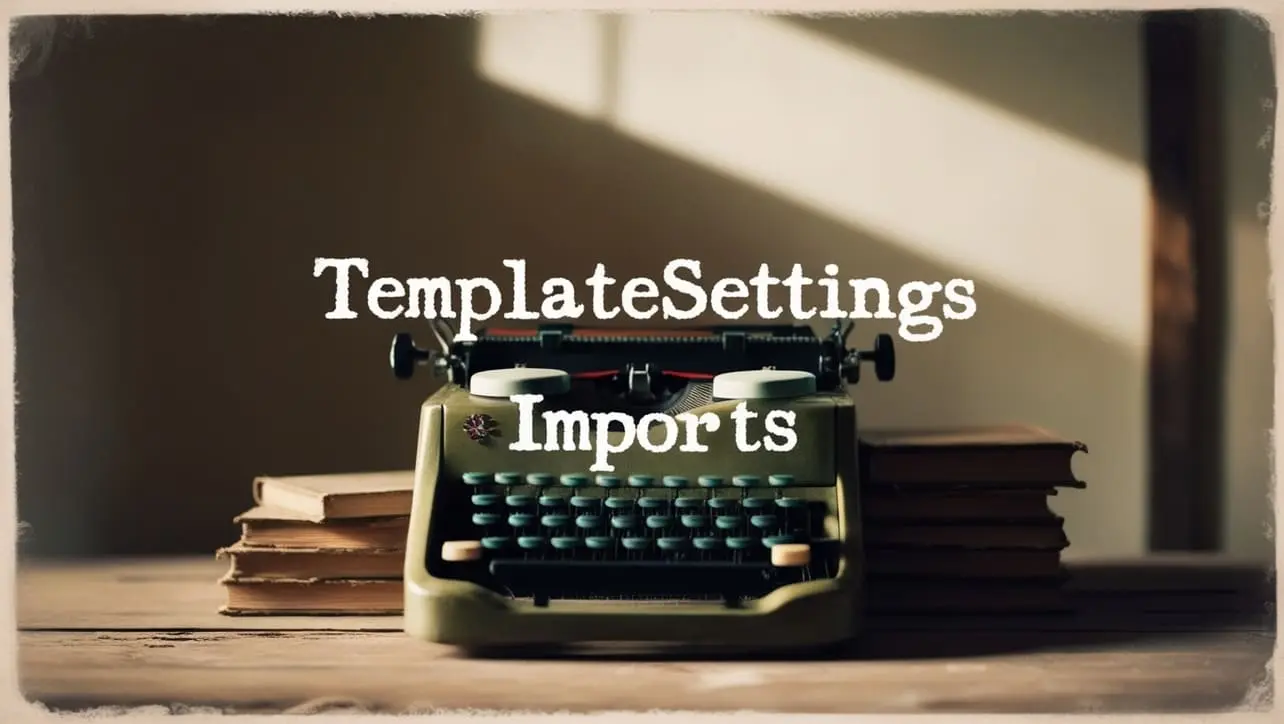
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isMap() Lang Method
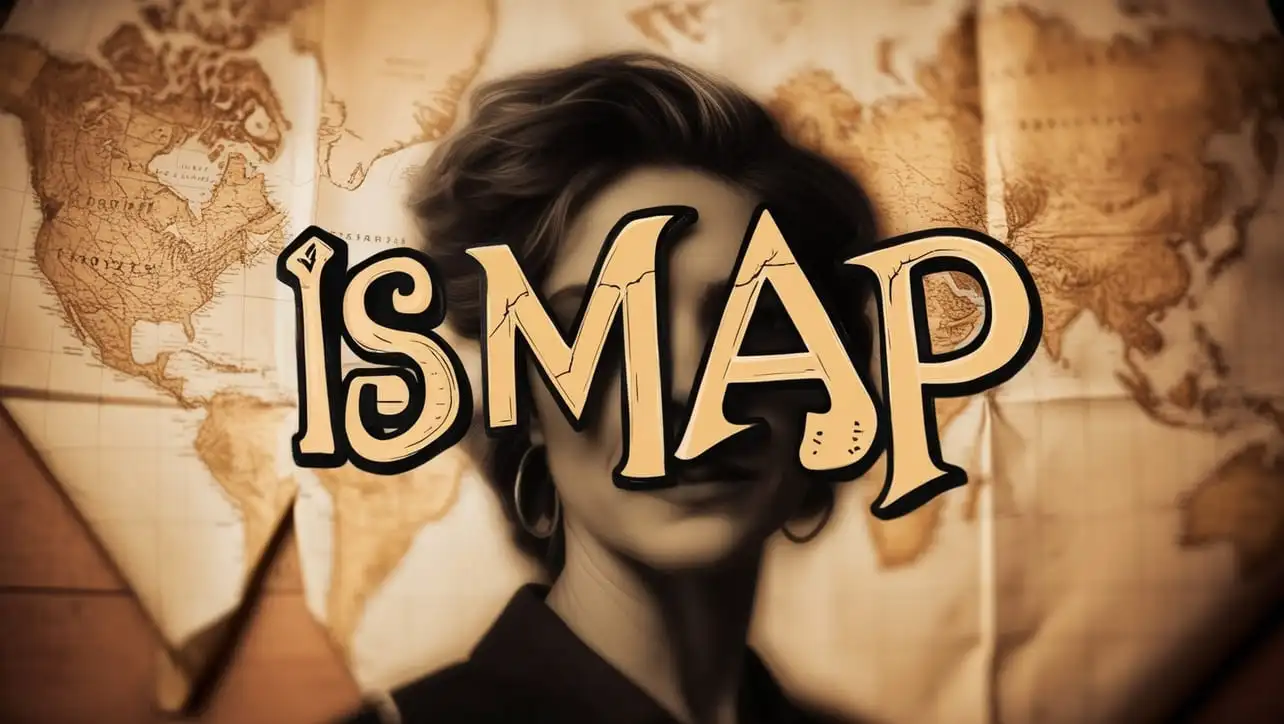
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, reliable data type checking is crucial for ensuring the integrity of your code. Lodash, a feature-rich utility library, provides a variety of functions for simplifying common programming tasks. Among these functions is the _.isMap()
method, an essential tool for determining whether a given value is a Map object.
Understanding and utilizing _.isMap()
can enhance the robustness and clarity of your JavaScript applications.
🧠 Understanding _.isMap() Method
The _.isMap()
method in Lodash is designed to ascertain whether a given value is a Map object or not. This is particularly useful in scenarios where you need to validate the type of an object before performing operations specific to Map instances.
💡 Syntax
The syntax for the _.isMap()
method is straightforward:
_.isMap(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isMap()
method:
const _ = require('lodash');
const mapInstance = new Map();
const isArrayResult = _.isMap(mapInstance);
console.log(isArrayResult);
// Output: true
In this example, the mapInstance is checked using _.isMap()
, confirming that it is indeed a Map object.
