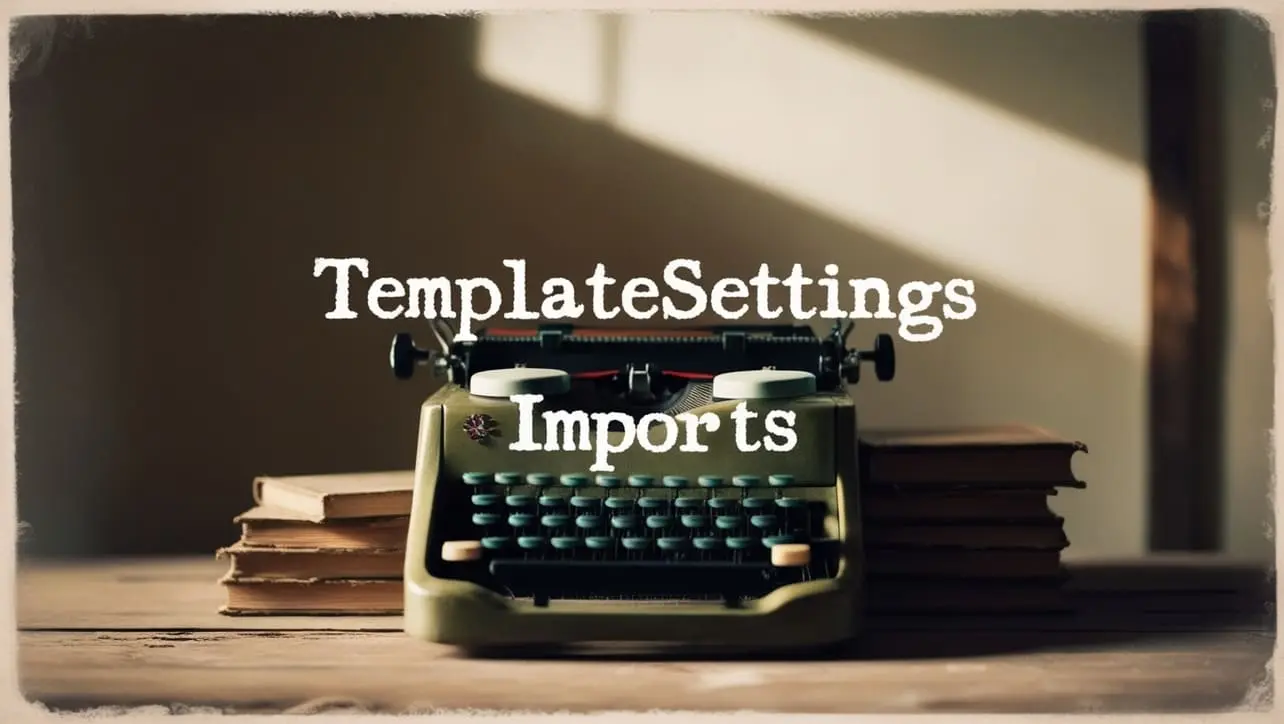
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.forOwnRight() Object Method
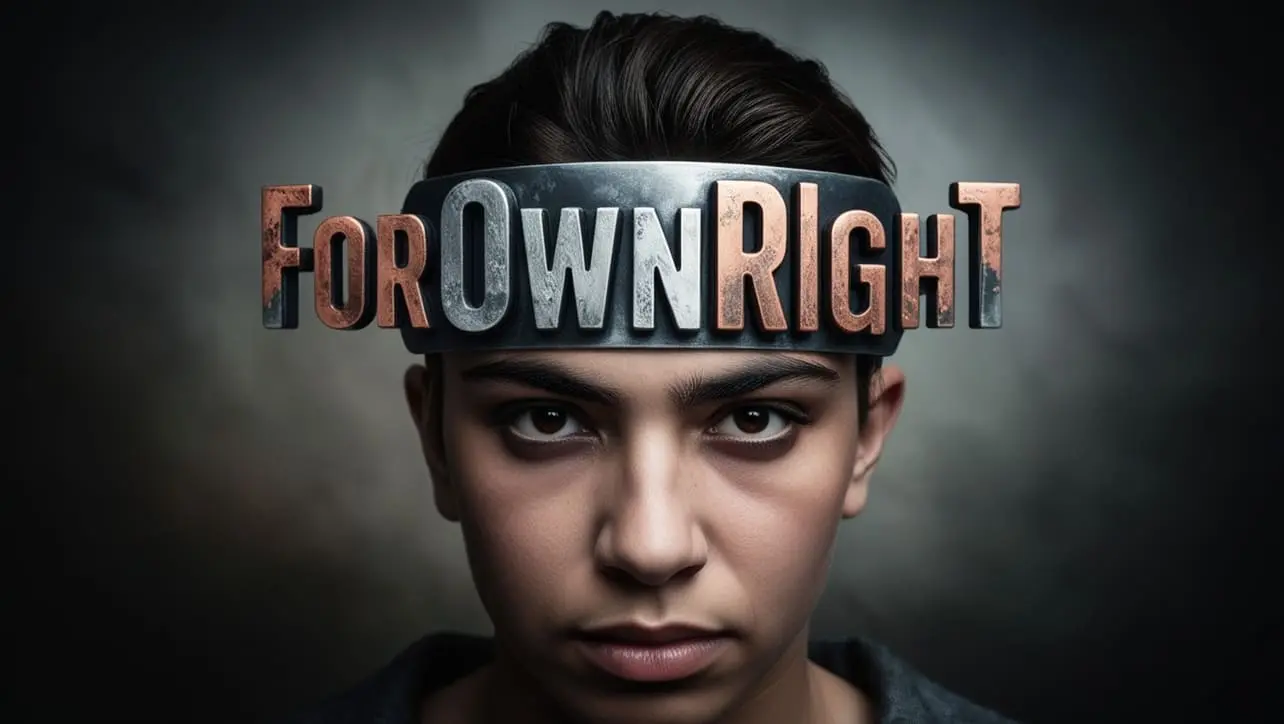
Photo Credit to CodeToFun
🙋 Introduction
In the diverse landscape of JavaScript development, effective manipulation of objects is crucial. Lodash, a powerful utility library, presents a variety of functions to simplify common tasks. Among these functions, the _.forOwnRight()
method stands out as a valuable tool for iterating over an object's own properties in reverse order.
This method provides flexibility and control, making it an essential asset for developers working with complex object structures.
🧠 Understanding _.forOwnRight() Method
The _.forOwnRight()
method in Lodash enables you to iterate over an object's own enumerable properties in reverse order. This is particularly useful when you need to perform operations on an object's properties in the opposite sequence of their creation. The method allows developers to apply a custom iteratee function to each property, providing a versatile approach to object manipulation.
💡 Syntax
The syntax for the _.forOwnRight()
method is straightforward:
_.forOwnRight(object, iteratee)
- object: The object to iterate over.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.forOwnRight()
method:
const _ = require('lodash');
const sampleObject = {
a: 1,
b: 2,
c: 3
};
_.forOwnRight(sampleObject, (value, key) => {
console.log(`${key}: ${value}`);
});
In this example, _.forOwnRight()
is used to iterate over the sampleObject in reverse order, logging each key-value pair to the console.
🏆 Best Practices
When working with the _.forOwnRight()
method, consider the following best practices:
Understand Property Enumeration Order:
Be aware that the order of property enumeration is not guaranteed in JavaScript objects.
