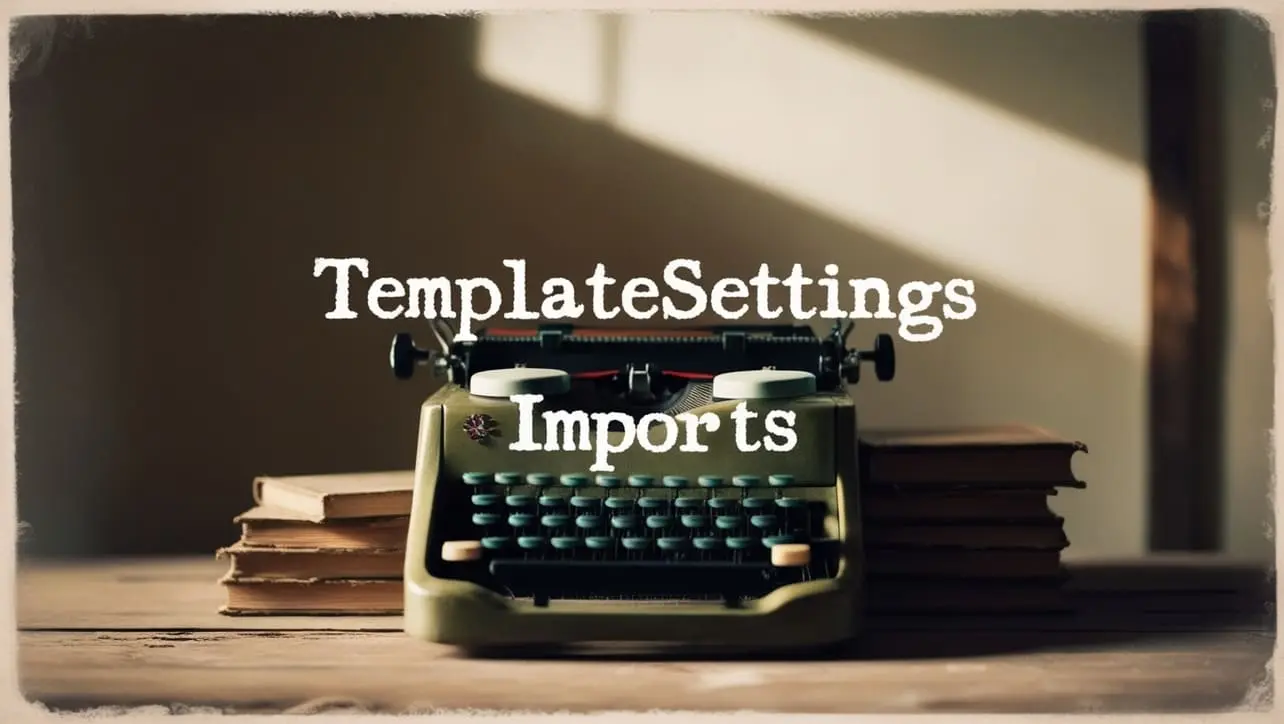
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isSet() Lang Method
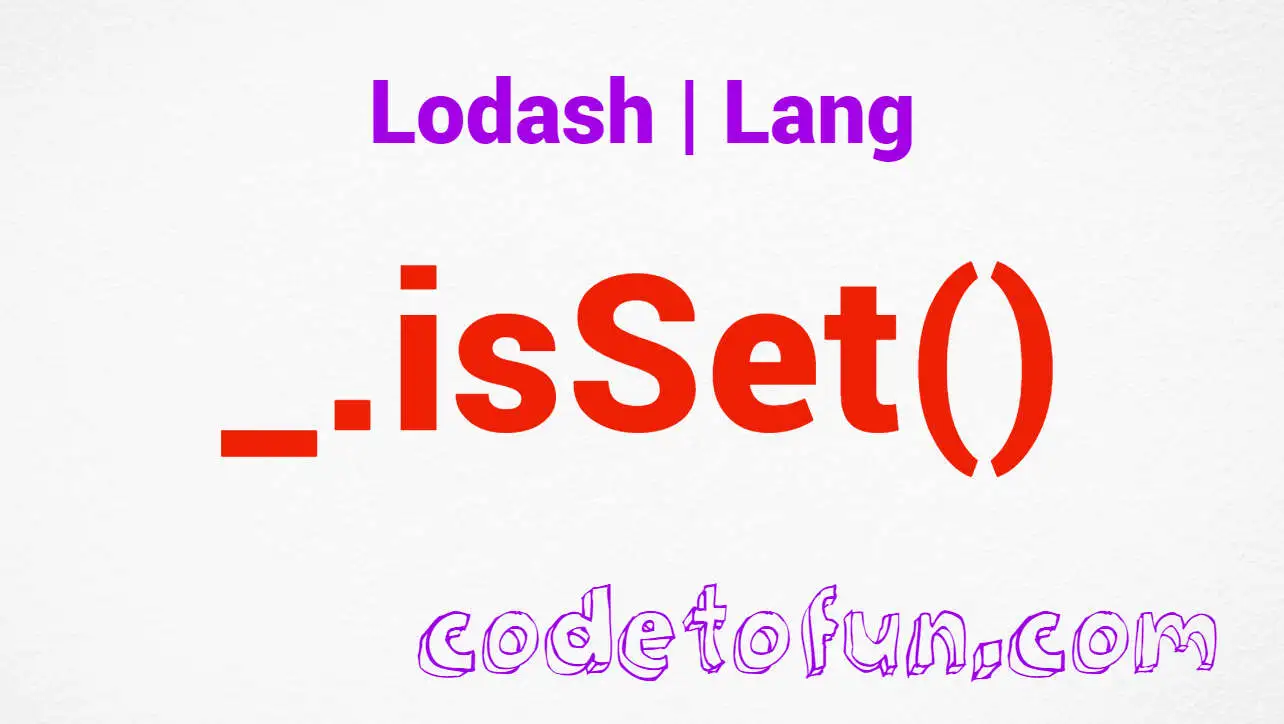
Photo Credit to CodeToFun
🙋 Introduction
In the expansive landscape of JavaScript development, effective type checking is essential. Lodash, a versatile utility library, provides a variety of functions to simplify common programming tasks. Among these functions is the _.isSet()
method, a valuable tool for checking whether a value is of the Set data type.
This method contributes to code reliability and clarity, aiding developers in creating robust applications.
🧠 Understanding _.isSet() Method
The _.isSet()
method in Lodash serves as a dedicated utility for determining whether a given value is a Set. Sets are a built-in object type in JavaScript introduced in ECMAScript 2015 (ES6), and they provide a collection of unique values. The _.isSet()
method simplifies the process of validating whether a value conforms to this specific data structure.
💡 Syntax
The syntax for the _.isSet()
method is straightforward:
_.isSet(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isSet()
method:
const _ = require('lodash');
const setInstance = new Set([1, 2, 3]);
const isArraySet = _.isSet(setInstance);
console.log(isArraySet);
// Output: true
In this example, the setInstance is a valid Set, and _.isSet()
returns true accordingly.
