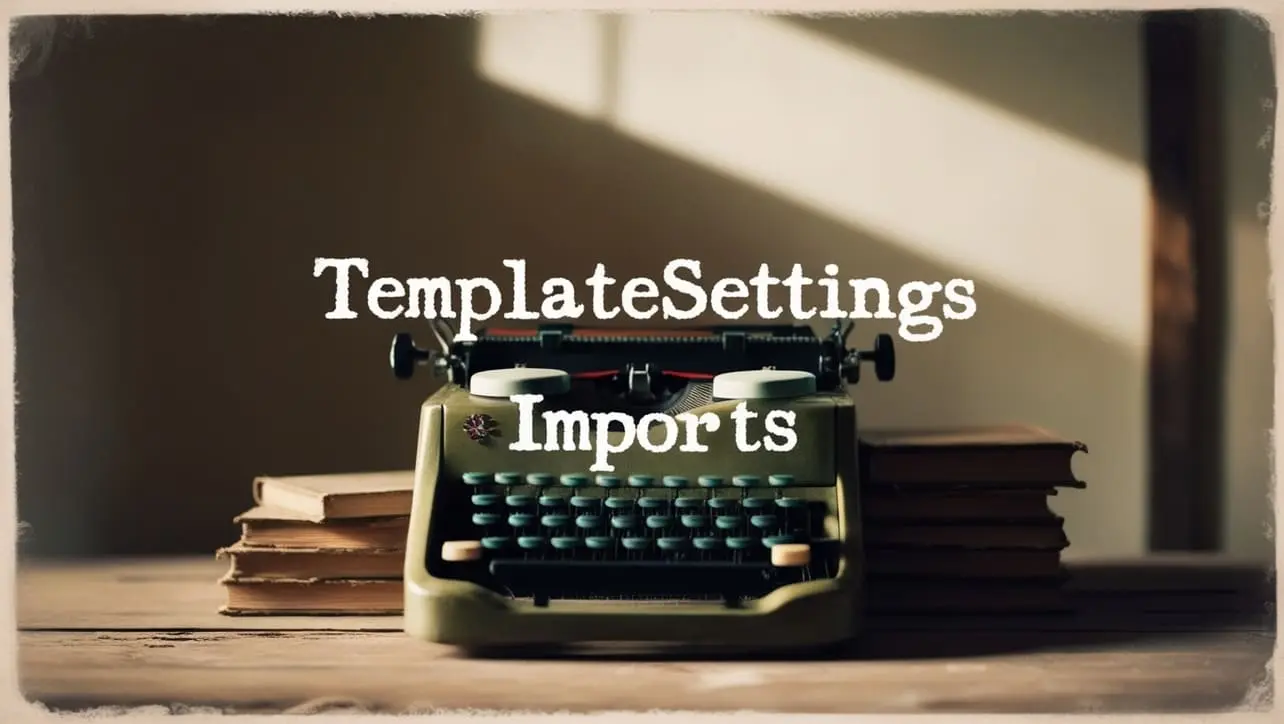
Lodash _.subtract() Math Method
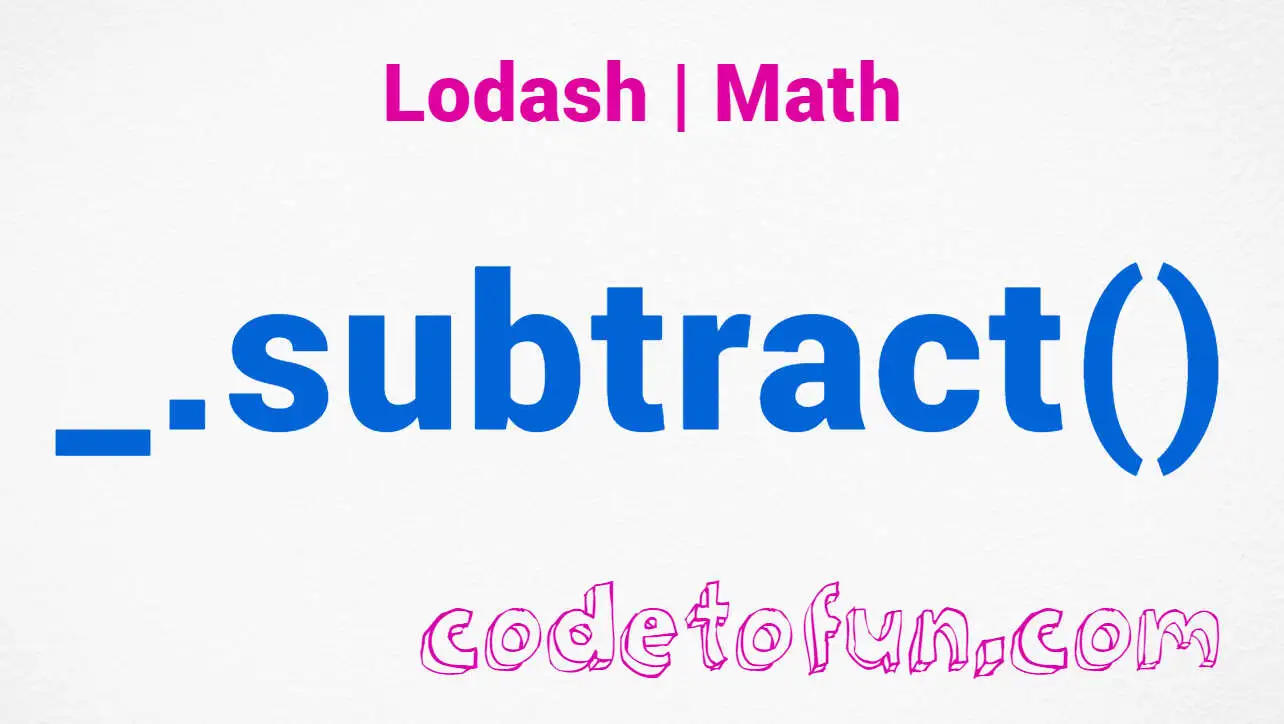
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript programming, mathematical operations are fundamental, and the Lodash library provides a set of utilities to simplify these tasks. Among them is the _.subtract()
method, a powerful tool for performing subtraction with precision and flexibility.
Whether you're dealing with numeric calculations or need to manipulate data, this method proves to be a valuable asset in your JavaScript toolkit.
🧠 Understanding _.subtract() Method
The _.subtract()
method in Lodash is designed for subtracting two numbers with precision, addressing common challenges associated with JavaScript's native arithmetic operations. This method is part of Lodash's efforts to provide reliable and accurate mathematical functions for developers.
💡 Syntax
The syntax for the _.subtract()
method is straightforward:
_.subtract(minuend, subtrahend)
- minuend: The number from which the subtrahend will be subtracted.
- subtrahend: The number to be subtracted from the minuend.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.subtract()
method:
const _ = require('lodash');
const result = _.subtract(10, 5);
console.log(result);
// Output: 5
In this example, _.subtract()
subtracts 5 from 10, yielding the result of 5.
🏆 Best Practices
When working with the _.subtract()
method, consider the following best practices:
Handling NaN Values:
Be aware that if either the minuend or subtrahend is NaN (Not a Number), the result will be NaN. Consider implementing validation checks to handle such scenarios.
example.jsCopiedconst invalidResult = _.subtract(10, 'abc'); console.log(isNaN(invalidResult)); // Output: true
Precision in Decimal Numbers:
When dealing with decimal numbers, be cautious of precision issues inherent in JavaScript. Consider using a library like Decimal.js for more precise calculations if needed.
example.jsCopiedconst decimalResult = _.subtract(0.1 + 0.2, 0.3); console.log(decimalResult); // Output: 5.551115123125783e-17 (due to JavaScript floating-point precision)
Handling Large Numbers:
JavaScript has limitations when dealing with large numbers. Be aware of potential overflow issues and consider using libraries like BigInt or BigNumber for handling extremely large numbers.
