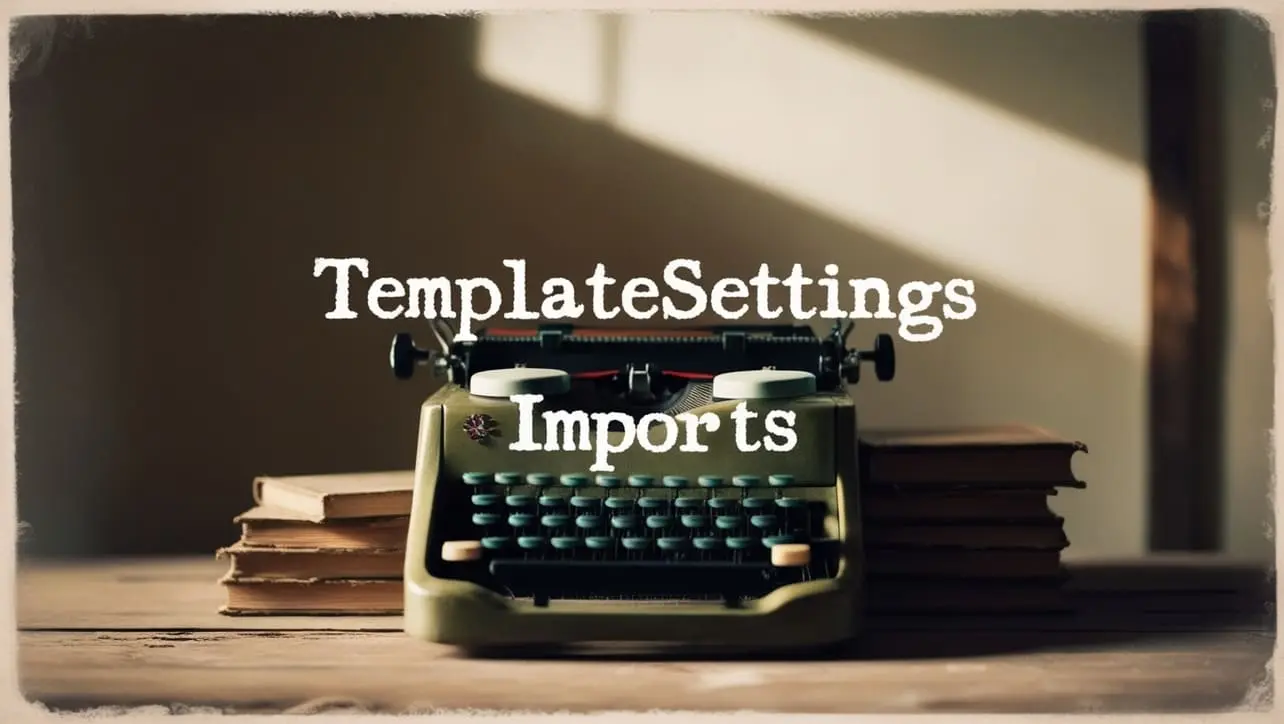
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isBuffer() Lang Method
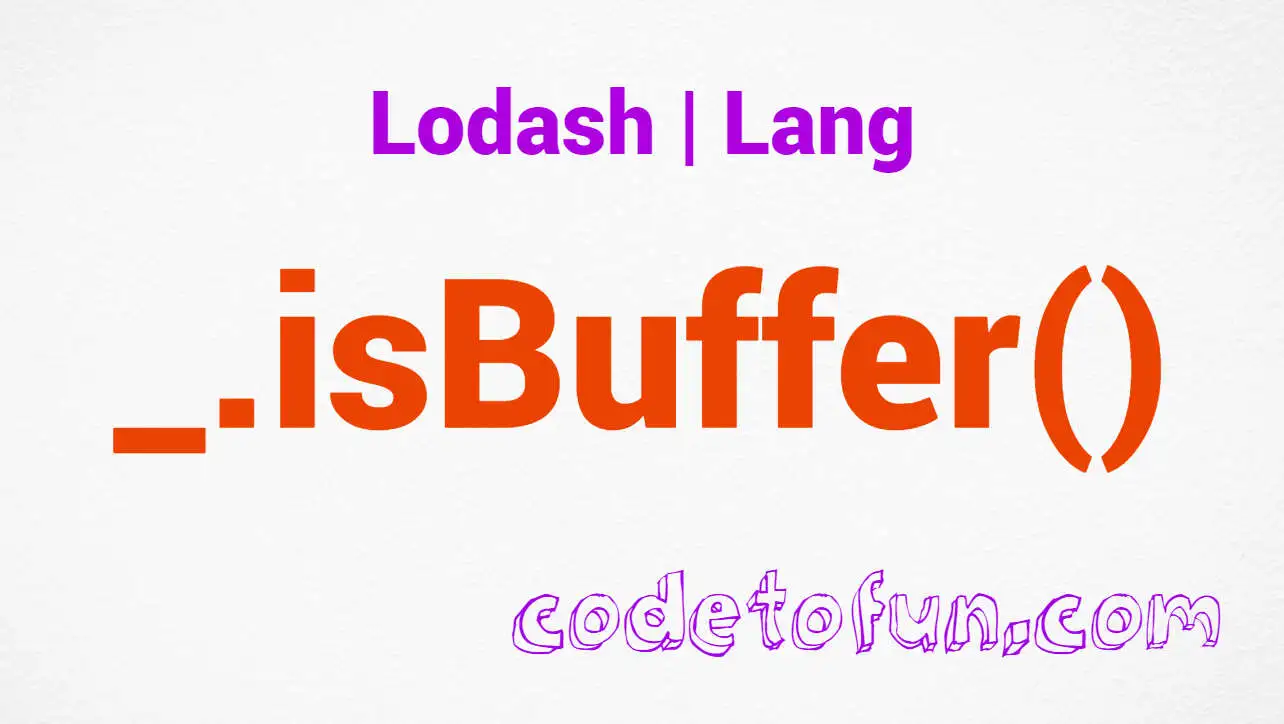
Photo Credit to CodeToFun
🙋 Introduction
When working with data in JavaScript, it's essential to have tools that help you identify the nature of your variables. In the vast landscape of utility libraries, Lodash stands out, providing developers with functions that simplify common tasks.
One such function is _.isBuffer()
, a method designed to determine whether a given value is a Buffer object. This method is particularly useful in scenarios involving binary data and stream processing.
🧠 Understanding _.isBuffer() Method
The _.isBuffer()
method in Lodash serves a simple yet crucial purpose – it checks if a given value is a Buffer object.
💡 Syntax
The syntax for the _.isBuffer()
method is straightforward:
_.isBuffer(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isBuffer()
method:
const _ = require('lodash');
const bufferData = Buffer.from('Hello, Lodash!');
const isBuffer = _.isBuffer(bufferData);
console.log(isBuffer);
// Output: true
In this example, bufferData is a Buffer object, and _.isBuffer()
correctly identifies it as such.
