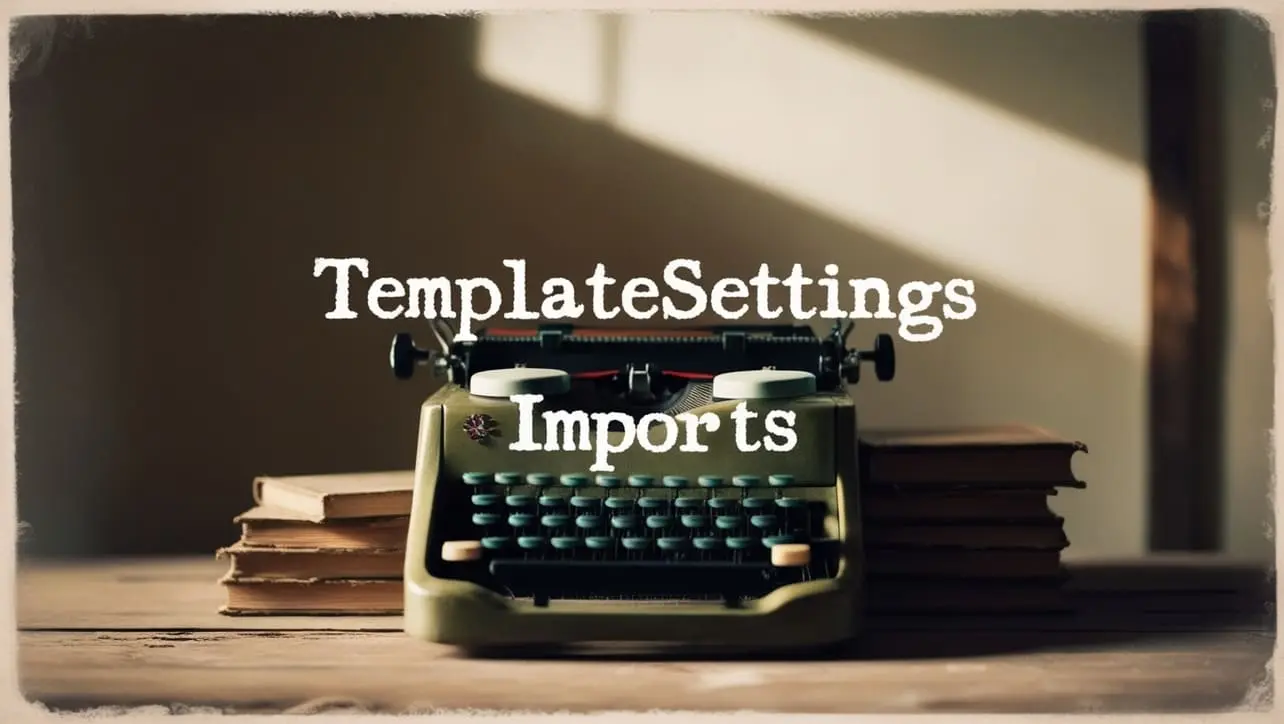
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isObject() Lang Method
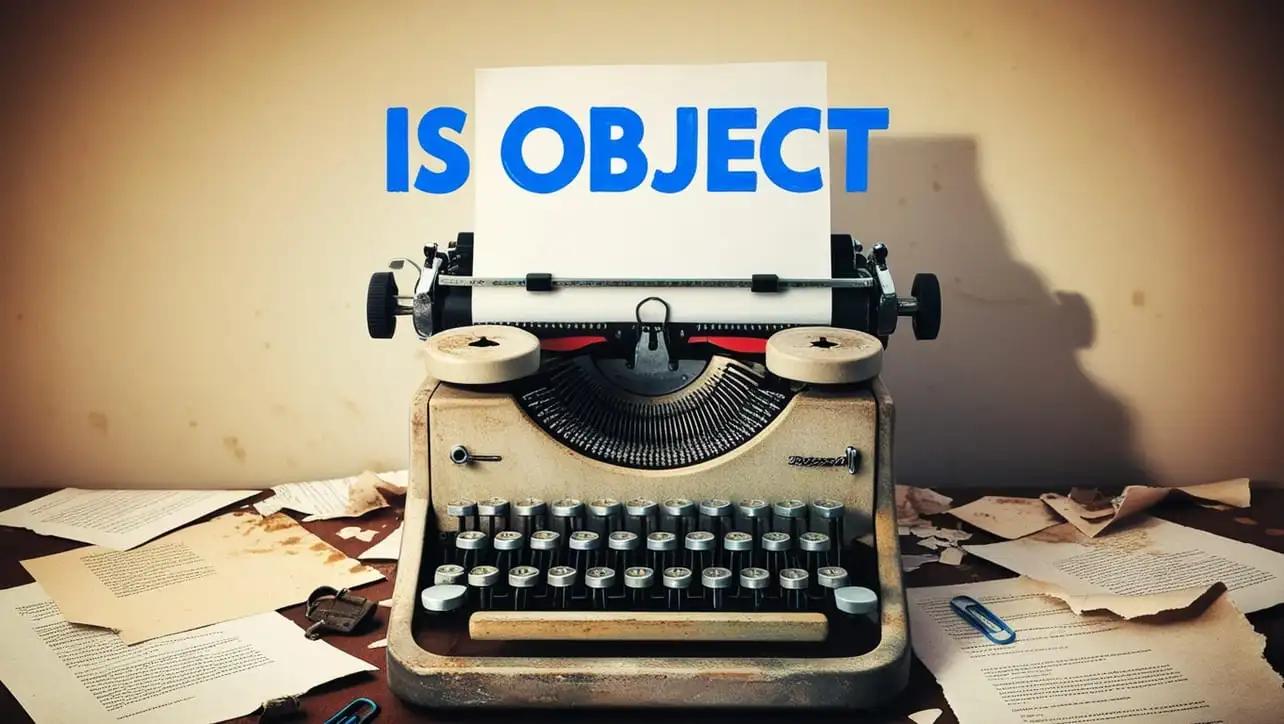
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, handling different data types is a common challenge. The Lodash library comes to the rescue with its versatile utility functions, and one such handy method is _.isObject()
.
This method provides a straightforward way to check whether a given value is an object or not, simplifying conditional logic and enhancing code reliability.
🧠 Understanding _.isObject() Method
The _.isObject()
method in Lodash is designed to determine whether a value is an object. It accounts for various edge cases, such as arrays and null values, offering a more comprehensive check than the native JavaScript typeof operator.
💡 Syntax
The syntax for the _.isObject()
method is straightforward:
_.isObject(value)
- value: The value to evaluate.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isObject()
method:
const _ = require('lodash');
const objectValue = { key: 'value' };
const arrayValue = [1, 2, 3];
const stringValue = 'Hello, Lodash!';
console.log(_.isObject(objectValue)); // Output: true
console.log(_.isObject(arrayValue)); // Output: true
console.log(_.isObject(stringValue)); // Output: false
In this example, _.isObject()
correctly identifies both the object and array as objects, while recognizing the string as a non-object.
