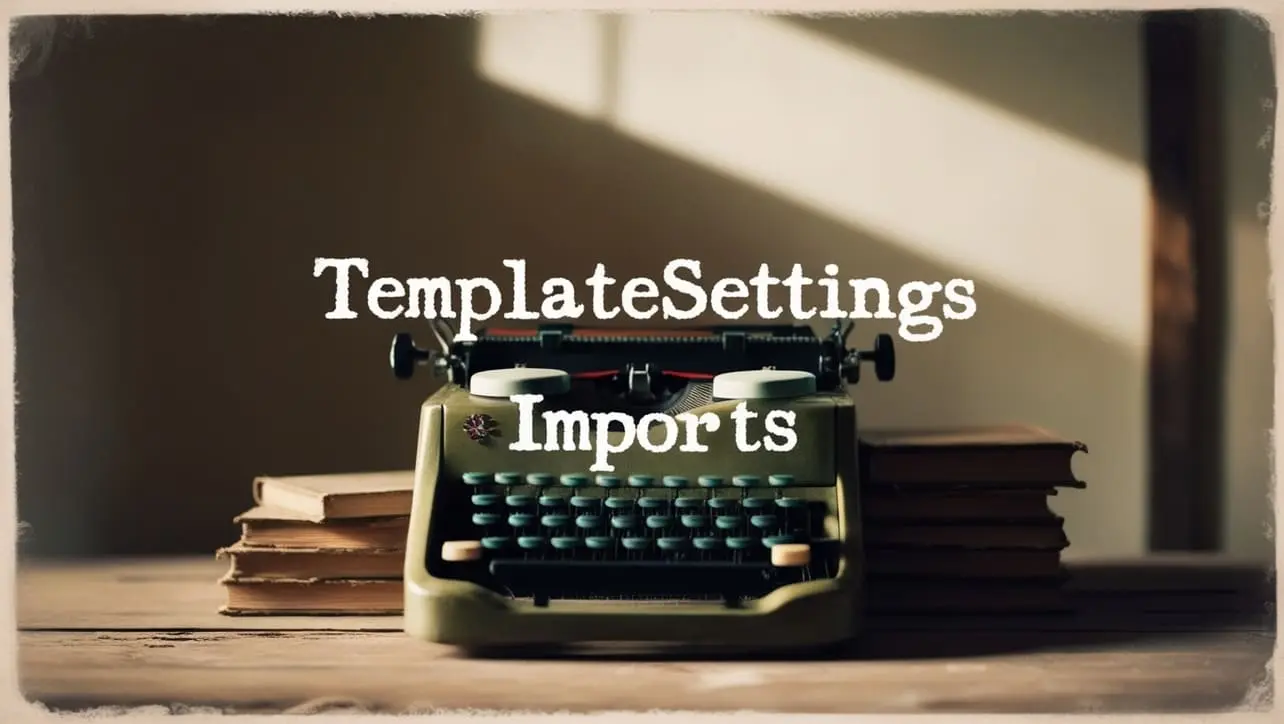
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.isArguments() Lang Method
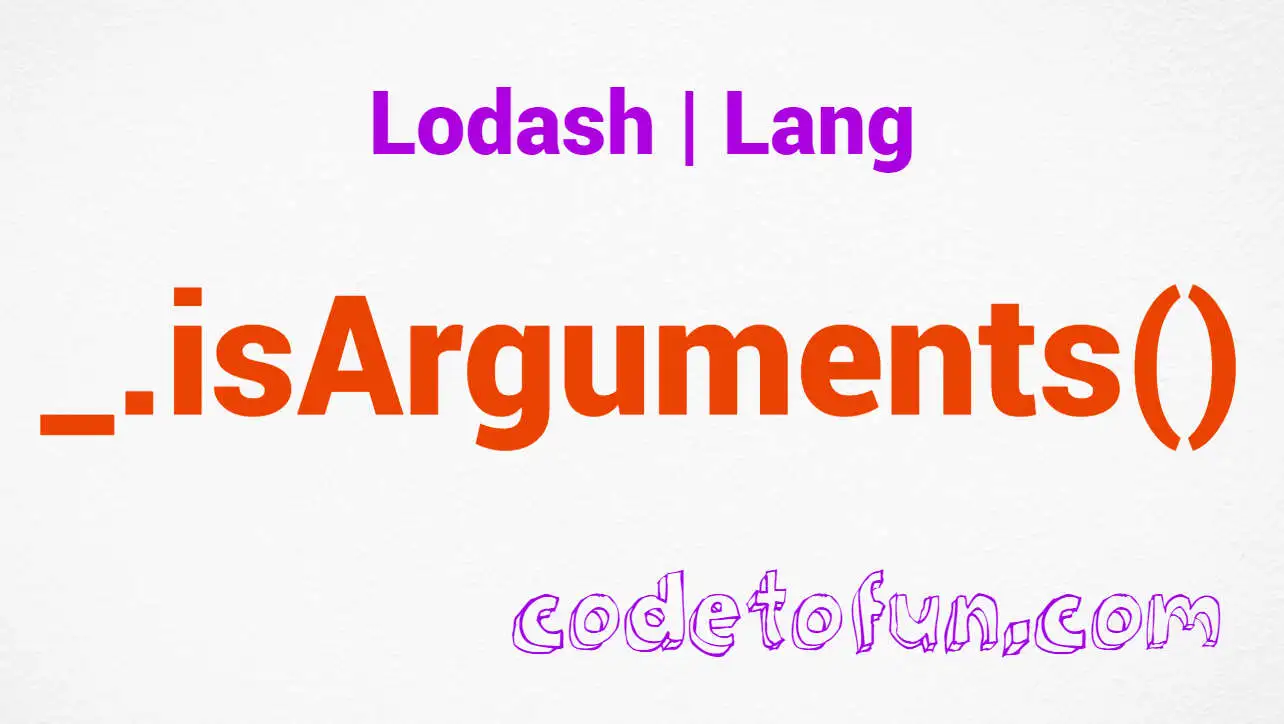
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript programming, understanding the nature of data is crucial for building robust applications. Lodash, a feature-rich utility library, provides the _.isArguments()
method as a means to identify if an object is an "arguments" object.
This method proves valuable in scenarios where you need to distinguish function arguments from other types of objects, contributing to more effective type checking and overall code quality.
🧠 Understanding _.isArguments() Method
The _.isArguments()
method in Lodash is designed to determine whether a given object is an "arguments" object, which is a special object available within functions that holds the function's arguments. By utilizing this method, developers can perform accurate type checking and handle function-specific scenarios with confidence.
💡 Syntax
The syntax for the _.isArguments()
method is straightforward:
_.isArguments(value)
- value: The value to check.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.isArguments()
method:
const _ = require('lodash');
function exampleFunction() {
console.log(_.isArguments(arguments));
}
exampleFunction(1, 2, 3);
// Output: true
In this example, the exampleFunction is defined with a call to _.isArguments(arguments), indicating that the provided value is indeed an "arguments" object.
