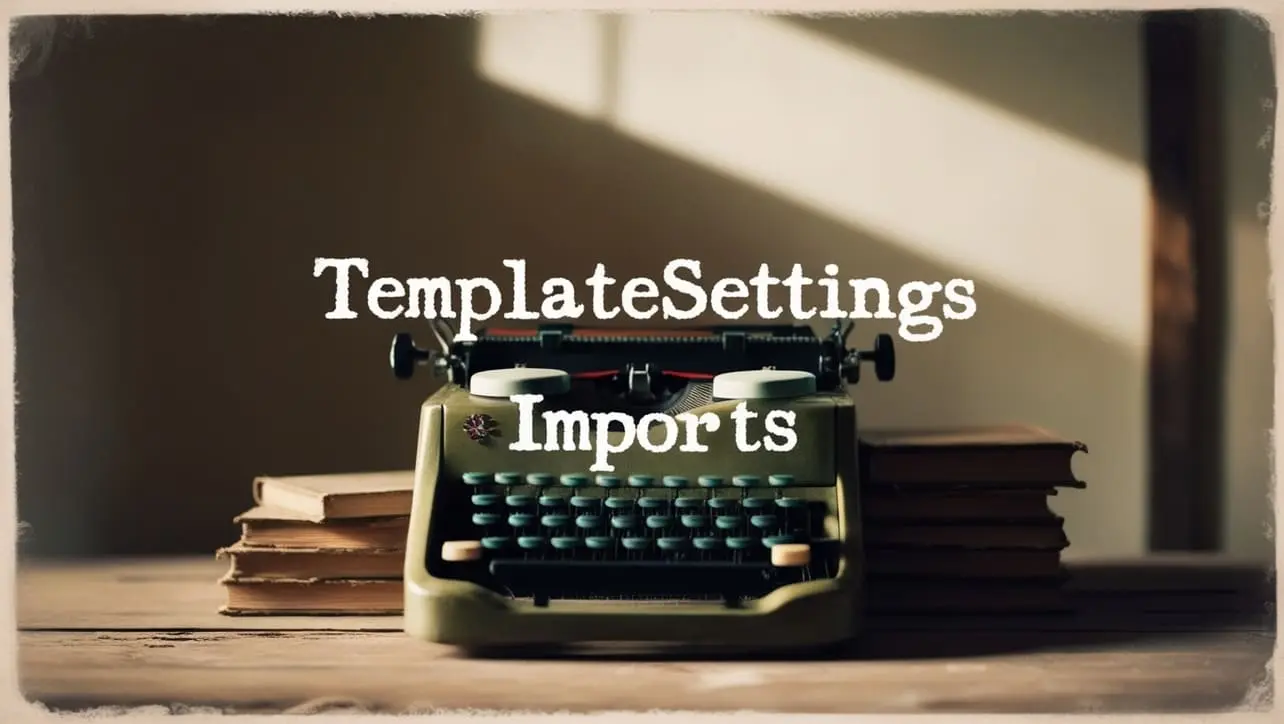
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isEqual() Lang Method
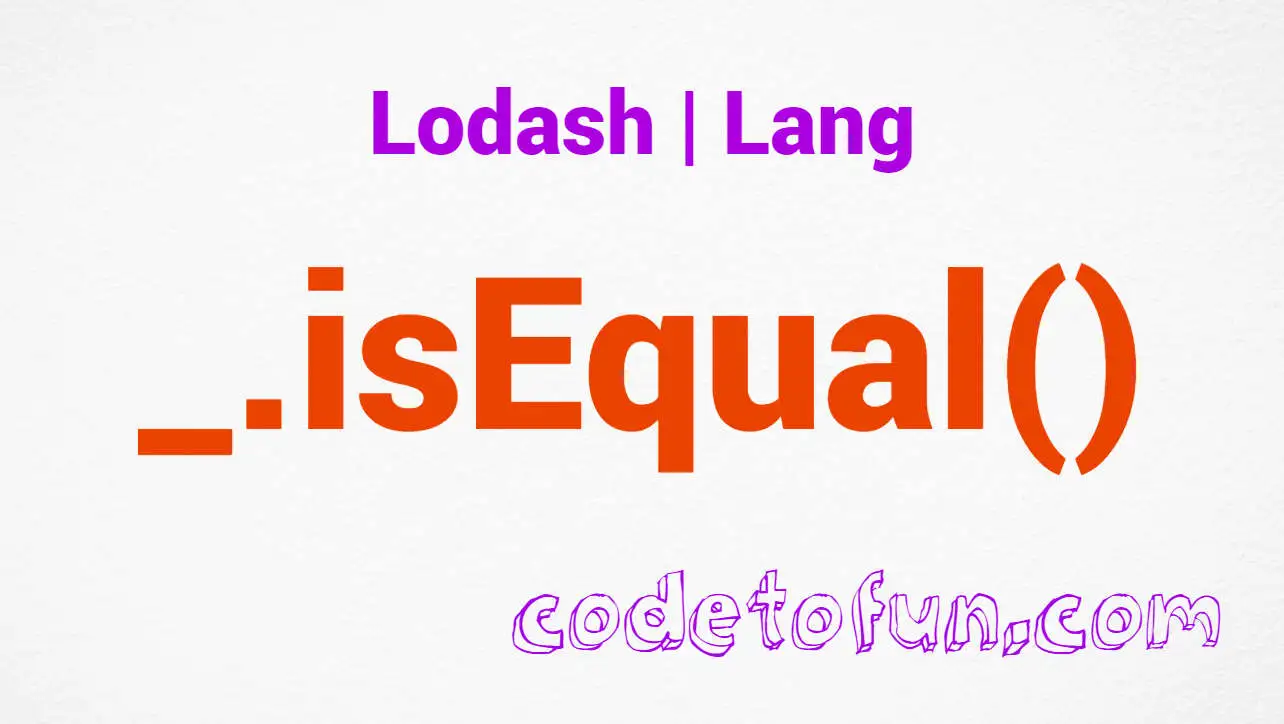
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, comparing objects and values is a common task. Lodash, a powerful utility library, provides the _.isEqual()
method to simplify and streamline the process of deep equality checks.
This method proves invaluable when dealing with complex data structures, ensuring accurate comparisons and enhancing the reliability of your code.
🧠 Understanding _.isEqual() Method
The _.isEqual()
method in Lodash is designed for deep equality checks between two values. Whether you're comparing simple values, arrays, or nested objects, this method traverses the structure to validate equality at every level. Its versatility and accuracy make it a go-to choice for developers who require robust equality comparisons in their projects.
💡 Syntax
The syntax for the _.isEqual()
method is straightforward:
_.isEqual(value, other)
- value: The value to compare.
- other: The other value to compare.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isEqual()
method:
const _ = require('lodash');
const obj1 = {
a: 1,
b: {
c: 2
}
};