🏆 Best Practices
When working with the _.isObject()
method, consider the following best practices:
Comprehensive Type Checking:
Use
_.isObject()
for comprehensive type checking, especially when dealing with values that might be objects, arrays, or other data types. This method provides a more accurate assessment compared to the typeof operator.example.jsCopiedconst value = /* ...some value... */ ; if (_.isObject(value)) { console.log('The value is an object or array.'); } else { console.log('The value is not an object or array.'); }
Null Values Consideration:
_.isObject()
considers null values as non-objects. If your use case requires treating null as a valid object, add an additional null check.example.jsCopiedconst value = /* ...some value... */ ; if (_.isObject(value) || value === null) { console.log('The value is an object or null.'); } else { console.log('The value is not an object or null.'); }
📚 Use Cases
Conditional Logic:
_.isObject()
is invaluable in conditional logic, allowing you to handle different data types appropriately within your application.example.jsCopiedfunction processData(data) { if (_.isObject(data)) { // Handle object data console.log('Processing object data:', data); } else { // Handle non-object data console.log('Unsupported data type:', typeof data); } } const objectData = { key: 'value' }; const stringData = 'Hello, Lodash!'; processData(objectData); processData(stringData);
Data Validation:
When validating user input or external data,
_.isObject()
can help ensure that the expected data type is received.example.jsCopiedfunction validateUserData(userData) { if (_.isObject(userData)) { // Process user data console.log('Valid user data received:', userData); } else { // Handle invalid data console.error('Invalid user data format.'); } } const validUserData = { username: 'john_doe', age: 25 }; const invalidUserData = 'Not an object'; validateUserData(validUserData); validateUserData(invalidUserData);
Iterating Over Objects:
Use
_.isObject()
to filter and iterate over objects within a collection, ensuring that only objects are processed.example.jsCopiedconst collection = [1, 'hello', { key: 'value' }, [1, 2, 3], { nested: { prop: 'data' } }]; collection.forEach(item => { if (_.isObject(item)) { console.log('Processing object:', item); } });
🎉 Conclusion
The _.isObject()
method in Lodash provides a reliable and comprehensive way to check whether a value is an object. By leveraging this utility function, JavaScript developers can enhance the robustness of their code, simplify type checking, and handle diverse data types with ease.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isObject()
method in your Lodash projects.
👨💻 Join our Community:
Author
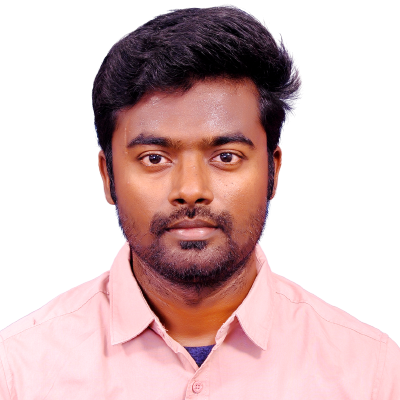
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
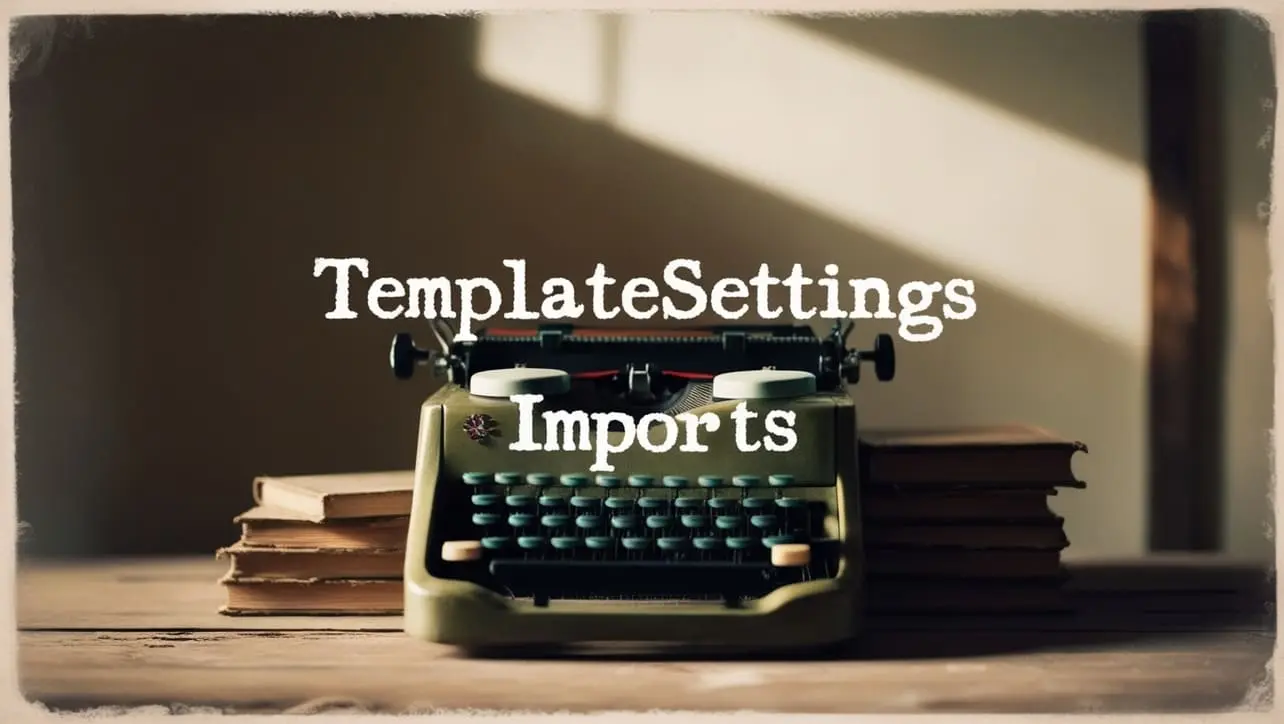
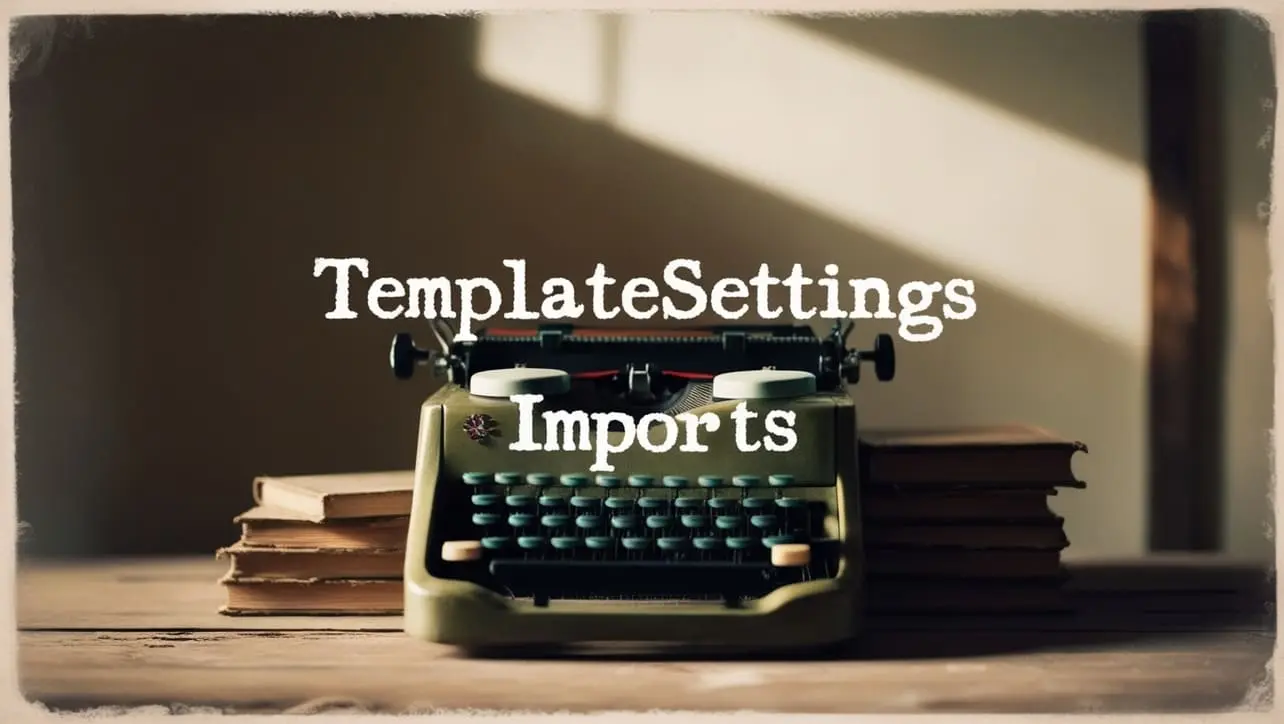
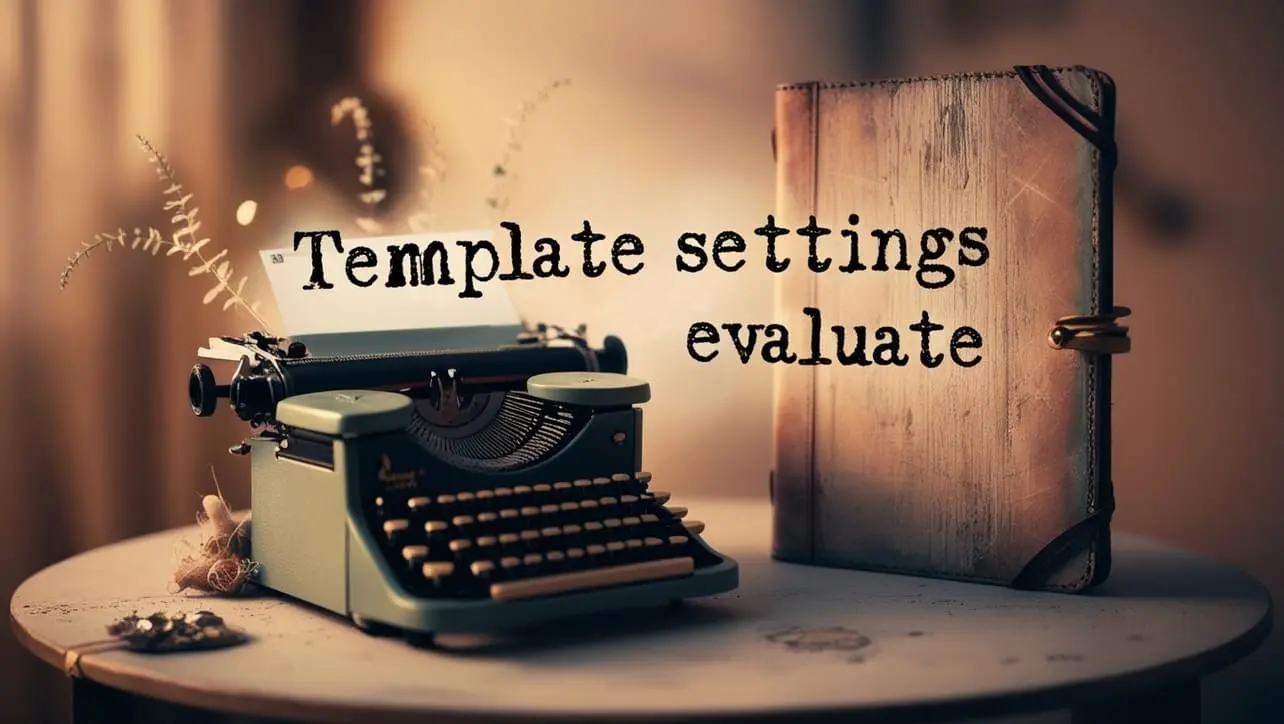
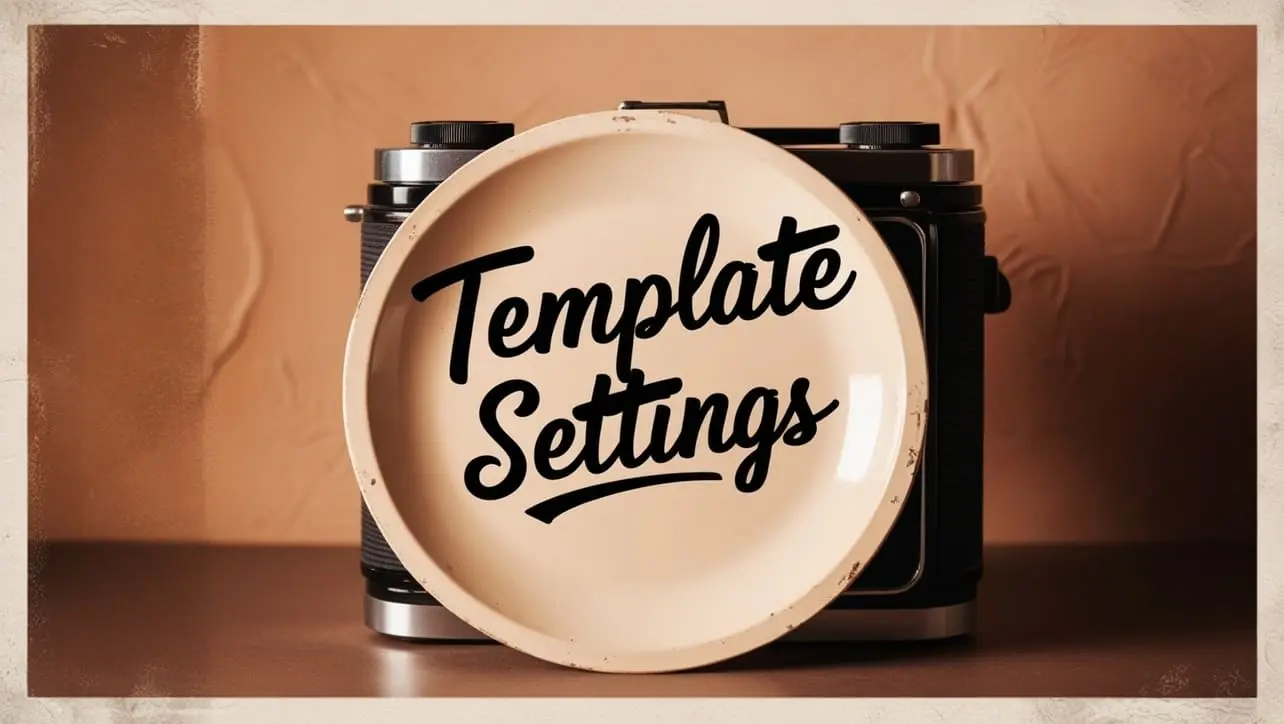
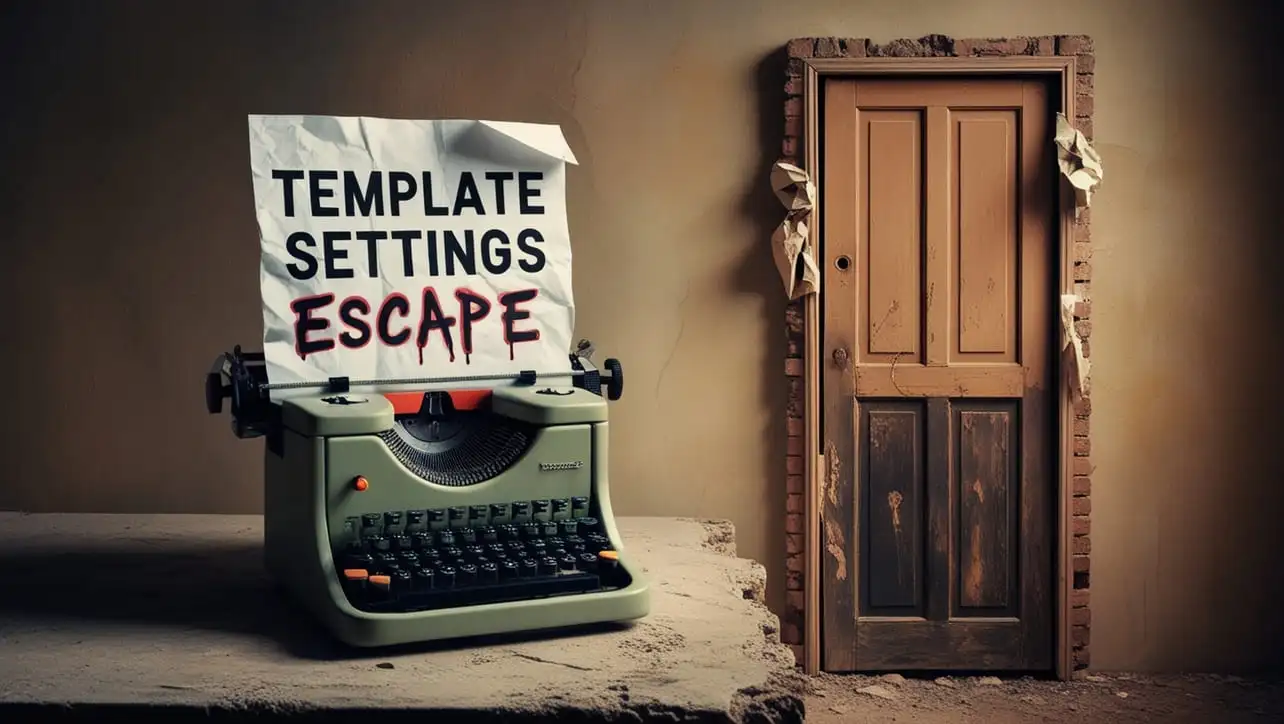
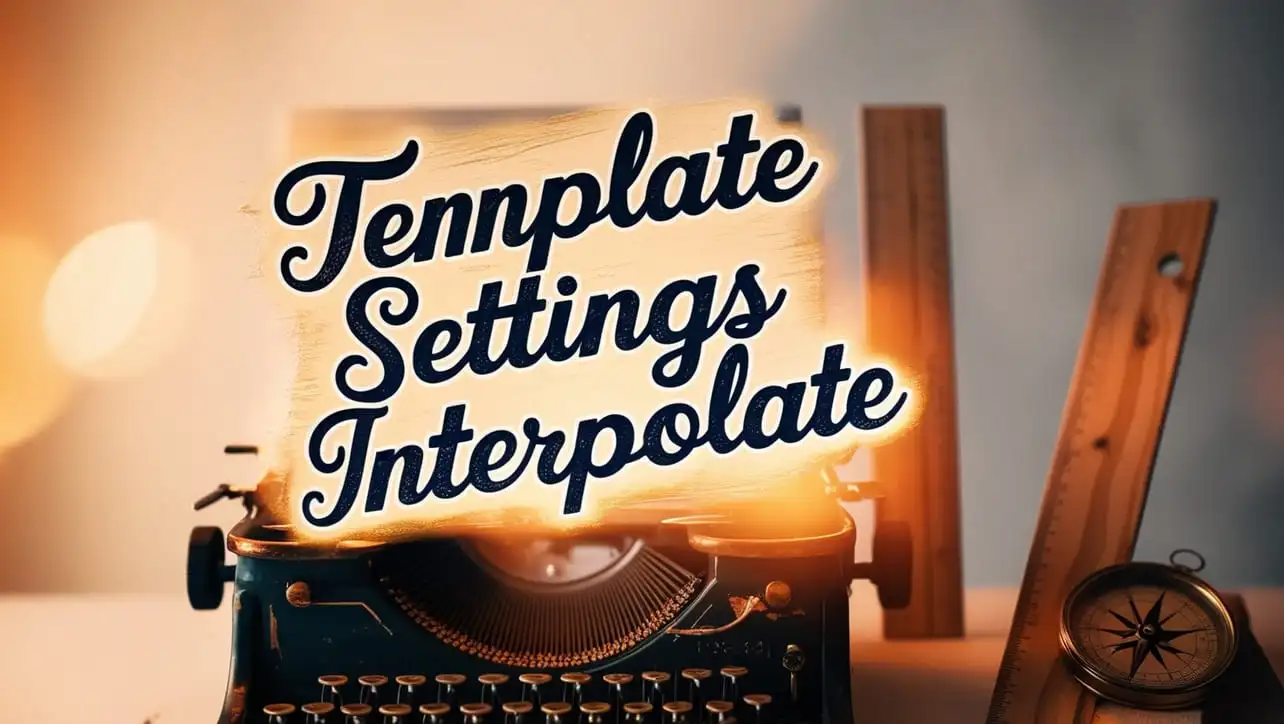
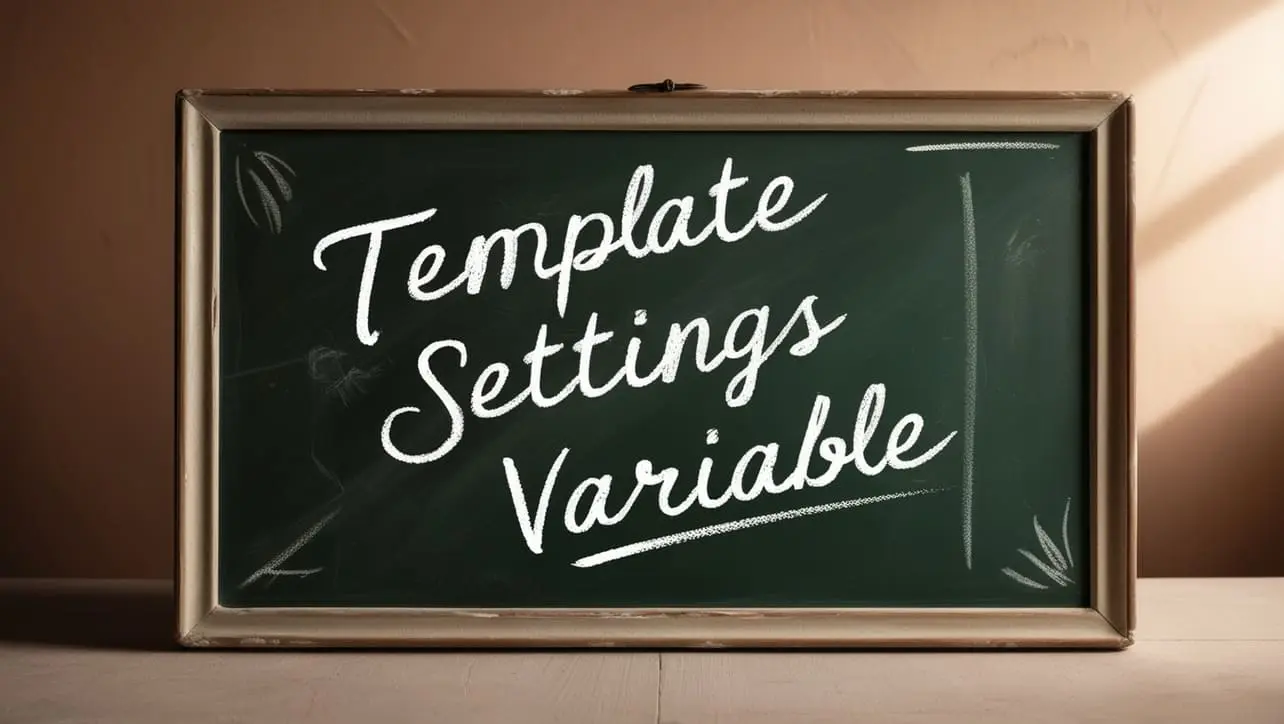
If you have any doubts regarding this article (Lodash _.isObject() Lang Method), please comment here. I will help you immediately.