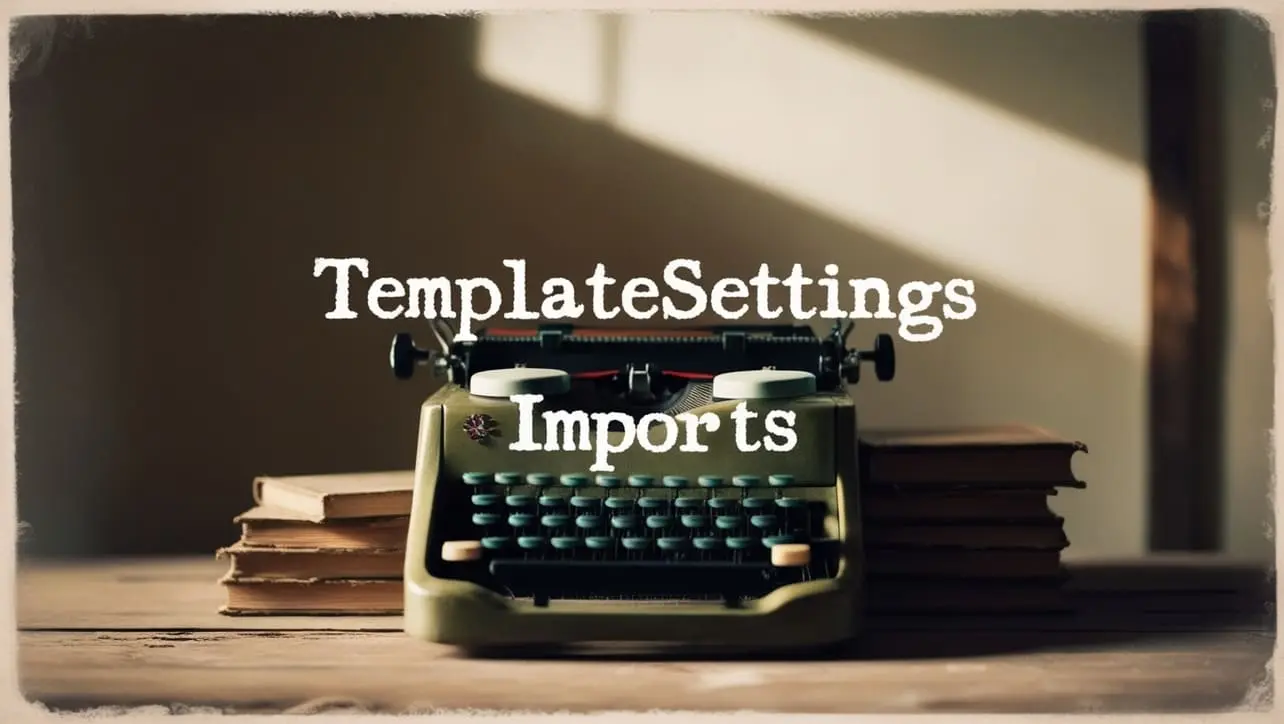
Lodash _.inRange() Number Method
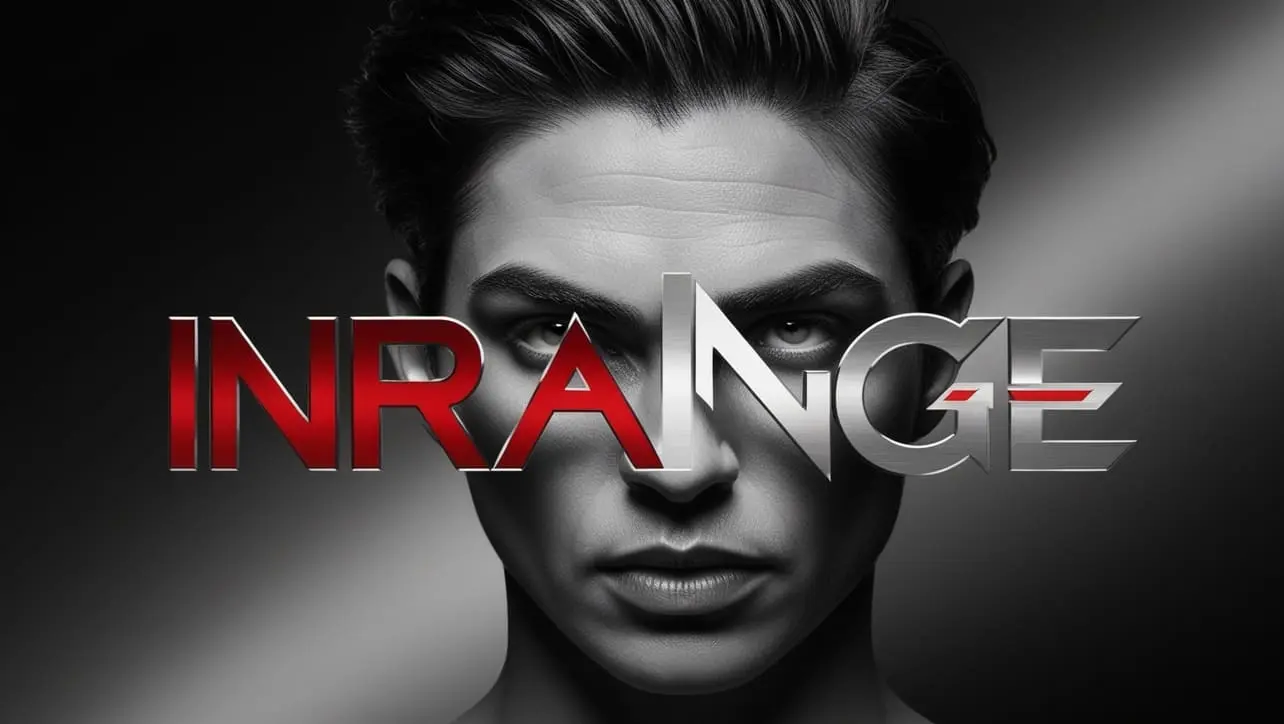
Photo Credit to CodeToFun
🙋 Introduction
When it comes to working with numeric values in JavaScript, precision and accuracy are key. The Lodash library, a powerhouse of utility functions, introduces the _.inRange()
method, designed to simplify the task of checking if a number is within a specified range.
Whether you're validating user inputs or implementing conditional logic, this method proves to be an invaluable tool for developers dealing with numeric ranges.
🧠 Understanding _.inRange() Method
The _.inRange()
method in Lodash allows you to determine if a given number falls within a specified range. This is particularly useful for scenarios where you need to validate input values or execute different code paths based on numeric conditions.
💡 Syntax
The syntax for the _.inRange()
method is straightforward:
_.inRange(number, [start=0], end)
- number: The number to check.
- start (Optional): The start of the range.
- end: The end of the range.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.inRange()
method:
const _ = require('lodash');
const checkNumber = 5;
const isInRange = _.inRange(checkNumber, 1, 10);
console.log(isInRange);
// Output: true
In this example, the checkNumber is evaluated using _.inRange()
to determine if it falls within the range of 1 (inclusive) to 10 (exclusive).
🏆 Best Practices
When working with the _.inRange()
method, consider the following best practices:
Specify Range Boundaries:
Always explicitly specify both the start and end boundaries of the range to avoid unexpected results. The
_.inRange()
method checks if the provided number is within the half-open interval [start, end).example.jsCopiedconst inputNumber = 15; const startRange = 10; const endRange = 20; const isWithinRange = _.inRange(inputNumber, startRange, endRange); console.log(isWithinRange); // Output: false
Handle Single-Argument Usage:
Be aware that if only one argument is provided, it is considered as the end value, and the start value defaults to 0. It's good practice to use the two-argument syntax for clarity.