🏆 Best Practices
When working with the _.isSet()
method, consider the following best practices:
Clarify Type Intent:
Use
_.isSet()
when you specifically want to check whether a value is a Set. This enhances code readability and clarifies the developer's intent in scenarios where different data types might be involved.example.jsCopiedconst potentialSet = new Set([4, 5, 6]); if (_.isSet(potentialSet)) { // Perform operations specific to Set console.log('It is a Set!'); } else { console.log('It is not a Set.'); }
Combine with Conditional Logic:
Integrate
_.isSet()
within conditional logic to handle different cases based on the type of the value. This ensures that your application responds appropriately to varying data structures.example.jsCopiedfunction processValue(value) { if (_.isSet(value)) { // Handle Set-specific logic console.log('Processing Set:', value); } else { console.log('Unsupported data type.'); } } const dataSet = new Set([7, 8, 9]); processValue(dataSet); const nonSetData = [10, 11, 12]; processValue(nonSetData);
Consider Type Coercion:
Be aware of type coercion when using
_.isSet()
. If a value can be coerced into a Set, the method will return true. Ensure that this behavior aligns with your expectations.example.jsCopiedconst coercedSet = _.isSet(new Set('123')); console.log(coercedSet); // Output: true
📚 Use Cases
Validating Function Parameters:
When designing functions that expect Set instances as parameters,
_.isSet()
can be used to validate the input, preventing unexpected data types from causing issues within the function.example.jsCopiedfunction processSet(inputSet) { if (_.isSet(inputSet)) { // Perform Set-specific operations console.log('Processing Set:', inputSet); } else { console.error('Invalid input. Expected a Set.'); } } const validSet = new Set([13, 14, 15]); processSet(validSet); const invalidInput = [16, 17, 18]; processSet(invalidInput);
Enhancing Type-Safe Operations:
By incorporating
_.isSet()
