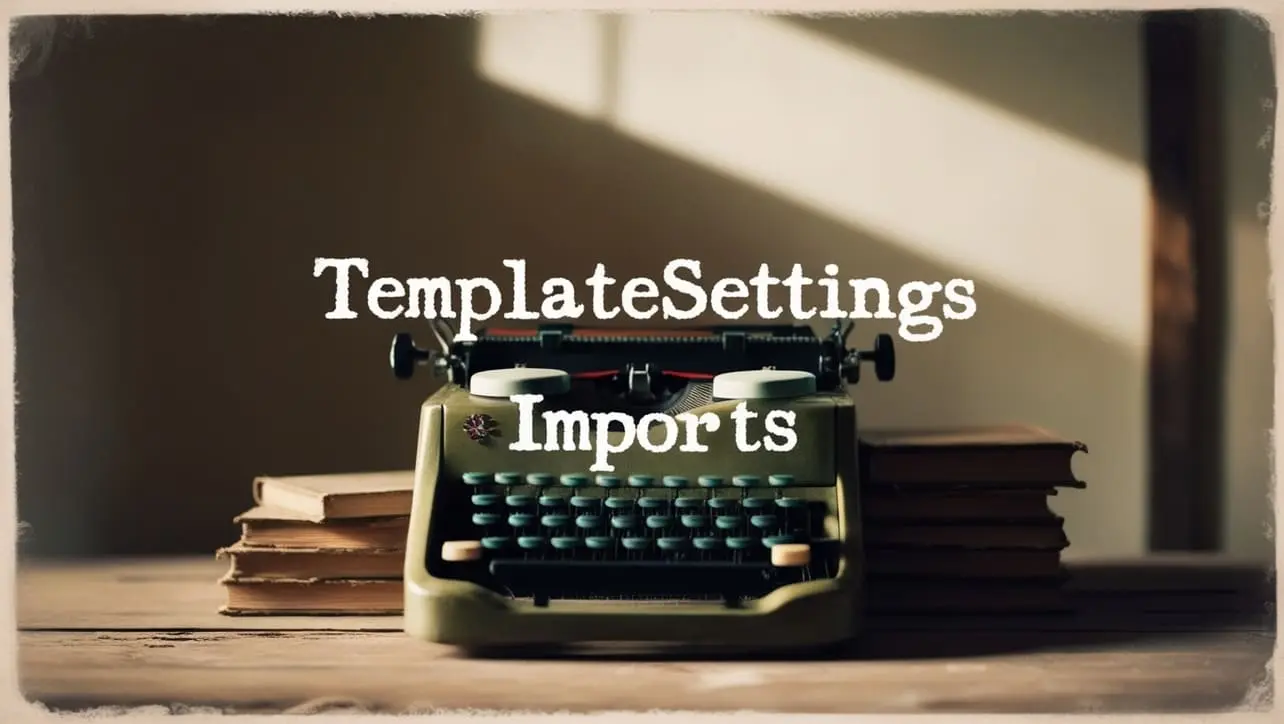
Lodash _.rest() Function Method
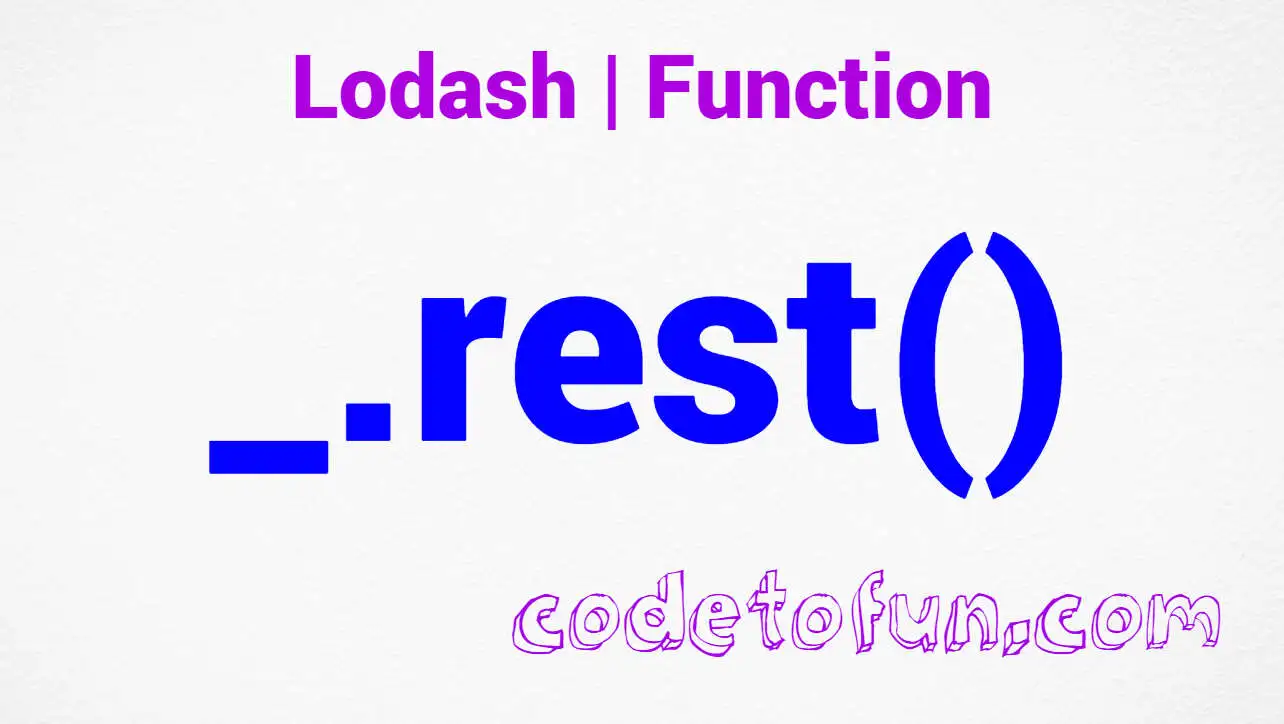
Photo Credit to CodeToFun
🙋 Introduction
In the ever-evolving landscape of JavaScript development, efficient array manipulation is a fundamental skill. Lodash, a versatile utility library, offers a range of functions to streamline these tasks. Among its arsenal is the _.rest()
function method, a tool designed to create a new array with all elements except the first.
This method proves invaluable for scenarios where excluding the initial elements of an array enhances data processing and readability.
🧠 Understanding _.rest() Method
The _.rest()
function method in Lodash extracts all elements from an array except the first one, creating a new array with the remaining elements. This can be particularly useful when you want to skip or exclude specific elements at the beginning of an array.
💡 Syntax
The syntax for the _.rest()
method is straightforward:
_.rest(array)
- array: The array from which to extract elements.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.rest()
method:
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5];
const newArray = _.rest(originalArray);
console.log(newArray);
// Output: [2, 3, 4, 5]
In this example, _.rest()
is applied to originalArray, resulting in a new array (newArray) that excludes the first element.
🏆 Best Practices
When working with the _.rest()
method, consider the following best practices:
Validate Array Length:
Before using
_.rest()
, validate that the input array has sufficient length to exclude elements. Attempting to extract elements from an empty array or an array with only one element may lead to unexpected results.example.jsCopiedconst emptyArray = []; const singleElementArray = [42]; const resultEmptyArray = _.rest(emptyArray); const resultSingleElementArray = _.rest(singleElementArray); console.log(resultEmptyArray); // Output: [] console.log(resultSingleElementArray); // Output: []
Use with Destructuring:
Leverage array destructuring in JavaScript to capture the rest of the elements conveniently.
