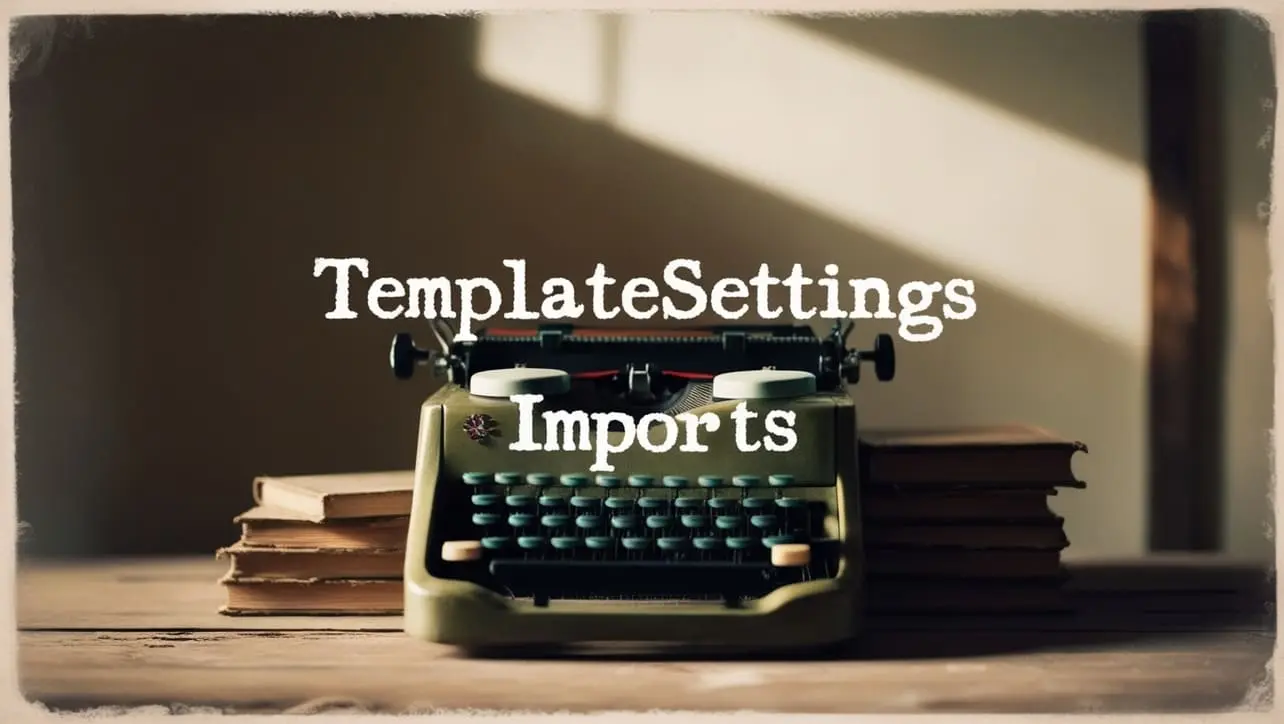
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toArray() Lang Method
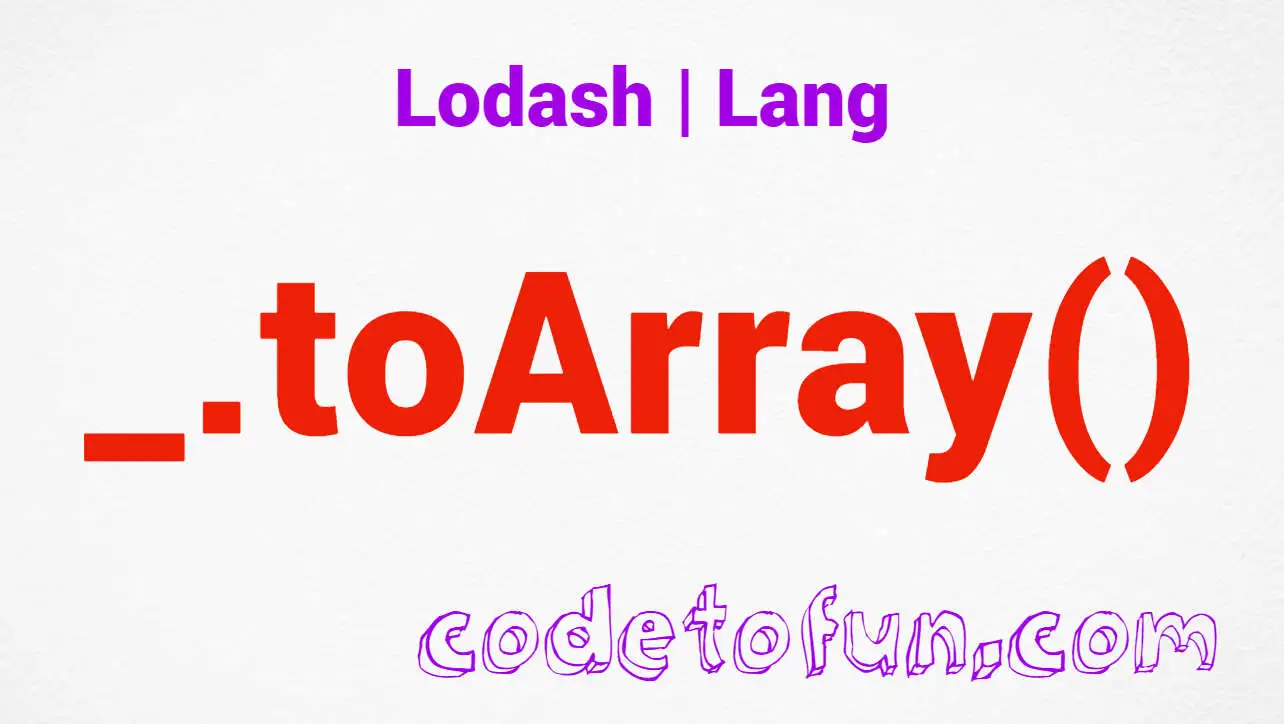
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript programming, Lodash stands out as a comprehensive utility library, offering a wide array of functions to simplify common tasks. Among these functions is the _.toArray()
method, a versatile tool for converting values into arrays.
This method provides a straightforward way to transform various data types into arrays, facilitating seamless data manipulation and compatibility.
🧠 Understanding _.toArray() Method
The _.toArray()
method in Lodash is designed to convert values into arrays, accommodating a diverse range of input types. Whether dealing with objects, strings, or array-like structures, this method streamlines the process of creating uniform arrays for further manipulation.
💡 Syntax
The syntax for the _.toArray()
method is straightforward:
_.toArray(value)
- value: The value to convert into an array.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toArray()
method:
const _ = require('lodash');
const exampleObject = { a: 1, b: 2, c: 3 };
const arrayFromObject = _.toArray(exampleObject);
console.log(arrayFromObject);
// Output: [1, 2, 3]
In this example, the exampleObject is converted into an array using _.toArray()
, resulting in an array containing the values of the object.
