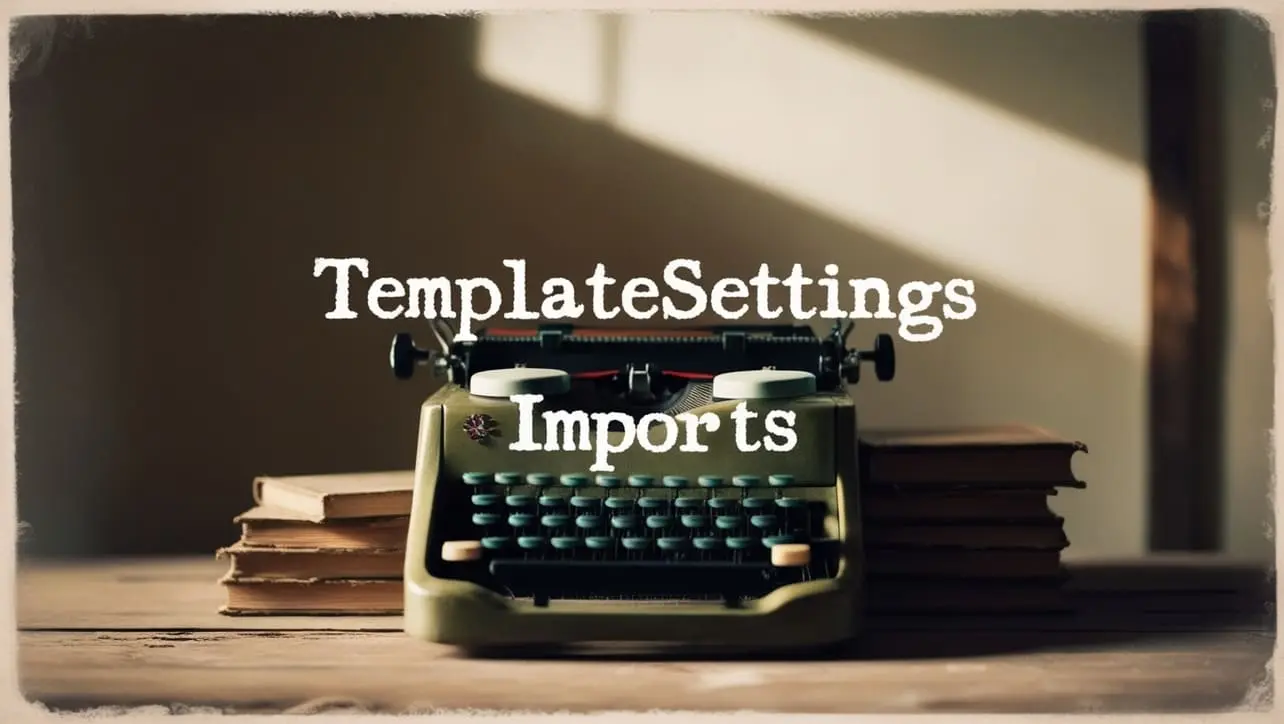
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.clone() Lang Method
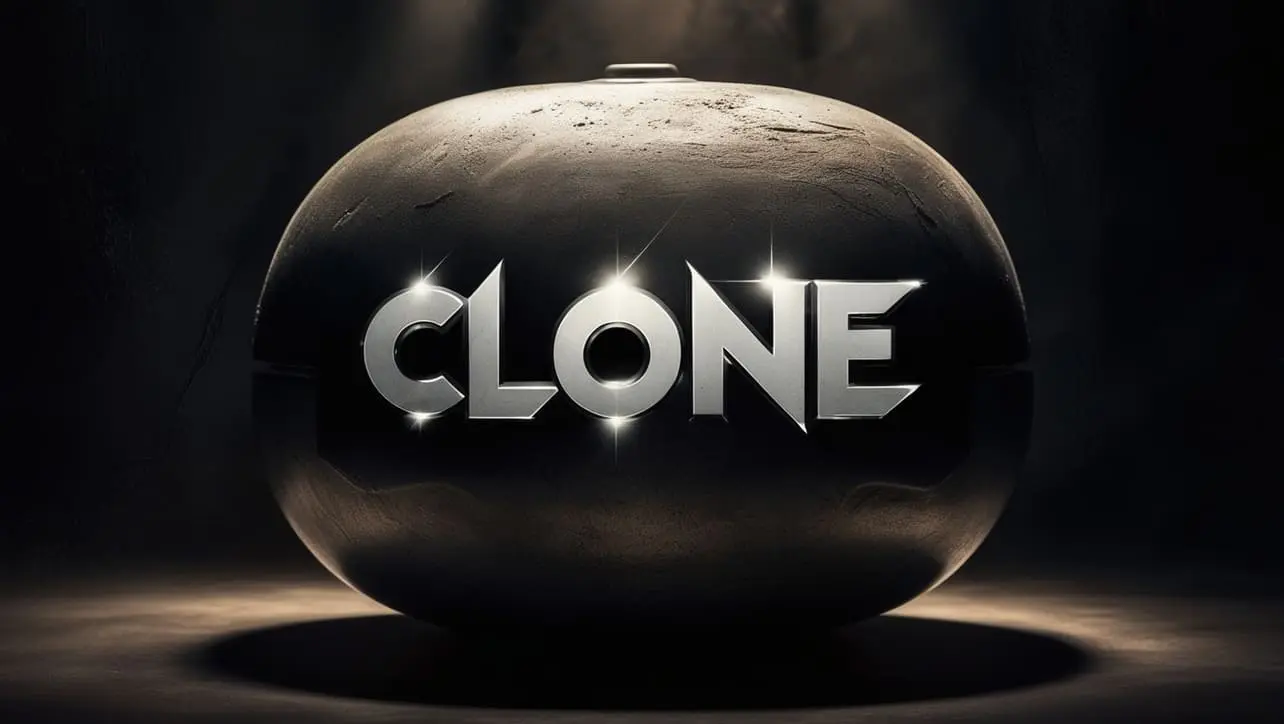
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript, effective handling of objects and arrays is crucial. Lodash, a powerful utility library, provides a multitude of functions to simplify these tasks. One such fundamental function is _.clone()
, part of the Lang category in Lodash.
This method is designed to create a shallow copy of an object or array, facilitating efficient data manipulation and reducing the risk of unintended side effects in your code.
🧠 Understanding _.clone() Method
The _.clone()
method in Lodash is a straightforward yet indispensable tool for duplicating objects and arrays without altering the original data. By creating a shallow copy, developers can work with the cloned data independently, making modifications without impacting the source.
💡 Syntax
The syntax for the _.clone()
method is straightforward:
_.clone(value)
- value: The value to clone.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.clone()
method:
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5];
const clonedArray = _.clone(originalArray);
console.log(clonedArray);
// Output: [1, 2, 3, 4, 5]
In this example, originalArray is cloned using _.clone()
, resulting in a new array, clonedArray, with identical elements.