🏆 Best Practices
When working with the _.isMap()
method, consider the following best practices:
Validating Map Instances:
Ensure that the value passed to
_.isMap()
is a valid Map instance. This method is specifically designed for checking whether an object is of type Map, and passing other data types may yield inaccurate results.example.jsCopiedconst nonMapObject = { key: 'value' }; const isMap = _.isMap(nonMapObject); console.log(isMap); // Output: false
Combining with Type Checks:
Combine
_.isMap()
with other type-checking methods when dealing with complex data structures. This helps in creating robust checks for various scenarios.example.jsCopiedconst mixedData = [1, 'two', new Map(), { key: 'value' }]; mixedData.forEach(item => { if (_.isMap(item)) { console.log('Found a Map:', item); } else if (_.isPlainObject(item)) { console.log('Found a Plain Object:', item); } // Add more conditions as needed for different types });
Handling Null and Undefined:
Take into account that
_.isMap()
returns false for null and undefined values. If needed, combine with additional checks for these cases.example.jsCopiedconst maybeMap = /* ...some variable that may be a Map or null... */ ; if (_.isMap(maybeMap) || maybeMap === null) { // Handle Map or null console.log('Valid Map or null:', maybeMap); } else { console.log('Not a Map or null:', maybeMap); }
📚 Use Cases
Validating Function Parameters:
When designing functions that expect a Map as a parameter, use
_.isMap()
to validate the input and ensure that the function operates as intended.example.jsCopiedfunction processMapData(data) { if (_.isMap(data)) { // Process the Map data console.log('Processing Map data:', data); } else { console.error('Invalid input. Expected a Map.'); } } const validMap = new Map(); const invalidData = { key: 'value' }; processMapData(validMap); processMapData(invalidData);
Dynamic Type Handling:
In situations where the type of an object is dynamic and needs to be handled accordingly,
_.isMap()
can be used to conditionally execute code based on the object's type.example.jsCopiedconst dynamicObject = /* ...some dynamically obtained object... */ ; if (_.isMap(dynamicObject)) { // Handle Map-specific operations console.log('Handling Map-specific operations:', dynamicObject); } else { // Handle other cases console.log('Handling other cases:', dynamicObject); }
Data Validation in Libraries:
When developing libraries or utilities that expect Map inputs, use
_.isMap()
to perform initial data validation, enhancing the robustness of your code.example.jsCopiedfunction processData(inputData) { if (!_.isMap(inputData)) { throw new Error('Invalid input. Expected a Map.'); } // Continue processing the Map data console.log('Processing Map data:', inputData); } const userProvidedData = /* ...some data provided by the user... */ ; try { processData(userProvidedData); } catch (error) { console.error(error.message); }
🎉 Conclusion
The _.isMap()
method in Lodash serves as a reliable tool for checking whether a given value is a Map object. By incorporating this method into your JavaScript applications, you can enhance the clarity, reliability, and robustness of your code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isMap()
method in your Lodash projects.
👨💻 Join our Community:
Author
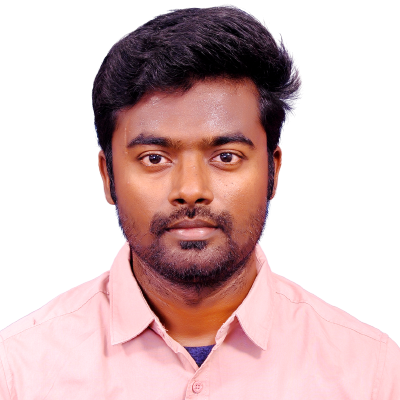
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
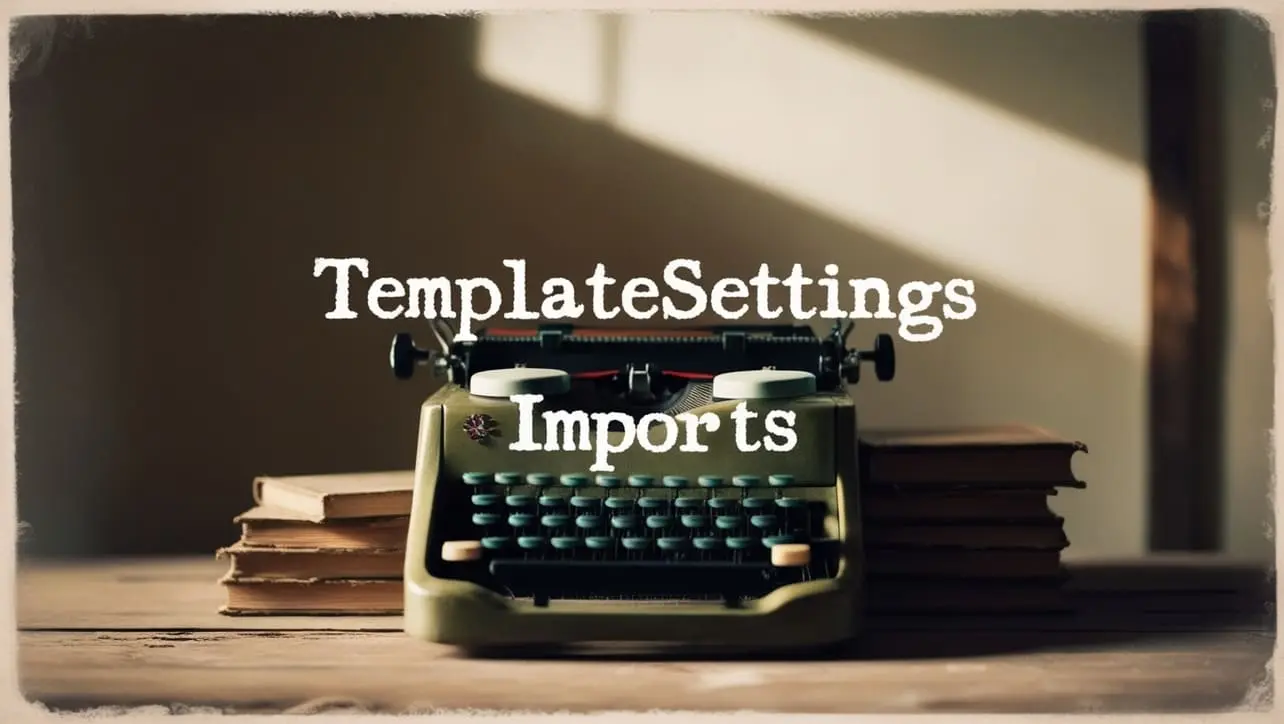
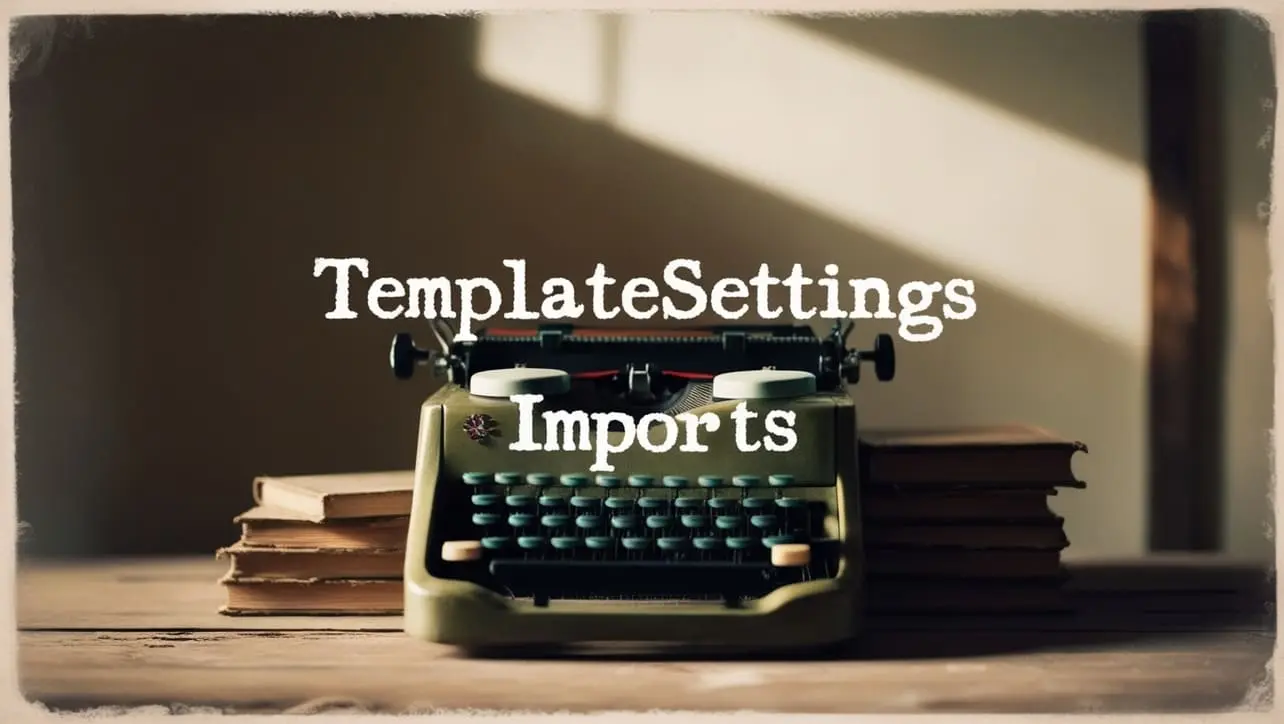
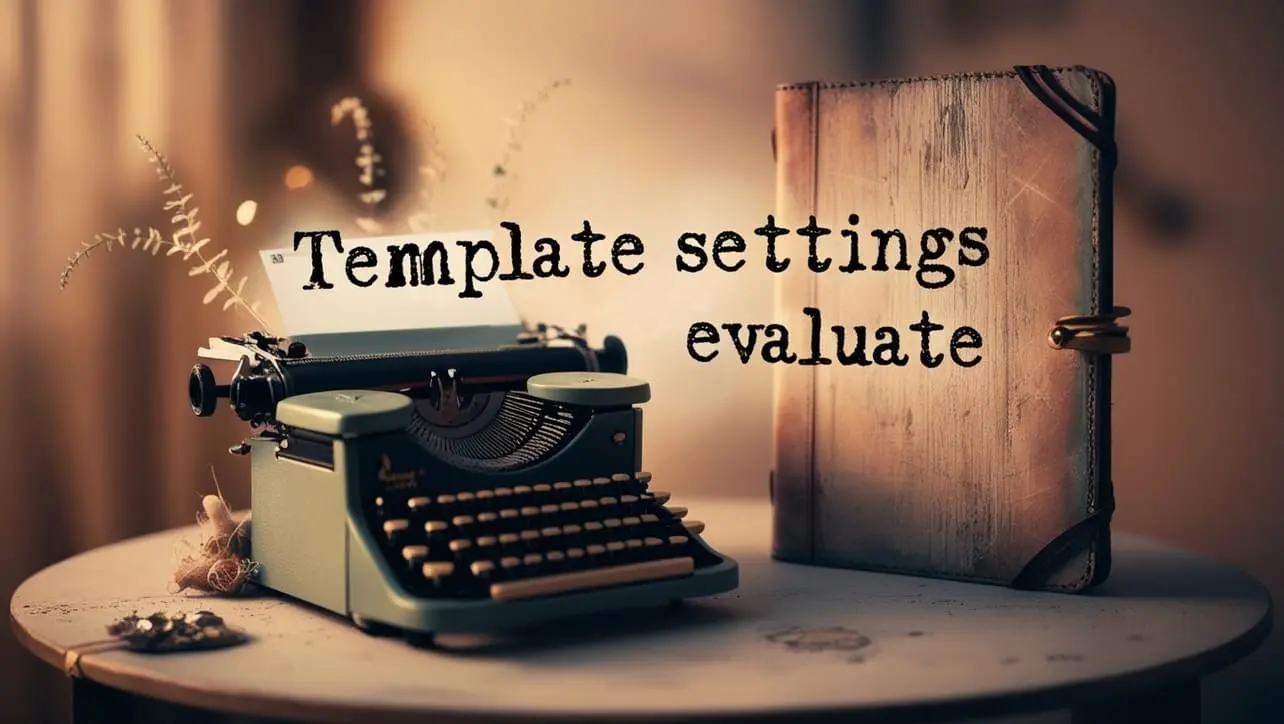
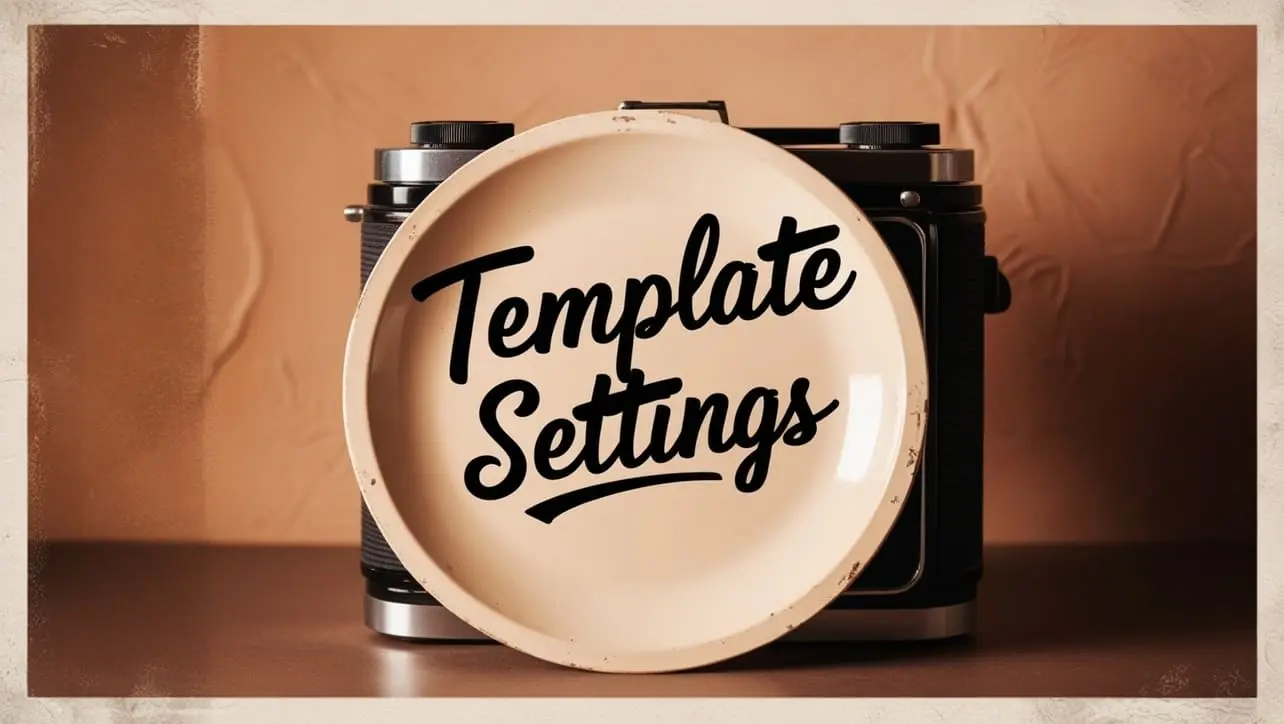
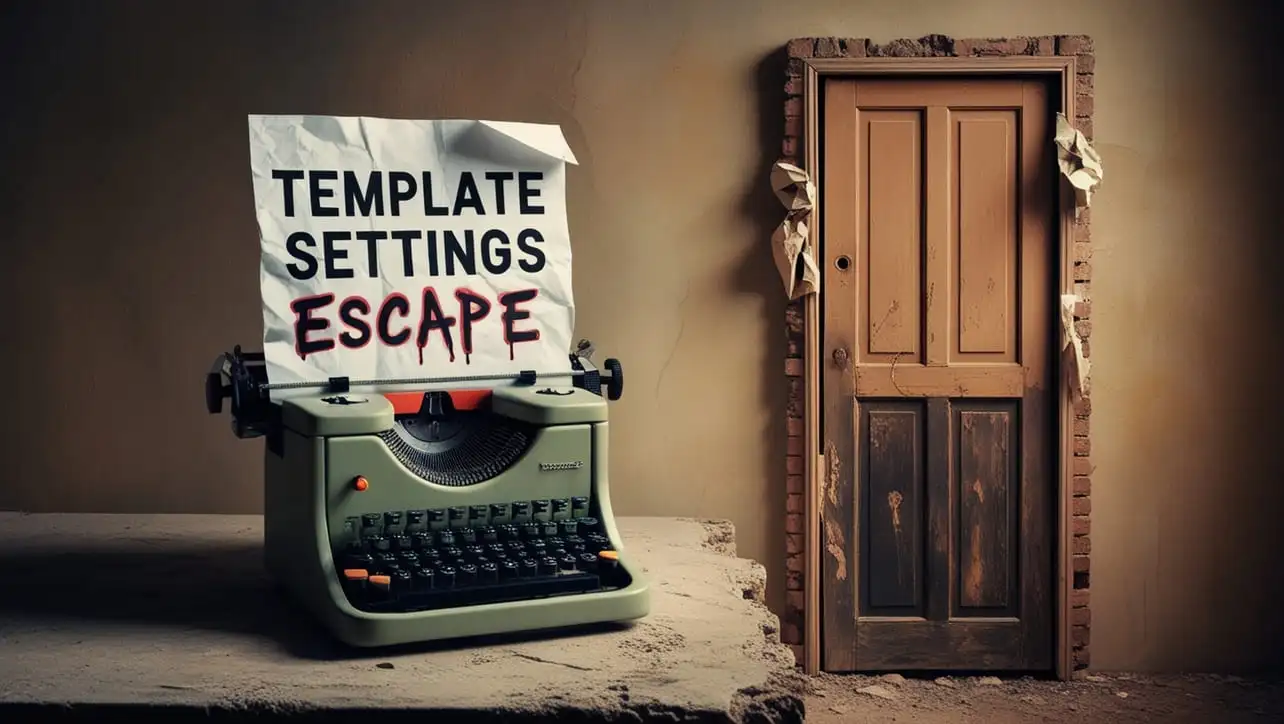
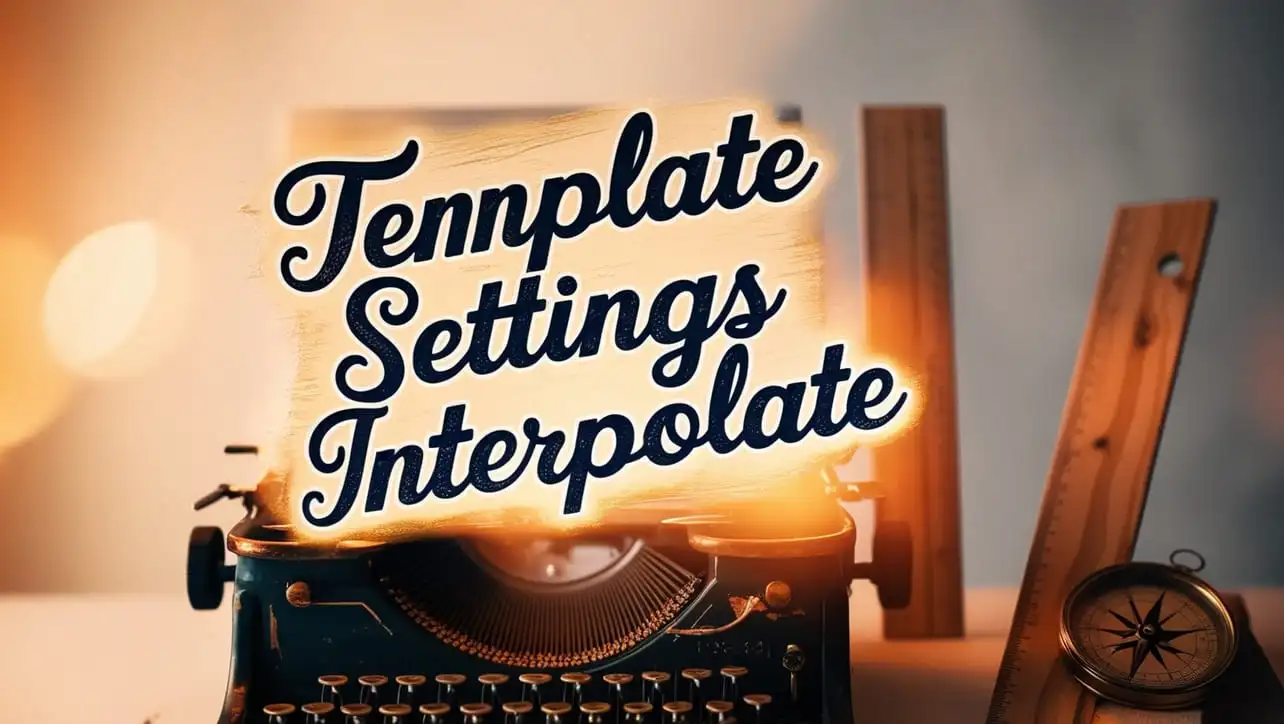
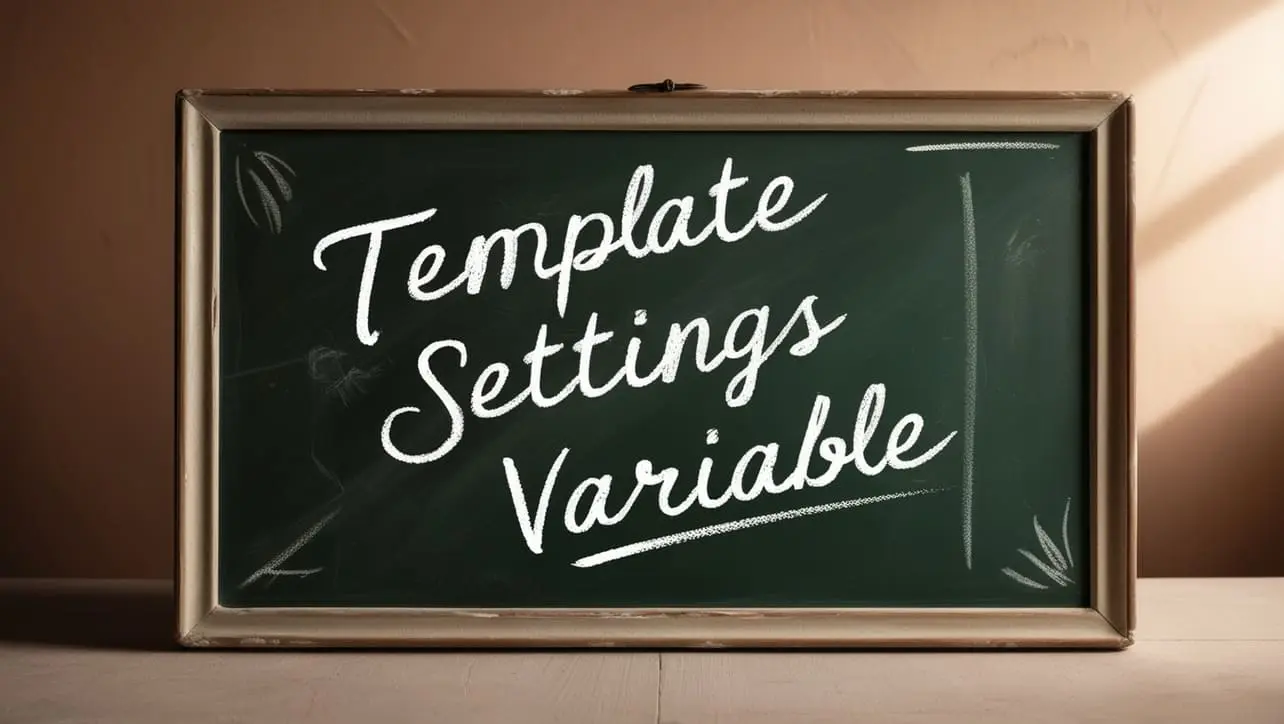
If you have any doubts regarding this article (Lodash _.isMap() Lang Method), please comment here. I will help you immediately.