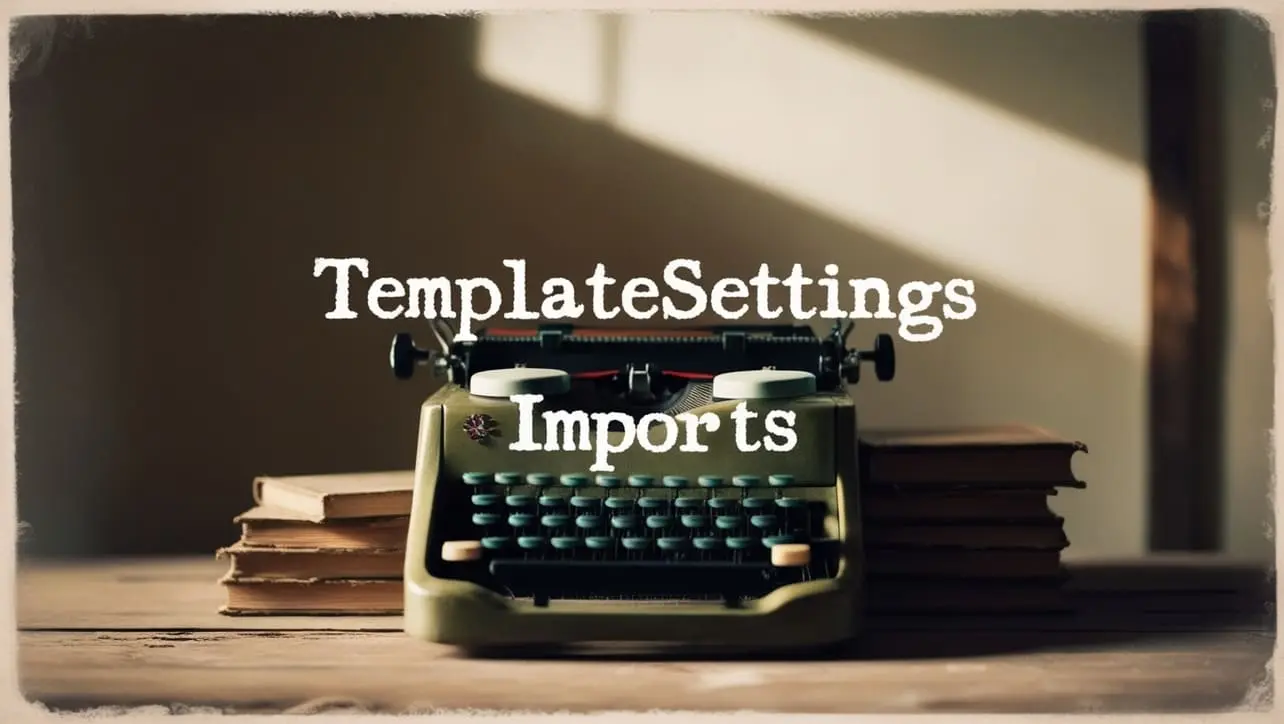
Lodash _.partialRight() Function Method
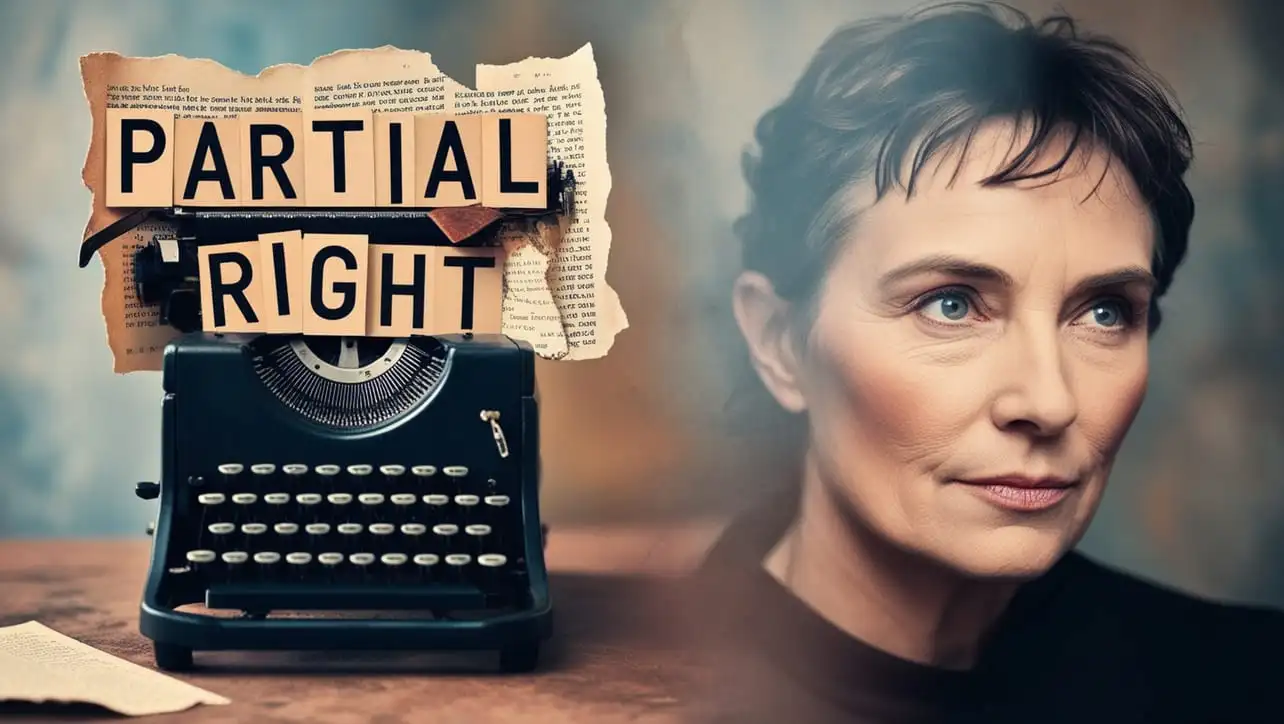
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript programming, enhancing the flexibility and reusability of functions is a common goal. Lodash, a powerful utility library, offers a variety of functions to streamline development tasks. Among these features is the _.partialRight()
method, a tool that allows developers to create partially applied functions with arguments fixed from the right.
This method is particularly useful for creating flexible and modular functions in a concise manner.
🧠 Understanding _.partialRight() Method
The _.partialRight()
method in Lodash is designed to create a partially applied function with arguments fixed from the right side. This means you can preset some arguments in a function, leaving others open for dynamic input when the partially applied function is invoked. This flexibility enables the creation of versatile and reusable functions.
💡 Syntax
The syntax for the _.partialRight()
method is straightforward:
_.partialRight(func, [partials])
- func: The function to partially apply arguments to.
- partials: The arguments to be fixed from the right side.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.partialRight()
method:
const _ = require('lodash');
// Original function
function greet(name, greeting, punctuation) {
return `${greeting}, ${name}${punctuation}`;
}
// Create a partially applied function with the greeting fixed to 'Hello'
const sayHello = _.partialRight(greet, 'Hello');
// Invoke the partially applied function with the dynamic name
const result = sayHello('John', '!');
console.log(result);
// Output: "Hello, John!"
