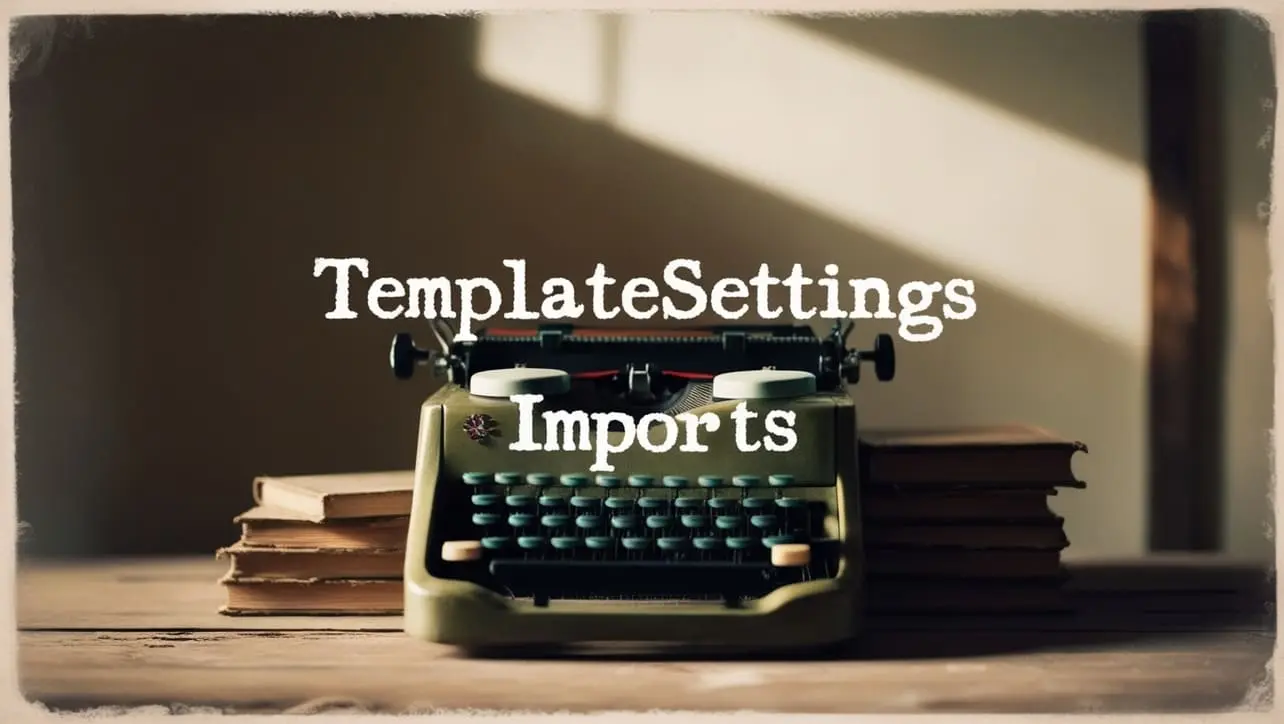
Lodash _.max() Math Method
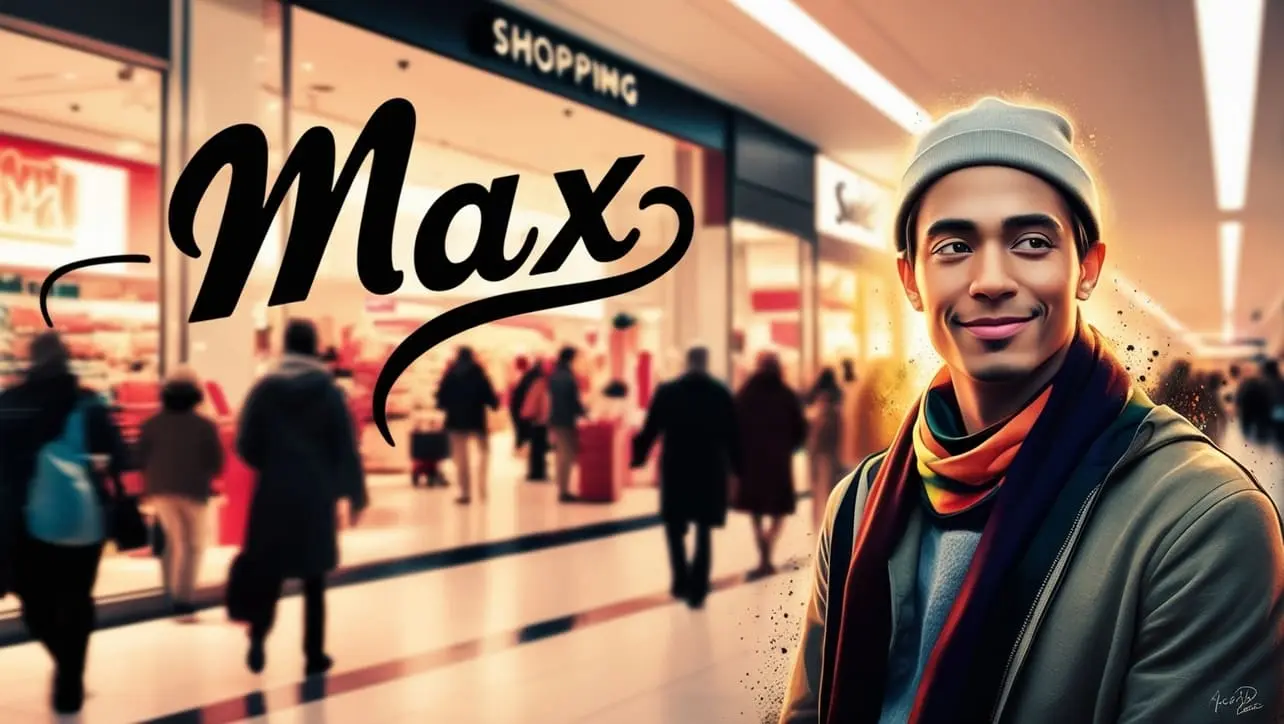
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript programming, efficient data analysis often involves finding the maximum value within an array. Lodash, a powerful utility library, provides the _.max()
method, a convenient tool for determining the maximum value in an array.
This method simplifies numeric computations and enhances the readability of your code, making it an invaluable asset for developers dealing with numeric datasets.
🧠 Understanding _.max() Method
The _.max()
method in Lodash is designed to find the maximum value within an array, whether it's an array of numbers, objects, or other comparable elements. This method simplifies the process of identifying the largest element, offering flexibility and ease of use.
💡 Syntax
The syntax for the _.max()
method is straightforward:
_.max(collection, [iteratee])
- collection: The collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.max()
method:
const _ = require('lodash');
const numericArray = [5, 12, 8, 20, 16, 10];
const maximumValue = _.max(numericArray);
console.log(maximumValue);
// Output: 20
In this example, the numericArray is processed by _.max()
, resulting in the identification of the maximum value, which is 20.
🏆 Best Practices
When working with the _.max()
method, consider the following best practices:
Numeric Arrays:
Ensure that the input array is composed of numeric values when using
_.max()
. While the method can handle other types of comparable elements, it is optimized for numeric computations.example.jsCopiedconst mixedArray = [15, '25', 10, 30, 'abc', 12]; const maxNumericValue = _.max(mixedArray); console.log(maxNumericValue); // Output: 30
Handling Objects:
When working with an array of objects, provide an iteratee function to specify the property for comparison. This allows you to find the maximum value based on a particular property of the objects.
example.jsCopiedconst objectArray = [ { id: 1, value: 15 }, { id: 2, value: 25 }, { id: 3, value: 10 }, ]; const maxObjectValue = _.max(objectArray, obj => obj.value); console.log(maxObjectValue); // Output: { id: 2, value: 25 }
Empty Arrays:
Handle scenarios where the input array might be empty. Provide appropriate default values or implement error handling to address such cases.
