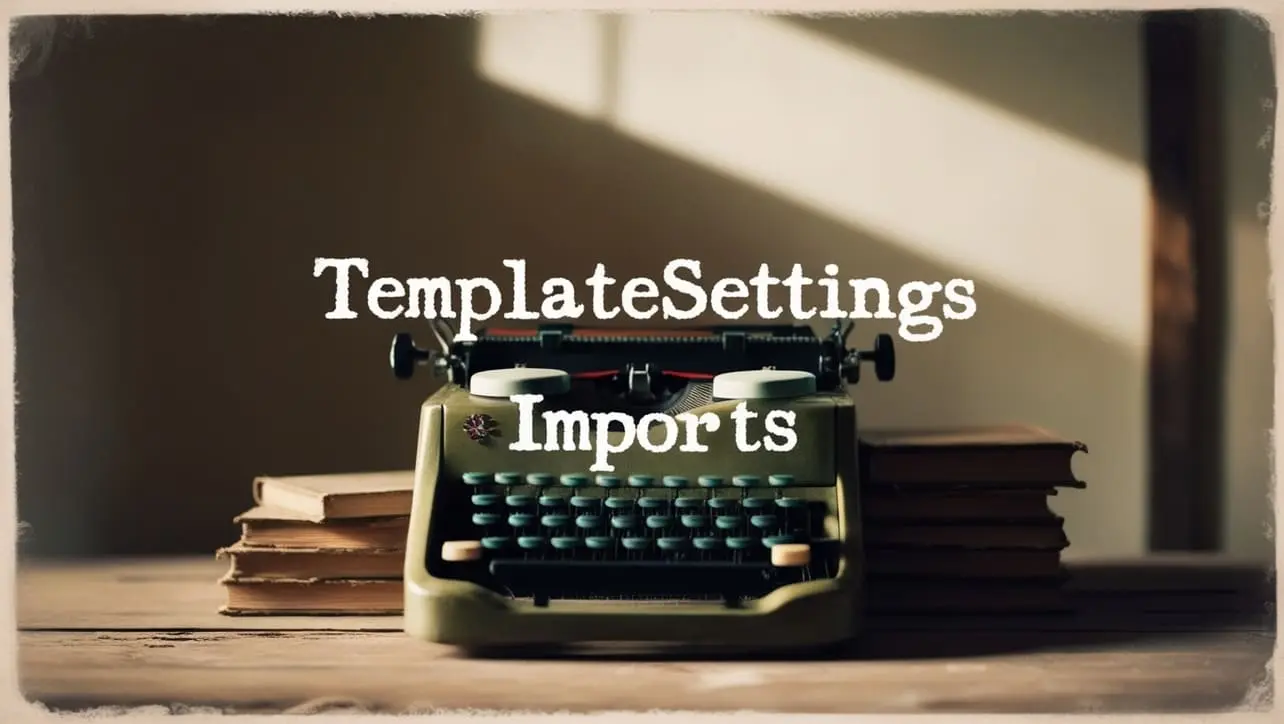
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isWeakMap() Lang Method
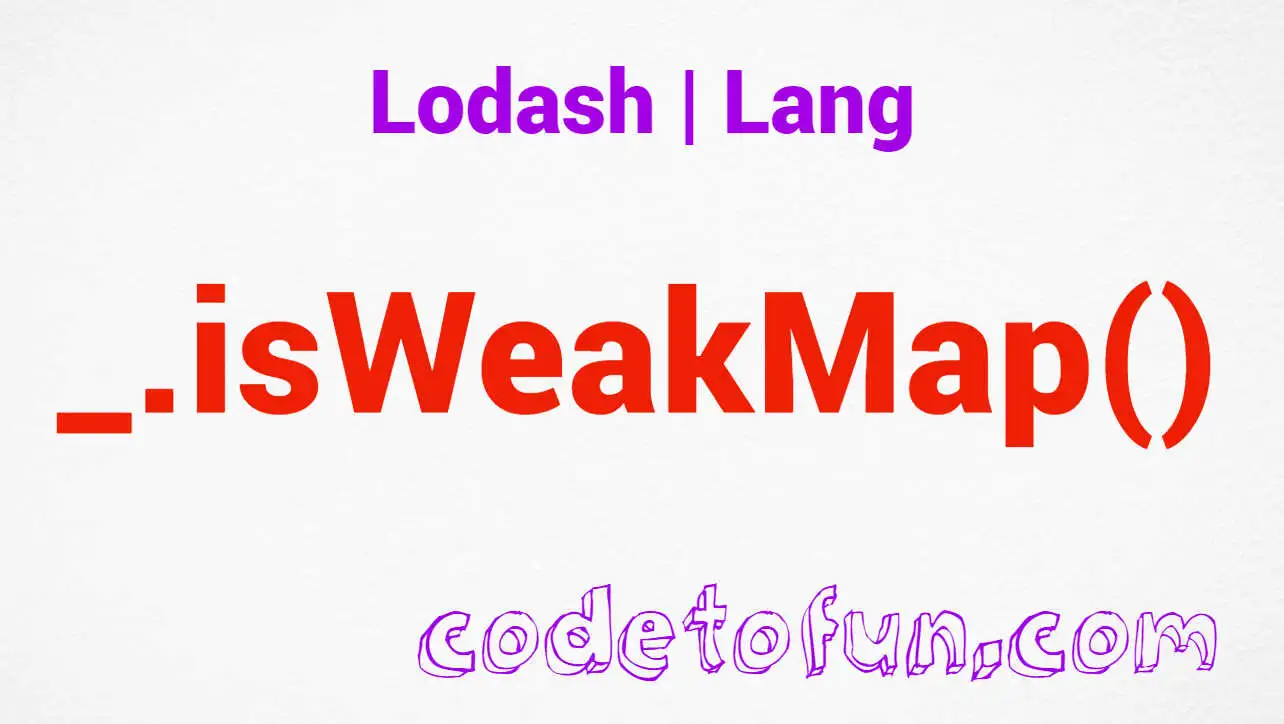
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript programming, robust type checking is essential. Lodash, a powerful utility library, provides various methods to facilitate these checks. Among them is the _.isWeakMap()
method, designed to determine whether a value is a WeakMap object.
This method proves invaluable when dealing with advanced data structures and ensuring the integrity of your code.
🧠 Understanding _.isWeakMap() Method
The _.isWeakMap()
method in Lodash is a type-checking utility that helps identify whether a given value is a WeakMap object. WeakMap is a built-in object in JavaScript that allows for the creation of key-value pairs, with keys being objects and values being arbitrary values. The weak reference nature of WeakMap makes it particularly useful for scenarios where you want to associate data with objects without preventing them from being garbage-collected.
💡 Syntax
The syntax for the _.isWeakMap()
method is straightforward:
_.isWeakMap(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isWeakMap()
method:
const _ = require('lodash');
const weakMap = new WeakMap();
const isWeakMap = _.isWeakMap(weakMap);
console.log(isWeakMap);
// Output: true
In this example, the isWeakMap variable is true because the weakMap is indeed a WeakMap object.
