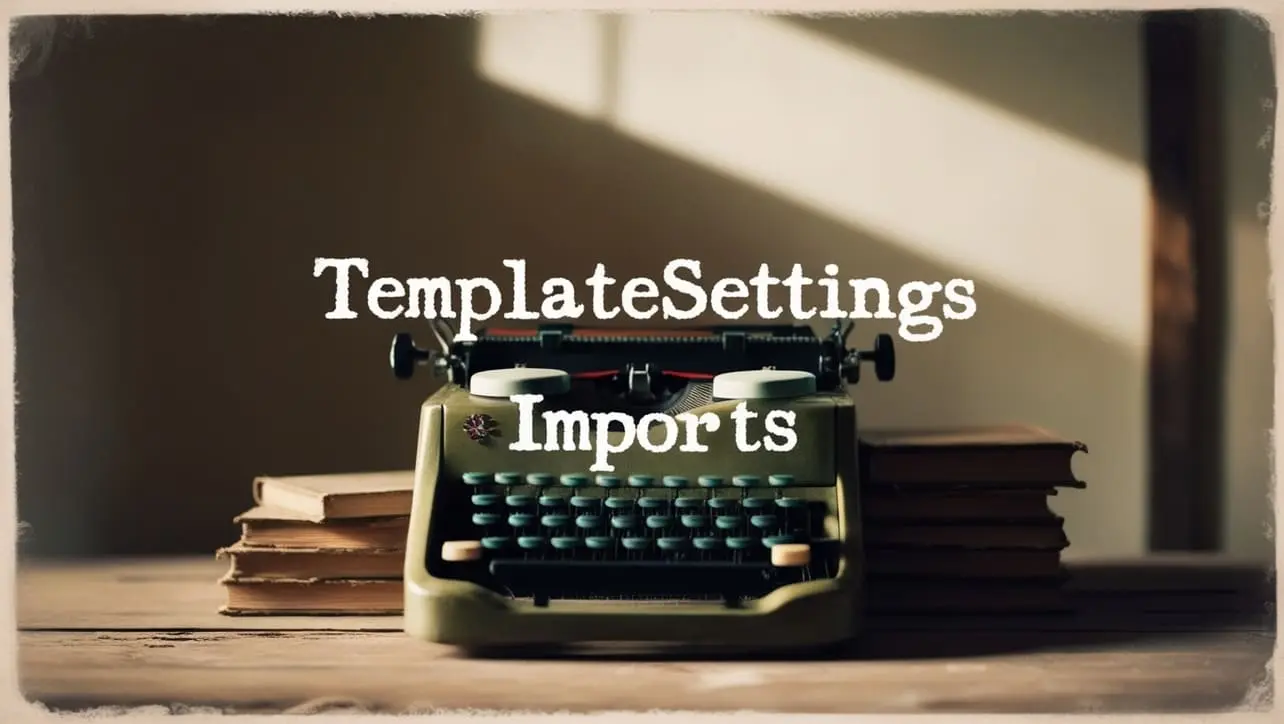
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isSymbol() Lang Method
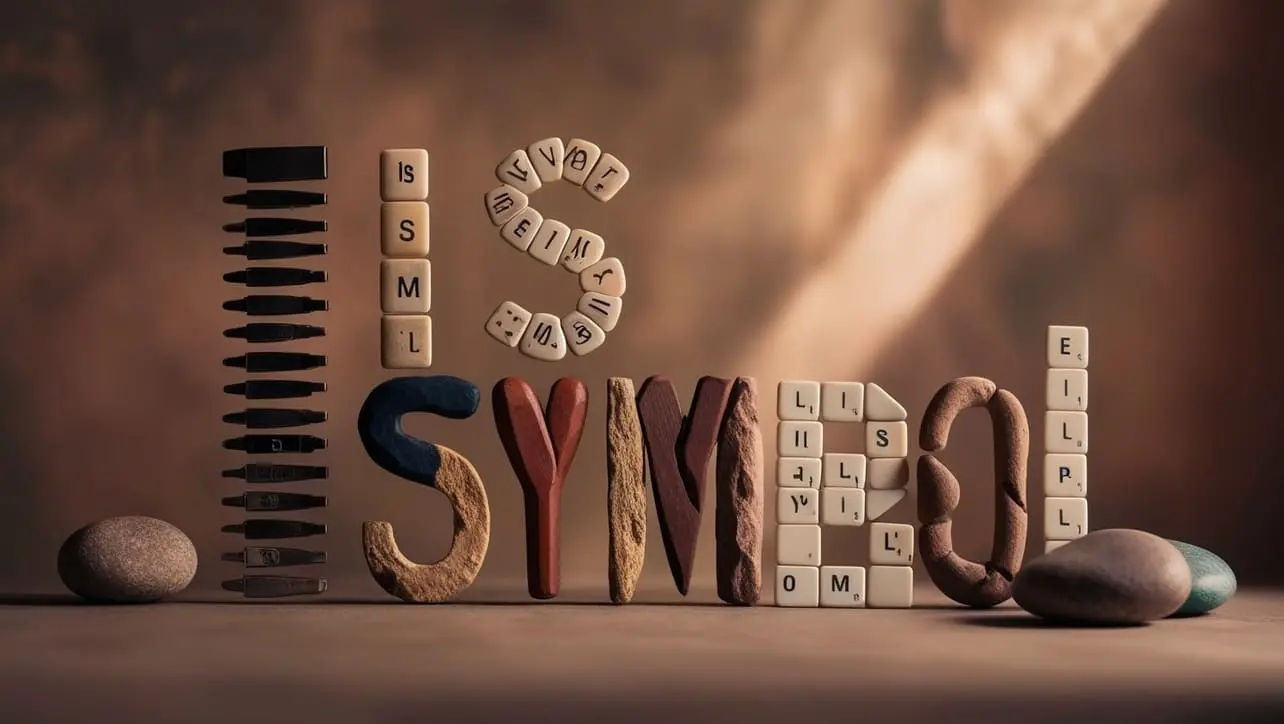
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, accurately identifying data types is crucial for writing robust and error-resistant code. Lodash, a versatile utility library, provides a variety of functions for efficient data manipulation. Among these functions is _.isSymbol()
, a Lang method designed to determine whether a given value is a Symbol.
This method proves invaluable when working with dynamic and heterogeneous data in JavaScript.
🧠 Understanding _.isSymbol() Method
The _.isSymbol()
method in Lodash checks if a given value is a Symbol data type. Symbols are unique and immutable primitive values often used as identifiers in object properties. This method simplifies the process of validating whether a value is specifically a Symbol.
💡 Syntax
The syntax for the _.isSymbol()
method is straightforward:
_.isSymbol(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isSymbol()
method:
const _ = require('lodash');
const symbolValue = Symbol('example');
const stringValue = 'example';
console.log(_.isSymbol(symbolValue)); // Output: true
console.log(_.isSymbol(stringValue)); // Output: false
In this example, _.isSymbol()
correctly identifies symbolValue as a Symbol and stringValue as not a Symbol.
