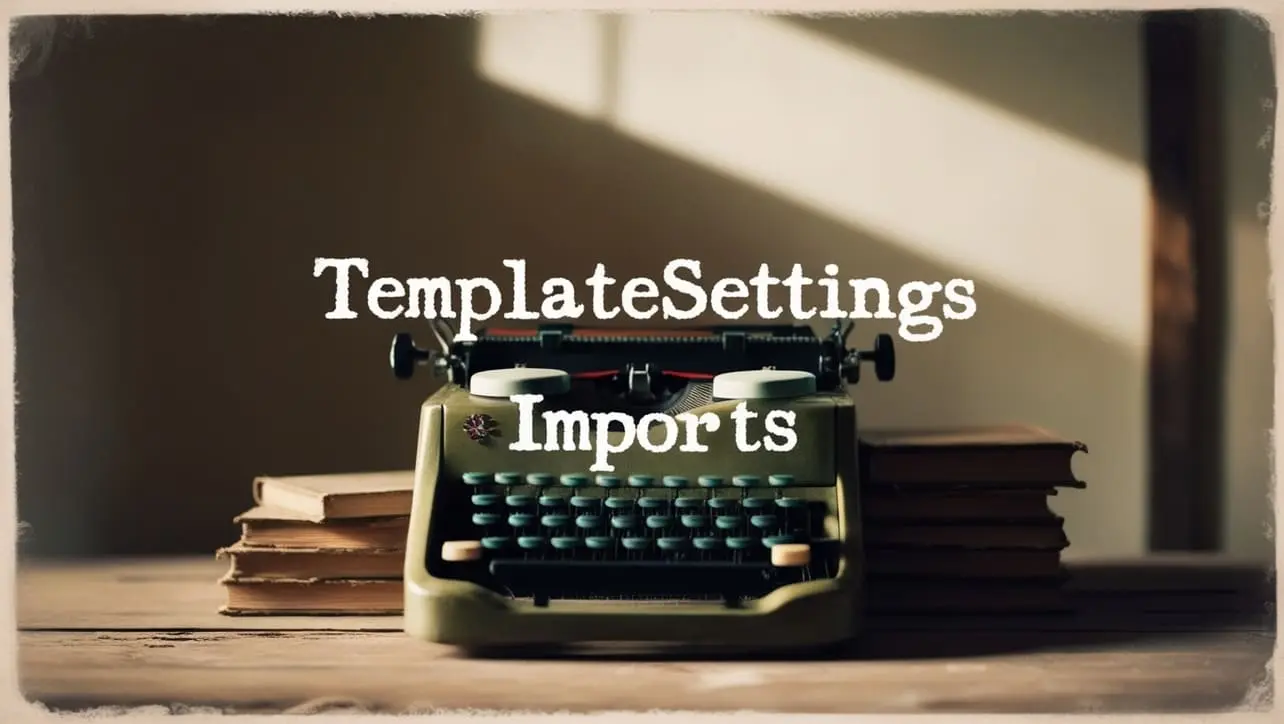
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isNull() Lang Method
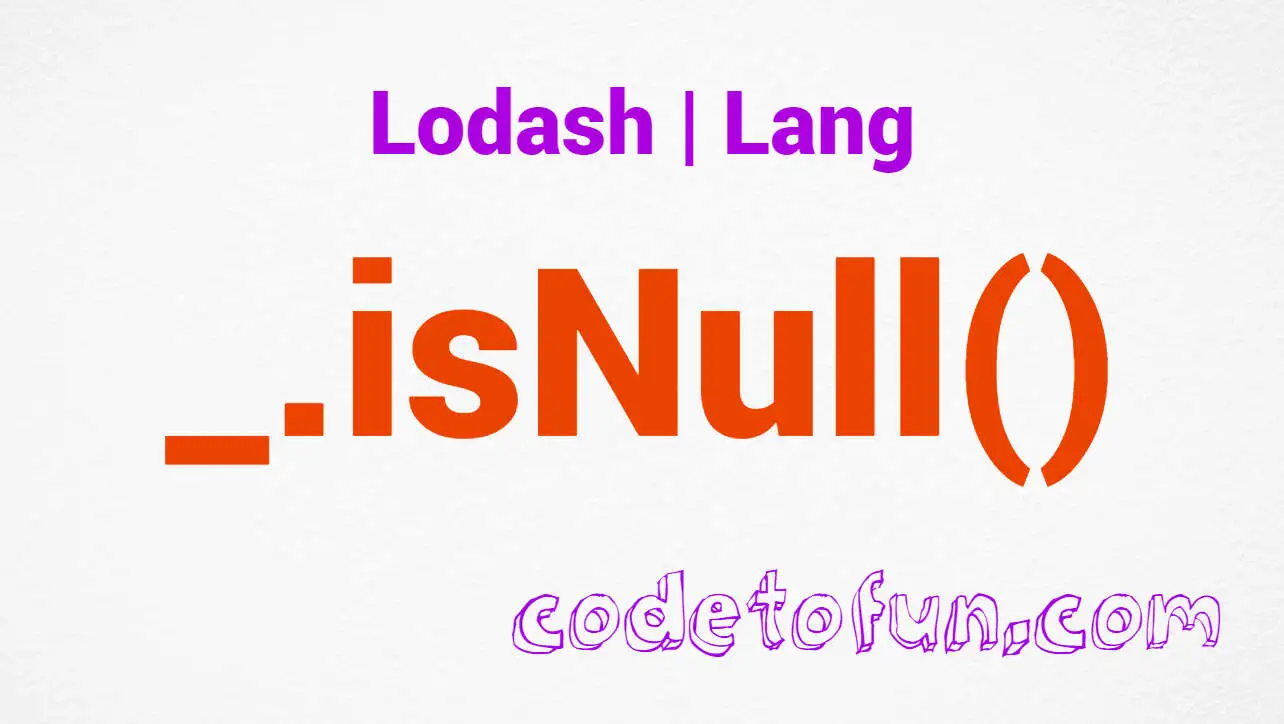
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, handling null values is a common challenge. Lodash, the utility library, comes to the rescue with its versatile set of functions. Among them is the _.isNull()
method, a straightforward tool for checking whether a value is null or not.
This method simplifies null-checking operations, making code more robust and readable.
🧠 Understanding _.isNull() Method
The _.isNull()
method in Lodash is designed to determine if a given value is null. It provides a reliable way to perform null checks, especially in scenarios where distinguishing between null and other falsy values is crucial.
💡 Syntax
The syntax for the _.isNull()
method is straightforward:
_.isNull(value)
- value: The value to check for null.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isNull()
method:
const _ = require('lodash');
const exampleValue = null;
const isNullValue = _.isNull(exampleValue);
console.log(isNullValue);
// Output: true
In this example, exampleValue is explicitly set to null, and _.isNull()
accurately identifies it as such.
