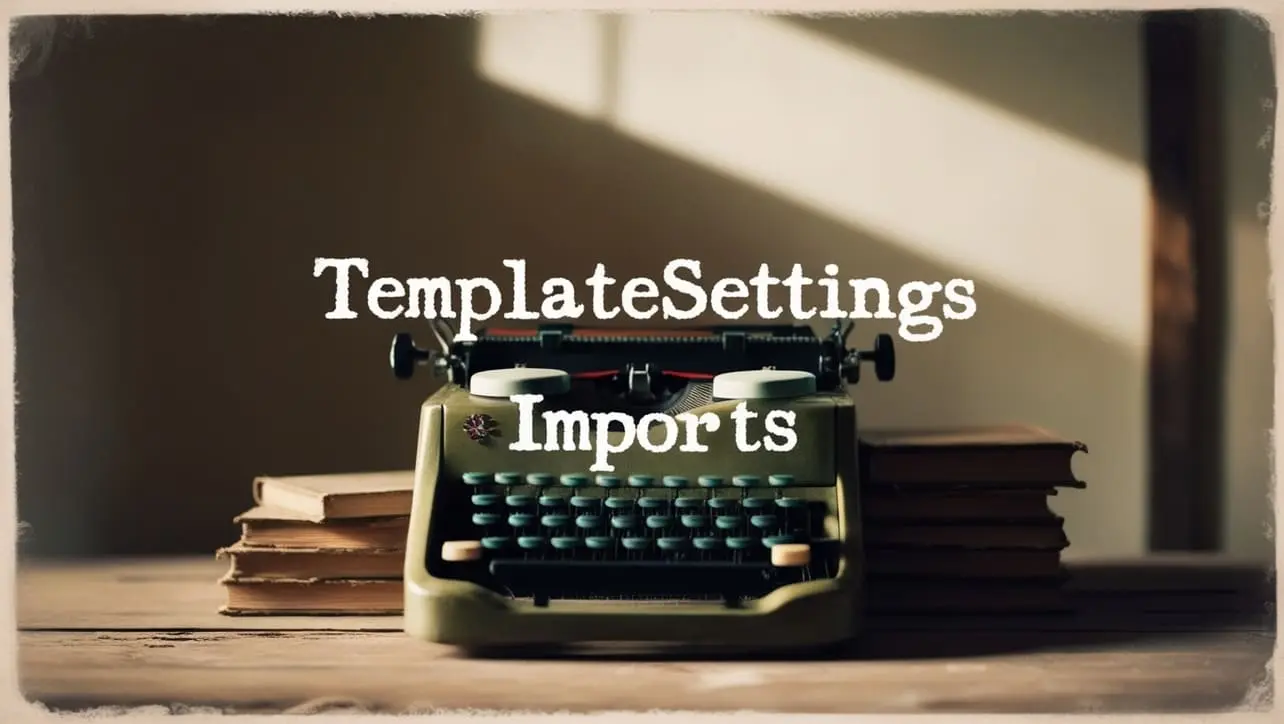
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.forInRight() Object Method
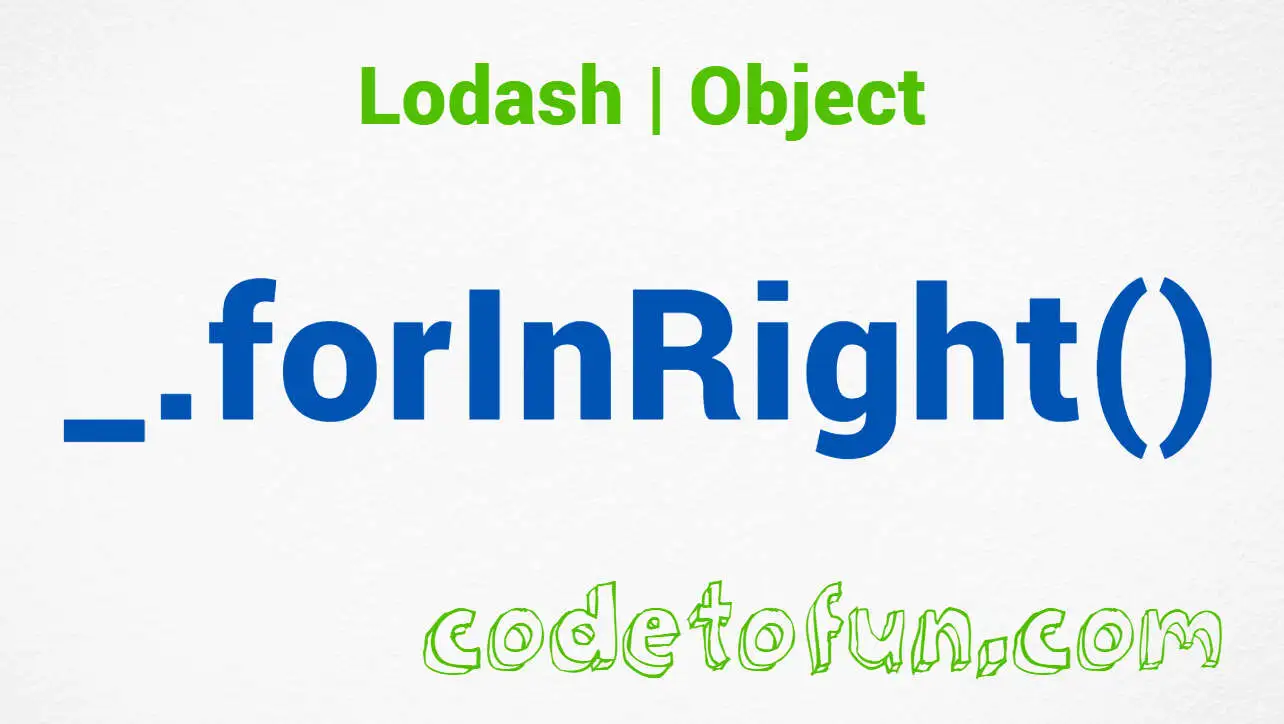
Photo Credit to CodeToFun
🙋 Introduction
When it comes to traversing and manipulating objects in JavaScript, Lodash provides an extensive set of utility functions. One such powerful method is _.forInRight()
, designed for iterating over an object's own and inherited enumerable string keyed properties in reverse order.
This method offers flexibility and ease of use, making it an invaluable tool for developers working with complex object structures.
🧠 Understanding _.forInRight() Method
The _.forInRight()
method in Lodash is part of the object iteration category, allowing you to iterate over an object's properties in reverse order. This method executes a provided function for each key-value pair, providing a convenient way to perform operations on objects.
💡 Syntax
The syntax for the _.forInRight()
method is straightforward:
_.forInRight(object, iteratee)
- object: The object to iterate over.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.forInRight()
method:
const _ = require('lodash');
const sampleObject = {
name: 'John',
age: 30,
city: 'New York',
};
_.forInRight(sampleObject, (value, key) => {
console.log(`${key}: ${value}`);
});
In this example, _.forInRight()
iterates over the sampleObject in reverse order, logging each key-value pair to the console.
