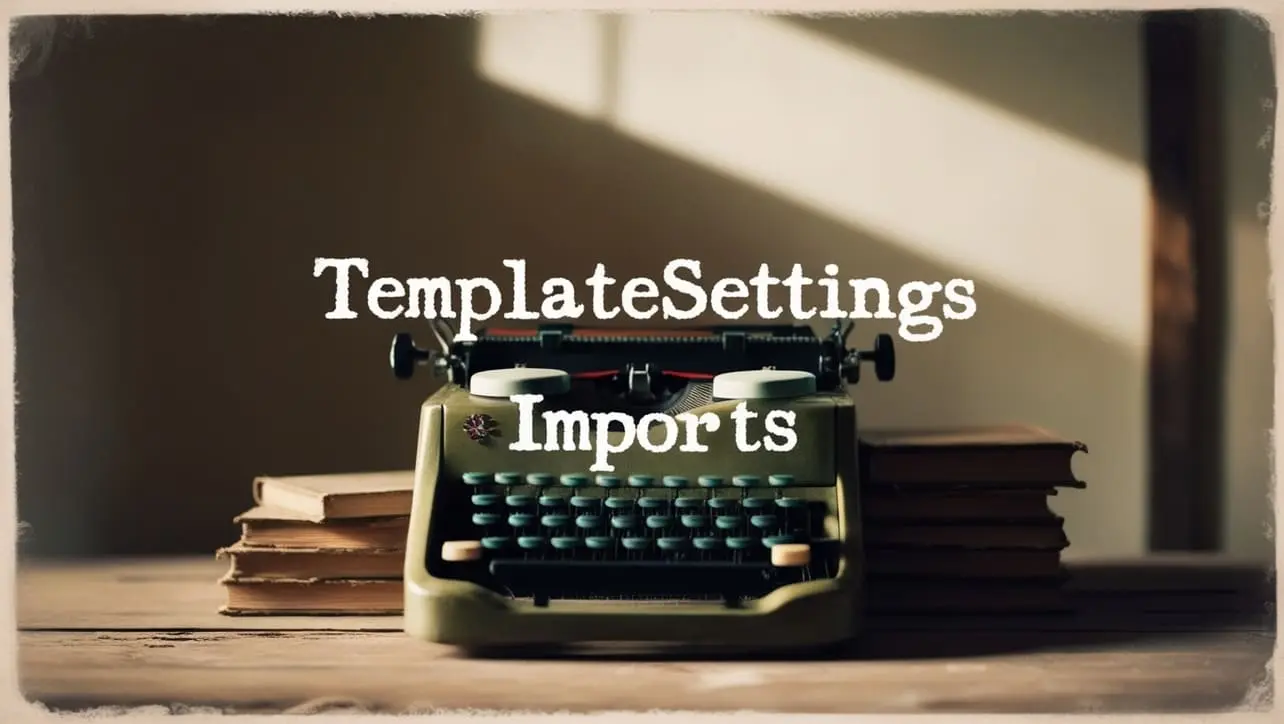
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isFinite() Lang Method
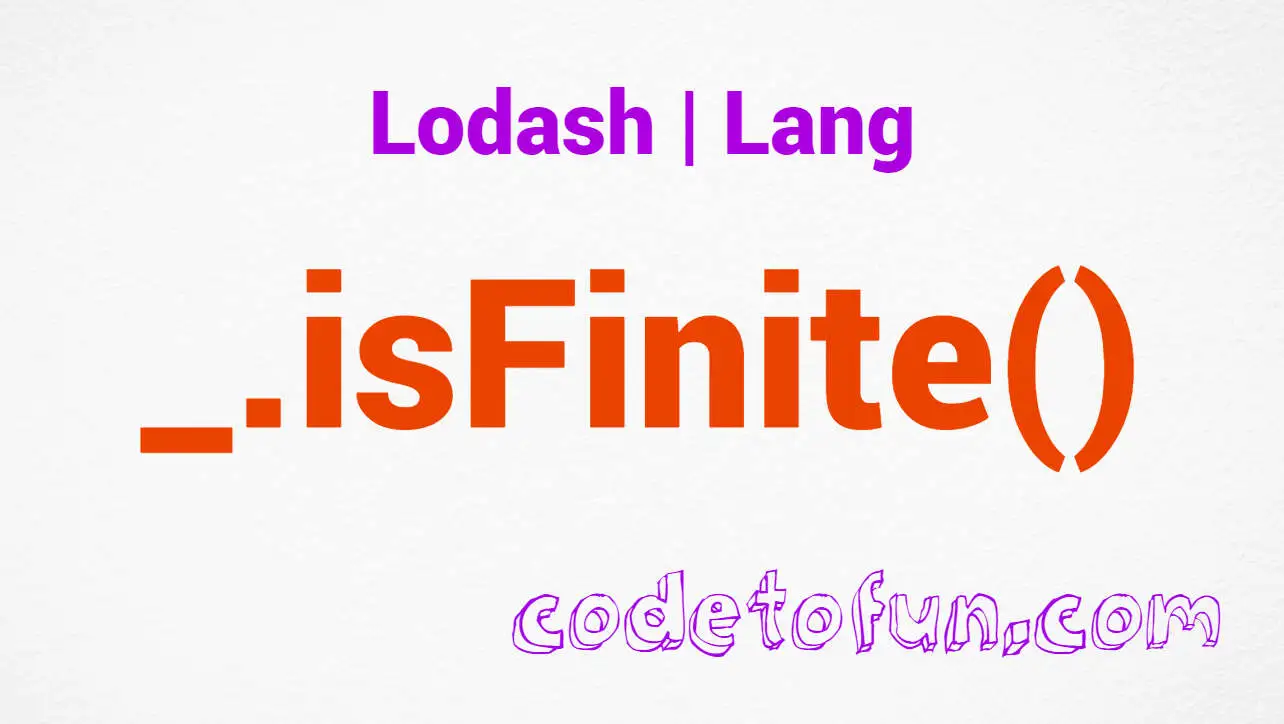
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, ensuring the validity of numeric values is crucial. Lodash, a powerful utility library, provides developers with a variety of functions to simplify common programming tasks. Among these functions is the _.isFinite()
method, designed to determine whether a given value is a finite number.
This method is essential for validating numeric data and preventing issues related to infinite or non-numeric values in your applications.
🧠 Understanding _.isFinite() Method
The _.isFinite()
method in Lodash checks if a value is a finite number, meaning it is not Infinity or -Infinity. It also ensures that the provided value is of the type number or a numeric string.
💡 Syntax
The syntax for the _.isFinite()
method is straightforward:
_.isFinite(value)
- value: The value to check for finiteness.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isFinite()
method:
const _ = require('lodash');
const finiteNumber = 42;
const infiniteNumber = Infinity;
const numericString = '123';
console.log(_.isFinite(finiteNumber)); // Output: true
console.log(_.isFinite(infiniteNumber)); // Output: false
console.log(_.isFinite(numericString)); // Output: true
In this example, _.isFinite()
is applied to different values, demonstrating its ability to distinguish between finite numbers, infinite numbers, and valid numeric strings.
🏆 Best Practices
When working with the _.isFinite()
method, consider the following best practices:
Validate User Input:
When dealing with user input that involves numeric data, use
_.isFinite()
to validate whether the input is a valid finite number before processing it further.example.jsCopiedconst userInput = /* ...retrieve user input... */ ; if (_.isFinite(userInput)) { // Process the valid finite number console.log('Processing input:', userInput); } else { // Handle invalid input console.error('Invalid numeric input'); }
Prevent Division by Zero:
Before performing division operations, ensure that the divisor is a finite and non-zero number to prevent potential issues like division by zero.