🏆 Best Practices
When working with the _.isNull()
method, consider the following best practices:
Clear Null Checks:
Use
_.isNull()
to make your null checks explicit and clear in your code. This enhances readability and reduces the likelihood of errors related to null values.example.jsCopiedconst possiblyNullValue = /* ...some variable... */ ; if (_.isNull(possiblyNullValue)) { // Handle null case console.log('Value is null.'); } else { // Continue processing non-null value console.log('Value is not null:', possiblyNullValue); }
Combined Checks:
Leverage
_.isNull()
in conjunction with other checks when needed. This is particularly useful when you want to ensure a value is both defined and not null.example.jsCopiedconst combinedCheckValue = /* ...some variable... */ ; if (_.isUndefined(combinedCheckValue) || _.isNull(combinedCheckValue)) { console.log('Value is either undefined or null.'); } else { console.log('Value is defined and not null:', combinedCheckValue); }
Consistent Null Handling:
Maintain consistency in null handling throughout your codebase by using
_.isNull()
consistently. This ensures a standardized approach to dealing with null values.example.jsCopiedfunction processValue(inputValue) { if (_.isNull(inputValue)) { console.log('Received null value. Skipping processing.'); return; } // Continue with processing non-null value console.log('Processing value:', inputValue); }
📚 Use Cases
Input Validation:
When validating user inputs or external data, use
_.isNull()
to explicitly check for null values, ensuring accurate and secure handling of input.example.jsCopiedfunction validateUserInput(userInput) { if (_.isNull(userInput)) { console.log('Invalid input. Please provide a non-null value.'); return false; } // Continue with validation for non-null input console.log('Validating input:', userInput); return true; }
Default Values:
Employ
_.isNull()