🏆 Best Practices
When working with the _.isArguments()
method, consider the following best practices:
Accurate Type Checking:
Use
_.isArguments()
for accurate type checking when dealing with function parameters. This ensures that your code can differentiate between an "arguments" object and other types of objects.example.jsCopiedfunction processArguments(arg) { if (_.isArguments(arg)) { // Handle "arguments" object console.log('Processing function arguments:', arg); } else { // Handle other types of objects console.log('Not a function arguments object:', arg); } } processArguments(arguments); // Output: Processing function arguments: [Arguments] { '0': 1, '1': 2, '2': 3 }
Function Parameter Validation:
When validating function parameters, use
_.isArguments()
to specifically check if an argument is the "arguments" object. This can be particularly useful in functions with variable parameters.example.jsCopiedfunction validateParameters() { for (let i = 0; i < arguments.length; i++) { if (_.isArguments(arguments[i])) { console.error('Invalid argument:', arguments[i]); return false; } } return true; } console.log(validateParameters(1, 'hello', [2, 3])); // Output: true console.log(validateParameters(1, arguments, [2, 3])); // Output: Invalid argument: [Arguments] { '0': 1, '1': [Arguments], '2': [ 2, 3 ] } // false
Compatibility with Other Libraries:
When working with other libraries or frameworks that may use or return "arguments" objects, leverage
_.isArguments()