🏆 Best Practices
When working with the _.isWeakMap()
method, consider the following best practices:
Accurate Type Checking:
Use
_.isWeakMap()
for accurate type checking when dealing with variables that may or may not be WeakMap objects. This ensures that your code behaves as expected and avoids potential runtime errors.example.jsCopiedfunction processData(data) { if (_.isWeakMap(data)) { // Process WeakMap data // ... } else { console.error('Invalid data type. Expected a WeakMap.'); } } const myData = /* ... fetch or generate WeakMap ... */ ; processData(myData);
Guard Clauses:
Implement guard clauses using
_.isWeakMap()
to handle specific scenarios gracefully. This can prevent unintended behavior and enhance the overall reliability of your code.example.jsCopiedfunction processUserData(userData) { if (!_.isWeakMap(userData)) { console.error('Invalid user data. Expected a WeakMap.'); return; } // Continue processing user data // ... } const userPreferences = /* ... fetch or generate WeakMap ... */ ; processUserData(userPreferences);
📚 Use Cases
Type Validation in Functions:
Utilize
_.isWeakMap()
to perform type validation within functions, ensuring that the expected data types are received.example.jsCopiedfunction processConfig(config) { if (!_.isWeakMap(config)) { console.error('Invalid configuration. Expected a WeakMap.'); return; } // Process configuration data // ... } const appConfig = /* ... fetch or generate WeakMap ... */ ; processConfig(appConfig);
Garbage Collection Awareness:
Use
_.isWeakMap()