example.jsCopiedconst largeResult = _.subtract(Number.MAX_SAFE_INTEGER, 1); console.log(largeResult); // Output: 9007199254740990 (correct result due to safe integer handling)
📚 Use Cases
Basic Arithmetic Operations:
_.subtract()
is ideal for basic subtraction operations, providing a consistent and reliable alternative to native JavaScript subtraction.example.jsCopiedconst basicResult = _.subtract(15, 7); console.log(basicResult); // Output: 8
Data Manipulation:
When working with datasets and needing to perform subtraction on numeric values,
_.subtract()
simplifies the process, ensuring accurate calculations.example.jsCopiedconst data = [10, 20, 30, 40]; const subtractedData = data.map(value => _.subtract(value, 5)); console.log(subtractedData); // Output: [5, 15, 25, 35]
Validation and Error Handling:
Utilize
_.subtract()
in scenarios where validation and error handling are critical, ensuring that your application gracefully handles unexpected input.example.jsCopiedfunction safeSubtraction(a, b) { if (typeof a !== 'number' || typeof b !== 'number') { return 'Invalid input'; } return _.subtract(a, b); } console.log(safeSubtraction(12, 'abc')); // Output: 'Invalid input'
🎉 Conclusion
The _.subtract()
method in Lodash is a reliable solution for performing subtraction in JavaScript, addressing common challenges associated with numeric calculations. Whether you're dealing with basic arithmetic or complex data manipulation, incorporating _.subtract()
into your code ensures precision and consistency.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.subtract()