_.forOwnRight()
iterates based on the order provided by Object.keys(). If order is crucial, consider using an array of key-value pairs.example.jsCopiedconst orderedObject = { b: 2, c: 3, a: 1 }; const orderedPairs = Object.entries(orderedObject); _.forOwnRight(orderedPairs, ([key, value]) => { console.log(`${key}: ${value}`); });
Use Custom Iteratee Functions:
Leverage the power of custom iteratee functions to tailor the behavior of
_.forOwnRight()
. This allows you to perform specific operations on each property during iteration.example.jsCopiedconst userPreferences = { theme: 'dark', fontSize: '16px', language: 'en' }; _.forOwnRight(userPreferences, (value, key) => { console.log(`Setting: ${key}, Value: ${value}`); // ... custom logic based on preferences ... });
Combine with Other Lodash Methods:
Explore the synergy between
_.forOwnRight()
and other Lodash methods to streamline complex operations on objects. Combinations with methods like _.mapValues() or _.transform() can lead to elegant and efficient code.example.jsCopiedconst data = { a: 10, b: 20, c: 30 }; const transformedObject = _.transform(data, (result, value, key) => { result[key] = value * 2; }, {}); _.forOwnRight(transformedObject, (value, key) => { console.log(`${key}: ${value}`); });
