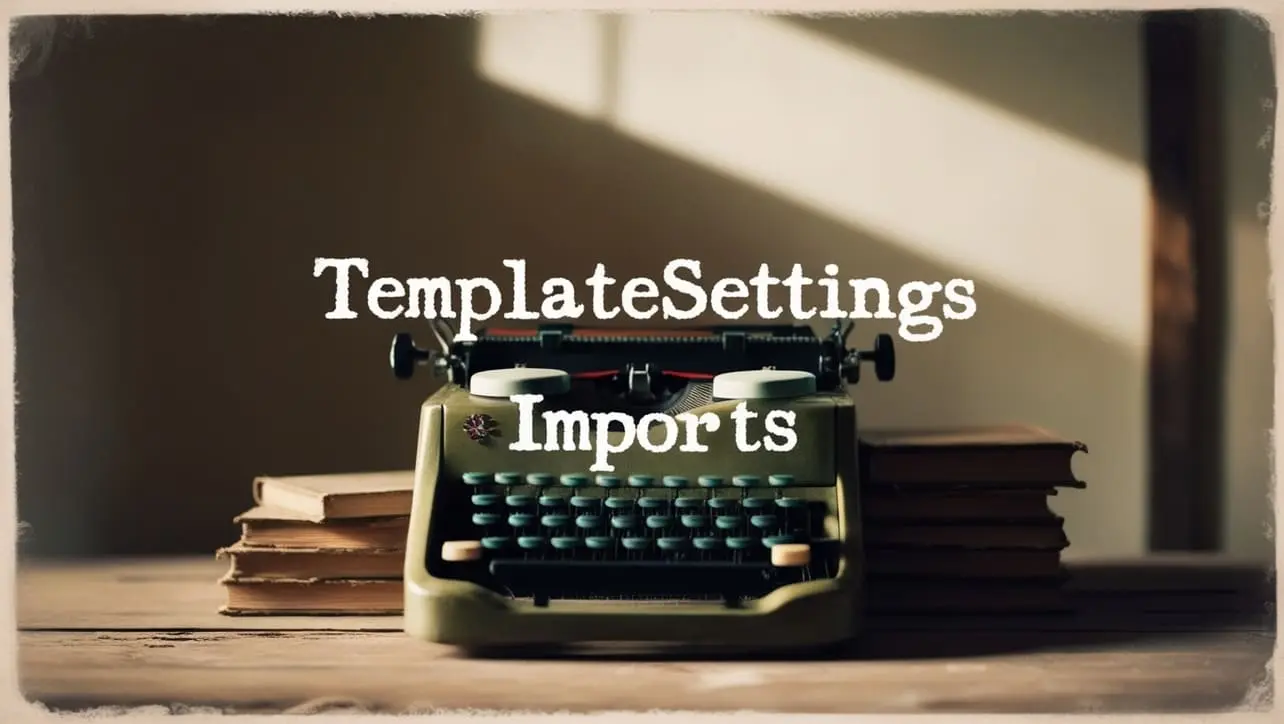
Lodash _.partial() Function Method
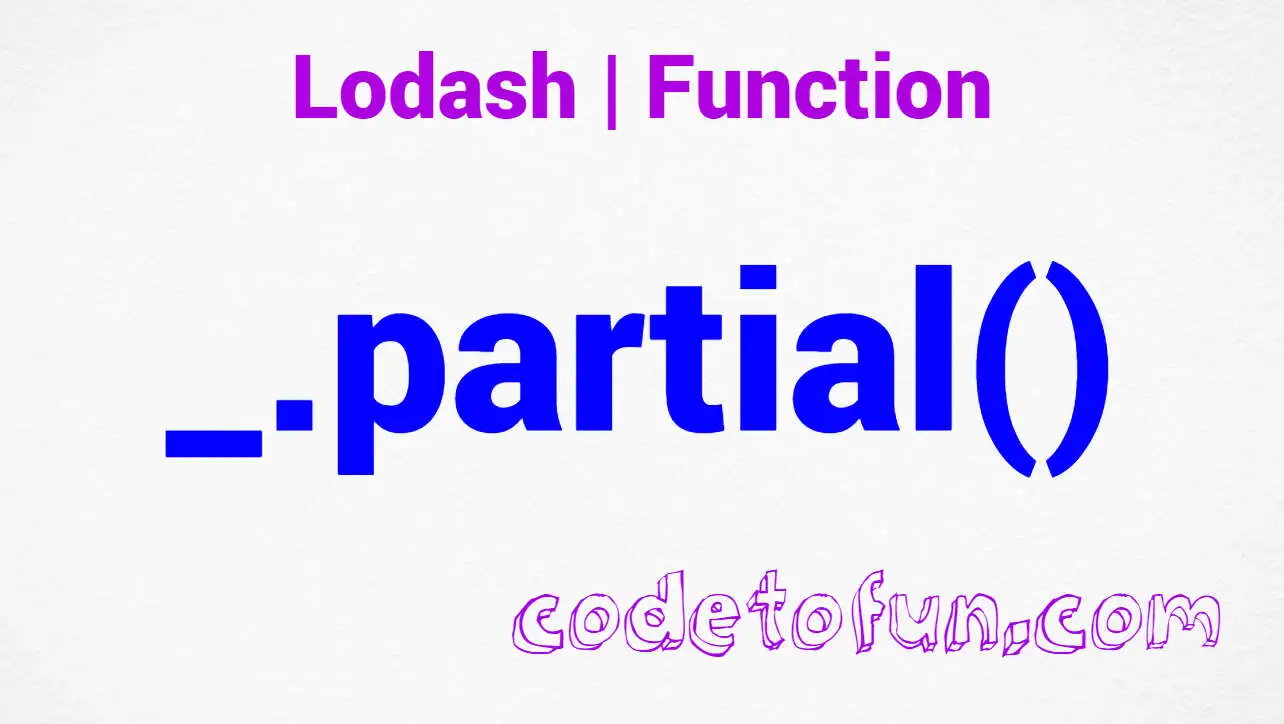
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript programming, flexibility and simplicity are key. Lodash, a feature-rich utility library, offers a multitude of functions to streamline development tasks. Among these functions is the _.partial()
method, a powerful tool for creating partially applied functions.
This method enhances code modularity and reusability, making it invaluable for developers seeking clean and efficient solutions.
🧠 Understanding _.partial() Method
The _.partial()
method in Lodash allows developers to create partially applied functions. A partially applied function is a new function with some of its arguments pre-filled, providing a convenient way to reuse and specialize functions for different use cases.
💡 Syntax
The syntax for the _.partial()
method is straightforward:
_.partial(func, [partials])
- func: The function to partially apply.
- partials (Optional): Arguments to be partially applied.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.partial()
method:
const _ = require('lodash');
// Original function
function greet(greeting, name) {
return `${greeting}, ${name}!`;
}
// Partially applied function
const greetHello = _.partial(greet, 'Hello');
console.log(greetHello('John'));
// Output: "Hello, John!"
In this example, we create a new function greetHello by partially applying the greet function with the argument Hello. Now, greetHello is a specialized function that always greets with "Hello".
🏆 Best Practices
When working with the _.partial()
method, consider the following best practices:
Maintain Function Modularity:
Use
_.partial()
to maintain modularity in your code. Break down complex functions into simpler, reusable parts by creating partially applied functions for common scenarios.example.jsCopiedconst _ = require('lodash'); // Original function function generateMessage(greeting, punctuation, name) { return `${greeting}, ${name}${punctuation}`; } // Partially applied functions const greetHello = _.partial(generateMessage, 'Hello', '!'); const greetGoodMorning = _.partial(generateMessage, 'Good morning', '.'); console.log(greetHello('John')); // Output: "Hello, John!" console.log(greetGoodMorning('Alice')); // Output: "Good morning, Alice."