In this example, the _.partialRight()
method is used to create a new function (sayHello) with the greeting fixed to 'Hello'. When the partially applied function is invoked with the dynamic name 'John', it produces the greeting message "Hello, John!".
🏆 Best Practices
When working with the _.partialRight()
method, consider the following best practices:
Clarify Intent with Named Functions:
When using
_.partialRight()
, consider creating named functions to enhance code readability and express the intent of the partially applied function.example.jsCopiedconst _ = require('lodash'); function formatCurrency(symbol, amount, precision) { // Function logic for formatting currency // ... } // Create a named partially applied function for formatting dollars const formatDollars = _.partialRight(formatCurrency, '$'); // Invoke the partially applied function const result = formatDollars(25.5, 2); console.log(result); // Output: "$25.50"
Reuse Partially Applied Functions:
Take advantage of the reusability of partially applied functions by using them in various contexts. This promotes modular code and reduces redundancy.
example.jsCopiedconst _ = require('lodash'); function generateGreeting(greeting, name) { return `${greeting}, ${name}!`; } // Create a partially applied function with the fixed greeting 'Welcome' const welcomeMessage = _.partialRight(generateGreeting, 'Welcome'); // Create another partially applied function with the fixed greeting 'Hello' const helloMessage = _.partialRight(generateGreeting, 'Hello'); // Invoke the partially applied functions console.log(welcomeMessage('Alice')); // Output: "Welcome, Alice!" console.log(helloMessage('Bob')); // Output: "Hello, Bob!"