example.jsCopiedconst emptyArray = []; const defaultValue = 0; const maxEmptyArrayValue = _.max(emptyArray) || defaultValue; console.log(maxEmptyArrayValue); // Output: 0
📚 Use Cases
Data Analysis:
_.max()
is particularly useful when performing data analysis tasks, such as finding the maximum value in a dataset. This can be beneficial for generating insights or making informed decisions based on numeric data.example.jsCopiedconst salesData = /* ...fetch sales data from a database or API... */; const maxSalesValue = _.max(salesData); console.log(maxSalesValue);
User Ratings:
In scenarios involving user ratings,
_.max()
can help identify the highest-rated item or user based on their numeric ratings.example.jsCopiedconst userRatings = [4.5, 3.2, 5, 4.8, 3.9]; const highestRating = _.max(userRatings); console.log(highestRating); // Output: 5
Dynamic Scaling:
When working with visualizations or dynamic scaling,
_.max()
can be utilized to determine the maximum value for proper scaling.example.jsCopiedconst dataForScaling = /* ...fetch dynamic data... */; const maximumScaleValue = _.max(dataForScaling); console.log(maximumScaleValue);
🎉 Conclusion
The _.max()
method in Lodash offers a straightforward and efficient way to find the maximum value within an array. Whether you're dealing with numeric datasets, object properties, or other comparable elements, this method provides a