example.jsCopiedconst numerator = 10; const divisor = /* ...retrieve divisor... */ ; if (_.isFinite(divisor) && divisor !== 0) { const result = numerator / divisor; console.log('Result of division:', result); } else { console.error('Invalid divisor for division operation'); }
Check Numeric Values in Arrays:
When working with arrays containing numeric values, use
_.isFinite()
to filter out non-finite numbers and ensure the integrity of your data.example.jsCopiedconst numericArray = [1, 2, '3', 4, Infinity, 'five']; const finiteNumbers = _.filter(numericArray, _.isFinite); console.log(finiteNumbers); // Output: [1, 2, '3', 4]
📚 Use Cases
Form Validation:
In web development, when validating form inputs that expect numeric values,
_.isFinite()
can be employed to ensure that the entered data is a valid finite number.example.jsCopiedconst submittedFormData = /* ...retrieve form data... */; const ageInput = submittedFormData.age; if (_.isFinite(ageInput)) { // Process the valid age input console.log("User's age is valid:", ageInput); } else { // Handle invalid age input console.error('Invalid age input'); }
Financial Calculations:
When performing financial calculations, it's crucial to validate the numerical inputs to avoid errors that may arise from non-finite or unexpected values.
example.jsCopiedconst principalAmount = /* ...retrieve principal amount... */ ; const interestRate = /* ...retrieve interest rate... */ ; if (_.isFinite(principalAmount) && _.isFinite(interestRate)) { // Perform financial calculations with valid inputs const totalAmount = principalAmount * (1 + interestRate); console.log('Total