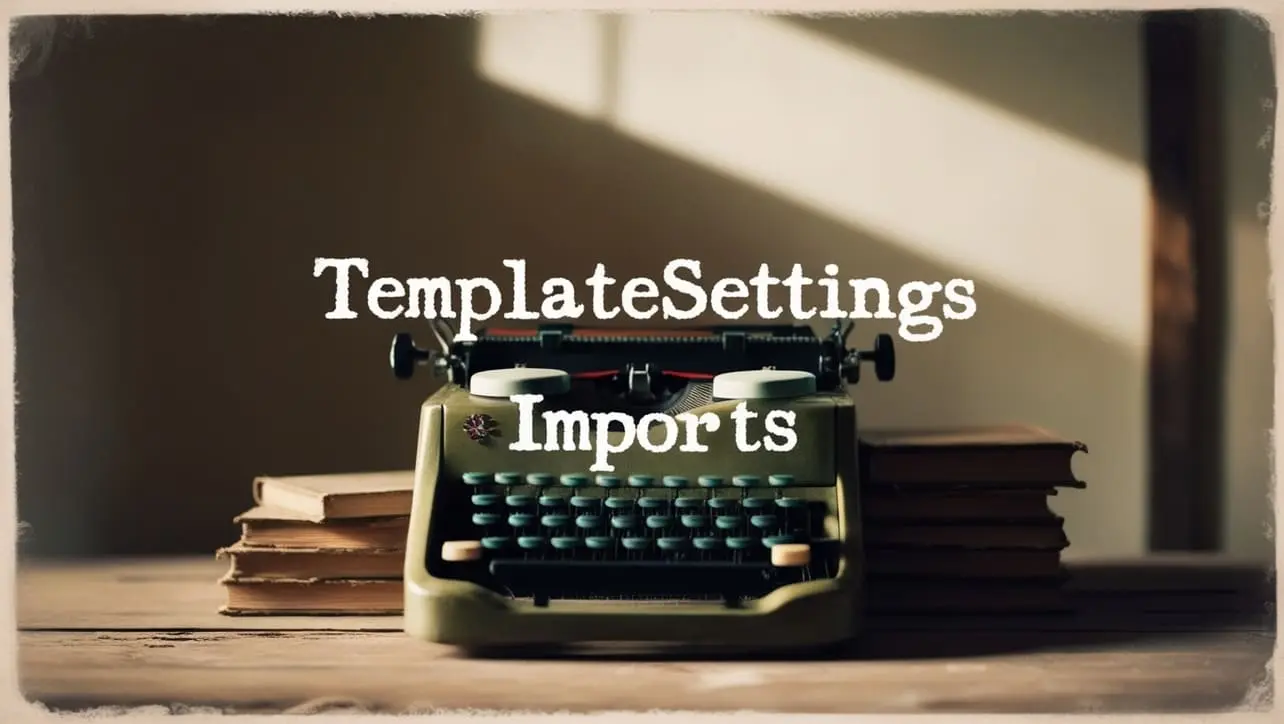
Lodash _.before() Function Method
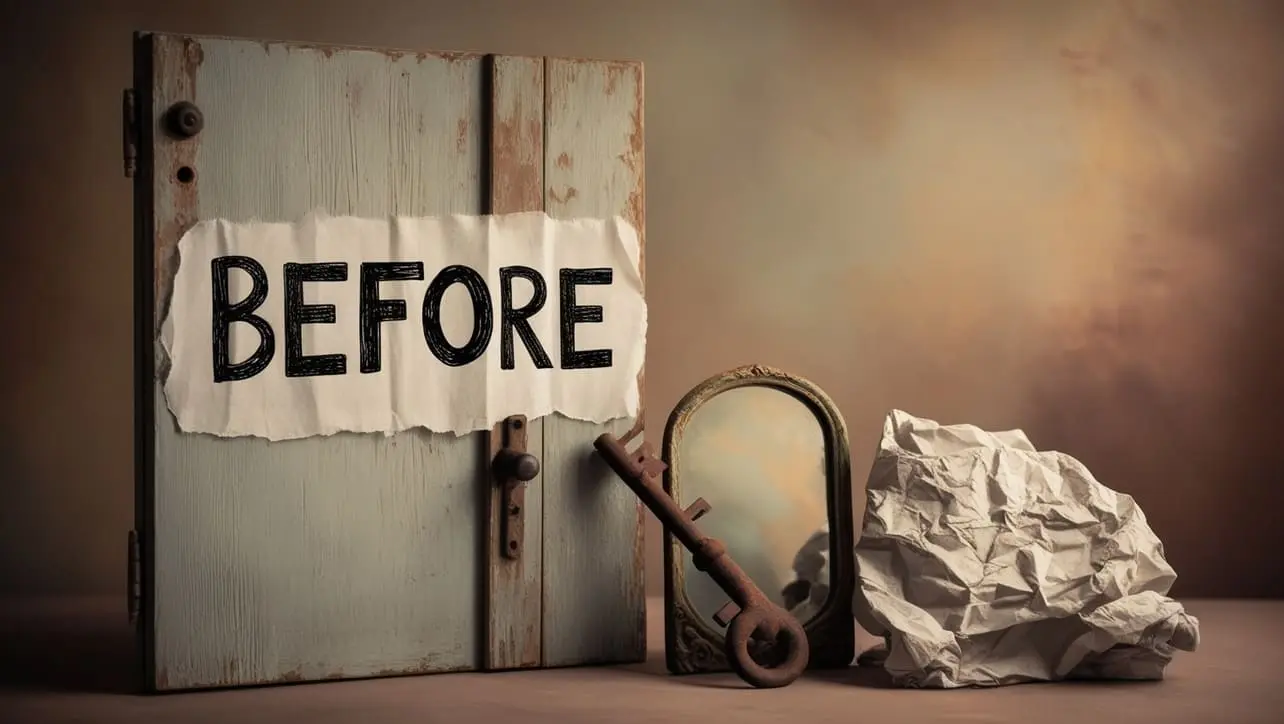
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, effective control over the invocation of functions can be crucial for certain scenarios. Enter Lodash, a comprehensive utility library that provides a variety of functions to simplify and optimize JavaScript code. Among these functions is the _.before()
method, a powerful tool for limiting the number of times a function can be called.
This method is particularly useful when you need to restrict the execution of a function to a specified threshold.
🧠 Understanding _.before() Method
The _.before()
method in Lodash is designed to create a function that invokes the provided function only until a specified number of calls have been made. Once the limit is reached, subsequent calls to the generated function will return the result of the final invocation. This can be beneficial for scenarios where you want to perform an action a certain number of times or under specific conditions.
💡 Syntax
The syntax for the _.before()
method is straightforward:
_.before(n, func)
- n: The number of calls at which the provided function will no longer be invoked.
- func: The function to be called.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.before()
method:
const _ = require('lodash');
// Create a function that logs a message and stops after 3 calls
const limitedFunction = _.before(3, () => {
console.log('Function is called!');
});
// Invoke the function multiple times
limitedFunction(); // Output: Function is called!
limitedFunction(); // Output: Function is called!
limitedFunction(); // Output: Function is called!
// Further invocations have no effect
limitedFunction(); // No output
In this example, the limitedFunction is created using _.before(3, ...), allowing it to be called only three times.
🏆 Best Practices
When working with the _.before()
method, consider the following best practices:
Define a Sensible Threshold:
When using
_.before()
, define a threshold (n) that makes sense for your use case. Setting an appropriate limit ensures that the function behaves as expected and doesn't unnecessarily restrict or allow too many invocations.example.jsCopiedconst limitedFunction = _.before(5, () => { console.log('Function is called!'); }); // Invoke the function multiple times limitedFunction(); // Output: Function is called! limitedFunction(); // Output: Function is called! limitedFunction(); // Output: Function is called! limitedFunction(); // Output: Function is called! limitedFunction(); // Output: Function is called! // Further invocations have no effect limitedFunction(); // No output
Use Case-Specific Functions:
Tailor the provided function (func) to perform the desired action within the specified limit. This ensures that the limited function serves its intended purpose effectively.