Maintain Function Flexibility:
When using
_.partialRight()
, be mindful of maintaining the flexibility of the original function by fixing only the necessary arguments. Avoid fixing all arguments unless absolutely required for your use case.example.jsCopiedconst _ = require('lodash'); function multiply(a, b, c) { return a * b * c; } // Create a partially applied function with the fixed value for 'c' const multiplyByTwo = _.partialRight(multiply, 2); // Invoke the partially applied function with dynamic values for 'a' and 'b' const result = multiplyByTwo(3, 4); console.log(result); // Output: 24
📚 Use Cases
Creating Specialized Functions:
Use
_.partialRight()
to create specialized versions of functions tailored for specific use cases. This promotes code modularity and allows for more expressive and focused functions.example.jsCopiedconst _ = require('lodash'); // Original function function calculateInterest(principal, rate, time) { return (principal * rate * time) / 100; } // Create a specialized version for calculating annual interest const calculateAnnualInterest = _.partialRight(calculateInterest, 1); // Invoke the specialized function with dynamic principal and rate const result = calculateAnnualInterest(1000, 5); console.log(result); // Output: 50
Simplifying Callback Functions:
When working with functions that accept callbacks, use
_.partialRight()
to simplify callback functions by fixing certain arguments.example.jsCopiedconst _ = require('lodash'); // Original function that accepts a callback function fetchData(url, callback) { // Logic to fetch data from the provided URL const data = /* ... */; callback(data); } // Create a partially applied function with the fixed URL const fetchFromAPI = _.partialRight(fetchData, 'https://api.example.com'); // Invoke the partially applied function with a dynamic callback fetchFromAPI(data => { console.log('Received data:', data); });
Enhancing Function Composition:
Leverage
_.partialRight()
to enhance function composition by creating partially applied functions that seamlessly fit into a chain of operations.example.jsCopiedconst _ = require('lodash'); // Original functions function add(a, b) { return a + b; } function square(n) { return n * n; } // Create a partially applied function for adding 5 to a value const add5 = _.partialRight(add, 5); // Create a composed function that squares the result of adding 5 const squareResult = _.flow([add5, square]); // Invoke the composed function with a dynamic value const result = squareResult(3); console.log(result); // Output: 64
🎉 Conclusion
The _.partialRight()
method in Lodash is a valuable tool for creating partially applied functions with fixed arguments from the right.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.partialRight()
method in your Lodash projects.
