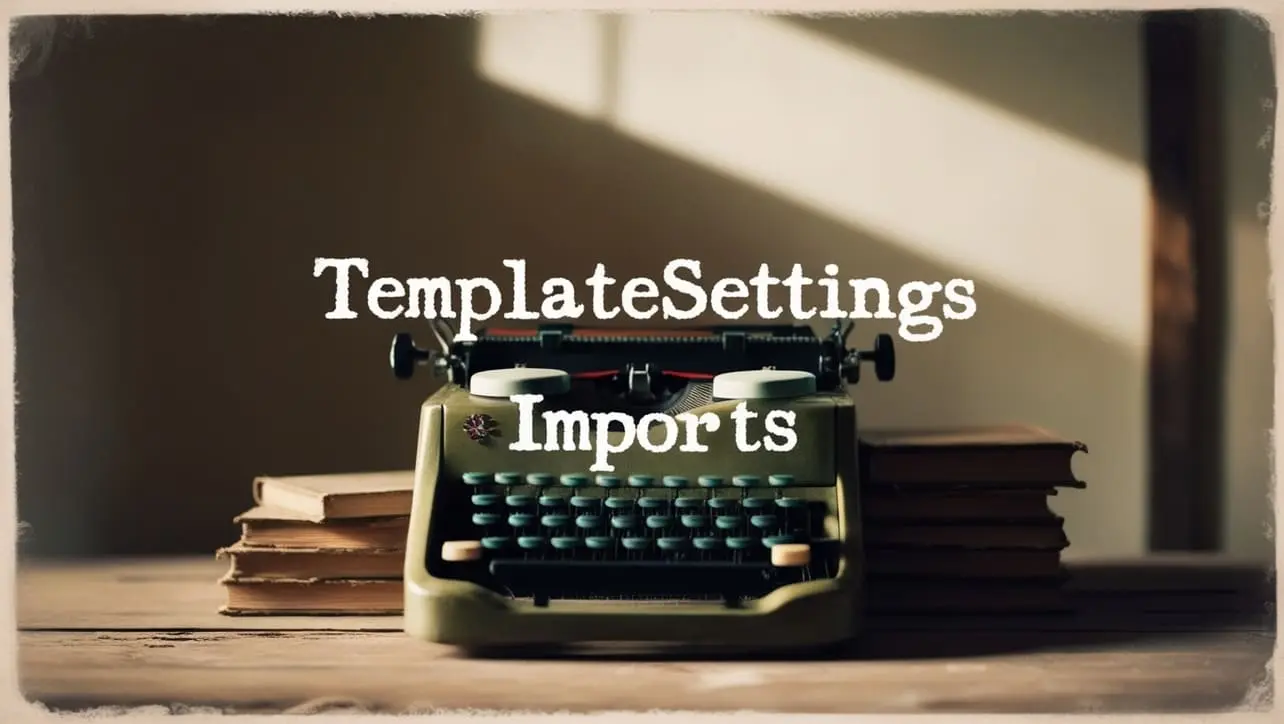
Lodash _.unary() Function Method
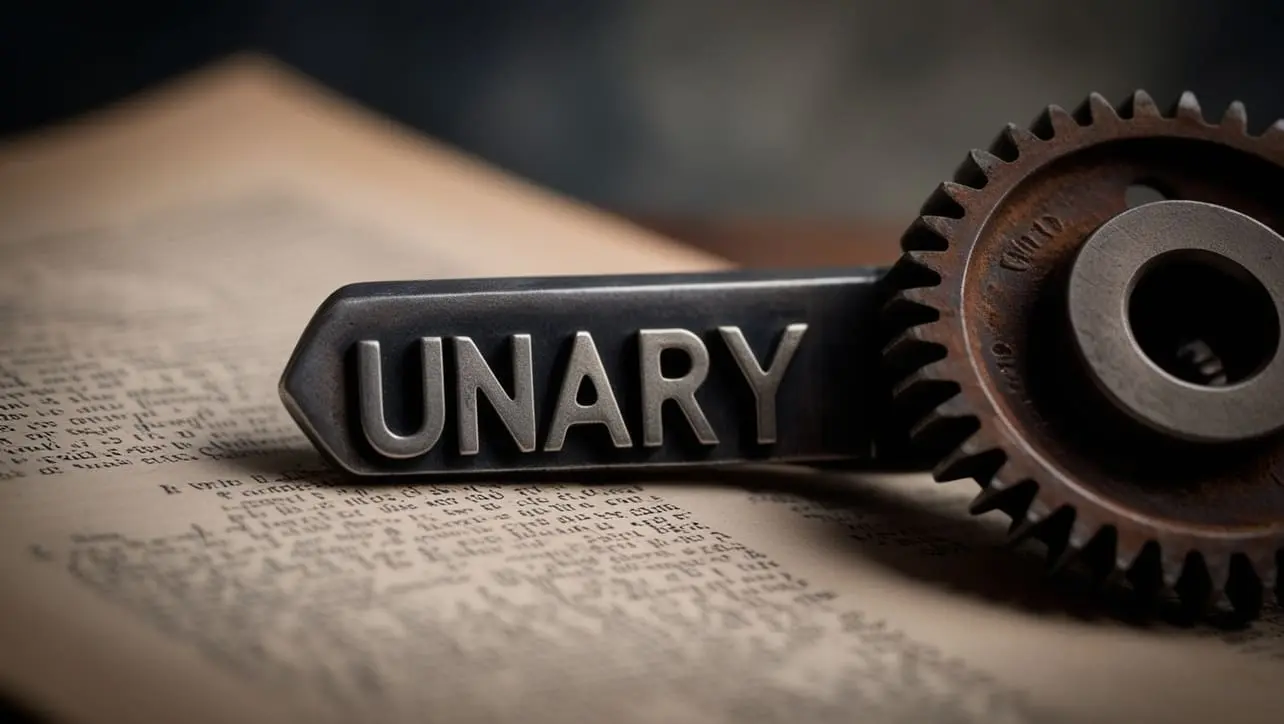
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript programming, fine-tuning functions is a common task. Lodash, a feature-rich utility library, introduces the _.unary()
function method to simplify and control the arity (number of arguments) of functions.
This method proves invaluable when you need to ensure a function receives only a specific number of arguments, enhancing code predictability and maintainability.
🧠 Understanding _.unary() Method
The _.unary()
method in Lodash is designed to create a new function that accepts only one argument, regardless of how many arguments are passed to it. This can be particularly useful when you want to adapt functions to work seamlessly in scenarios where a single argument is expected.
💡 Syntax
The syntax for the _.unary()
method is straightforward:
_.unary(func)
- func: The function to be wrapped.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.unary()
method:
const _ = require('lodash');
// Original function that accepts multiple arguments
const multiArgFunction = (a, b, c) => a + b + c;
// Wrap the function with _.unary()
const unaryFunction = _.unary(multiArgFunction);
console.log(unaryFunction(1, 2, 3));
// Output: 1 (only the first argument is considered)
In this example, the original function multiArgFunction accepts three arguments, but when wrapped with _.unary()
, it becomes unaryFunction, which only considers the first argument.
🏆 Best Practices
When working with the _.unary()
method, consider the following best practices:
Controlling Function Arity:
Use
_.unary()
when you need precise control over the number of arguments a function should receive. This can help prevent unexpected behavior and improve the clarity of your code.example.jsCopiedconst originalFunction = (a, b, c) => /* ... */; // Wrap the function with _.unary() for consistent behavior const unaryFunction = _.unary(originalFunction); console.log(unaryFunction(1, 2, 3)); // Only considers the first argument
Adapting Functions:
Adapt existing functions to work seamlessly in contexts where only a single argument is expected. This is particularly useful when dealing with APIs or higher-order functions that operate on unary functions.