amount after interest:', totalAmount); } else { console.error('Invalid input for financial calculation'); }
Filtering Numeric Data:
When working with datasets, use
_.isFinite()
to filter out non-finite numbers and ensure that only valid numeric values are included in your analysis or computations.example.jsCopiedconst dataset = [42, 56, 'invalid', Infinity, 78, 'NaN']; const validNumericData = _.filter(dataset, _.isFinite); console.log(validNumericData); // Output: [42, 56, 78]
🎉 Conclusion
The _.isFinite()
method in Lodash is a valuable tool for JavaScript developers, offering a straightforward way to check the finiteness of numeric values. By incorporating this method into your code, you can enhance the reliability and robustness of applications that involve numeric data.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isFinite()
method in your Lodash projects.
👨💻 Join our Community:
Author
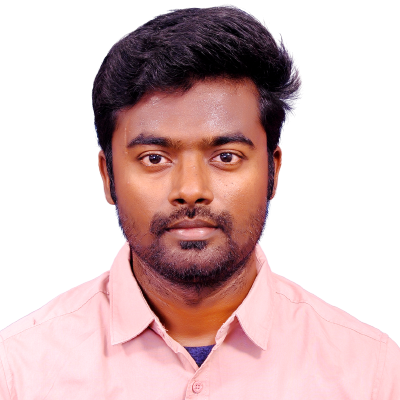
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
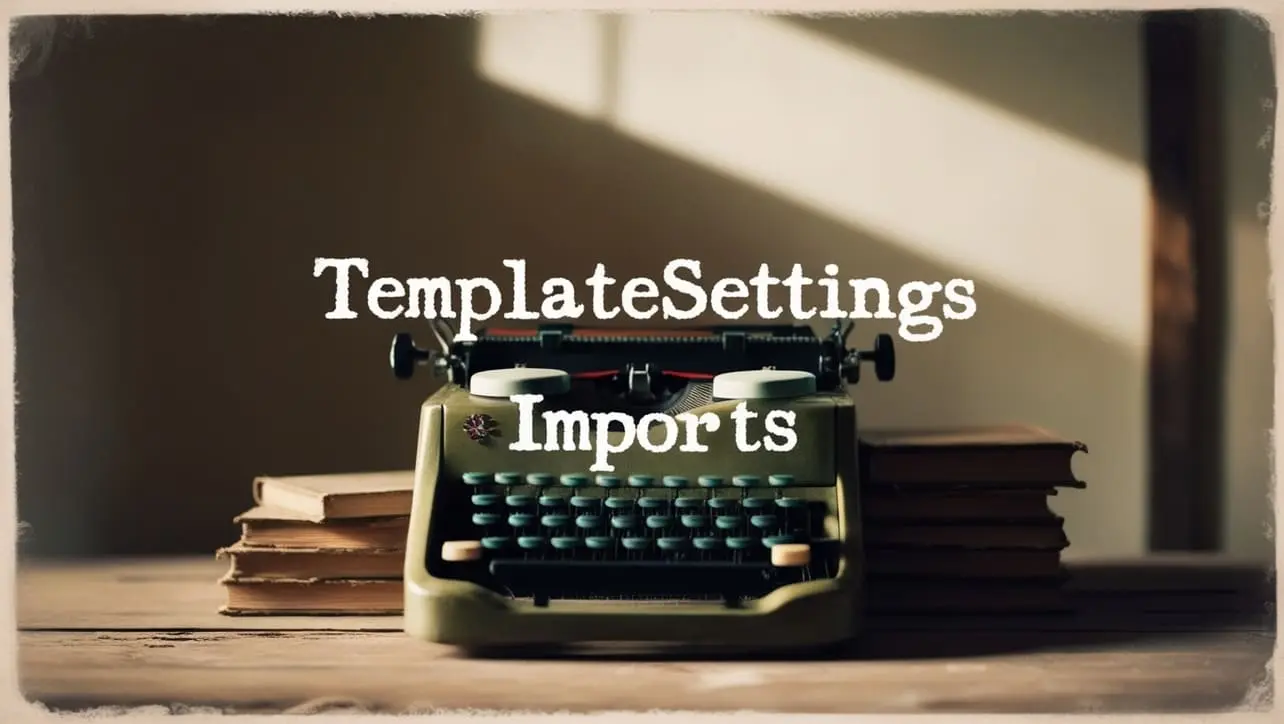
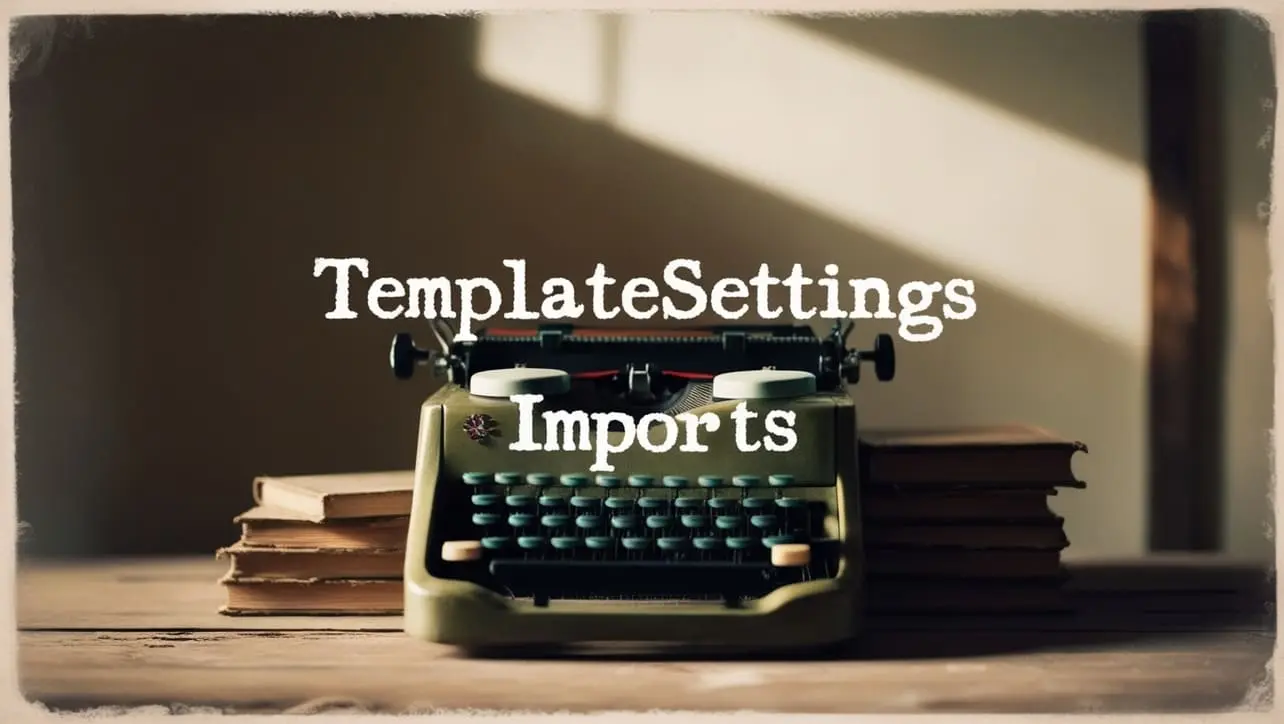
