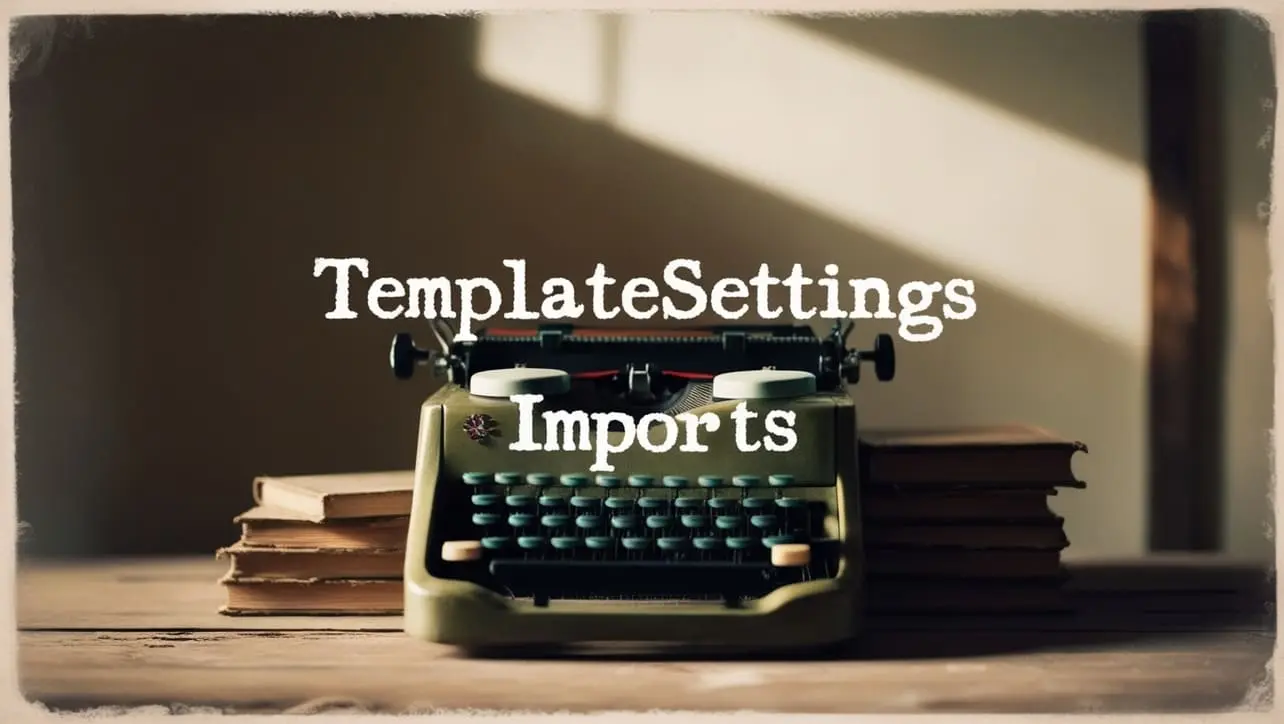
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.findKey() Object Method
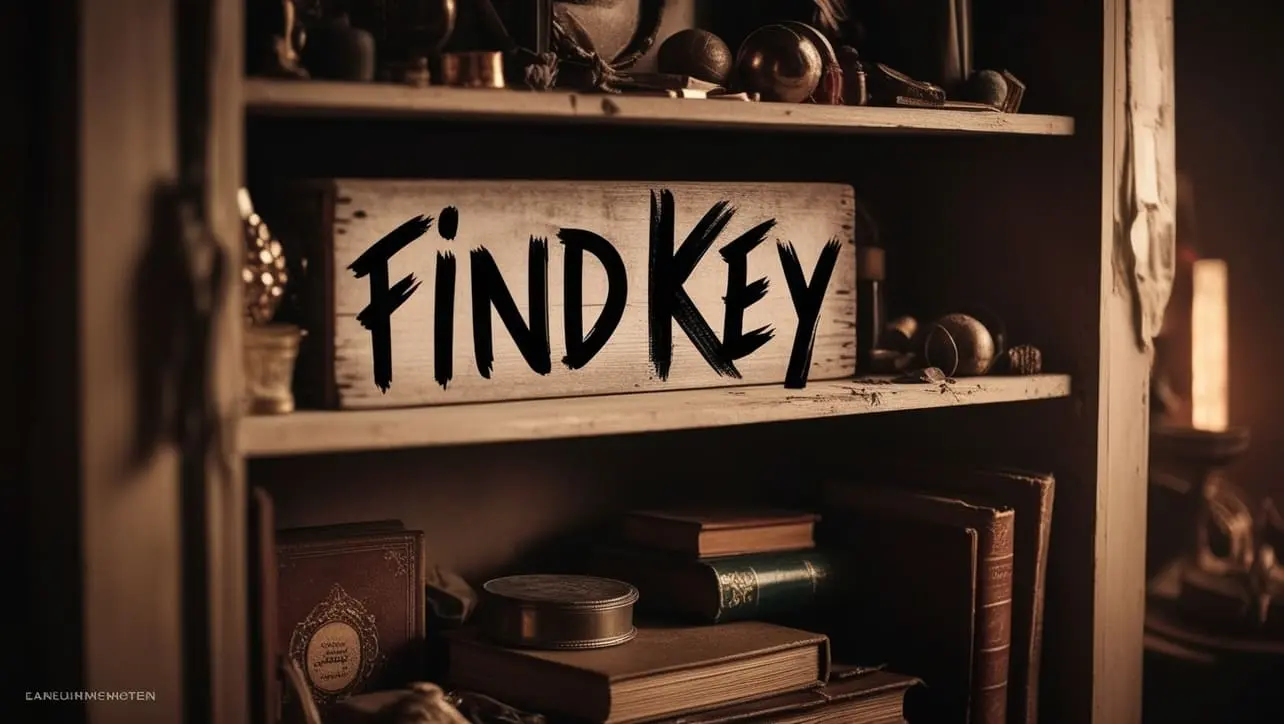
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficient manipulation of objects is a fundamental aspect of creating robust applications. Lodash, a powerful utility library, provides a plethora of functions to simplify object handling. Among these functions is the _.findKey()
method, a versatile tool for searching through objects based on a provided predicate.
This method is invaluable for developers seeking an elegant solution to find keys that match specific criteria.
🧠 Understanding _.findKey() Method
The _.findKey()
method in Lodash is designed to search for a key in an object that satisfies a given condition. This allows developers to locate the key that corresponds to a particular value or meets a custom criterion.
💡 Syntax
The syntax for the _.findKey()
method is straightforward:
_.findKey(object, [predicate])
- object: The object to search.
- predicate (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.findKey()
method:
const _ = require('lodash');
const sampleObject = {
apple: {
color: 'red',
type: 'fruit'
},
banana: {
color: 'yellow',
type: 'fruit'
},
carrot: {
color: 'orange',
type: 'vegetable'
},
};
const key = _.findKey(sampleObject, {
color: 'yellow'
});
console.log(key);
// Output: banana
In this example, _.findKey()
is used to find the key associated with an object having the specified color property.
🏆 Best Practices
When working with the _.findKey()
method, consider the following best practices:
Understand the Object Structure:
Before applying
_.findKey()
, have a clear understanding of the structure of the object. Knowing the structure helps in creating effective predicates for accurate key detection.example.jsCopiedconst complexObject = { user: { details: { name: 'John Doe', age: 30, address: { city: 'Example City', country: 'Example Country' }, }, }, isAdmin: false, }; const keyInComplexObject = _.findKey(complexObject, { 'user.details.age': 30 }); console.log(keyInComplexObject); // Output: user
Use Custom Predicates:
Take advantage of the predicate parameter to create custom conditions for key identification. This allows for flexible and dynamic searches based on specific criteria.