to set default values or take alternative actions when a value is null.example.jsCopiedfunction processUserDetails(userDetails) { const name = _.isNull(userDetails.name) ? 'Guest' : userDetails.name; const age = _.isNull(userDetails.age) ? 'Not specified' : userDetails.age; console.log('Name:', name); console.log('Age:', age); }
Conditional Rendering:
In user interfaces, use
_.isNull()
to conditionally render or handle components based on whether a value is null.example.jsCopiedfunction renderUserDetails(userDetails) { return ( <div> {_.isNull(userDetails) ? ( <p>No user details available.</p> ) : ( <div> <p>Name: {userDetails.name}</p> <p>Age: {userDetails.age}</p> </div> )} </div> ); }
🎉 Conclusion
The _.isNull()
method in Lodash is a valuable tool for simplifying null checks in JavaScript. By incorporating this method into your code, you can enhance clarity, readability, and consistency when dealing with null values. Whether you're validating inputs, setting default values, or rendering components conditionally, _.isNull()
provides a reliable solution for null-related operations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isNull()
method in your Lodash projects.
👨💻 Join our Community:
Author
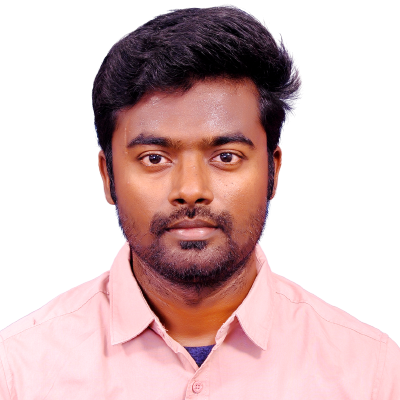
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
