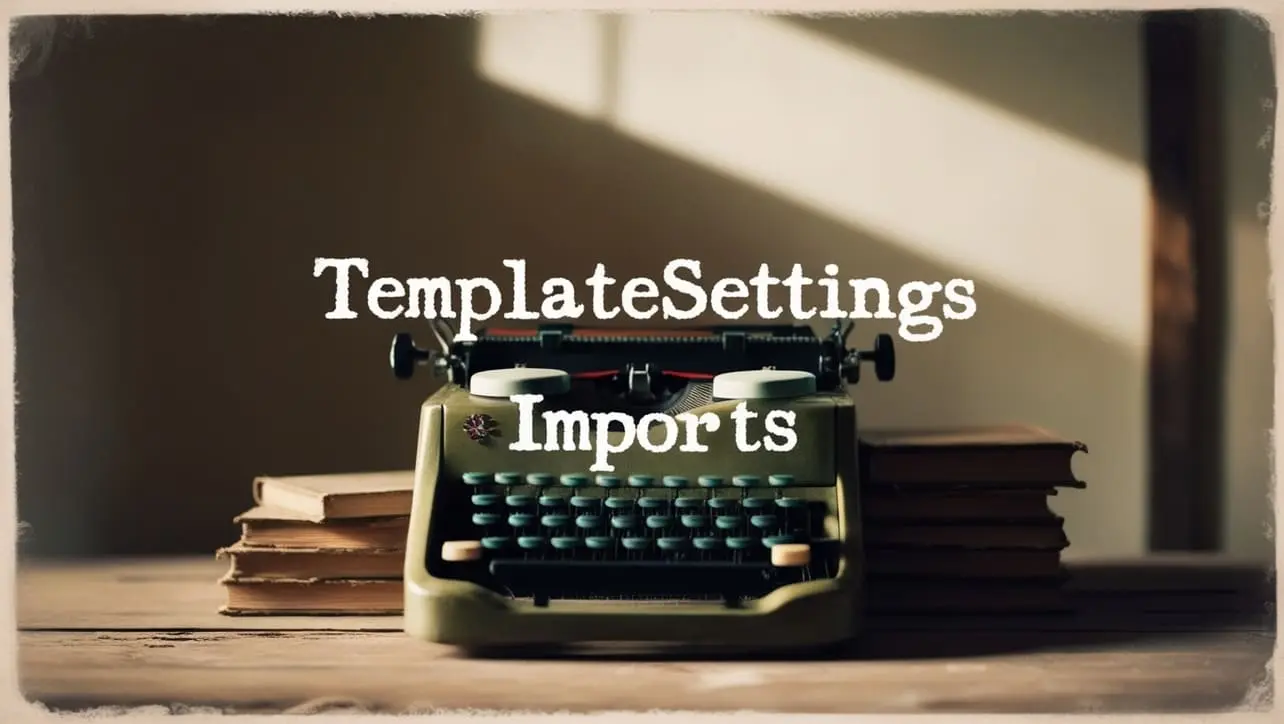
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.cloneDeepWith() Lang Method
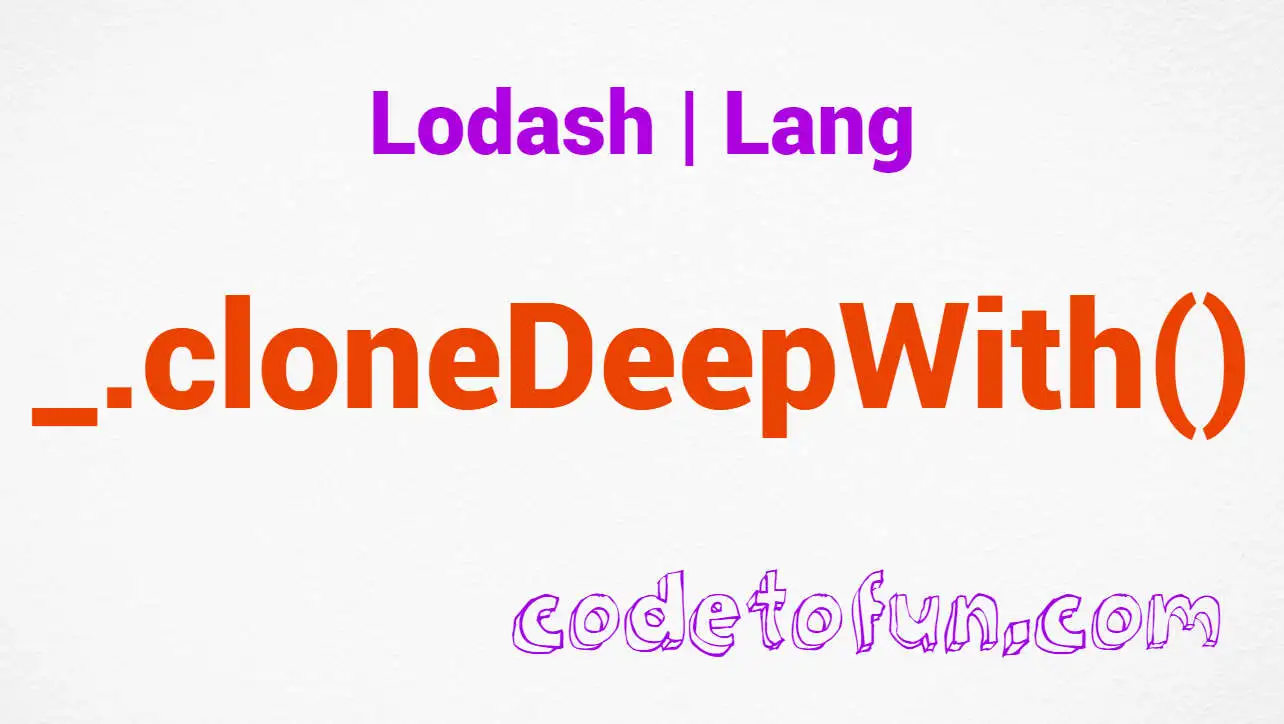
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, managing complex objects and data structures often requires careful handling of references. Lodash, a comprehensive utility library, provides a solution with the _.cloneDeepWith()
method. This method goes beyond a shallow copy, allowing developers to create deep clones of objects with customizable cloning behavior.
Understanding and harnessing the power of _.cloneDeepWith()
can significantly enhance your ability to work with intricate data structures in a flexible and tailored manner.
🧠 Understanding _.cloneDeepWith() Method
The _.cloneDeepWith()
method in Lodash enables the creation of deep clones with a customizable cloning function. Unlike a shallow copy, which only creates a new reference to the top-level object, _.cloneDeepWith()
recursively clones nested objects, providing a complete duplicate with the option to define specific cloning logic.
💡 Syntax
The syntax for the _.cloneDeepWith()
method is straightforward:
_.cloneDeepWith(value, [customizer])
- value: The value to recursively clone.
- customizer (Optional): The function invoked to customize cloning.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.cloneDeepWith()
method:
const _ = require('lodash');
const originalObject = {
id: 1,
name: 'John',
address: {
city: 'New York',
country: 'USA',
},
};
const customCloner = (value) => {
if (_.isPlainObject(value)) {
// Clone only if the object has a 'country' property
return value.hasOwnProperty('country') ? _.cloneDeep(value) : undefined;
}
};
const clonedObject = _.cloneDeepWith(originalObject, customCloner);
console.log(clonedObject);
In this example, the customCloner function is used to specify that only objects with a 'country' property should be deeply cloned. The result is a new object with the same structure, excluding properties that don't meet the custom cloning criteria.
🏆 Best Practices
When working with the _.cloneDeepWith()
method, consider the following best practices:
Custom Cloning Logic:
Take advantage of the customizer function to define specific cloning logic for different types of objects. This allows you to tailor the cloning process according to your application's requirements.