const obj2 = {
a: 1,
b: {
c: 2
}
};
const isEqual = _.isEqual(obj1, obj2);
console.log(isEqual);
// Output: true
In this example, obj1 and obj2 are deeply compared using _.isEqual()
, and the result is true as both objects have the same structure and values.
🏆 Best Practices
When working with the _.isEqual()
method, consider the following best practices:
Deep Comparison:
Leverage
_.isEqual()
for deep comparison of objects and arrays. This ensures that nested structures are thoroughly examined for equality.example.jsCopiedconst array1 = [1, 2, { a: 3, b: { c: 4 } }]; const array2 = [1, 2, { a: 3, b: { c: 4 } }]; const isEqualArrays = _.isEqual(array1, array2); console.log(isEqualArrays); // Output: true
Handling Circular References:
_.isEqual()
gracefully handles circular references, making it suitable for comparing objects with complex interdependencies.example.jsCopiedconst circularObj1 = { a: 1 }; const circularObj2 = { a: 1 }; circularObj1.b = circularObj1; circularObj2.b = circularObj2; const isEqualCircular = _.isEqual(circularObj1, circularObj2); console.log(isEqualCircular); // Output: true
Customizing Comparison Logic:
For advanced scenarios, you can customize the comparison logic using _.isEqualWith().