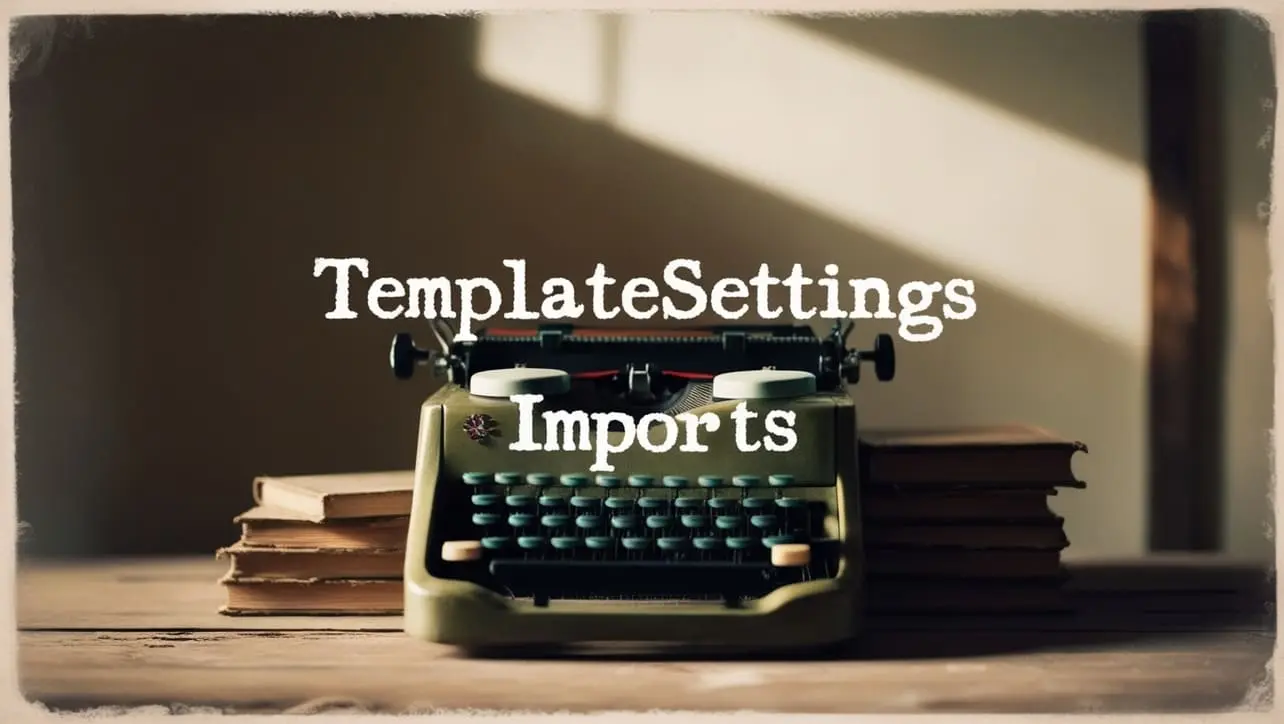
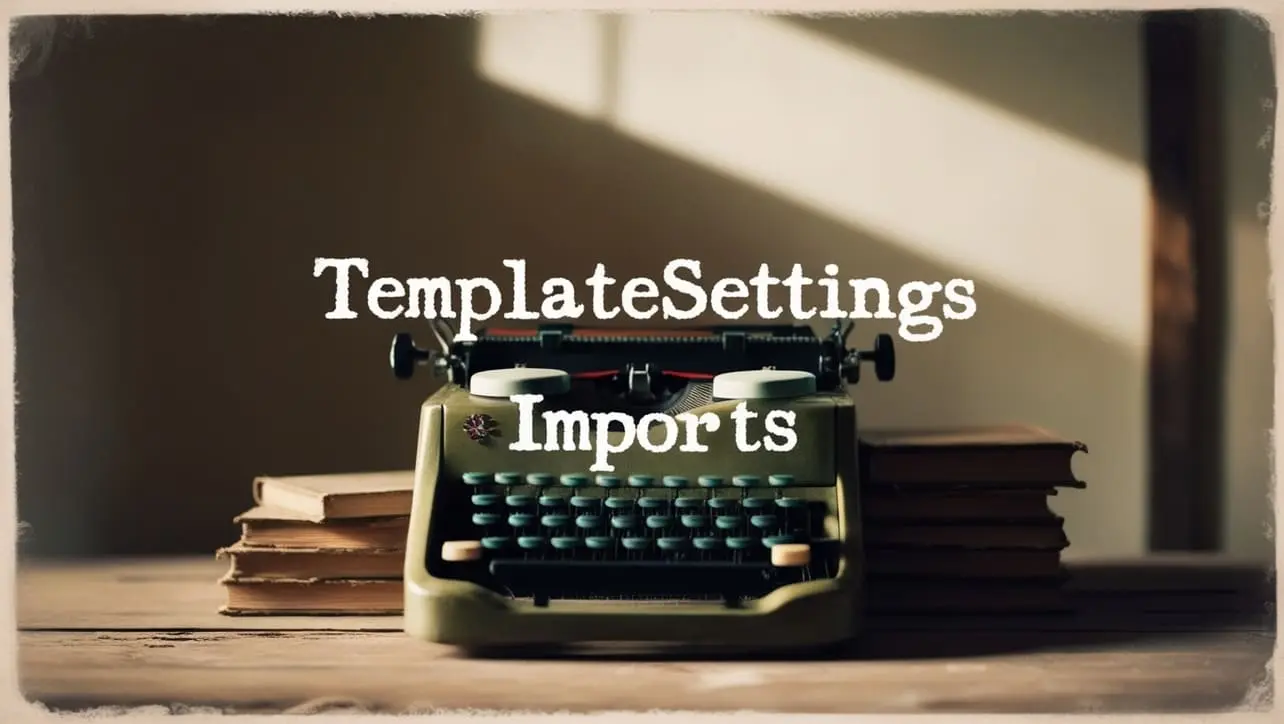
Lodash _.templateSettings.imports Property
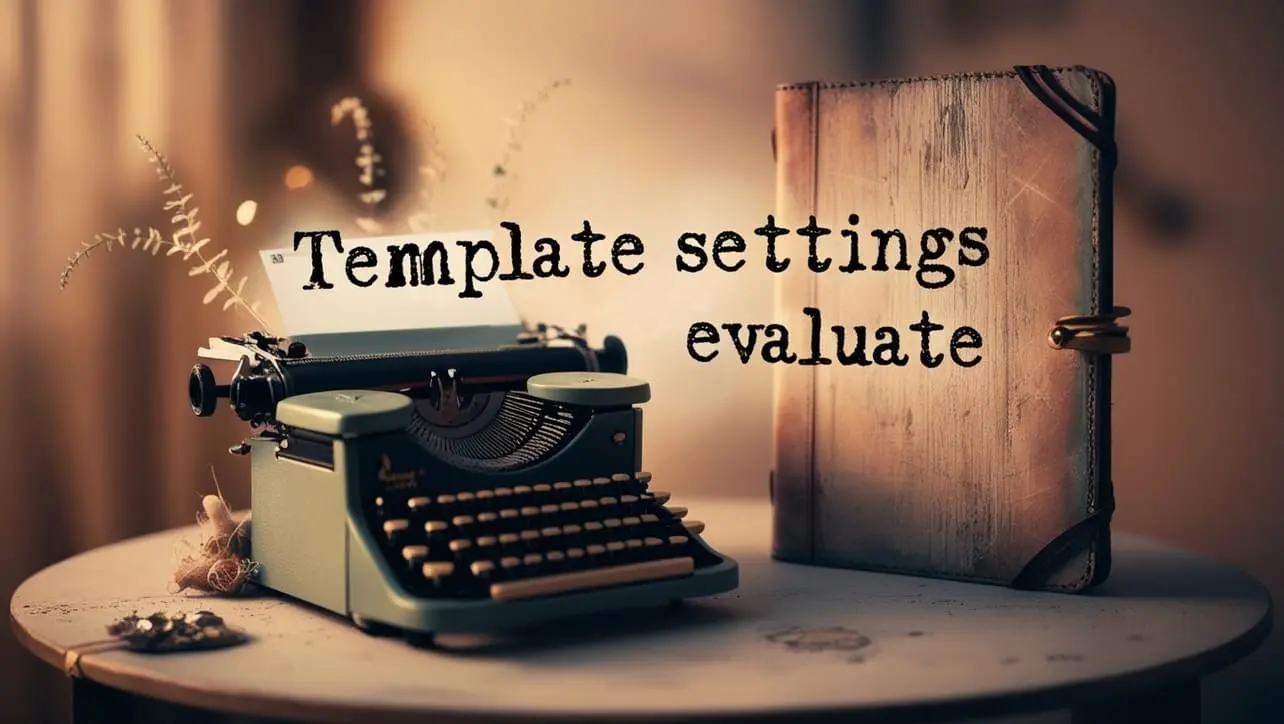
Lodash _.templateSettings.evaluate Property
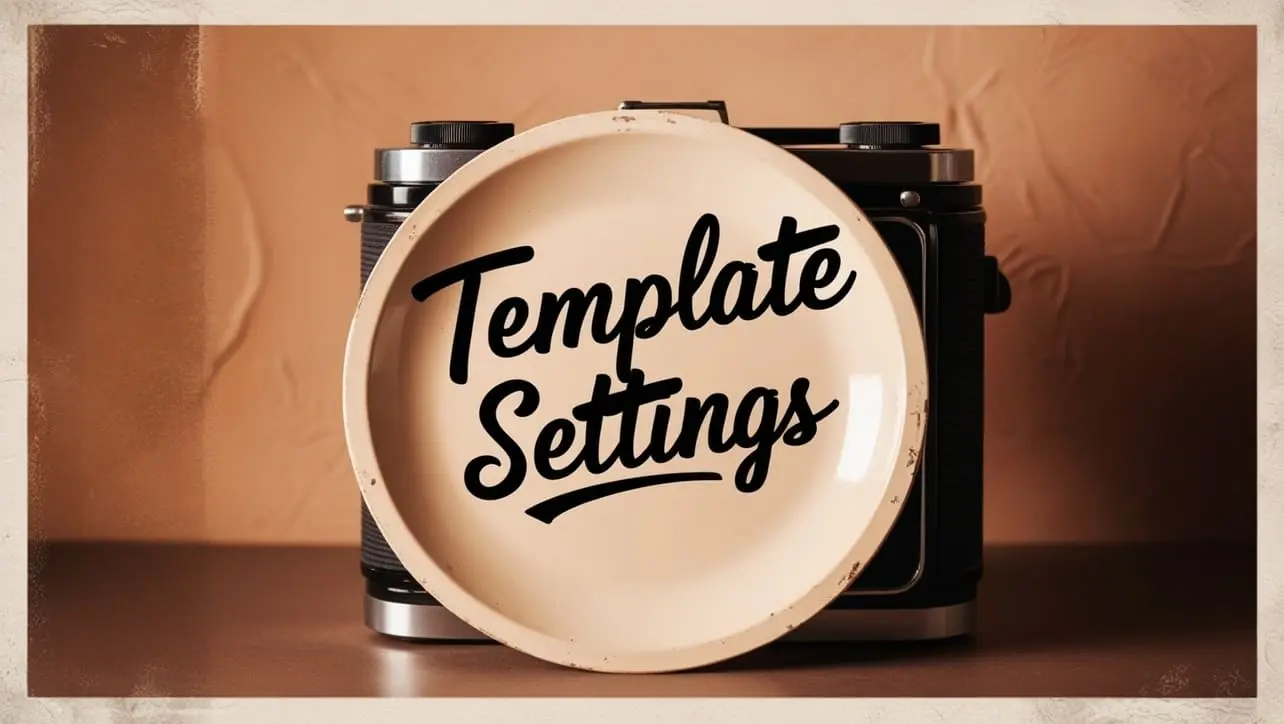
Lodash _.templateSettings Property
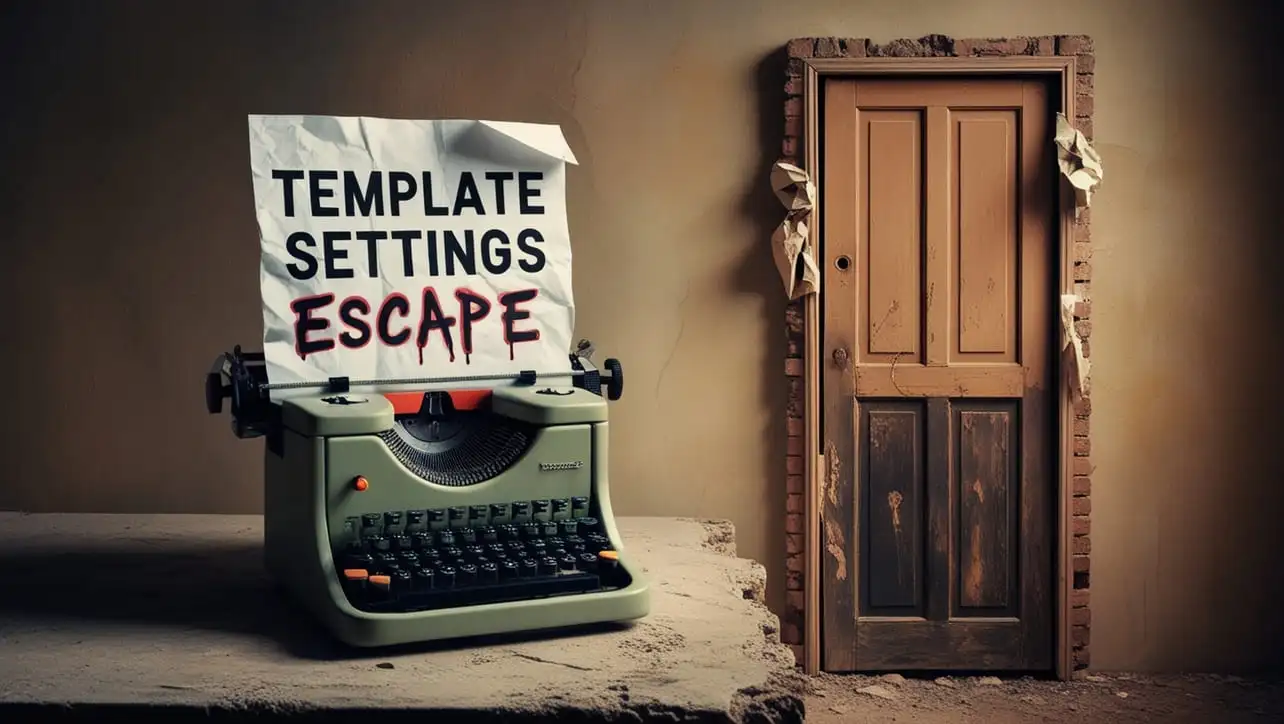
Lodash _.templateSettings.escape Property
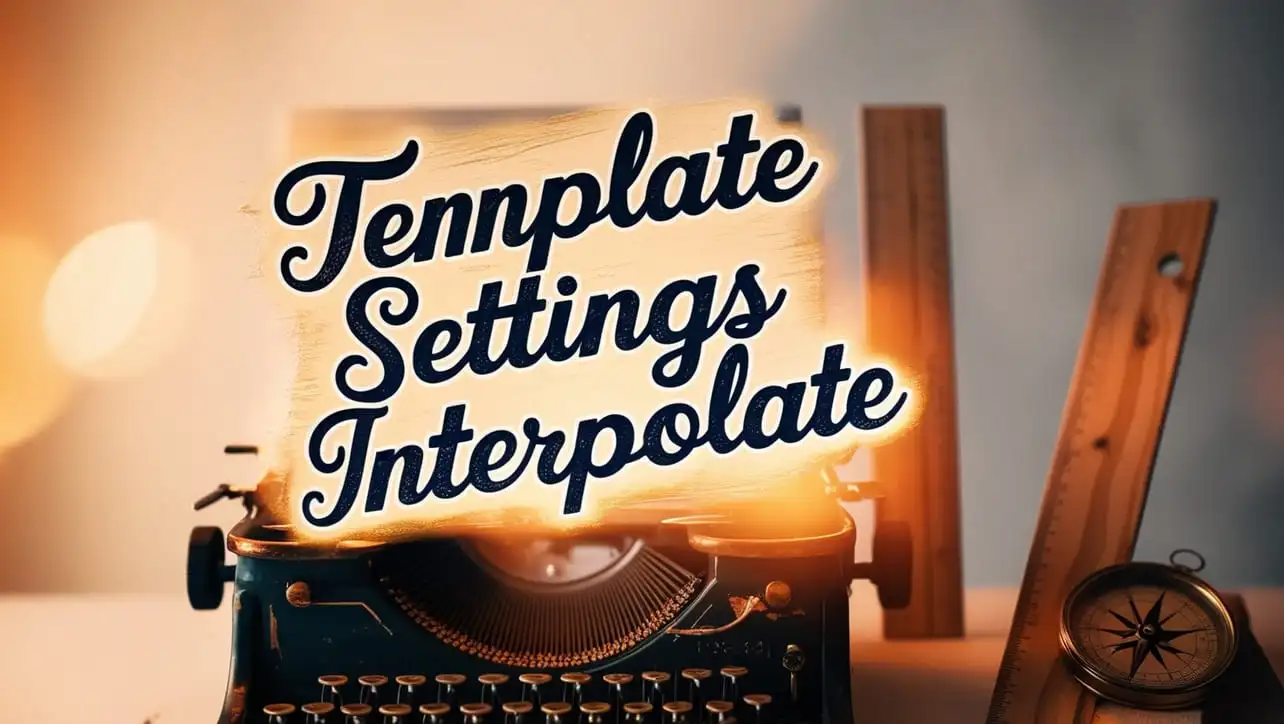
Lodash _.templateSettings.interpolate Property
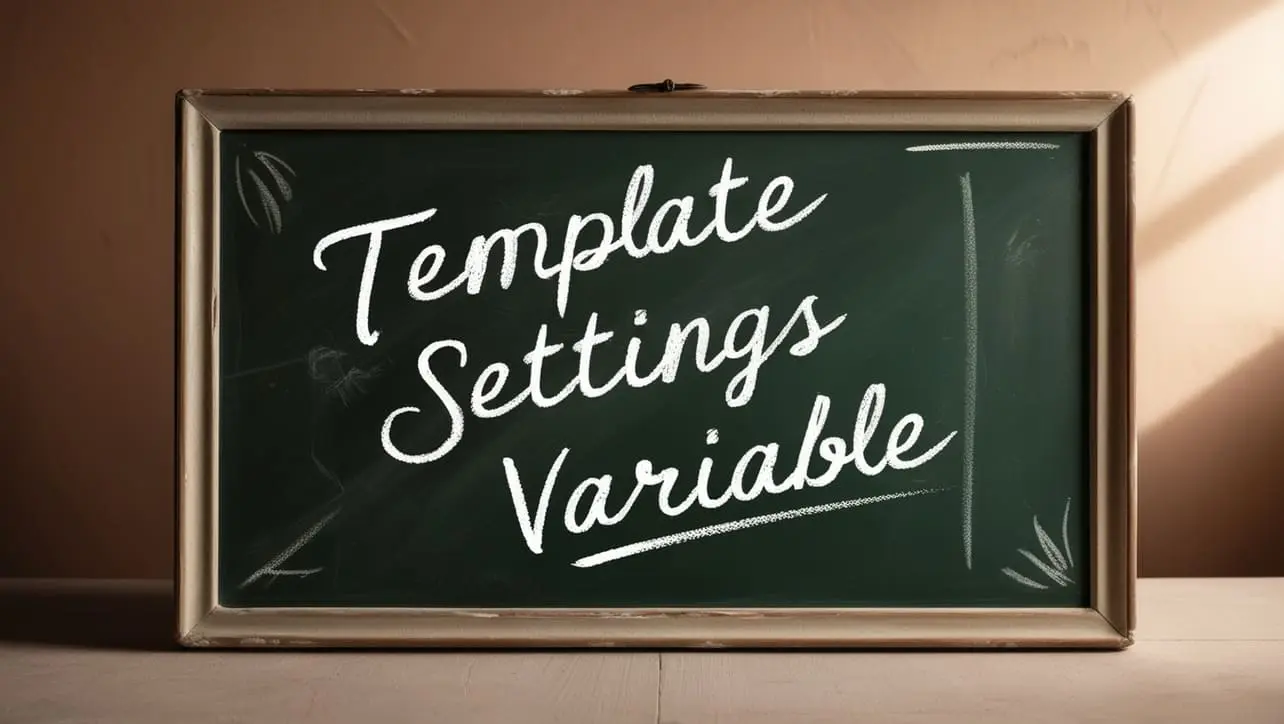
If you have any doubts regarding this article (Lodash _.isNull() Lang Method), please comment here. I will help you immediately.