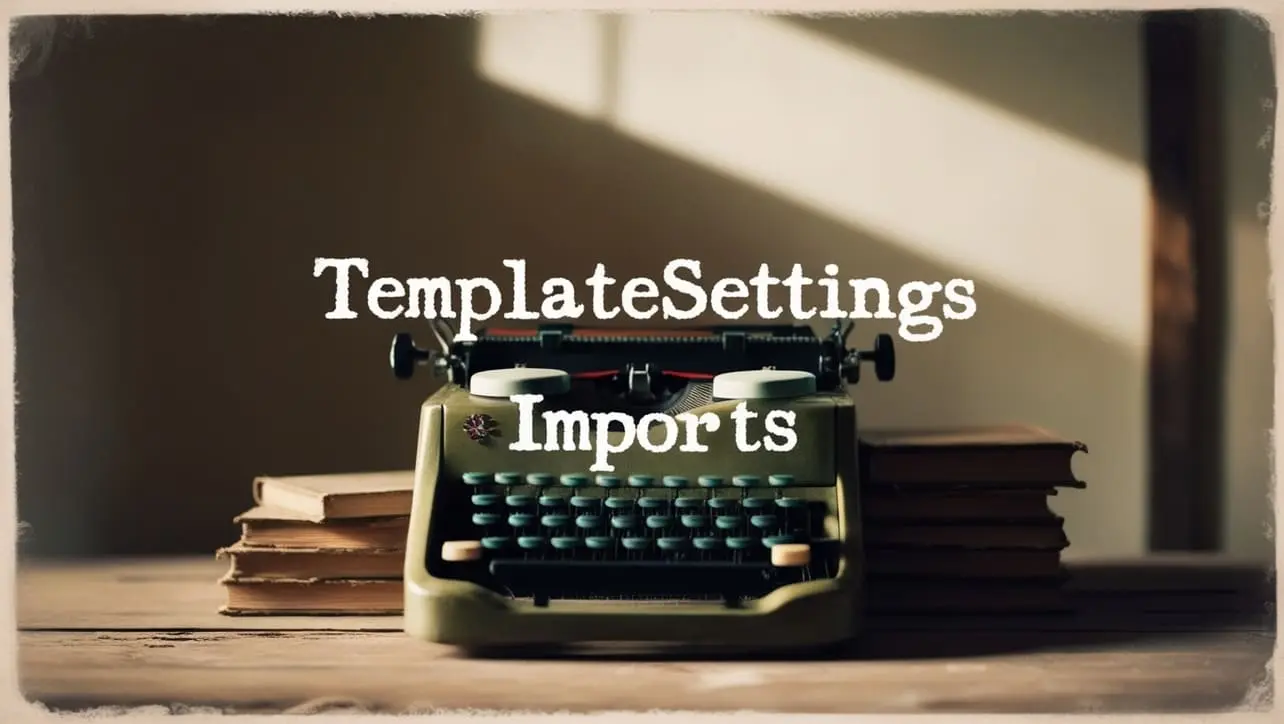
Lodash _.floor() Math Method
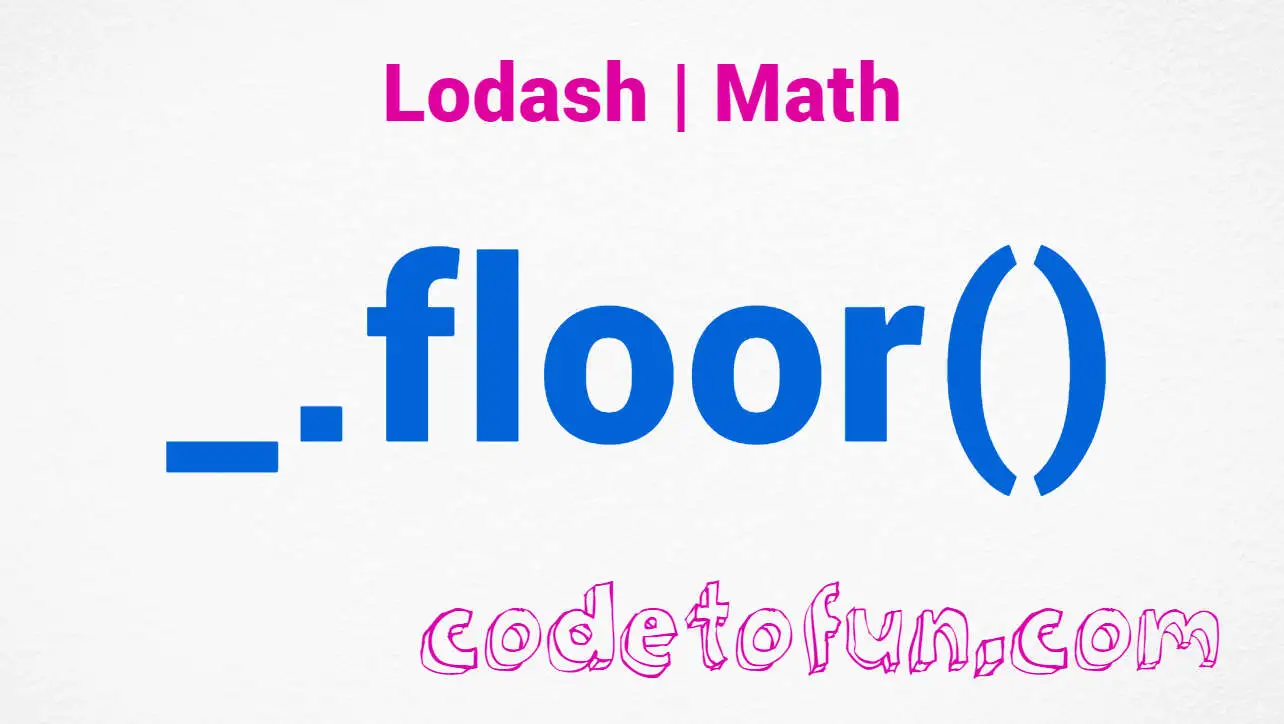
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, precise numeric operations are crucial. Lodash, a powerful utility library, provides the _.floor()
method, leveraging the capabilities of the underlying Math.floor() function.
This method allows developers to round down numeric values to the nearest integer, providing a reliable solution for scenarios where integer precision is essential.
🧠 Understanding _.floor() Method
The _.floor()
method in Lodash is a straightforward yet valuable tool for obtaining the floor of a numeric value. Whether you're dealing with financial calculations, data transformations, or any situation where rounding down is necessary, _.floor()
simplifies the process with ease.
💡 Syntax
The syntax for the _.floor()
method is straightforward:
_.floor(number, [precision=0])
- number: The numeric value to round down.
- precision (Optional): The precision of the rounding (default is 0).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.floor()
method:
const _ = require('lodash');
const originalValue = 9.75;
const roundedDownValue = _.floor(originalValue);
console.log(roundedDownValue);
// Output: 9
In this example, the originalValue is rounded down to the nearest integer using _.floor()
.
🏆 Best Practices
When working with the _.floor()