example.jsCopiedconst originalArray = [1, 2, 3, 4, 5]; const [firstElement, ...restElements] = originalArray; console.log(firstElement); // Output: 1 console.log(restElements); // Output: [2, 3, 4, 5]
Combine with Other Lodash Methods:
Integrate
_.rest()
with other Lodash methods to perform more complex array manipulations.example.jsCopiedconst originalArray = [1, 2, 3, 4, 5]; const modifiedArray = _.map(_.rest(originalArray), num => num * 2); console.log(modifiedArray); // Output: [4, 6, 8, 10]
📚 Use Cases
Removing Header Rows:
In scenarios where you have an array representing a table and want to exclude the header row,
_.rest()
proves handy.example.jsCopiedconst tableData = [ ['Name', 'Age', 'Country'], ['John Doe', 30, 'USA'], ['Jane Smith', 25, 'Canada'], ]; const bodyRows = _.rest(tableData); console.log(bodyRows);
Pagination:
For paginated displays, excluding the first set of elements can be useful to navigate through pages effectively.
example.jsCopiedconst allData = /* ...fetch data from API or elsewhere... */; const itemsPerPage = 10; const currentPageData = _.rest(allData, itemsPerPage); console.log(currentPageData);
Dynamic Function Parameters:
When dealing with functions that accept variable arguments,
_.rest()
can help capture and process the remaining parameters.example.jsCopiedfunction