🏆 Best Practices
When working with the _.isBuffer()
method, consider the following best practices:
Validating Buffer Objects:
Use
_.isBuffer()
to validate whether a variable is a Buffer object before performing operations specific to binary data. This helps prevent errors and ensures that your code works as intended.example.jsCopiedconst potentialBuffer = /* ...some data... */; if (_.isBuffer(potentialBuffer)) { // Perform buffer-specific operations console.log('Valid Buffer object:', potentialBuffer); } else { console.log('Not a Buffer object:', potentialBuffer); }
Safeguarding Binary Operations:
Before engaging in binary operations or dealing with raw data, employ
_.isBuffer()
to confirm that the variable is indeed a Buffer. This practice enhances the robustness of your code.example.jsCopiedconst data = /* ...some data... */; if (_.isBuffer(data)) { // Perform binary operations safely const reversedData = Buffer.from(data).reverse(); console.log('Reversed Buffer data:', reversedData); } else { console.log('Not a Buffer object. Unable to perform binary operations.'); }
Handling User Input:
When dealing with user input or external data sources, use
_.isBuffer()
to validate that the received data conforms to the expected Buffer format. This helps prevent potential security vulnerabilities.example.jsCopiedconst userInput = /* ...user input or external data... */; if (_.isBuffer(userInput)) { // Process the Buffer data securely console.log('Received valid Buffer data:', userInput); } else { console.error('Invalid input. Expected Buffer data.'); }
📚 Use Cases
Binary Data Processing:
_.isBuffer()
is invaluable when working with binary data, ensuring that your code only processes data in the expected Buffer format.example.jsCopiedconst rawData = /* ...raw binary data... */; if (_.isBuffer(rawData)) { // Process binary data securely const processedData = /* ...your binary processing logic... */; console.log('Processed binary data:', processedData); } else { console.error('Invalid binary data. Expected Buffer format.'); }