to handle these cases gracefully.example.jsCopiedconst externalLibraryResult = /* ...result from an external library... */ ; if (_.isArguments(externalLibraryResult)) { console.log('Handling external library result as "arguments" object:', externalLibraryResult); } else { console.log('Processing external library result:', externalLibraryResult); }
📚 Use Cases
Function Overloading:
In scenarios where function overloading or variable parameters are employed,
_.isArguments()
can help identify when the "arguments" object is being used.example.jsCopiedfunction overloadedFunction() { if (_.isArguments(arguments)) { console.log('Function overloaded with variable parameters:', arguments); // Handle variable parameters } else { console.log('Function with specific parameters:', arguments); // Handle specific parameters } } overloadedFunction(1, 2, 3); // Output: Function overloaded with variable parameters: [Arguments] { '0': 1, '1': 2, '2': 3 }
Custom Type Checking:
When building custom type-checking functions, incorporate
_.isArguments()
to enhance the accuracy of your type-checking mechanisms.example.jsCopiedfunction isCustomType(value) { return _.isArguments(value) ? 'ArgumentsObject' : typeof value; } console.log(isCustomType(arguments)); // Output: ArgumentsObject
Conditional Logic in Functions:
Within functions, use
_.isArguments()
to conditionally execute logic based on whether the function is invoked with the "arguments" object.example.jsCopiedfunction