example.jsCopiedconst customCloner = (value) => { if (_.isArray(value)) { // Clone arrays, but limit the length to 5 elements return value.slice(0, 5); } else if (_.isPlainObject(value)) { // Clone objects, excluding properties with keys starting with '_' return _.omitBy(_.cloneDeep(value), (_, key) => key.startsWith('_')); } }; const originalData = /* ...complex data structure... */ ; const clonedData = _.cloneDeepWith(originalData, customCloner); console.log(clonedData);
Handling Circular References:
Be cautious when dealing with circular references in objects. The default behavior of
_.cloneDeepWith()
is to handle circular references, but if you have specific requirements, you can customize the cloning process accordingly.example.jsCopiedconst circularObject = { prop: 'value' }; circularObject.circularRef = circularObject; const customCloner = (value) => { if (_.isObject(value) && value.circularRef === value) { // Custom handling for circular reference return { circularRef: 'handled' }; } }; const clonedCircularObject = _.cloneDeepWith(circularObject, customCloner); console.log(clonedCircularObject);
Preserve Prototype Chains:
Consider whether to preserve prototype chains during cloning. By default, Lodash's
_.cloneDeepWith()
maintains prototype chains, but you can customize this behavior if needed.example.jsCopiedconst originalWithPrototype = Object.create({ prototypeProp: 'value' }); originalWithPrototype.customProp = 'customValue'; const customCloner = (value) => { if (Object.getPrototypeOf(value)) { // Exclude properties from the prototype chain return _.omit(value, Object.getOwnPropertyNames(Object.getPrototypeOf(value))); } }; const clonedWithoutPrototype = _.cloneDeepWith(originalWithPrototype, customCloner); console.log(clonedWithoutPrototype);
