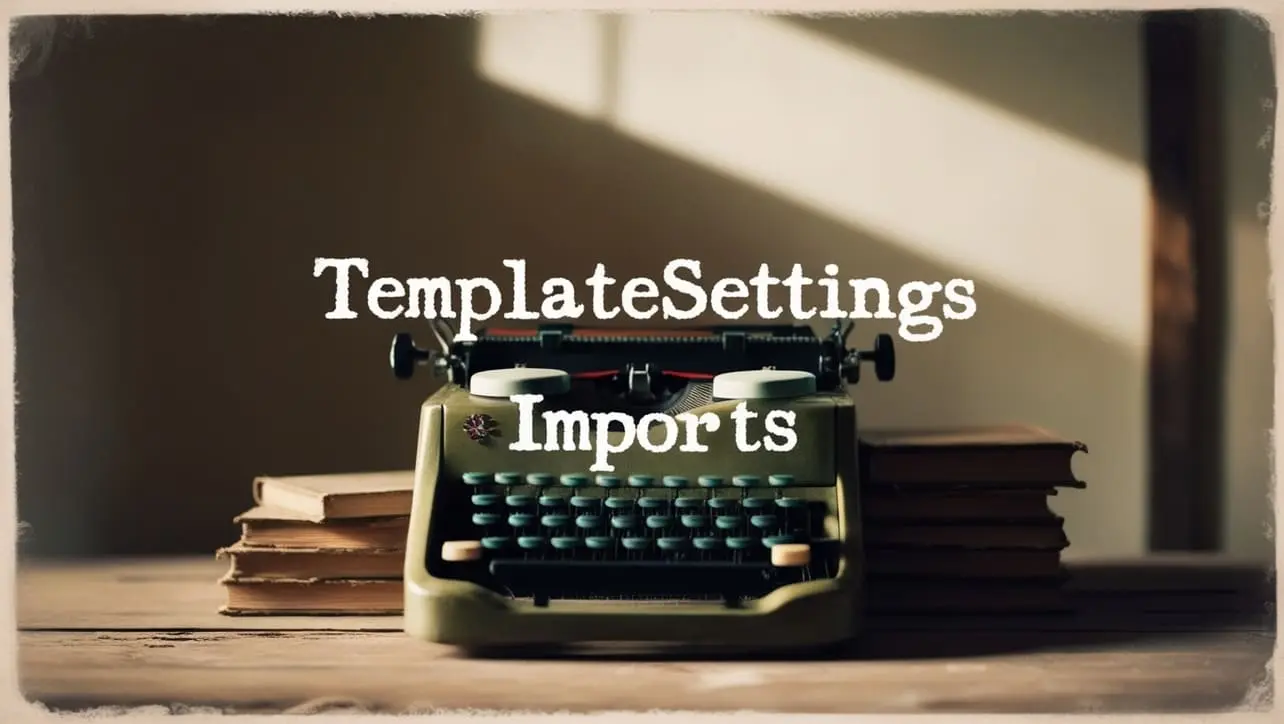
Lodash _.once() Function Method
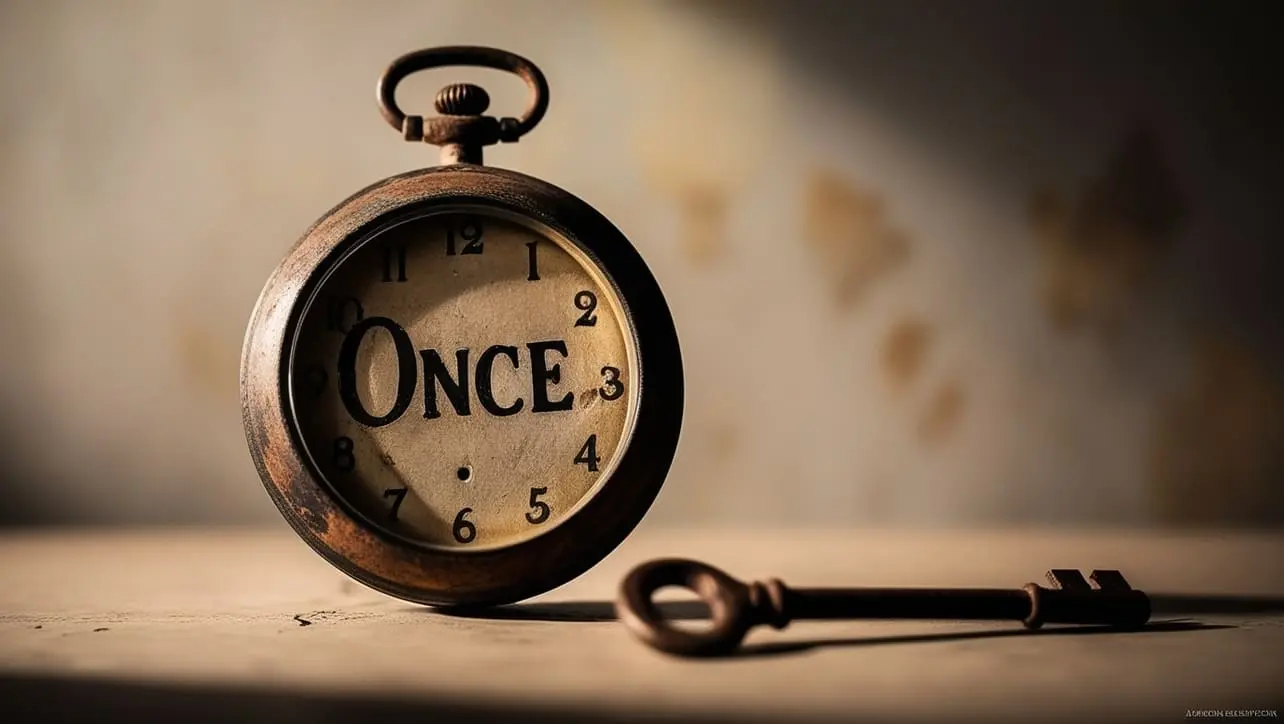
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript development, managing the execution of functions is a common challenge. Lodash, a comprehensive utility library, offers a solution in the form of the _.once()
method.
This method ensures that a given function is invoked only once, regardless of how many times it is called. _.once()
is a powerful tool for scenarios where you want to guarantee a function's single execution, such as initializing resources, setting configurations, or preventing redundant operations.
🧠 Understanding _.once() Method
The _.once()
method in Lodash creates a version of a function that can only be called once. Subsequent calls to the function will return the result of the initial invocation. This behavior is particularly useful when you want to ensure that a function with potentially side effects is executed exactly once.
💡 Syntax
The syntax for the _.once()
method is straightforward:
_.once(func)
- func: The function to be invoked once.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.once()
method:
const _ = require('lodash');
const initializeApp = _.once(() => {
console.log('Application Initialized');
// Additional initialization logic goes here
});
initializeApp(); // Output: Application Initialized
initializeApp(); // (No Output)
In this example, initializeApp is a function created using _.once()
. Despite being called multiple times, it only produces output on the first invocation.