example.jsCopiedconst customComparison = (value, other) => { // Custom logic for comparison return /* result of comparison */; }; const customEqual = _.isEqualWith(obj1, obj2, customComparison); console.log(customEqual);
📚 Use Cases
State Comparison in React:
In React applications,
_.isEqual()
can be utilized to compare state objects, ensuring efficient updates and preventing unnecessary re-renders.example.jsCopiedclass MyComponent extends React.Component { state = /* ...initial state... */ ; componentDidUpdate(prevProps, prevState) { if (!_.isEqual(this.state, prevState)) { // State has changed, perform necessary actions } } // ... rest of the component ... }
Unit Testing:
When writing unit tests,
_.isEqual()
is handy for comparing expected and actual results, especially when dealing with complex data structures.example.jsCopiedconst expectedData = /* ...expected data... */; const actualData = /* ...function result or received data... */; const testResult = _.isEqual(expectedData, actualData); console.log(testResult);
Merging State in Redux:
In a Redux environment,
_.isEqual()
can aid in determining whether the state has changed, helping optimize the implementation of reducers.example.jsCopiedconst rootReducer = (state, action) => { switch (action.type) { case 'UPDATE_DATA': if (_.isEqual(state.data, action.payload)) { return state; // No need to create a new state if the data is the same } return { ...state, data: action.payload }; // ... other cases ... } };
🎉 Conclusion
The _.isEqual()
method in Lodash is a versatile tool for deep equality comparisons in JavaScript. Whether you're working with React state, conducting unit tests, or managing complex data structures, this method provides a reliable solution for ensuring equality. Embrace the power of deep comparison with _.isEqual()