method, consider the following best practices:
Understand Rounding Behavior:
Be aware of how rounding behaves with positive and negative numbers.
_.floor()
always rounds towards negative infinity, meaning it always moves towards the lower value.example.jsCopiedconst positiveNumber = 7.9; const negativeNumber = -7.9; const roundedPositive = _.floor(positiveNumber); const roundedNegative = _.floor(negativeNumber); console.log(roundedPositive); // Output: 7 console.log(roundedNegative); // Output: -8
Precision Considerations:
When working with decimals, consider the precision parameter. It determines the number of decimal places to preserve in the result.
example.jsCopiedconst decimalValue = 15.6789; const roundedValueDefaultPrecision = _.floor(decimalValue); const roundedValueCustomPrecision = _.floor(decimalValue, 2); console.log(roundedValueDefaultPrecision); // Output: 15 console.log(roundedValueCustomPrecision); // Output: 15.67
Input Validation:
Ensure that the input to
_.floor()
is a valid numeric value. Unexpected inputs may result in unexpected output or errors.example.jsCopiedconst invalidInput = 'not a number'; if (typeof invalidInput === 'number' && !isNaN(invalidInput)) { const roundedInvalidInput = _.floor(invalidInput); console.log(roundedInvalidInput); } else { console.error('Invalid input for _.floor()'); }
📚 Use Cases
Financial Calculations:
In financial applications, precise rounding is crucial.
