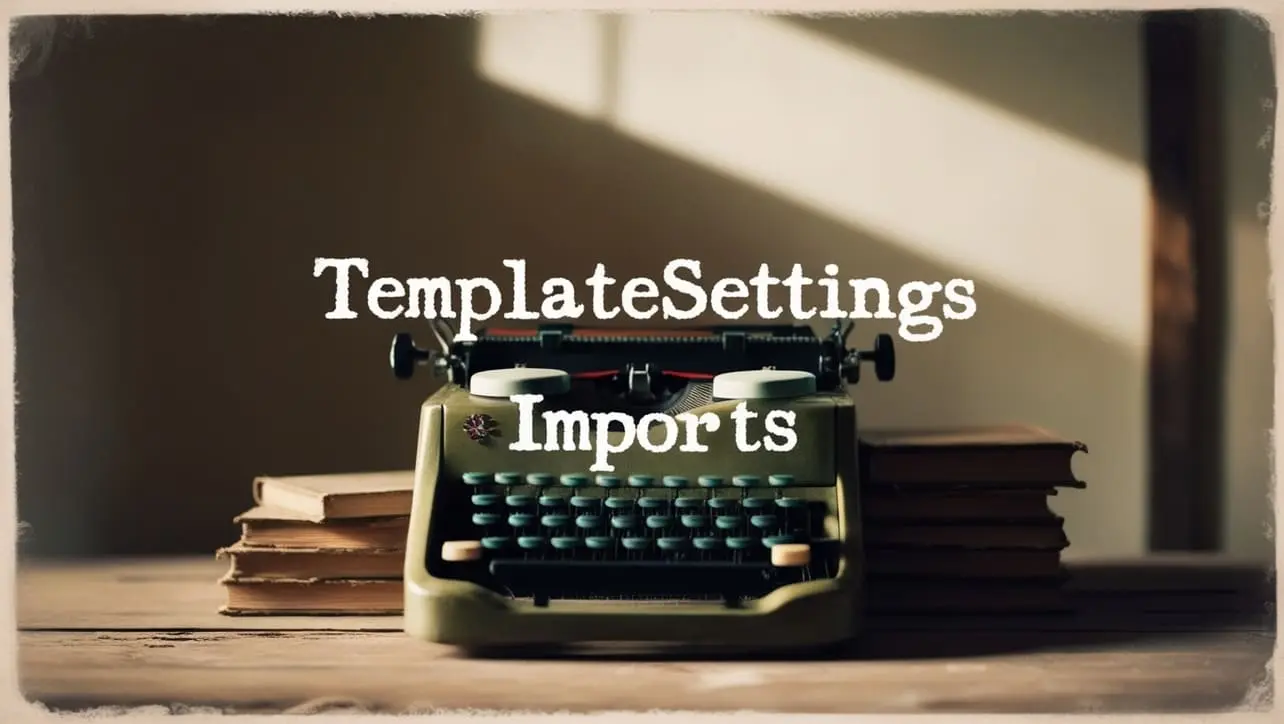
Lodash _.curryRight() Function Method
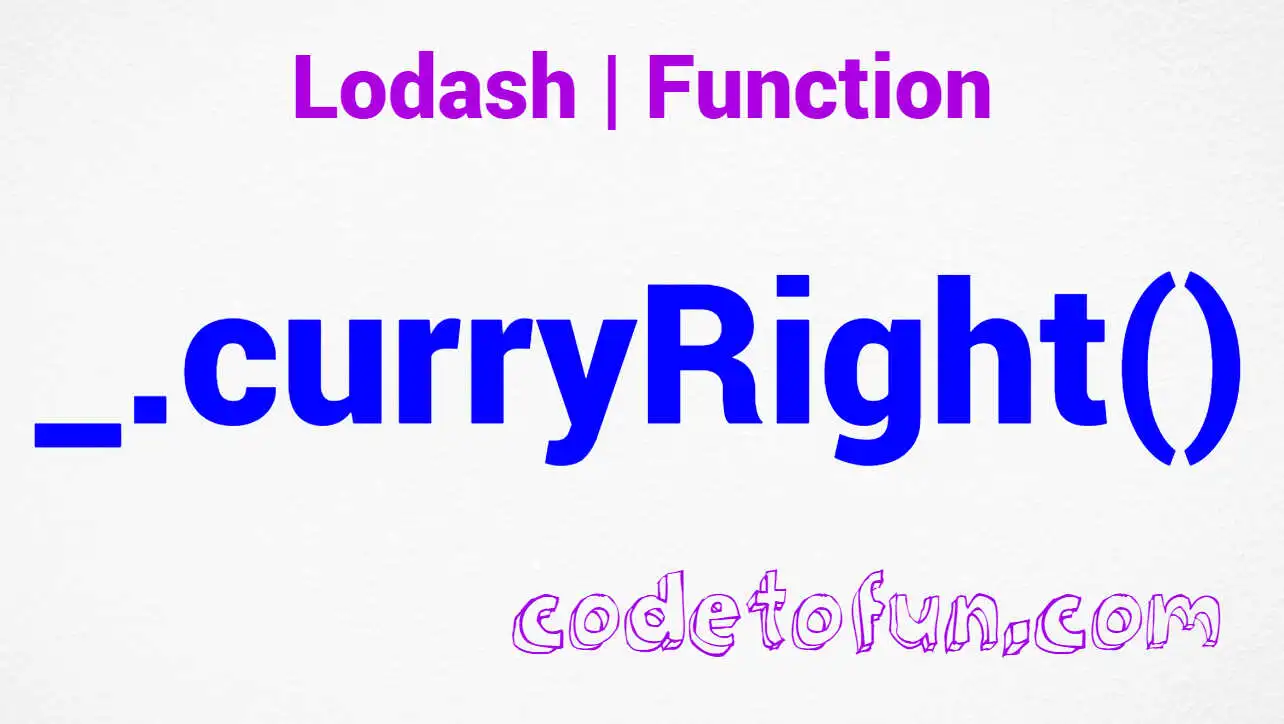
Photo Credit to CodeToFun
🙋 Introduction
In the realm of functional programming, currying is a powerful technique that transforms a function with multiple arguments into a series of functions with a single argument. Lodash, the versatile utility library, provides the _.curryRight()
method, allowing developers to create curried functions where arguments can be supplied in a reverse order.
This enhances code flexibility and promotes a functional programming paradigm.
🧠 Understanding _.curryRight() Method
The _.curryRight()
method in Lodash is designed to create a curried function, enabling partial application of arguments from right to left. This means that you can gradually apply arguments, and the resulting function can be invoked with the remaining arguments later. This method empowers developers to build more reusable and flexible functions.
💡 Syntax
The syntax for the _.curryRight()
method is straightforward:
_.curryRight(func, [arity=func.length])
- func: The function to curry.
- arity: The number of arguments the curried function should accept.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.curryRight()
method:
const _ = require('lodash');
// Original function
function greet(name, greeting, punctuation) {
return `${greeting}, ${name}${punctuation}`;
}
// Curried function using _.curryRight()
const curriedGreet = _.curryRight(greet);
// Applying arguments
const greetHello = curriedGreet('Hello');
const greetHi = greetHello('Hi');
console.log(greetHi('John', '!'));
// Output: "Hello, John! Hi"
In this example, the greet function is curried using _.curryRight()
, allowing for the gradual application of arguments.