logRemainingArgs(firstArg, ...restArgs) { console.log('First argument:', firstArg); console.log('Remaining arguments:', restArgs); } logRemainingArgs('Hello', 'World', 42, true);
🎉 Conclusion
The _.rest()
function method in Lodash provides a concise way to create a new array excluding the first elements. Whether you're skipping header rows, implementing pagination, or dynamically handling function parameters, _.rest()
proves to be a versatile tool for array manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.rest()
method in your Lodash projects.
👨💻 Join our Community:
Author
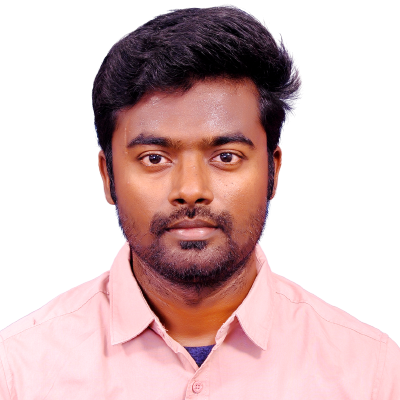
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
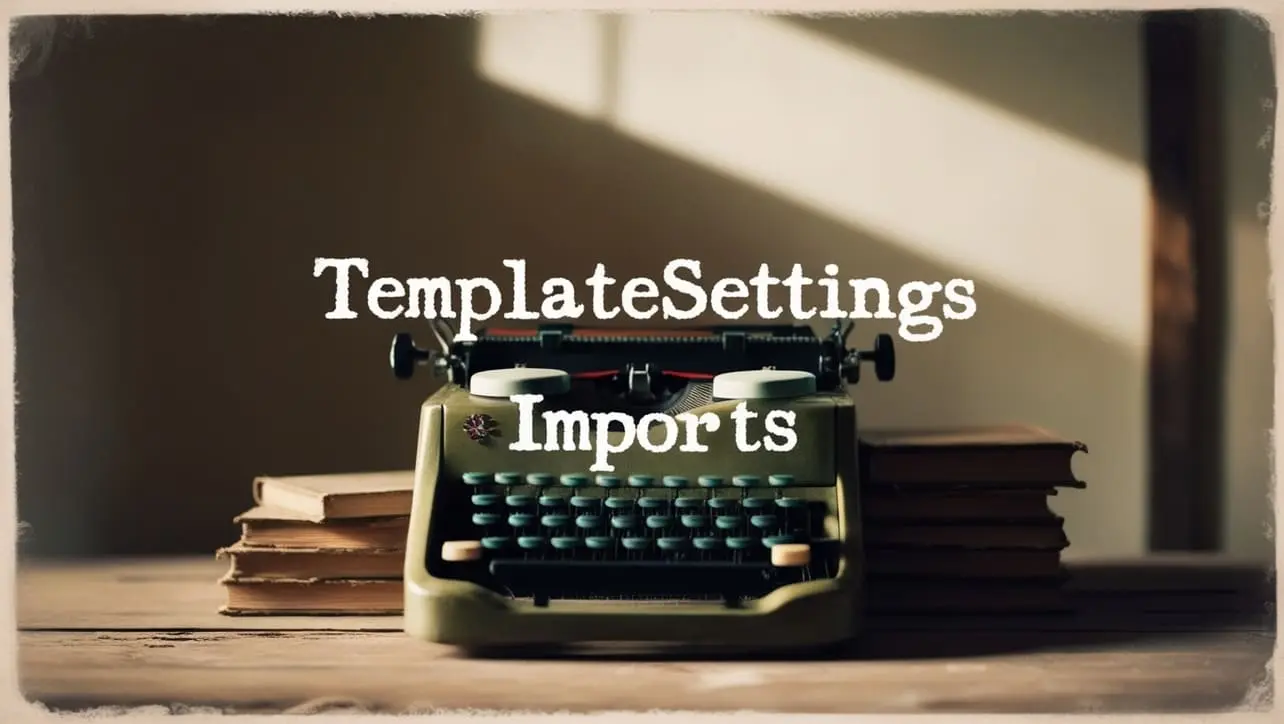
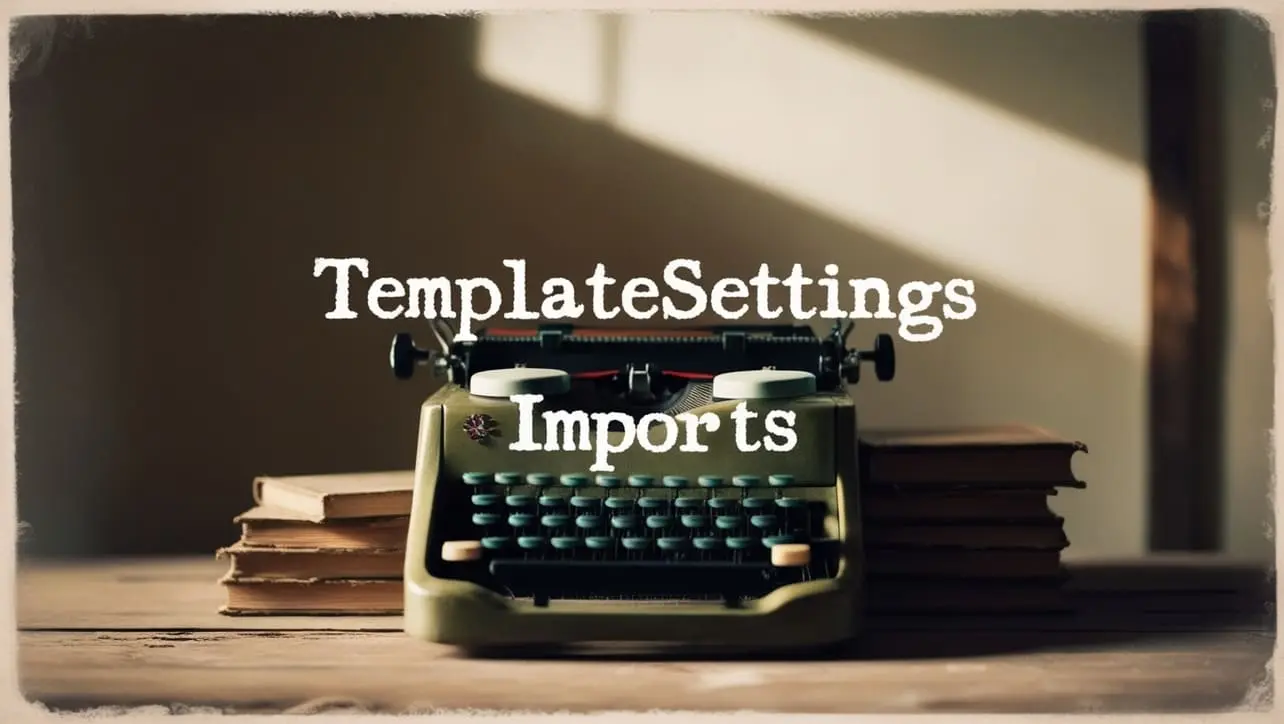
Lodash _.templateSettings.imports Property
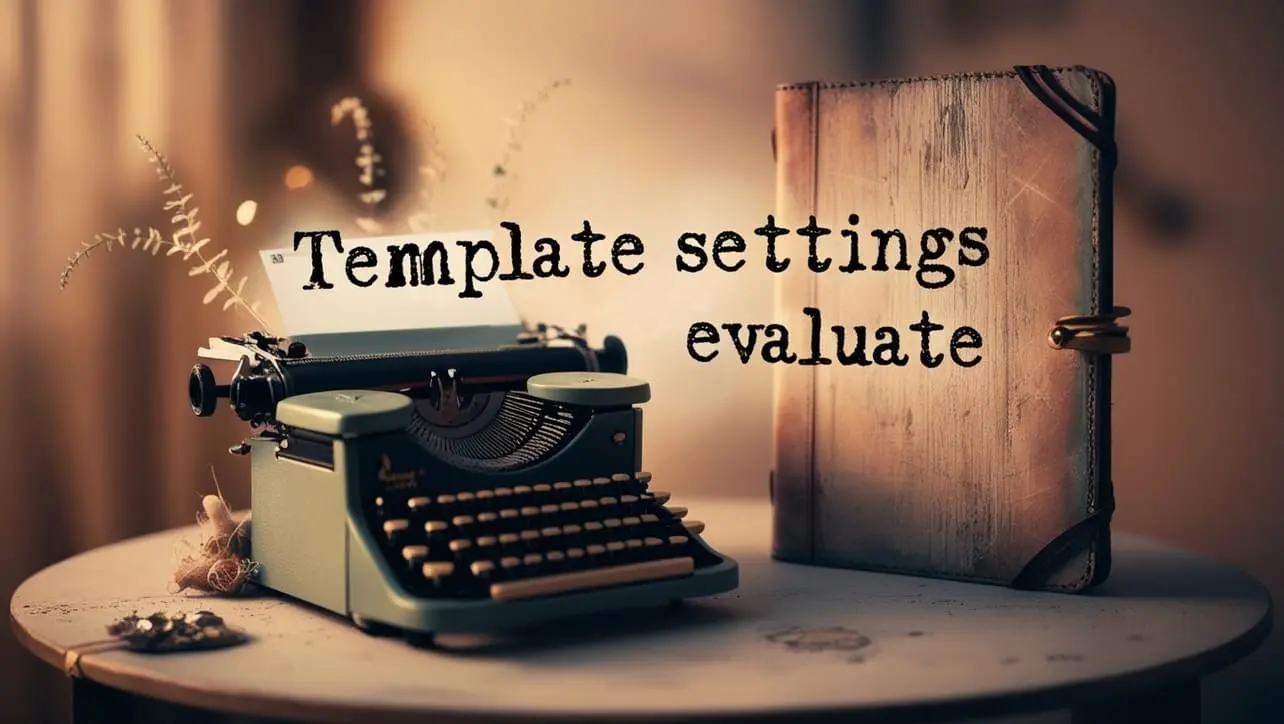
Lodash _.templateSettings.evaluate Property