versatile solution for identifying the largest element in your data.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.max()
method in your Lodash projects.
👨💻 Join our Community:
Author
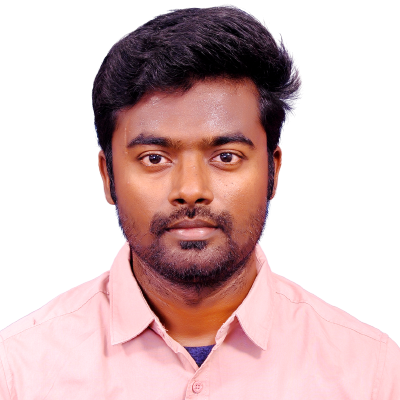
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
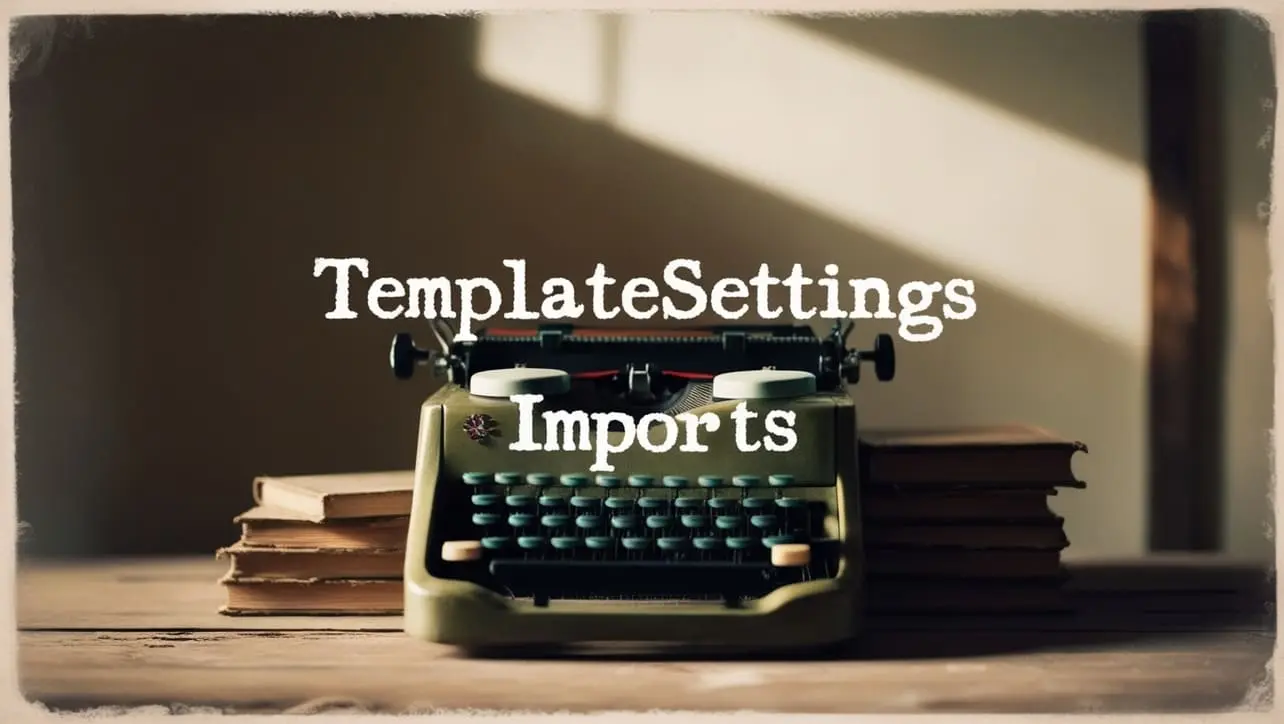
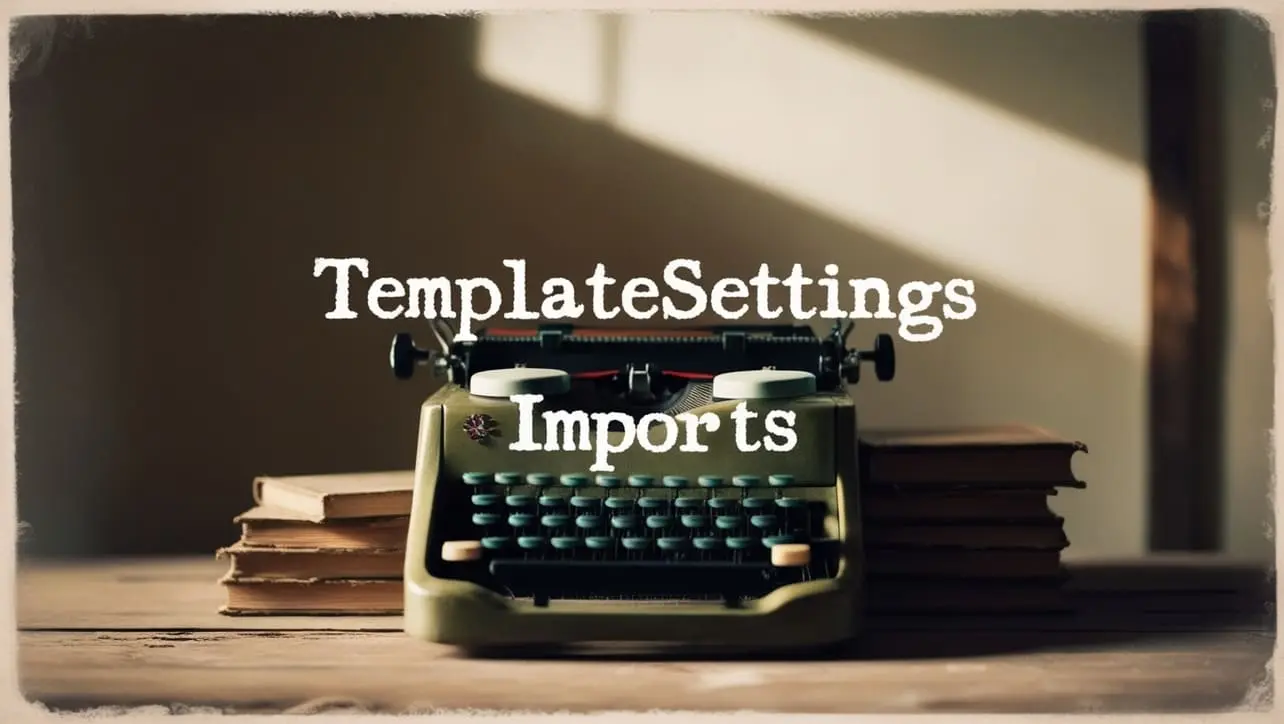