👨💻 Join our Community:
Author
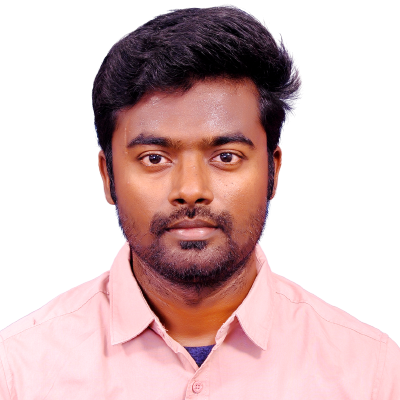
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
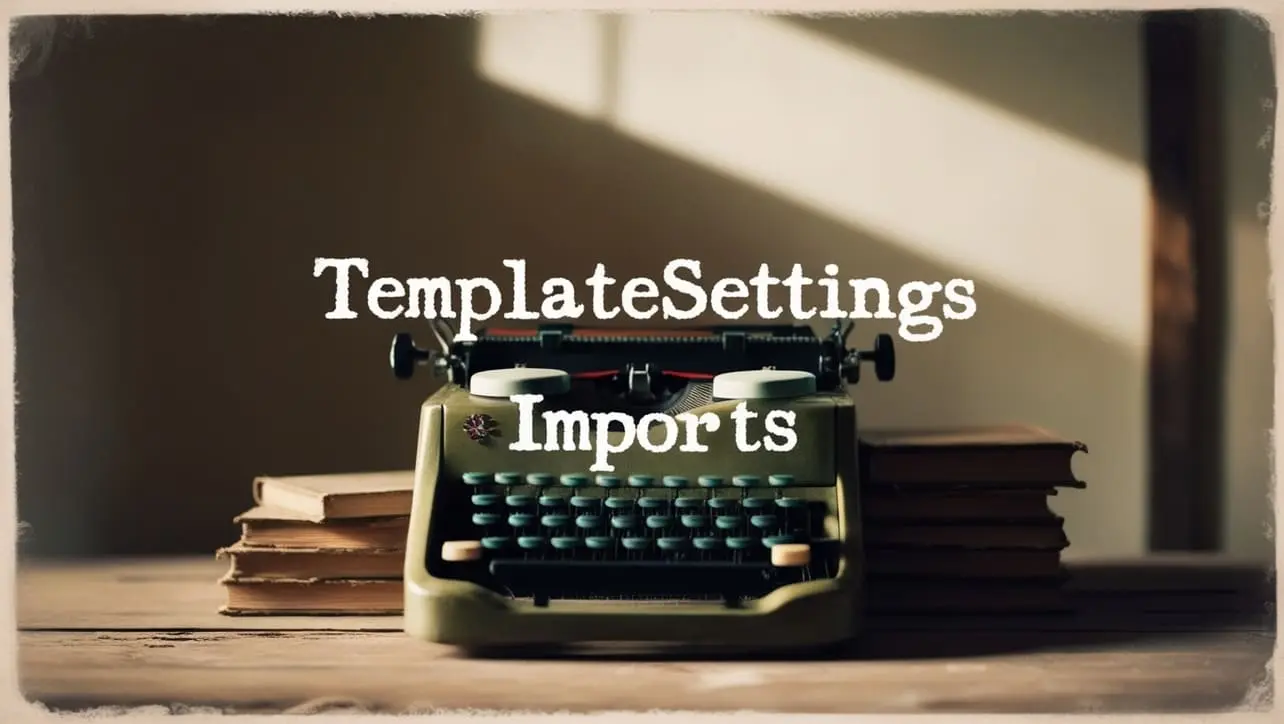
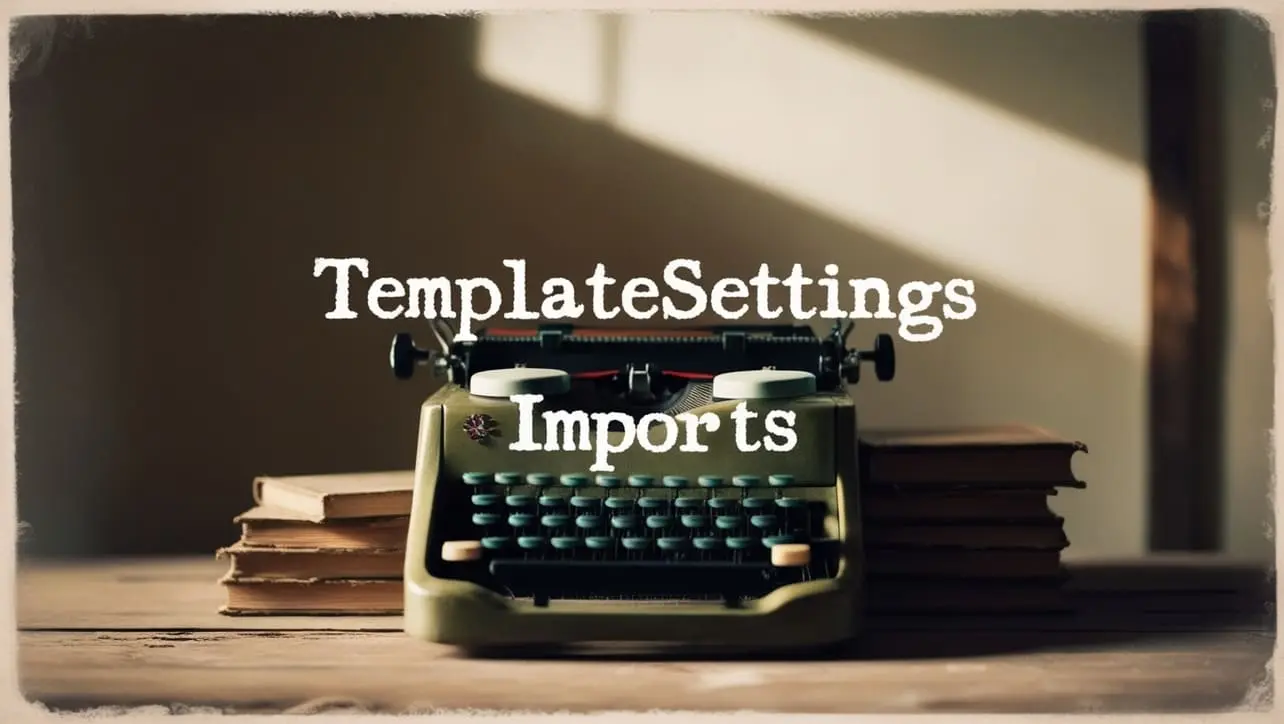