to be aware of whether a given value is a WeakMap, especially in scenarios where garbage collection and memory management are critical.example.jsCopiedfunction trackResource(resource) { if (_.isWeakMap(resource)) { // Track the resource using WeakMap // ... } else { console.warn('Resource tracking skipped. WeakMap expected.'); } } const monitoredResource = /* ... fetch or generate WeakMap ... */ ; trackResource(monitoredResource);
🎉 Conclusion
The _.isWeakMap()
method in Lodash provides a reliable means of identifying whether a given value is a WeakMap object. Whether you're performing type checks, implementing guard clauses, or ensuring proper memory management, this method proves to be a valuable asset in the toolkit of any JavaScript developer.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isWeakMap()
method in your Lodash projects.
👨💻 Join our Community:
Author
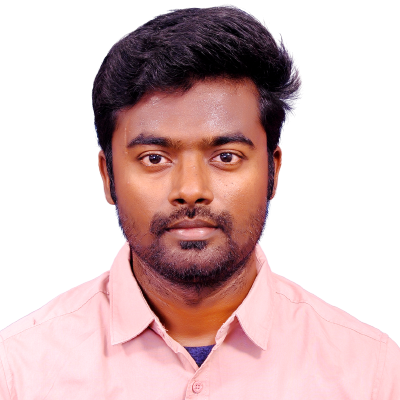
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
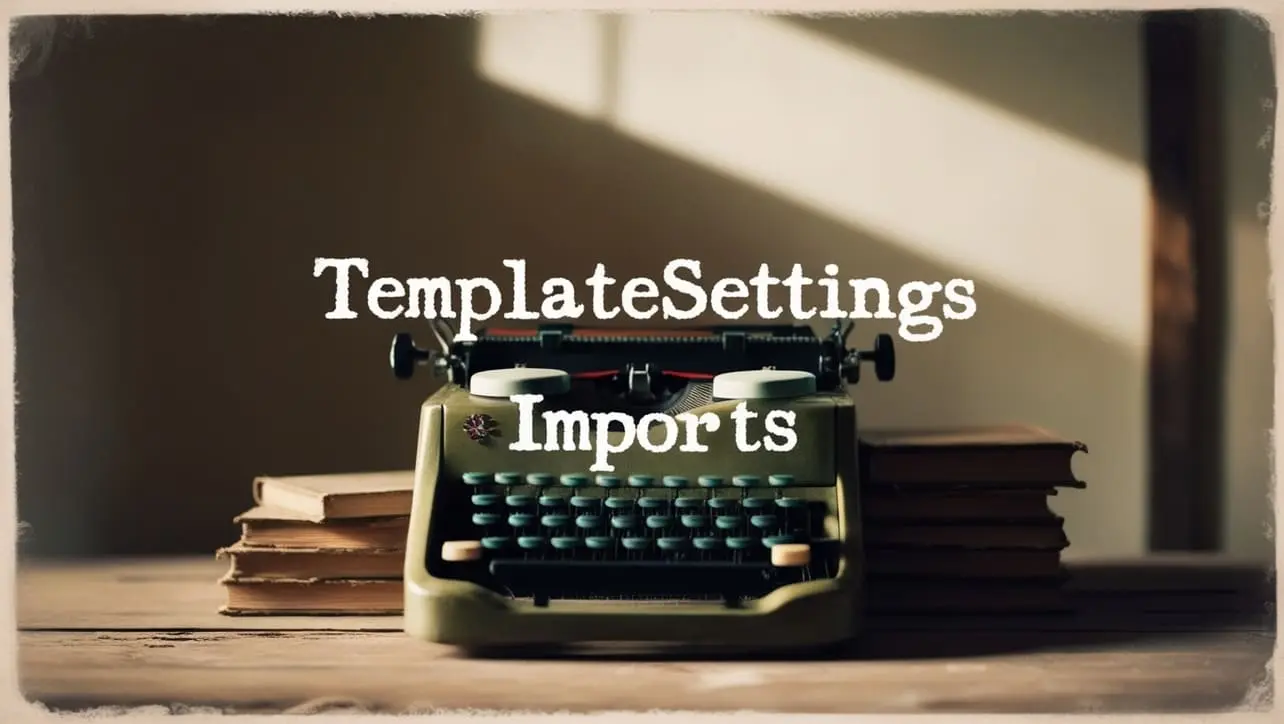
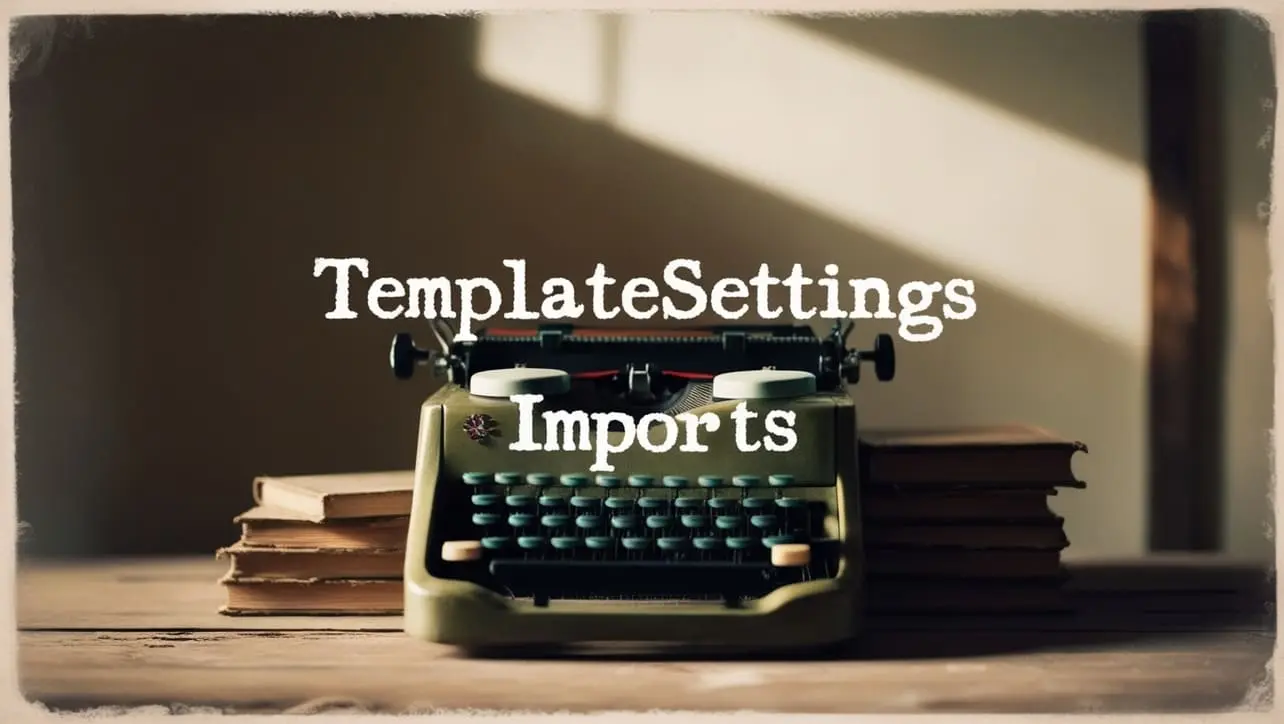
Lodash _.templateSettings.imports Property