🏆 Best Practices
When working with the _.isSymbol()
method, consider the following best practices:
Validate Symbol Type:
Ensure that you are specifically dealing with Symbols when using
_.isSymbol()
. This method is designed to identify Symbols, and using it with other data types may lead to unexpected results.example.jsCopiedconst valueToCheck = /* ...retrieve a value... */ ; if (_.isSymbol(valueToCheck)) { // Handle the case where the value is a Symbol console.log('The value is a Symbol.'); } else { // Handle the case where the value is not a Symbol console.log('The value is not a Symbol.'); }
Combine with Type Checks:
Combine
_.isSymbol()
with other type-checking methods to create more comprehensive validation logic.example.jsCopiedconst valueToCheck = /* ...retrieve a value... */ ; if (_.isSymbol(valueToCheck)) { // Handle the case where the value is a Symbol console.log('The value is a Symbol.'); } else if (_.isString(valueToCheck)) { // Handle the case where the value is a String console.log('The value is a String.'); } else { // Handle other cases console.log('The value is neither a Symbol nor a String.'); }
Use in Conditional Statements:
Leverage
_.isSymbol()
in conditional statements to control the flow of your code based on the data type.example.jsCopiedconst valueToCheck = /* ...retrieve a value... */ ; if (_.isSymbol(valueToCheck)) { // Perform actions specific to Symbols console.log('Processing Symbol data...'); } else { // Handle other cases console.log('Invalid data type.'); }
📚 Use Cases
Object Property Identification:
When working with objects that use Symbols as property identifiers,
_.isSymbol()
can be employed to verify the data type before accessing or manipulating those properties.example.jsCopiedconst obj = { [Symbol('property')]: 'value', regularProperty: 'another value', }; for (const key in obj) { if (_.isSymbol(key)) { console.log('Symbol