example.jsCopiedconst originalApiFunction = (callback) => /* ... */; // Wrap the function with _.unary() for compatibility const unaryApiFunction = _.unary(originalApiFunction); console.log(unaryApiFunction(value => /* ... */)); // Ensures only one argument is passed
Enhancing Readability:
When dealing with functions that naturally work with a single argument, using
_.unary()
can enhance the readability of your code by explicitly signaling the intended arity.example.jsCopiedconst filterWithPredicate = _.unary(items => items.filter(item => /* ... */)); const data = /* ...fetch data from API or elsewhere... */; const filteredData = filterWithPredicate(data);
📚 Use Cases
Array Filtering:
When working with array filtering functions,
_.unary()
can be employed to ensure that the predicate function receives only one argument at a time, even though the filter function typically provides multiple arguments.example.jsCopiedconst filterWithUnary = _.unary((item) => /* ... */); const data = /* ...fetch data from API or elsewhere... */; const filteredData = data.filter(filterWithUnary);
API Request Handlers:
In scenarios where API request handlers expect callbacks with a fixed number of arguments,
_.unary()
can ensure that the callbacks receive only the necessary data, simplifying request handling logic.example.jsCopiedfunction handleApiResponse(data) { console.log('Received API response:', data); } fetch('https://api.example.com/data') .then(response => response.json()) .then(_.unary(handleApiResponse)) .catch(error => console.error('Error fetching data:', error));
🎉 Conclusion