🏆 Best Practices
When working with the _.curryRight()
method, consider the following best practices:
Understand Currying Basics:
Ensure a solid understanding of currying basics before applying
_.curryRight()
. Familiarize yourself with the concept of transforming a function with multiple arguments into a series of single-argument functions.example.jsCopied// Non-curried function function add(a, b, c) { return a + b + c; } // Curried function using _.curryRight() const curriedAdd = _.curryRight(add); console.log(curriedAdd(1)(2)(3)); // Output: 6
Consider Argument Order:
When using
_.curryRight()
, be mindful of the argument order. Arguments are applied from right to left, so consider the logical order of your function's arguments.example.jsCopiedconst divide = (a, b) => a / b; // Curried function using _.curryRight() const curriedDivide = _.curryRight(divide); const divideBy2 = curriedDivide(2); console.log(divideBy2(10)); // Output: 5
Partial Application Benefits:
Leverage the benefits of partial application enabled by
_.curryRight()
. This allows you to create specialized functions by supplying a subset of arguments, promoting code reusability.example.jsCopiedconst power = (base, exponent) => Math.pow(base, exponent); // Curried function using _.curryRight() const curriedPower = _.curryRight(power); const square = curriedPower(2); console.log(square(3)); // Output: 9
📚 Use Cases
Flexible Function Composition:
Use
_.curryRight()
to create functions that can be easily composed, allowing for a more flexible and modular code structure.example.jsCopiedconst add = (a, b) => a + b; const multiply = (a, b) => a * b; // Curried functions using _.curryRight() const curriedAdd = _.curryRight(add); const curriedMultiply = _.curryRight(multiply); const addThenMultiply = _.flowRight(curriedMultiply(3), curriedAdd(2)); console.log(addThenMultiply(5)); // Output: 21
Dynamic Function Generation:
Dynamically generate functions with varying behavior by using
_.curryRight()