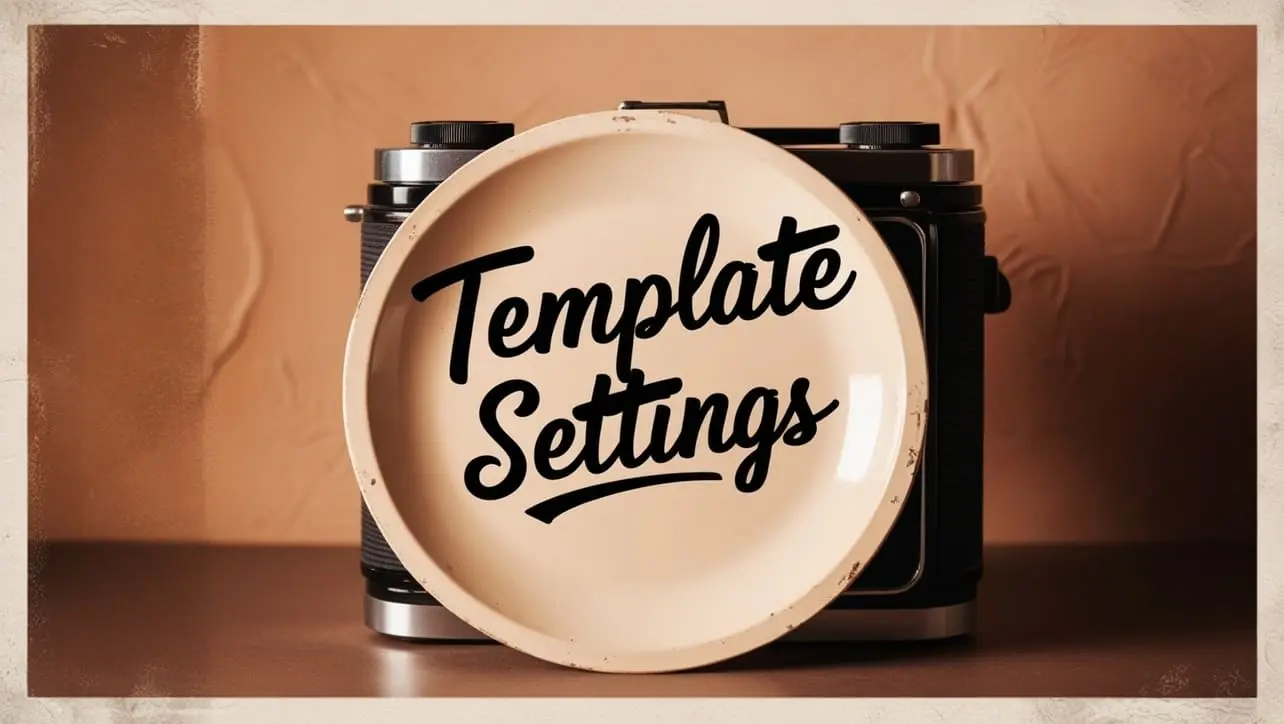
Lodash _.templateSettings Property
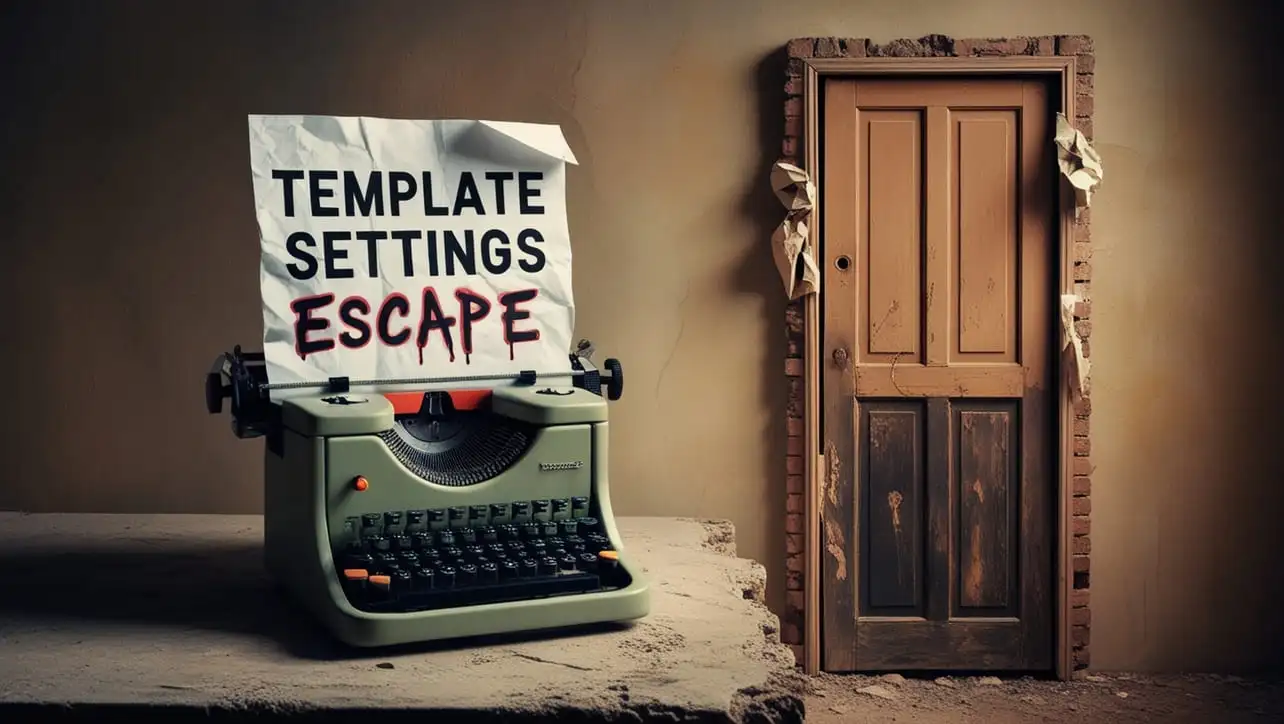
Lodash _.templateSettings.escape Property
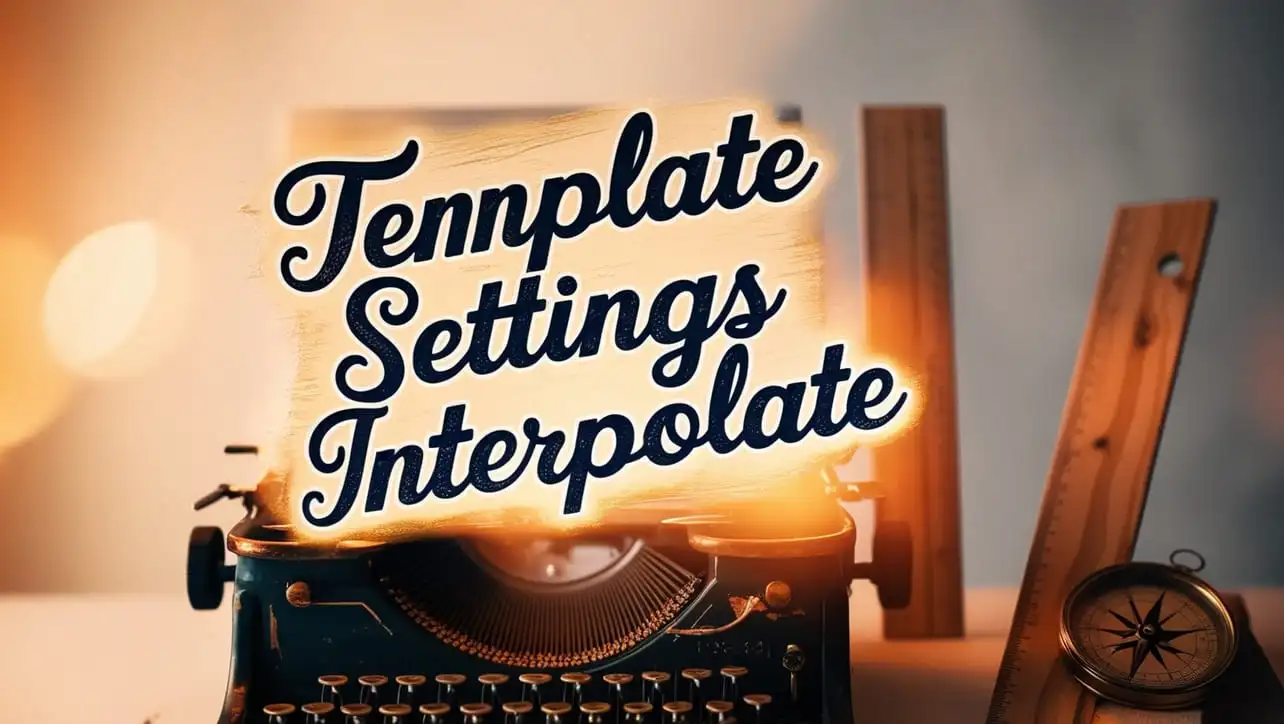
Lodash _.templateSettings.interpolate Property
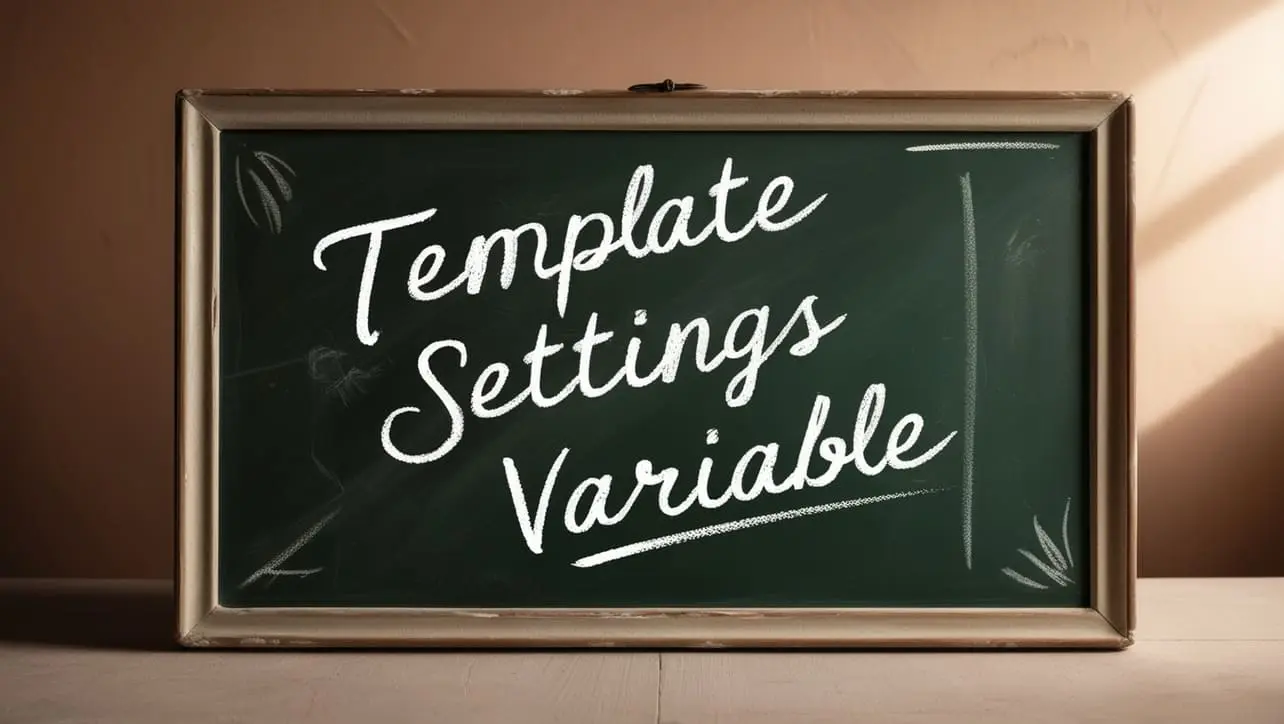
If you have any doubts regarding this article (Lodash _.rest() Function Method), please comment here. I will help you immediately.