example.jsCopiedconst printMessageBeforeLimit = _.before(3, (message) => { console.log(message); }); // Invoke the function with different messages printMessageBeforeLimit('First message'); // Output: First message printMessageBeforeLimit('Second message'); // Output: Second message printMessageBeforeLimit('Third message'); // Output: Third message // Further invocations have no effect printMessageBeforeLimit('Extra message'); // No output
Combine with Conditions:
Combine
_.before()
with conditional logic within the provided function for more dynamic behavior. This allows you to control the execution based on specific conditions.example.jsCopiedconst limitedFunctionWithCondition = _.before(2, (value) => { if (value > 0) { console.log('Positive value detected!'); } }); // Invoke the function with different values limitedFunctionWithCondition(5); // Output: Positive value detected! limitedFunctionWithCondition(-2); // Output: Positive value detected! // Further invocations have no effect limitedFunctionWithCondition(10); // No output
📚 Use Cases
Rate Limiting:
_.before()
is valuable for implementing rate-limiting functionality, where you want to restrict the frequency of certain actions, such as API calls or user interactions.example.jsCopiedconst performLimitedAction = _.before(3, () => { // Perform an action, e.g., make an API call console.log('API call made!'); }); // Trigger the action based on user interactions performLimitedAction(); // Output: API call made! performLimitedAction(); // Output: API call made! performLimitedAction(); // Output: API call made! // Further triggers have no effect performLimitedAction(); // No output
Initialization Tasks:
Use
_.before()
for limiting initialization tasks in situations where certain setup actions need to be performed only a specified number of times.example.jsCopiedconst initializeApp = _.before(1, () => { // Perform initialization tasks console.log('App initialized!'); }); // Initialize the app initializeApp(); // Output: App initialized! // Further invocations have no effect initializeApp(); // No output
User Interaction Handling:
In scenarios where you want to handle user interactions up to a certain point,
_.before()
can be employed to control the behavior of the interaction handler.example.jsCopiedconst handleUserInteraction = _.before(2, () => { // Handle user interaction, e.g., display a message console.log('User interaction handled!'); }); // Trigger user interactions handleUserInteraction(); // Output: User interaction handled! handleUserInteraction(); // Output: User interaction handled! // Further triggers have no effect handleUserInteraction(); // No output
🎉 Conclusion
The _.before()
method in Lodash provides a convenient way to limit the number of times a function can be called. Whether you're implementing rate limiting, controlling initialization tasks, or managing user interactions, _.before()
offers a versatile tool for achieving precise control over function invocations in your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.before()
method in your Lodash projects.