🏆 Best Practices
When working with the _.clone()
method, consider the following best practices:
Understand Shallow Copy:
Be aware that
_.clone()
creates a shallow copy. While it duplicates the top-level object or array, nested objects or arrays within the original data are still references. This means modifications to nested structures will affect both the original and cloned data.example.jsCopiedconst originalObject = { key: { nestedKey: 'value' } }; const clonedObject = _.clone(originalObject); clonedObject.key.nestedKey = 'modifiedValue'; console.log(originalObject.key.nestedKey); // Output: 'modifiedValue'
Handle Circular References:
_.clone()
can handle circular references in objects, preventing infinite recursion. However, be cautious when working with objects containing circular structures, as it may impact performance.example.jsCopiedconst circularObject = { key: 'value' }; circularObject.circularReference = circularObject; const clonedCircularObject = _.clone(circularObject); console.log(clonedCircularObject.circularReference === clonedCircularObject); // Output: true
Consider Deep Clone for Nested Structures:
If your data involves complex nested structures, consider using _.cloneDeep() instead of
_.clone()
to create a deep copy. This ensures that all nested structures are duplicated, avoiding shared references.example.jsCopiedconst originalNestedObject = { key: { nestedKey: 'value' } }; const deepClonedObject = _.cloneDeep(originalNestedObject); deepClonedObject.key.nestedKey = 'modifiedValue'; console.log(originalNestedObject.key.nestedKey); // Output: 'value'
📚 Use Cases
Immutability in State Management:
_.clone()
is valuable in state management scenarios, especially in frameworks like React or Vue.js. When working with application state, creating a cloned copy ensures immutability, helping prevent unintentional state mutations.example.jsCopiedconst currentState = /* ...current state