📚 Use Cases
Cleanup Operations:
Perform cleanup operations on an object by using
_.forOwnRight()
to iterate over properties and remove or modify them based on specific conditions.example.jsCopiedconst userData = { name: 'John', age: 25, isAdmin: false, lastLogin: null }; _.forOwnRight(userData, (value, key, obj) => { if(value === null || value === undefined) { delete obj[key]; } }); console.log(userData); // Output: { name: 'John', age: 25, isAdmin: false }
Configurable Settings:
Use
_.forOwnRight()
to iterate over a settings object, allowing users to customize their preferences, and applying those preferences in reverse order for priority.example.jsCopiedconst userSettings = { theme: 'light', fontSize: '14px', language: 'fr' }; _.forOwnRight(userSettings, (value, key) => { // Apply user settings in reverse order for priority console.log(`Applying Setting: ${key}, Value: ${value}`); // ... apply settings ... });
Data Transformation:
Utilize
_.forOwnRight()
in conjunction with other Lodash methods for data transformation, converting object properties based on specific criteria.example.jsCopiedconst temperatureData = { Monday: 30, Tuesday: 32, Wednesday: 28, Thursday: 26 }; const convertedTemperatures = _.mapValues(temperatureData, value => (value - 32) * (5 / 9)); _.forOwnRight(convertedTemperatures, (value, key) => { console.log(`${key}: ${value.toFixed(2)}°C`); });
🎉 Conclusion
The _.forOwnRight()
method in Lodash provides a powerful and flexible approach to iterating over an object's own properties in reverse order. Whether you're performing cleanup operations, configuring settings, or transforming data, this method offers a valuable tool for efficient object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.forOwnRight()