🏆 Best Practices
When working with the _.toArray()
method, consider the following best practices:
Convert Objects to Arrays:
Utilize
_.toArray()
to efficiently convert objects into arrays. This can be particularly handy when you need to extract values from objects for further processing.example.jsCopiedconst sampleObject = { x: 10, y: 20, z: 30 }; const arrayFromObject = _.toArray(sampleObject); console.log(arrayFromObject); // Output: [10, 20, 30]
Handle Array-Like Structures:
_.toArray()
is not limited to traditional arrays or objects; it can also handle array-like structures such as the arguments object or strings.example.jsCopiedfunction exampleFunction() { const argumentsArray = _.toArray(arguments); console.log(argumentsArray); } exampleFunction(1, 'two', { three: 3 }); // Output: [1, 'two', { three: 3 }]
Ensure Consistent Output:
When using
_.toArray()
with different data types, ensure that the resulting arrays have consistent structures for smoother downstream operations.example.jsCopiedconst mixedData = [42, 'hello', { key: 'value' }]; const arrayFromMixedData = _.toArray(mixedData); console.log(arrayFromMixedData); // Output: [42, 'hello', { key: 'value' }]
📚 Use Cases
Object Value Extraction:
_.toArray()
is particularly useful when you need to extract values from objects, creating an array for easy access and manipulation.example.jsCopiedconst userPreferences = { theme: 'dark', fontSize: 16, language: 'en' }; const preferencesArray = _.toArray(userPreferences); console.log(preferencesArray); // Output: ['dark', 16, 'en']
String to Character Array:
When dealing with strings,
_.toArray()
