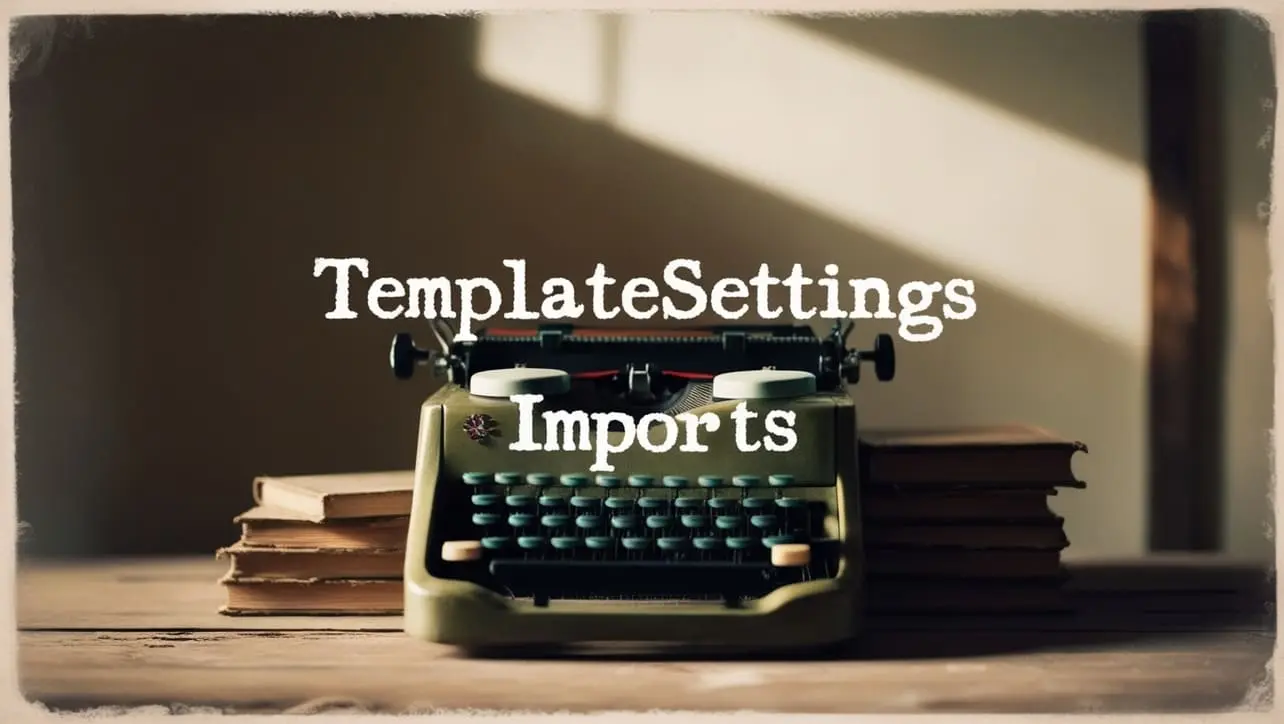
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.at() Object Method
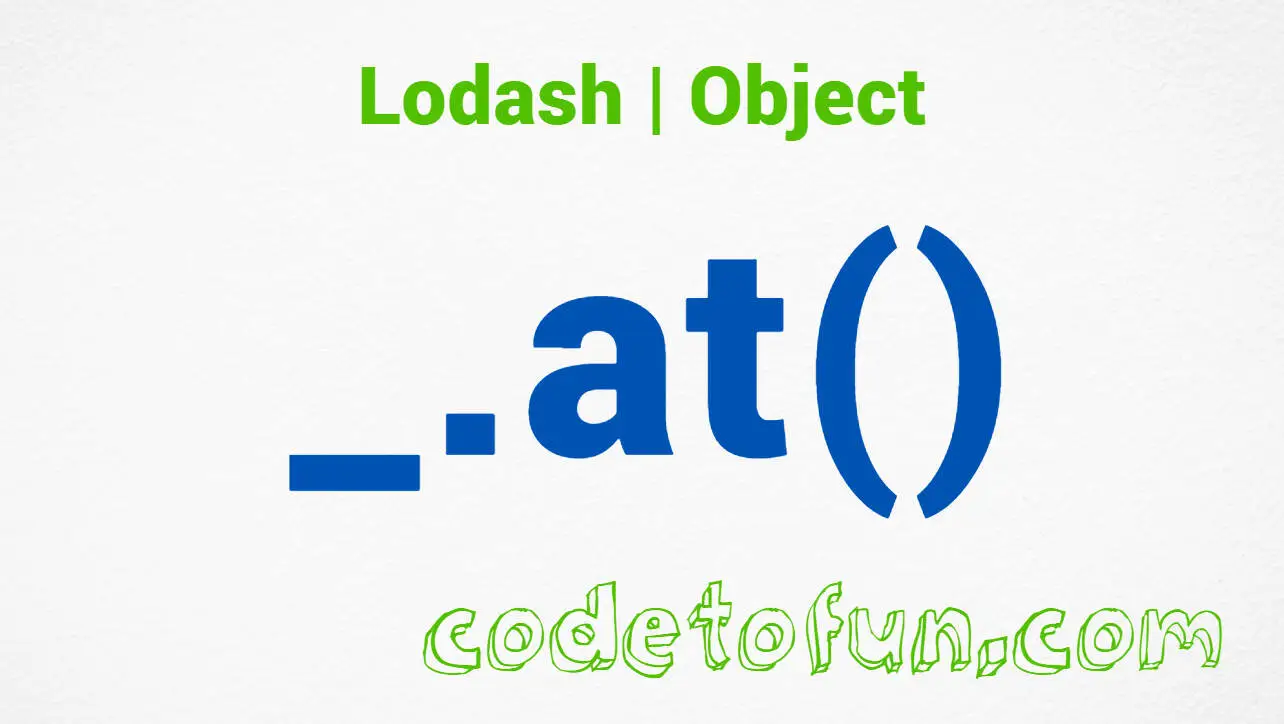
Photo Credit to CodeToFun
🙋 Introduction
When it comes to working with JavaScript objects, efficient extraction of specific values is a common requirement. Lodash, a powerful utility library, provides a solution to this with the _.at()
method.
This method allows developers to retrieve values from an object based on specified paths, streamlining the process of accessing nested properties and simplifying data extraction.
🧠 Understanding _.at() Method
The _.at()
method in Lodash is designed for selectively extracting values from an object based on provided paths. This can be particularly useful when dealing with complex nested structures, reducing the need for multiple statements to access desired properties.
💡 Syntax
The syntax for the _.at()
method is straightforward:
_.at(object, [paths])
- object: The object from which to extract values.
- paths: An array or multiple string arguments representing the paths to extract.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.at()
method:
const _ = require('lodash');
const user = {
id: 1,
name: 'John Doe',
address: {
city: 'New York',
zip: '10001',
},
email: 'john@example.com',
};
const selectedValues = _.at(user, ['name', 'address.city', 'email']);
console.log(selectedValues);
// Output: ['John Doe', 'New York', 'john@example.com']
In this example, the _.at()
method is used to extract values from the user object based on specified paths.
🏆 Best Practices
When working with the _.at()
method, consider the following best practices:
Check Object Existence:
Before using
_.at()
, ensure that the target object exists to prevent runtime errors. This is particularly important when working with dynamic data or user inputs.example.jsCopiedconst potentialObject = /* ...get an object from a dynamic source... */ ; if (potentialObject && typeof potentialObject === 'object') { const selectedValues = _.at(potentialObject, ['property1', 'property2']); console.log(selectedValues); } else { console.error('Invalid object'); }
Handle Non-existent Paths:
Account for non-existent paths to avoid unexpected results. Lodash gracefully handles undefined or non-existent paths, but it's good practice to validate paths, especially in dynamic scenarios.