📚 Use Cases
Complex Data Structures:
_.cloneDeepWith()
is particularly useful when working with complex data structures containing nested objects, arrays, or custom types. Customizing the cloning process ensures a tailored and accurate duplication of intricate data.example.jsCopiedclass CustomType { constructor(value) { this.value = value; } } const originalData = { array: [1, 2, 3], customObject: new CustomType('original'), }; const customCloner = (value) => { if (value instanceof CustomType) { // Customize cloning for custom types return new CustomType(`cloned-${value.value}`); } }; const clonedData = _.cloneDeepWith(originalData, customCloner); console.log(clonedData);
Data Sanitization:
When dealing with data from external sources, such as APIs or user inputs,
_.cloneDeepWith()
can be employed to create sanitized copies of the data by selectively cloning and modifying certain properties.example.jsCopiedconst userData = /* ...fetch user data from an API... */ ; const sanitizeUserData = (value) => { if (_.isString(value)) { // Truncate strings to a maximum length return value.slice(0, 50); } else if (_.isPlainObject(value)) { // Exclude sensitive information from the cloned object return _.omit(value, ['password', 'creditCardNumber']); } }; const sanitizedUserData = _.cloneDeepWith(userData, sanitizeUserData); console.log(sanitizedUserData);
Immutable State in Redux:
In a Redux or state management context,
_.cloneDeepWith()
can aid in creating immutable copies of state objects. Customizing the cloning process allows for controlled modifications or