property found:', key); } else { console.log('Regular property found:', key); } }
Custom Type Checking:
In scenarios where you need to perform custom type checking within your application,
_.isSymbol()
can play a crucial role.example.jsCopiedfunction processData(value) { if (_.isSymbol(value)) { // Process Symbol data console.log('Processing Symbol data...'); } else { // Handle other cases console.log('Invalid data type.'); } } const symbolValue = Symbol('example'); processData(symbolValue); const stringValue = 'example'; processData(stringValue);
Dynamic Code Paths:
Use
_.isSymbol()
to create dynamic code paths based on the data type of a given value.example.jsCopiedfunction processValue(value) { if (_.isSymbol(value)) { console.log('Processing Symbol data...'); } else { console.log('Processing other data types...'); } } const dynamicValue = /* ...retrieve a dynamic value... */ ; processValue(dynamicValue);
🎉 Conclusion
The _.isSymbol()
method in Lodash provides a reliable way to identify Symbol data types in JavaScript. Whether you're working with object properties, performing custom type checks, or dynamically handling different data types, _.isSymbol()
can enhance the precision and reliability of your code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isSymbol()
method in your Lodash projects.
👨💻 Join our Community:
Author
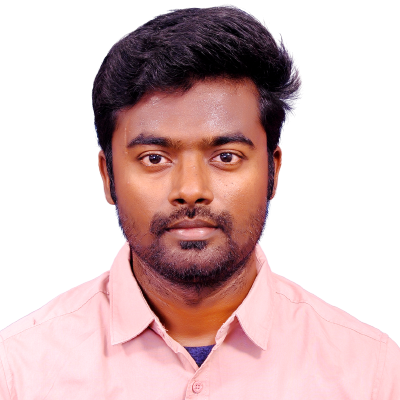
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
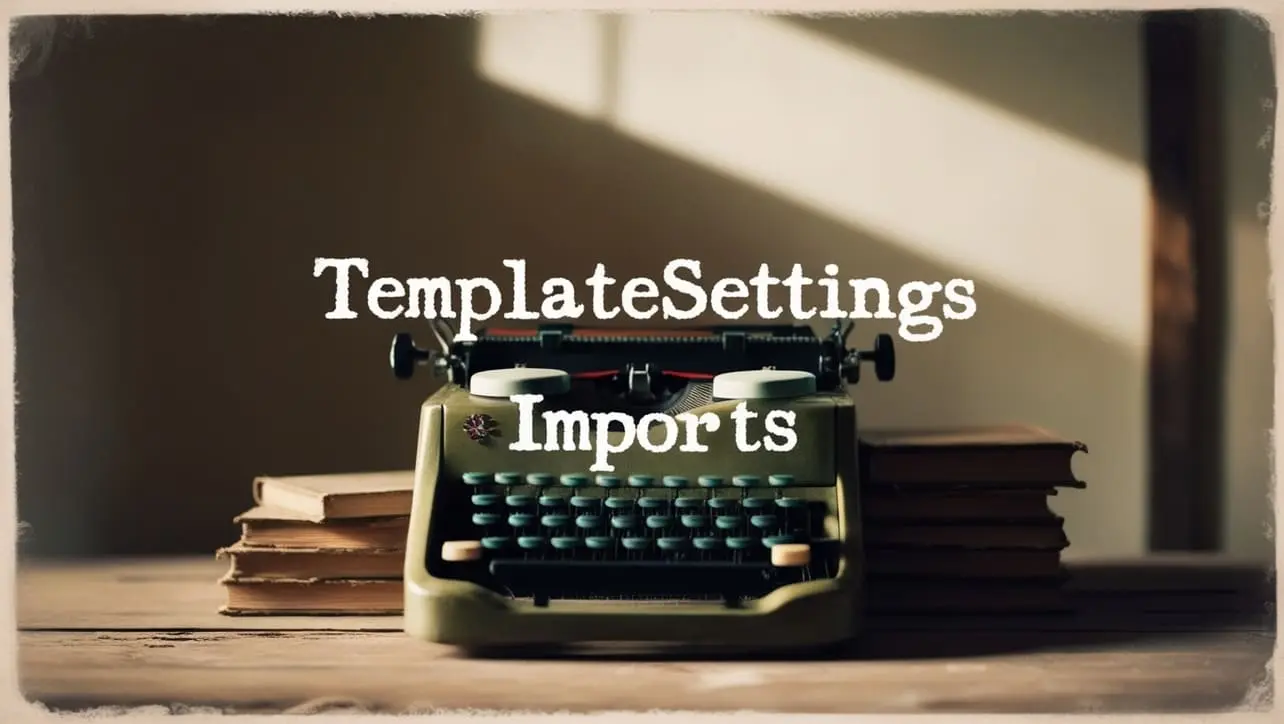
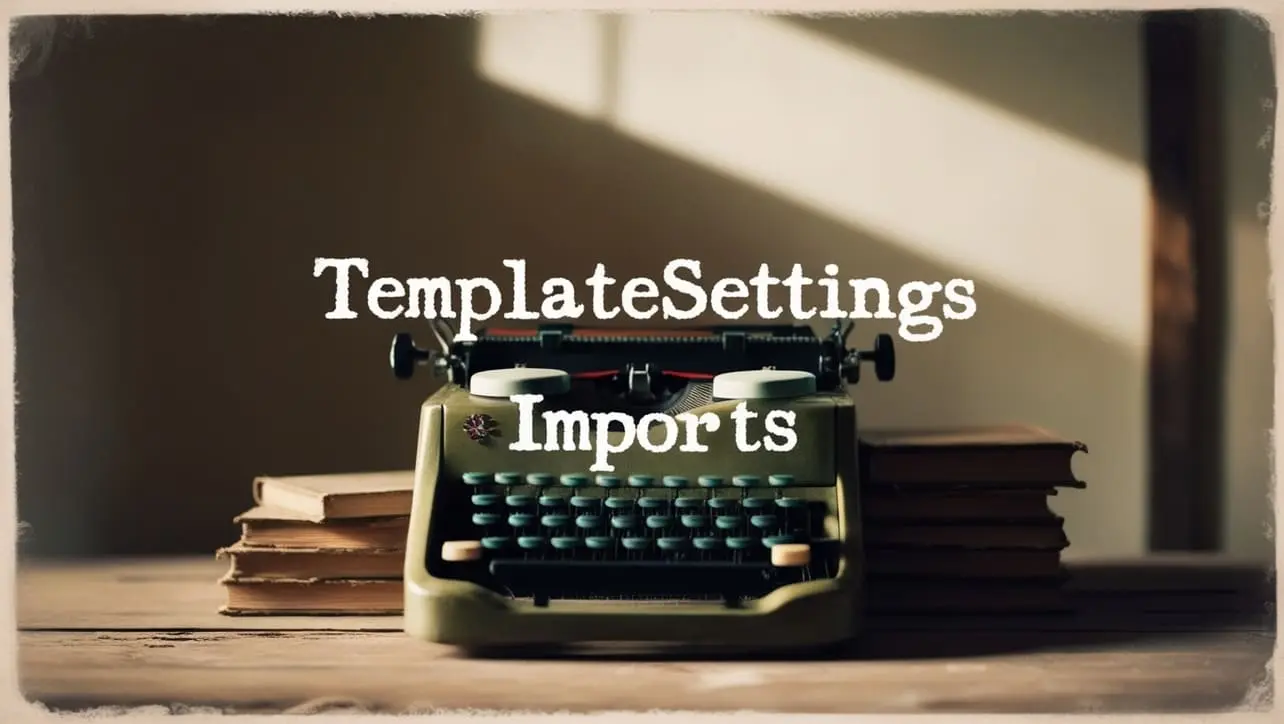
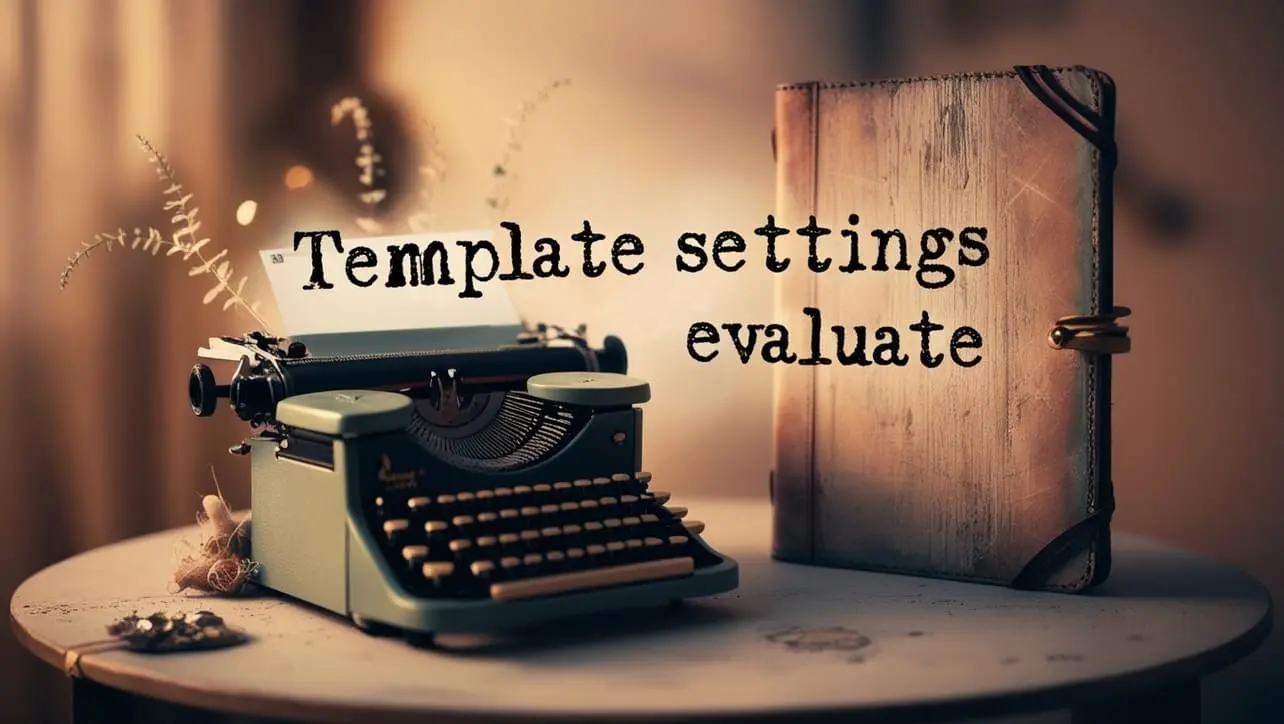
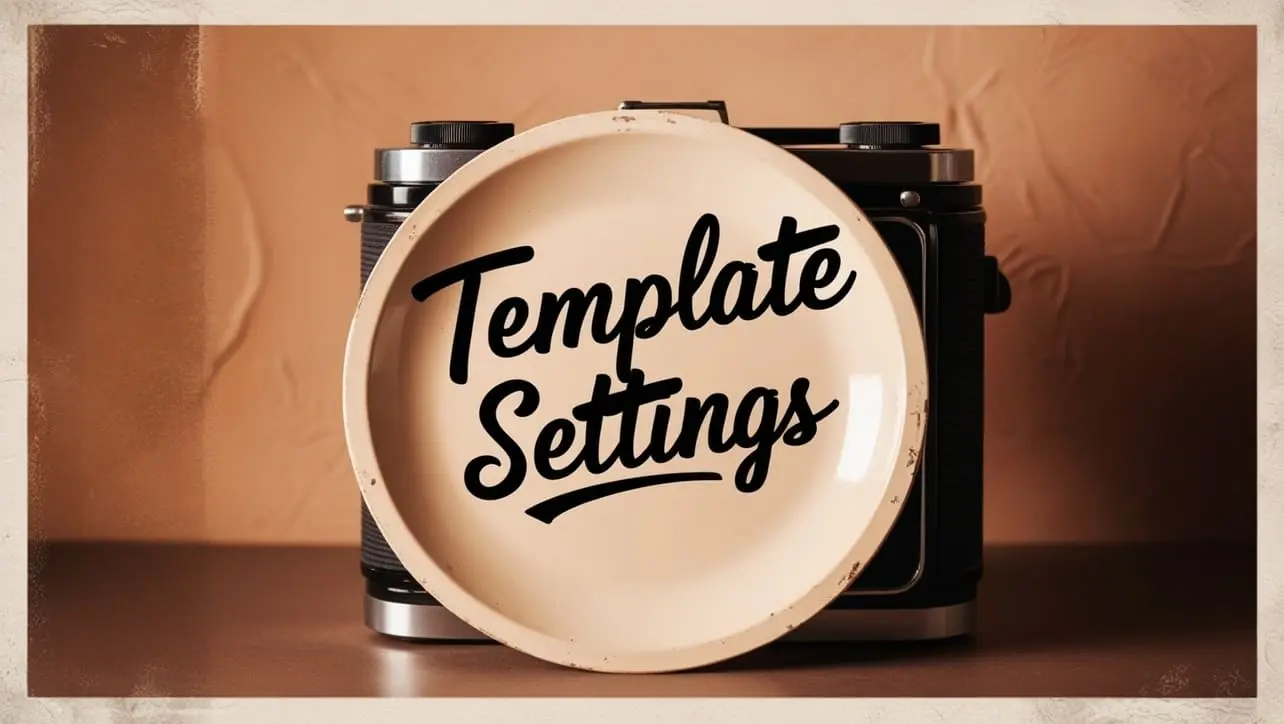
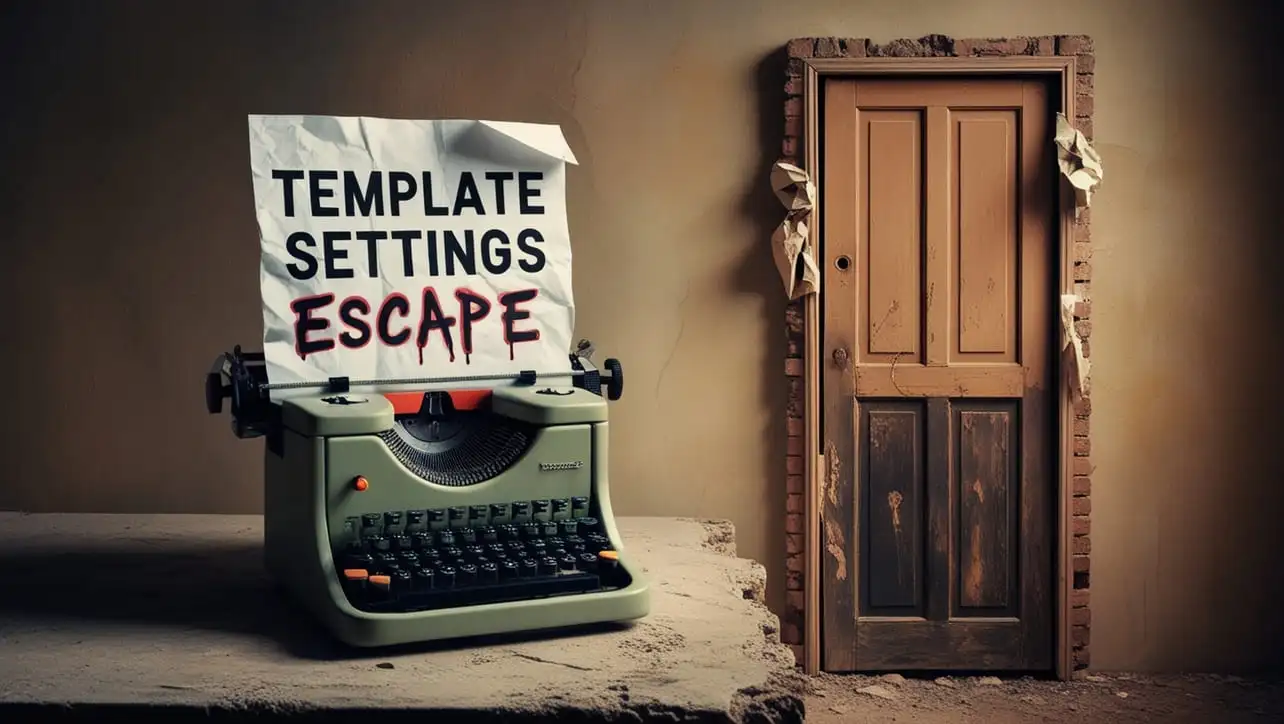
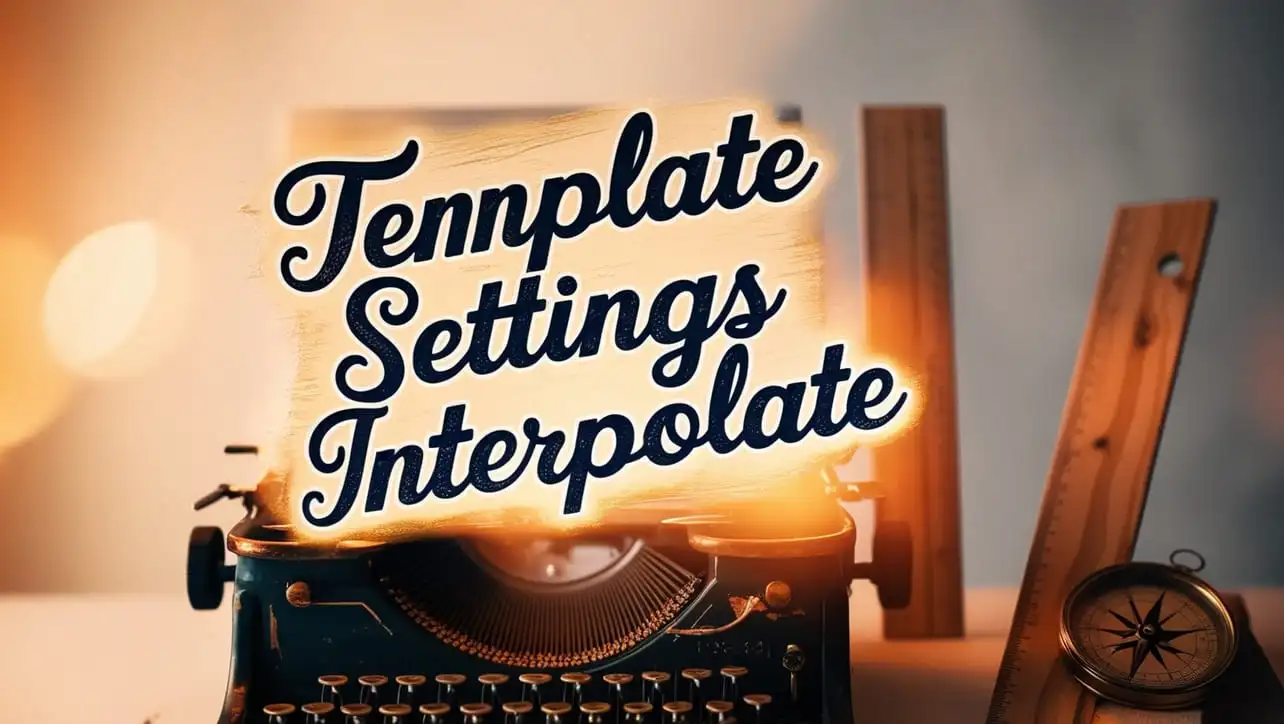
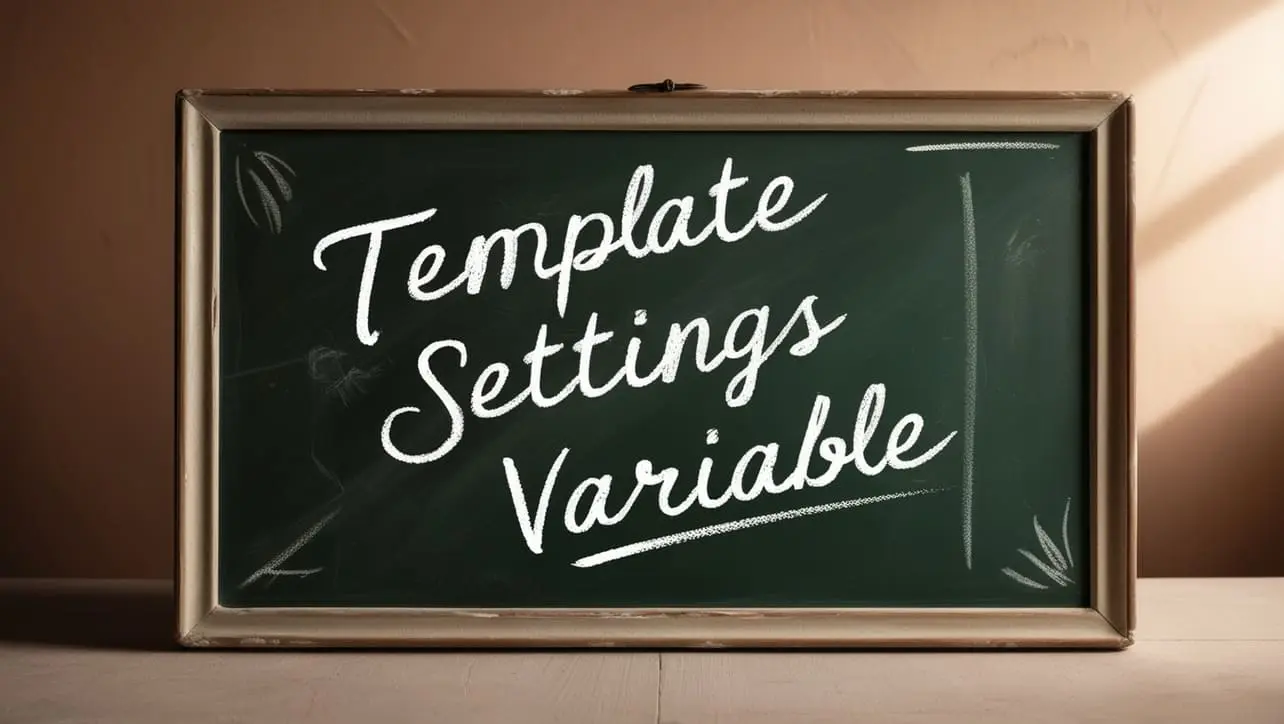
If you have any doubts regarding this article (Lodash _.isSymbol() Lang Method), please comment here. I will help you immediately.