_.floor()
can be utilized for rounding down monetary values to ensure accurate calculations.example.jsCopiedconst transactionAmount = 49.99; const roundedTransactionAmount = _.floor(transactionAmount); console.log(roundedTransactionAmount); // Output: 49
Data Normalization:
When normalizing data, rounding down numeric values can be essential. This is particularly useful in scenarios where integer representation is desired.
example.jsCopiedconst rawScores = [85.4, 92.7, 88.1, 95.9]; const roundedScores = rawScores.map(score => _.floor(score)); console.log(roundedScores); // Output: [85, 92, 88, 95]
User Interface Adjustments:
For UI elements that require precise positioning or sizing, rounding down using
_.floor()
can be beneficial to ensure consistent and pixel-perfect rendering.example.jsCopiedconst originalHeight = 150.75; const roundedDownHeight = _.floor(originalHeight); console.log(roundedDownHeight); // Output: 150
🎉 Conclusion
The _.floor()
method in Lodash simplifies numeric rounding by rounding down values to the nearest integer. Whether you're dealing with financial data, data normalization, or UI adjustments, _.floor()
provides a reliable and convenient solution for rounding precision.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.floor()
method in your Lodash projects.
👨💻 Join our Community:
Author
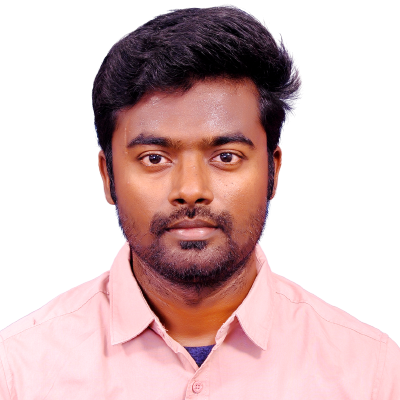
