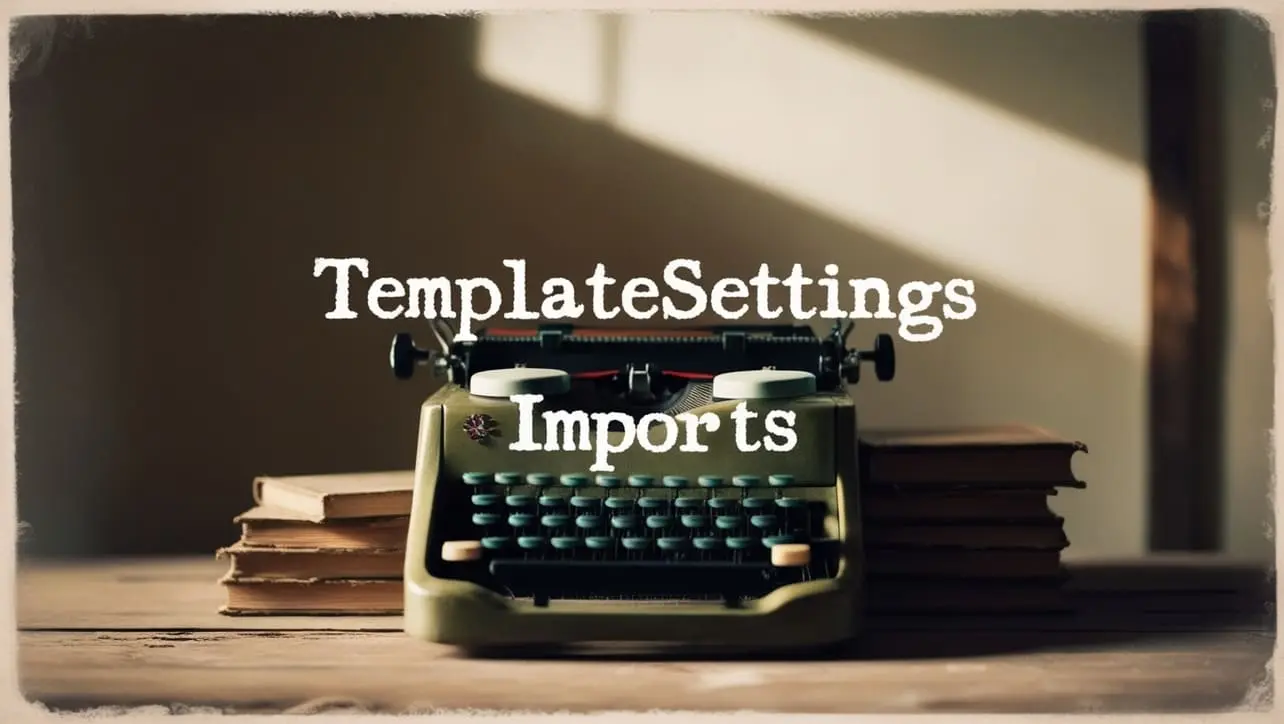
Lodash _.divide() Math Method
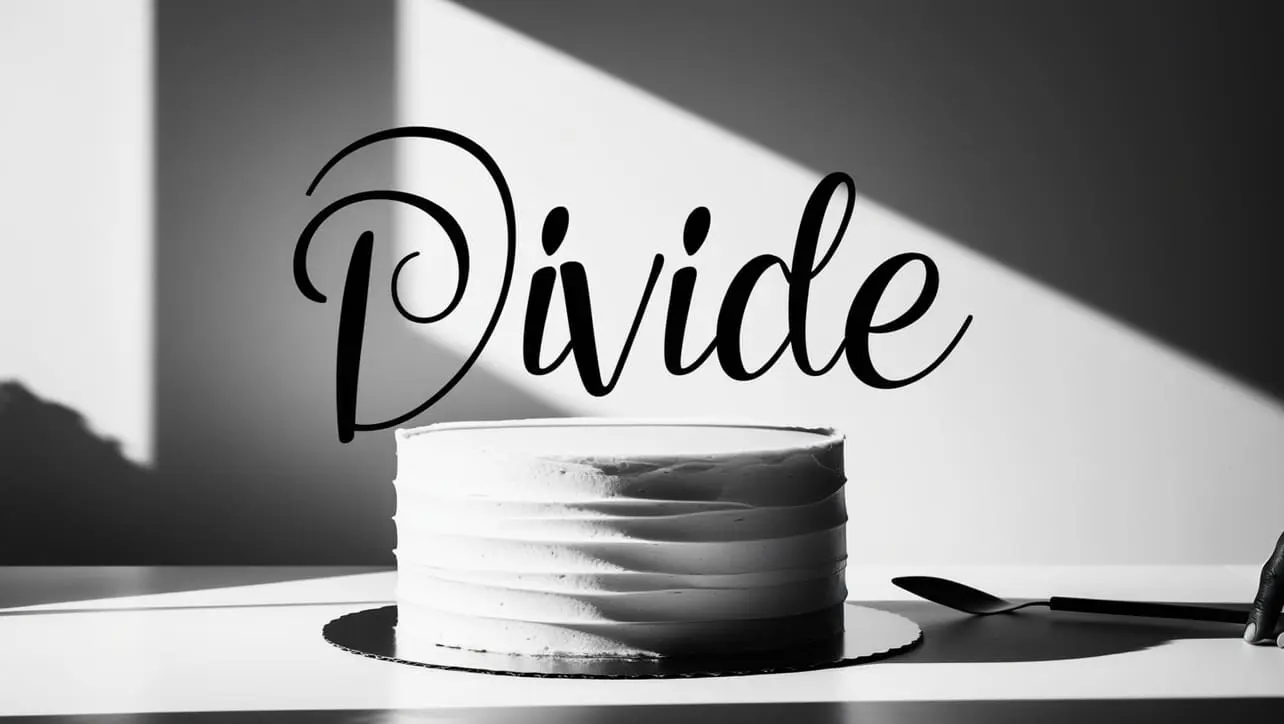
Photo Credit to CodeToFun
🙋 Introduction
When it comes to mathematical operations in JavaScript, Lodash steps in as a versatile utility library, providing convenient functions to simplify numeric calculations. Among these functions, the _.divide()
method stands out as a powerful tool for dividing numbers.
This method not only performs division but also handles edge cases gracefully, making it a valuable asset for developers dealing with numeric computations.
🧠 Understanding _.divide() Method
The _.divide()
method in Lodash is designed to divide two numbers. What sets it apart is its ability to handle scenarios where division by zero might occur, preventing unexpected errors in your code.
💡 Syntax
The syntax for the _.divide()
method is straightforward:
_.divide(dividend, divisor)
- dividend: The number to be divided.
- divisor: The number by which the dividend is to be divided.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.divide()
method:
const _ = require('lodash');
const result = _.divide(10, 2);
console.log(result);
// Output: 5
In this example, _.divide(10, 2) divides 10 by 2, yielding a result of 5.
🏆 Best Practices
When working with the _.divide()
method, consider the following best practices:
Handling Division by Zero:
Use
_.divide()
when there's a possibility of dividing by zero, as it gracefully handles such scenarios by returning Infinity or -Infinity instead of throwing an error.example.jsCopiedconst result = _.divide(10, 0); console.log(result); // Output: Infinity
Numeric Type Coercion:
Ensure that the dividend and divisor parameters are numeric values.