Reuse Functions with Fixed Arguments:
Leverage
_.partial()
to reuse functions with fixed arguments. This reduces redundant code and promotes a cleaner and more maintainable codebase.example.jsCopiedconst _ = require('lodash'); // Original function function power(base, exponent) { return Math.pow(base, exponent); } // Partially applied function with fixed exponent const square = _.partial(power, _, 2); console.log(square(5)); // Output: 25 console.log(square(3)); // Output: 9
Enhance Readability:
Use
_.partial()
to enhance the readability of your code by explicitly stating the partially applied arguments. This makes the code more self-explanatory and easier to understand.example.jsCopiedconst _ = require('lodash'); // Original function function createPerson(firstName, lastName, age) { // ... create person object } // Partially applied function for creating users const createUser = _.partial(createPerson, _, _, 'user'); console.log(createUser('John', 'Doe')); // Output: { firstName: 'John', lastName: 'Doe', age: 'user' }
📚 Use Cases
Function Specialization:
_.partial()
is excellent for function specialization. Create variations of functions tailored to specific scenarios by partially applying relevant arguments.example.jsCopiedconst _ = require('lodash'); // Original function function calculateTotal(price, taxRate) { return price + (price * taxRate); } // Partially applied functions for different tax rates const calculateTotalWithVAT = _.partial(calculateTotal, _, 0.2); const calculateTotalWithSalesTax = _.partial(calculateTotal, _, 0.1); console.log(calculateTotalWithVAT(100)); // Output: 120 console.log(calculateTotalWithSalesTax(100)); // Output: 110
Code Optimization:
Use
_.partial()
for code optimization by creating specialized functions with commonly used arguments. This can lead to cleaner and more efficient code.example.jsCopiedconst _ = require('lodash'); // Original function function fetchData(endpoint, options) { // ... fetch data from the specified endpoint with provided options } // Partially applied