🏆 Best Practices
When working with the _.forInRight()
method, consider the following best practices:
Reverse Order Iteration:
Take advantage of
_.forInRight()
to iterate over object properties in reverse order when order matters. This can be beneficial in scenarios where the order of operations is critical.example.jsCopiedconst userPreferences = { language: 'English', theme: 'Dark', fontSize: 'Medium', }; _.forInRight(userPreferences, (value, key) => { console.log(`${key}: ${value}`); });
Handle Inherited Properties:
Be aware that
_.forInRight()
iterates over both own and inherited properties of an object. If you only want to iterate over an object's own properties, consider using _.forOwnRight().example.jsCopiedclass Animal { constructor(name) { this.name = name; } } Animal.prototype.sound = 'Unknown'; const cat = new Animal('Whiskers'); _.forInRight(cat, (value, key) => { console.log(`${key}: ${value}`); });
Utilize Callback Function:
Use the provided iteratee function to perform custom operations on each key-value pair during iteration. This provides a flexible way to tailor the behavior of
_.forInRight()
to your specific needs.example.jsCopiedconst dataObject = { key1: 'value1', key2: 'value2', key3: 'value3', }; _.forInRight(dataObject, (value, key) => { console.log(`Uppercase ${key}: ${value.toUpperCase()}`); });