example.jsCopiedconst users = { user1: { age: 25, isAdmin: true }, user2: { age: 30, isAdmin: false }, user3: { age: 22, isAdmin: true }, }; const adminKey = _.findKey(users, user => user.isAdmin); console.log(adminKey); // Output: user1
Handle Edge Cases:
Consider scenarios where the object may be empty or the predicate does not match any keys. Implement appropriate error handling or default behaviors to address these edge cases.
example.jsCopiedconst emptyObject = {}; const nonMatchingPredicate = _.findKey(emptyObject, { property: 'value' }); console.log(nonMatchingPredicate); // Output: undefined
📚 Use Cases
Finding a Key by Value:
_.findKey()
is particularly useful when you need to find a key based on the value associated with it. This is common in scenarios where you want to locate a specific item in a collection.example.jsCopiedconst ages = { person1: 25, person2: 30, person3: 22, }; const targetAgeKey = _.findKey(ages, age => age === 30); console.log(targetAgeKey); // Output: person2
Dynamic Key Detection:
When dealing with dynamic data structures,
_.findKey()
enables dynamic key detection based on changing conditions or criteria.example.jsCopiedconst dynamicData = { optionA: { enabled: true, value: 'A' }, optionB: { enabled: false, value: 'B' }, optionC: { enabled: true, value: 'C' }, }; const enabledOptionKey = _.findKey(dynamicData, option => option.enabled); console.log(enabledOptionKey); // Output: optionA