function for fetching user data const fetchUserData = _.partial(fetchData, '/users', { headers: { Authorization: 'Bearer TOKEN' } }); // Later in the code... const userData = fetchUserData();
Event Handling:
When working with event handlers,
_.partial()
can be used to create specialized handler functions with predefined behavior.example.jsCopiedconst _ = require('lodash'); // Original event handler function handleButtonClick(event, buttonText) { // ... handle button click with specified text } // Partially applied functions for different button texts const handleSaveButtonClick = _.partial(handleButtonClick, _, 'Save'); const handleCancelButtonClick = _.partial(handleButtonClick, _, 'Cancel'); // Attach handlers to buttons saveButton.addEventListener('click', handleSaveButtonClick); cancelButton.addEventListener('click', handleCancelButtonClick);
🎉 Conclusion
The _.partial()
method in Lodash empowers developers with the ability to create partially applied functions, promoting code modularity, reusability, and readability. Whether you're specializing functions, optimizing code, or enhancing event handling, _.partial()
is a versatile tool in your JavaScript development toolkit.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.partial()
method in your Lodash projects.
👨💻 Join our Community:
Author
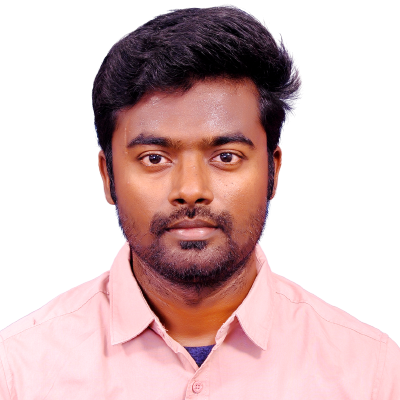