The _.unary()
function method in Lodash offers a convenient way to control the arity of functions, ensuring they receive only a specific number of arguments.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.unary()
method in your Lodash projects.
👨💻 Join our Community:
Author
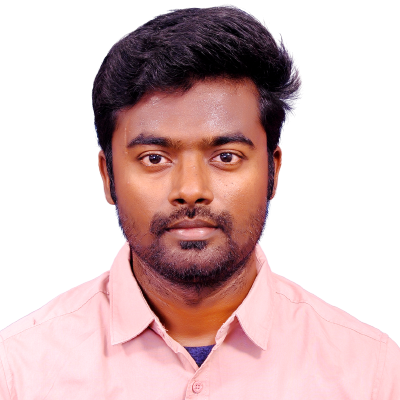
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
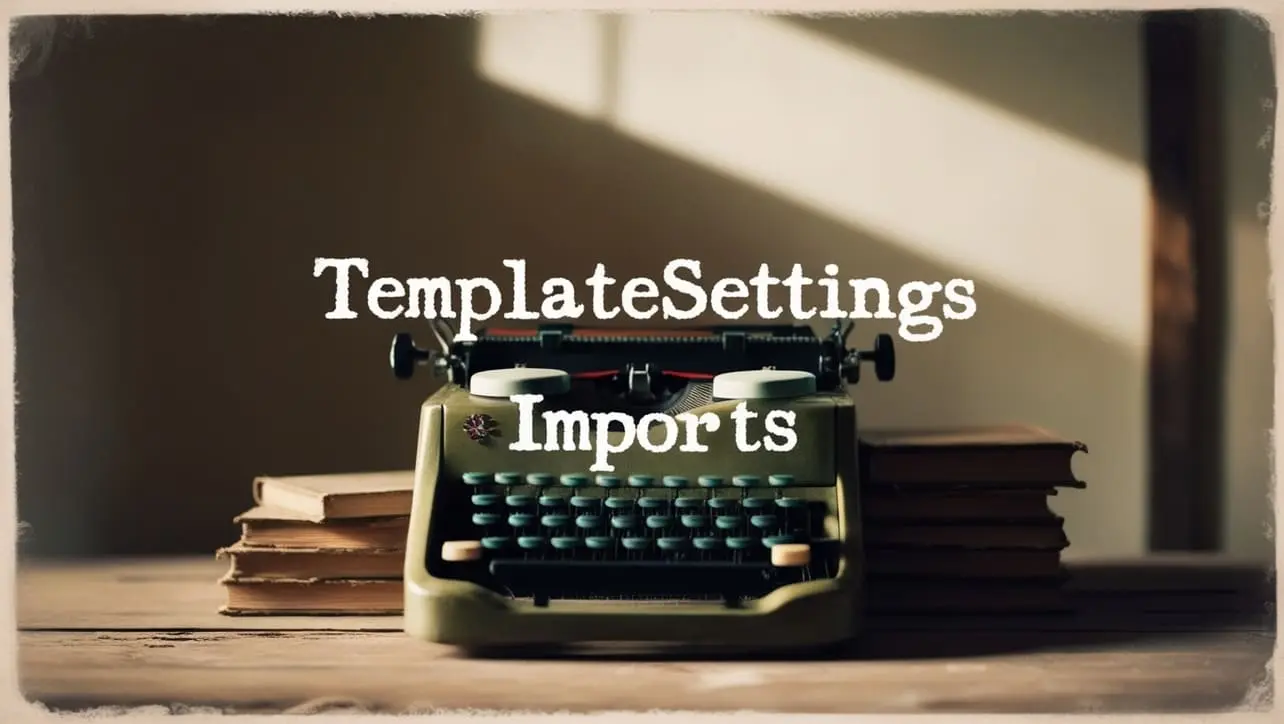
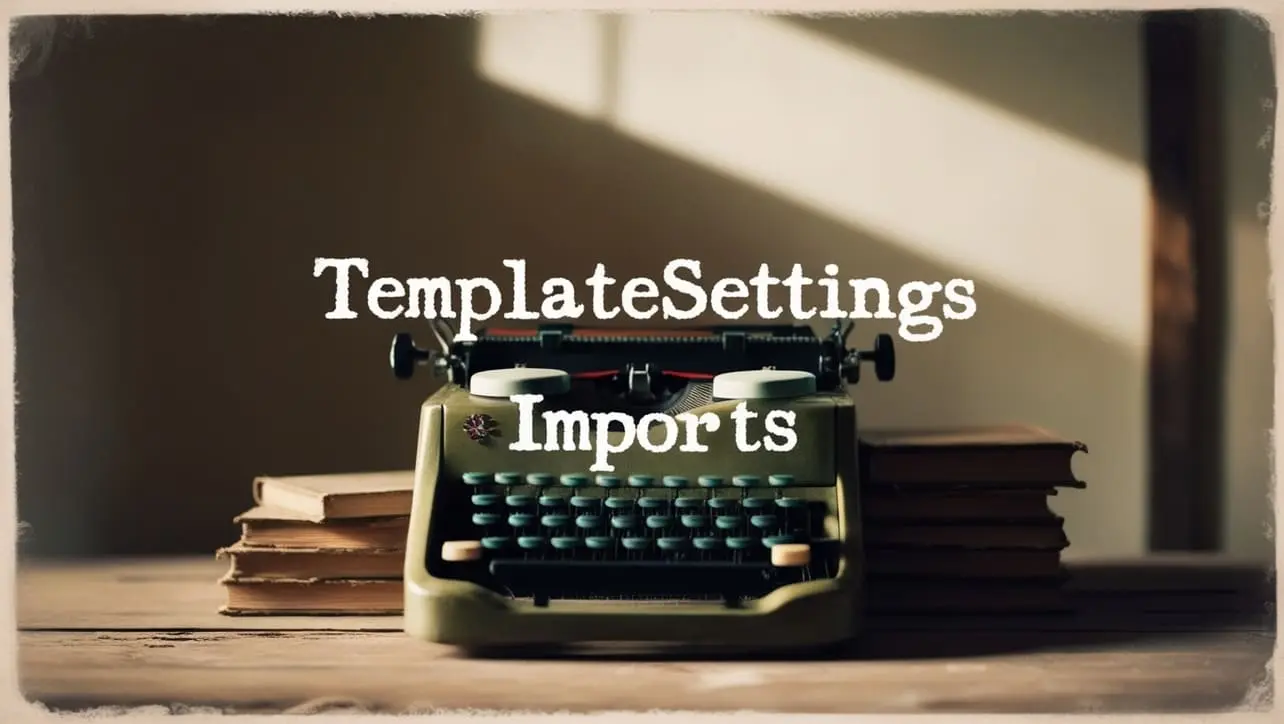
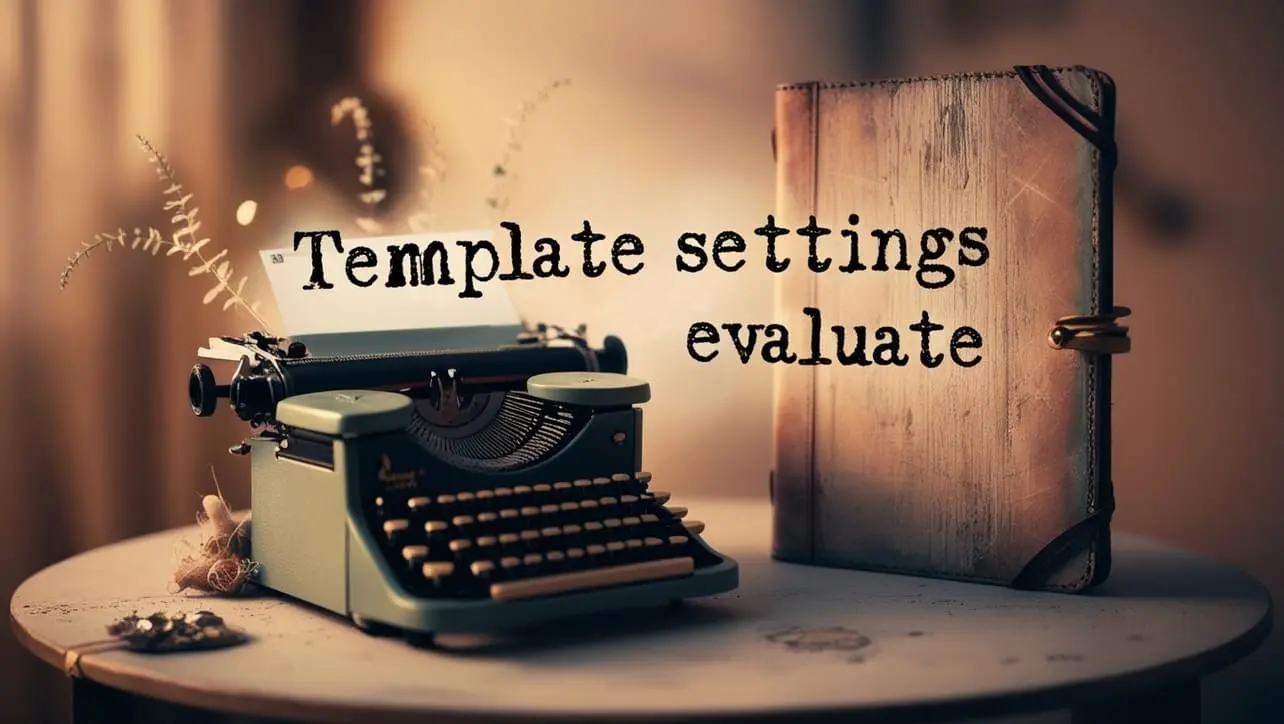
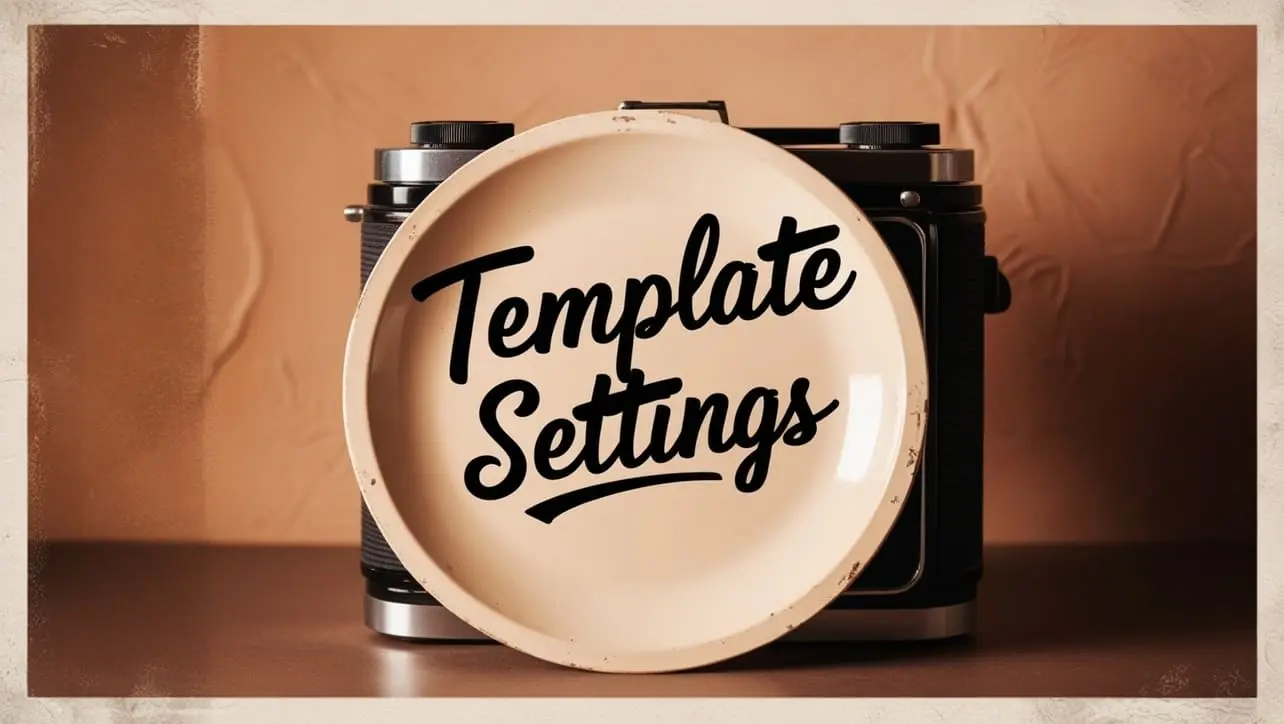
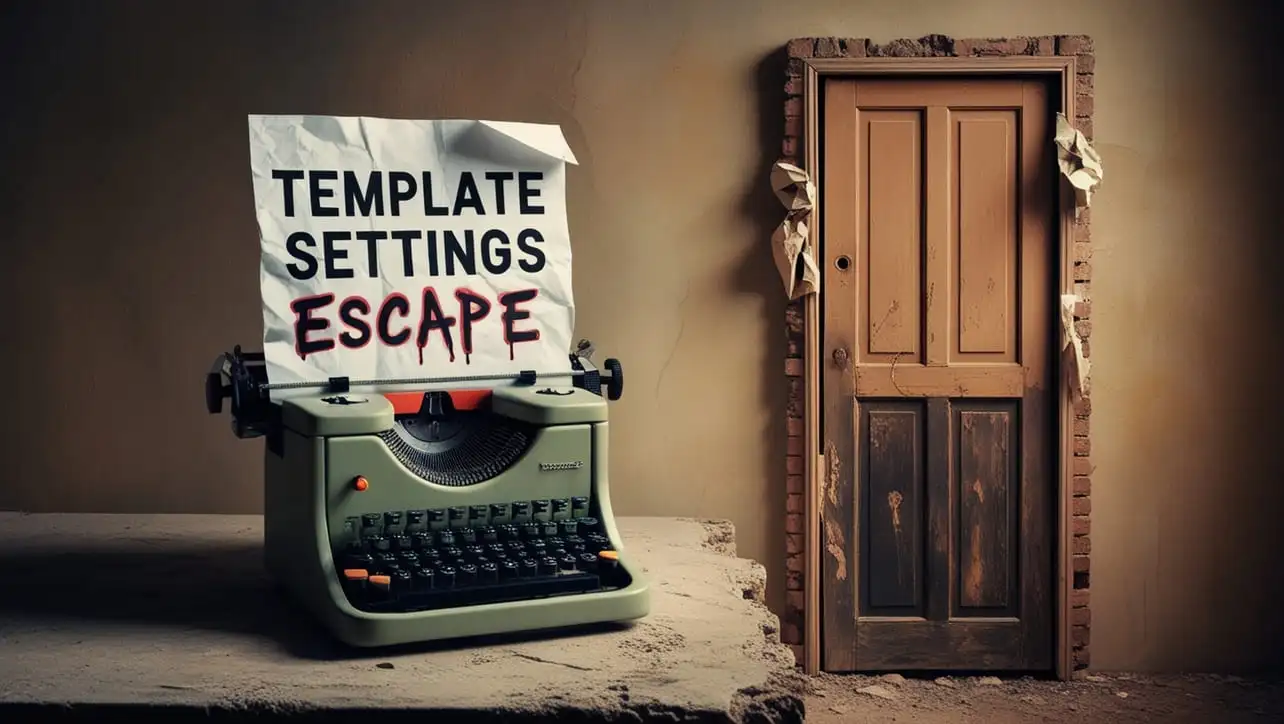
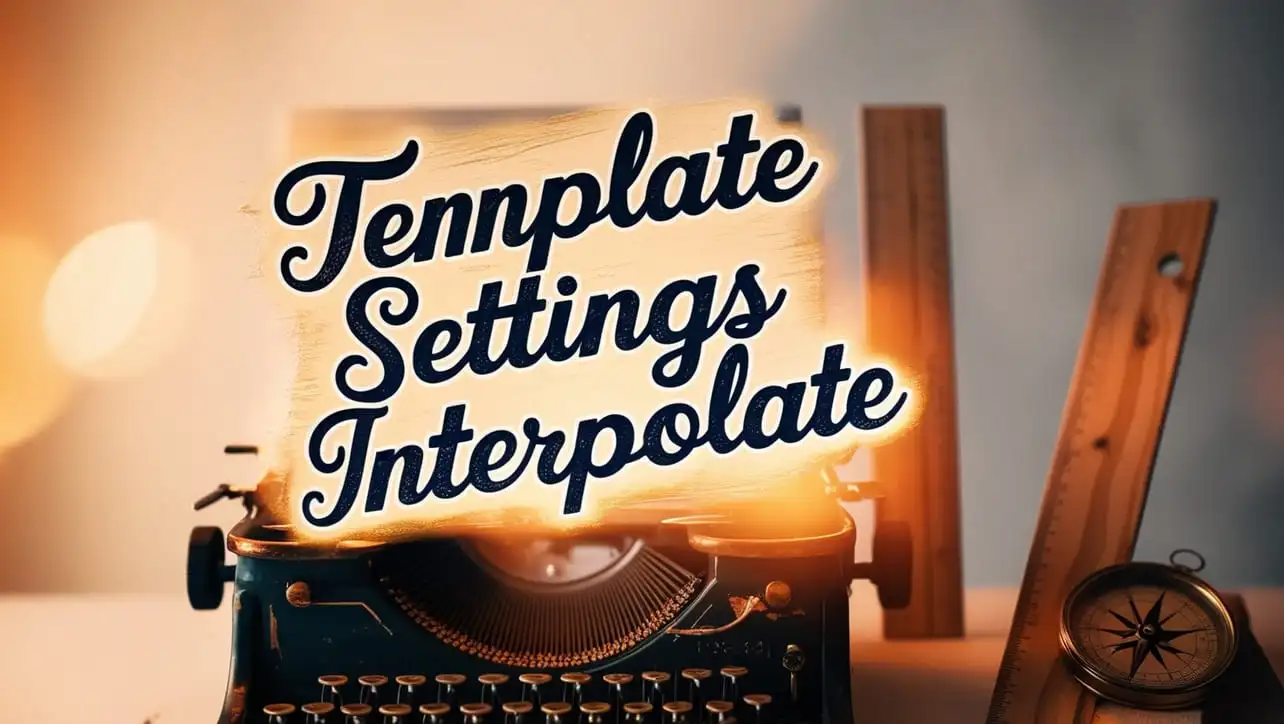
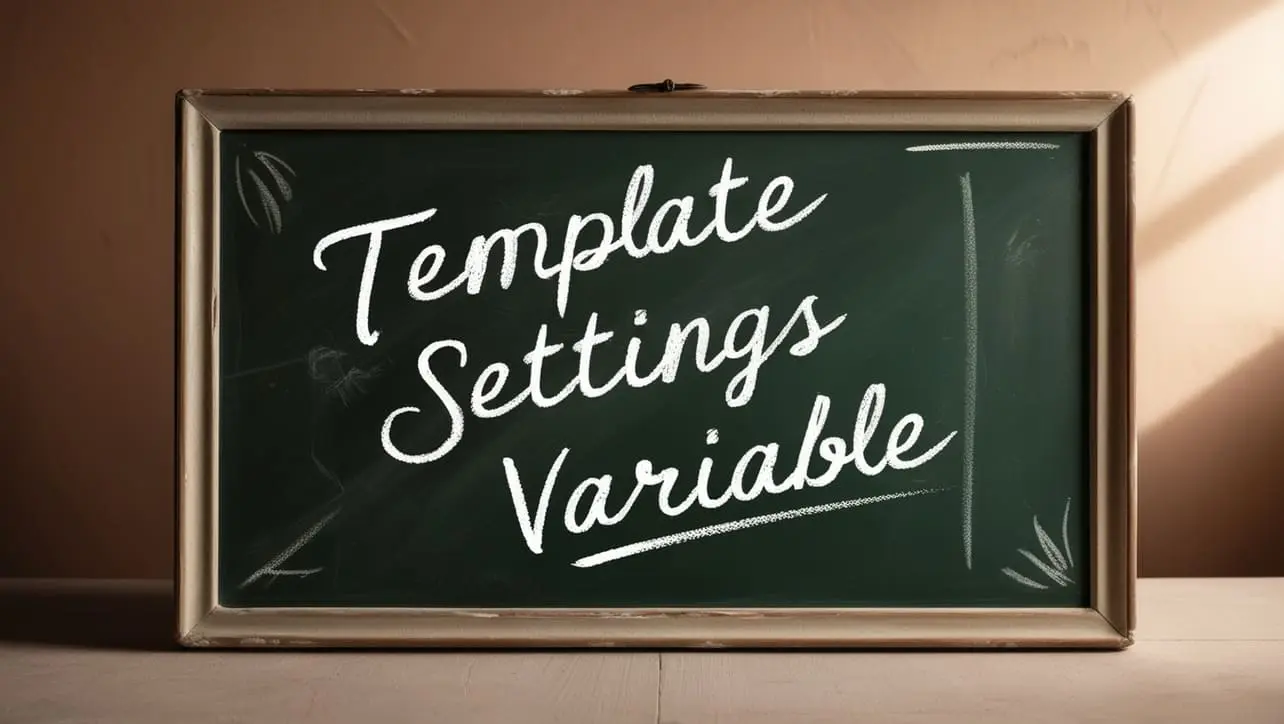
If you have any doubts regarding this article (Lodash _.unary() Function Method), please comment here. I will help you immediately.