📚 Use Cases
Data Transformation:
_.forInRight()
can be used for transforming data within an object, especially when the order of transformation matters.example.jsCopiedconst numericalData = { a: 5, b: 10, c: 15, }; _.forInRight(numericalData, (value, key, obj) => { obj[key] = value * 2; }); console.log(numericalData); // Output: { a: 10, b: 20, c: 30 }
Configuration Initialization:
When initializing configurations or settings,
_.forInRight()
can help ensure that the last defined property takes precedence.example.jsCopiedconst defaultConfig = { theme: 'Light', fontSize: 'Medium', }; const userConfig = { fontSize: 'Large', language: 'Spanish', }; _.forInRight(userConfig, (value, key) => { defaultConfig[key] = value; }); console.log(defaultConfig); // Output: { theme: 'Light', fontSize: 'Large', language: 'Spanish' }
Cleanup Operations:
Perform cleanup operations on an object by using
_.forInRight()
to iterate in reverse order, ensuring dependencies or references are properly handled.example.jsCopiedconst cleanupObject = { data: '...', connection: /* ... */ , resource: /* ... */ , }; _.forInRight(cleanupObject, (value, key, obj) => { // Perform cleanup operations, e.g., disconnecting, releasing resources, etc. delete obj[key]; }); console.log(cleanupObject); // Output: {}
