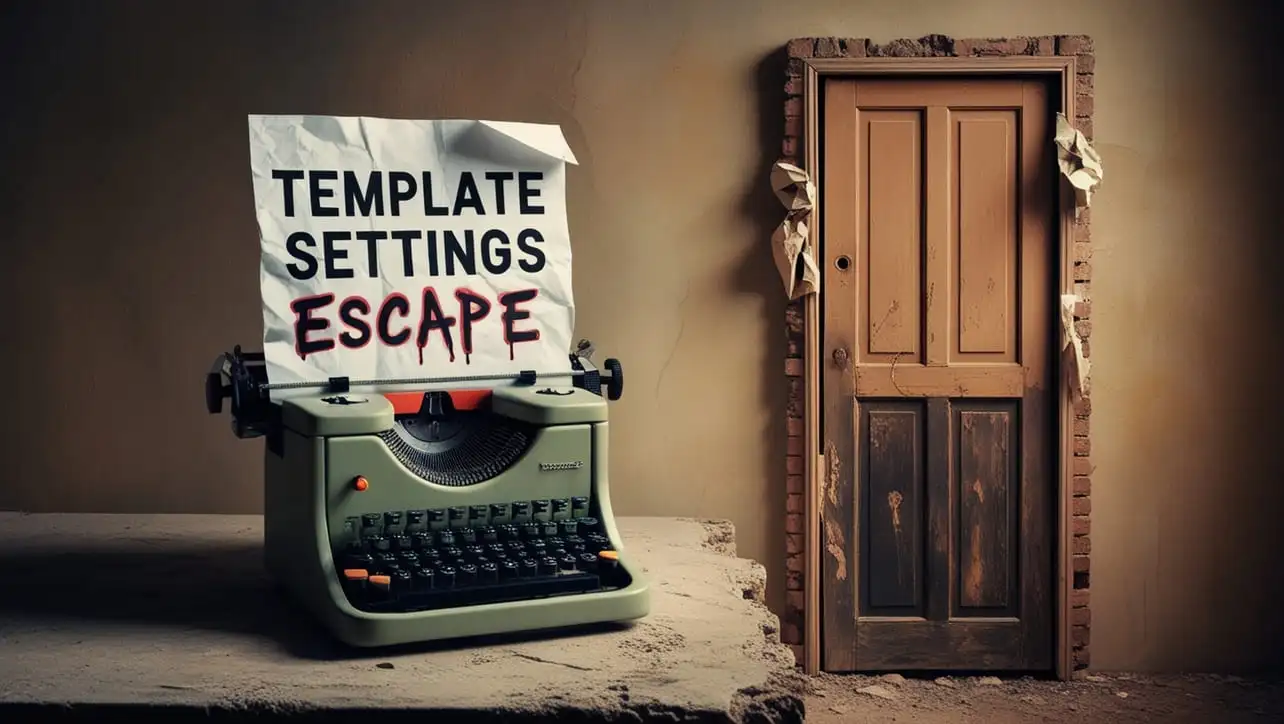
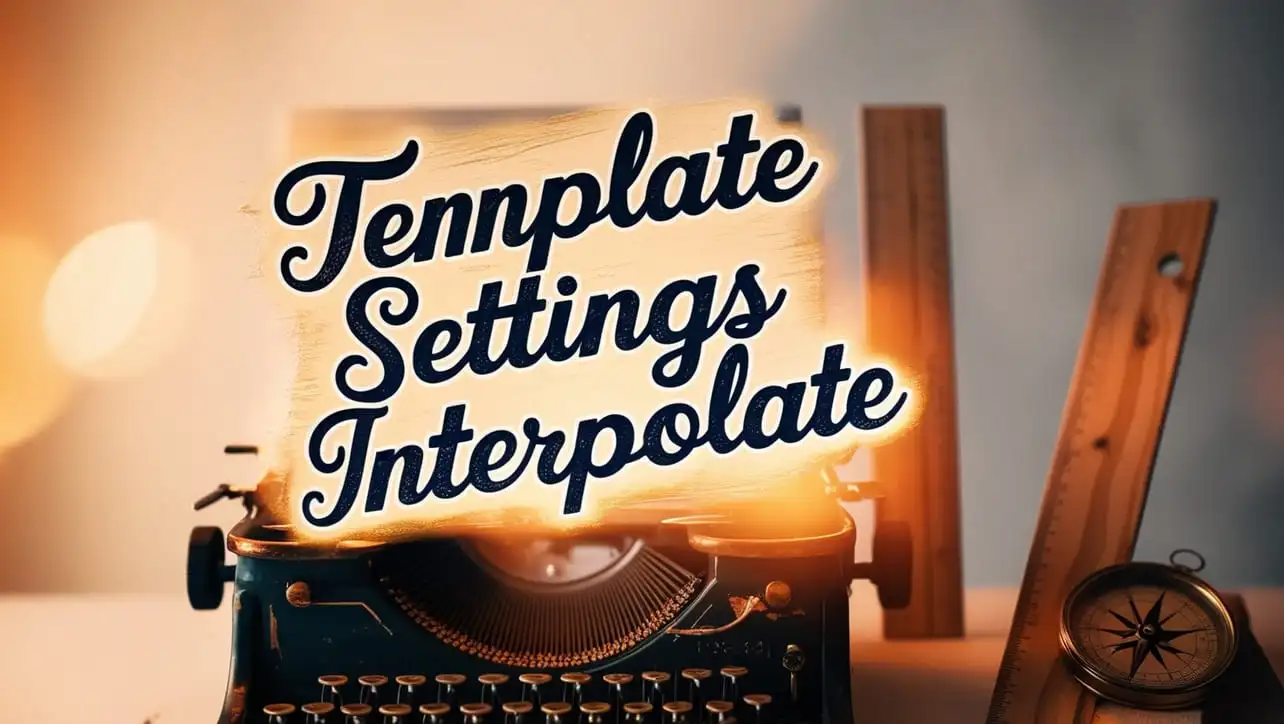
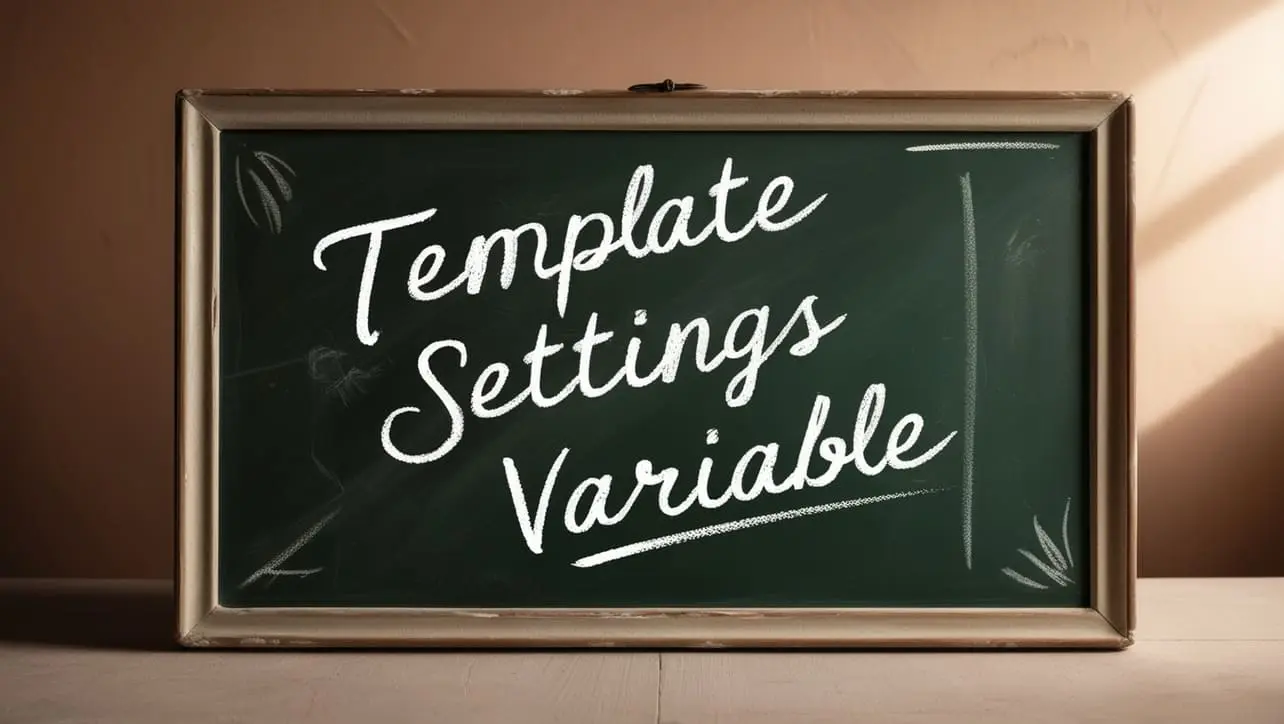
If you have any doubts regarding this article (Lodash _.invert() Object Method), please comment here. I will help you immediately.