can be employed to convert a string into an array of its individual characters.example.jsCopiedconst sampleString = 'Lodash'; const charArray = _.toArray(sampleString); console.log(charArray); // Output: ['L', 'o', 'd', 'a', 's', 'h']
Handling Arguments in Functions:
Functions with variable arguments can benefit from
_.toArray()
to convert the arguments object into a more manageable array.example.jsCopiedfunction processArguments() { const argsArray = _.toArray(arguments); // ... perform operations on argsArray } processArguments('arg1', 'arg2', 'arg3');
🎉 Conclusion
The _.toArray()
method in Lodash proves to be a valuable asset for JavaScript developers, offering a simple yet powerful way to convert various data types into arrays. Whether dealing with objects, strings, or array-like structures, this method streamlines the conversion process, enhancing the flexibility and compatibility of your code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toArray()
method in your Lodash projects.
👨💻 Join our Community:
Author
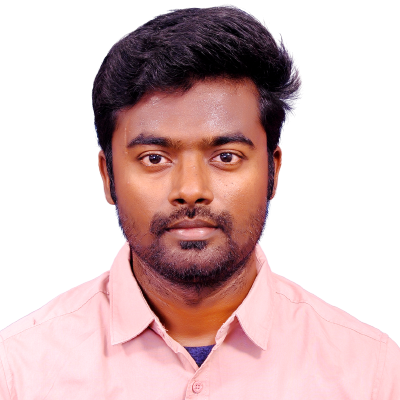
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
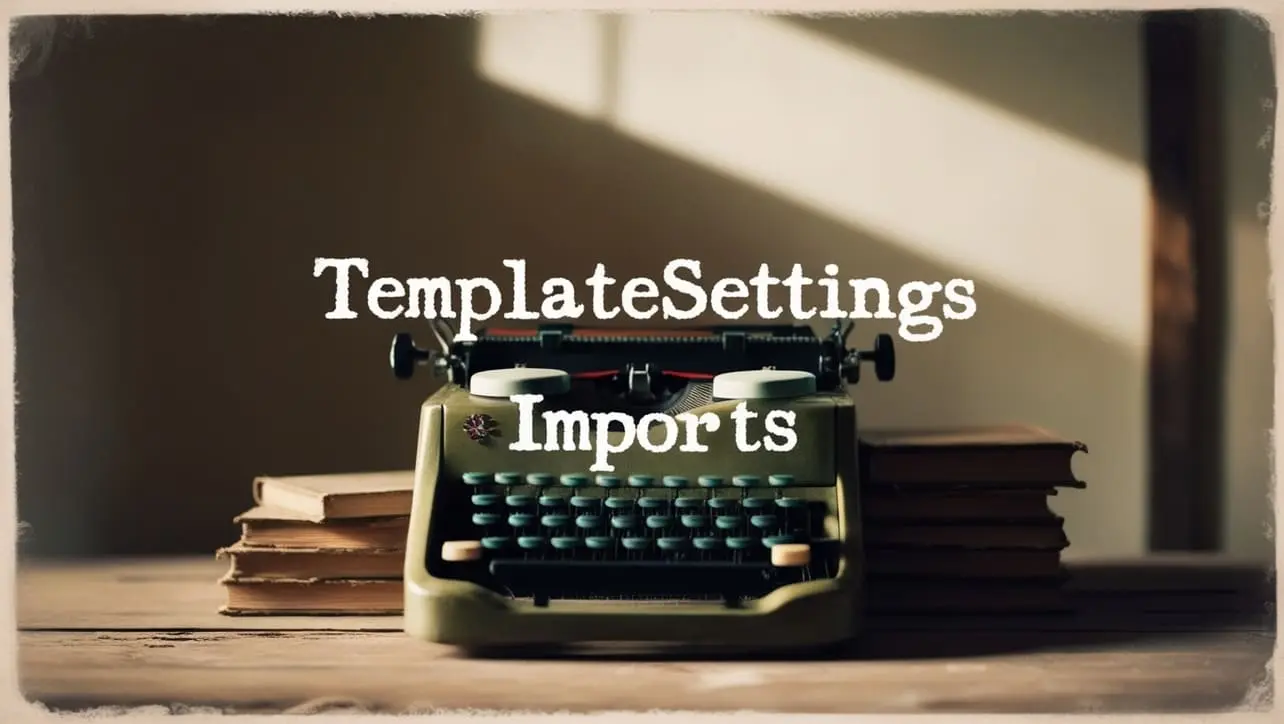
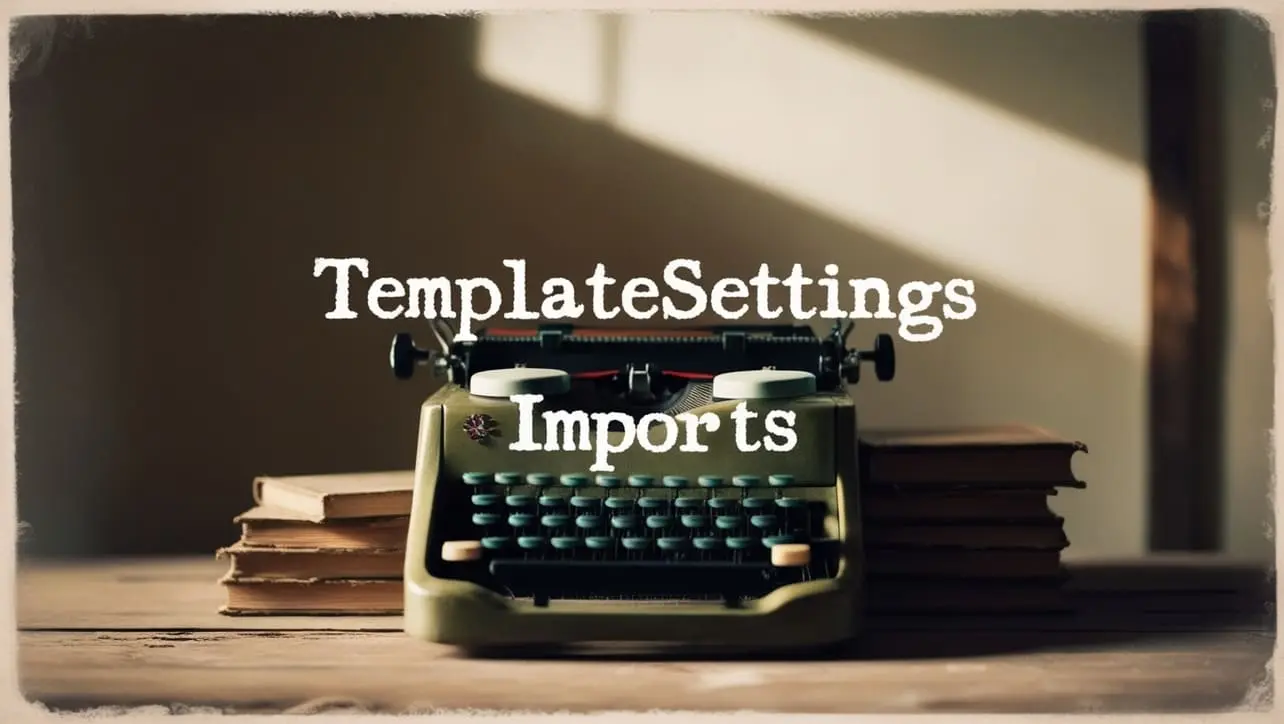