Stream Handling:
When dealing with streams and file I/O,
_.isBuffer()
helps you validate whether the incoming data is in Buffer format before further processing.example.jsCopiedconst incomingStreamData = /* ...data from a stream... */; if (_.isBuffer(incomingStreamData)) { // Handle stream data safely console.log('Received valid Buffer data from the stream:', incomingStreamData); } else { console.error('Invalid stream data. Expected Buffer format.'); }
Type Checking in Functions:
In functions that expect Buffer input, use
_.isBuffer()
to perform type checking and ensure the correct data format.example.jsCopiedfunction processData(bufferInput) { if (_.isBuffer(bufferInput)) { // Process the Buffer data console.log('Processing Buffer data:', bufferInput); } else { console.error('Invalid input. Expected Buffer data.'); } } const inputData = /* ...some data... */; processData(inputData);
🎉 Conclusion
The _.isBuffer()
method in Lodash serves as a reliable tool for identifying Buffer objects in JavaScript. By incorporating it into your code, you can ensure data integrity, enhance security, and streamline operations involving binary data.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isBuffer()
method in your Lodash projects.
👨💻 Join our Community:
Author
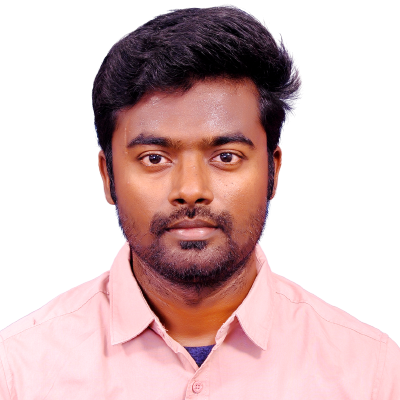