exclusions, maintaining the integrity of the application state.example.jsCopiedconst initialState = /* ...define initial state for Redux... */ ; const customCloner = (value) => { if (_.isArray(value)) { // Freeze arrays to ensure immutability return Object.freeze(_.cloneDeep(value)); } else if (_.isPlainObject(value)) { // Freeze objects, excluding certain properties return Object.freeze(_.omit(_.cloneDeep(value), ['volatileProperty'])); } }; const nextState = _.cloneDeepWith(initialState, customCloner); console.log(nextState);
🎉 Conclusion
The _.cloneDeepWith()
method in Lodash empowers developers to create deep clones of objects with customizable cloning logic. Whether dealing with intricate data structures, circular references, or the need for immutable state, this method provides a versatile solution for managing complex objects in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.cloneDeepWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
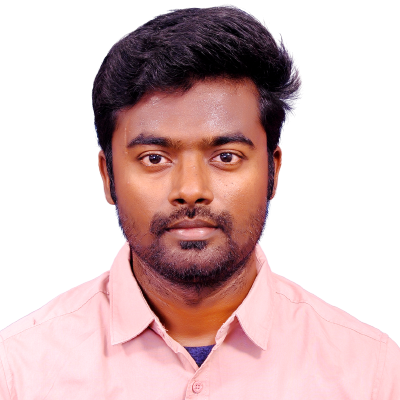
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
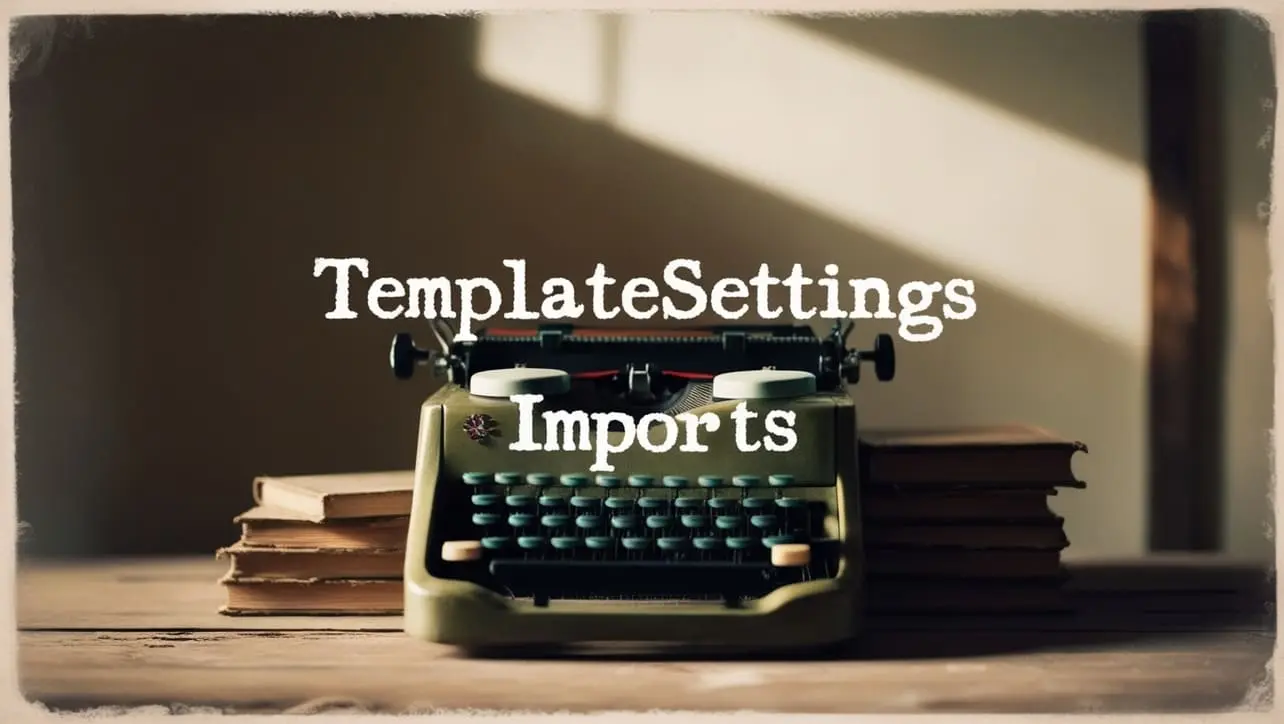
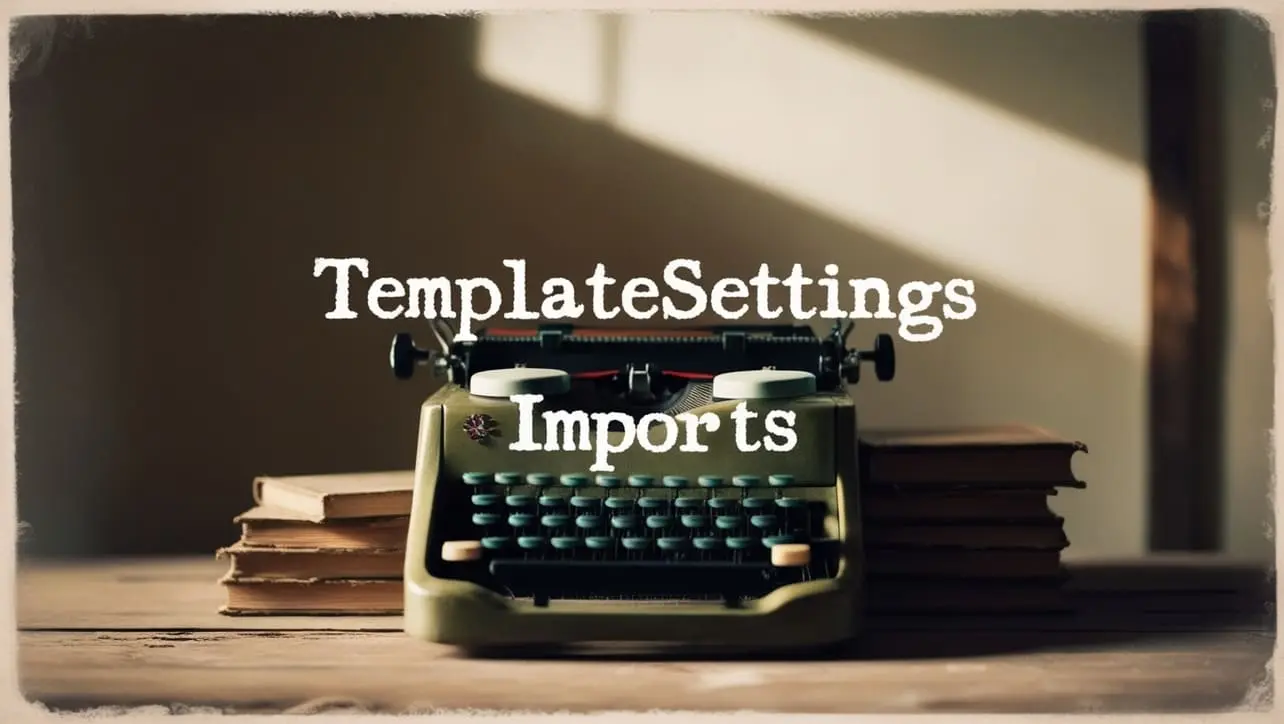
Lodash _.templateSettings.imports Property
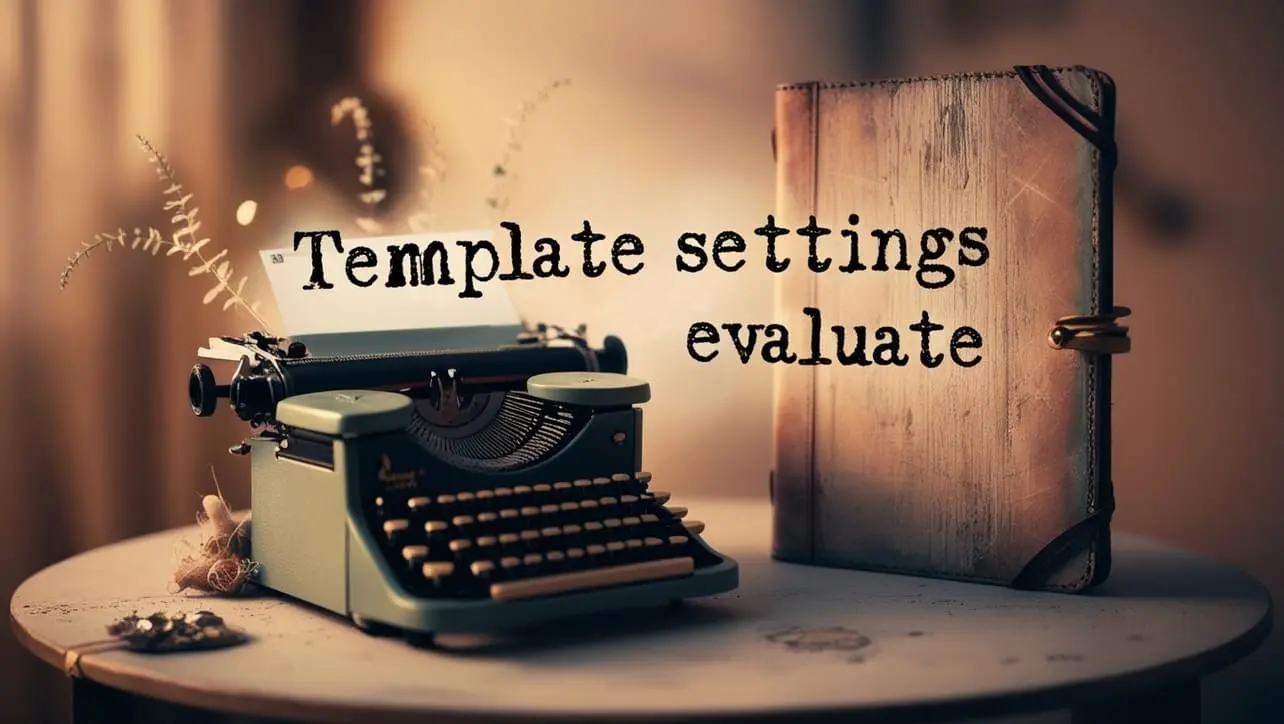
Lodash _.templateSettings.evaluate Property
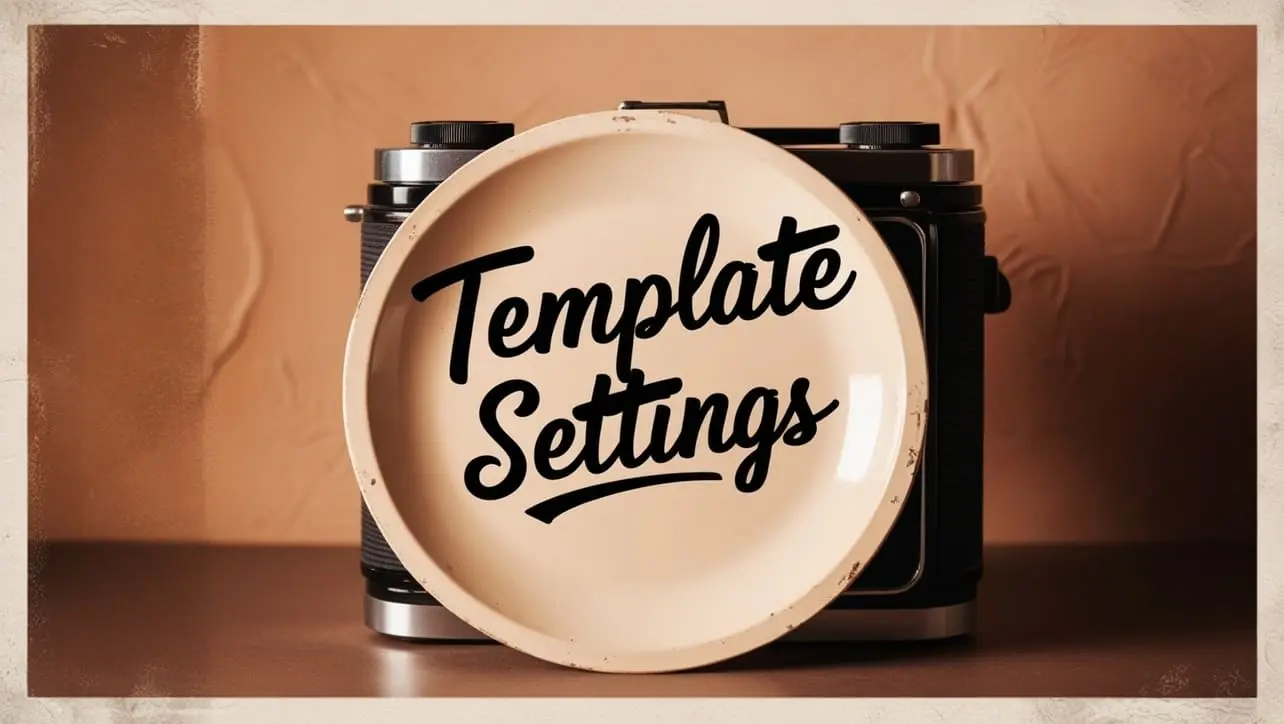
Lodash _.templateSettings Property
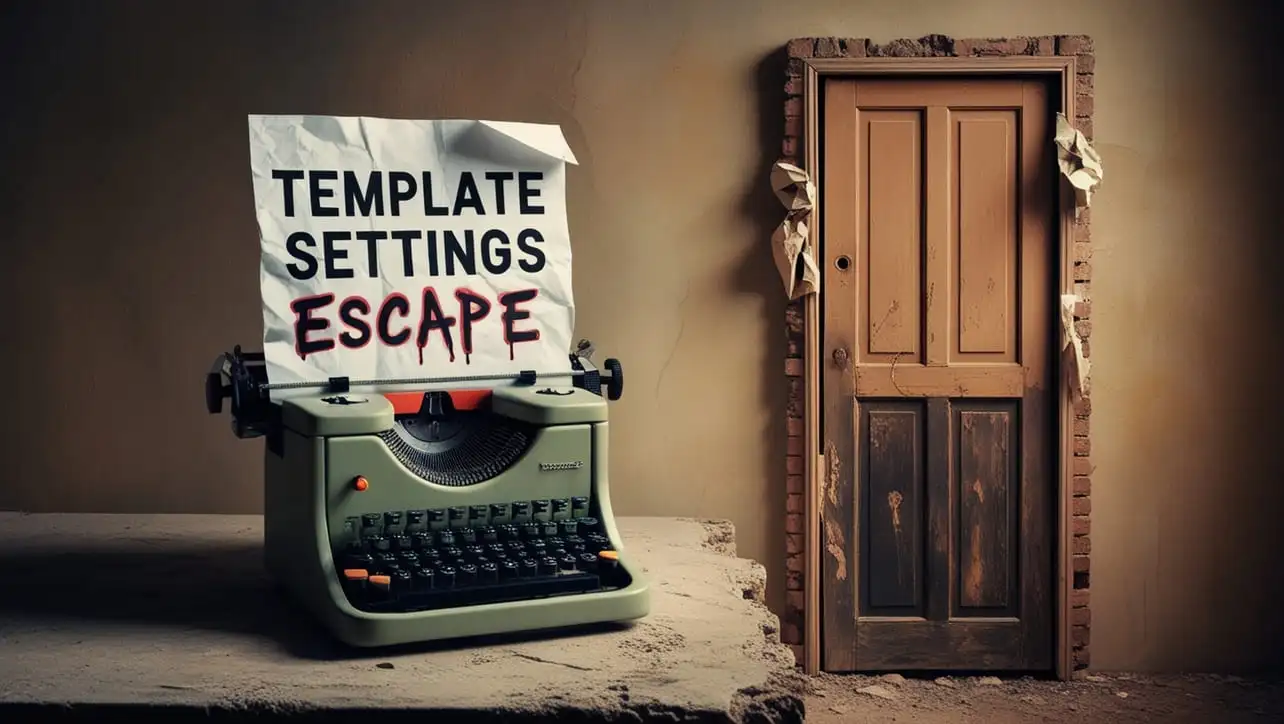
Lodash _.templateSettings.escape Property
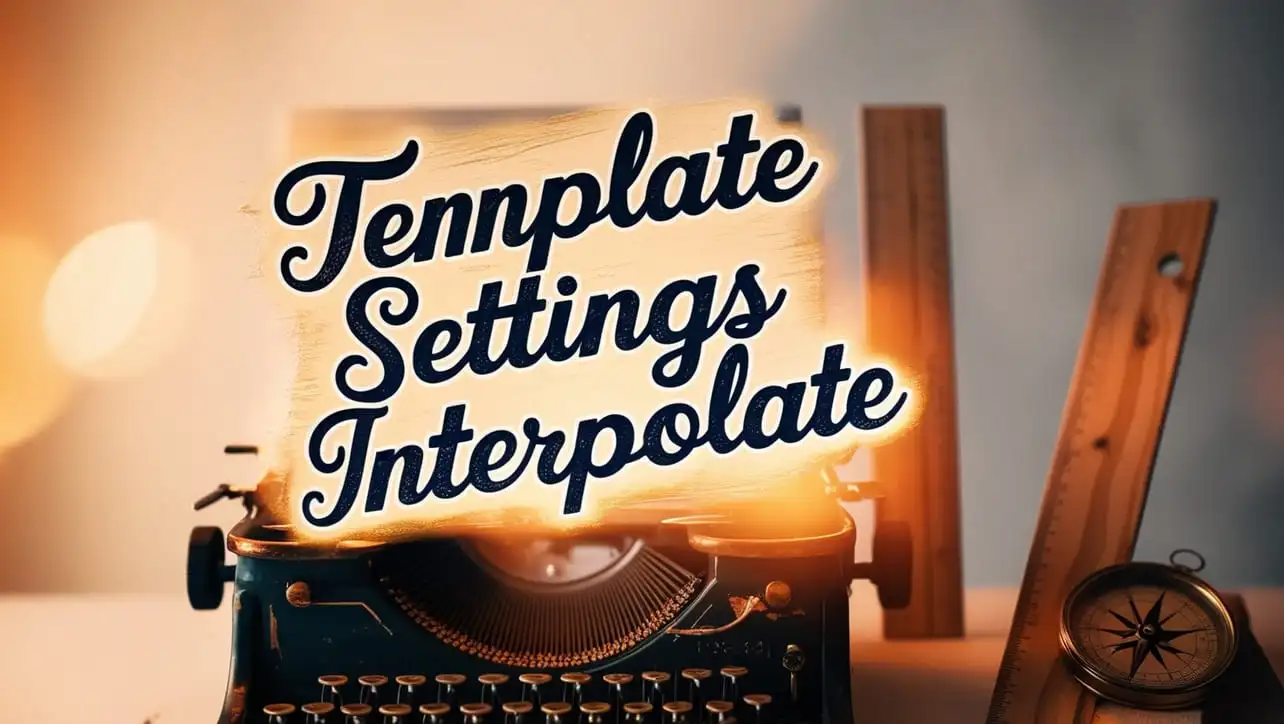
Lodash _.templateSettings.interpolate Property
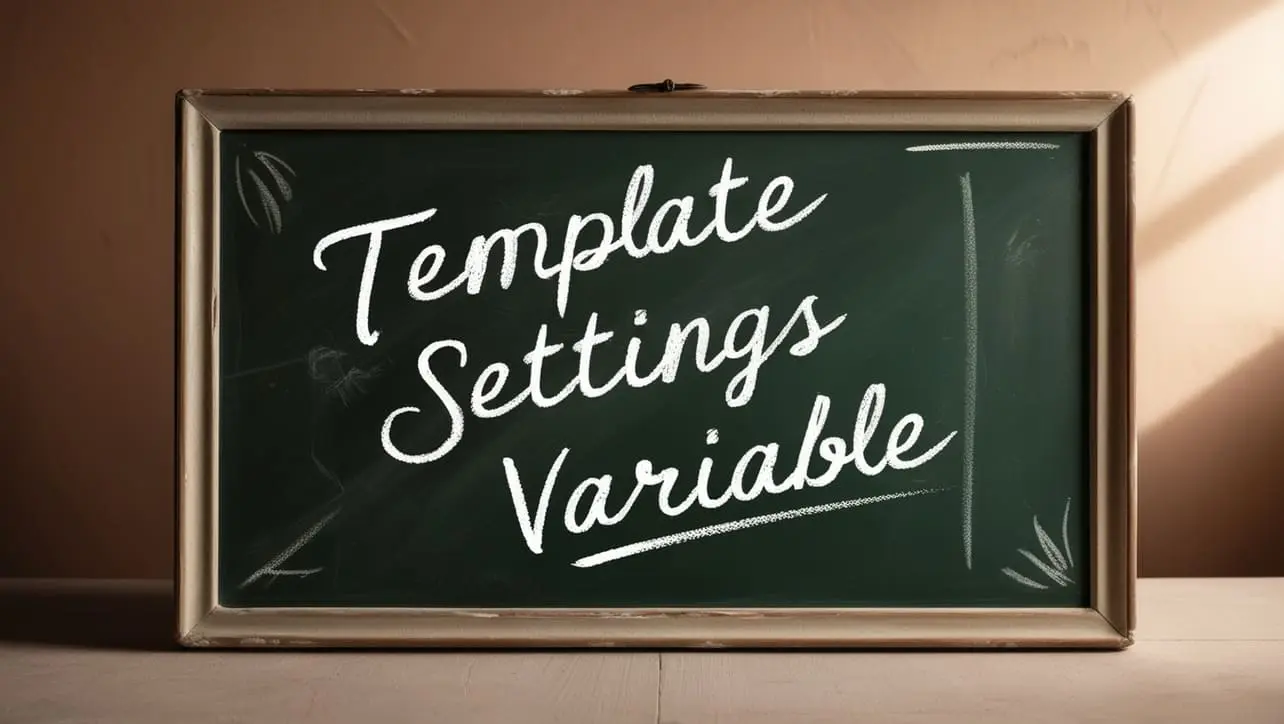
If you have any doubts regarding this article (Lodash _.cloneDeepWith() Lang Method), please comment here. I will help you immediately.