Lodash _.templateSettings.imports Property
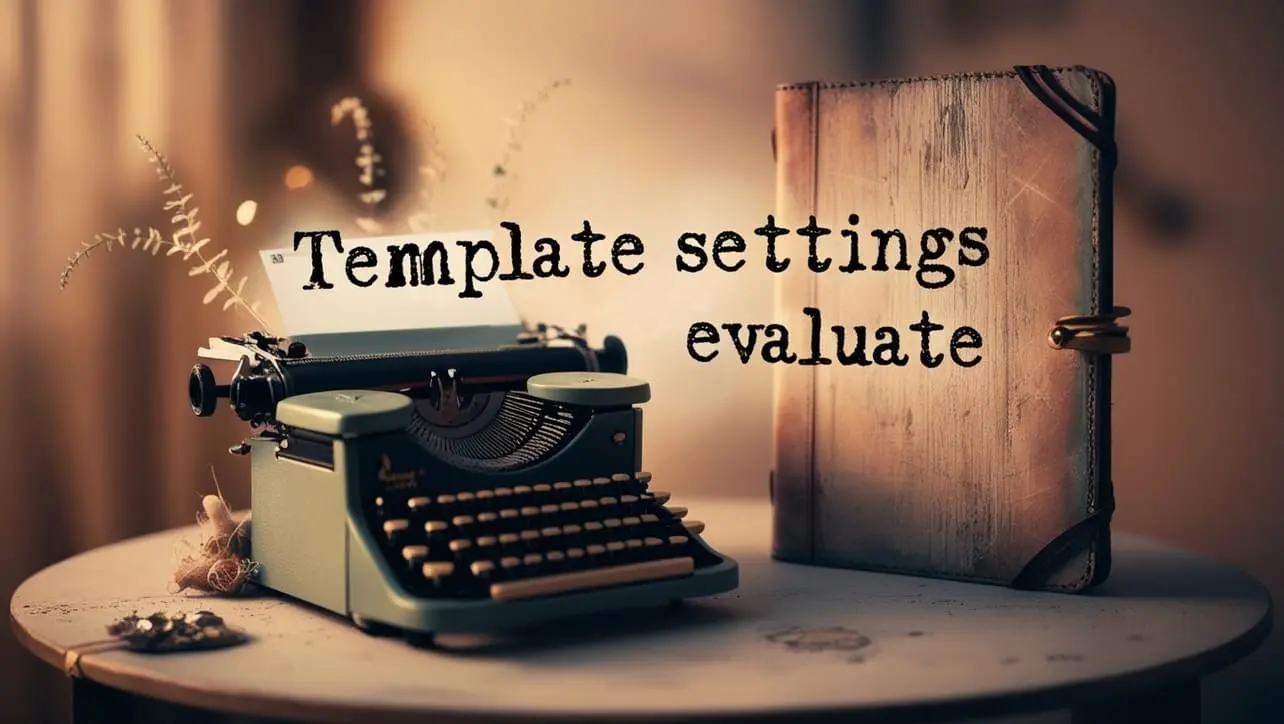
Lodash _.templateSettings.evaluate Property
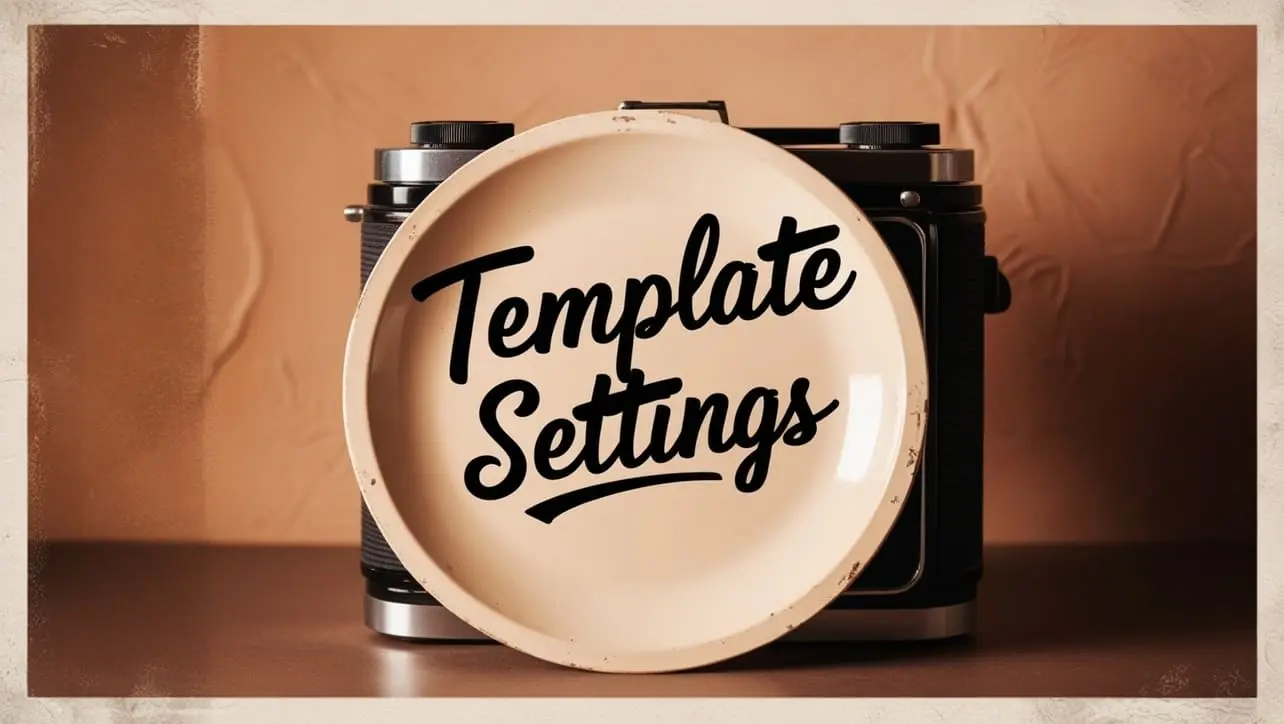
Lodash _.templateSettings Property
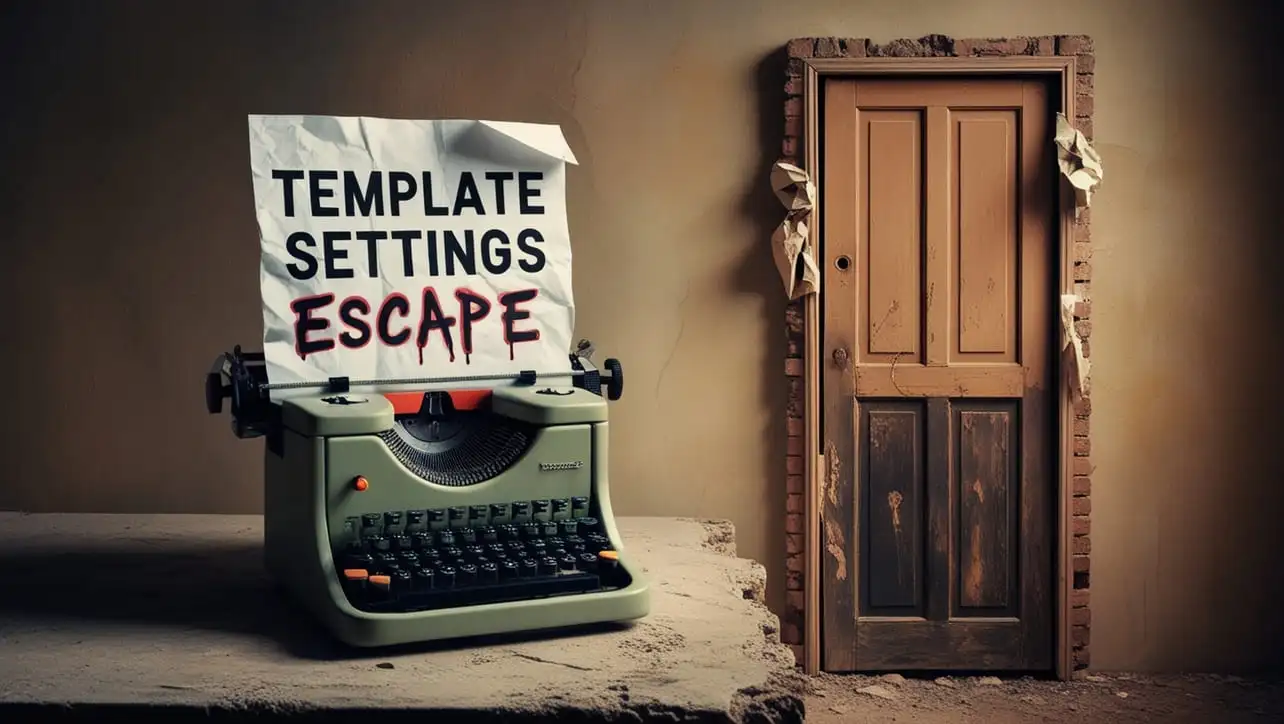
Lodash _.templateSettings.escape Property
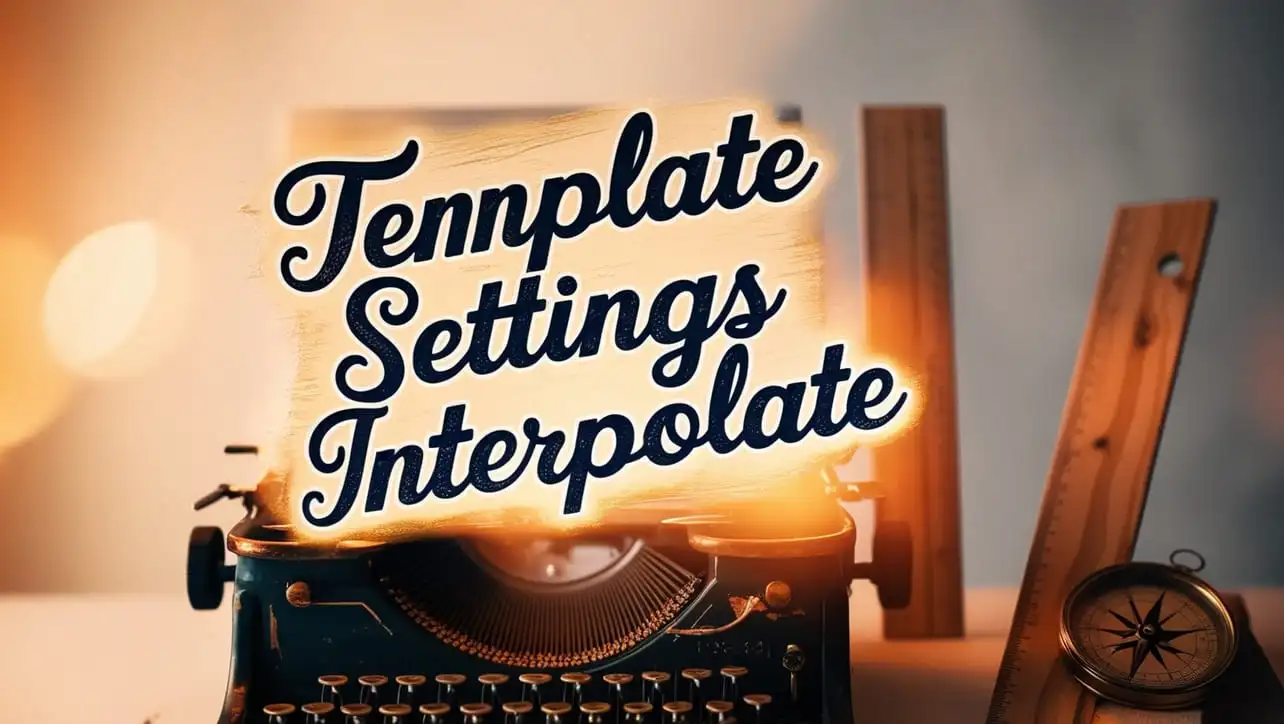
Lodash _.templateSettings.interpolate Property
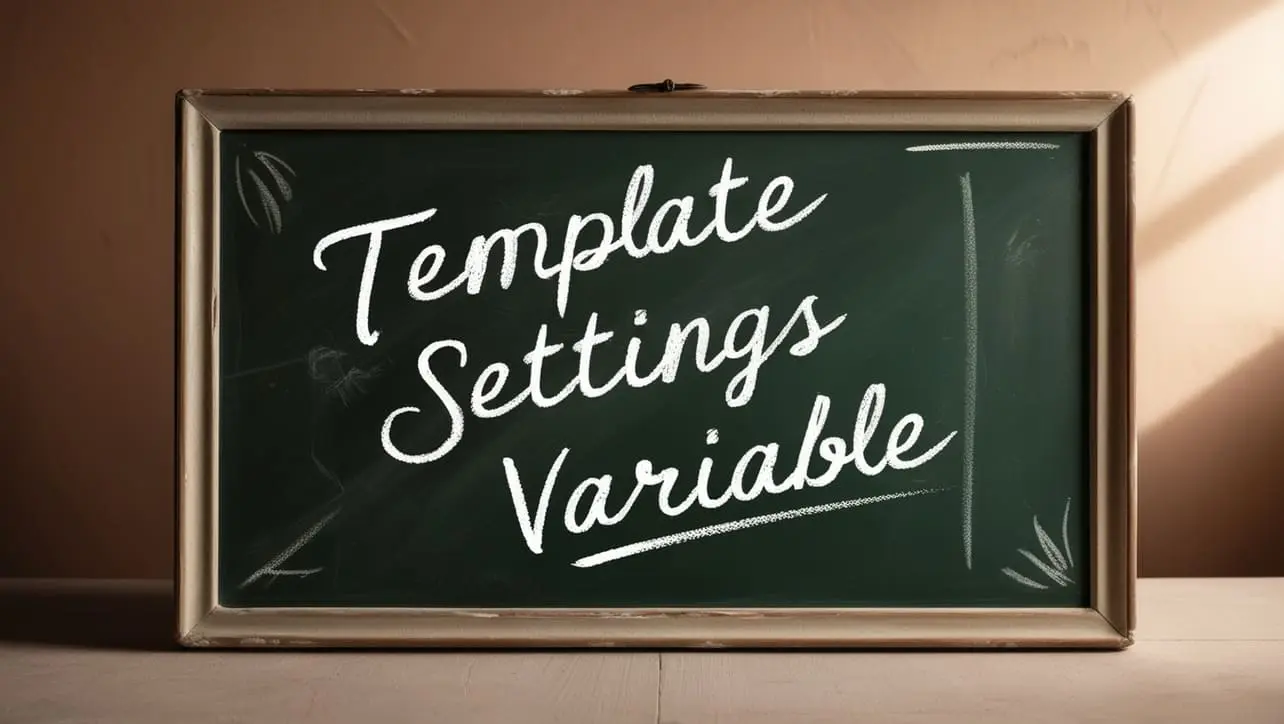
If you have any doubts regarding this article (Lodash _.toArray() Lang Method), please comment here. I will help you immediately.