in scenarios where Set-specific operations are required, you can ensure type safety and prevent potential runtime errors.example.jsCopiedfunction performSetOperation(setA, setB) { if (_.isSet(setA) && _.isSet(setB)) { // Perform operations like union, intersection, etc. const unionResult = new Set([...setA, ...setB]); console.log('Union Result:', unionResult); } else { console.error('Invalid input. Expected Sets.'); } } const set1 = new Set([19, 20, 21]); const set2 = new Set([20, 21, 22]); performSetOperation(set1, set2); const nonSetInput = [23, 24, 25]; performSetOperation(set1, nonSetInput);
Data Processing Pipelines:
In data processing pipelines,
_.isSet()
can be utilized to filter out values that are not Sets, ensuring that subsequent operations are performed only on valid data.example.jsCopiedconst inputData = [new Set([26, 27, 28]), 'invalid', new Set([29, 30])]; const validSets = inputData.filter(value => _.isSet(value)); console.log('Valid Sets:', validSets);
🎉 Conclusion
The _.isSet()
method in Lodash provides a concise and reliable solution for determining whether a value is of the Set data type. By incorporating this method into your code, you enhance the clarity of your type-checking logic and contribute to the overall robustness of your JavaScript applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isSet()
method in your Lodash projects.
👨💻 Join our Community:
Author
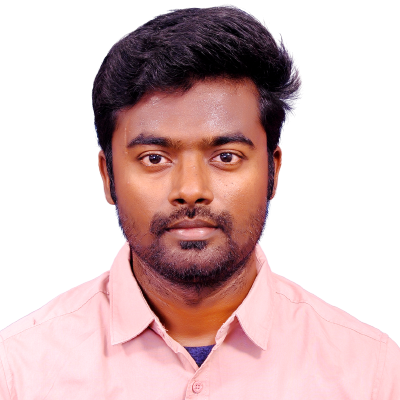