to enhance the accuracy and robustness of your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isEqual()
method in your Lodash projects.
👨💻 Join our Community:
Author
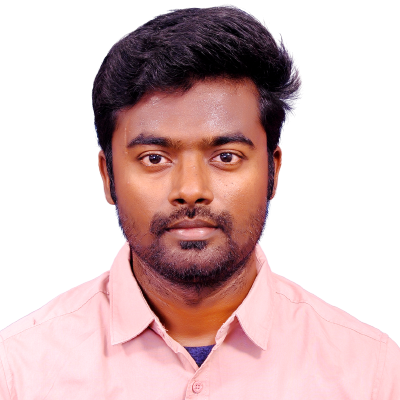
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
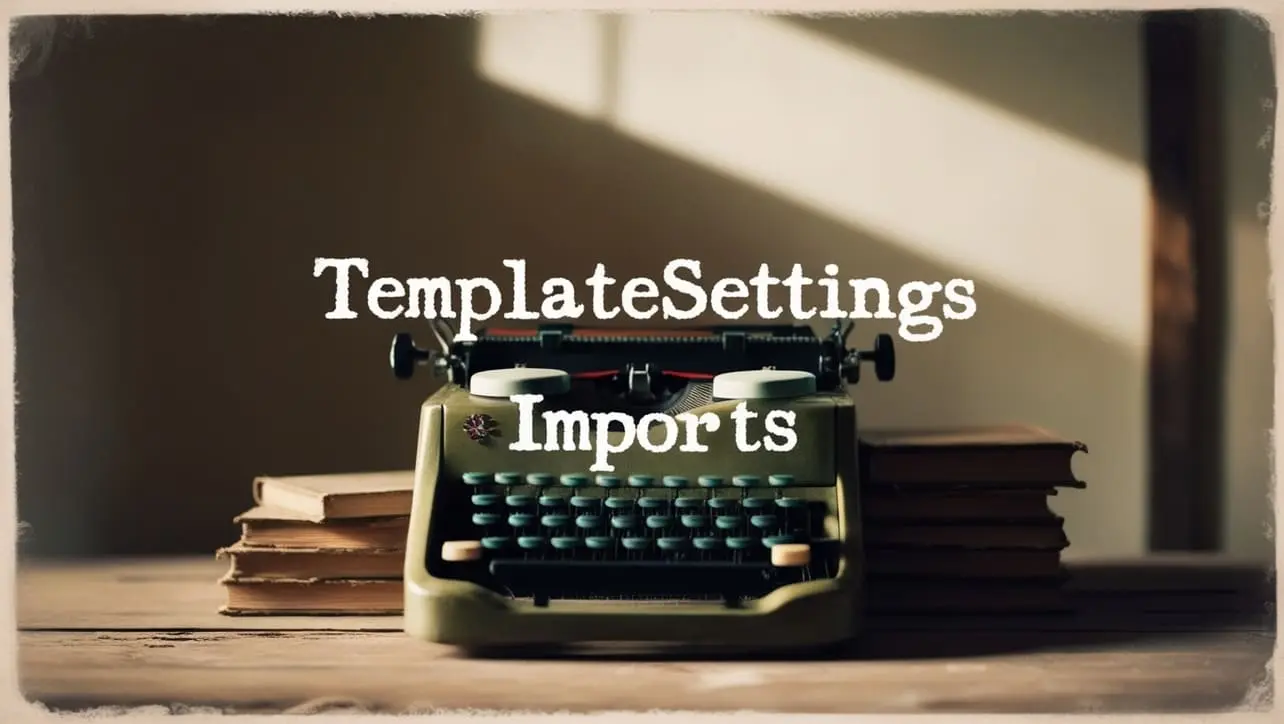
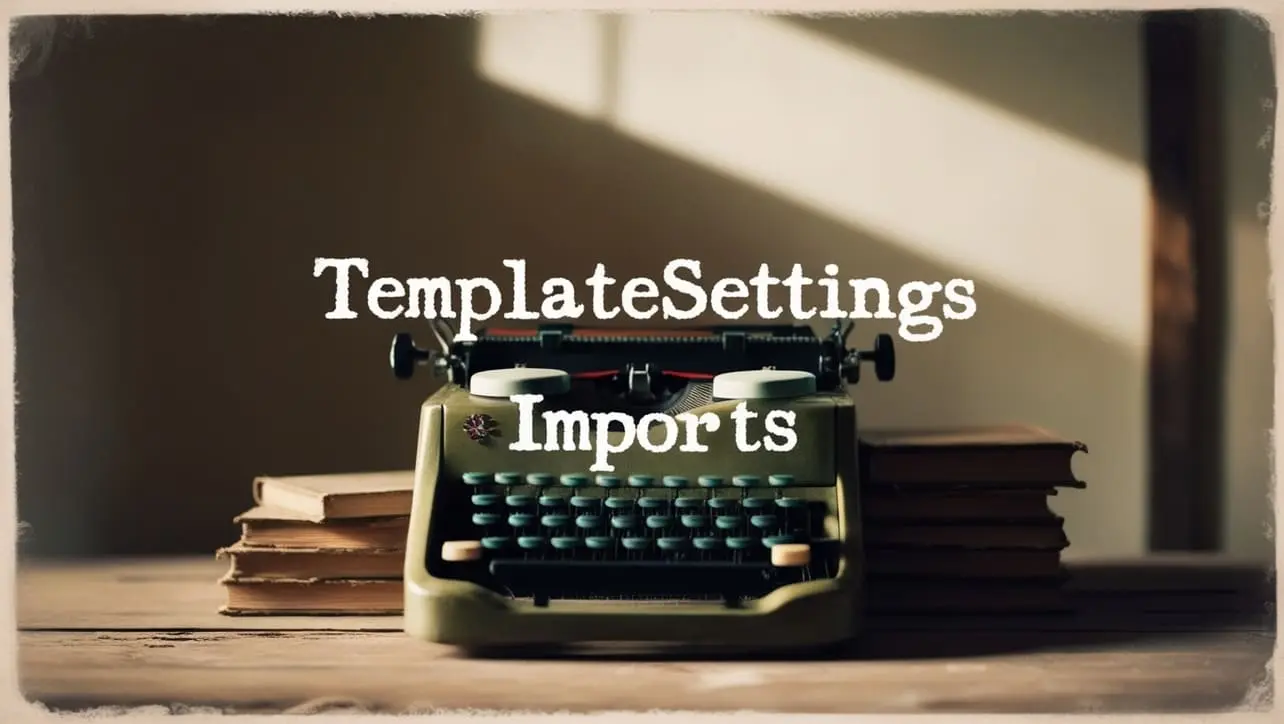
Lodash _.templateSettings.imports Property
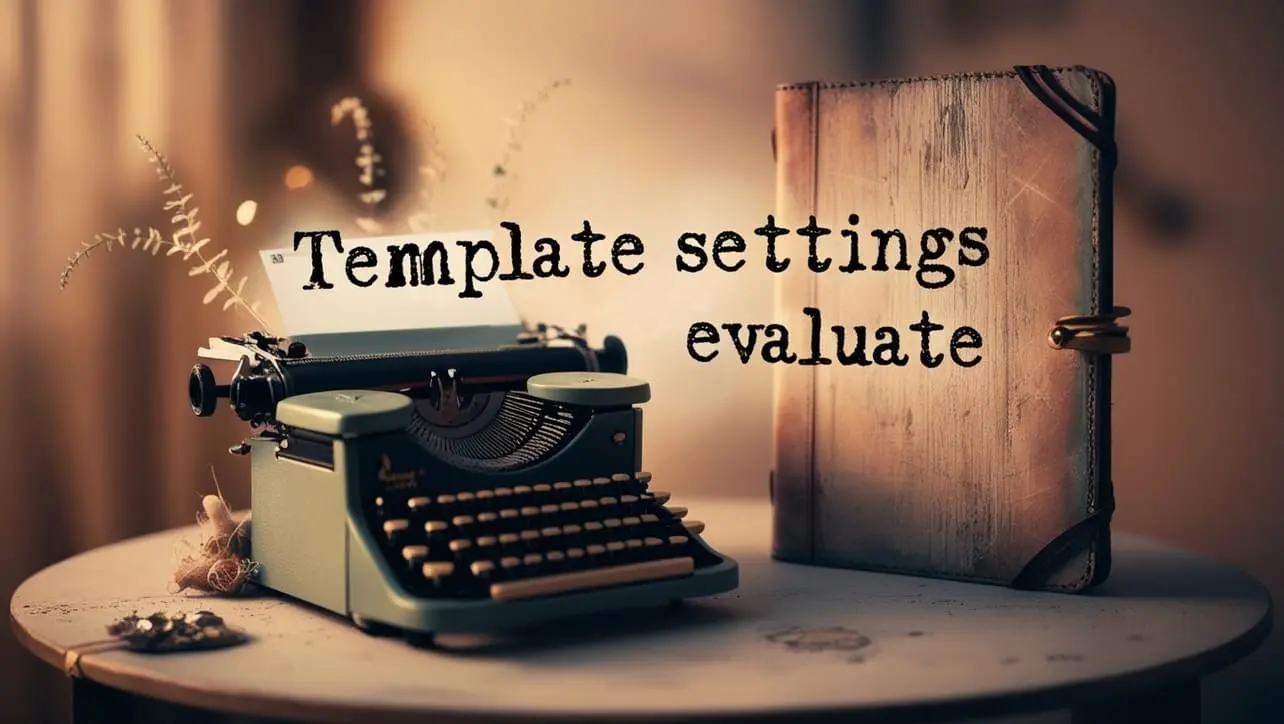