. This allows you to create specialized functions based on specific use cases.example.jsCopiedconst createFormatter = (format, value) => `${value.toFixed(2)} ${format}`; // Curried function using _.curryRight() const curriedFormatter = _.curryRight(createFormatter); const formatToCurrency = curriedFormatter('USD'); console.log(formatToCurrency(25.5)); // Output: "25.50 USD"
Reusable Function Factories:
Build reusable function factories with
_.curryRight()
, enabling the creation of functions tailored to different scenarios.example.jsCopiedconst exponentiateBy = (exponent, base) => Math.pow(base, exponent); // Curried function using _.curryRight() const curriedExponentiate = _.curryRight(exponentiateBy); const square = curriedExponentiate(2); console.log(square(4)); // Output: 16
🎉 Conclusion
The _.curryRight()
method in Lodash provides developers with a powerful tool for creating curried functions with a reversed order of argument application. By understanding and applying this method judiciously, you can enhance code flexibility, promote functional programming practices, and build more modular and reusable functions.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.curryRight()
method in your Lodash projects.
👨💻 Join our Community:
Author
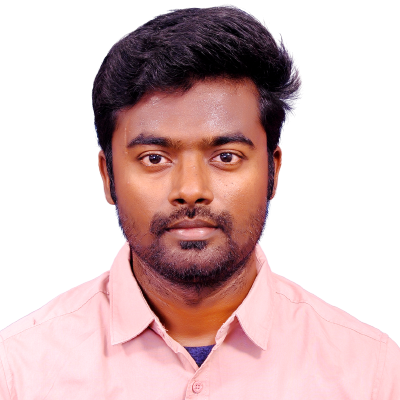