example.jsCopiedconst selectedValues = _.at(user, ['name', 'address.country']); console.log(selectedValues); // Output: ['John Doe', undefined] (country path does not exist)
Utilize Multiple Paths:
Take advantage of the ability to specify multiple paths in a single call to
_.at()
. This can significantly reduce the number of statements needed to extract specific values from an object.example.jsCopiedconst selectedValues = _.at(user, ['name', 'address.city', 'email']); console.log(selectedValues); // Output: ['John Doe', 'New York', 'john@example.com']
📚 Use Cases
Form Data Extraction:
In scenarios involving form data,
_.at()
can be used to selectively extract values based on the form's structure, simplifying data handling.example.jsCopiedconst formData = { user: { firstName: 'Alice', lastName: 'Smith', address: { city: 'San Francisco', zip: '94105', }, email: 'alice@example.com', }, // ...other form fields... }; const userFields = _.at(formData, ['user.firstName', 'user.address.city', 'user.email']); console.log(userFields);
API Response Parsing:
When working with complex API responses,
_.at()
can streamline the process of extracting specific data fields, enhancing readability and reducing the need for verbose code.example.jsCopiedconst apiResponse = /* ...fetch data from API... */; const extractedData = _.at(apiResponse, ['data.user.name', 'data.user.email', 'data.posts']); console.log(extractedData);
Dynamic Data Navigation:
In applications with dynamic data structures,
_.at()
enables dynamic navigation through objects, allowing for flexible and concise code.example.jsCopiedconst dynamicPath = /* ...determine path dynamically... */; const dynamicValue = _.at(user, [dynamicPath]); console.log(dynamicValue);
🎉 Conclusion
The _.at()
method in Lodash is a valuable tool for selectively extracting values from objects based on specified paths. Whether you're dealing with nested data structures, form handling, or API responses, _.at()
provides a convenient and efficient way to access the exact values you need.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.at()
method in your Lodash projects.
👨💻 Join our Community:
Author
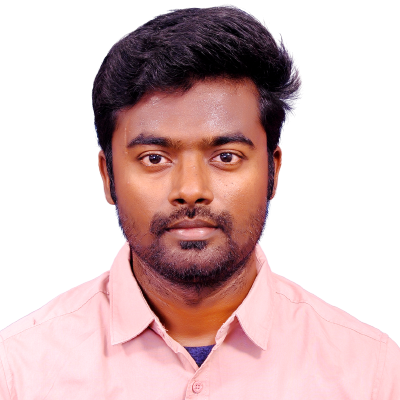
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
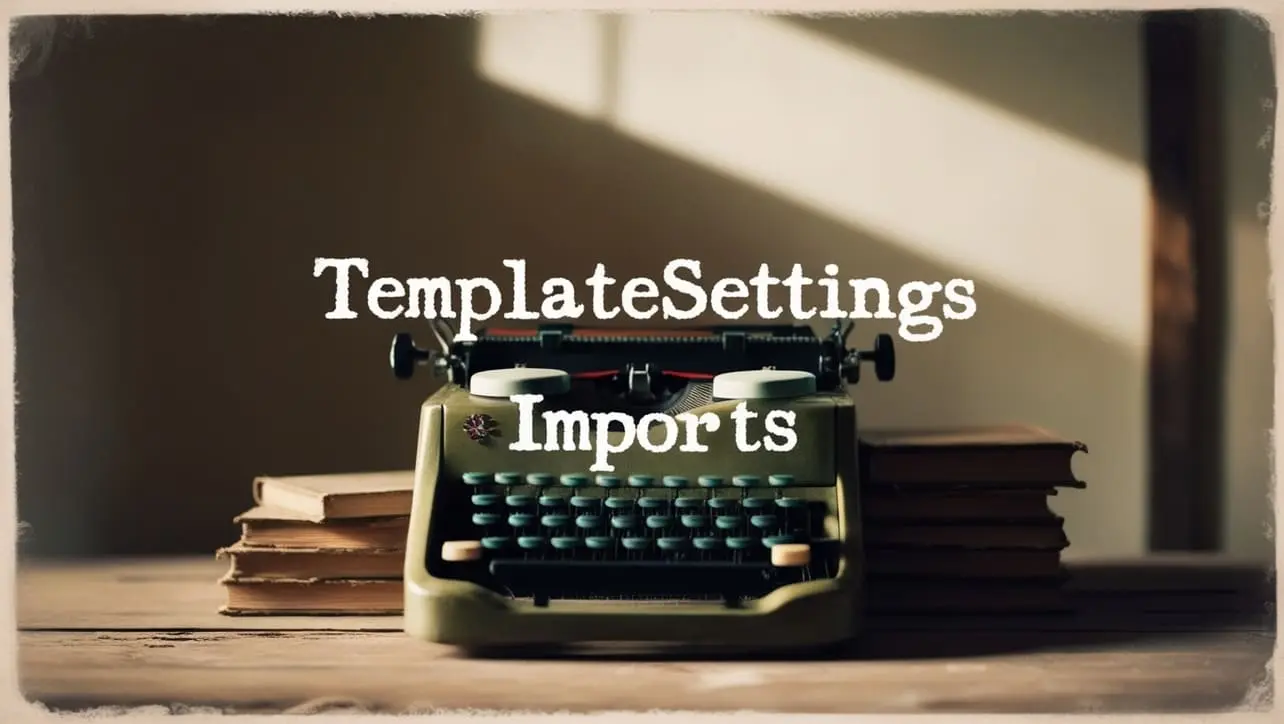
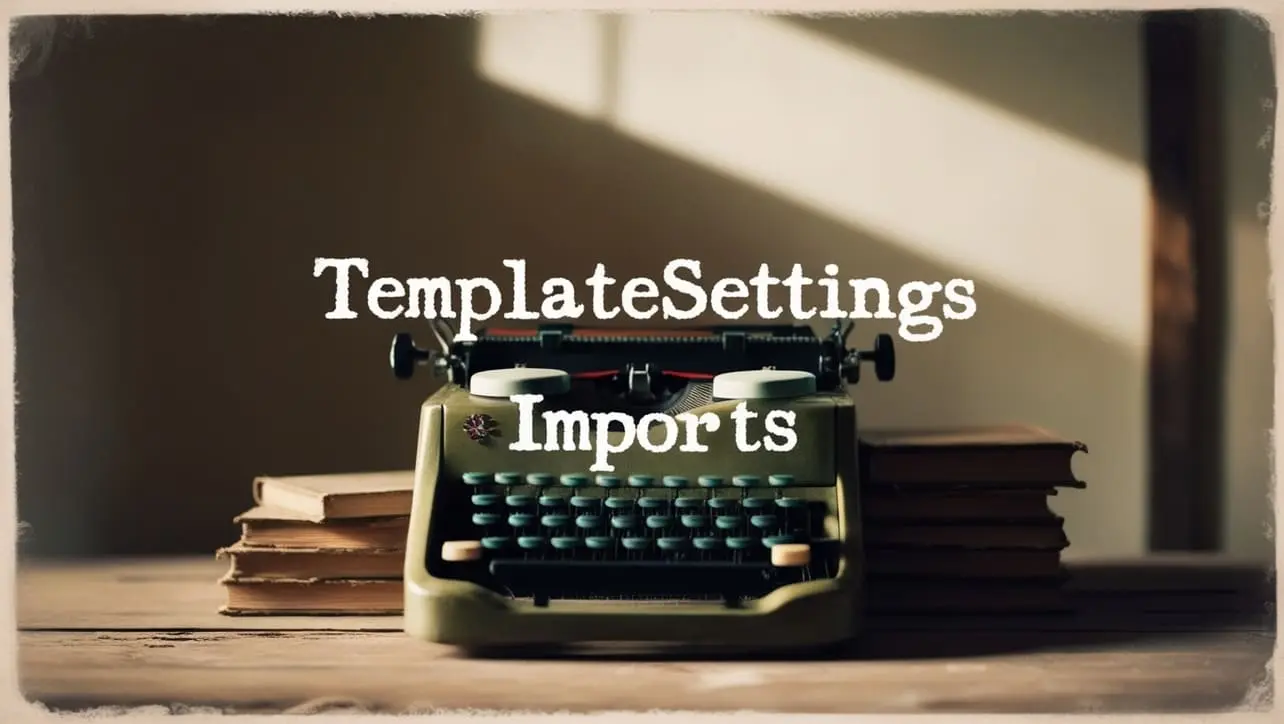
Lodash _.templateSettings.imports Property