Lodash _.templateSettings.evaluate Property
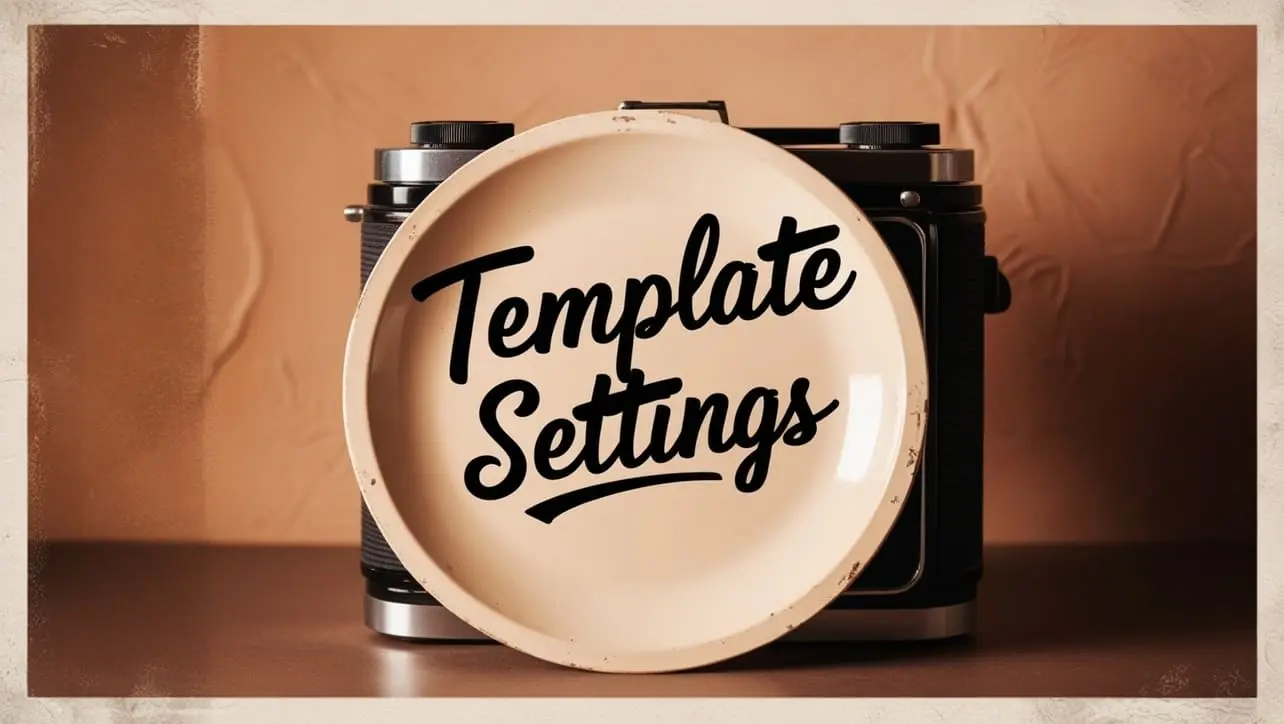
Lodash _.templateSettings Property
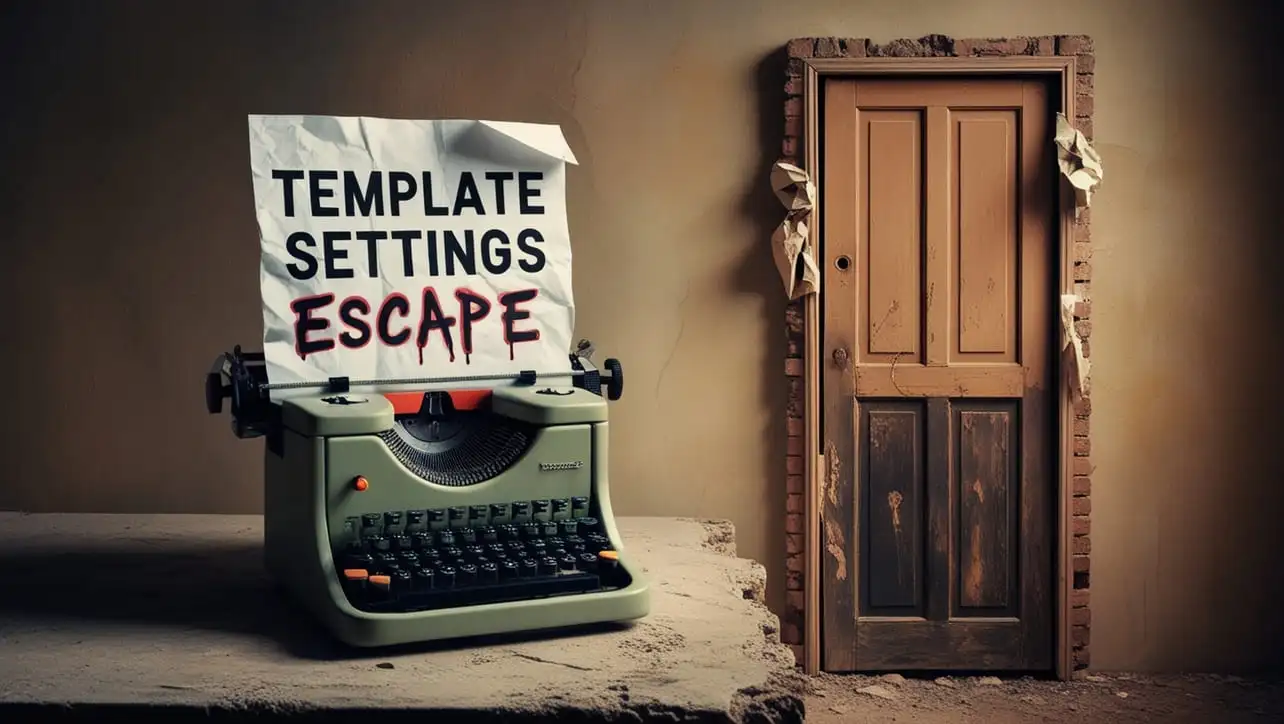
Lodash _.templateSettings.escape Property
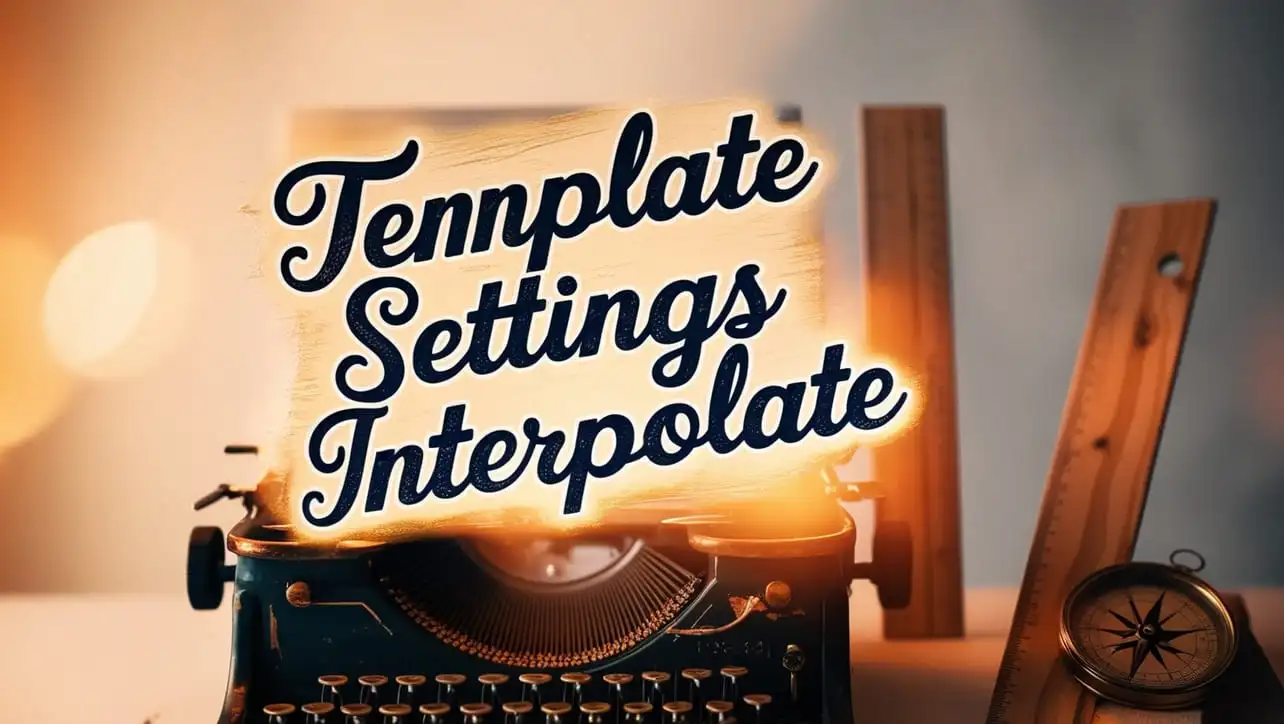
Lodash _.templateSettings.interpolate Property
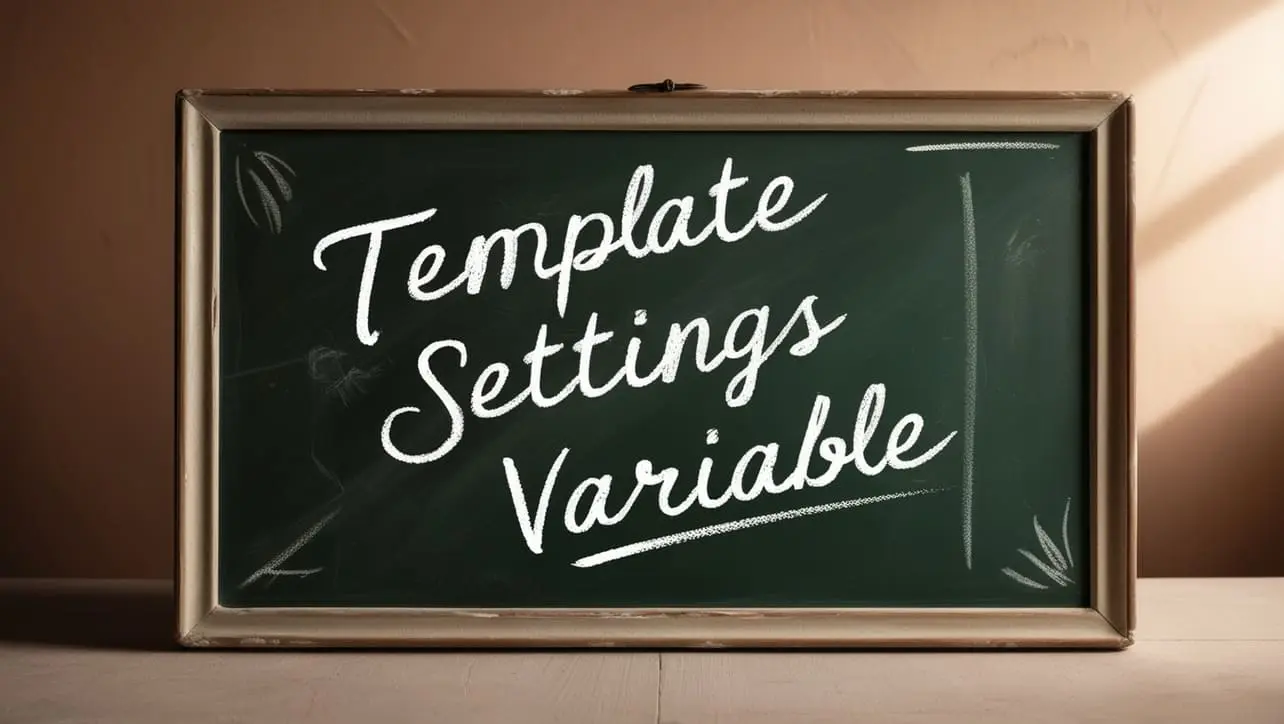
If you have any doubts regarding this article (Lodash _.isEqual() Lang Method), please comment here. I will help you immediately.