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
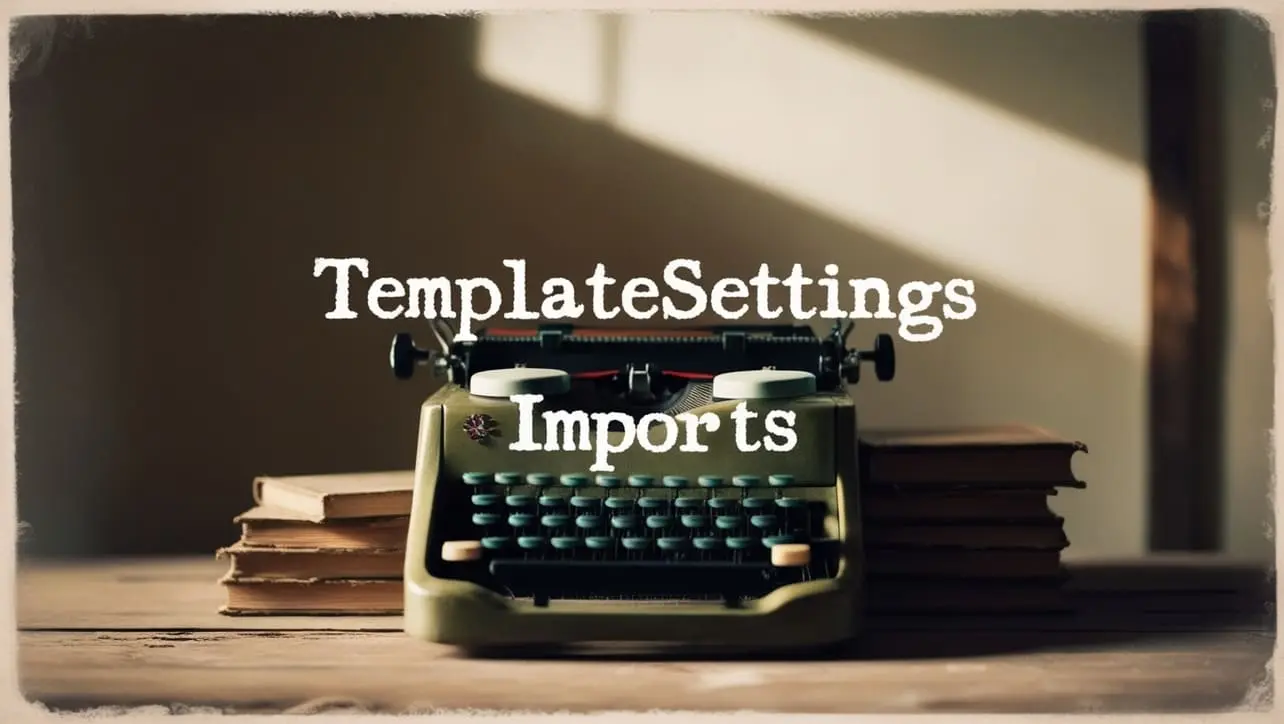
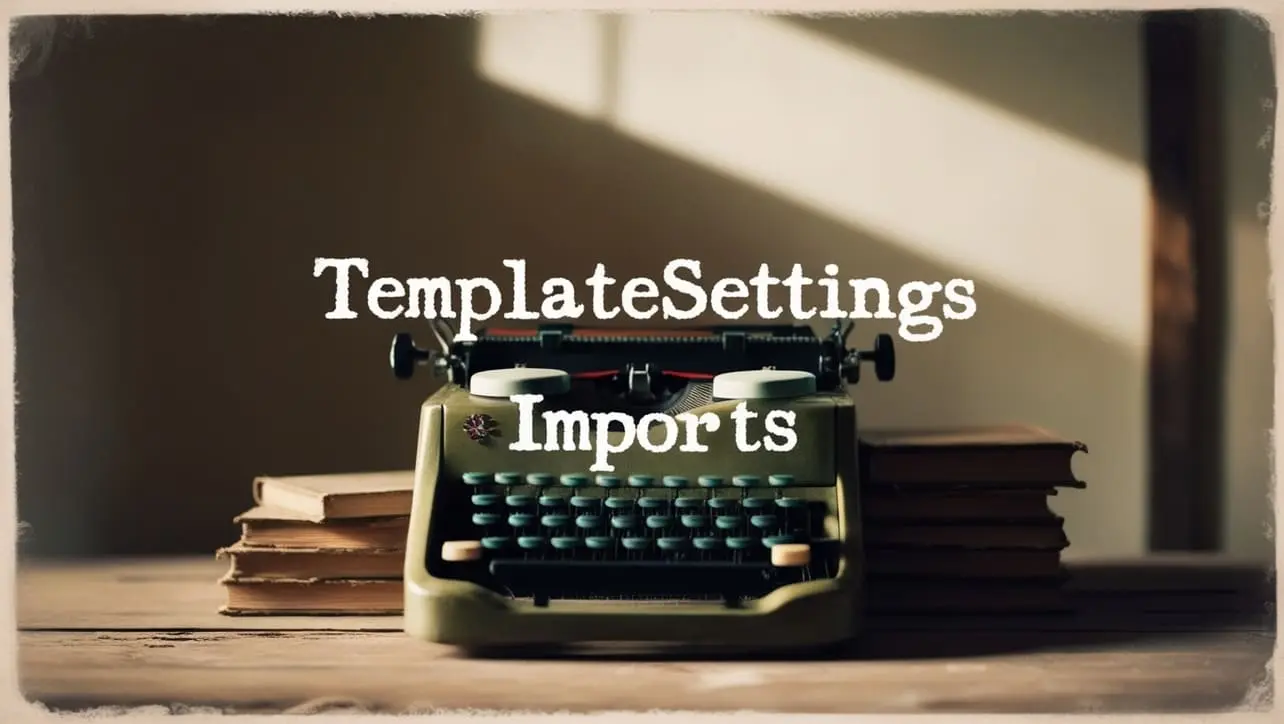
Lodash _.templateSettings.imports Property
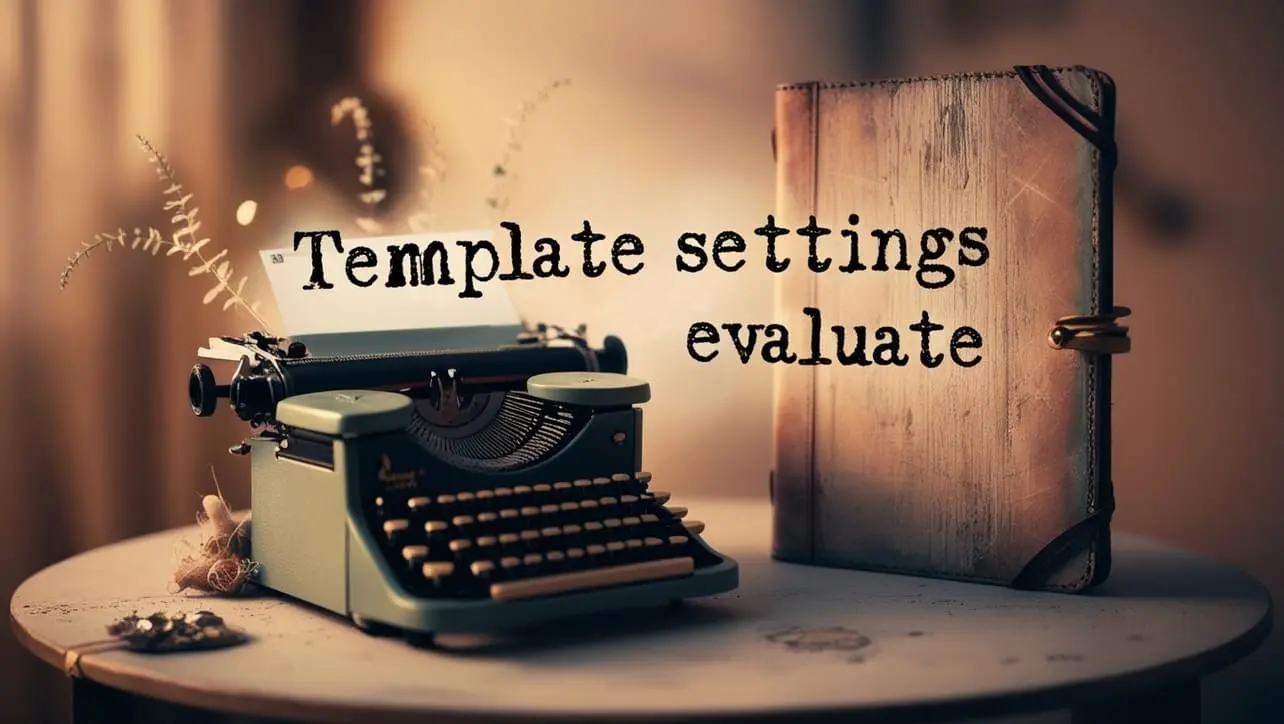
Lodash _.templateSettings.evaluate Property
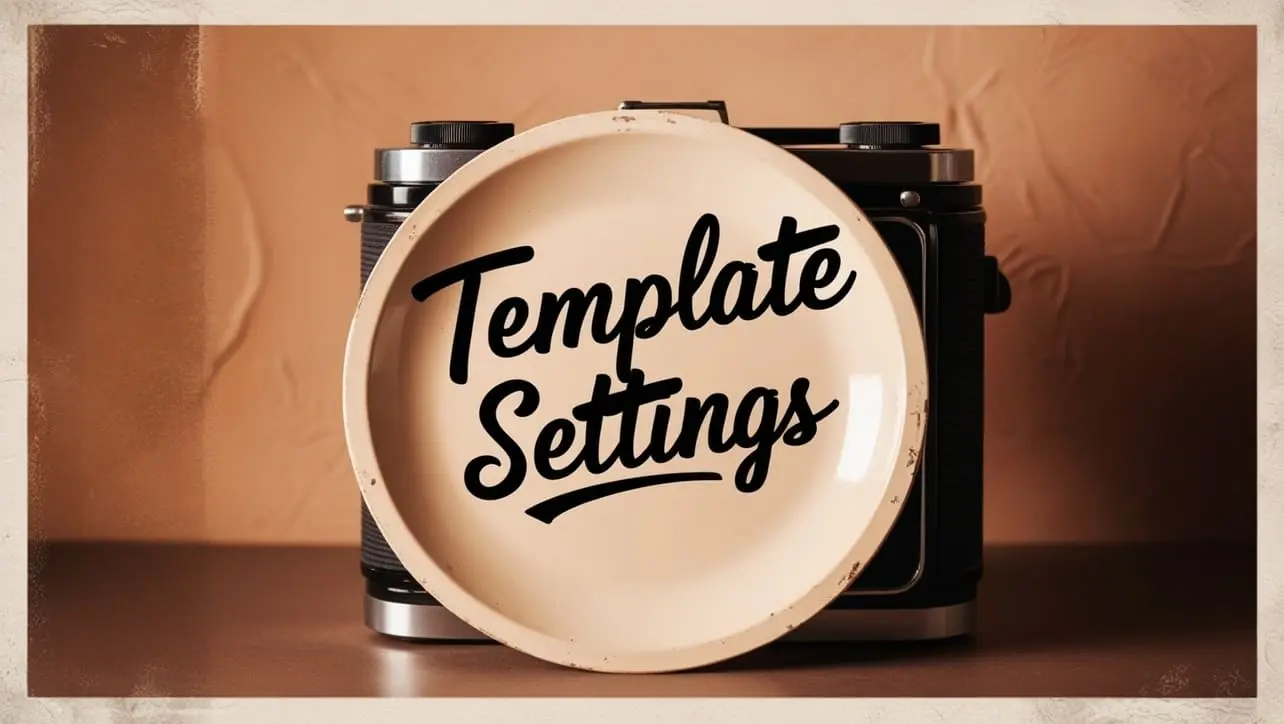
Lodash _.templateSettings Property
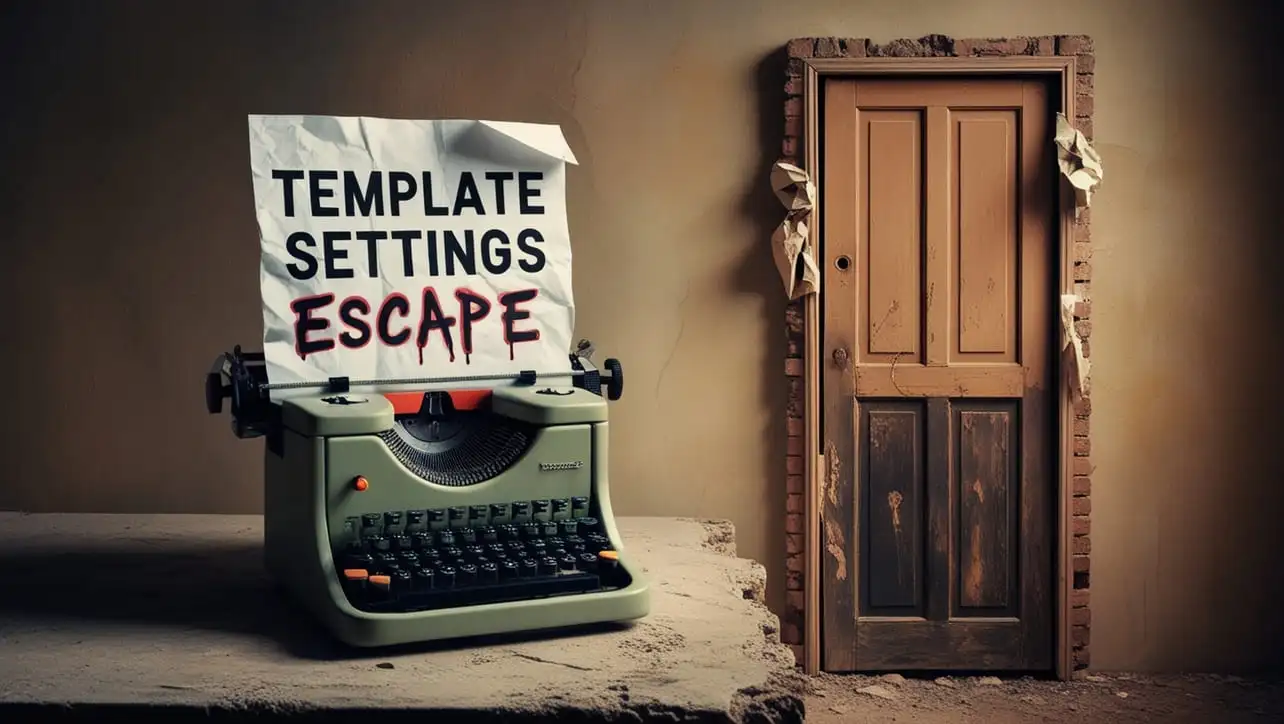
Lodash _.templateSettings.escape Property
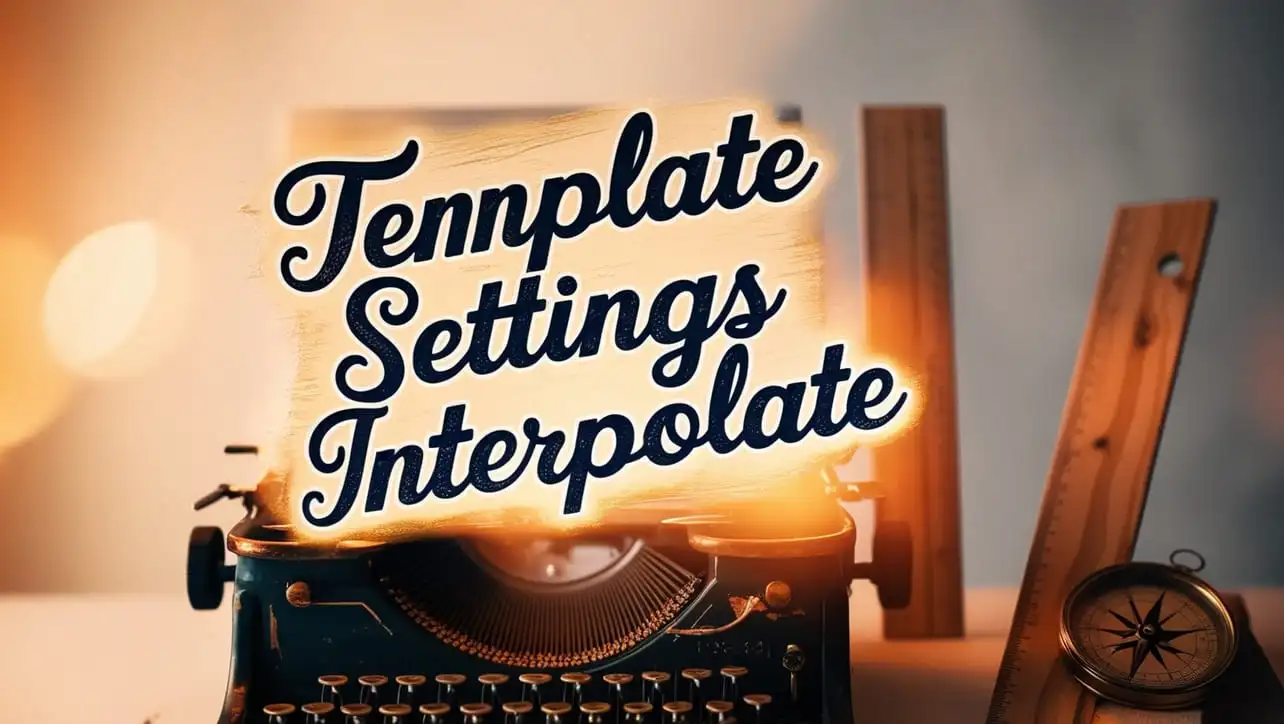
Lodash _.templateSettings.interpolate Property
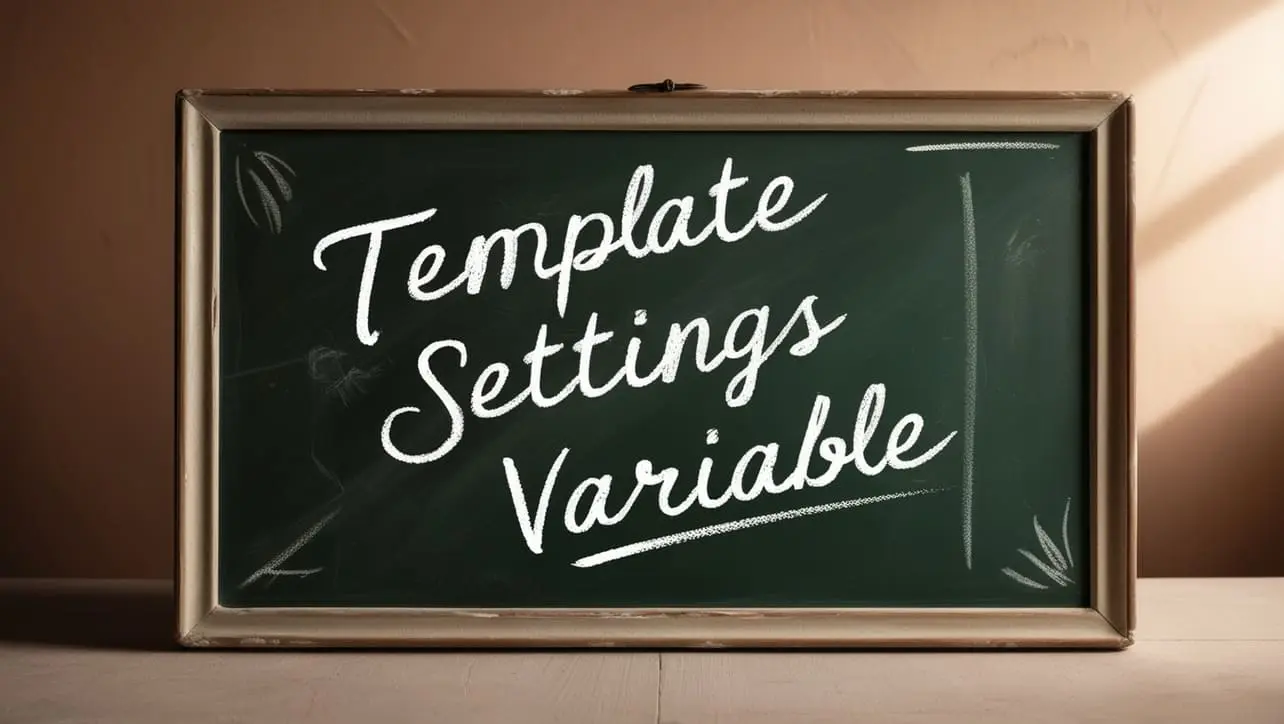
If you have any doubts regarding this article (Lodash _.isSet() Lang Method), please comment here. I will help you immediately.