Key Detection in Nested Objects:
For objects with nested structures,
_.findKey()
proves valuable in searching for keys deep within the hierarchy.example.jsCopiedconst nestedObject = { user: { details: { name: 'Jane Doe', age: 28, isAdmin: false, }, }, admin: { details: { name: 'Admin User', age: 35, isAdmin: true, }, }, }; const adminKeyInNestedObject = _.findKey(nestedObject, { 'user.details.isAdmin': true }); console.log(adminKeyInNestedObject); // Output: admin
🎉 Conclusion
The _.findKey()
method in Lodash provides a robust solution for searching through objects and identifying keys based on specified conditions. Whether you're searching for a key by value, implementing dynamic key detection, or navigating nested object structures, _.findKey()
offers a versatile and efficient approach.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.findKey()
method in your Lodash projects.
👨💻 Join our Community:
Author
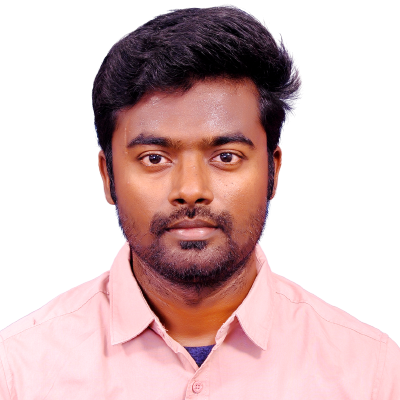