method in your Lodash projects.
👨💻 Join our Community:
Author
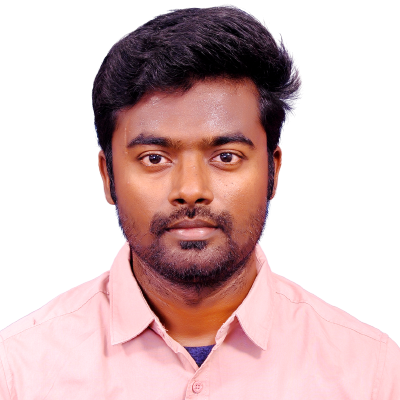
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
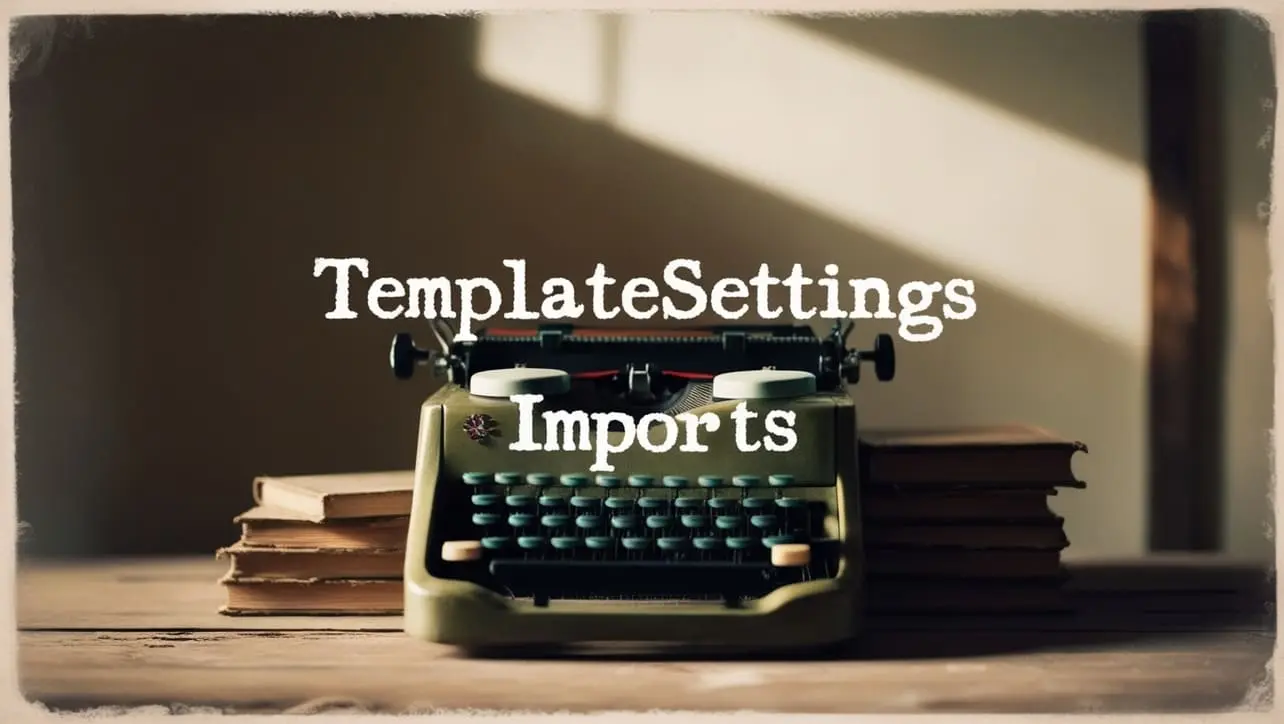
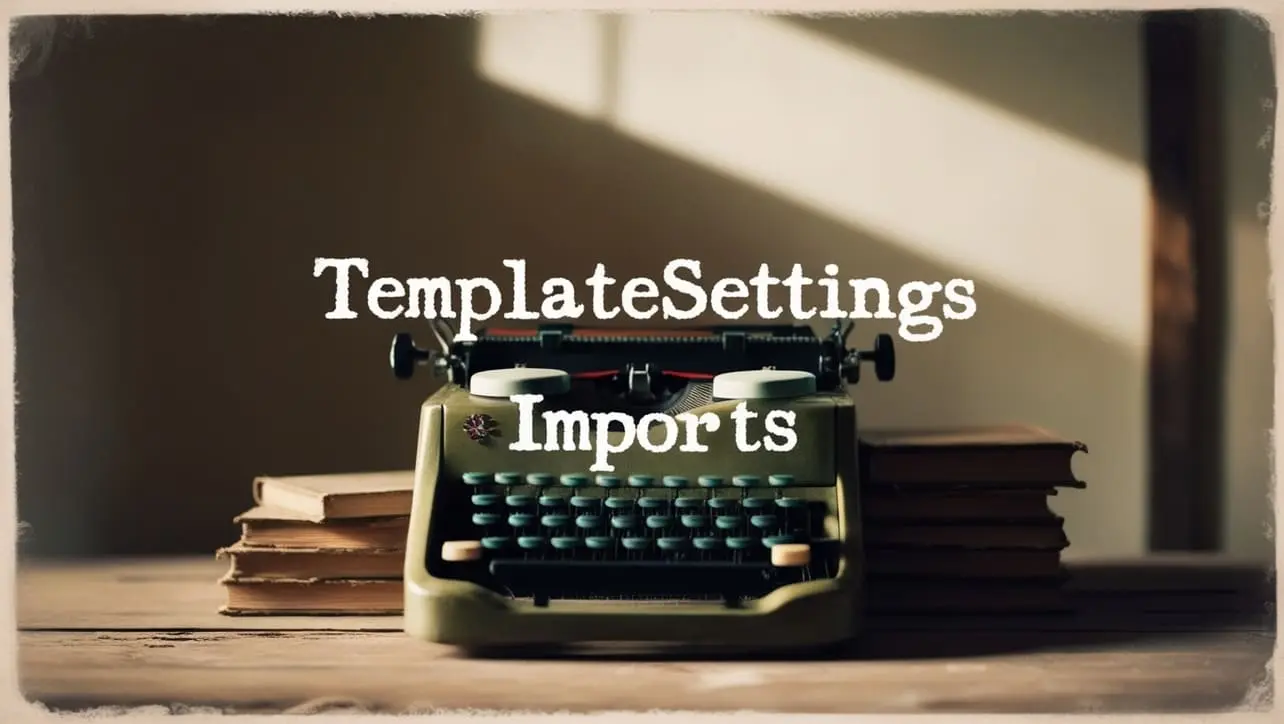
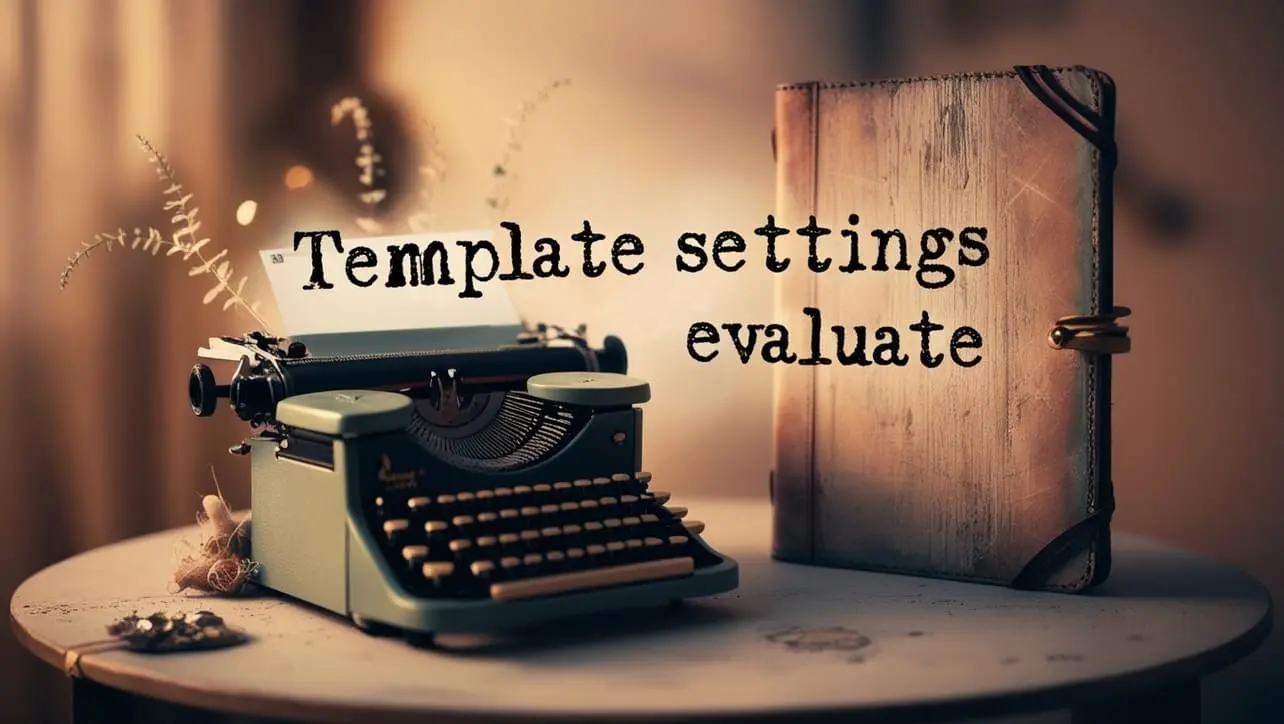
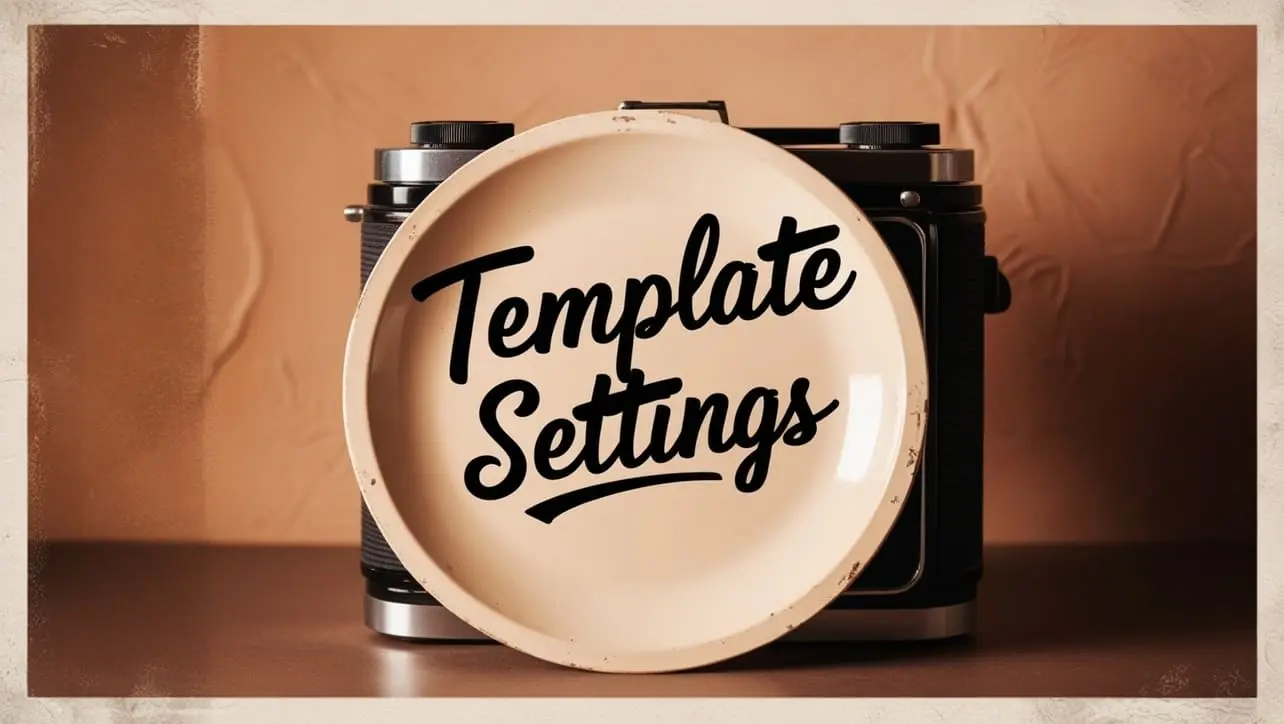
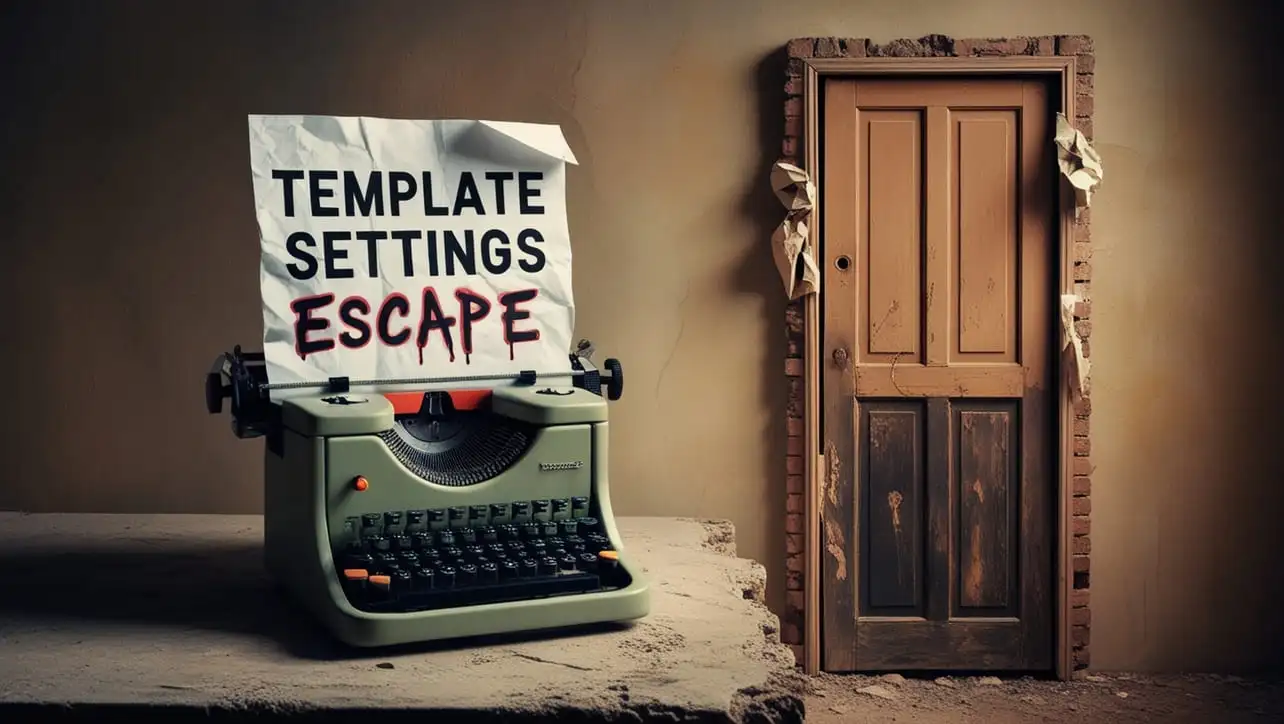
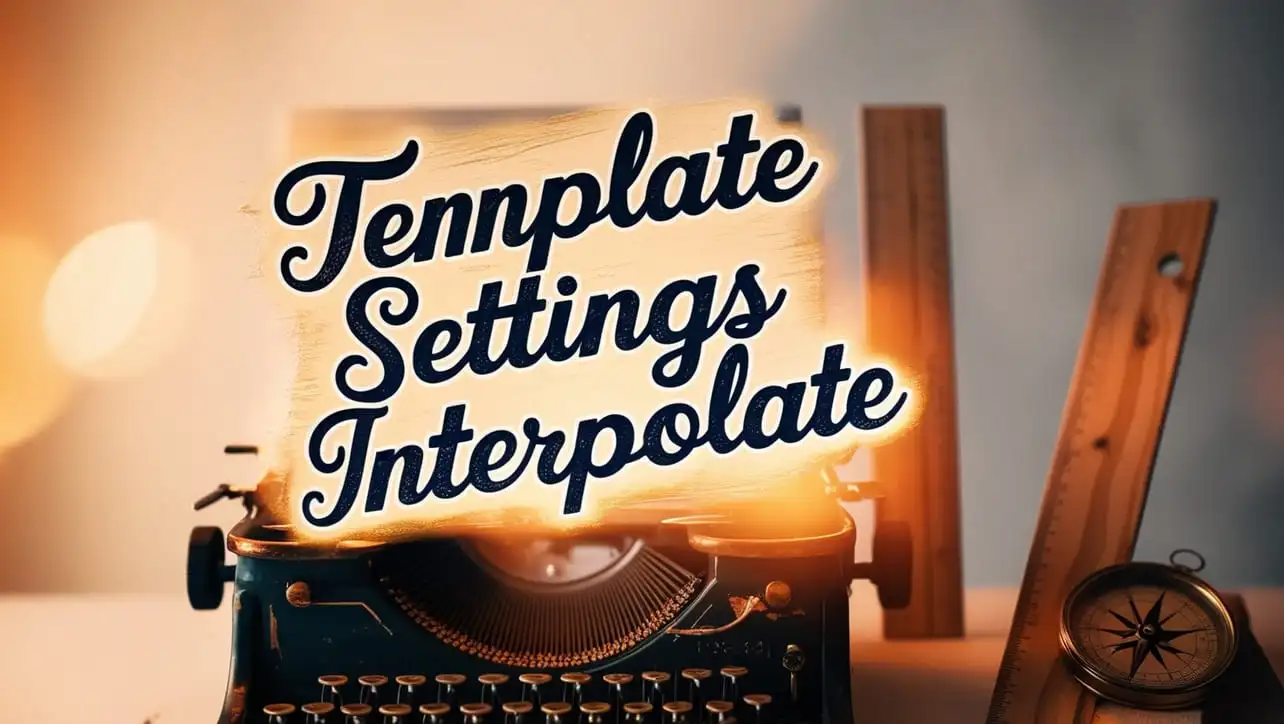
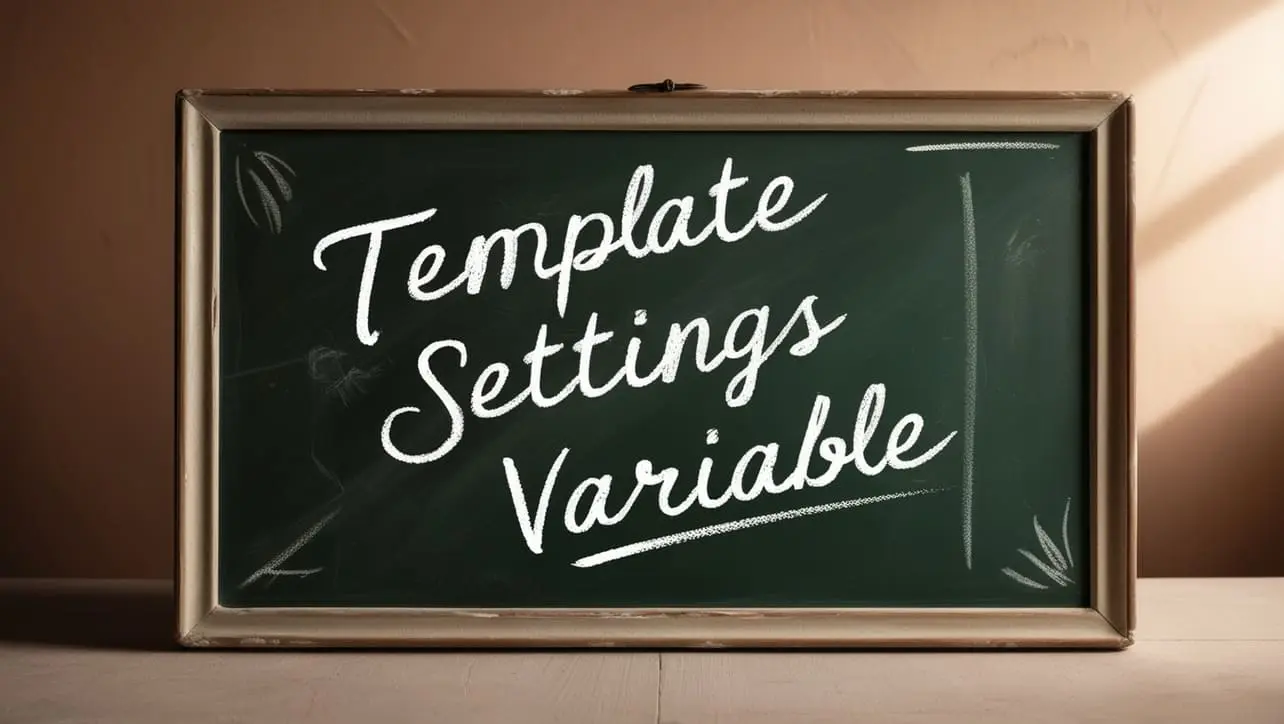
If you have any doubts regarding this article (Lodash _.forOwnRight() Object Method), please comment here. I will help you immediately.