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
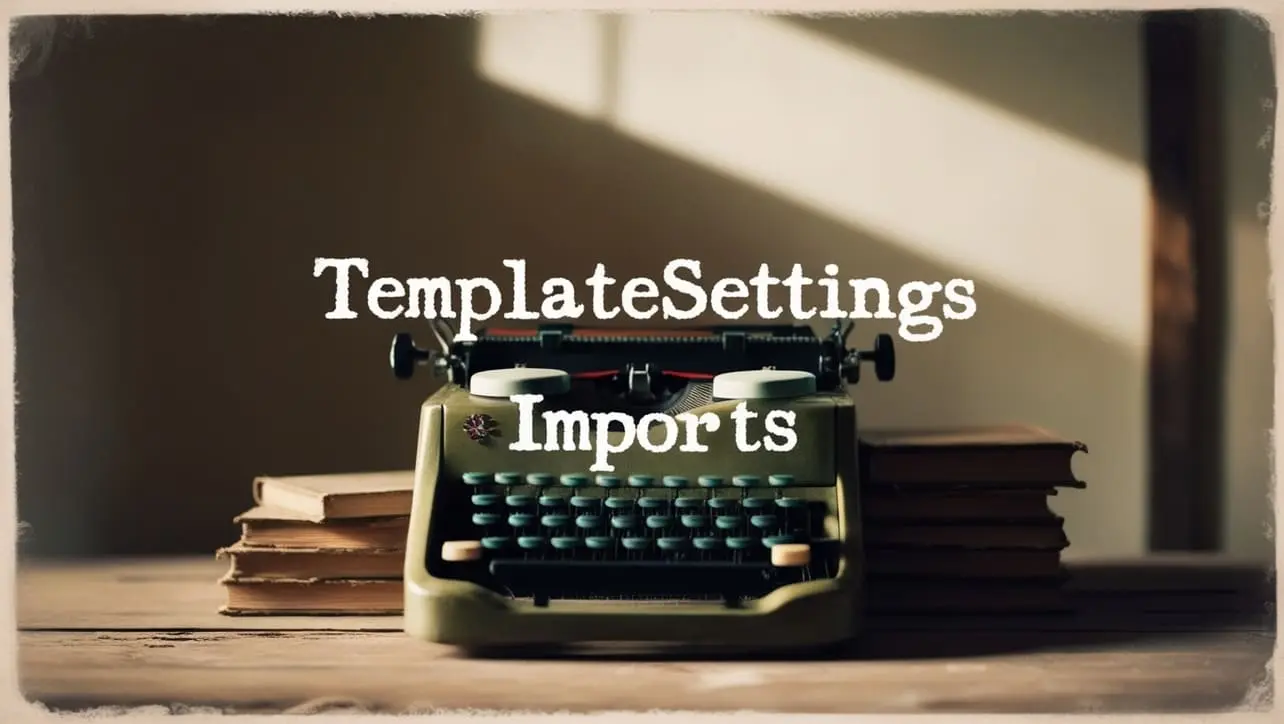
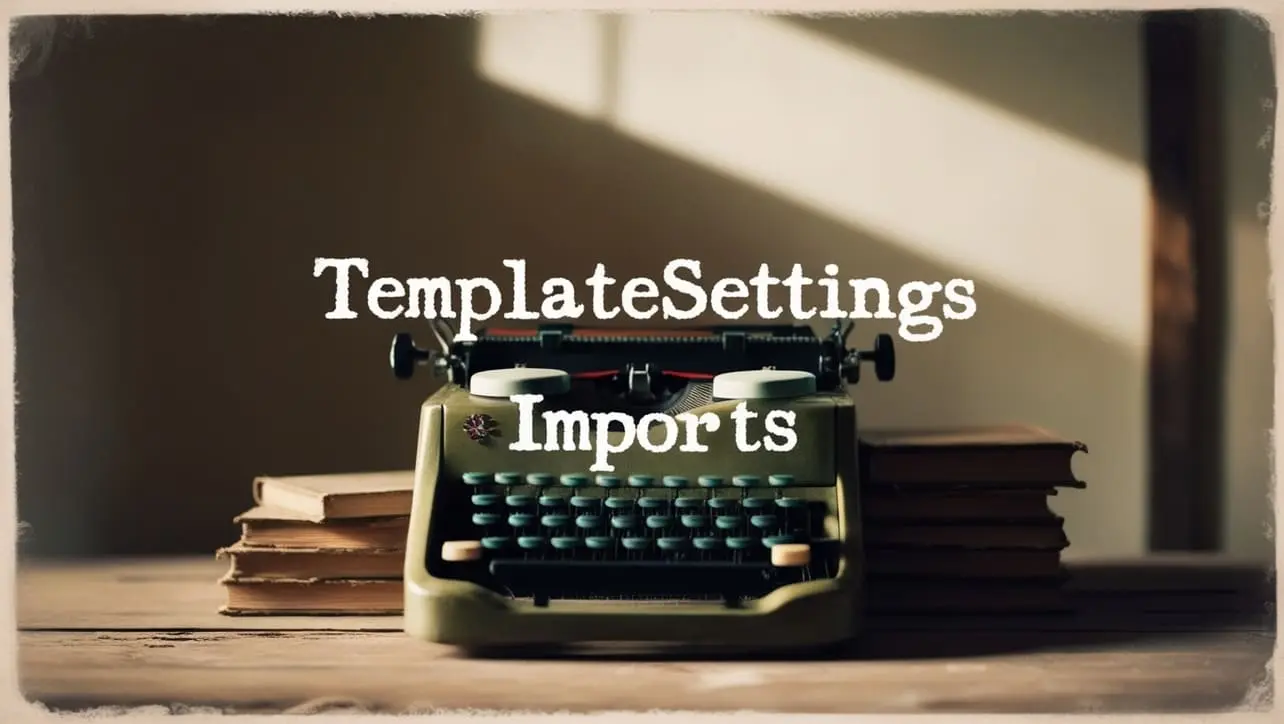
Lodash _.templateSettings.imports Property
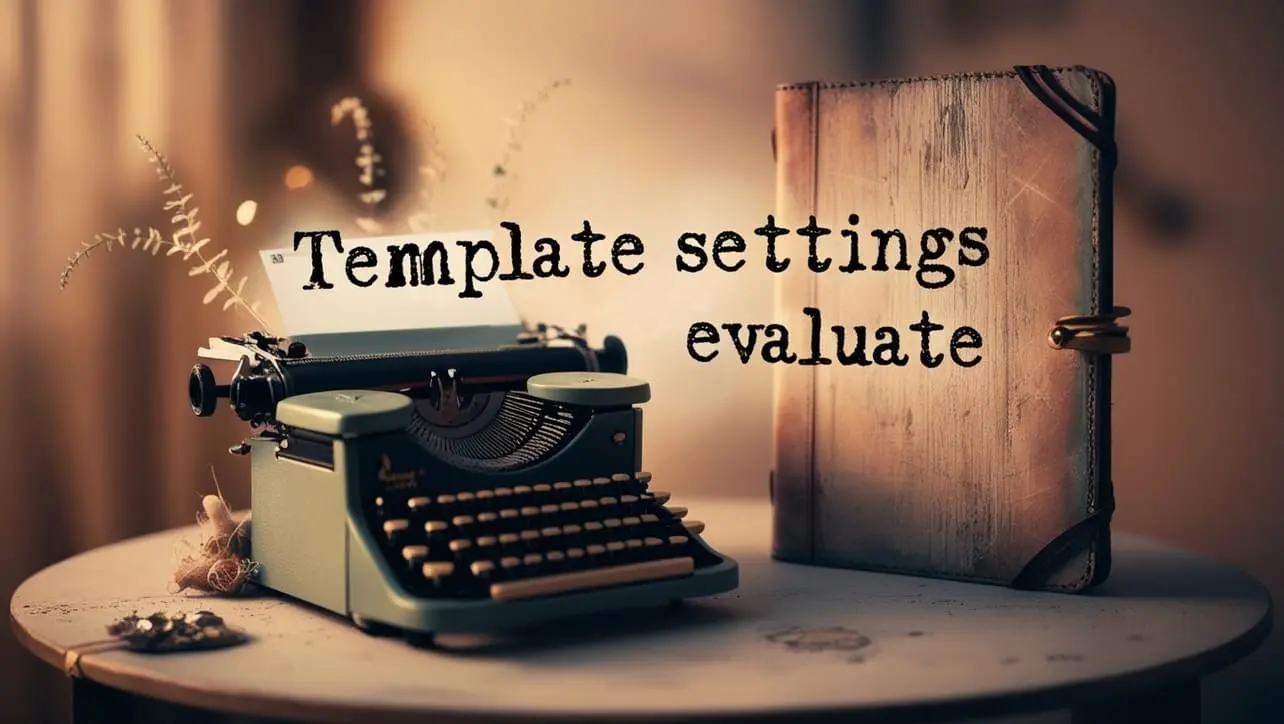
Lodash _.templateSettings.evaluate Property
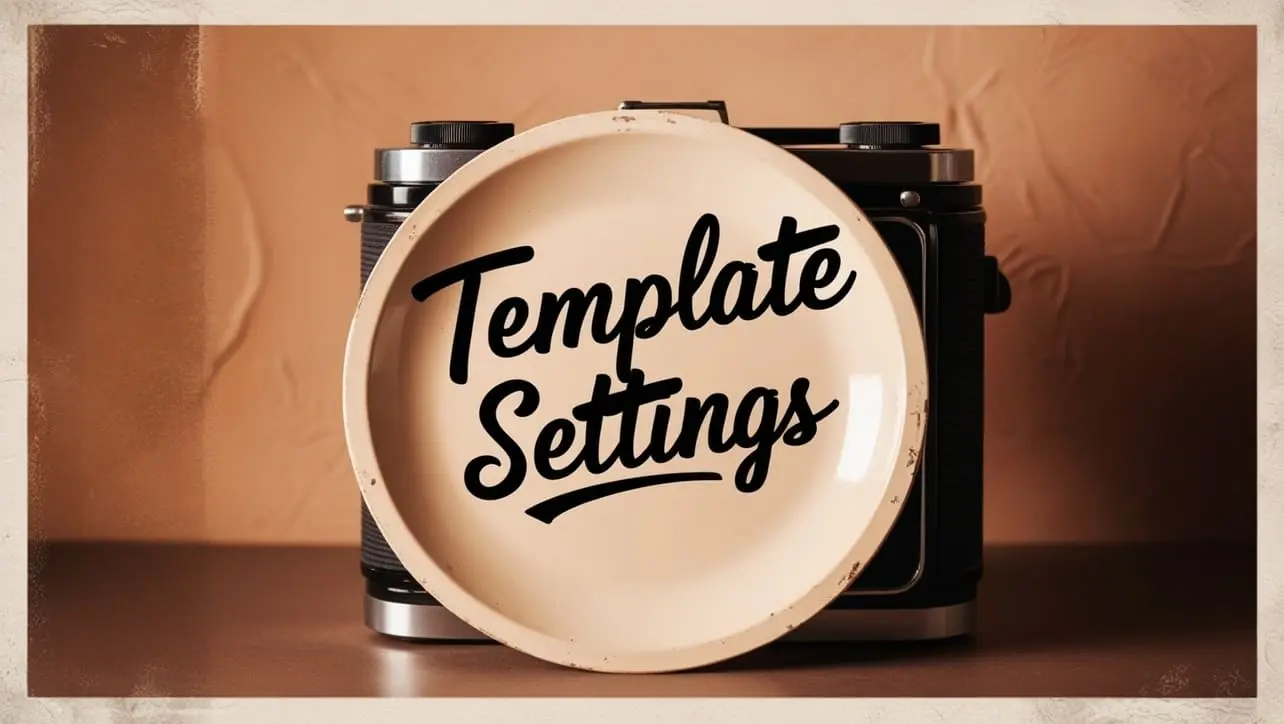
Lodash _.templateSettings Property
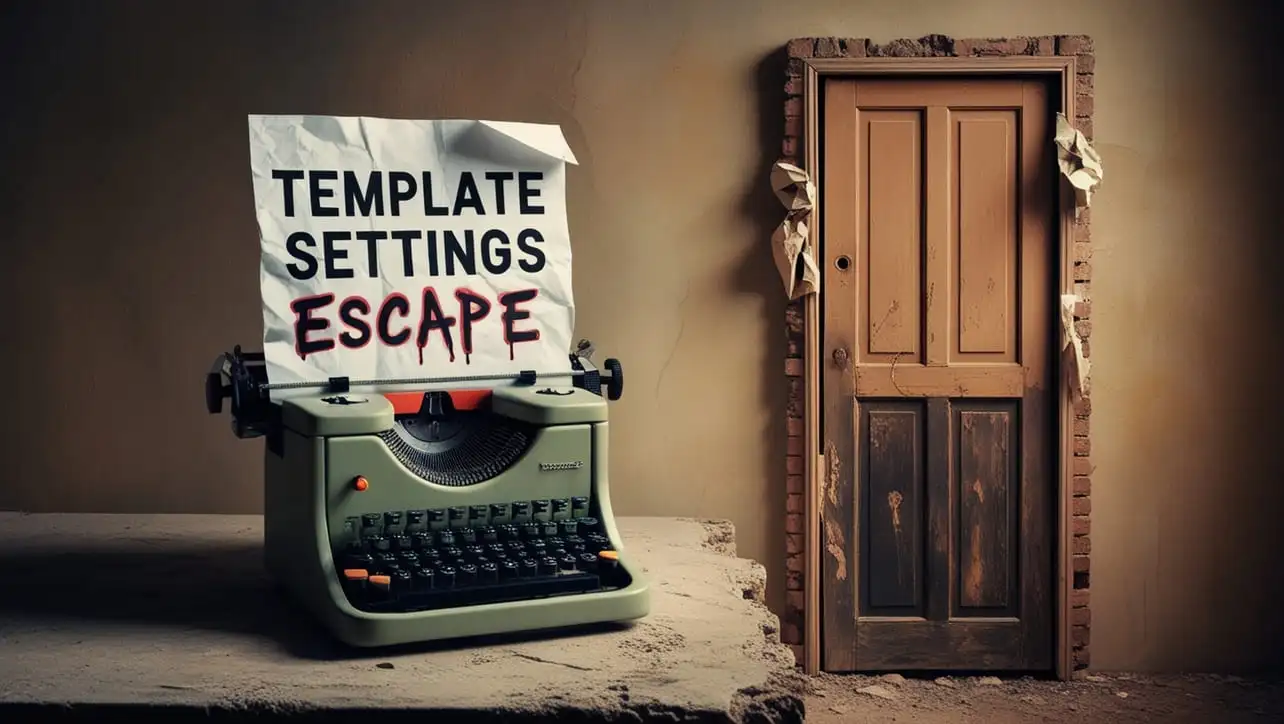
Lodash _.templateSettings.escape Property
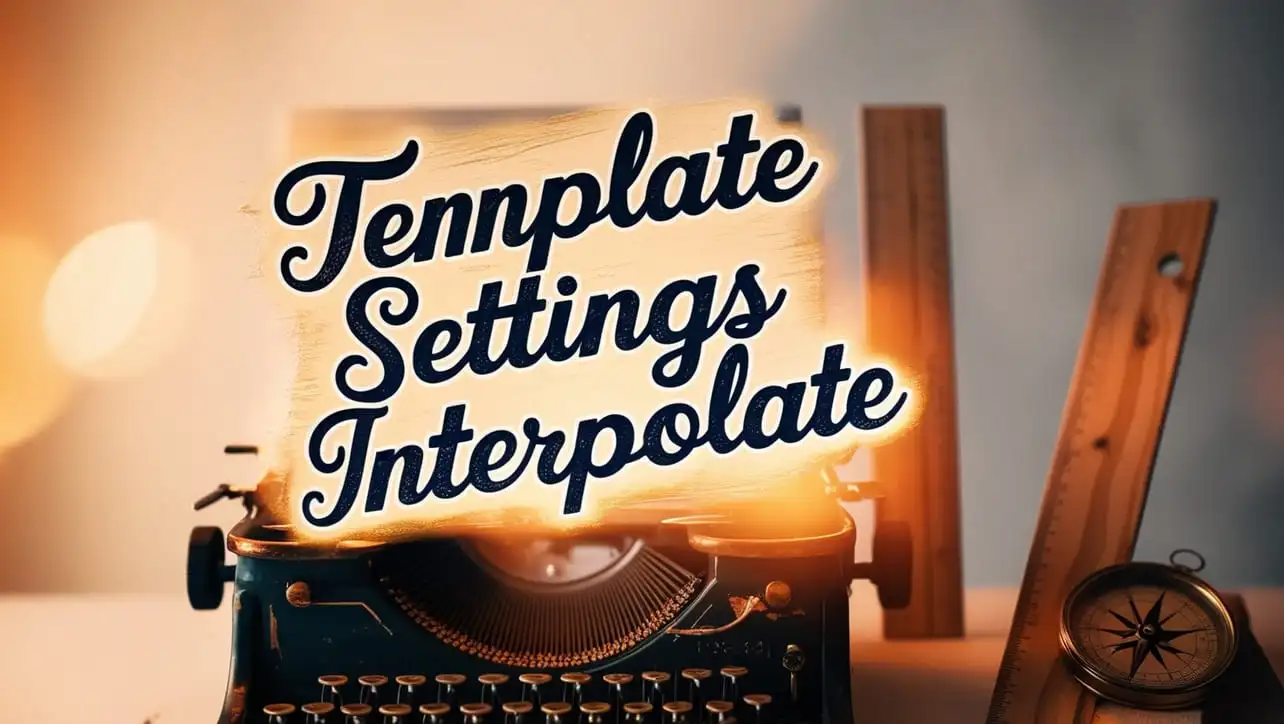
Lodash _.templateSettings.interpolate Property
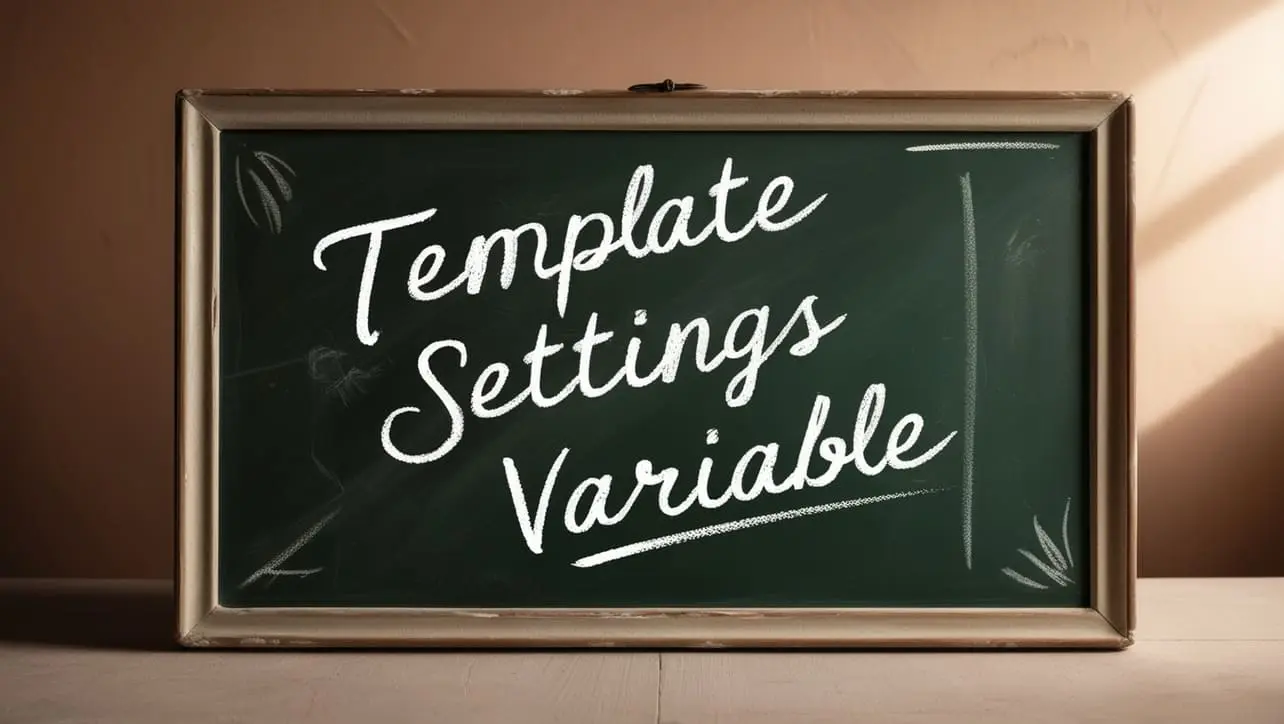
If you have any doubts regarding this article (Lodash _.isBuffer() Lang Method), please comment here. I will help you immediately.