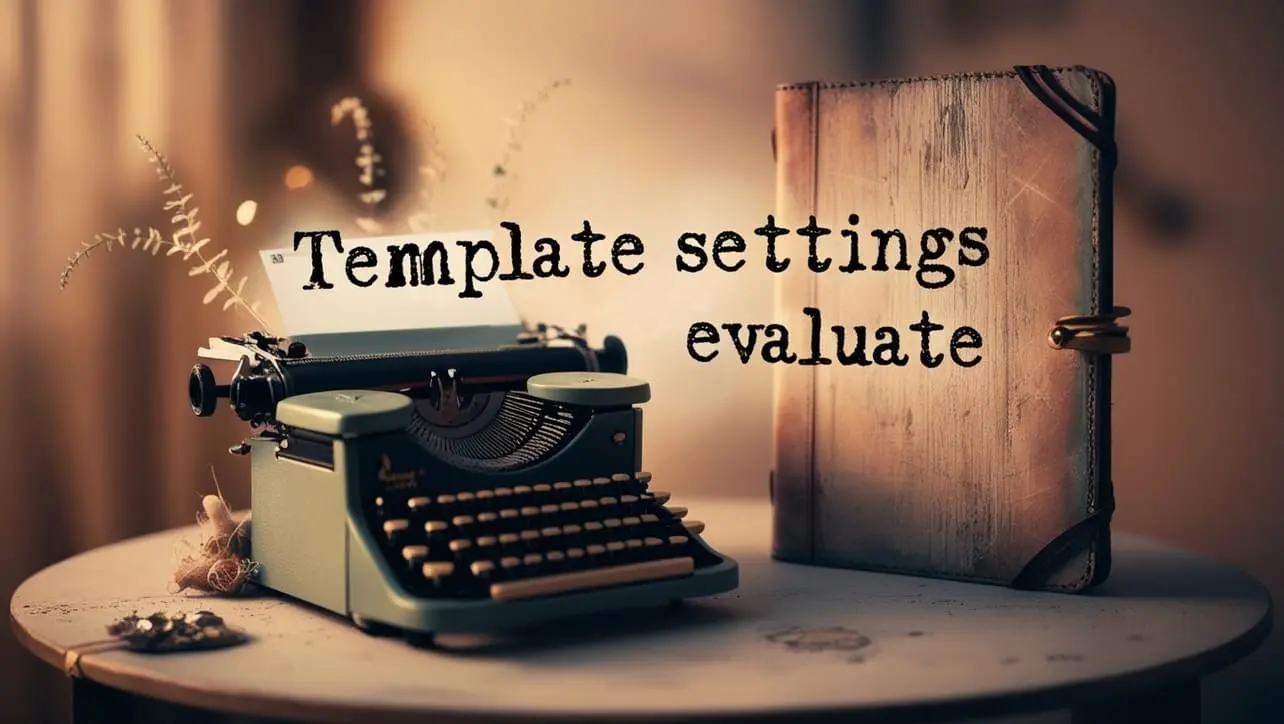
Lodash _.templateSettings.evaluate Property
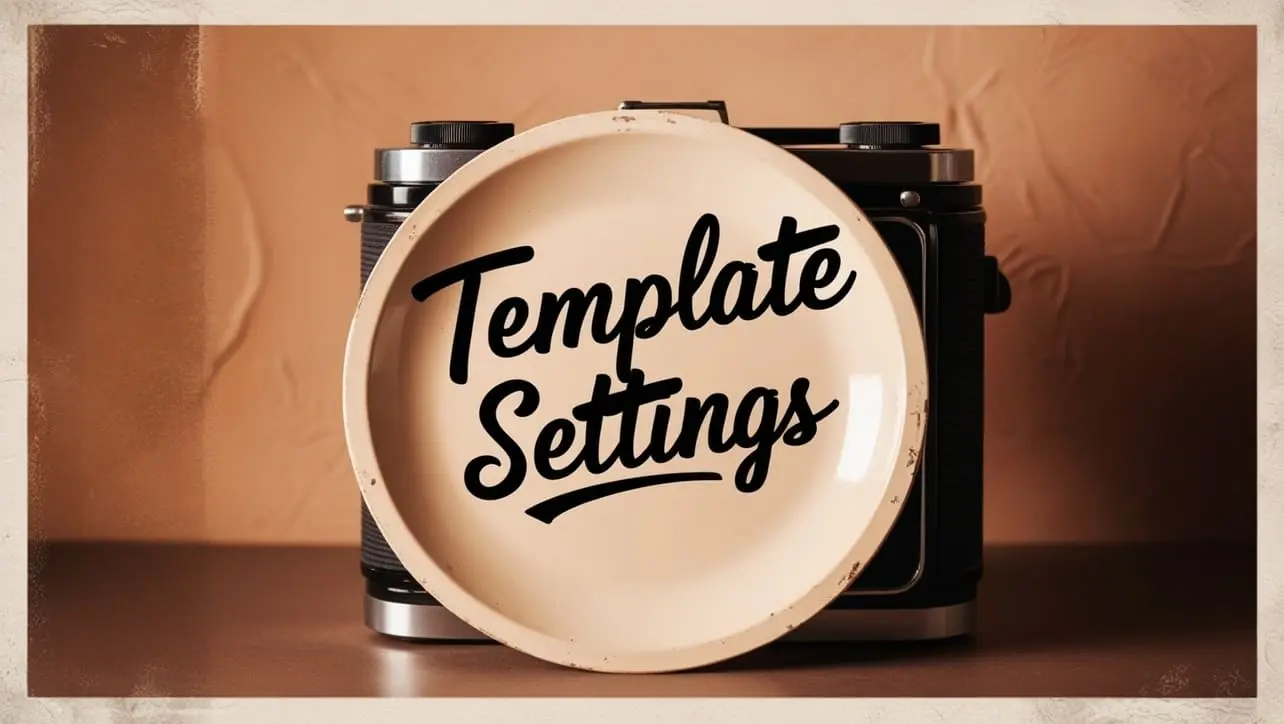
Lodash _.templateSettings Property
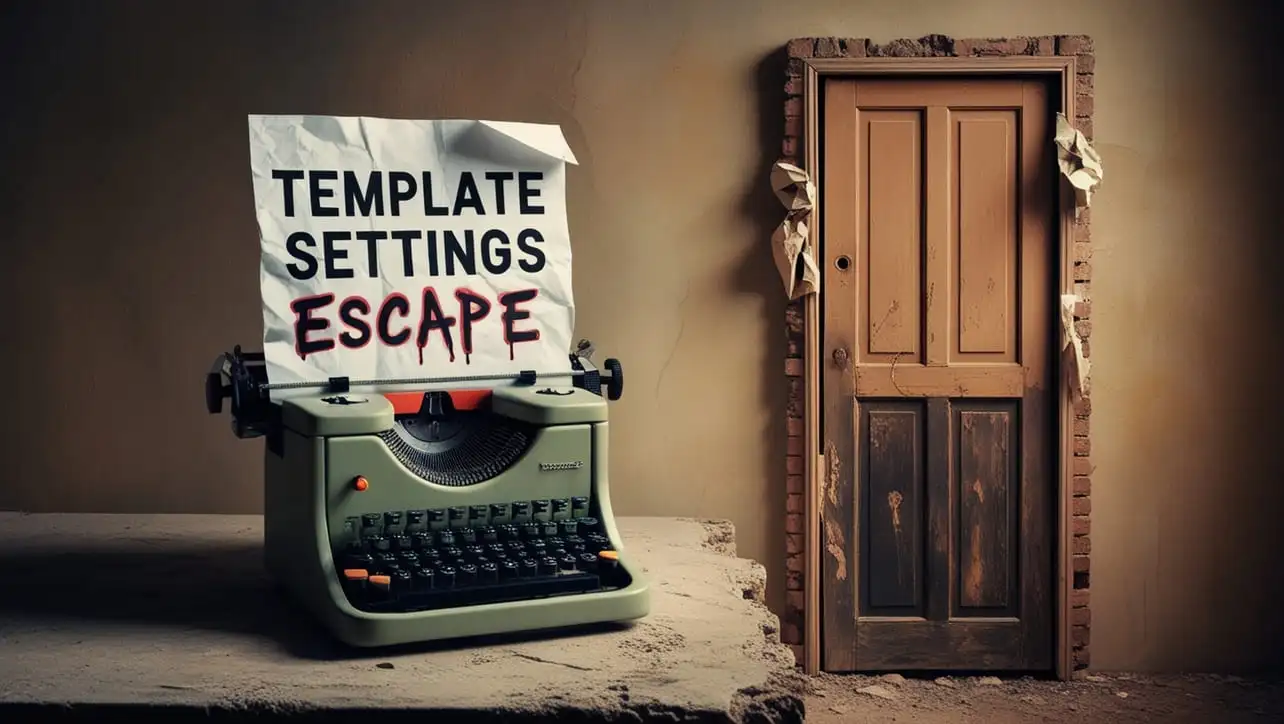
Lodash _.templateSettings.escape Property
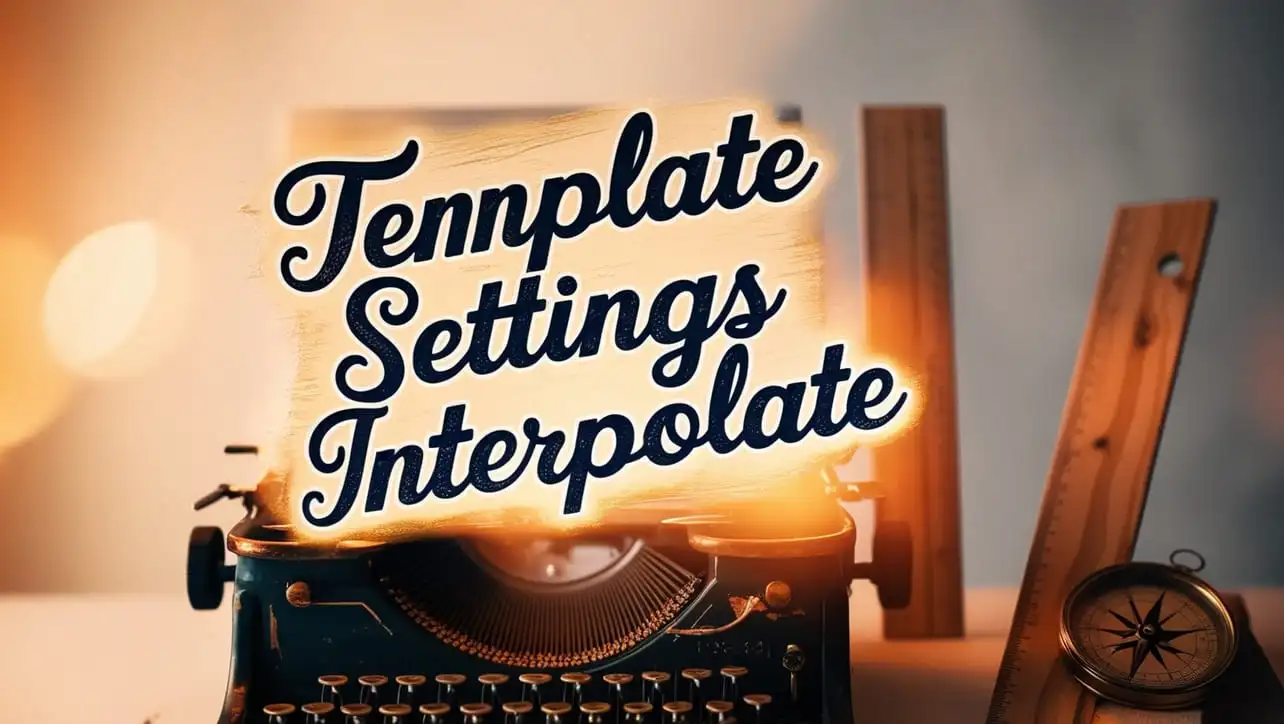
Lodash _.templateSettings.interpolate Property
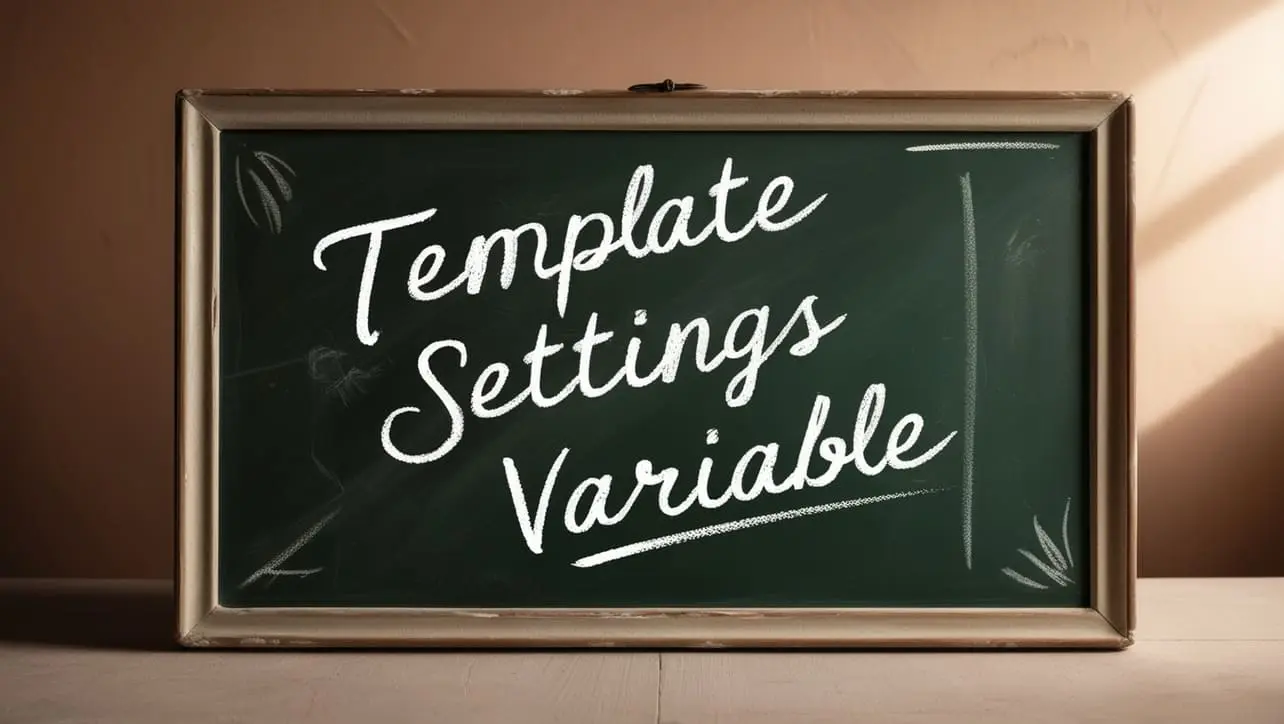
If you have any doubts regarding this article (Lodash _.isWeakMap() Lang Method), please comment here. I will help you immediately.