object... */; const newState = _.clone(currentState); // Modify newState without affecting currentState
Configuration Objects:
When dealing with configuration objects,
_.clone()
allows you to create copies for different instances or variations without altering the original configuration.example.jsCopiedconst baseConfig = /* ...base configuration object... */; const customConfig = _.clone(baseConfig); // Customize customConfig for a specific scenario
Undo Functionality in Applications:
In applications requiring undo functionality,
_.clone()
can be used to create snapshots of the state. These snapshots serve as points to revert to, providing a simple undo mechanism.example.jsCopiedconst stateHistory = []; function saveSnapshot() { const snapshot = _.clone( /* ...current application state... */ ); stateHistory.push(snapshot); } function undo() { if (stateHistory.length > 0) { const previousState = stateHistory.pop(); // Apply previousState to revert the application state } }
🎉 Conclusion
The _.clone()
method in Lodash, nestled within the Lang category, is a versatile and essential tool for JavaScript developers. By providing a straightforward means of creating shallow copies of objects and arrays, it contributes to code maintainability and reduces the likelihood of unintended side effects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.clone()
method in your Lodash projects.
👨💻 Join our Community:
Author
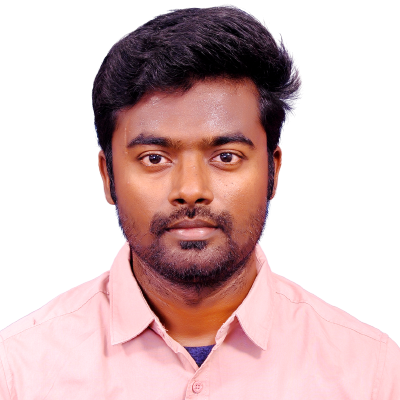
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
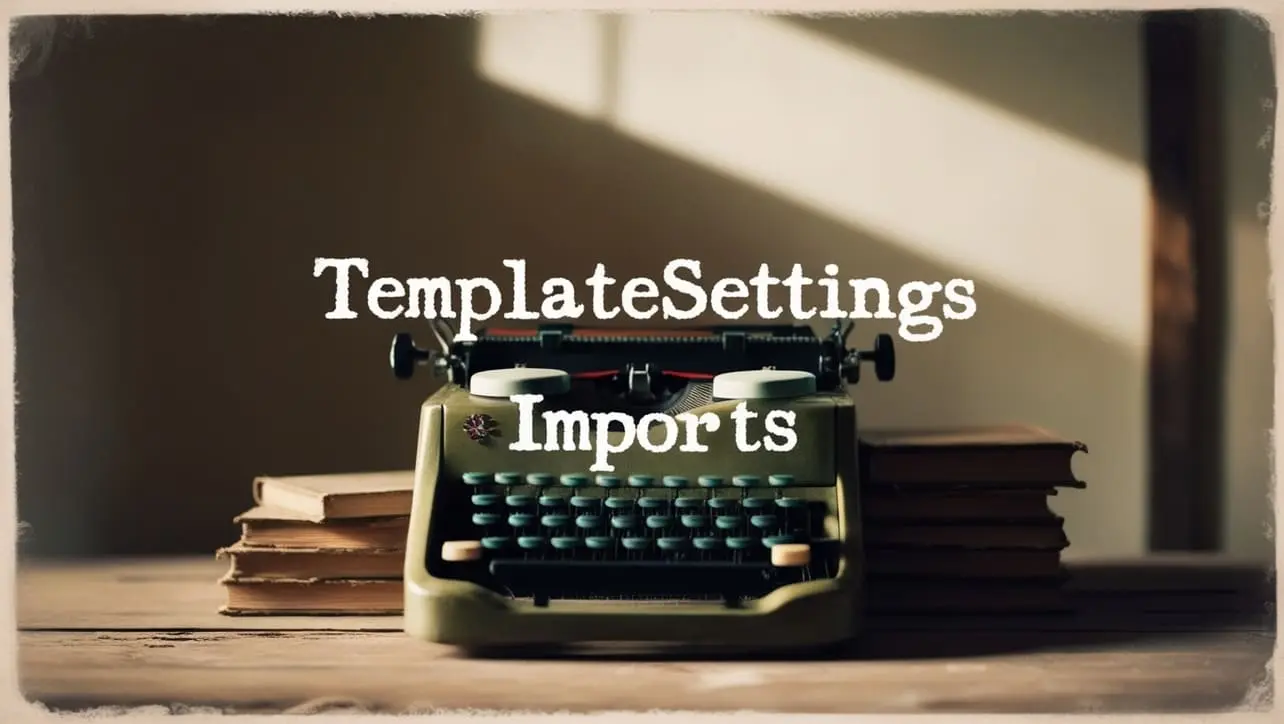
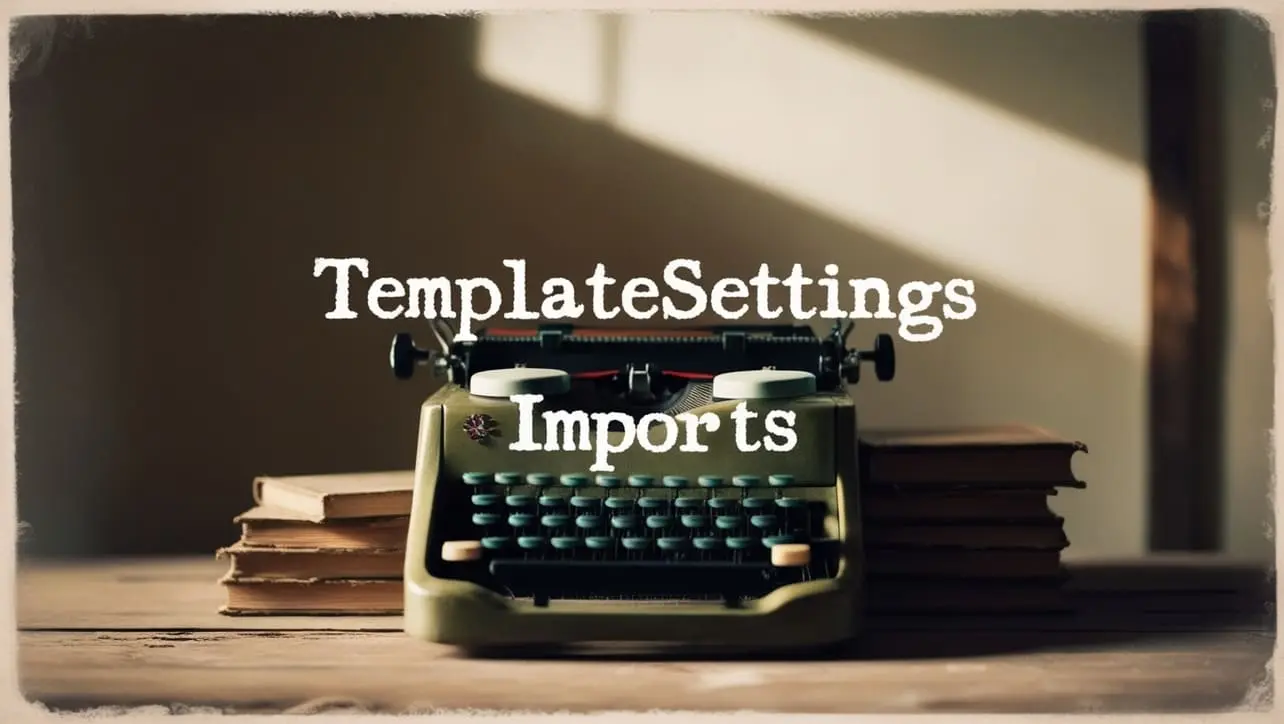
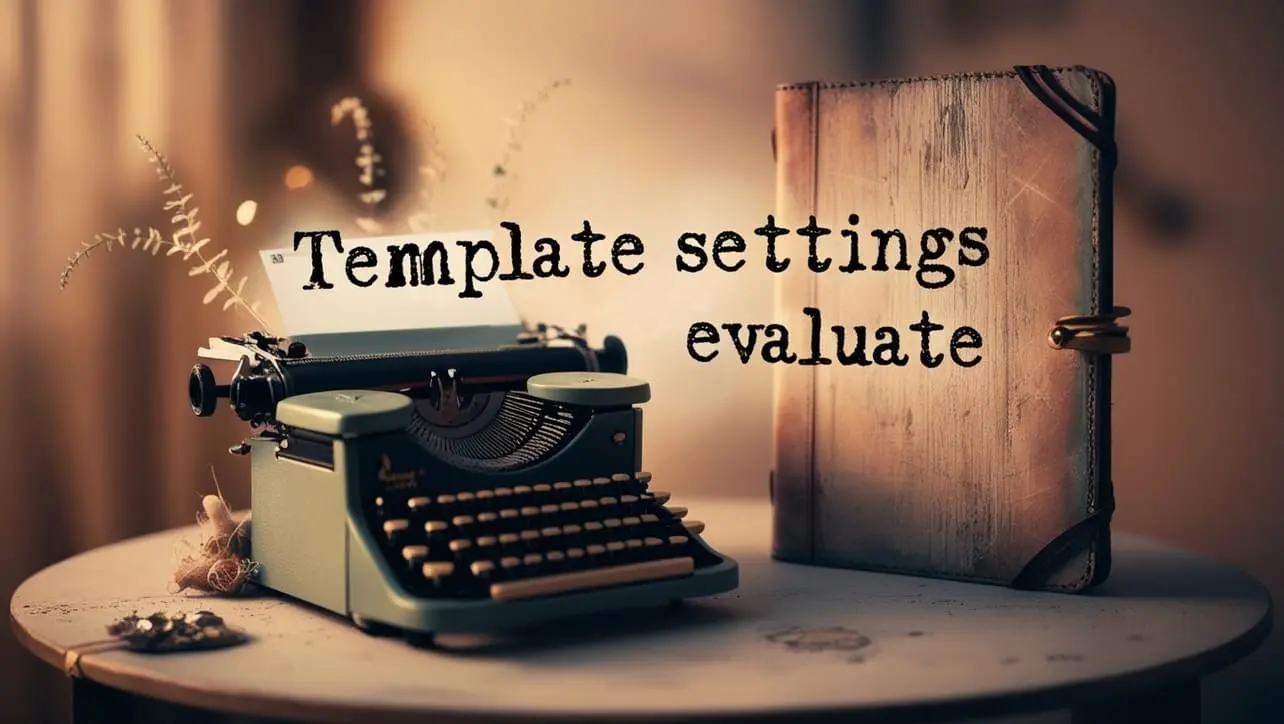
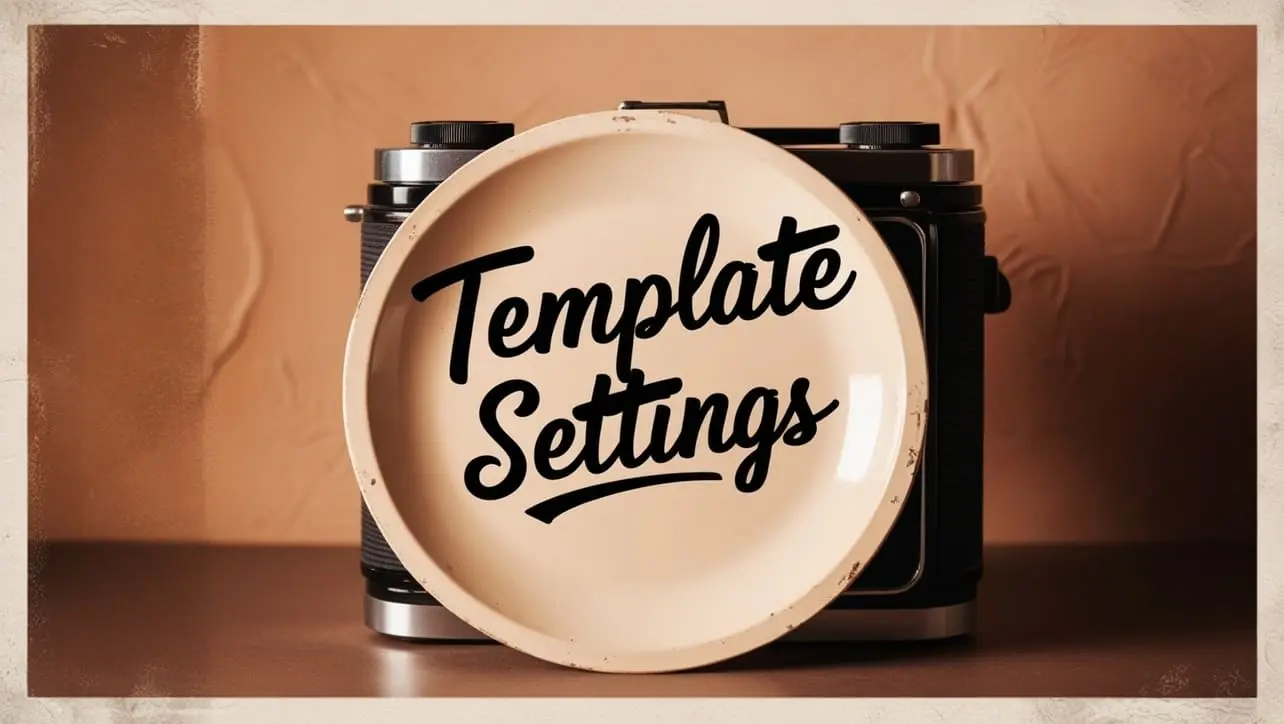
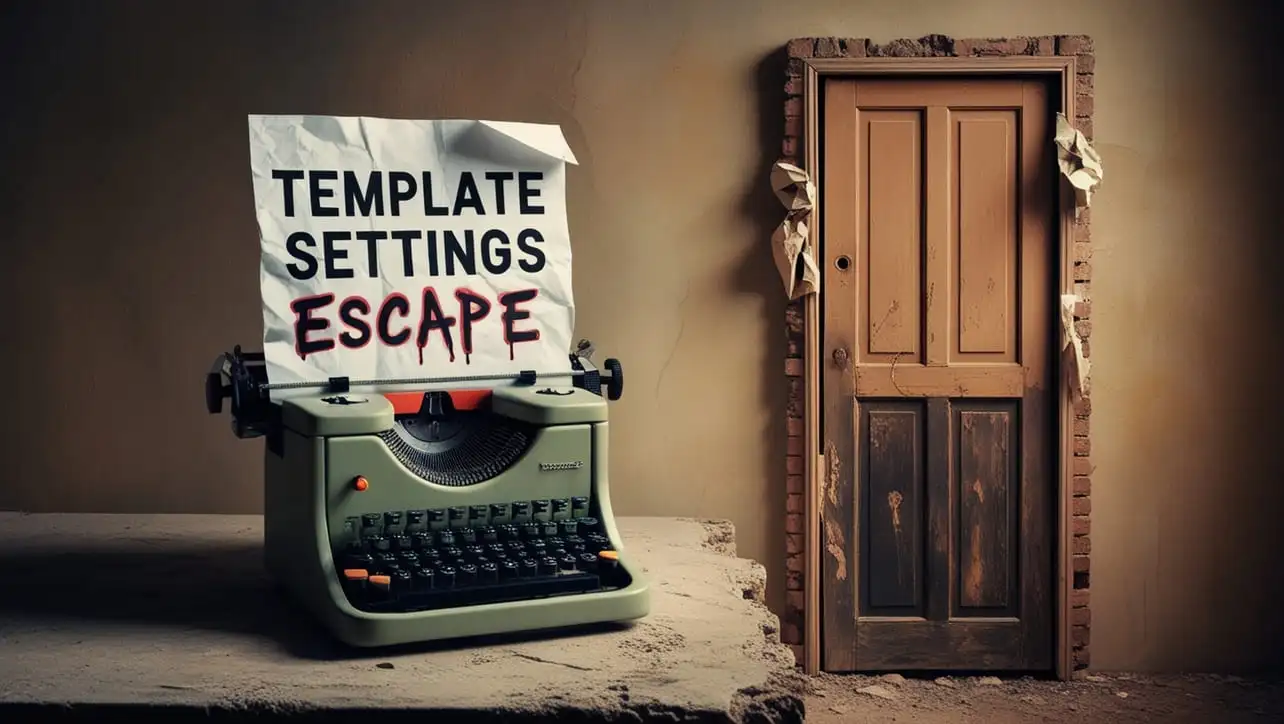
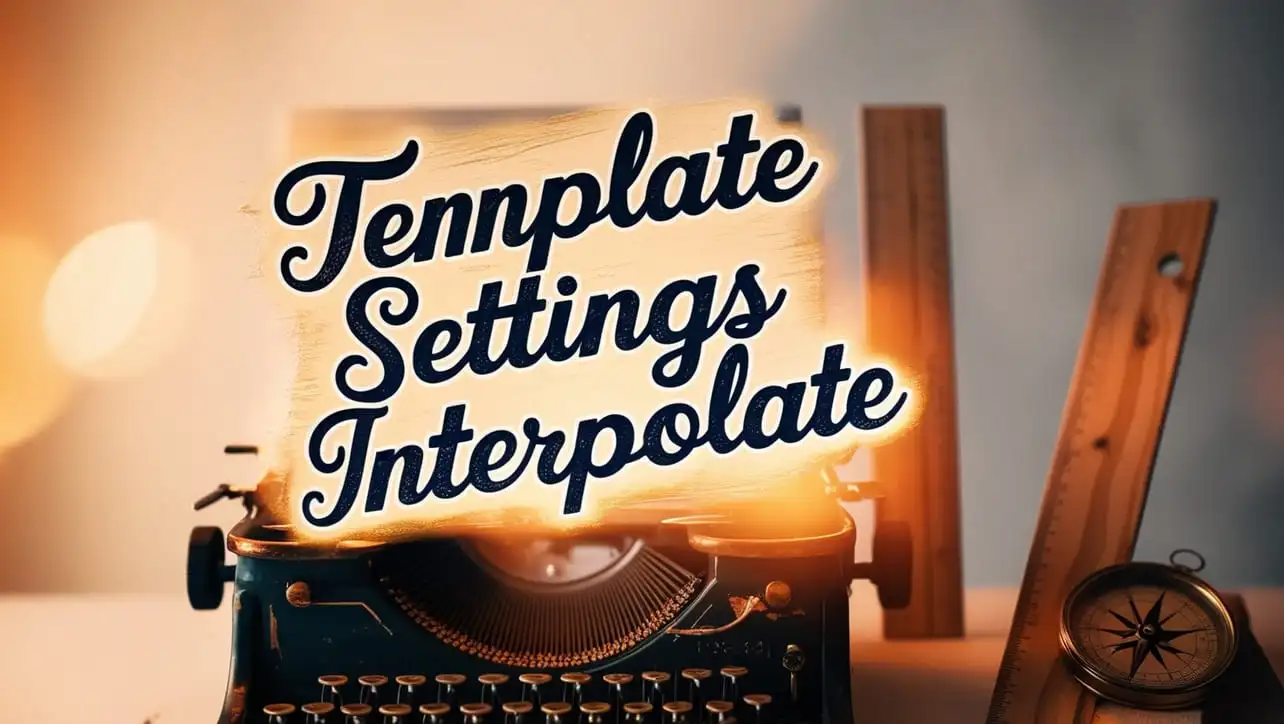
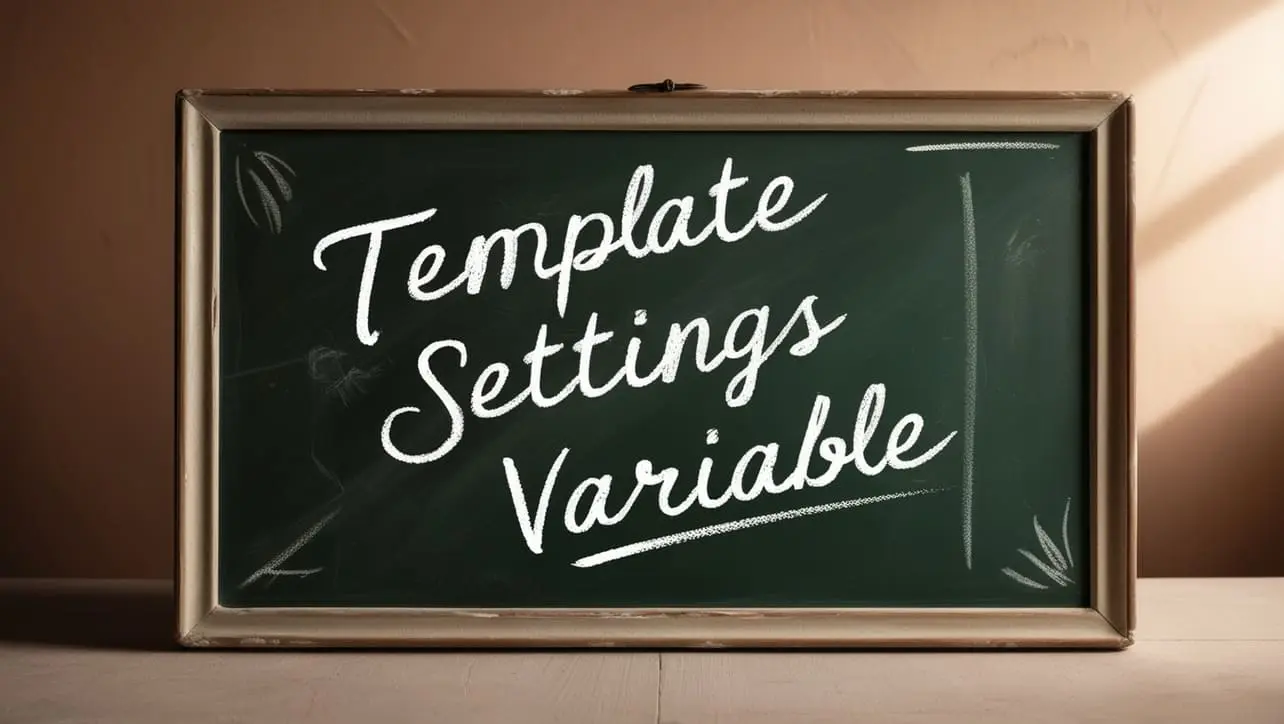
If you have any doubts regarding this article (Lodash _.clone() Lang Method), please comment here. I will help you immediately.