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
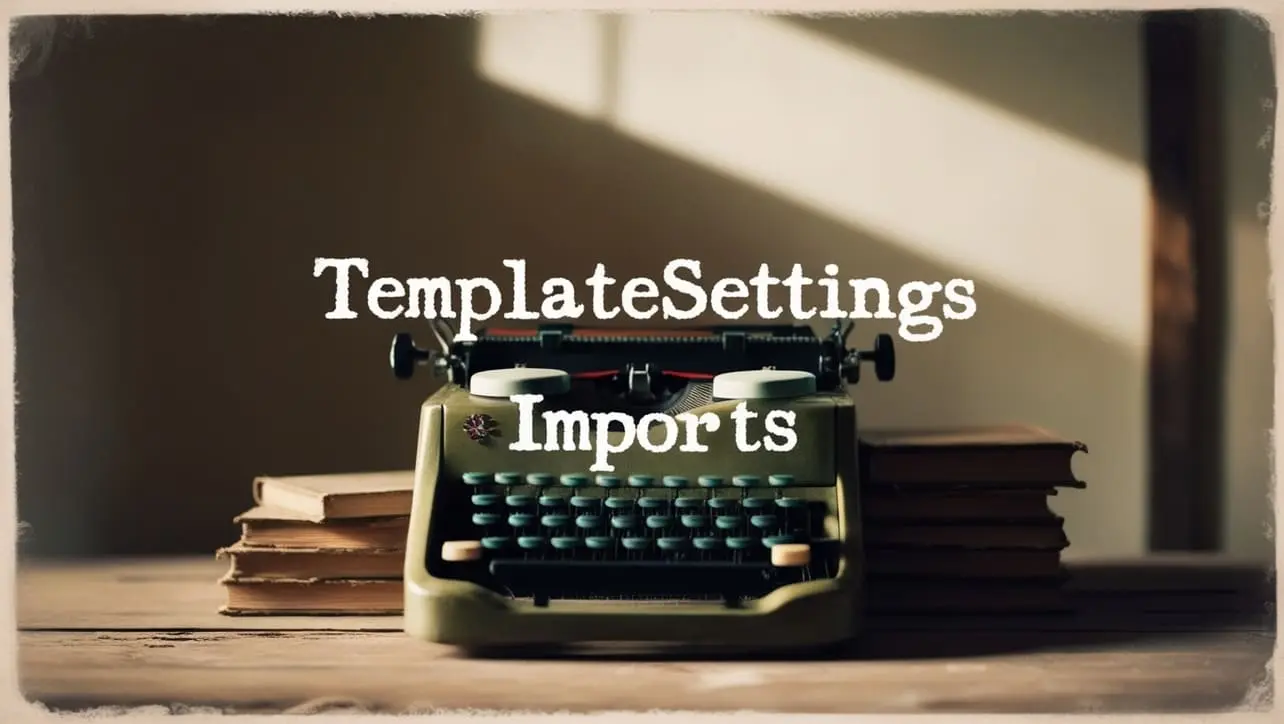
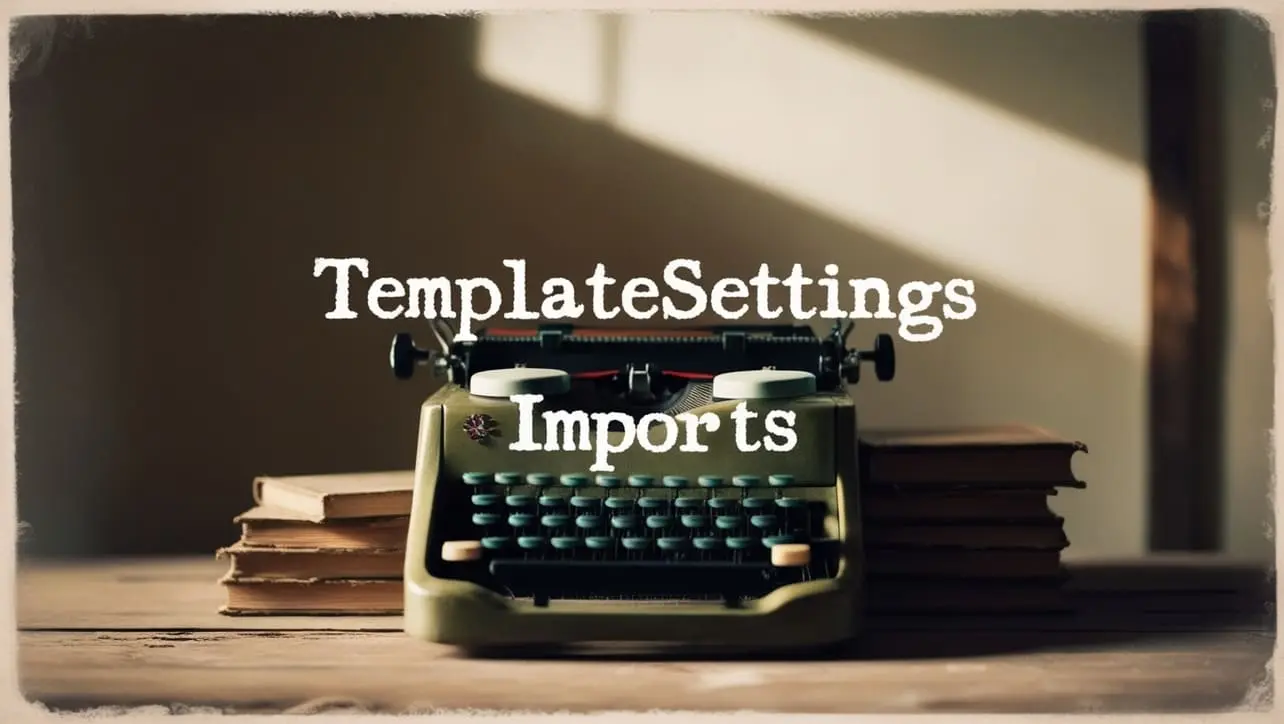
Lodash _.templateSettings.imports Property
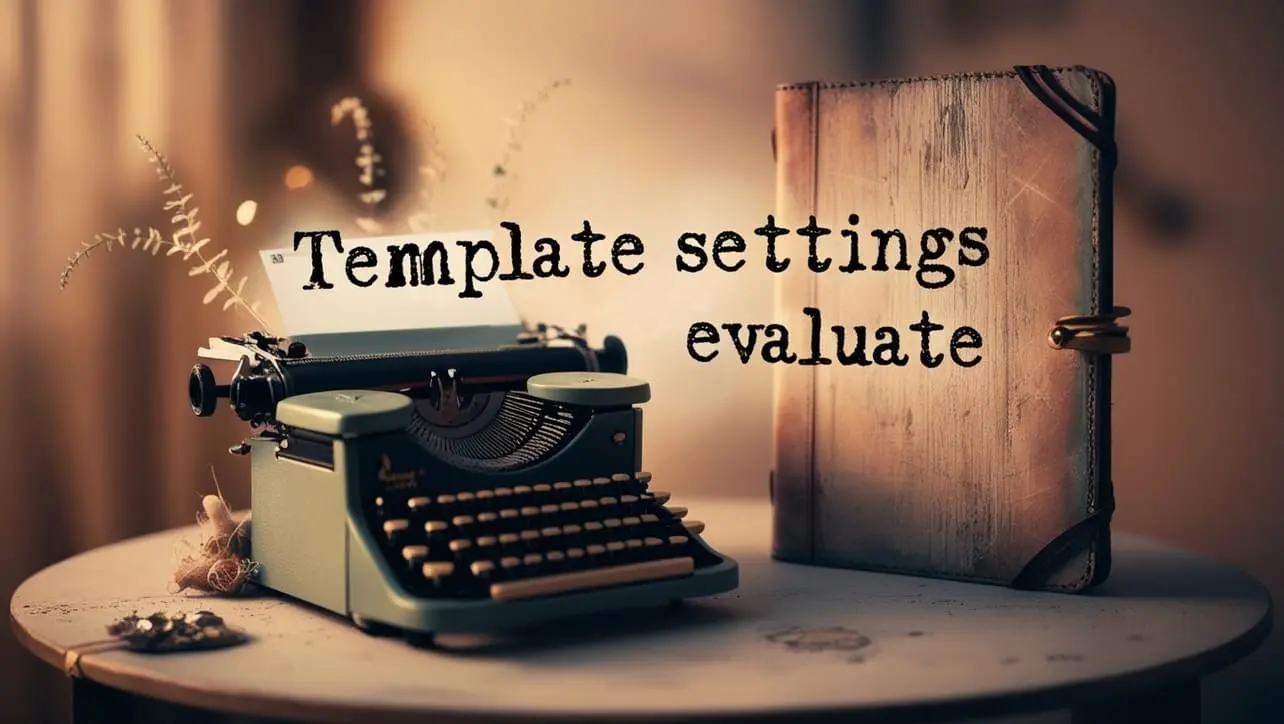
Lodash _.templateSettings.evaluate Property
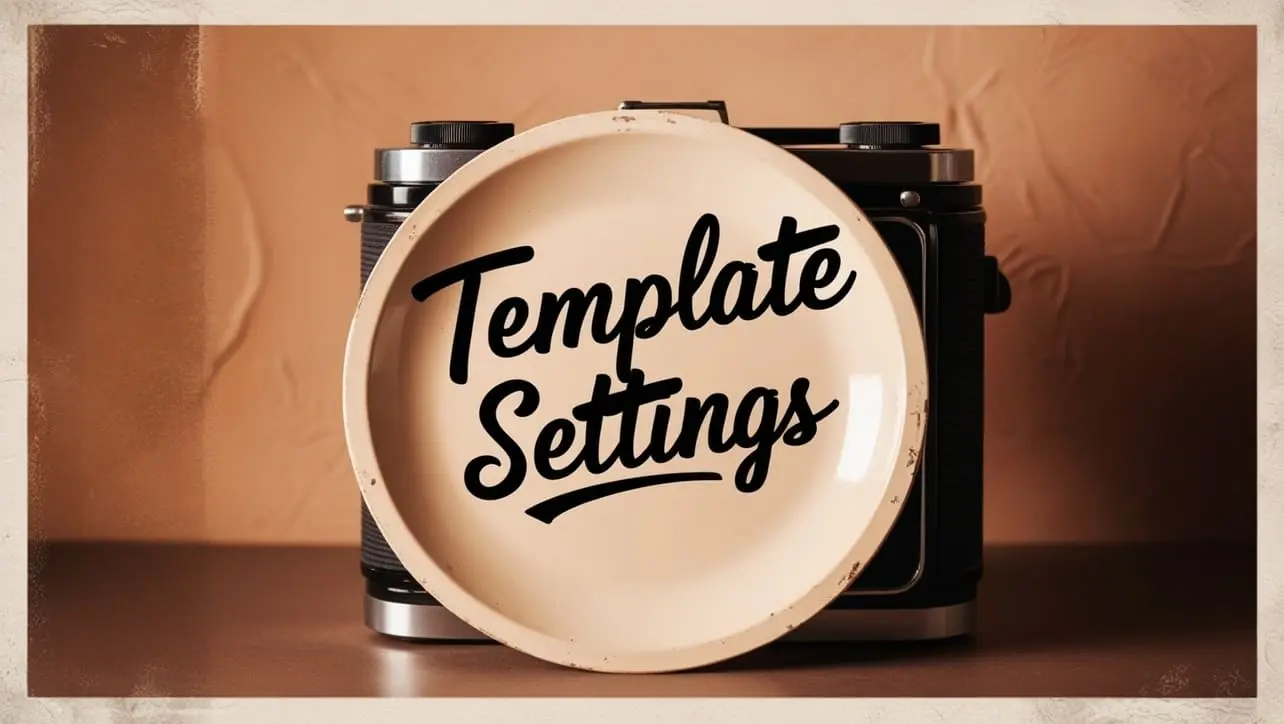
Lodash _.templateSettings Property
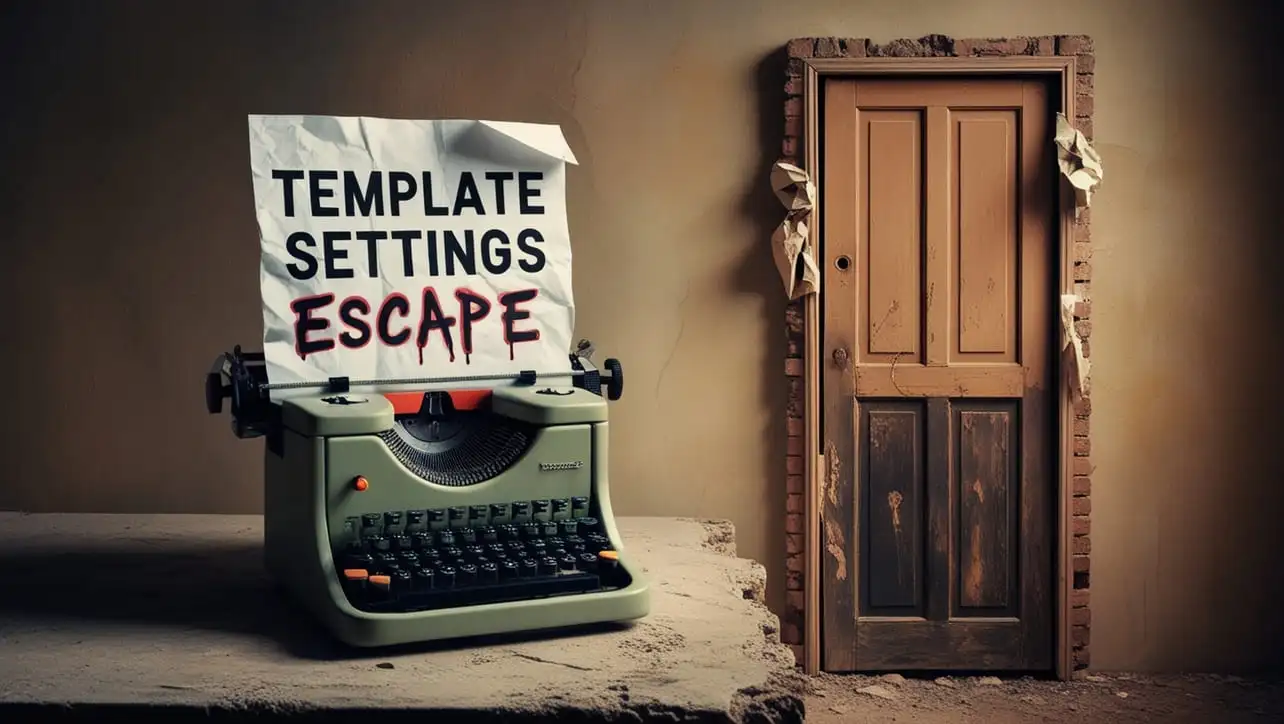
Lodash _.templateSettings.escape Property
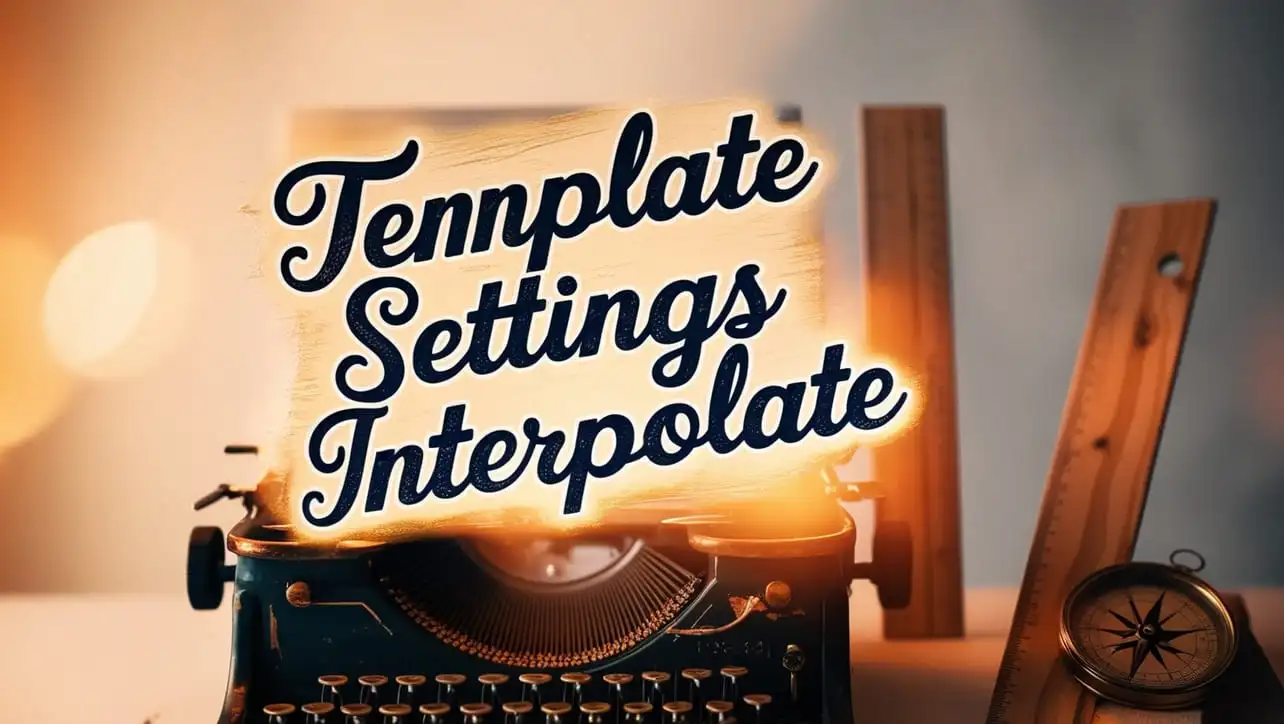
Lodash _.templateSettings.interpolate Property
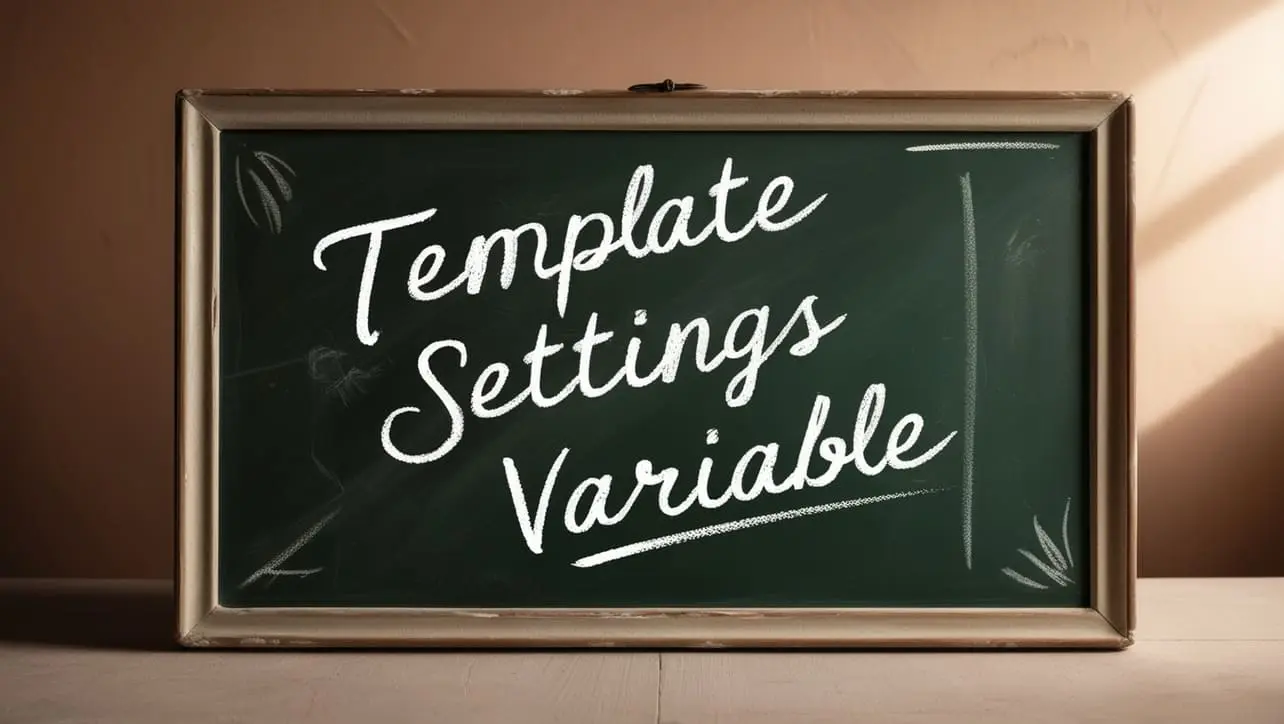
If you have any doubts regarding this article (Lodash _.curryRight() Function Method), please comment here. I will help you immediately.