_.divide()
performs automatic type coercion, but it's good practice to provide explicit numeric values.example.jsCopiedconst result = _.divide('10', 2); console.log(result); // Output: 5
Precision and Rounding:
Be mindful of precision in division results. If precision is crucial, consider rounding the result using additional methods or libraries.
example.jsCopiedconst result = _.round(_.divide(1, 3), 2); console.log(result); // Output: 0.33
📚 Use Cases
Handling User Input:
When dealing with user input for mathematical operations,
_.divide()
can be employed to perform division while mitigating the risk of errors caused by unexpected input.example.jsCopiedconst userInput = /* ...get user input... */ ; const numericValue = parseFloat(userInput); if (!isNaN(numericValue)) { const result = _.divide(numericValue, 2); console.log(result); } else { console.error('Invalid numeric input'); }
Proportional Distribution:
In scenarios where you need to distribute values proportionally,
_.divide()
can be a handy tool to calculate proportions based on specific ratios.example.jsCopiedconst totalValue = 100; const ratioA = 2; const ratioB = 3; const portionA = _.divide(totalValue * ratioA, ratioA + ratioB); const portionB = _.divide(totalValue * ratioB, ratioA + ratioB); console.log(portionA, portionB);
Calculating Percentages:
For calculating percentages or dividing values based on a percentage,
_.divide()
provides a straightforward solution.example.jsCopiedconst totalAmount = 500; const percentage = 20; const portion = _.divide(totalAmount * percentage, 100); console.log(portion); // Output: 100
🎉 Conclusion
The _.divide()
method in Lodash simplifies division operations in JavaScript, offering not only a convenient way