processInput(input) { if (_.isArguments(input)) { console.log('Processing function arguments:', input); // Handle "arguments" object } else { console.log('Processing regular input:', input); // Handle regular input } } processInput(1, 2, 3); // Output: Processing function arguments: [Arguments] { '0': 1, '1': 2, '2': 3 }
🎉 Conclusion
The _.isArguments()
method in Lodash provides a reliable means to identify "arguments" objects within JavaScript functions. By incorporating this method into your code, you can improve type checking, handle function-specific scenarios, and enhance the overall robustness of your applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.isArguments()
method in your Lodash projects.
👨💻 Join our Community:
Author
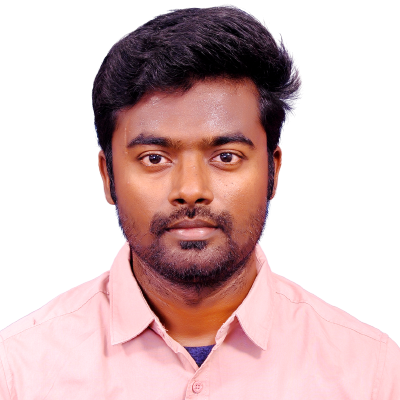
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
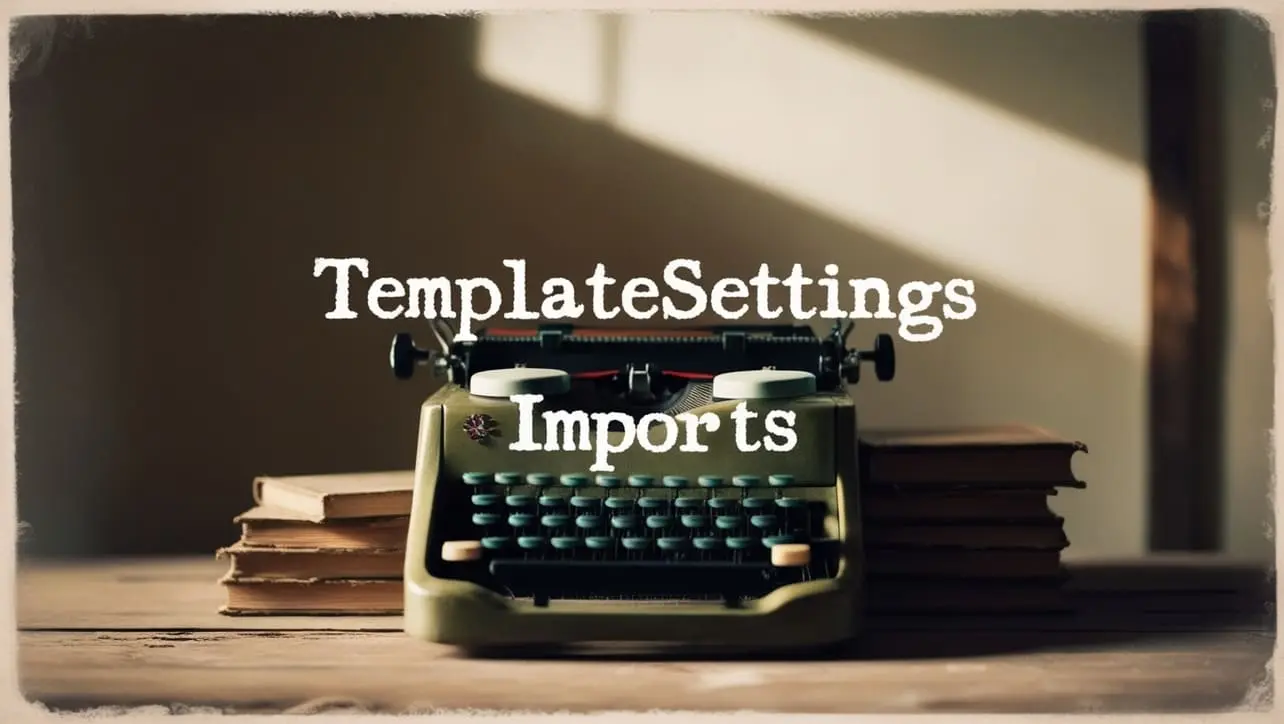
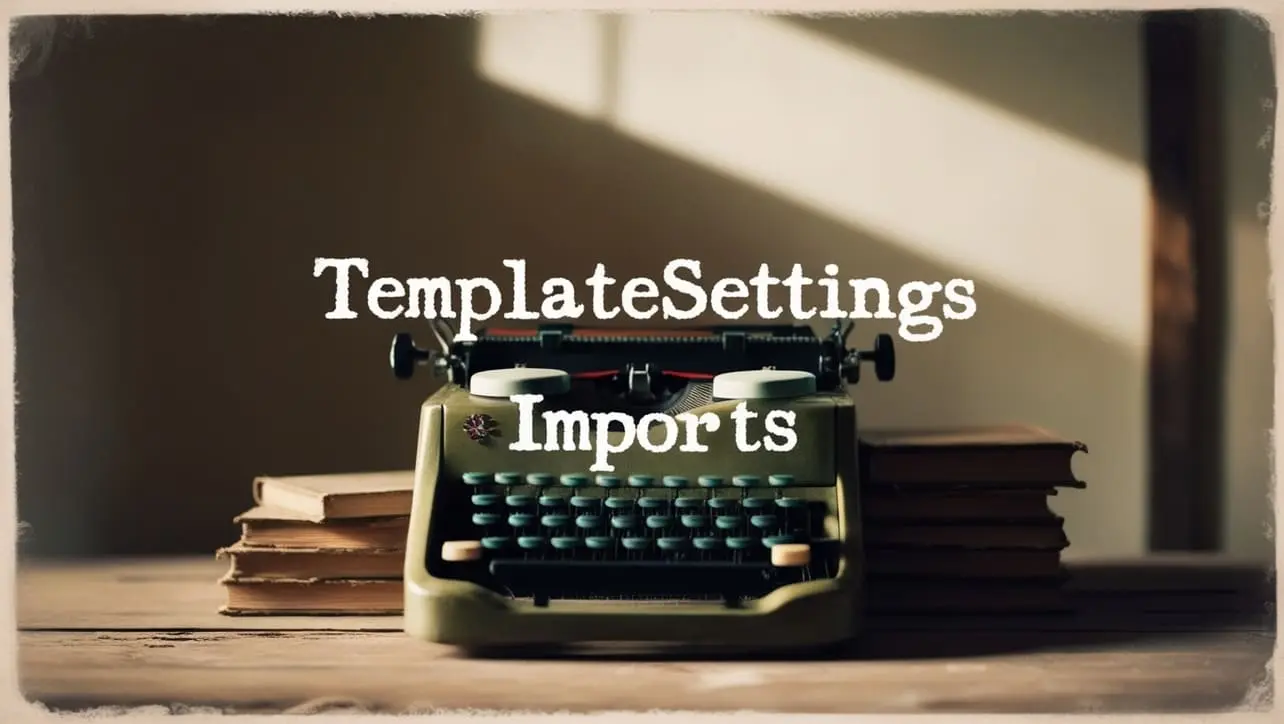
Lodash _.templateSettings.imports Property
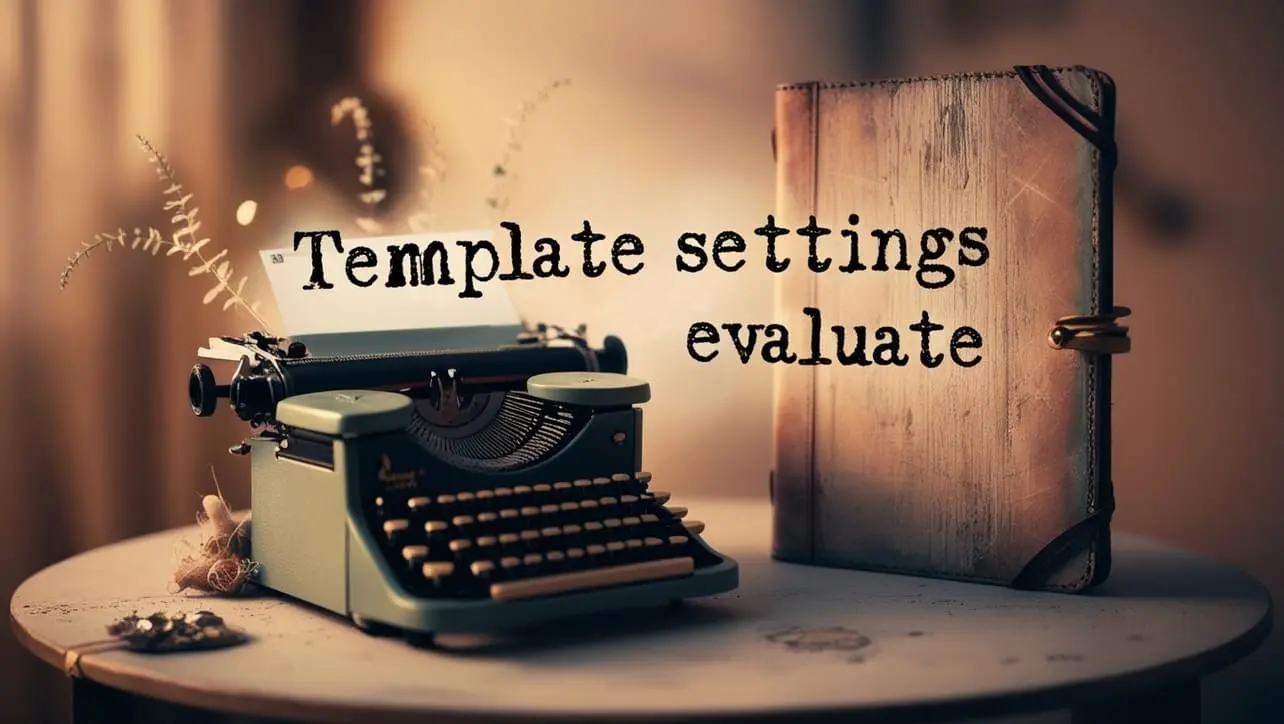
Lodash _.templateSettings.evaluate Property
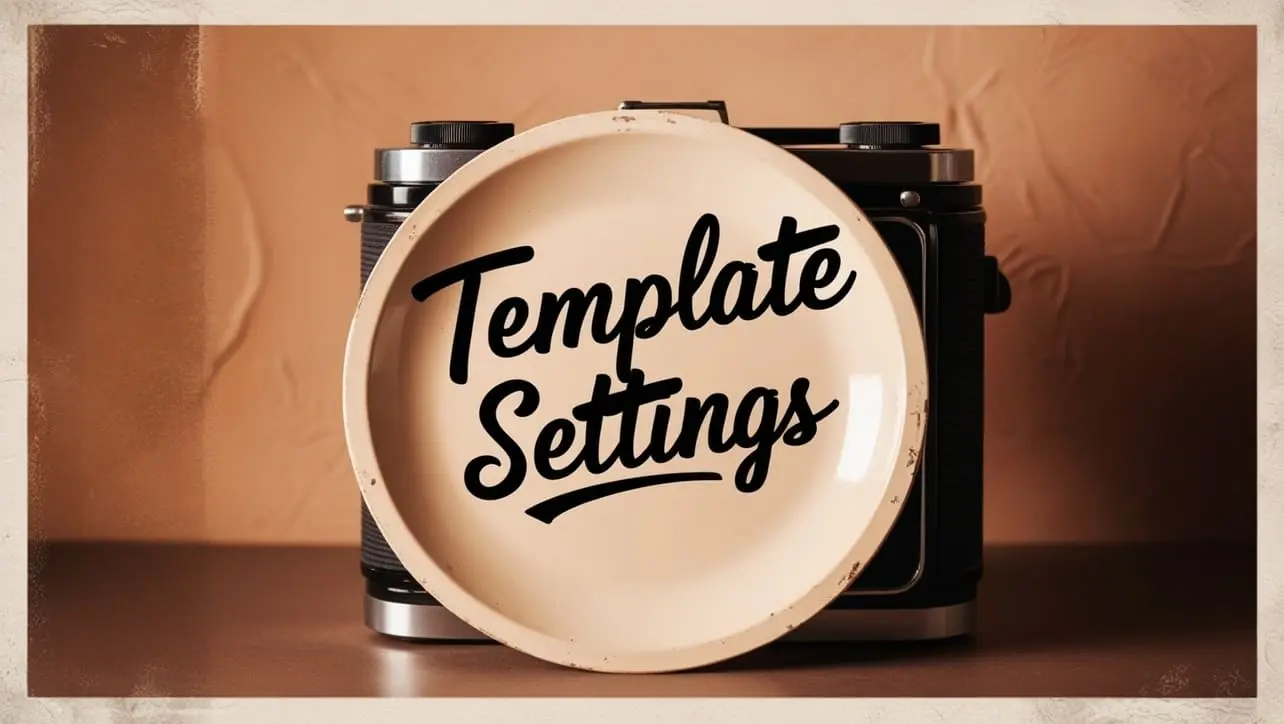
Lodash _.templateSettings Property
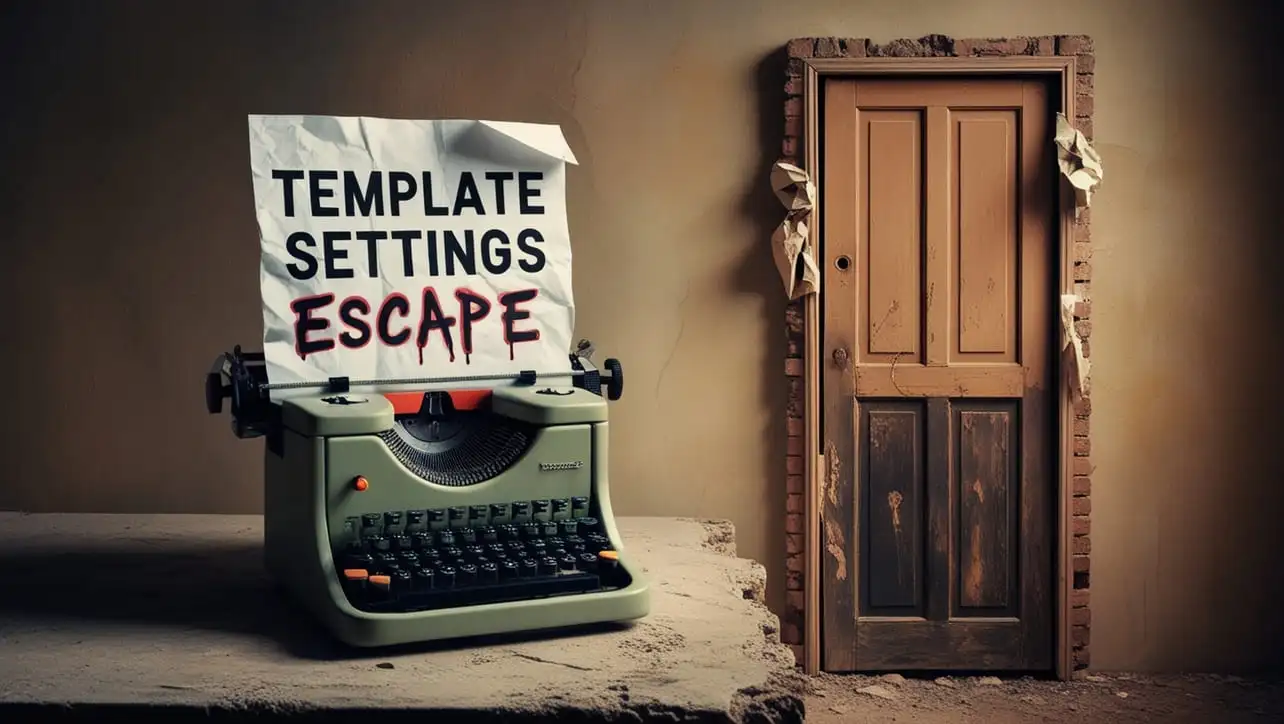
Lodash _.templateSettings.escape Property
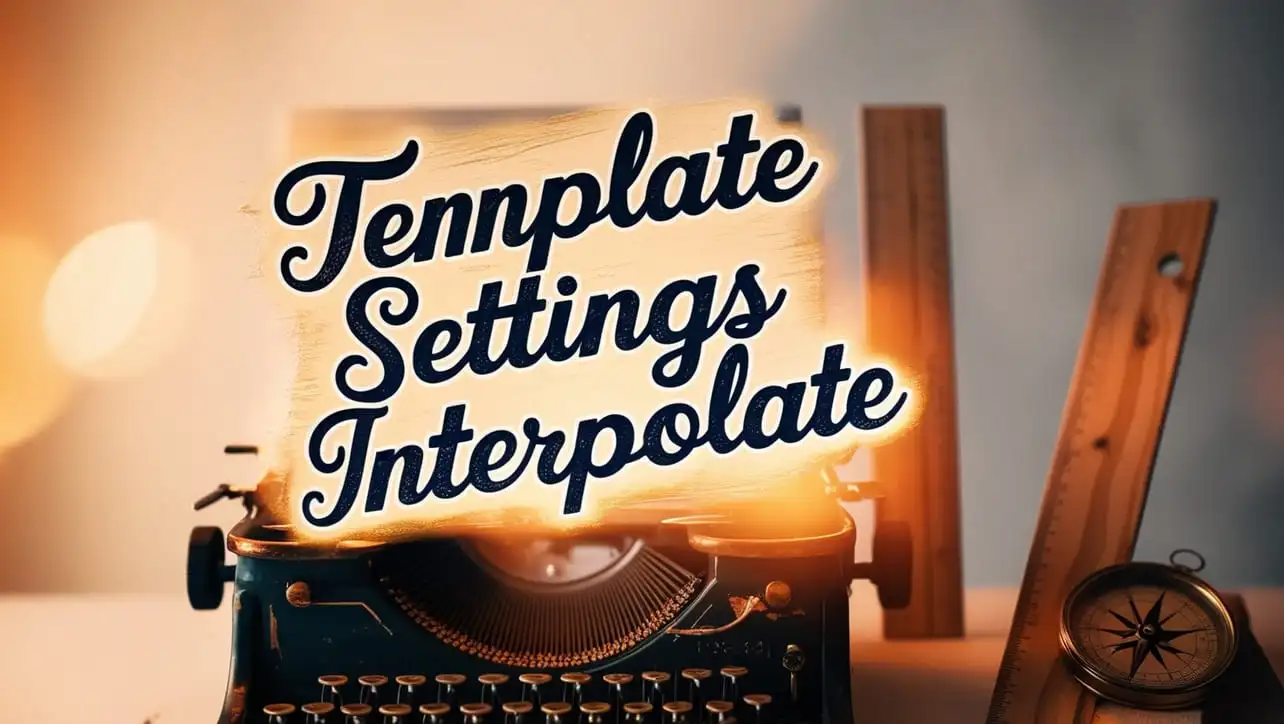
Lodash _.templateSettings.interpolate Property
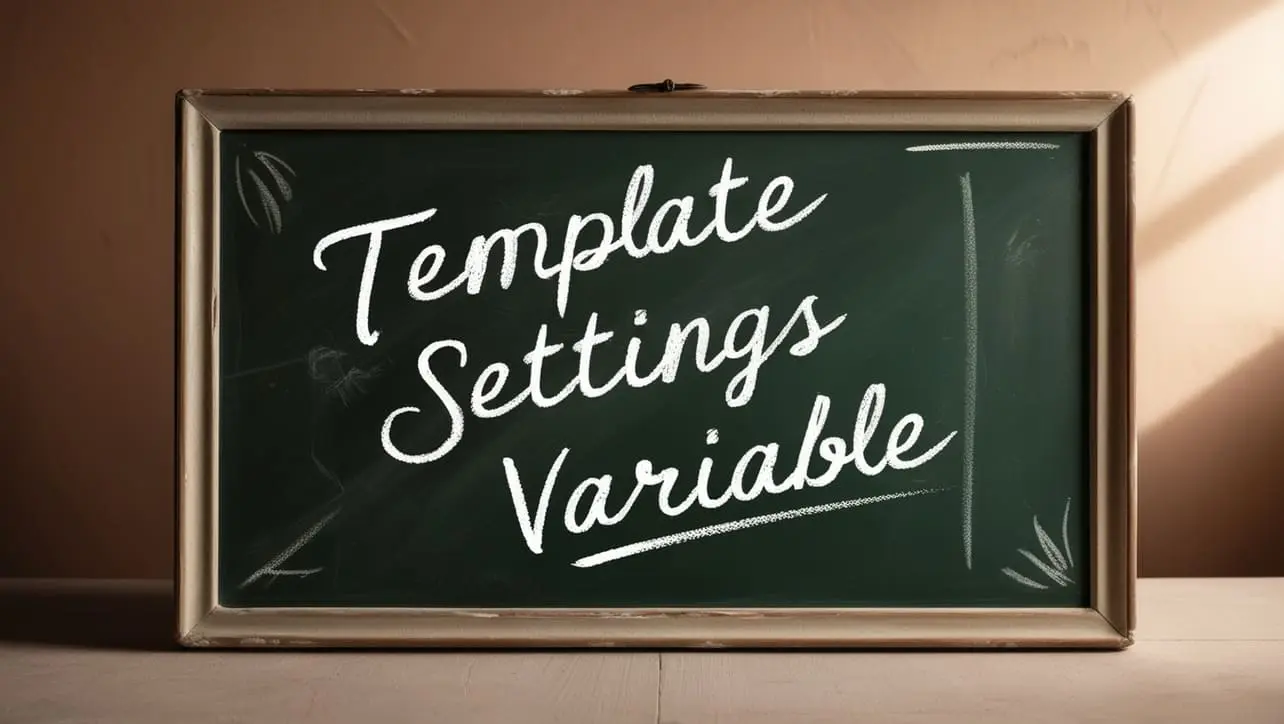
If you have any doubts regarding this article (Lodash _.isArguments() Lang Method), please comment here. I will help you immediately.