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
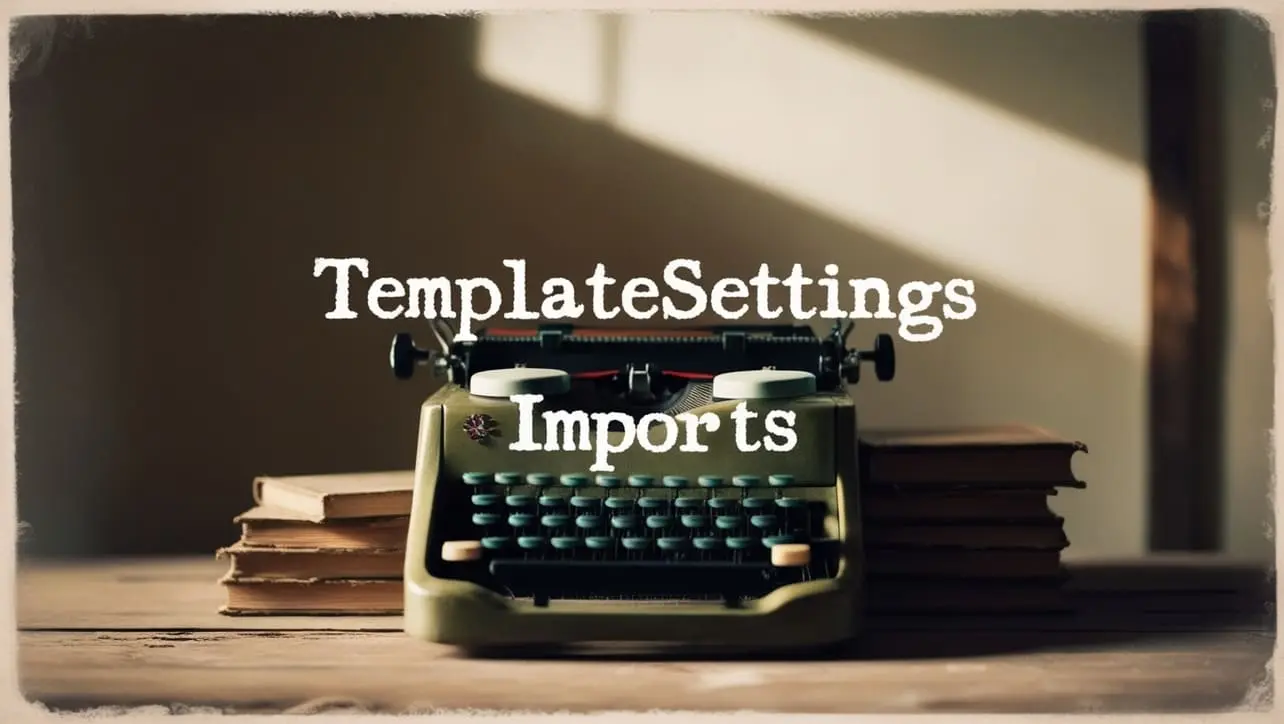
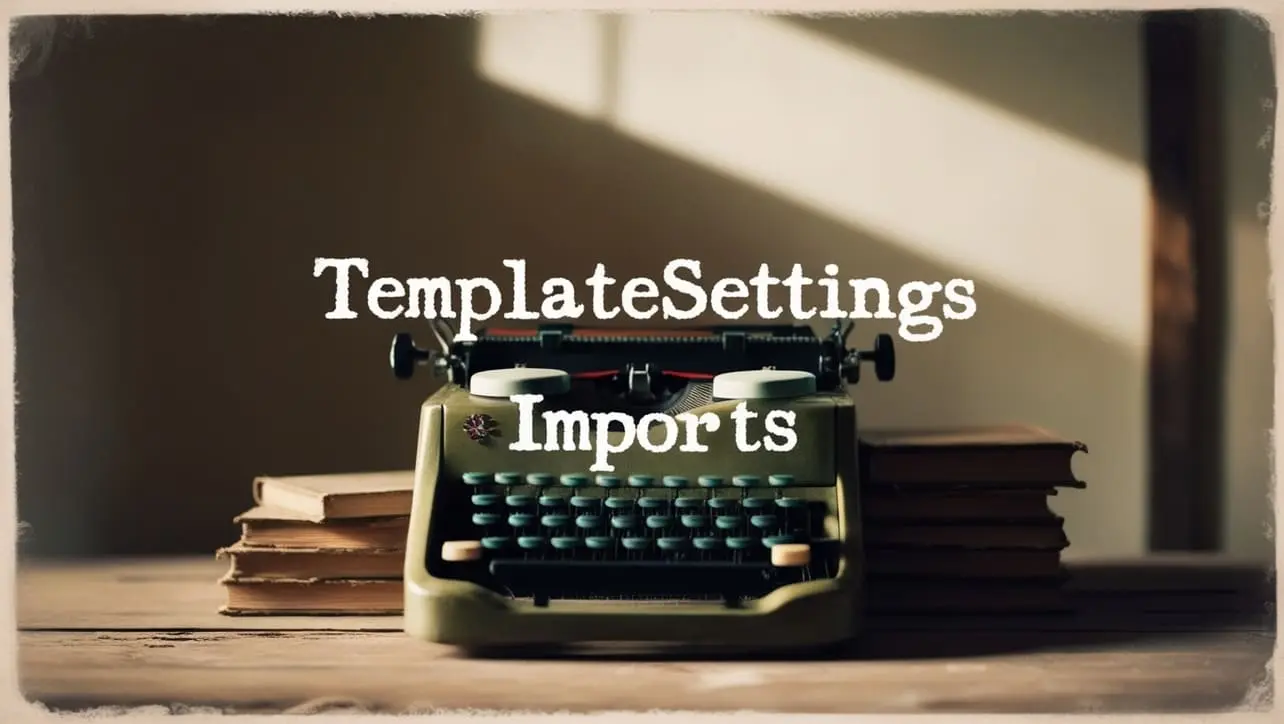
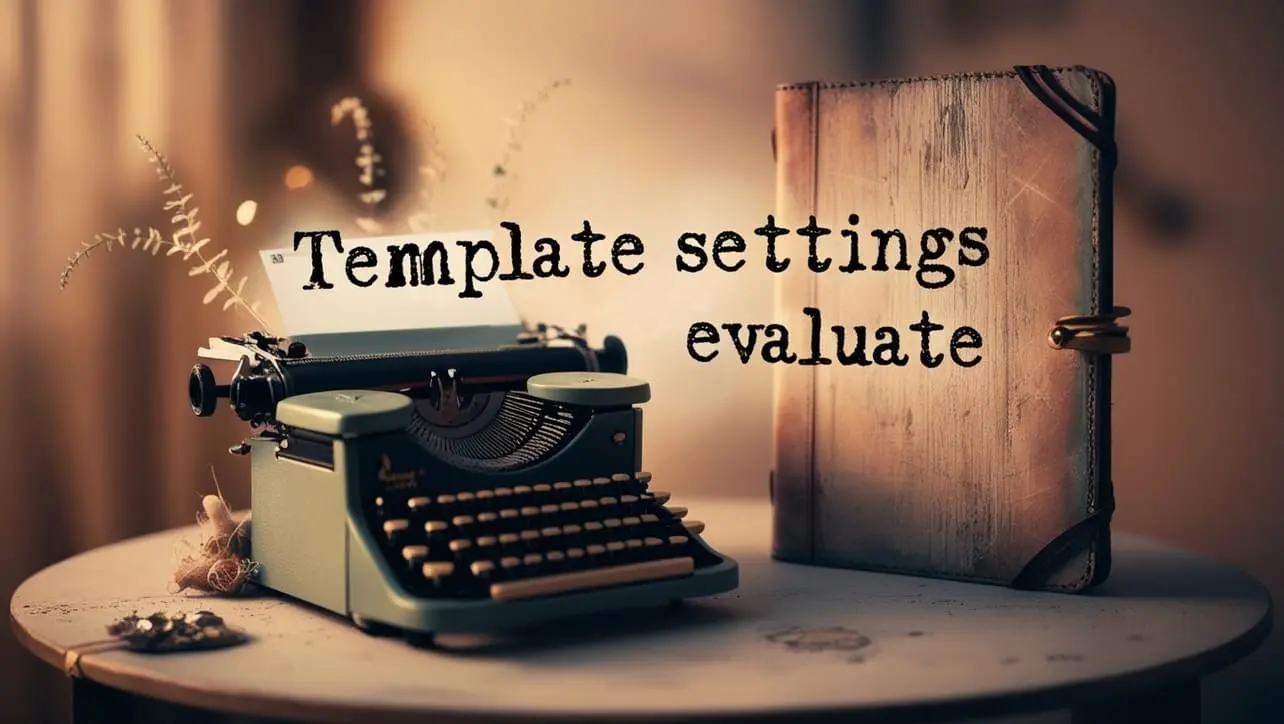
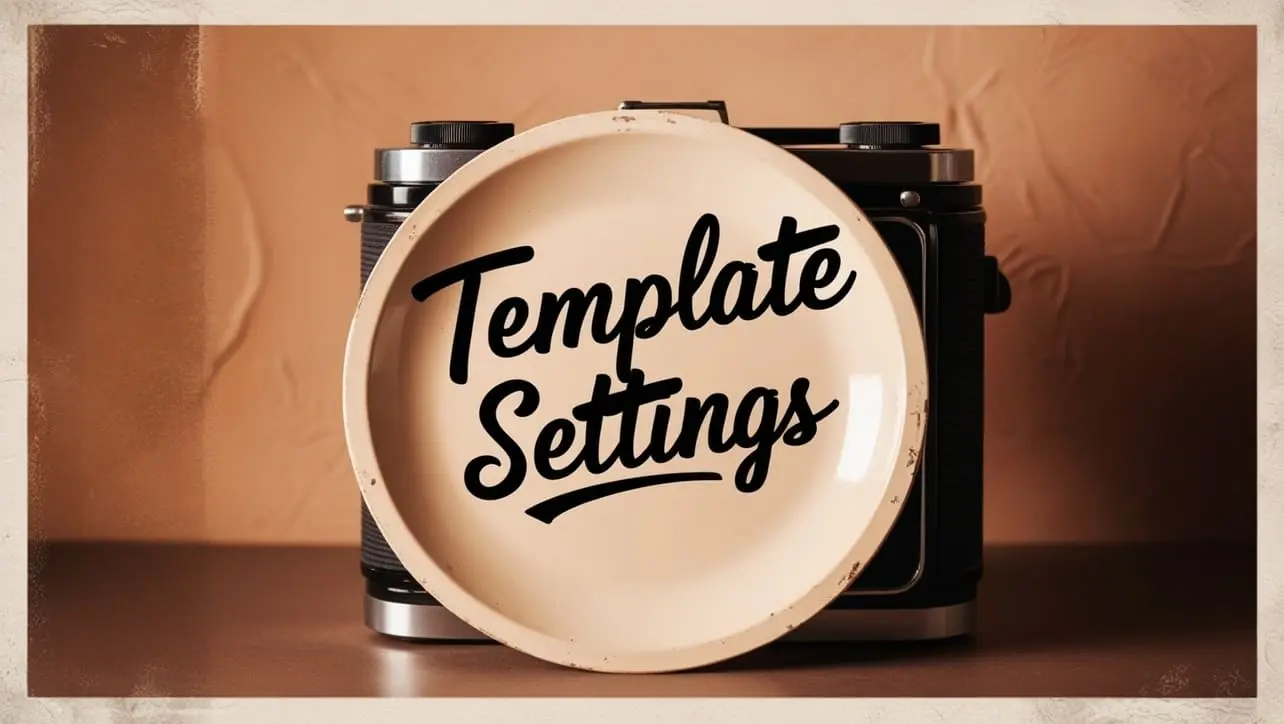
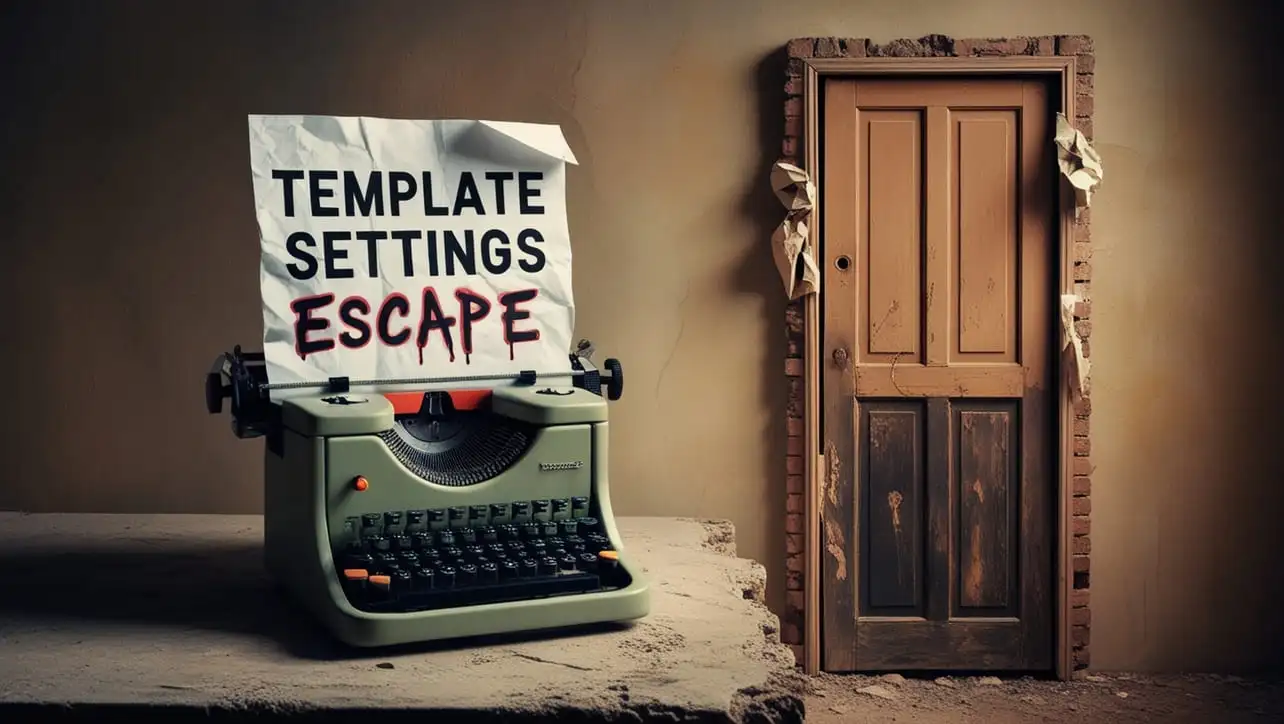
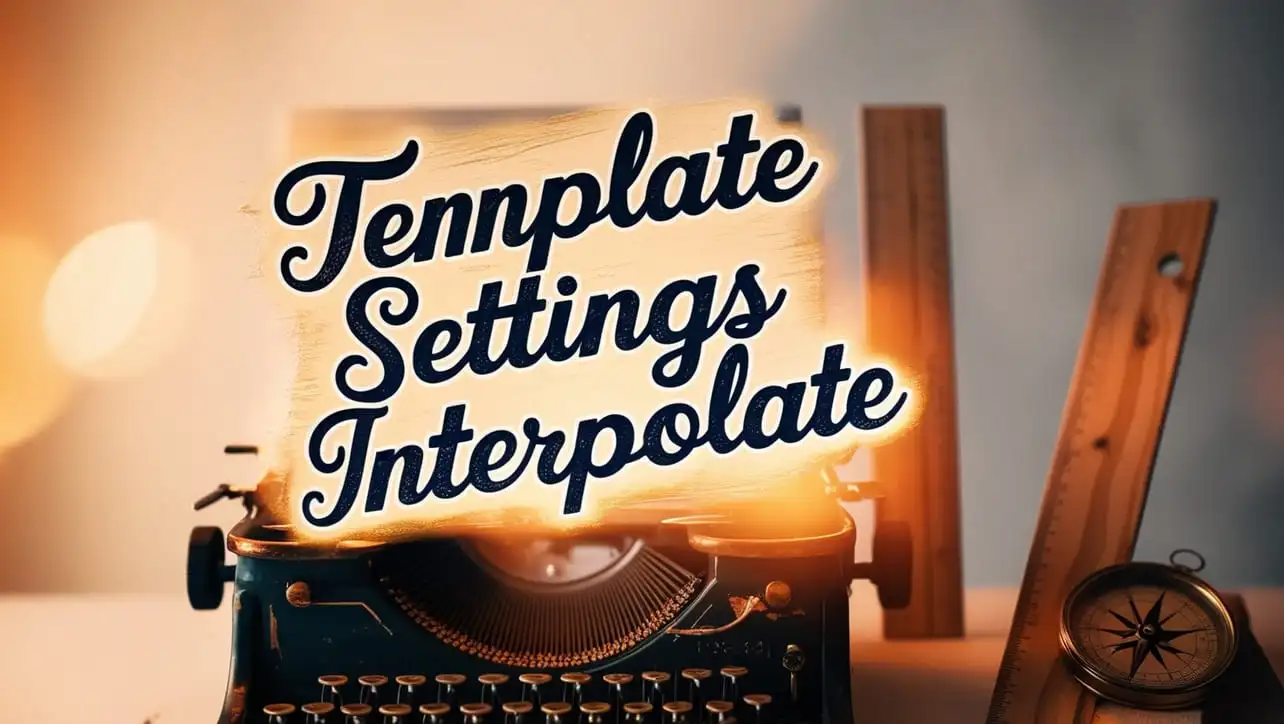
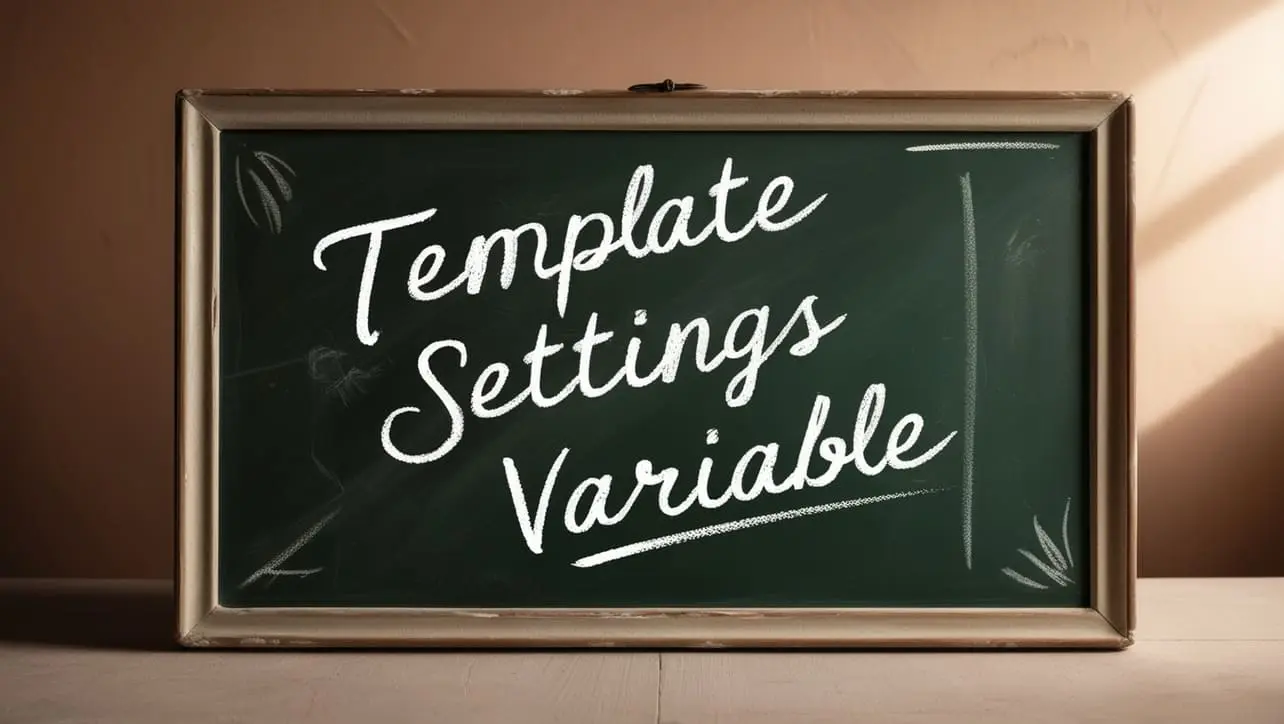
If you have any doubts regarding this article (Lodash _.findKey() Object Method), please comment here. I will help you immediately.