example.jsCopiedconst singleArgumentCheck = _.inRange(5); console.log(singleArgumentCheck); // Output: true
Use in Conditional Statements:
Leverage
_.inRange()
in conditional statements for concise and readable code when checking numeric conditions.example.jsCopiedconst userInput = /* ...get user input... */; if (_.inRange(userInput, 1, 100)) { console.log('Input is within the valid range.'); } else { console.log('Input is outside the valid range.'); }
📚 Use Cases
User Input Validation:
Validate user input to ensure it falls within an acceptable numeric range. This is particularly useful in scenarios like form submissions.
example.jsCopiedconst userInput = /* ...get user input... */ ; const isValidInput = _.inRange(userInput, 0, 100); if (isValidInput) { // Proceed with further processing console.log('Input is valid.'); } else { console.log('Invalid input. Please enter a number between 0 and 100.'); }
Conditional Rendering:
Determine whether a numeric value is within a specific range to conditionally render elements in a user interface.
example.jsCopiedconst numericValue = /* ...get numeric value... */; if (_.inRange(numericValue, 10, 20)) { // Render special content for the specified range console.log('Rendering special content.'); } else { console.log('No special content to render.'); }
Game Development:
In game development, use
_.inRange()
to check if a player's score or position is within certain thresholds to trigger specific in-game events.example.jsCopiedconst playerScore = /* ...get player score... */; if (_.inRange(playerScore, 1000, 2000)) { console.log('Player achieved a high score!'); } else { console.log('Player score is below the threshold.'); }
🎉 Conclusion
The _.inRange()
method in Lodash simplifies numeric range validation, providing a straightforward