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
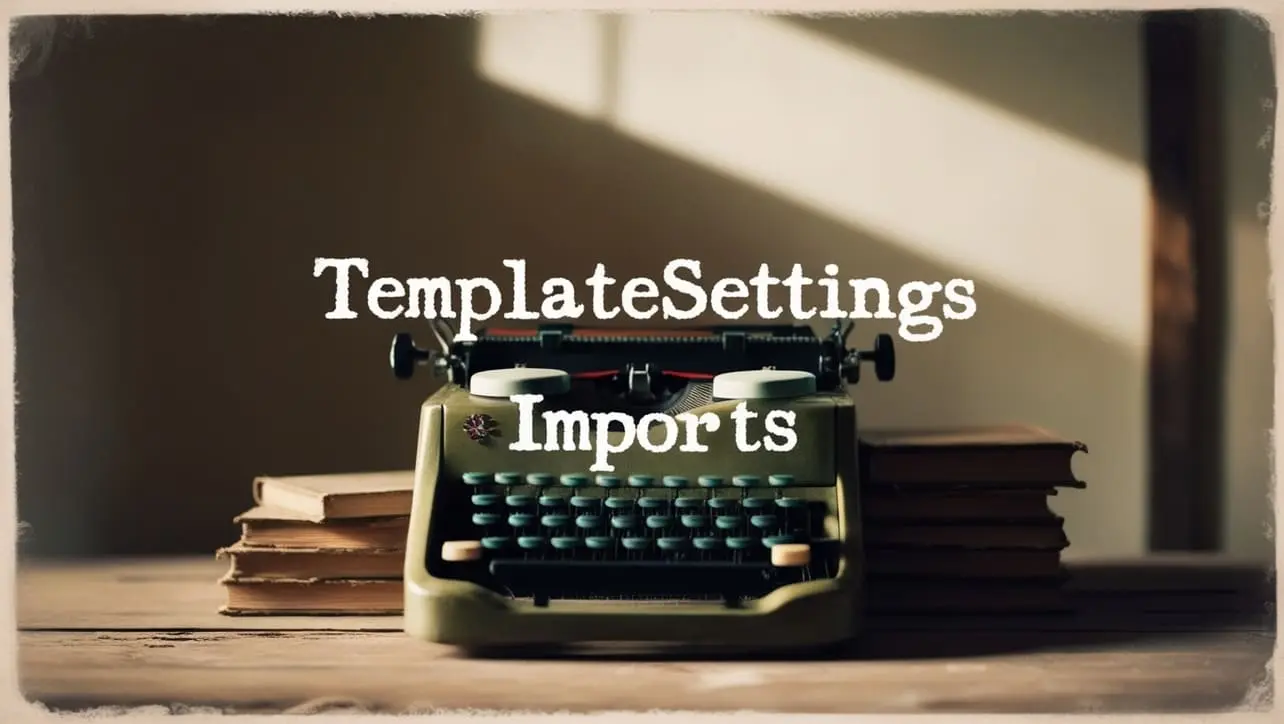
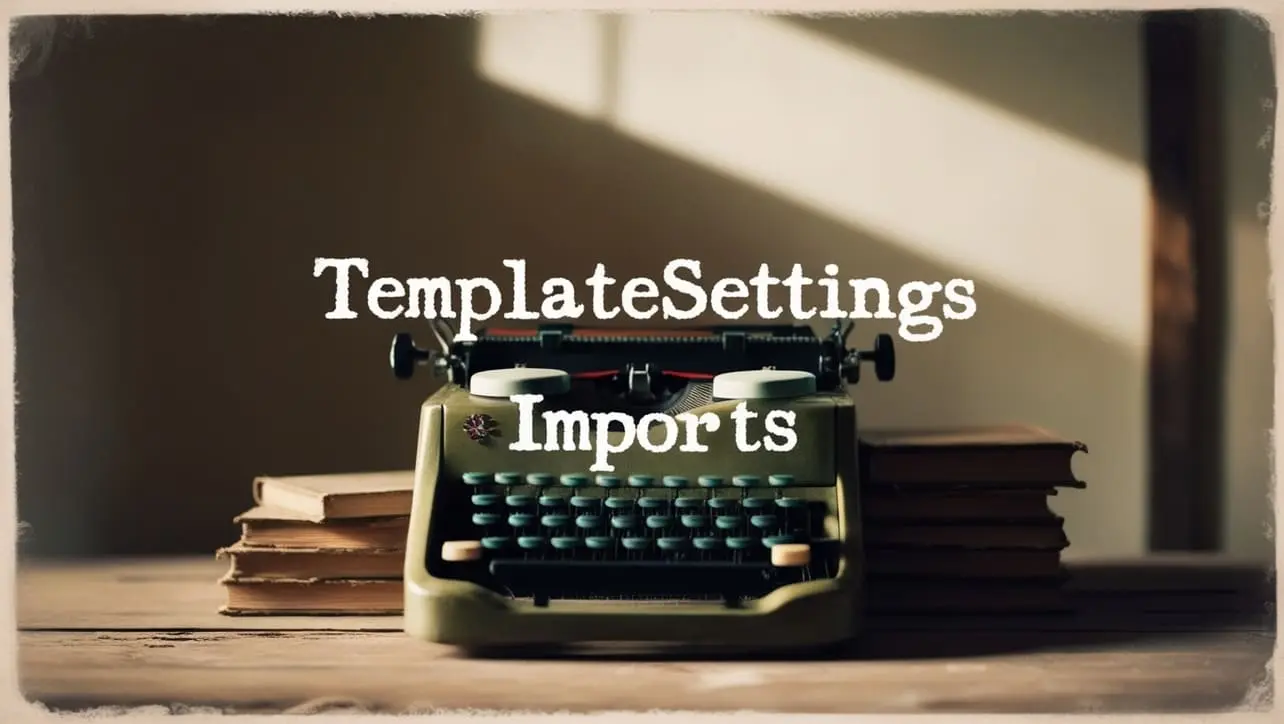
Lodash _.templateSettings.imports Property
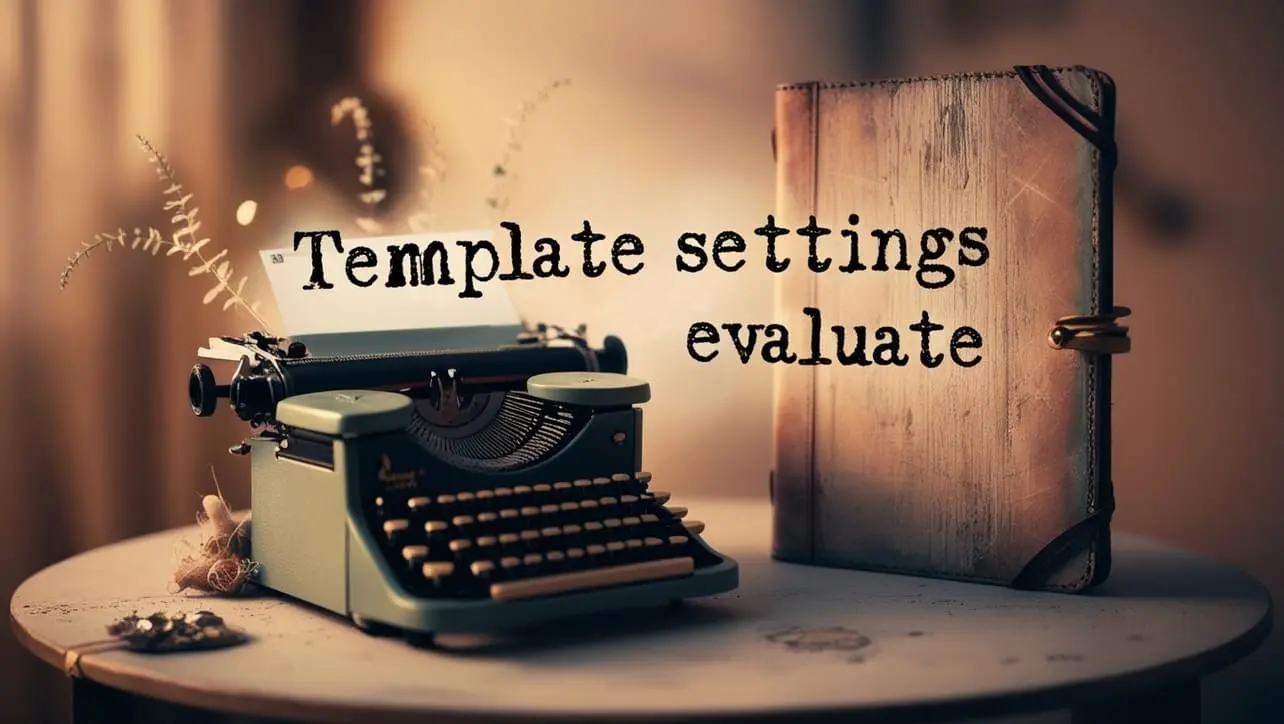
Lodash _.templateSettings.evaluate Property
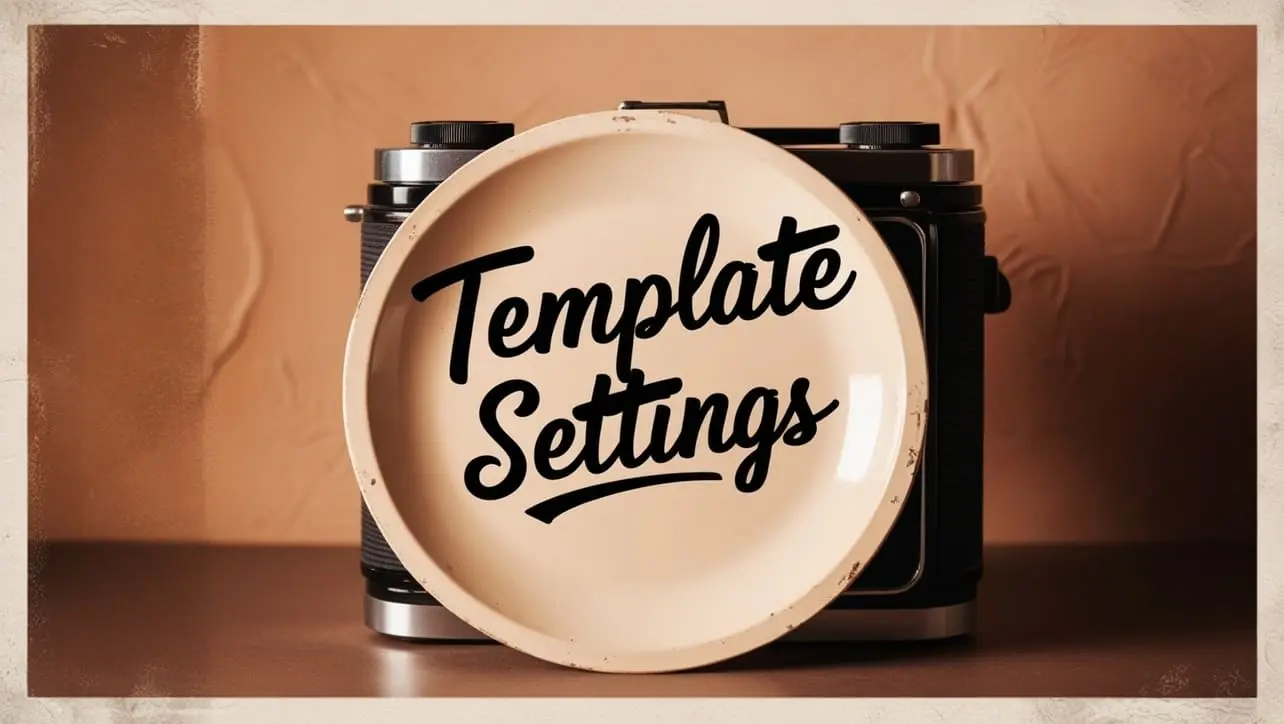
Lodash _.templateSettings Property