🏆 Best Practices
When working with the _.once()
method, consider the following best practices:
Initialization Tasks:
Use
_.once()
for functions that perform initialization tasks, ensuring these tasks are executed only once, even if the function is called from various parts of your code.example.jsCopiedconst initializeConfig = _.once(() => { console.log('Config Initialized'); // Configuration logic goes here }); initializeConfig(); // Output: Config Initialized initializeConfig(); // (No Output)
Resource Allocation:
When dealing with resource allocation or setup,
_.once()
can be employed to guarantee that resources are allocated only once, preventing unnecessary overhead.example.jsCopiedconst allocateResources = _.once(() => { console.log('Resources Allocated'); // Resource allocation logic goes here }); allocateResources(); // Output: Resources Allocated allocateResources(); // (No Output)
Preventing Redundant Operations:
In scenarios where executing a function multiple times may lead to redundant or expensive operations,
_.once()
offers a clean solution.example.jsCopiedconst performExpensiveOperation = _.once(() => { console.log('Expensive Operation Performed'); // Expensive operation logic goes here }); performExpensiveOperation(); // Output: Expensive Operation Performed performExpensiveOperation(); // (No Output)
📚 Use Cases
Initialization in Single Page Applications (SPAs):
In SPAs, use
_.once()
to initialize core components or services, ensuring that initialization code runs only once during the application's lifetime.example.jsCopiedconst initializeSPA = _.once(() => { console.log('SPA Initialized'); // SPA initialization logic goes here }); initializeSPA(); // Output: SPA Initialized initializeSPA(); // (No Output)