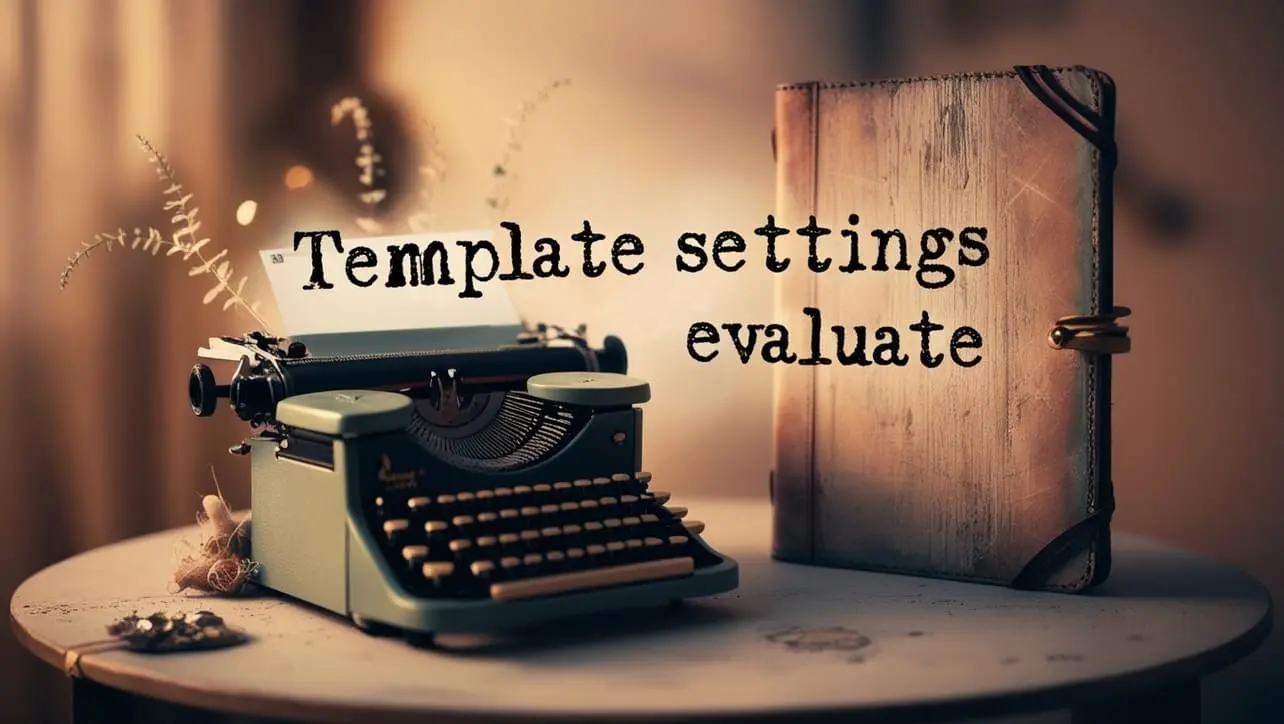
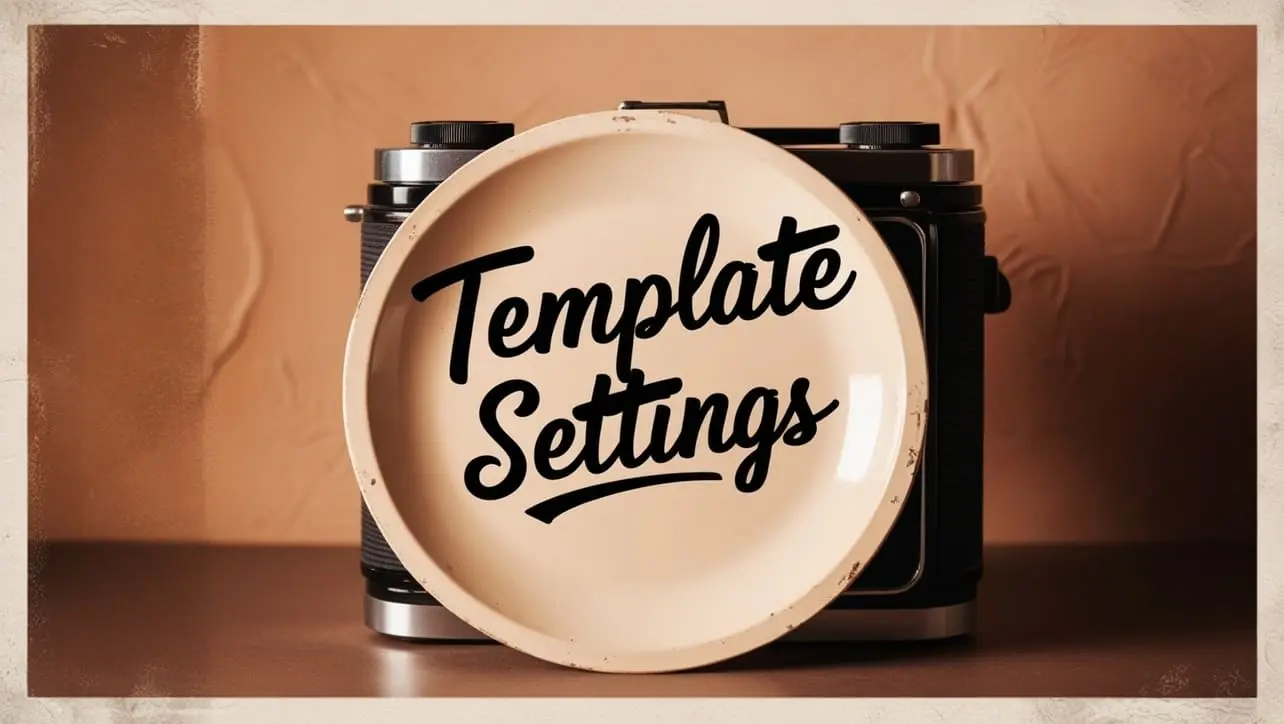
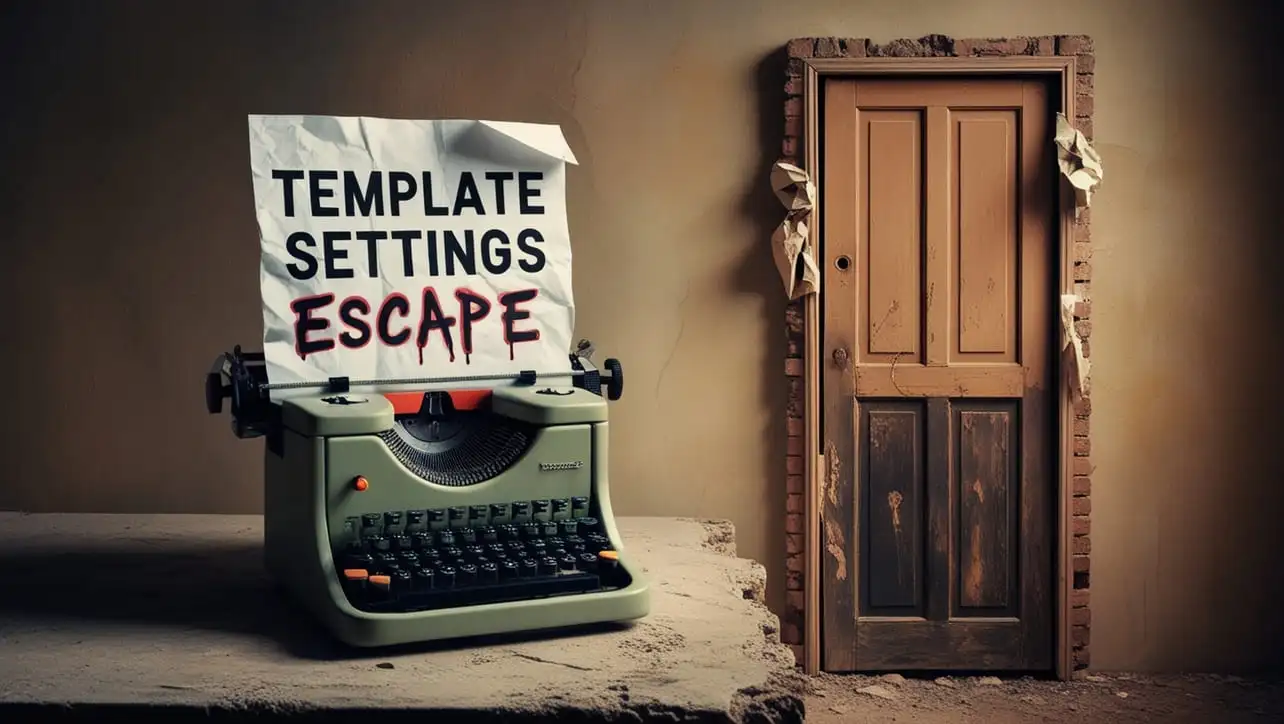