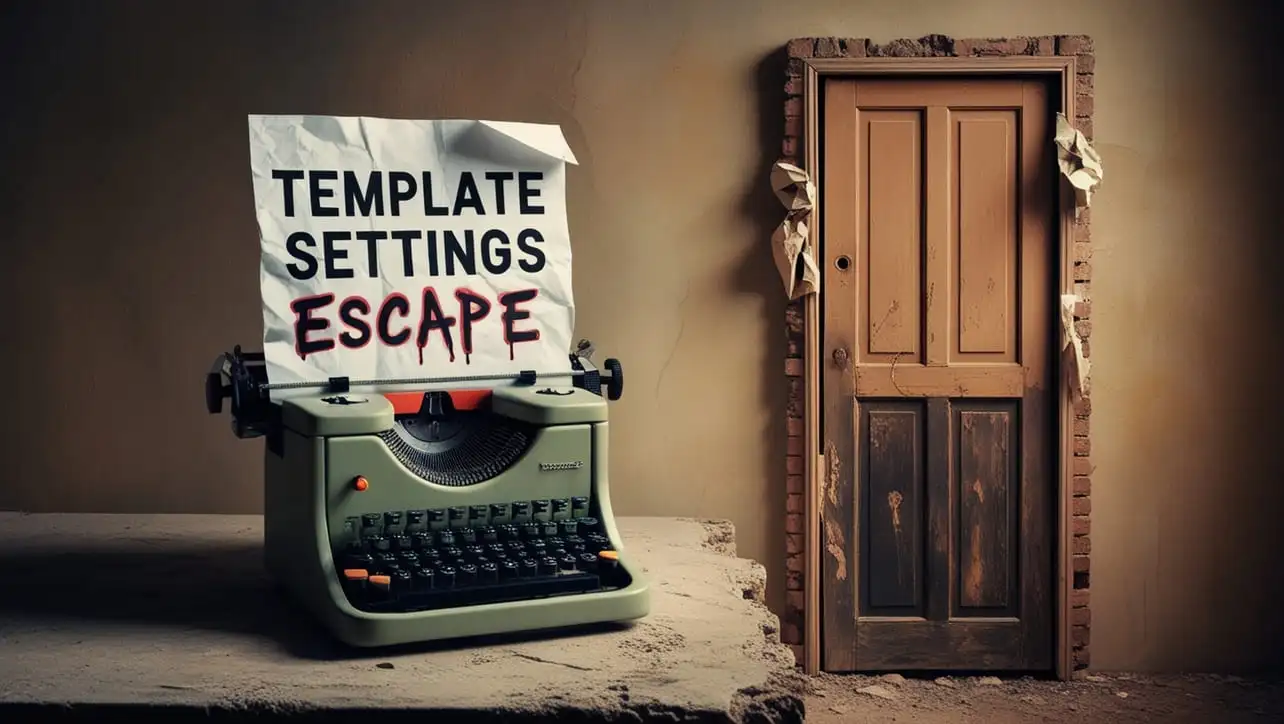
Lodash _.templateSettings.escape Property
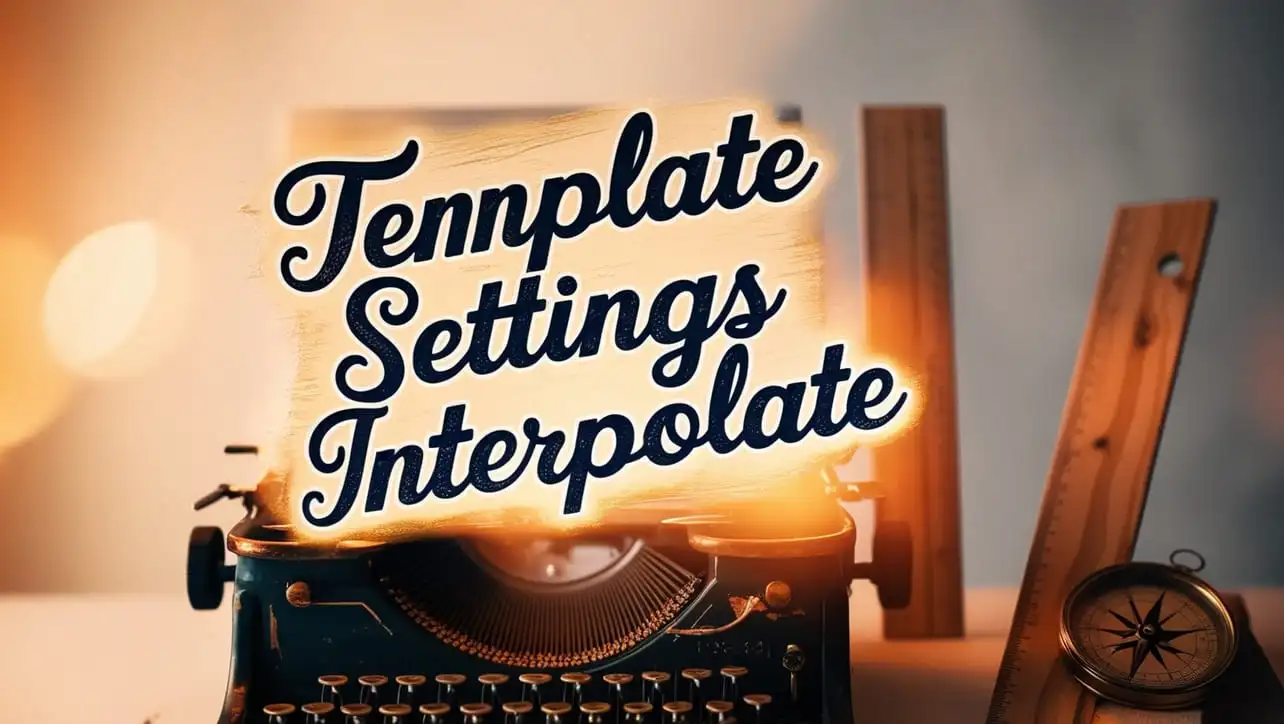
Lodash _.templateSettings.interpolate Property
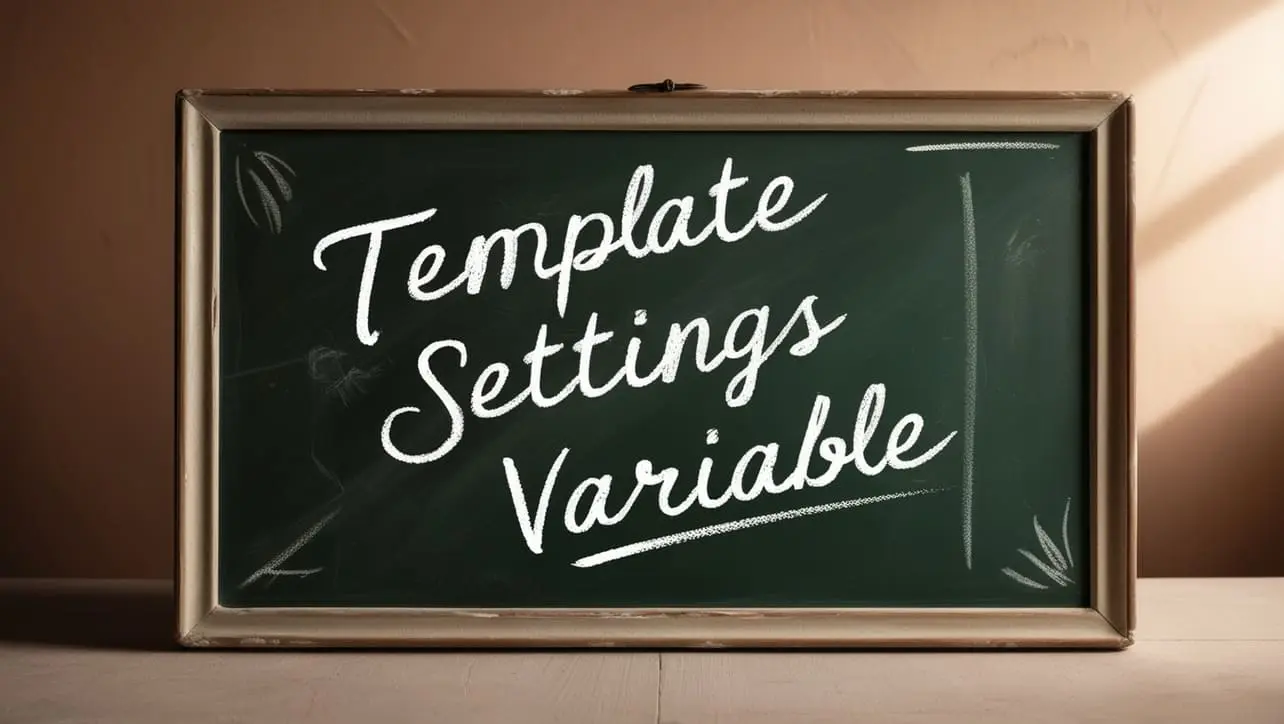
If you have any doubts regarding this article (Lodash _.floor() Math Method), please comment here. I will help you immediately.