solution for checking if a number falls within a specified interval. Whether you're validating user inputs, implementing conditional logic, or working on game development, this method proves to be a versatile and efficient tool for numeric comparisons in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.inRange()
method in your Lodash projects.
👨💻 Join our Community:
Author
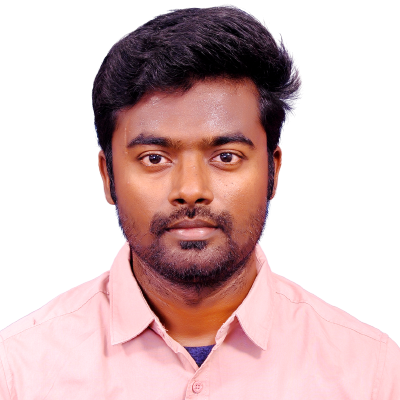
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
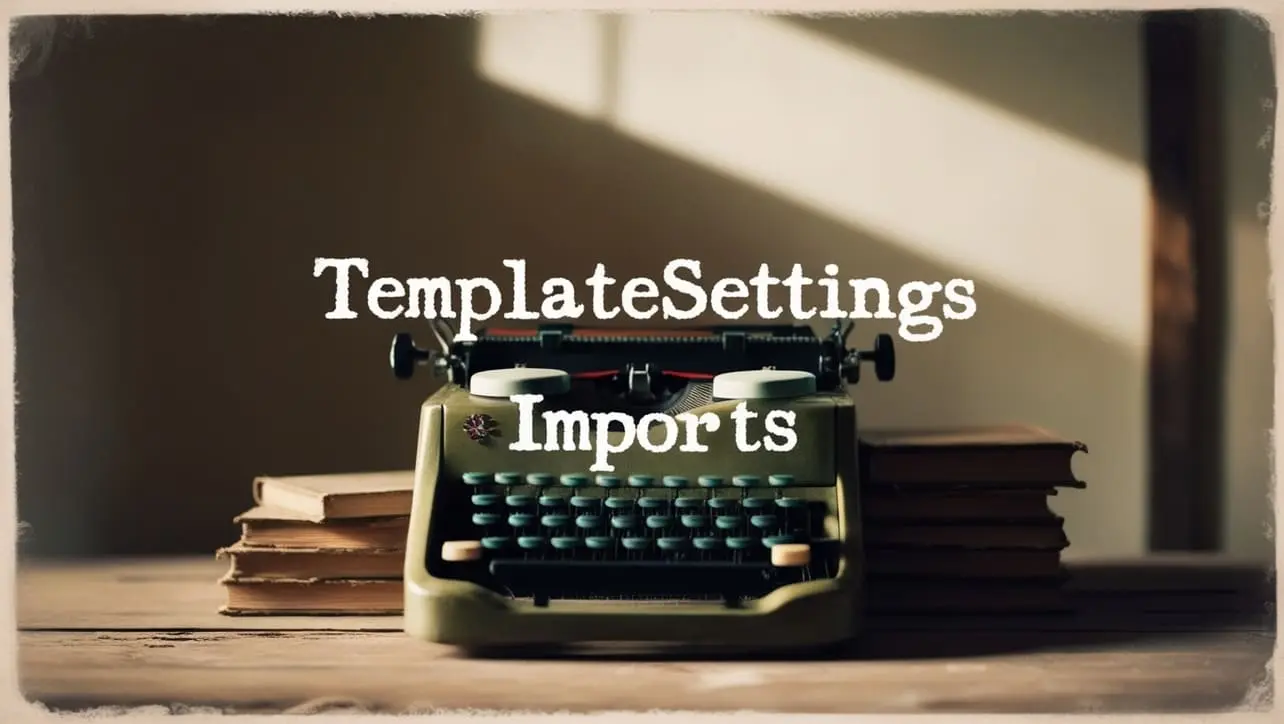
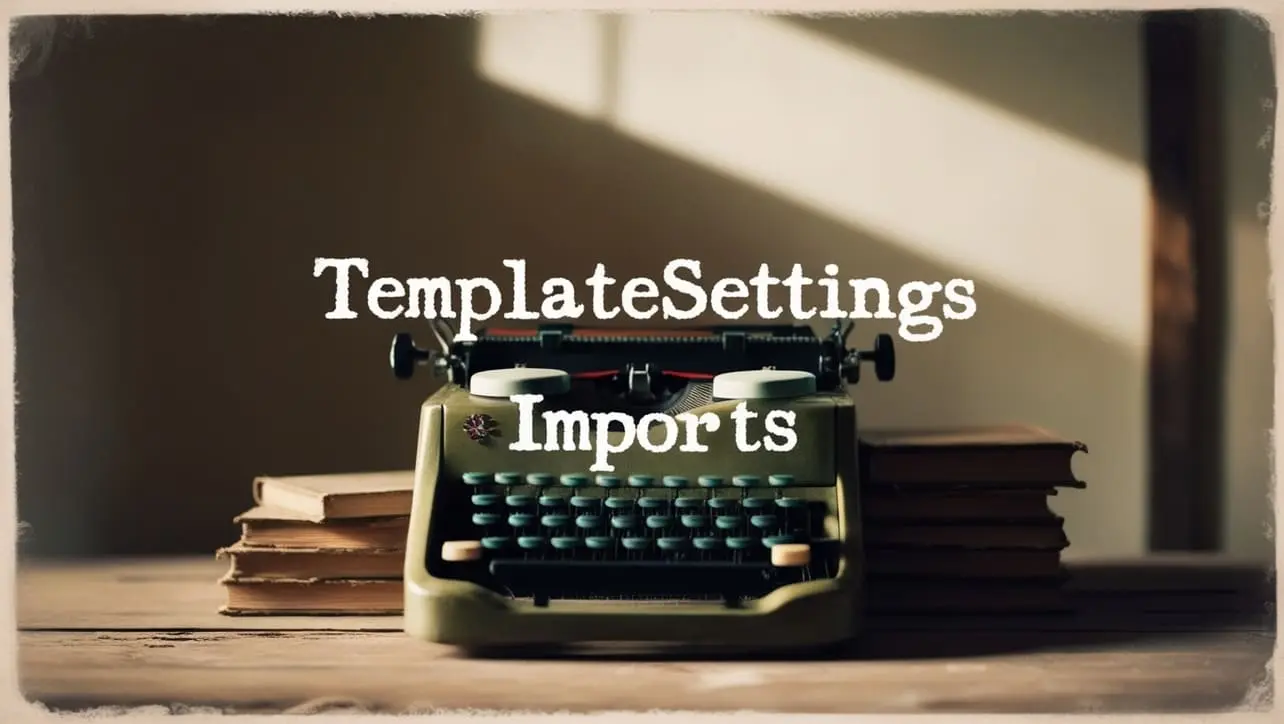
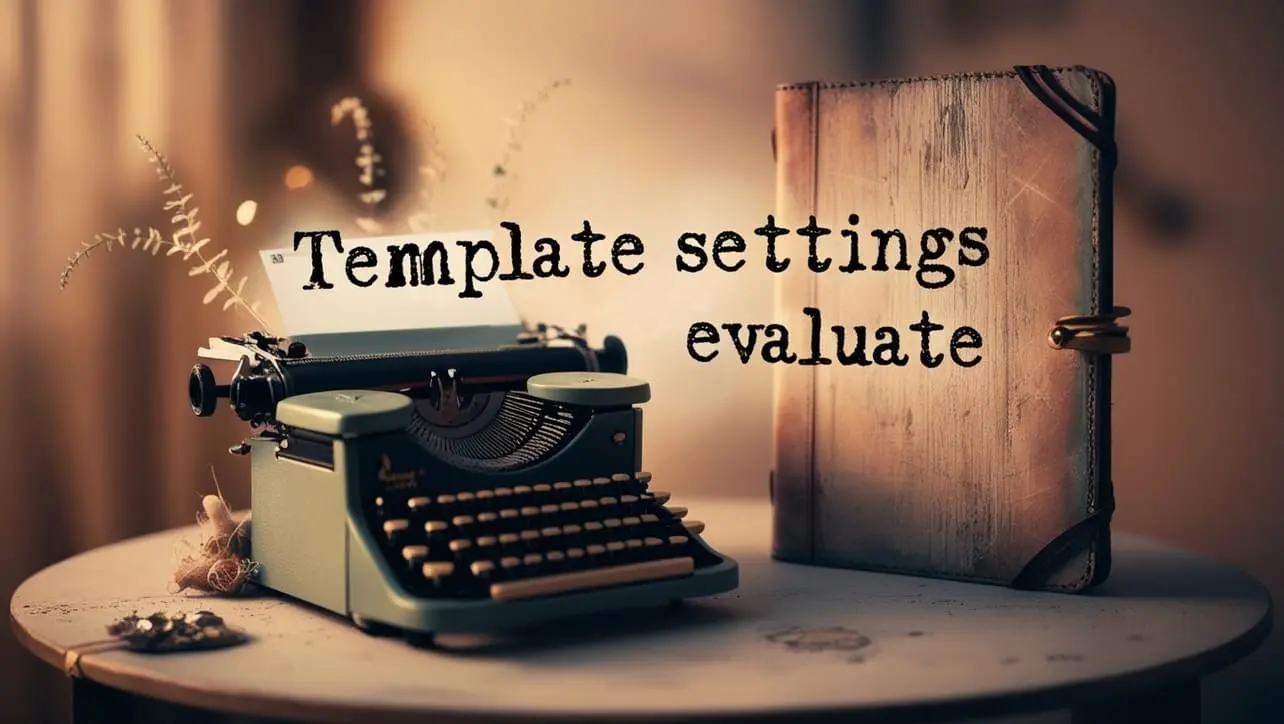
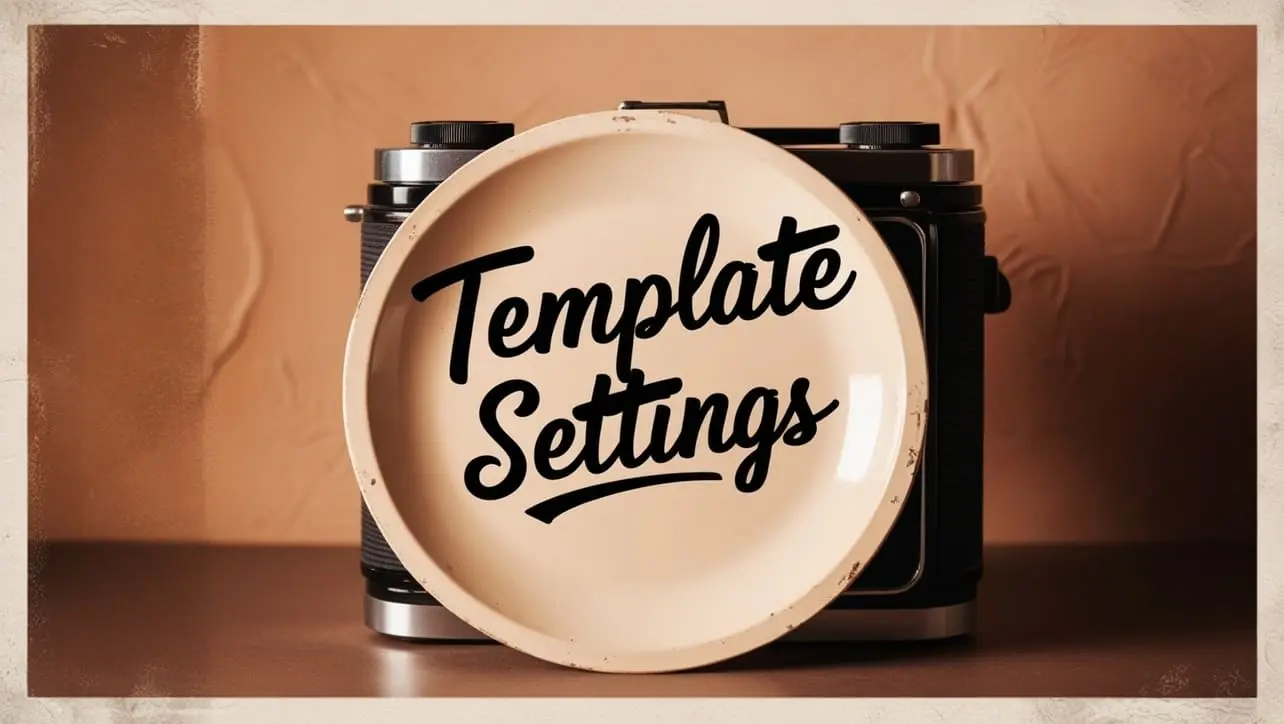
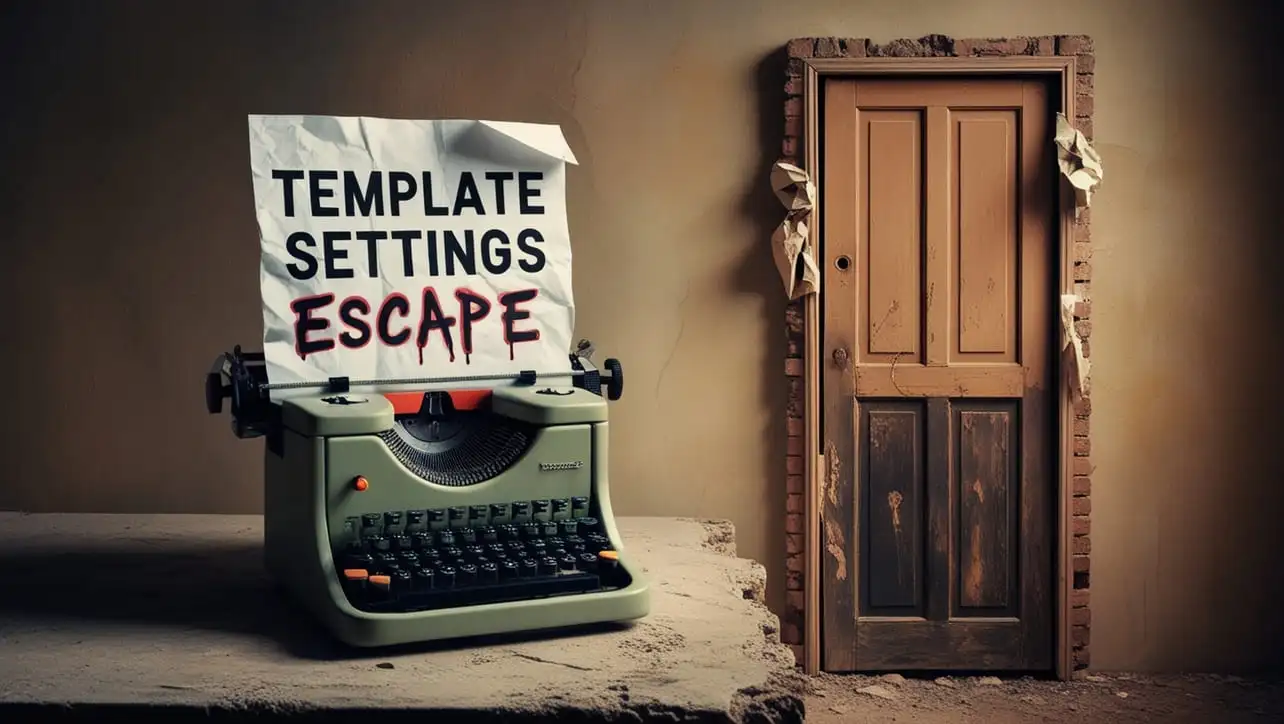
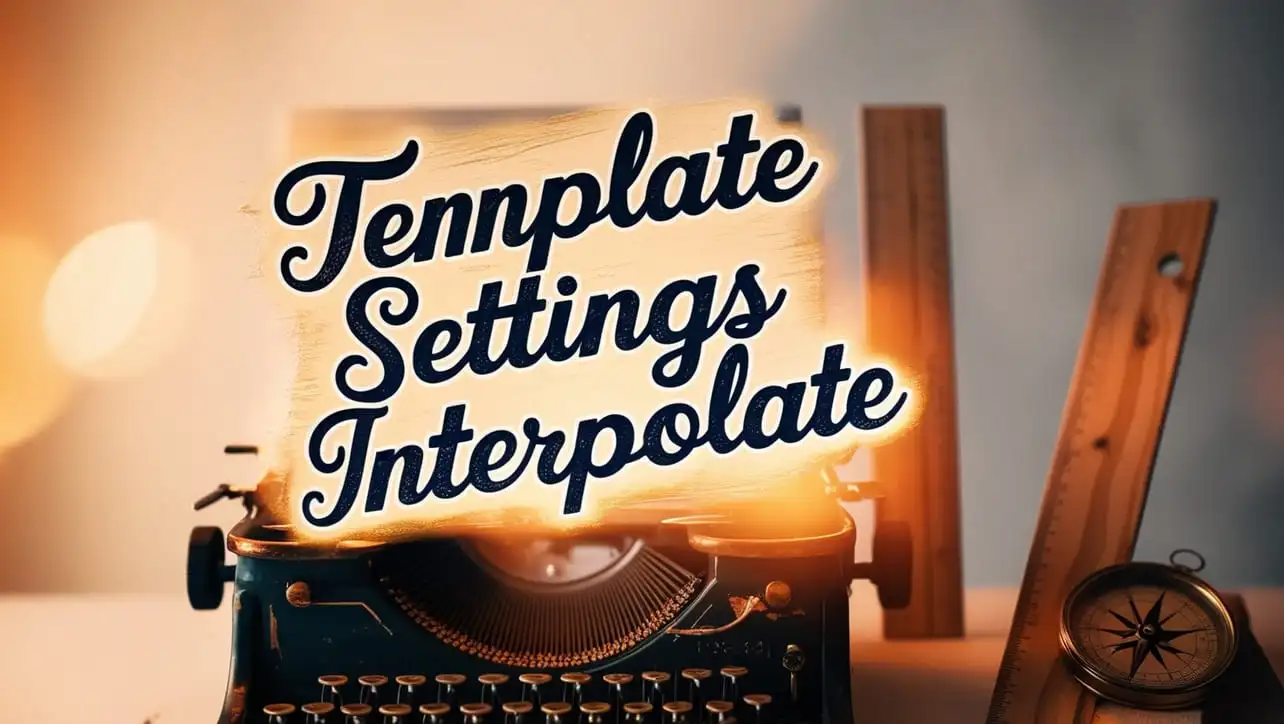
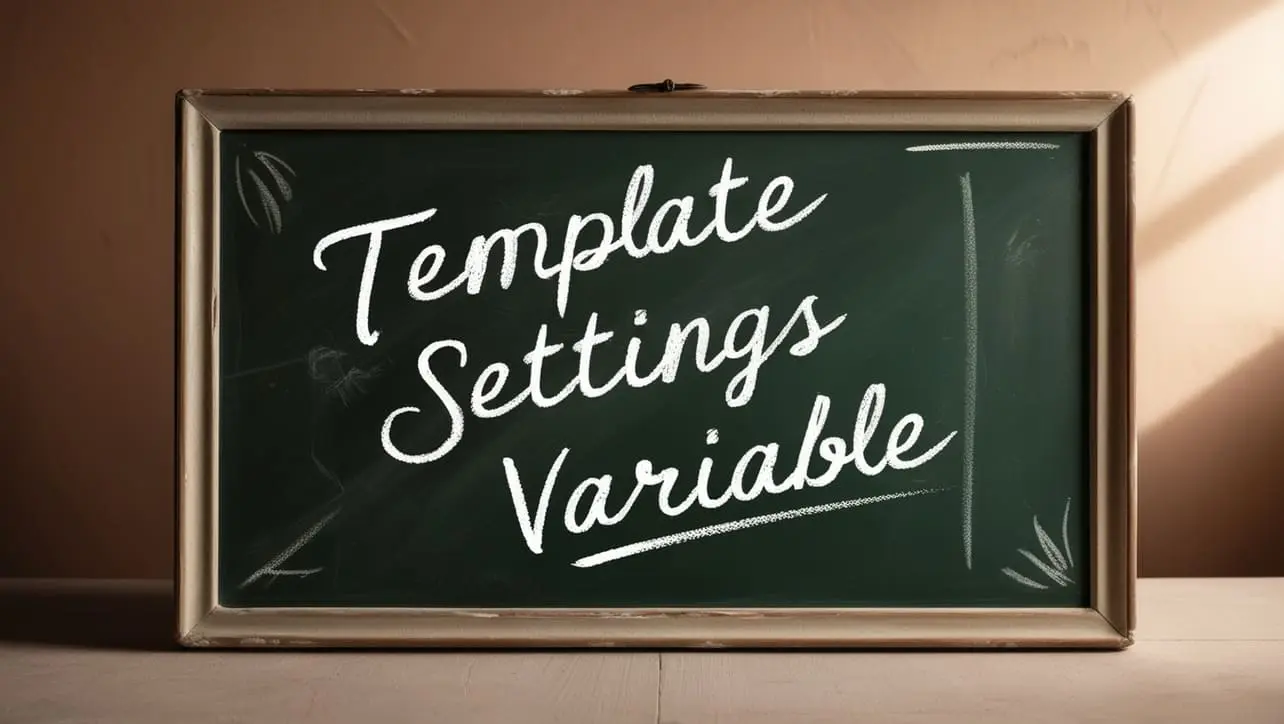
If you have any doubts regarding this article (Lodash _.inRange() Number Method), please comment here. I will help you immediately.