Event Handling Setup:
When setting up event handlers, especially in scenarios where multiple components may attempt to set up the same handler,
_.once()
can ensure that the event handling logic is attached only once.example.jsCopiedconst setupEventHandler = _.once(() => { console.log('Event Handler Set Up'); // Event handling logic goes here }); setupEventHandler(); // Output: Event Handler Set Up setupEventHandler(); // (No Output)
Lazy Initialization:
For functions that involve lazy initialization,
_.once()
is a convenient way to ensure that the initialization logic is executed only when needed.example.jsCopiedconst lazyInitialization = _.once(() => { console.log('Lazy Initialization Performed'); // Lazy initialization logic goes here }); // Some code that may or may not trigger lazyInitialization()
🎉 Conclusion
The _.once()
method in Lodash provides a reliable mechanism for ensuring that a function is executed only once. Whether you're dealing with initialization tasks, resource allocation, or preventing redundant operations, _.once()
offers a clean and efficient solution.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.once()
method in your Lodash projects.
👨💻 Join our Community:
Author
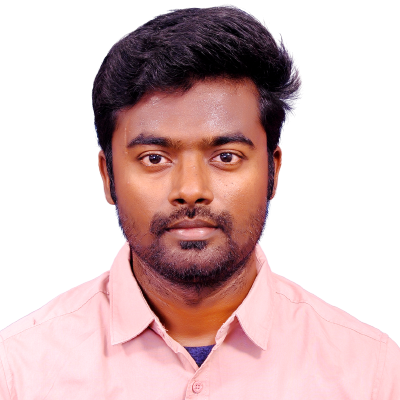
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
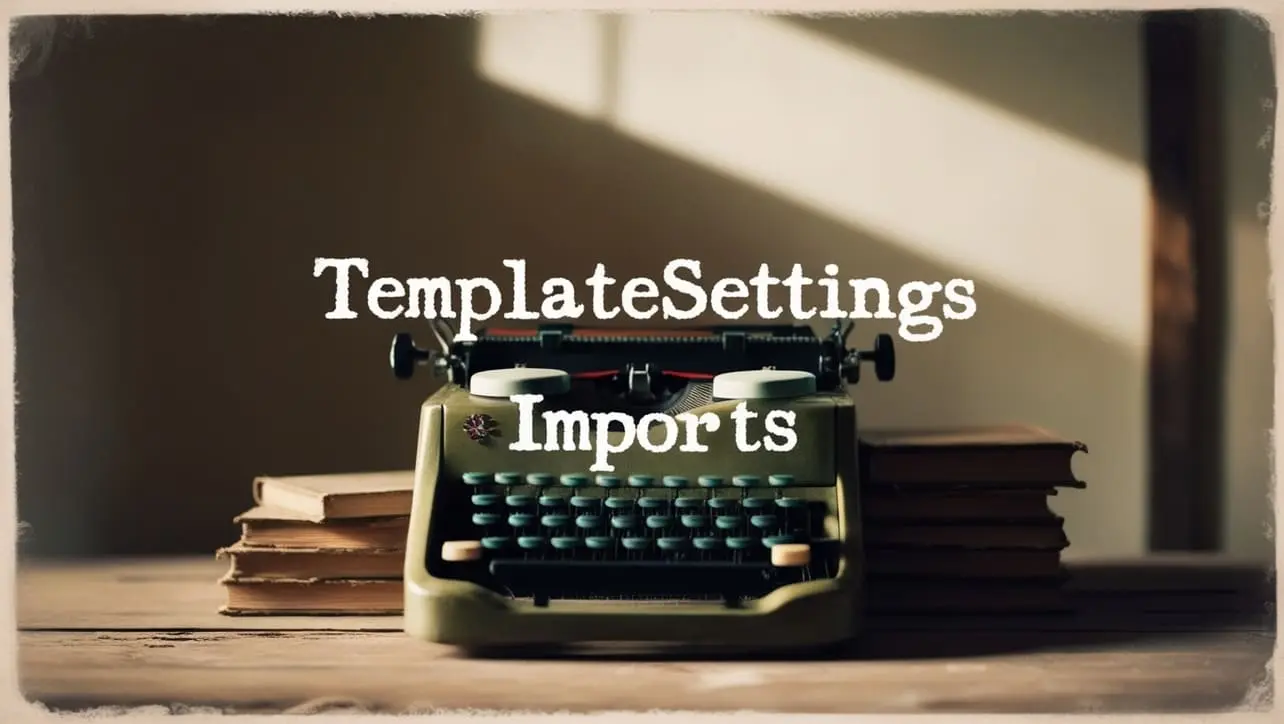
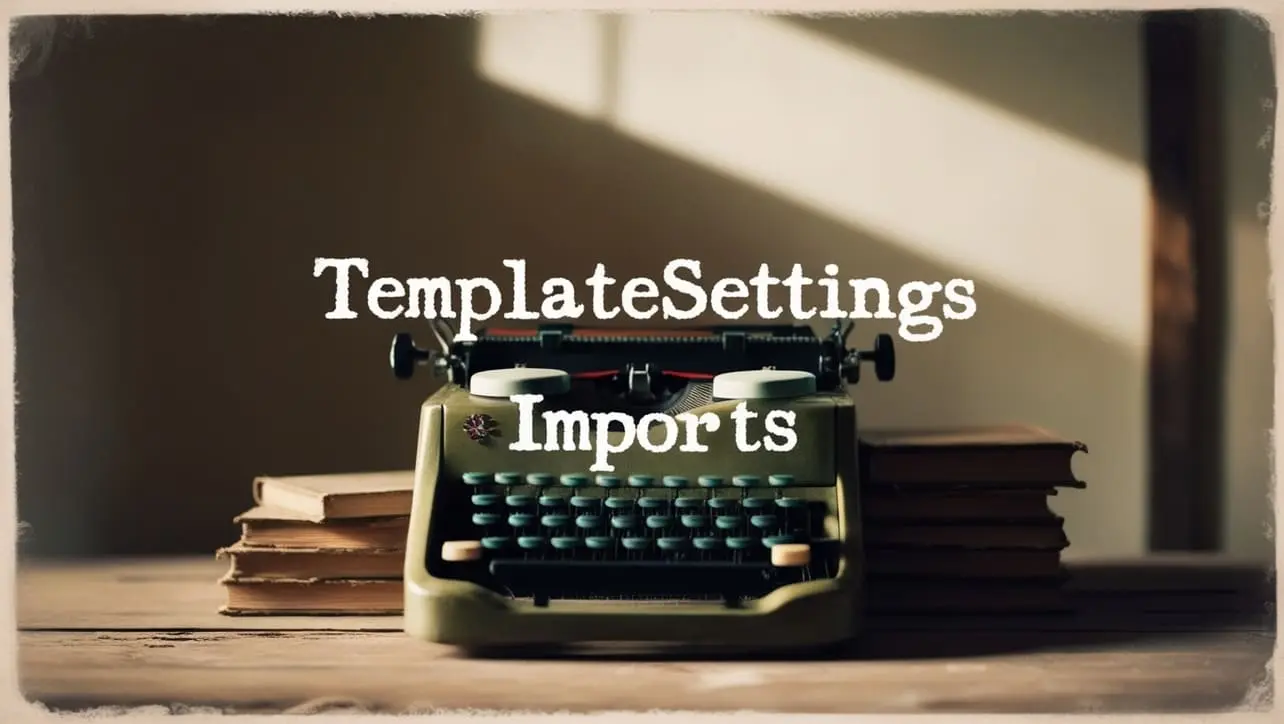
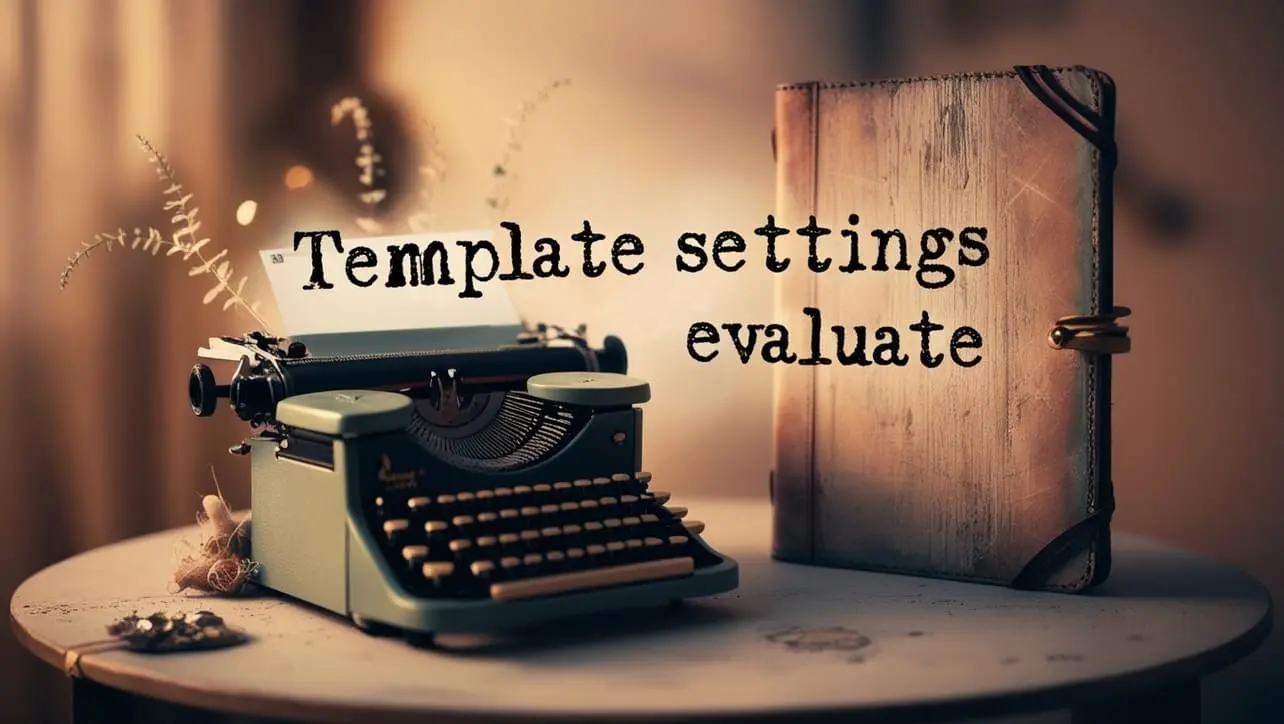
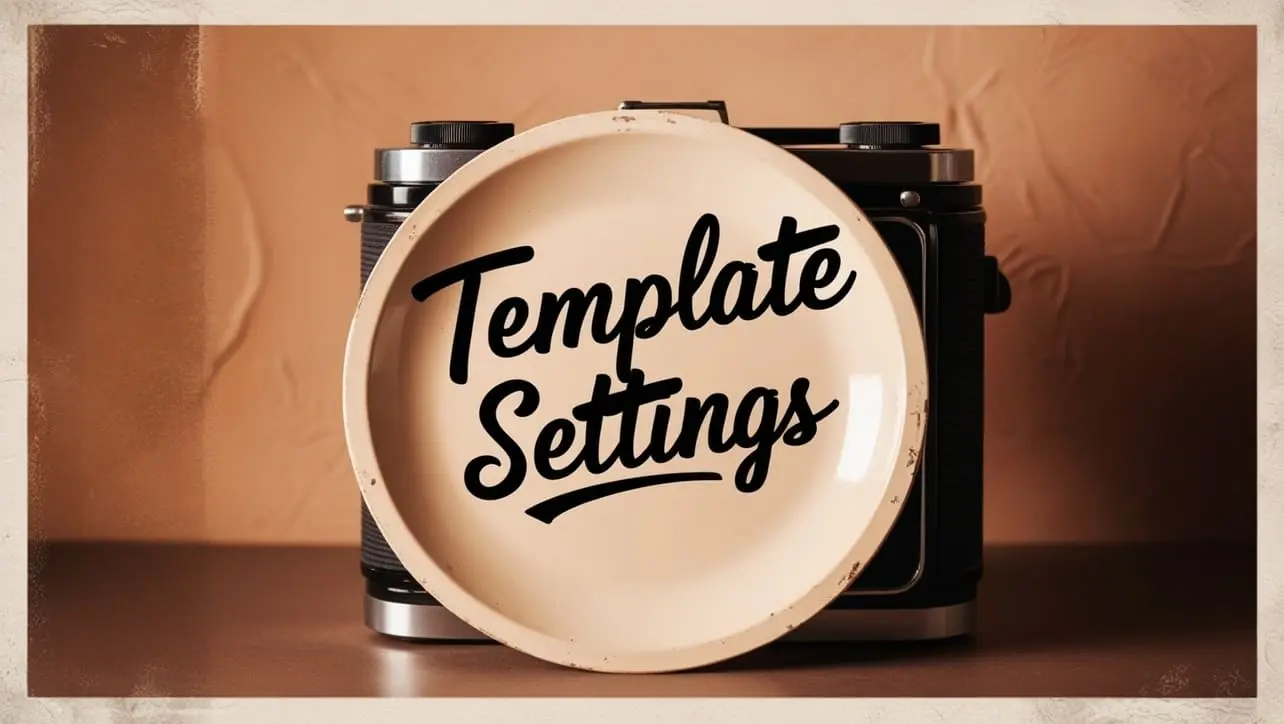
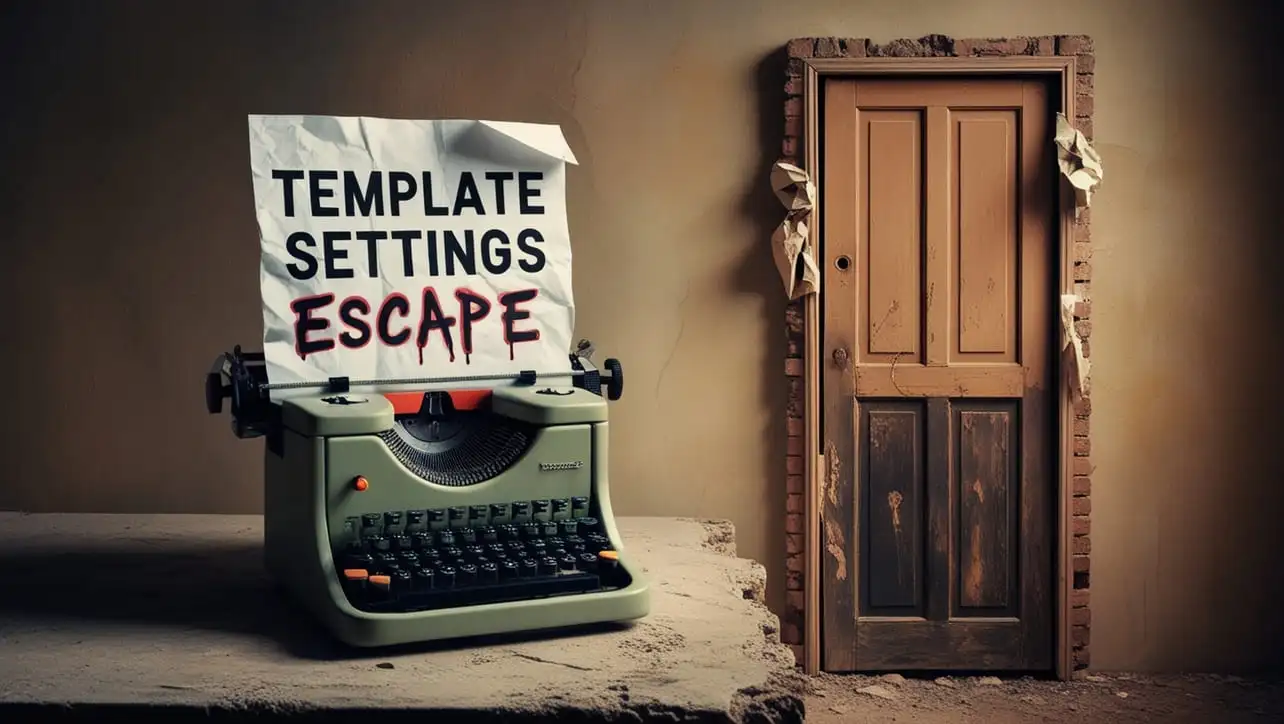
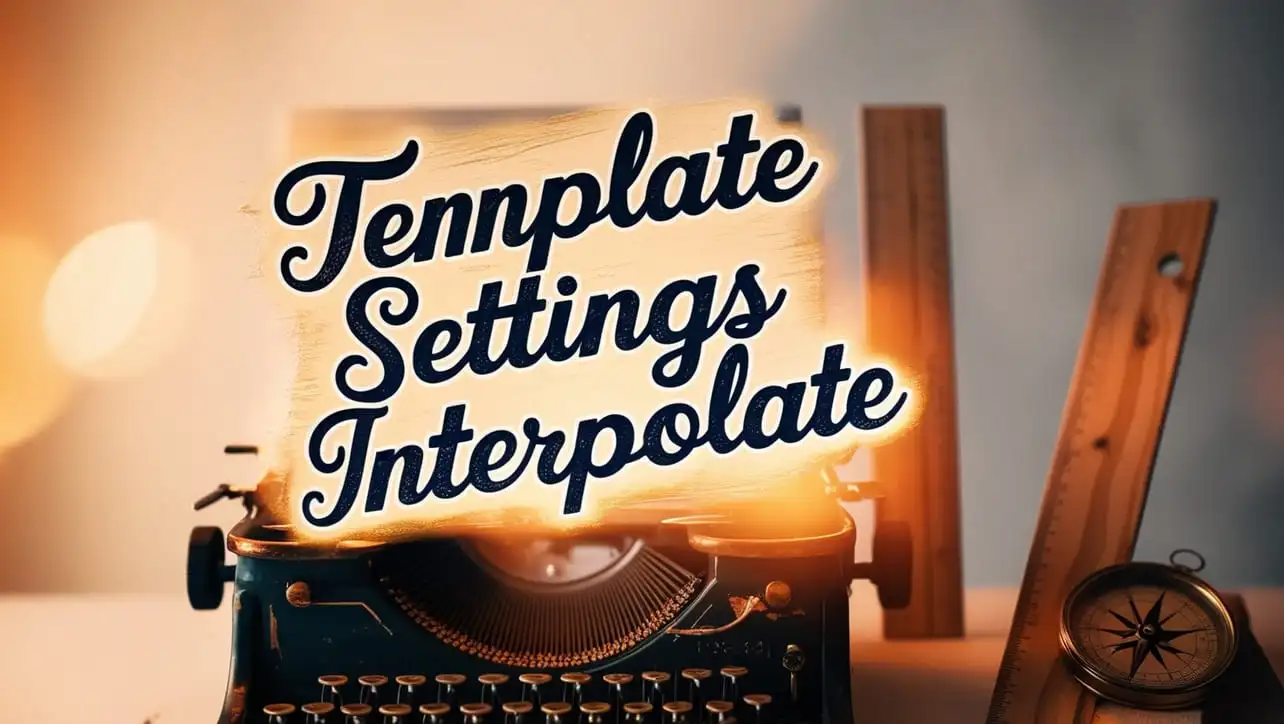
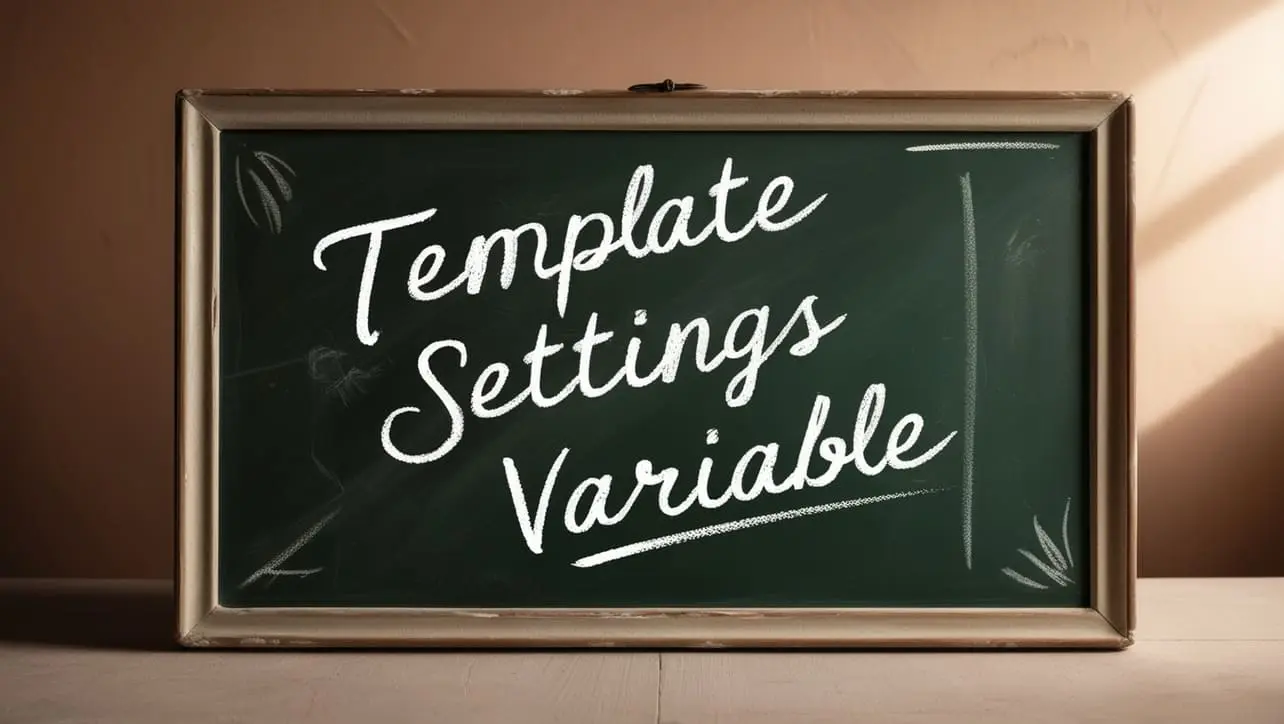
If you have any doubts regarding this article (Lodash _.once() Function Method), please comment here. I will help you immediately.