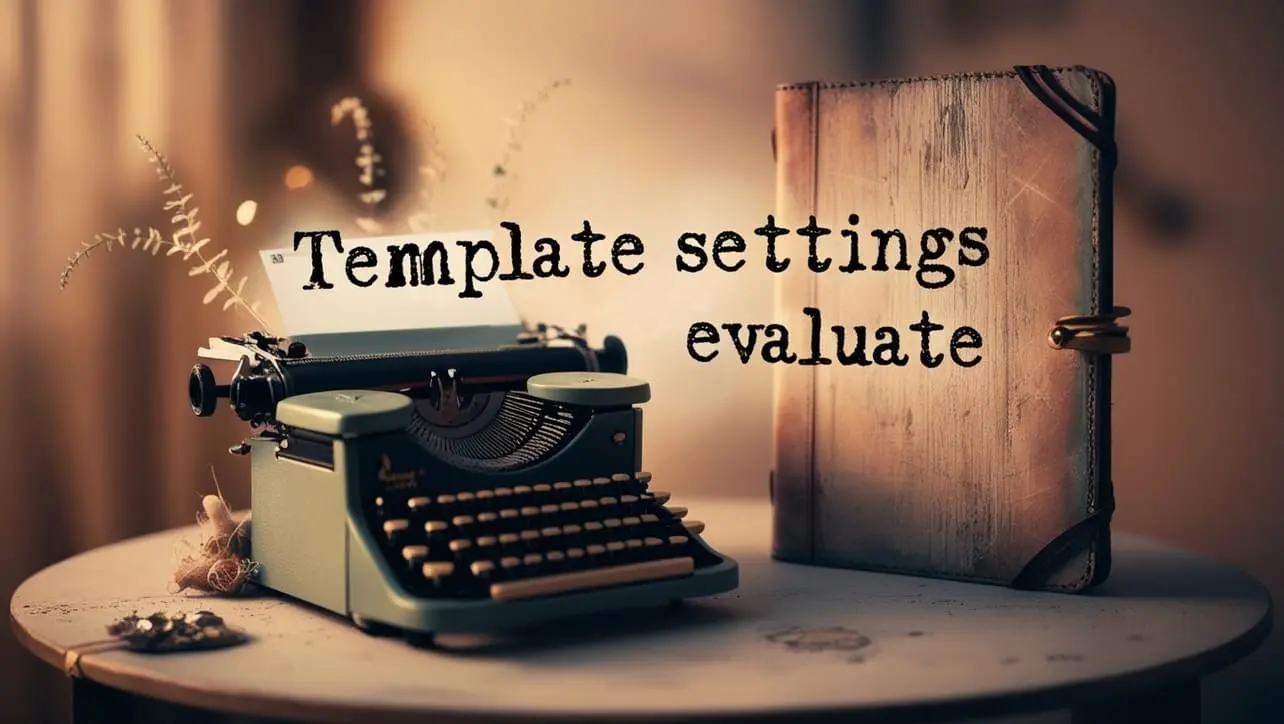
Lodash _.templateSettings.evaluate Property
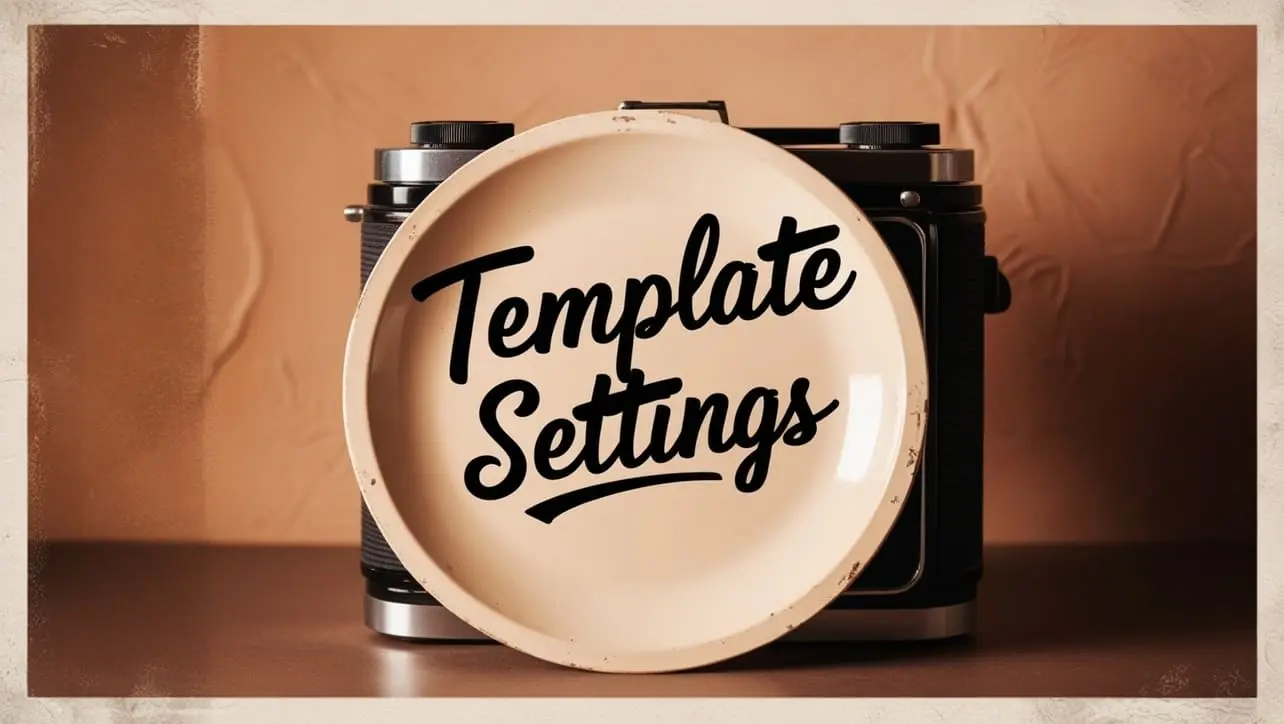
Lodash _.templateSettings Property
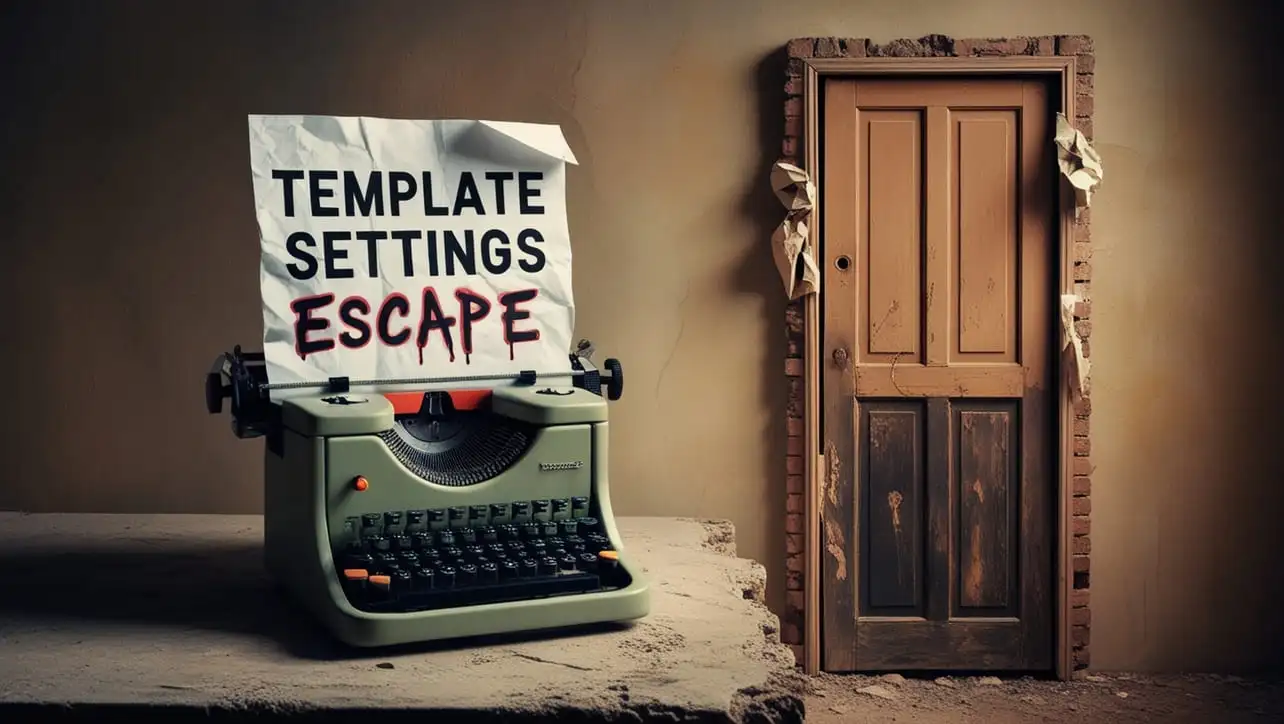
Lodash _.templateSettings.escape Property
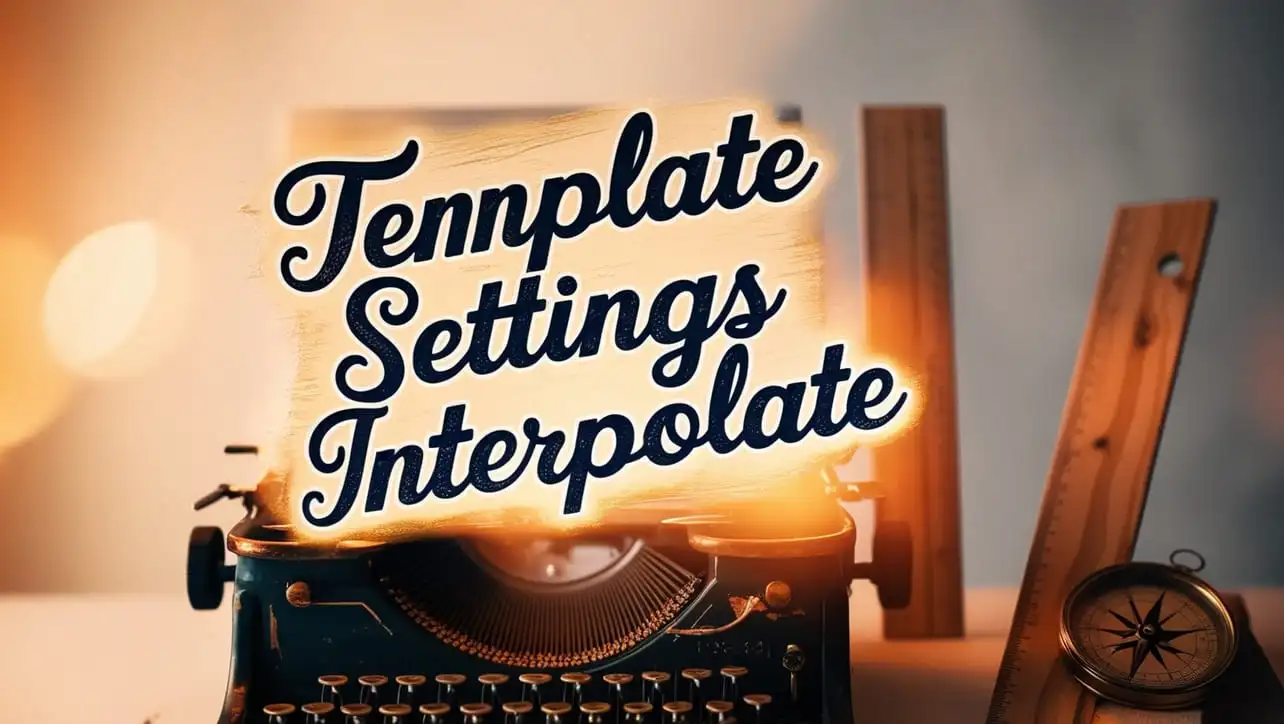
Lodash _.templateSettings.interpolate Property
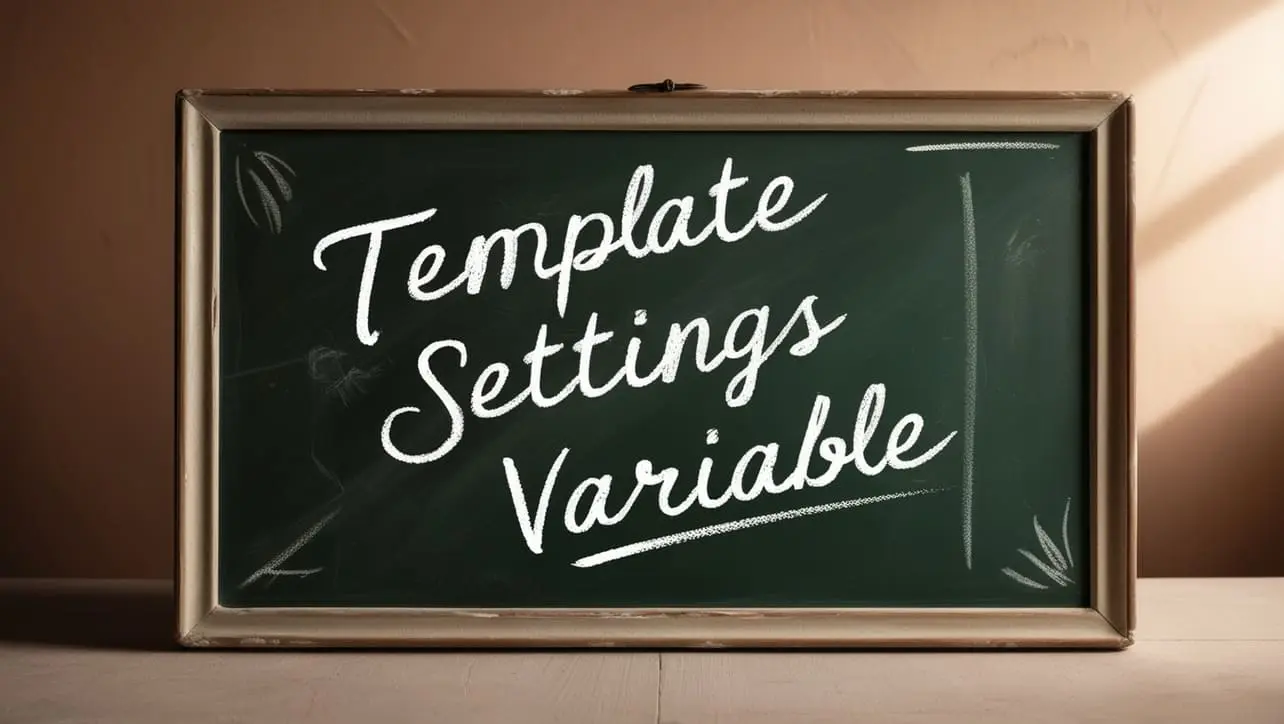
If you have any doubts regarding this article (Lodash _.at() Object Method), please comment here. I will help you immediately.