method in your Lodash projects.
👨💻 Join our Community:
Author
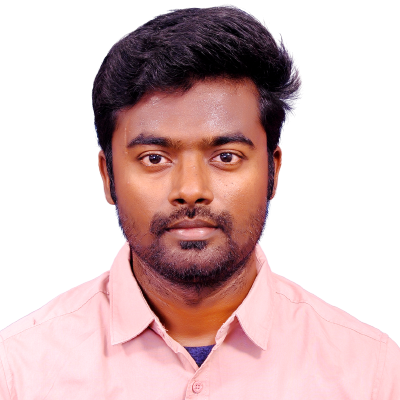
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
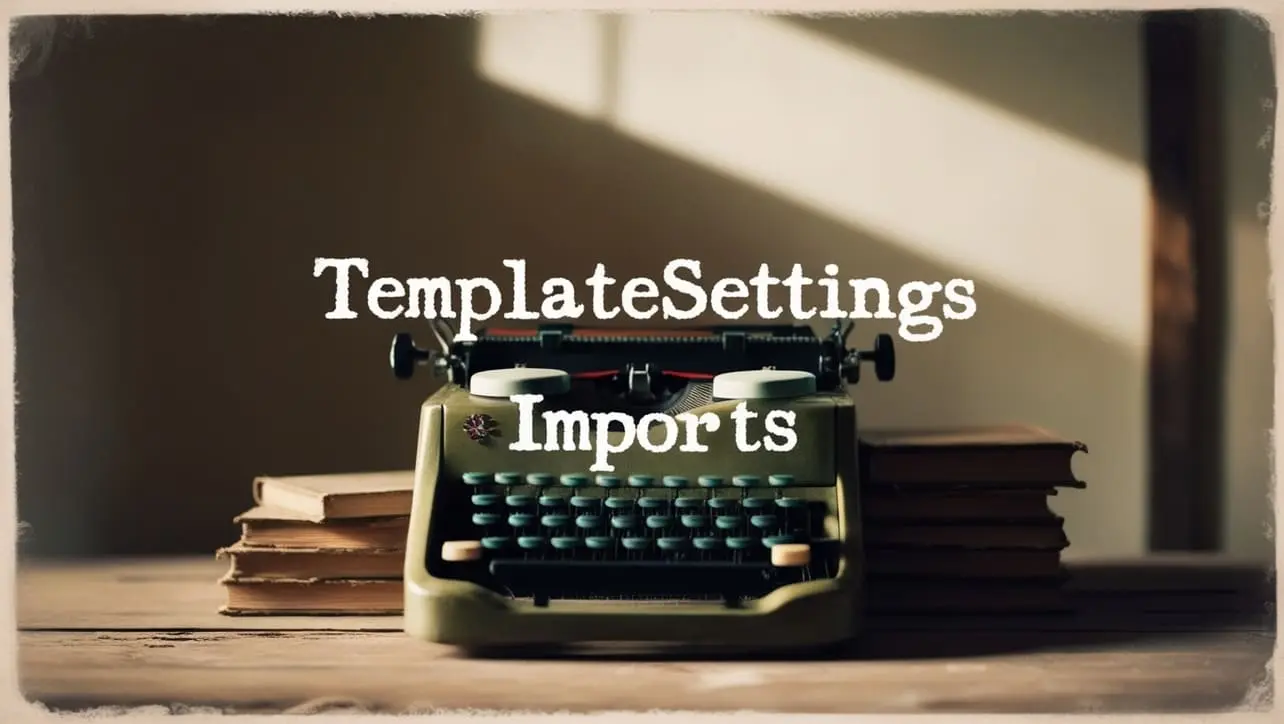
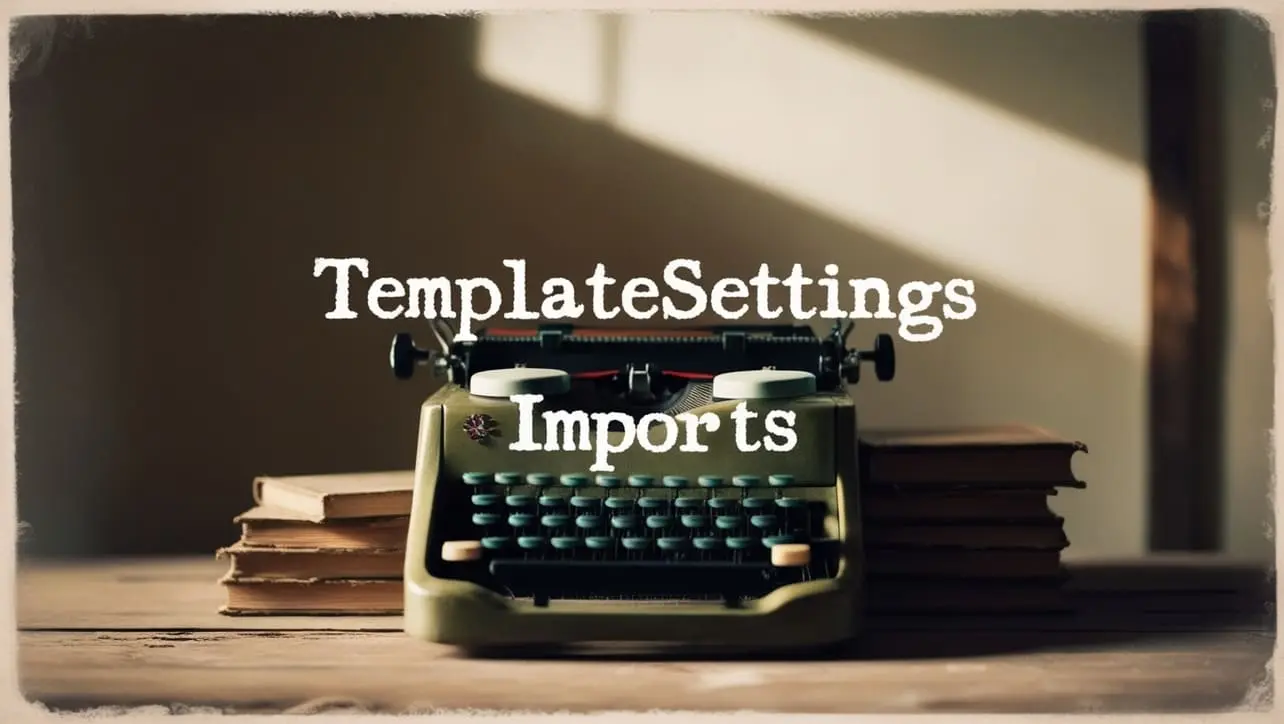
Lodash _.templateSettings.imports Property
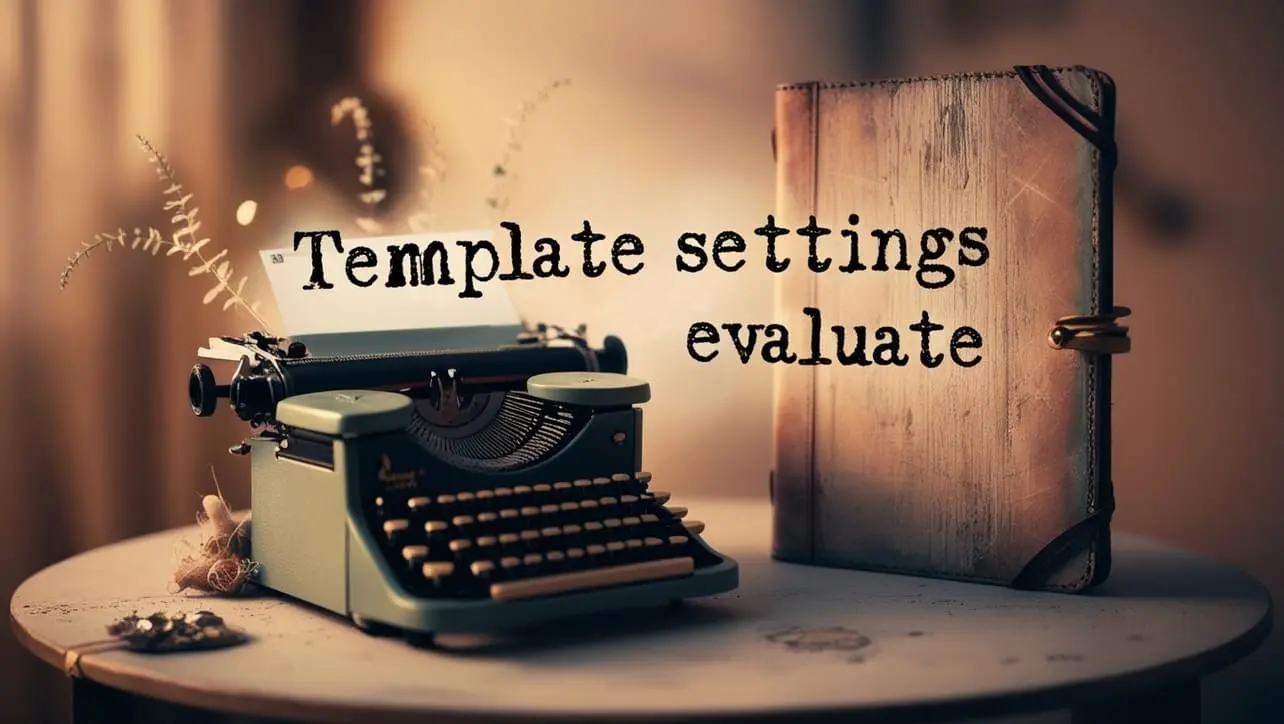
Lodash _.templateSettings.evaluate Property
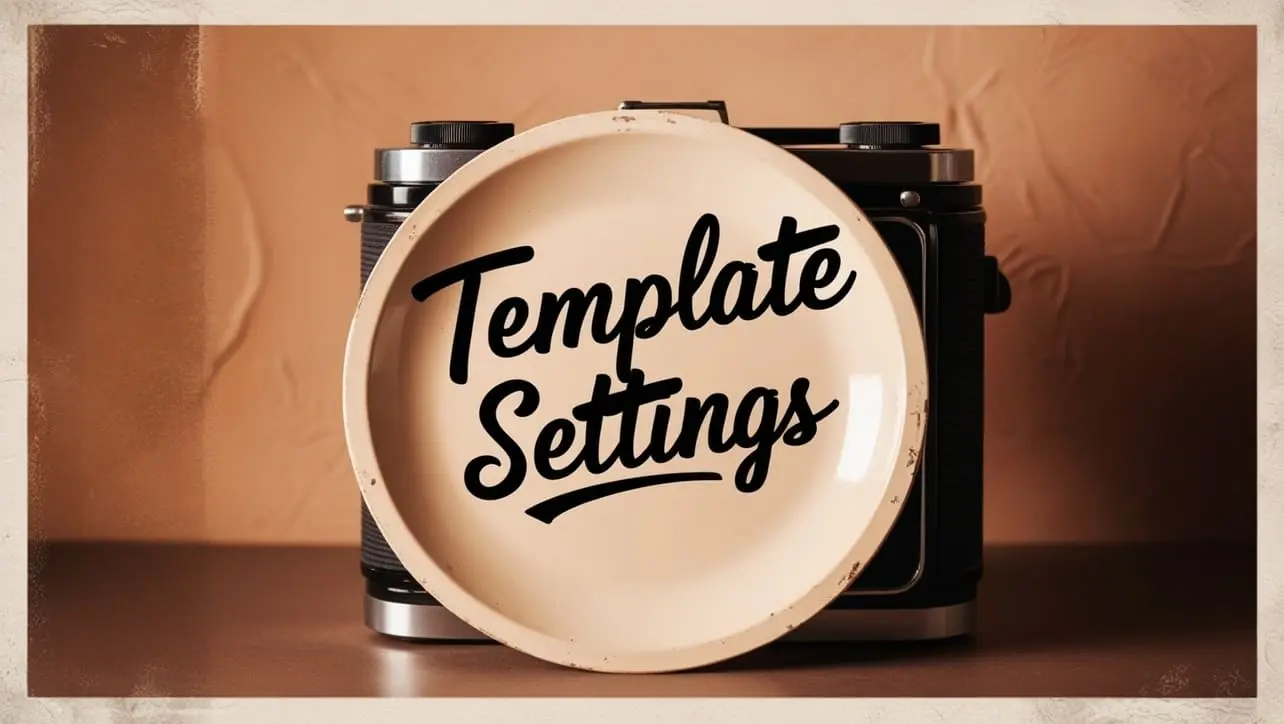