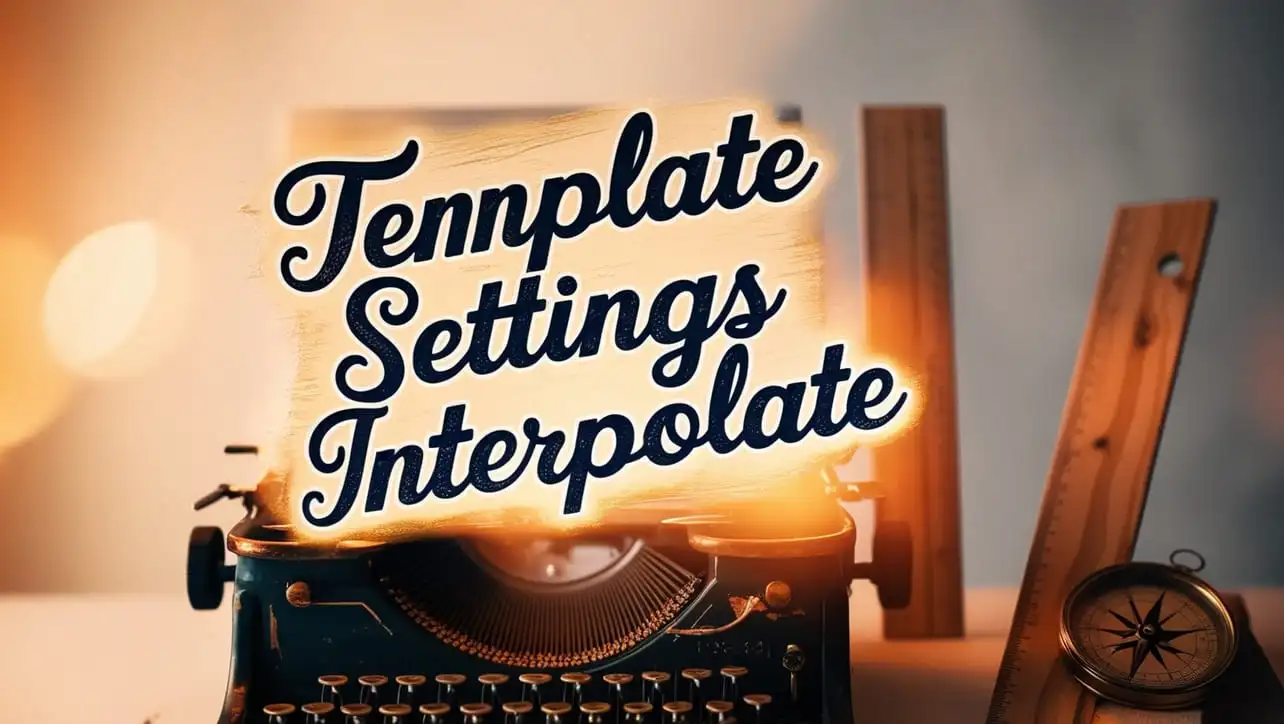
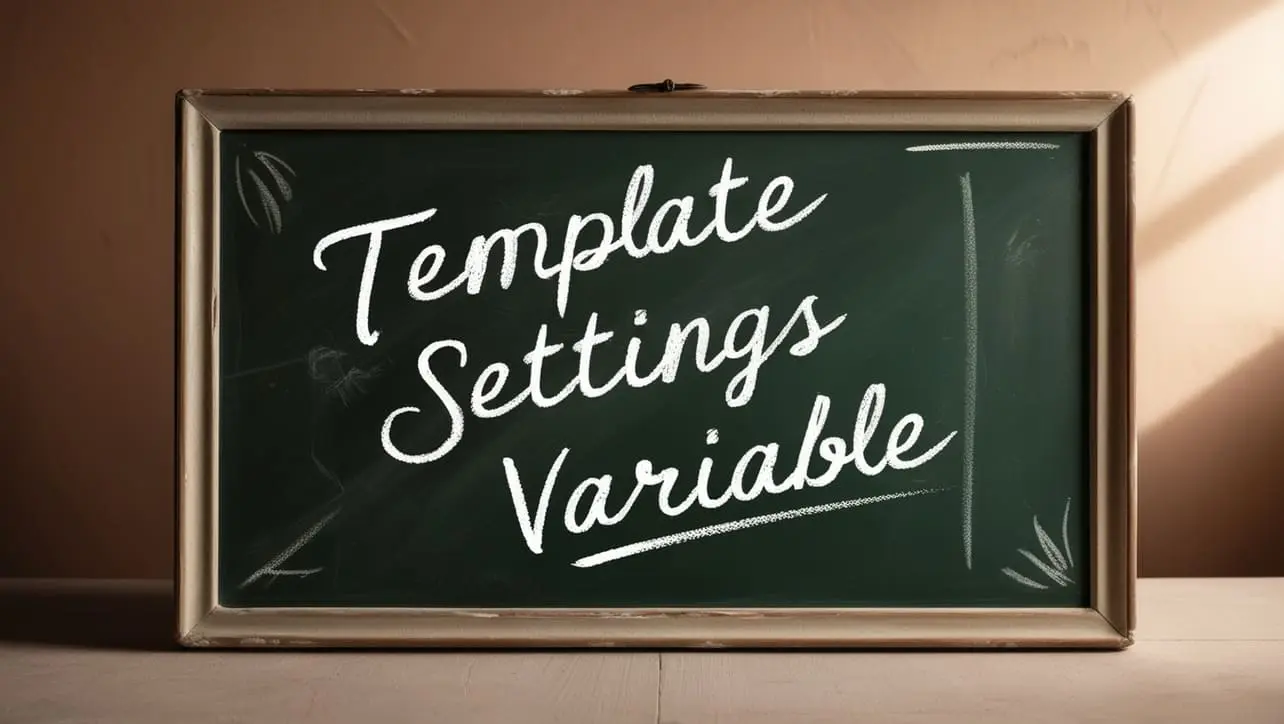
If you have any doubts regarding this article (Lodash _.partialRight() Function Method), please comment here. I will help you immediately.