🎉 Conclusion
The _.forInRight()
method in Lodash is a versatile tool for iterating over object properties in reverse order, offering developers the flexibility to perform various operations. Whether you need to transform data, handle configurations, or perform cleanup operations, _.forInRight()
provides a convenient and efficient solution.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.forInRight()
method in your Lodash projects.
👨💻 Join our Community:
Author
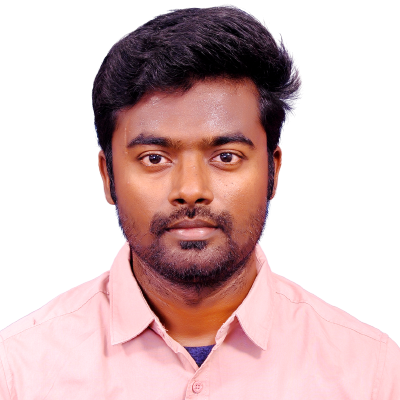
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
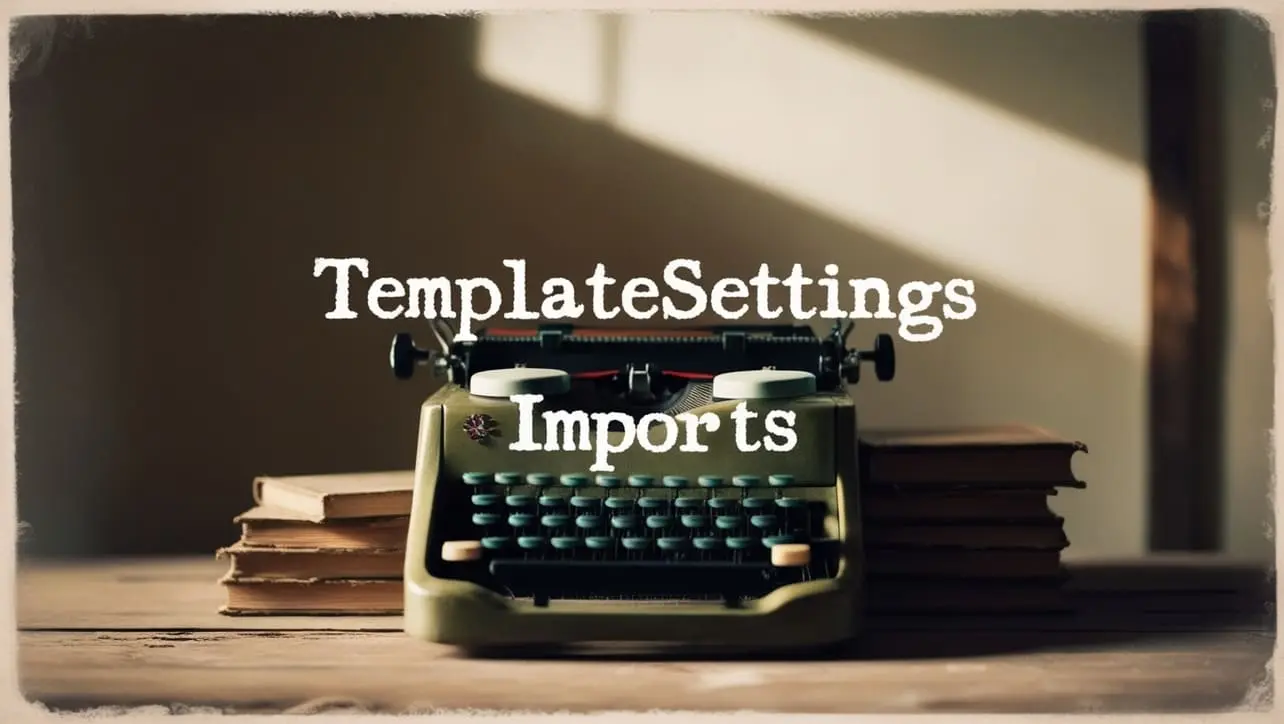
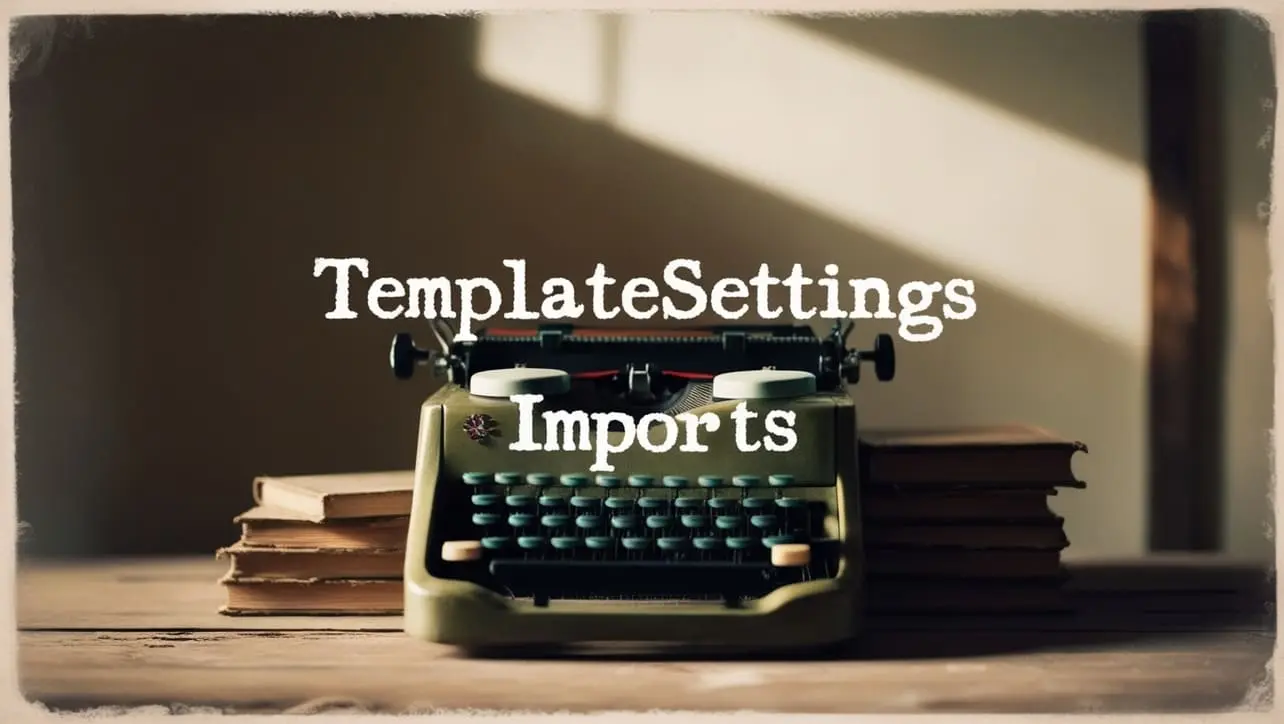
Lodash _.templateSettings.imports Property
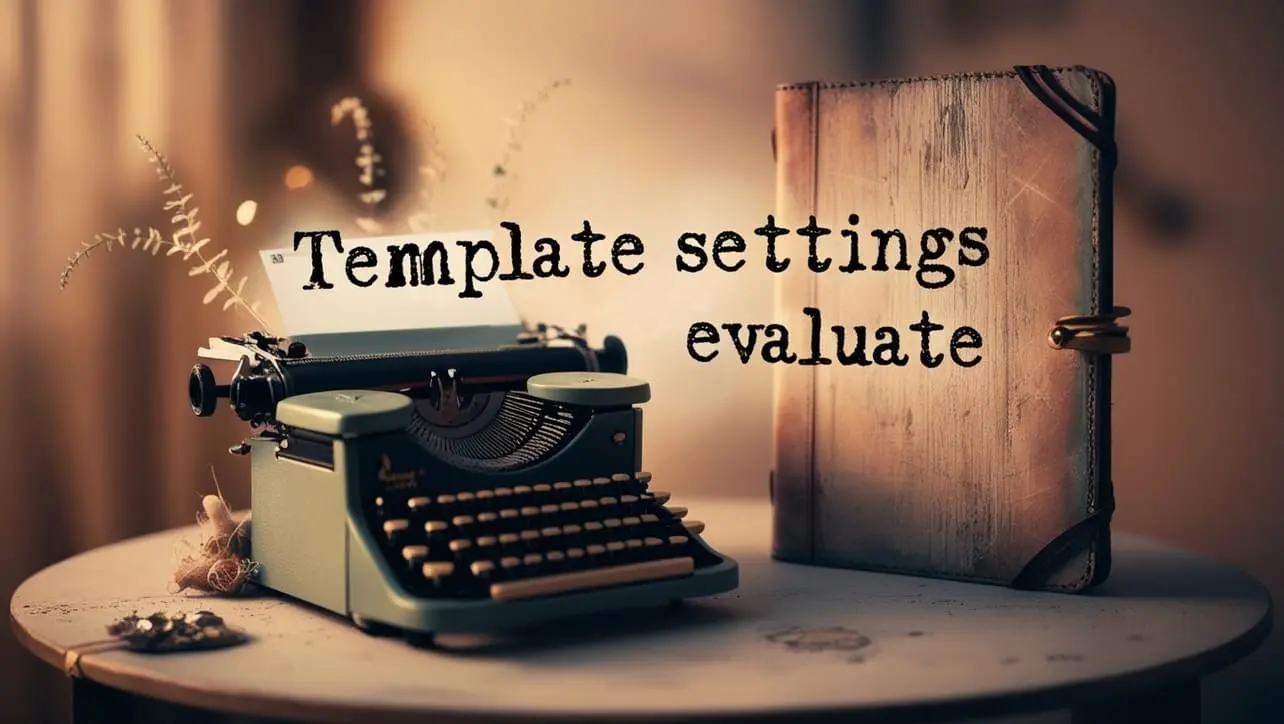
Lodash _.templateSettings.evaluate Property
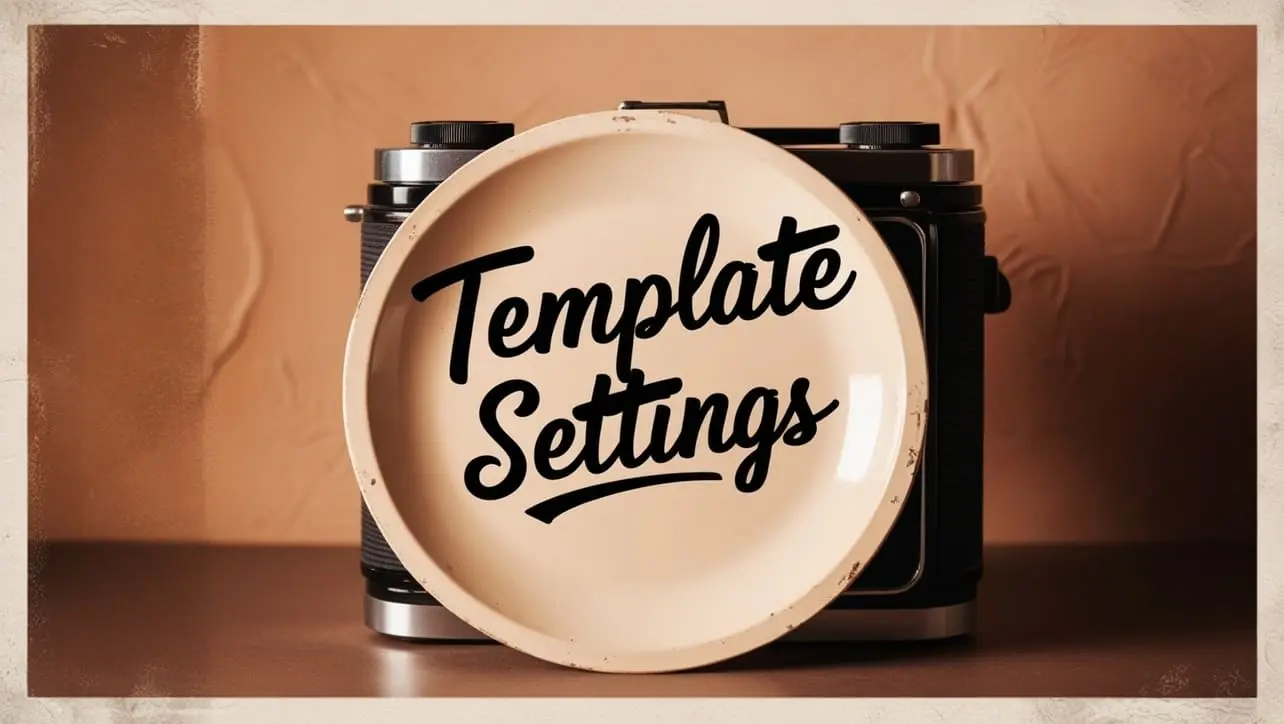
Lodash _.templateSettings Property
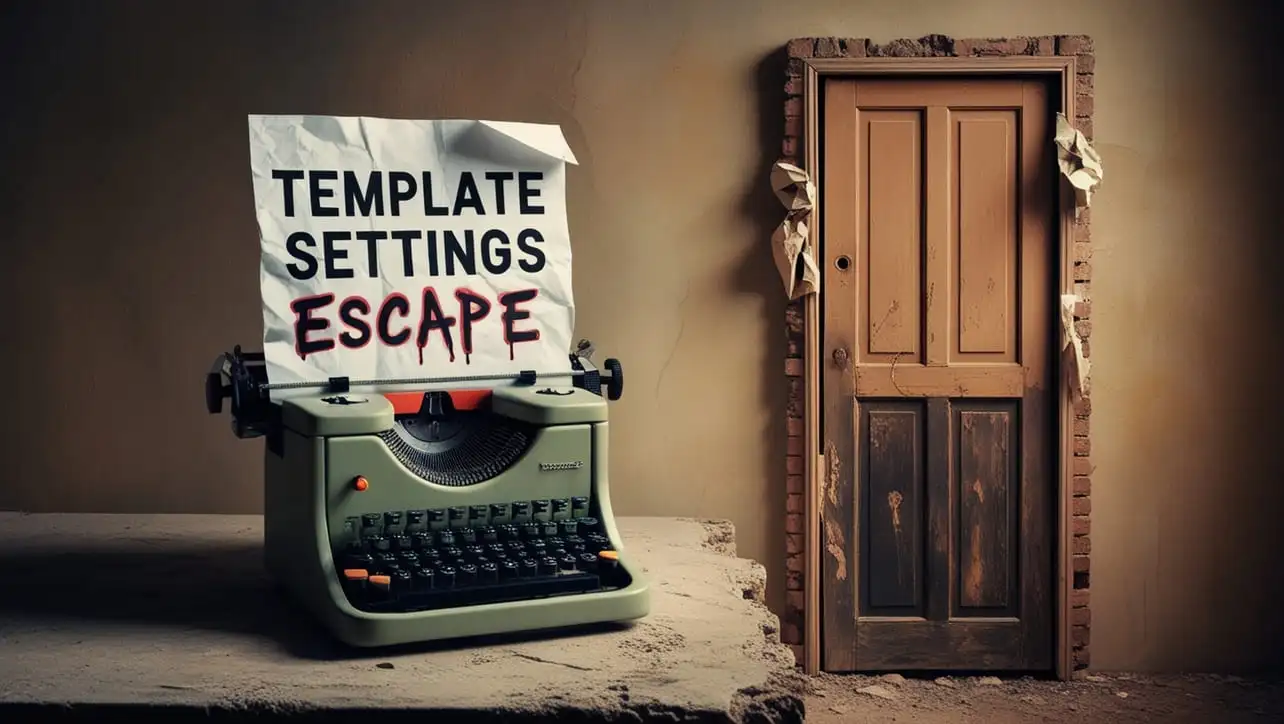
Lodash _.templateSettings.escape Property
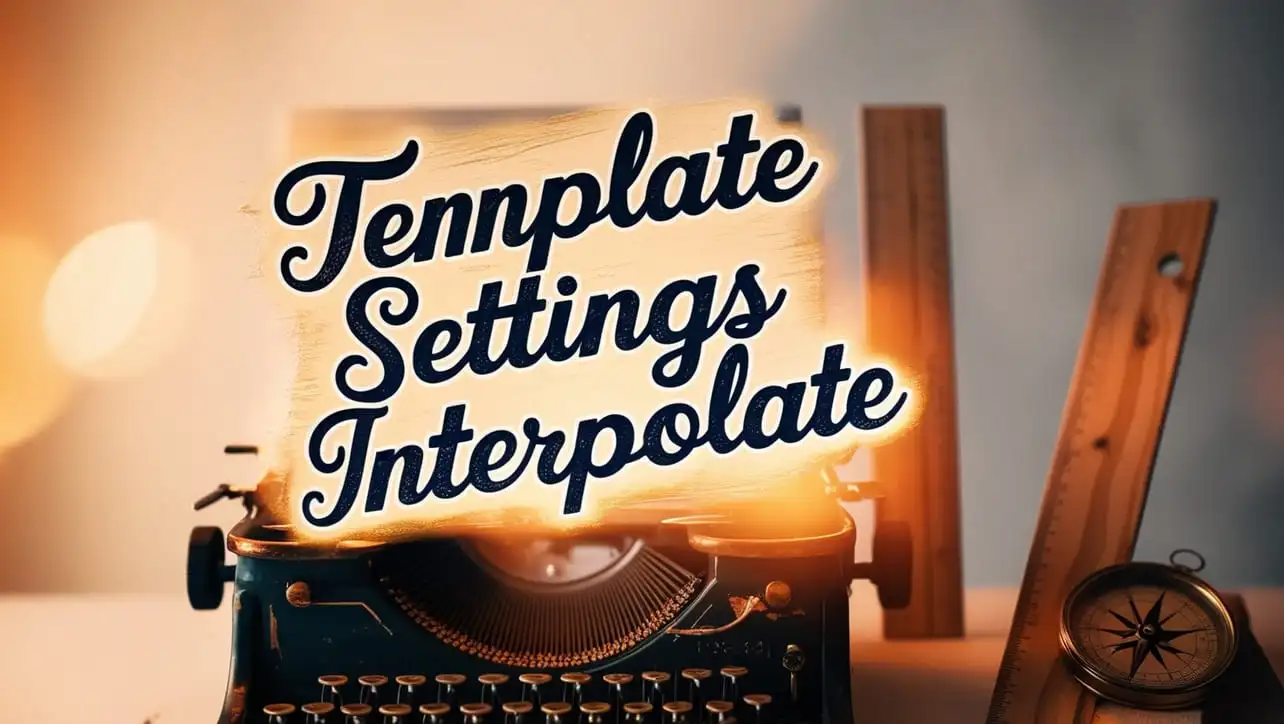
Lodash _.templateSettings.interpolate Property
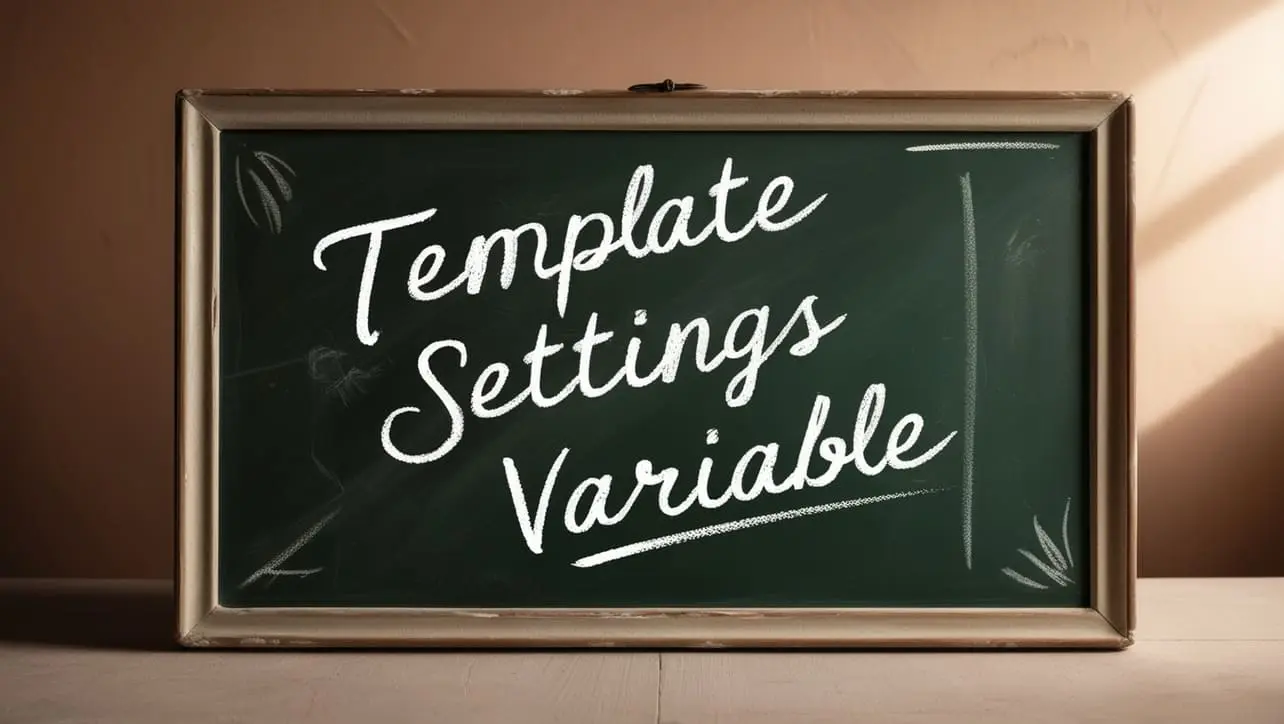
If you have any doubts regarding this article (Lodash _.forInRight() Object Method), please comment here. I will help you immediately.