Lodash _.templateSettings Property
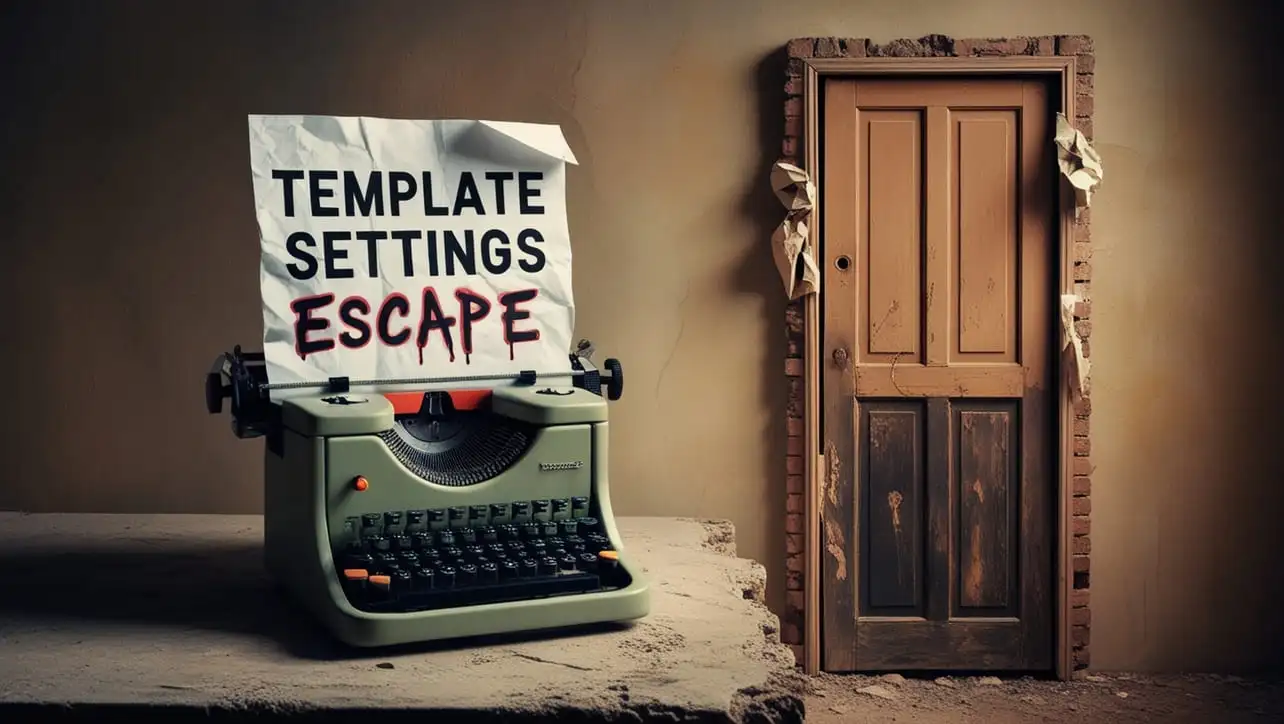
Lodash _.templateSettings.escape Property
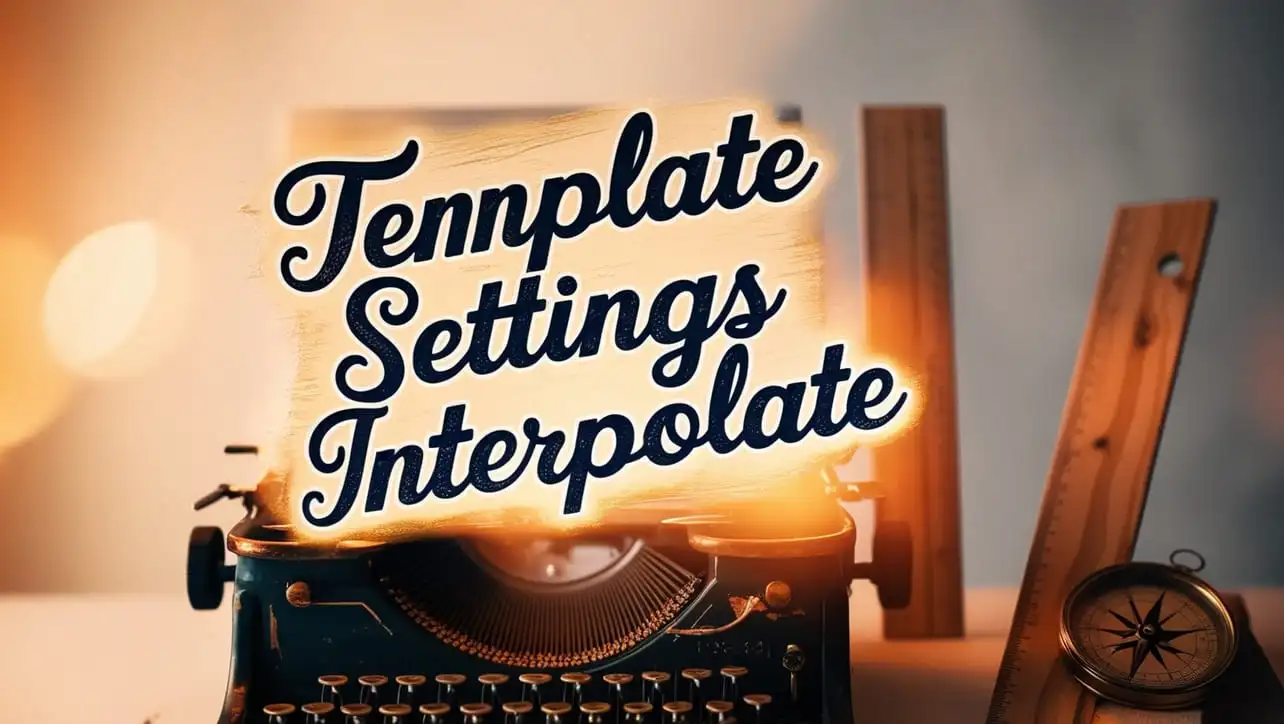
Lodash _.templateSettings.interpolate Property
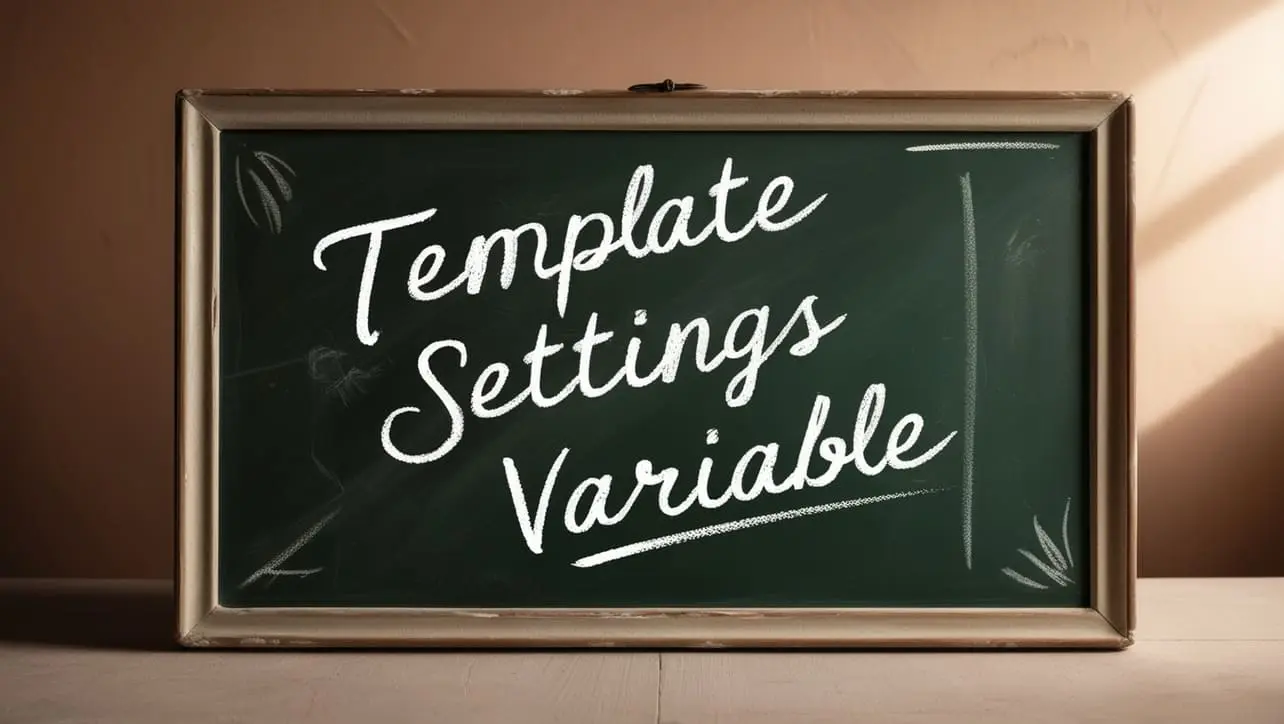
If you have any doubts regarding this article (Lodash _.subtract() Math Method), please comment here. I will help you immediately.