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
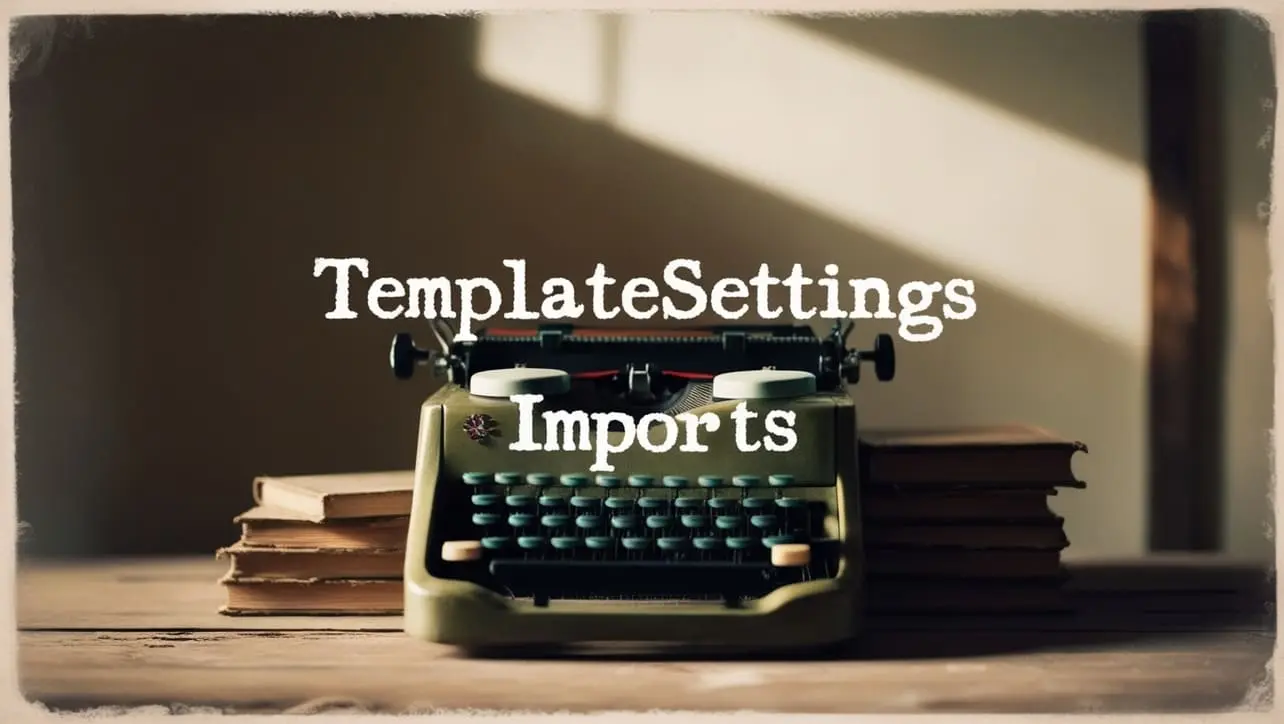
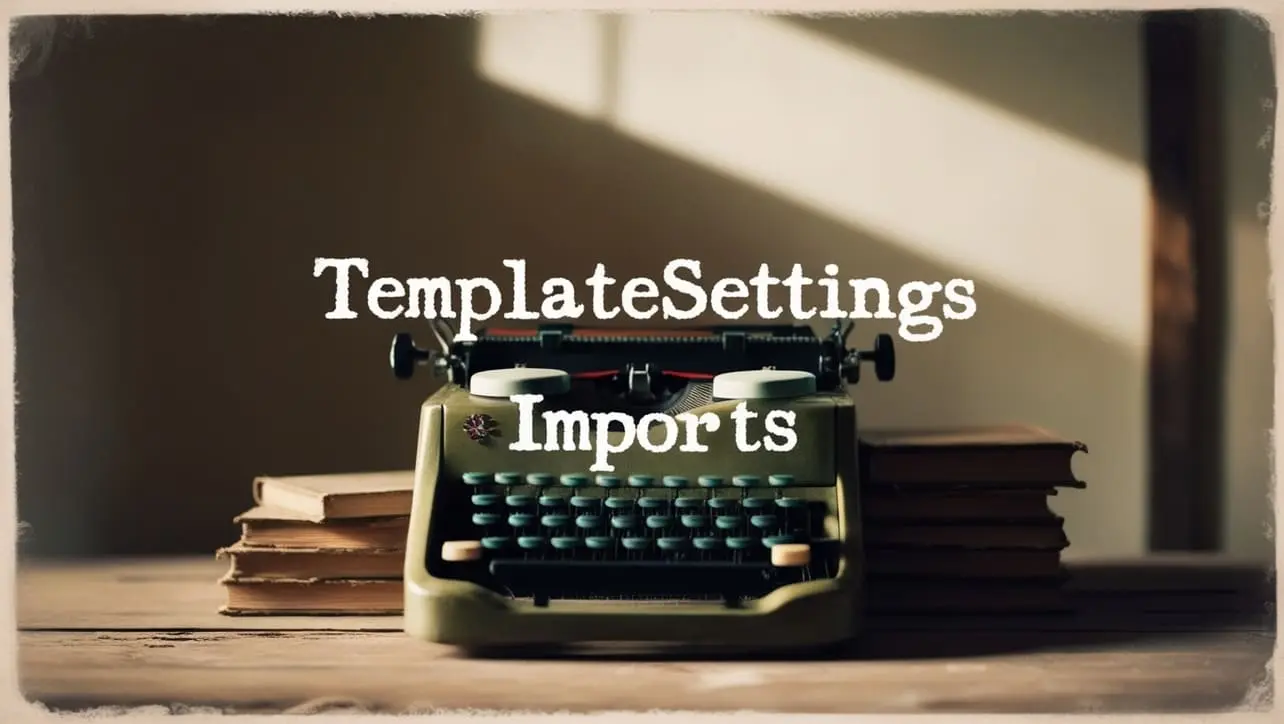
Lodash _.templateSettings.imports Property
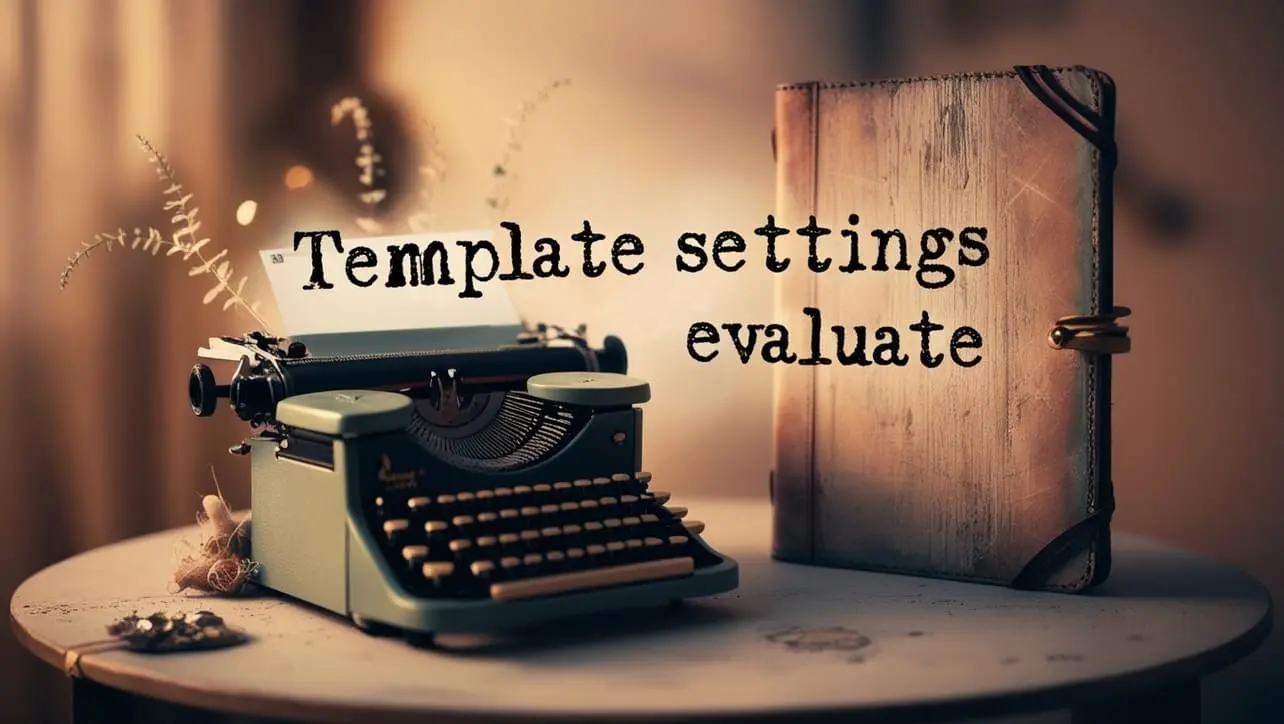
Lodash _.templateSettings.evaluate Property
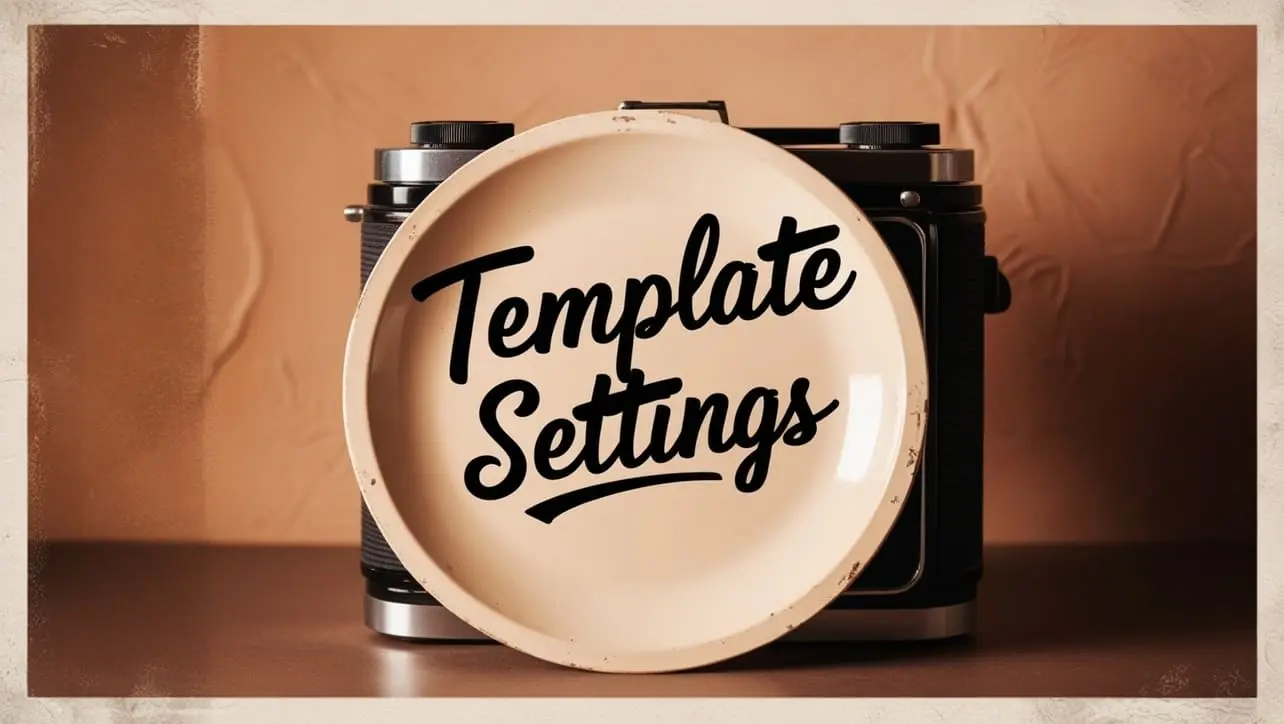
Lodash _.templateSettings Property
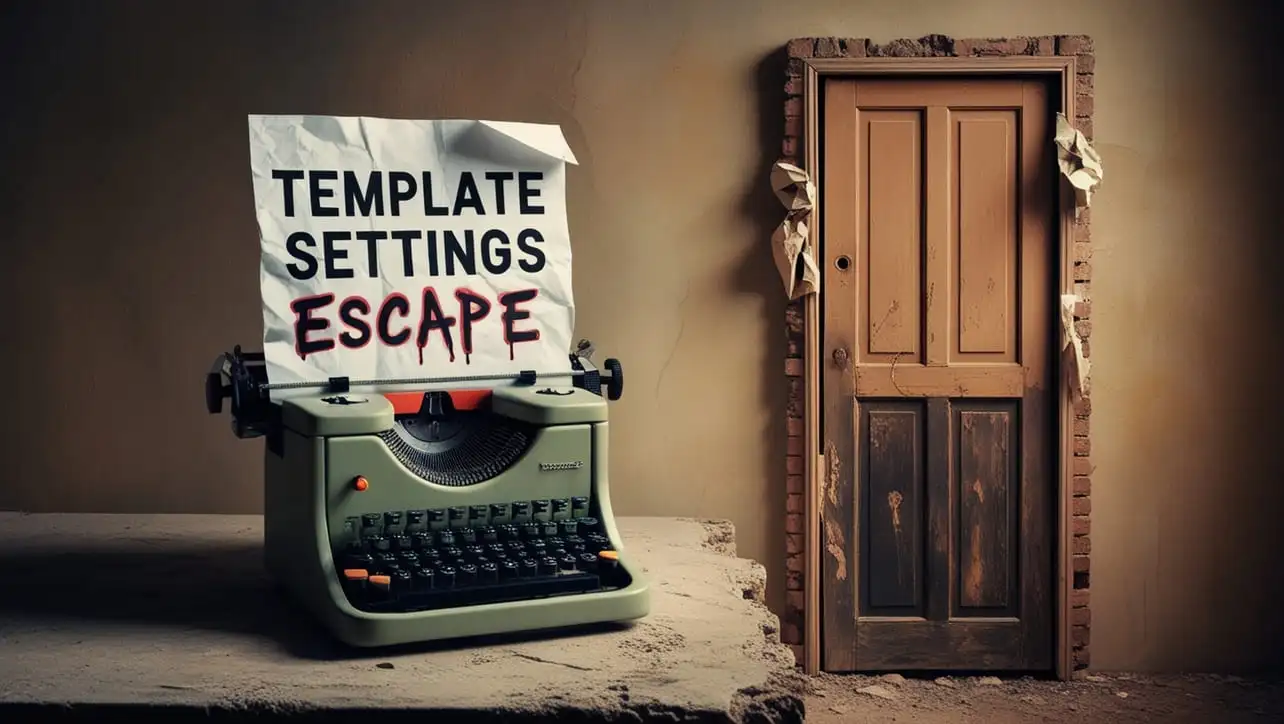
Lodash _.templateSettings.escape Property
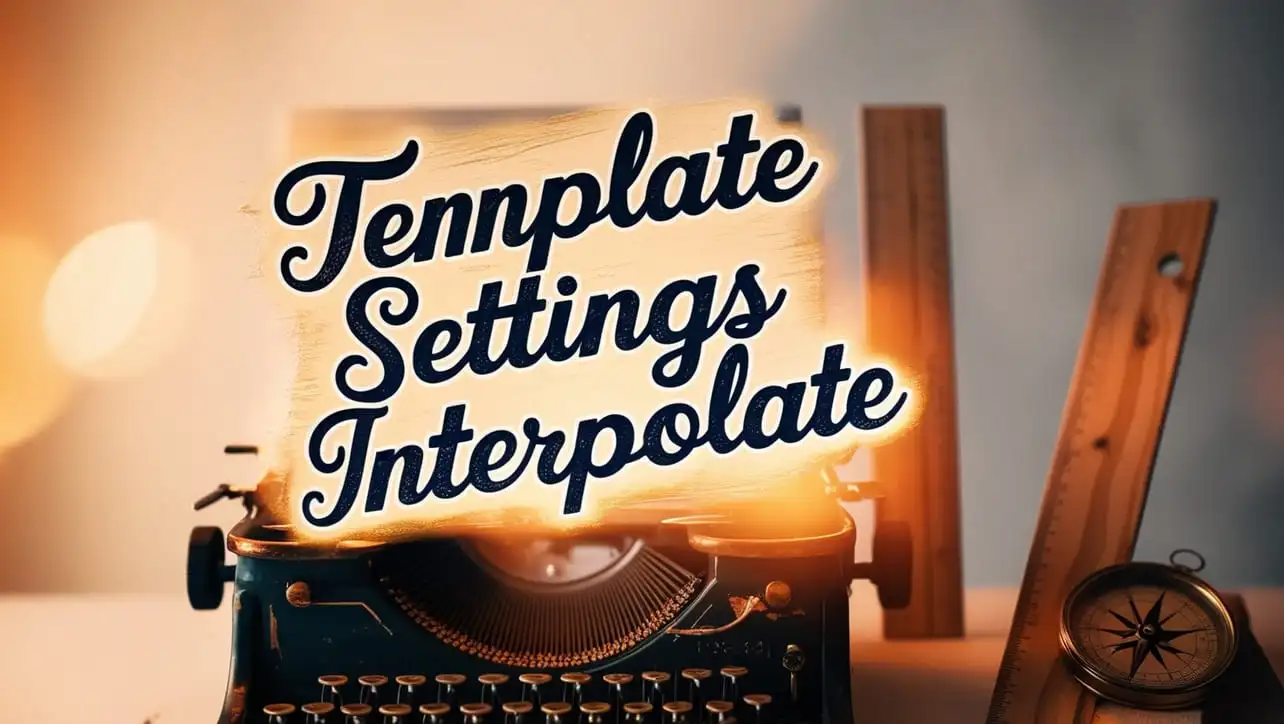
Lodash _.templateSettings.interpolate Property
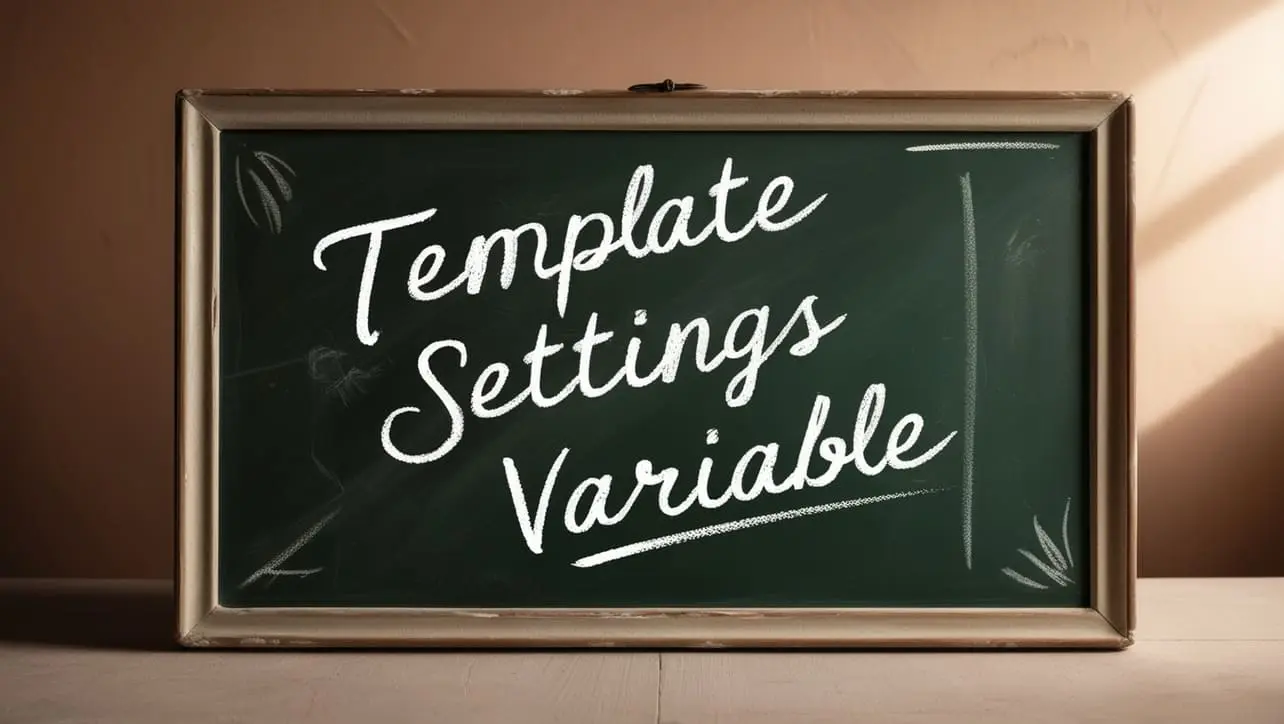
If you have any doubts regarding this article (Lodash _.partial() Function Method), please comment here. I will help you immediately.