Lodash _.templateSettings.imports Property
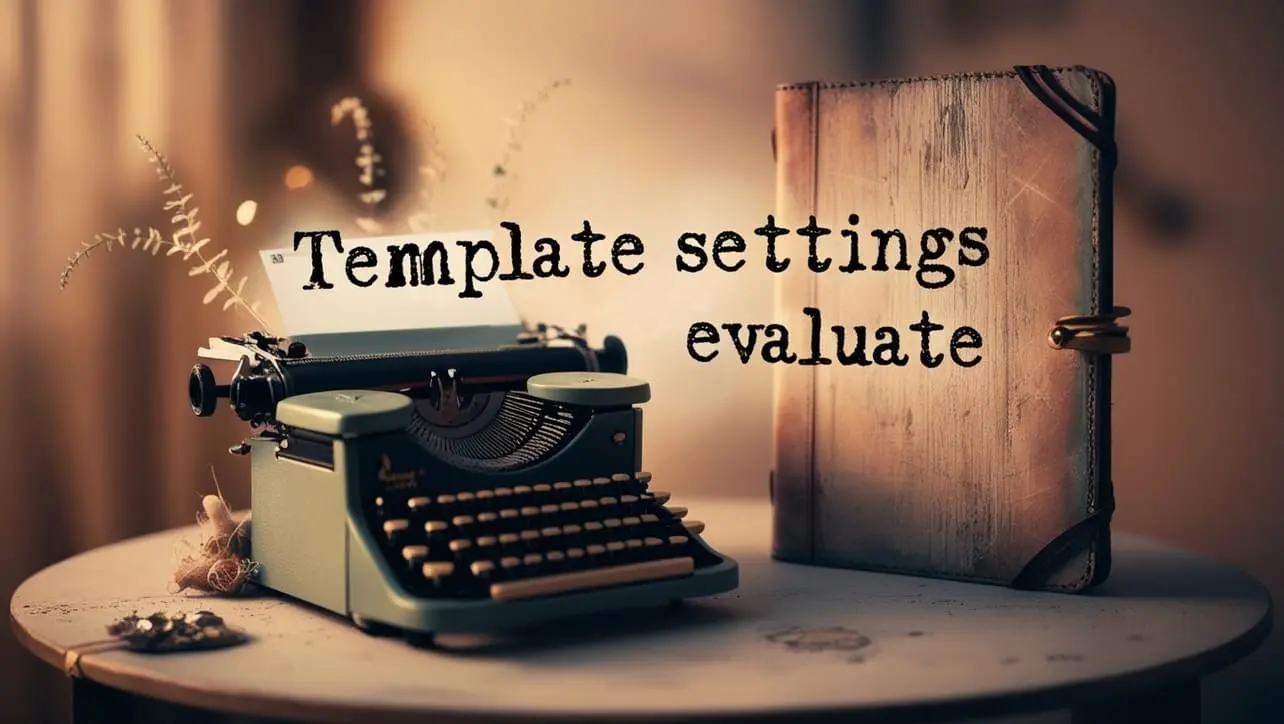
Lodash _.templateSettings.evaluate Property
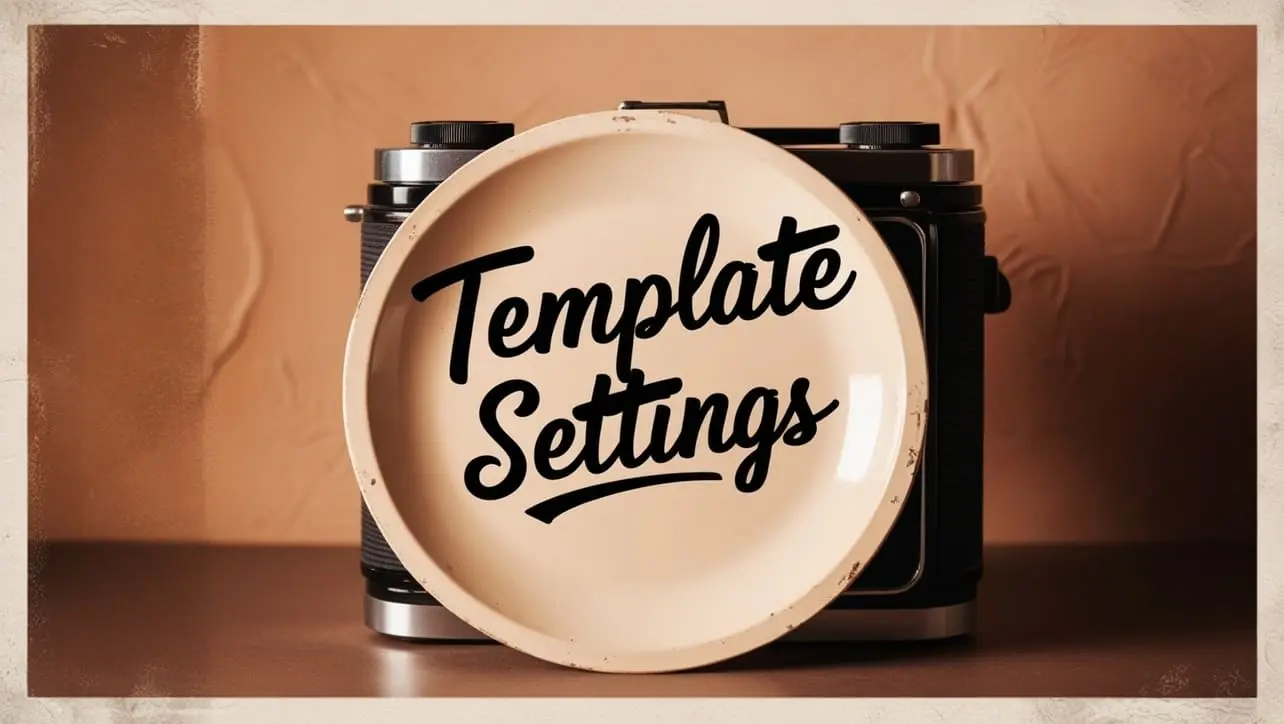
Lodash _.templateSettings Property
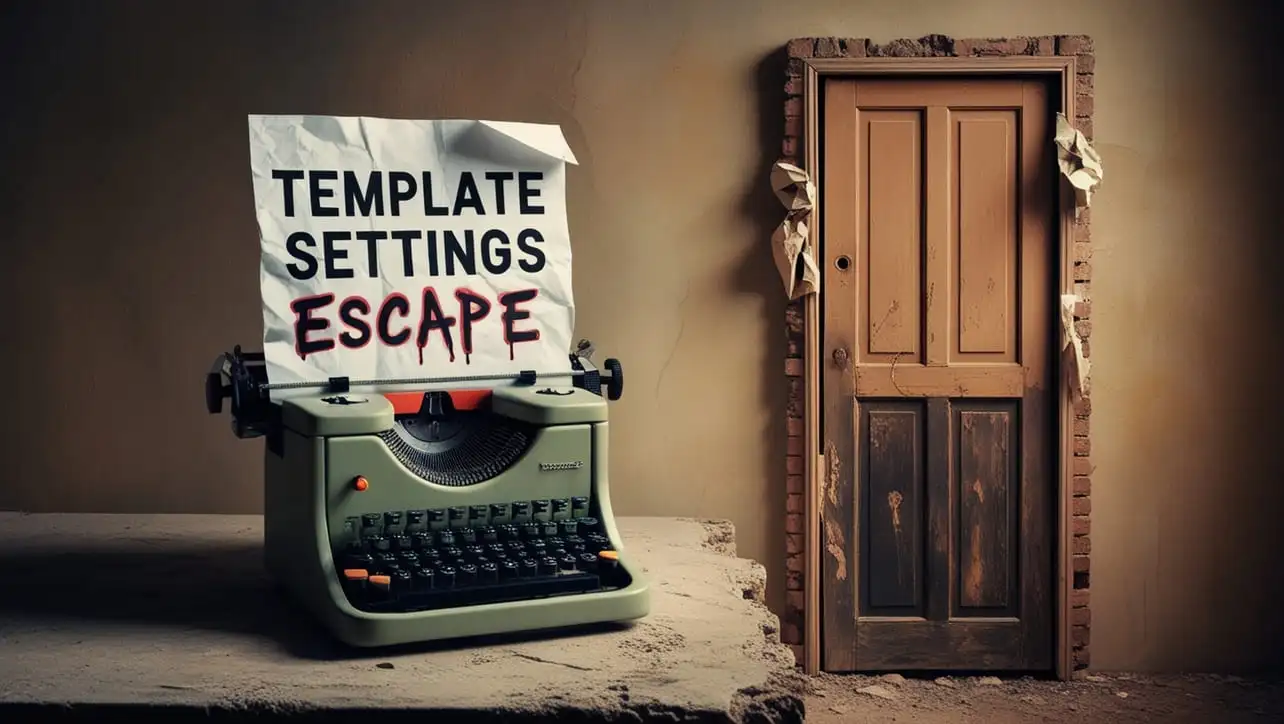
Lodash _.templateSettings.escape Property
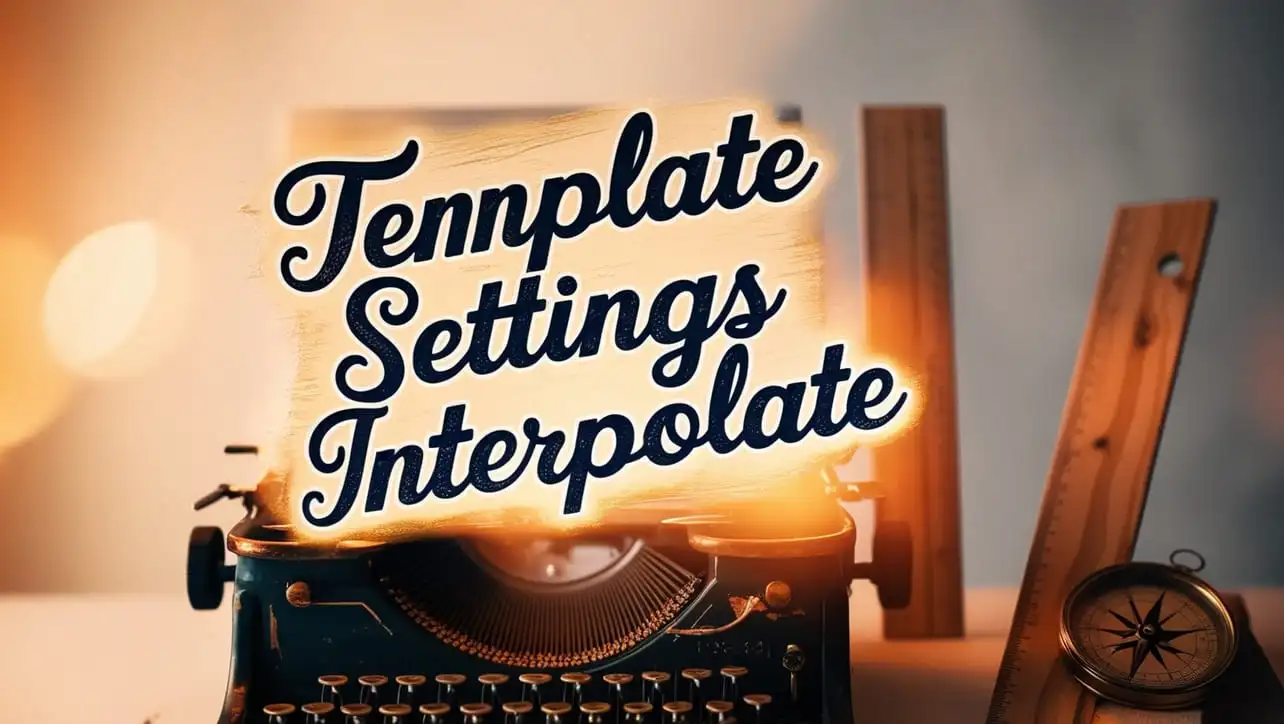
Lodash _.templateSettings.interpolate Property
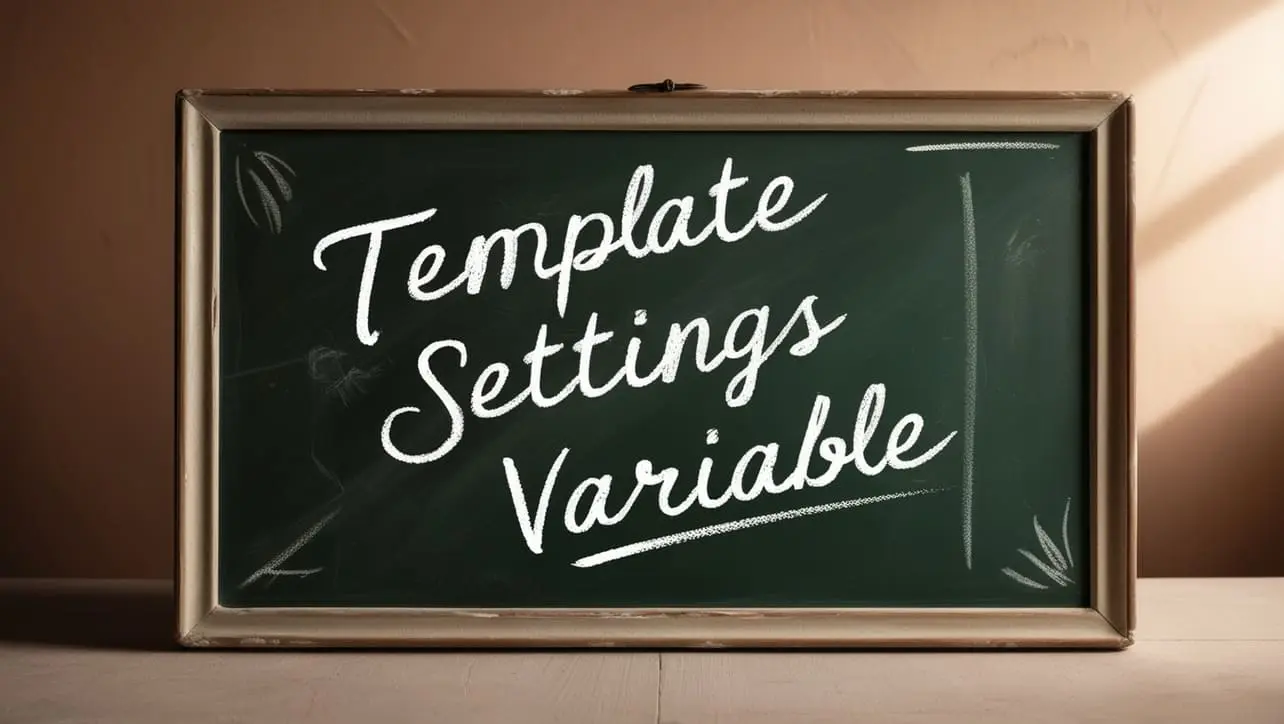
If you have any doubts regarding this article (Lodash _.isFinite() Lang Method), please comment here. I will help you immediately.