to divide numbers but also handling edge cases with finesse. Whether you're working with user input, proportional distribution, or percentage calculations, _.divide()
proves to be a reliable companion for your numeric computations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.divide()
method in your Lodash projects.
👨💻 Join our Community:
Author
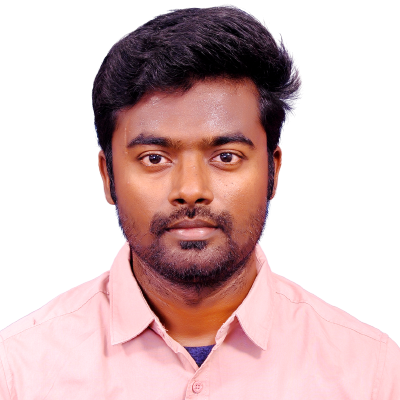
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
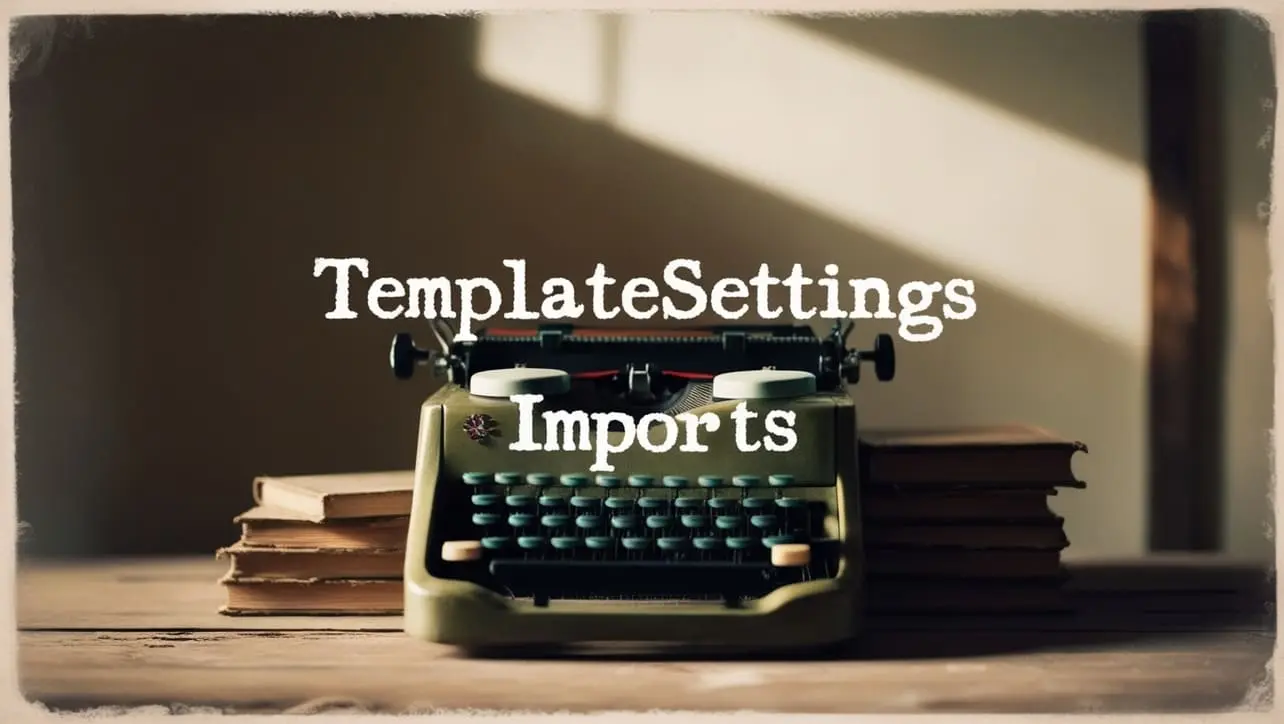
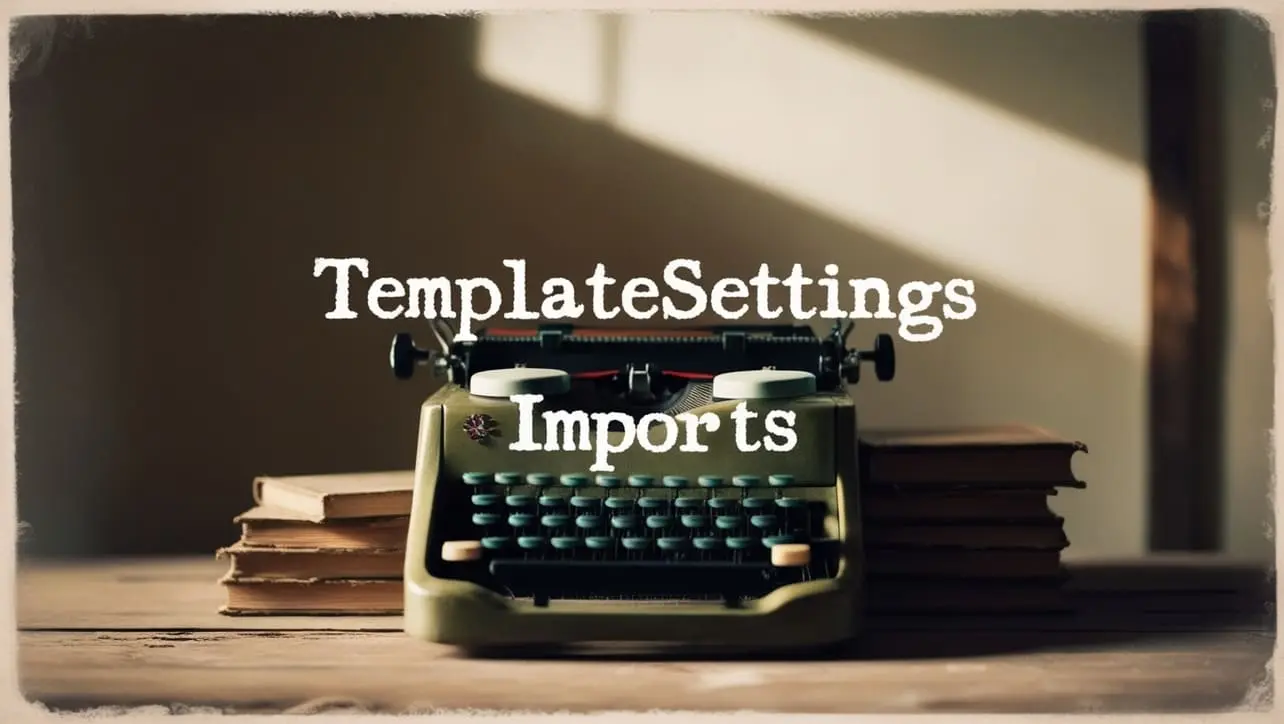
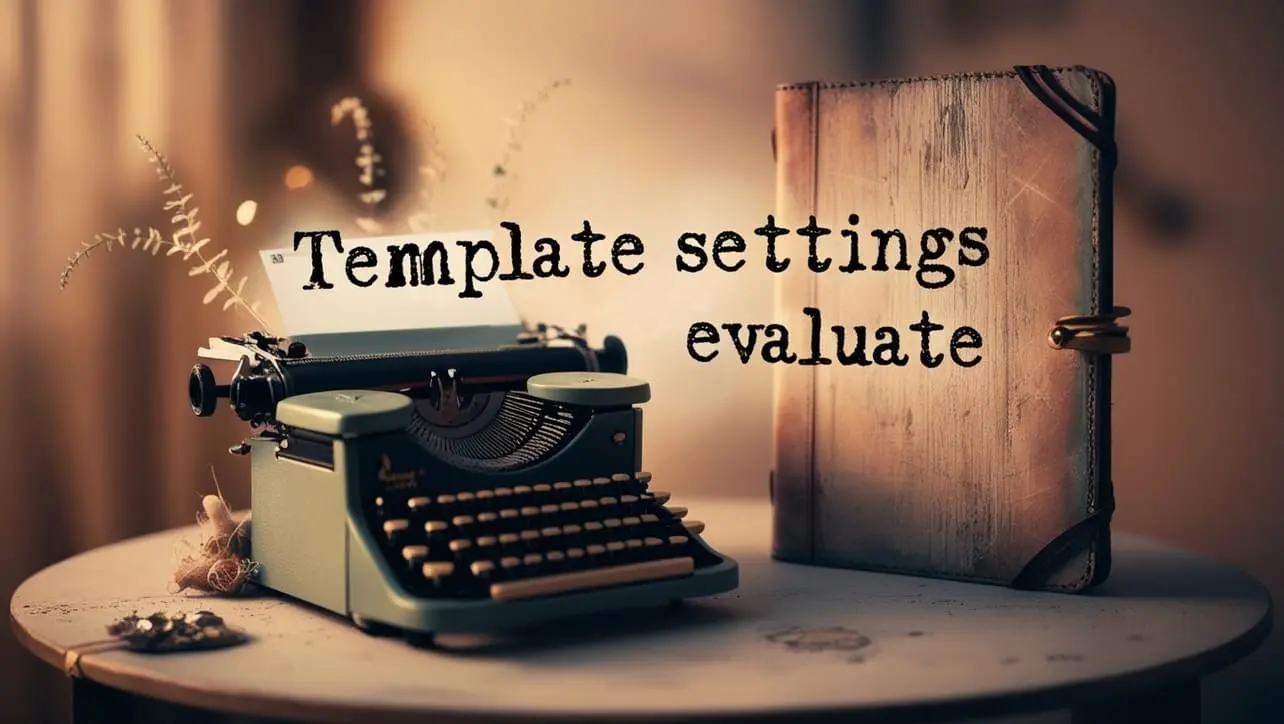
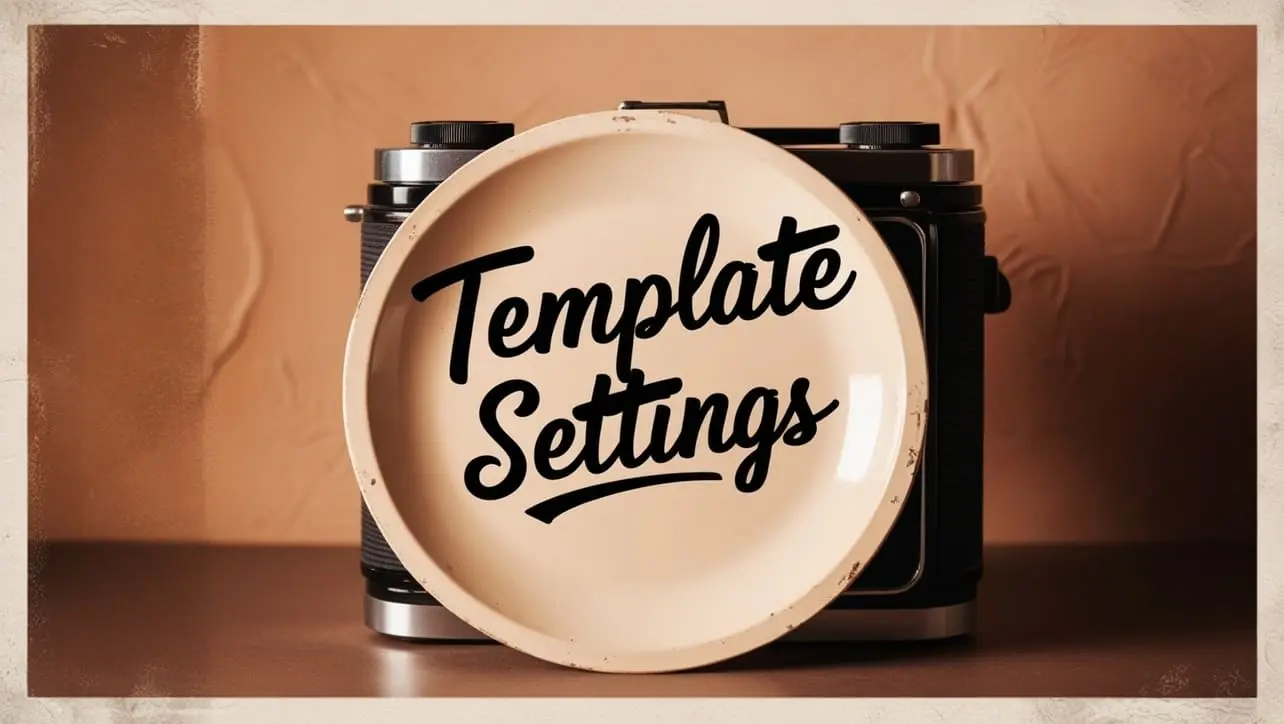
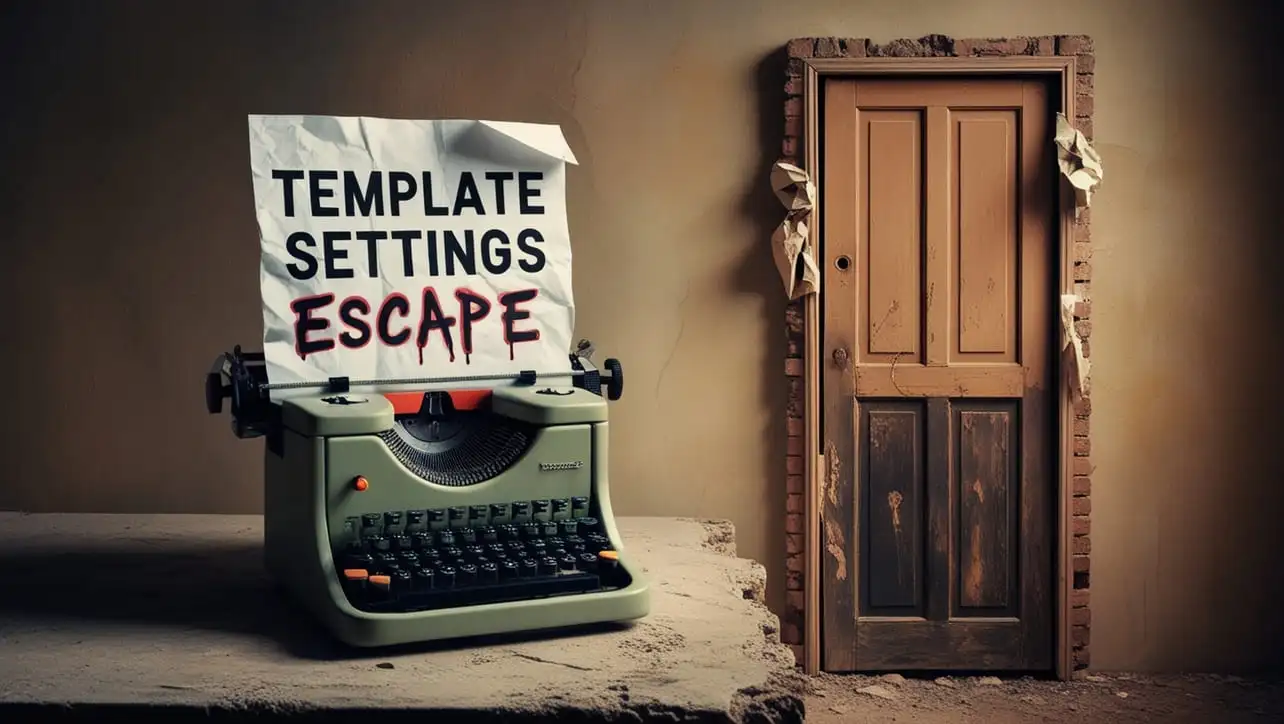
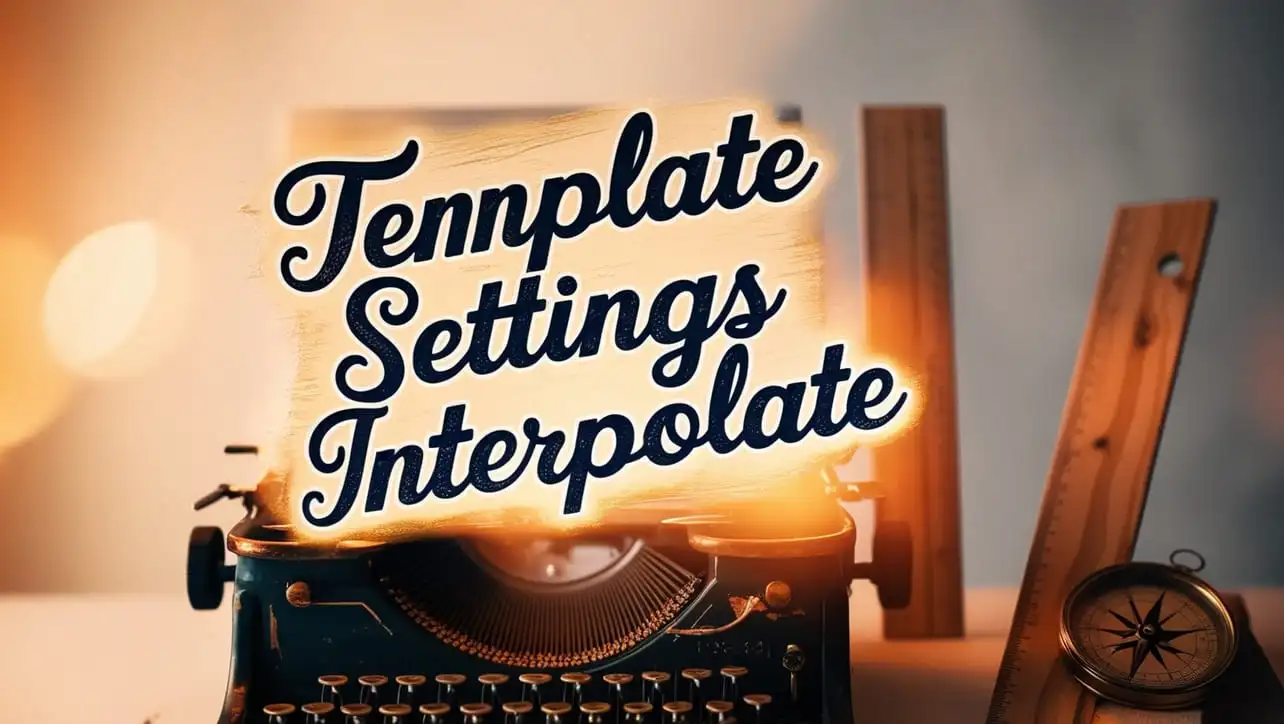
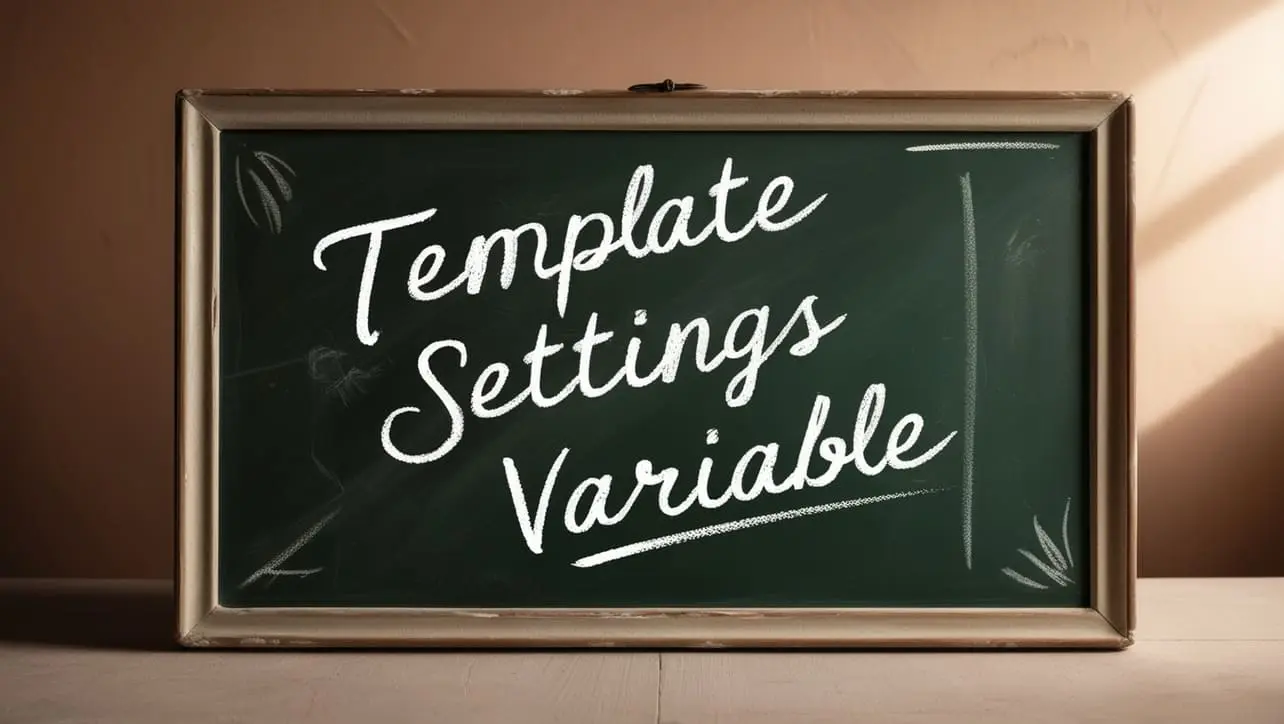
If you have any doubts regarding this article (Lodash _.divide() Math Method), please comment here. I will help you immediately.