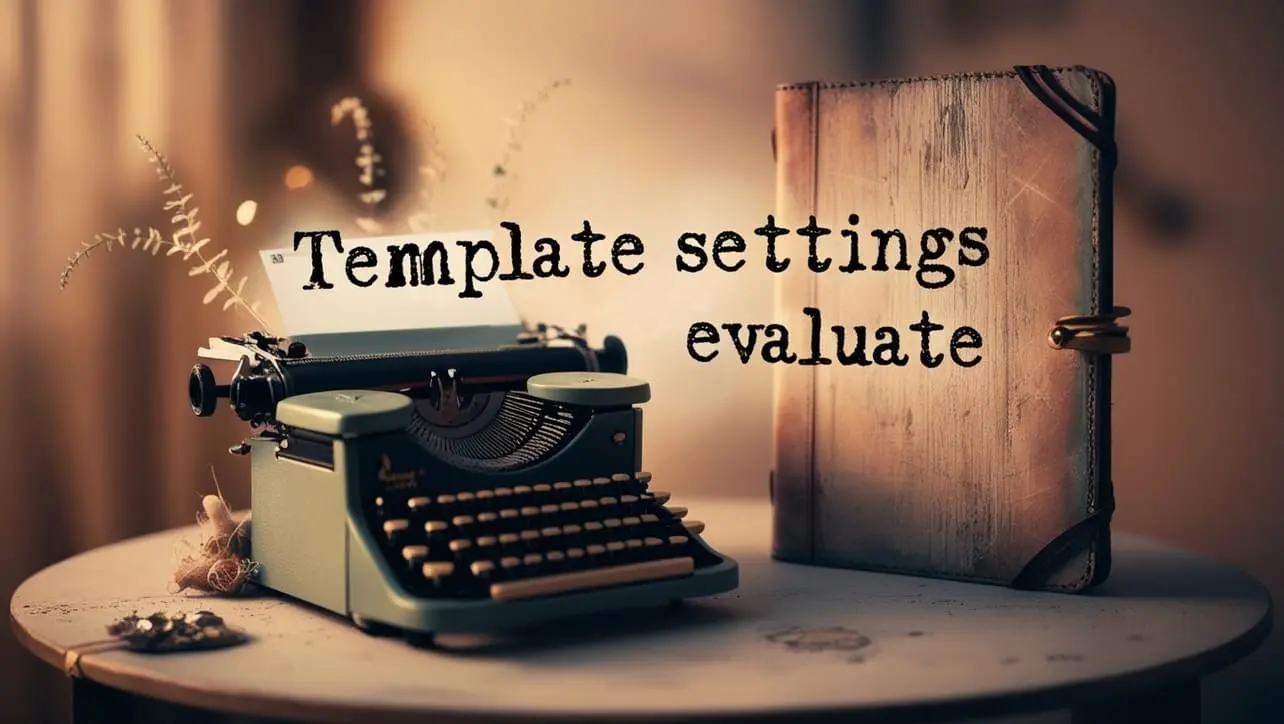
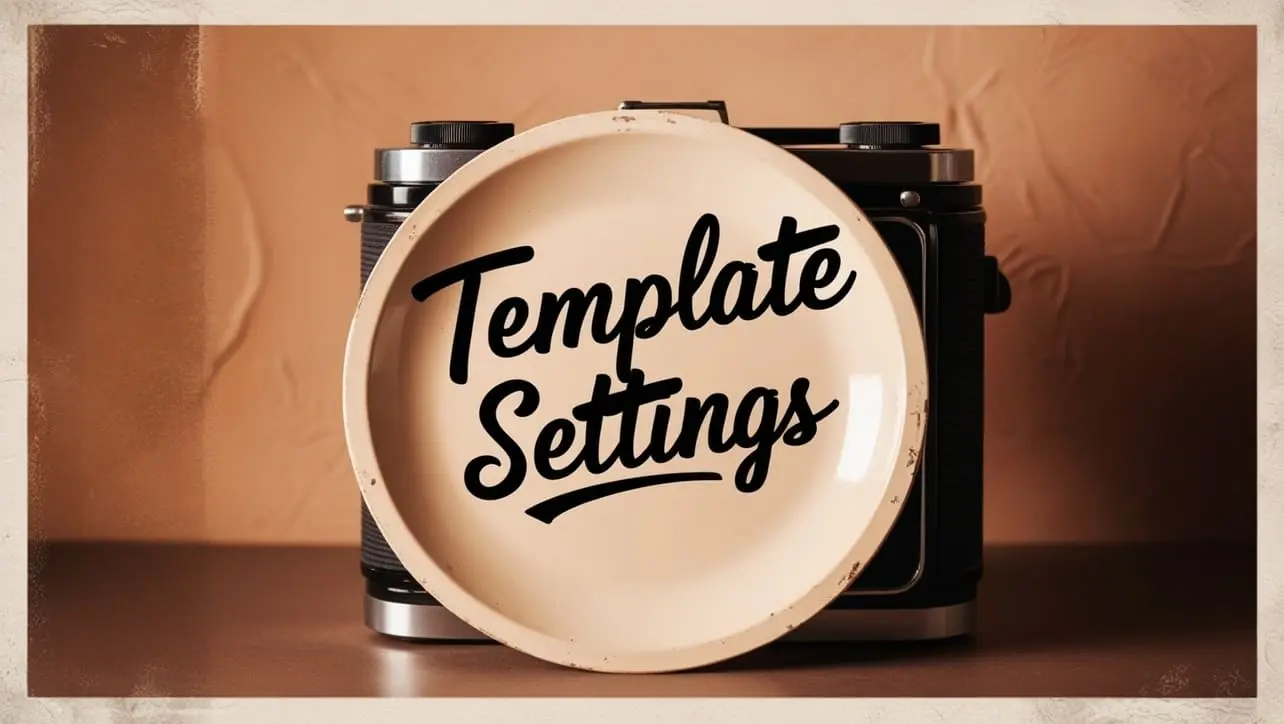
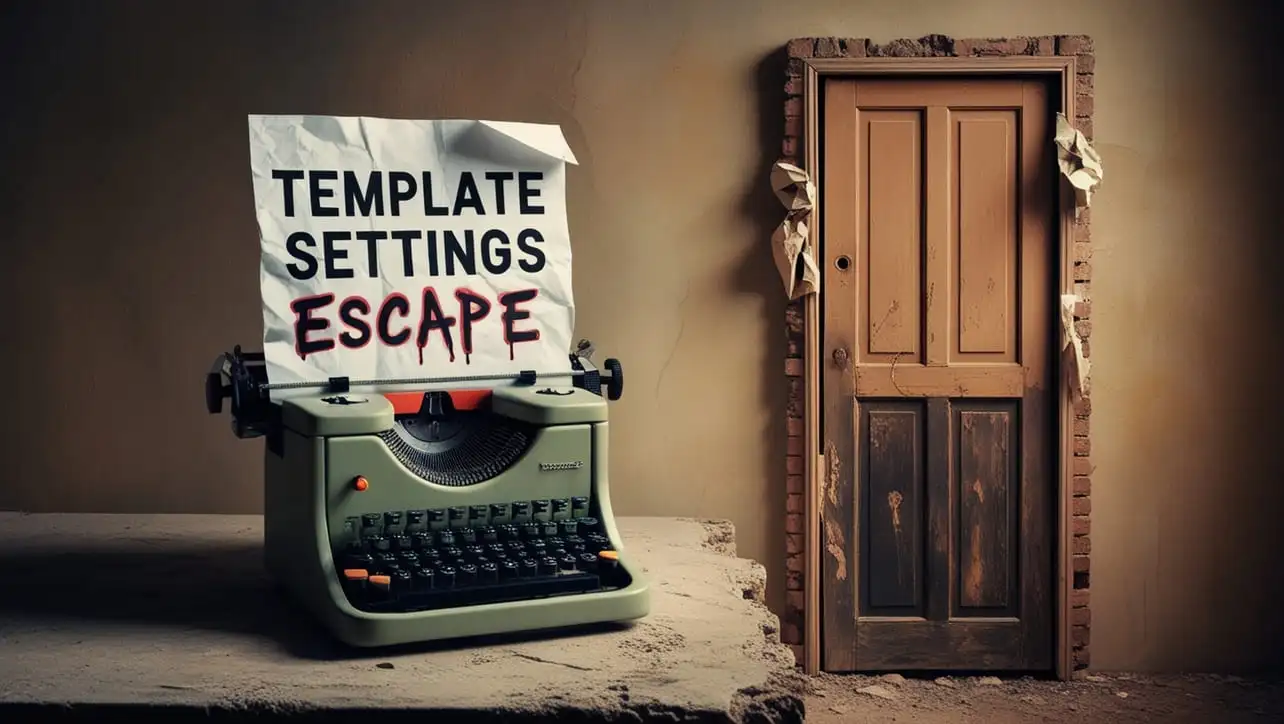
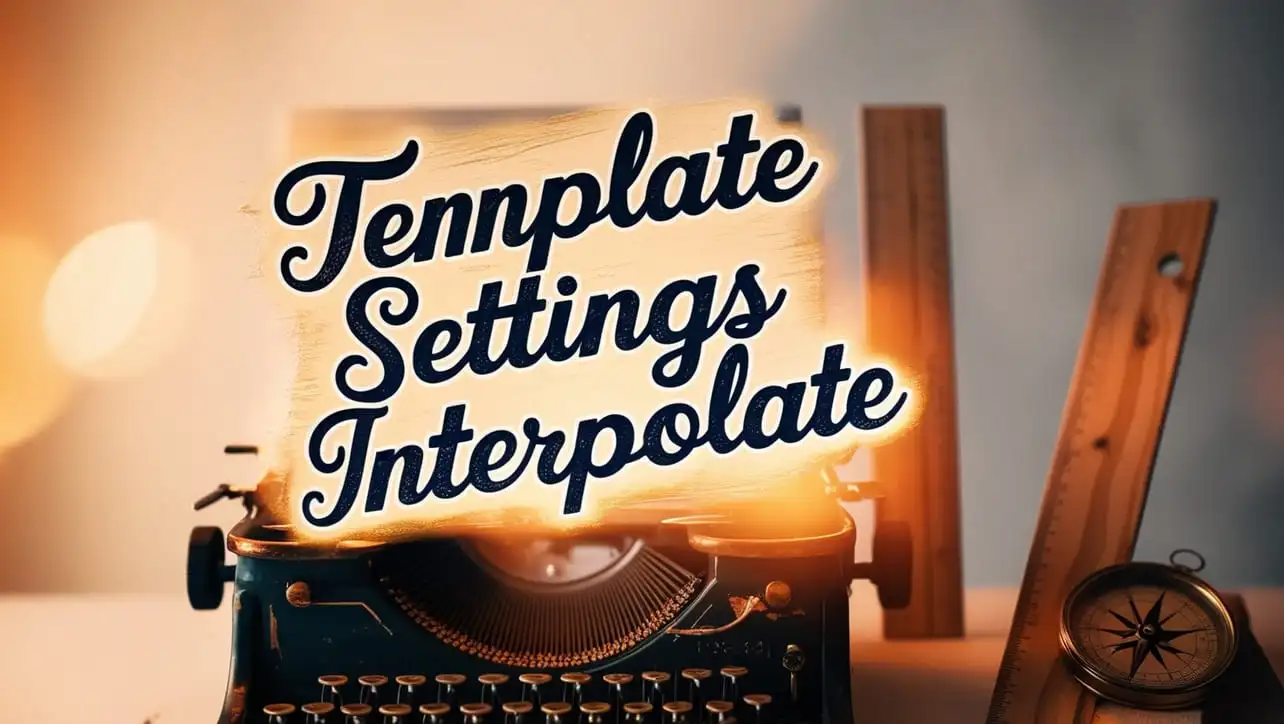
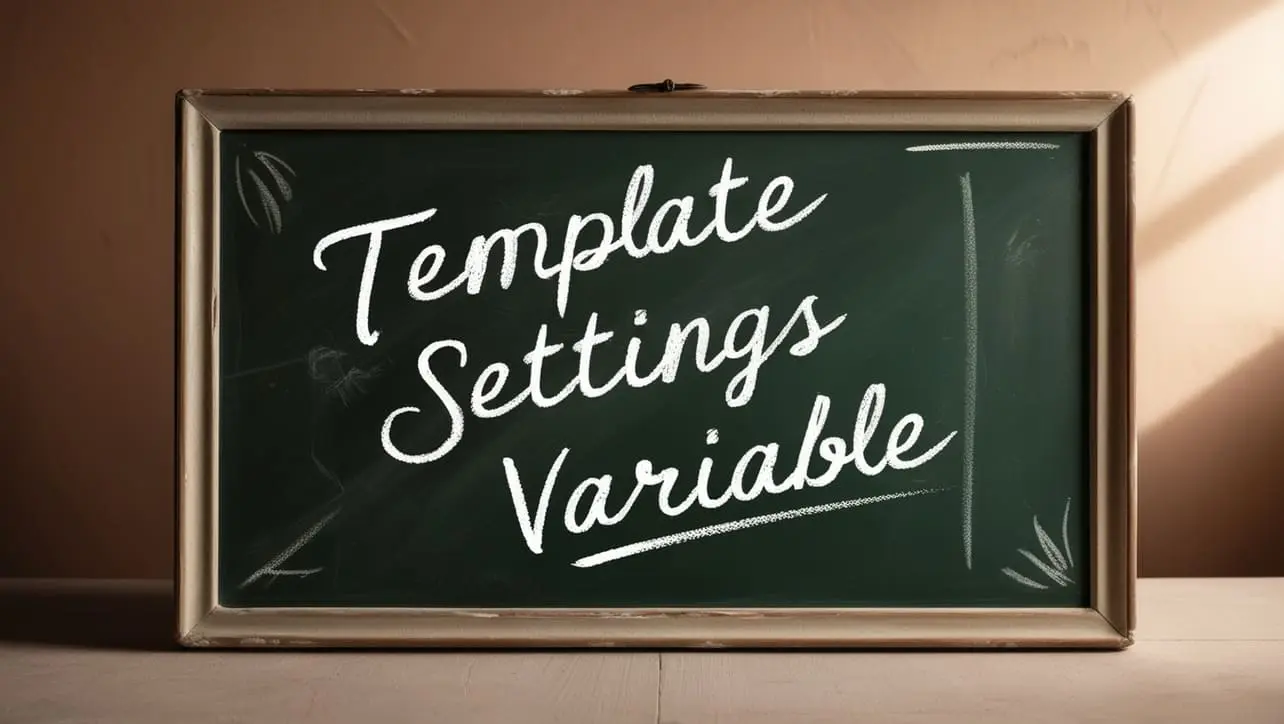
If you have any doubts regarding this article (Lodash _.max() Math Method), please comment here. I will help you immediately.