👨💻 Join our Community:
Author
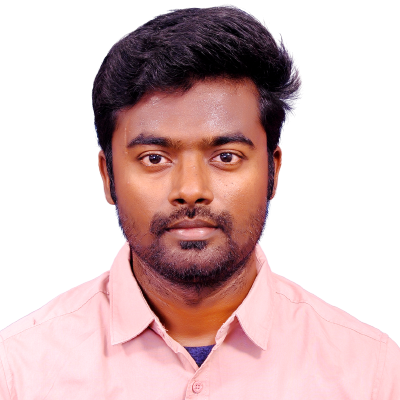
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
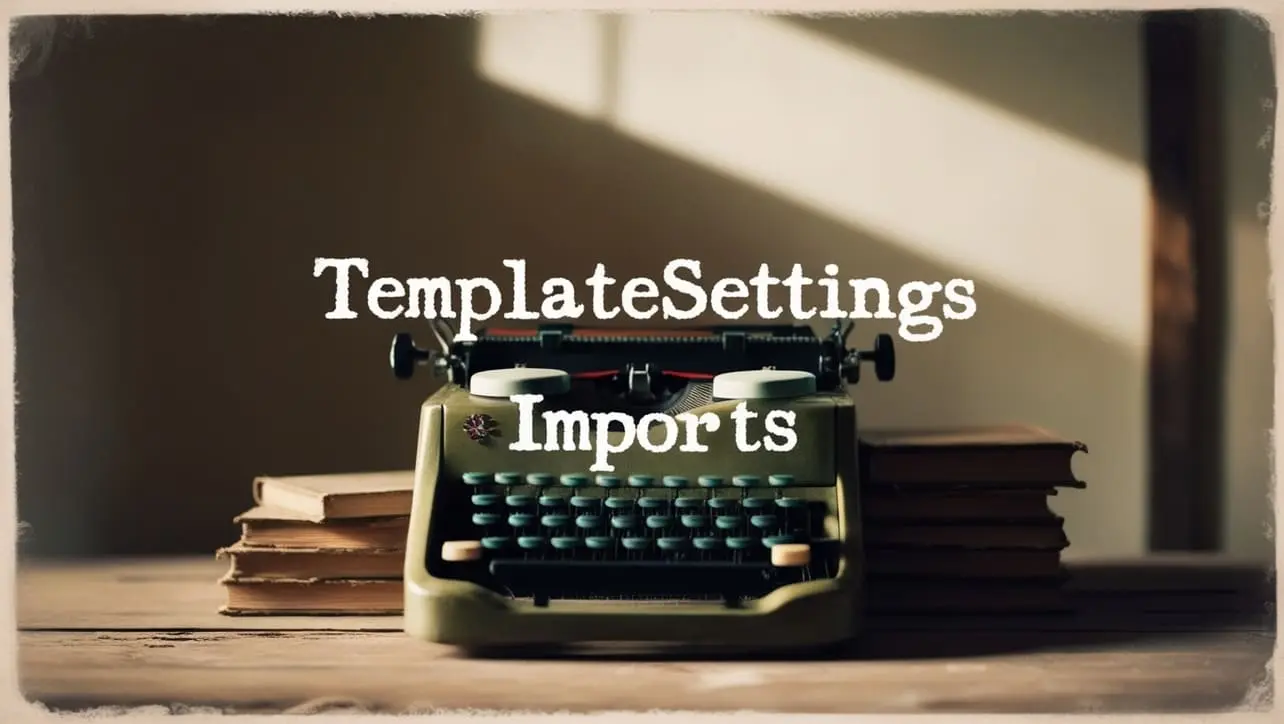
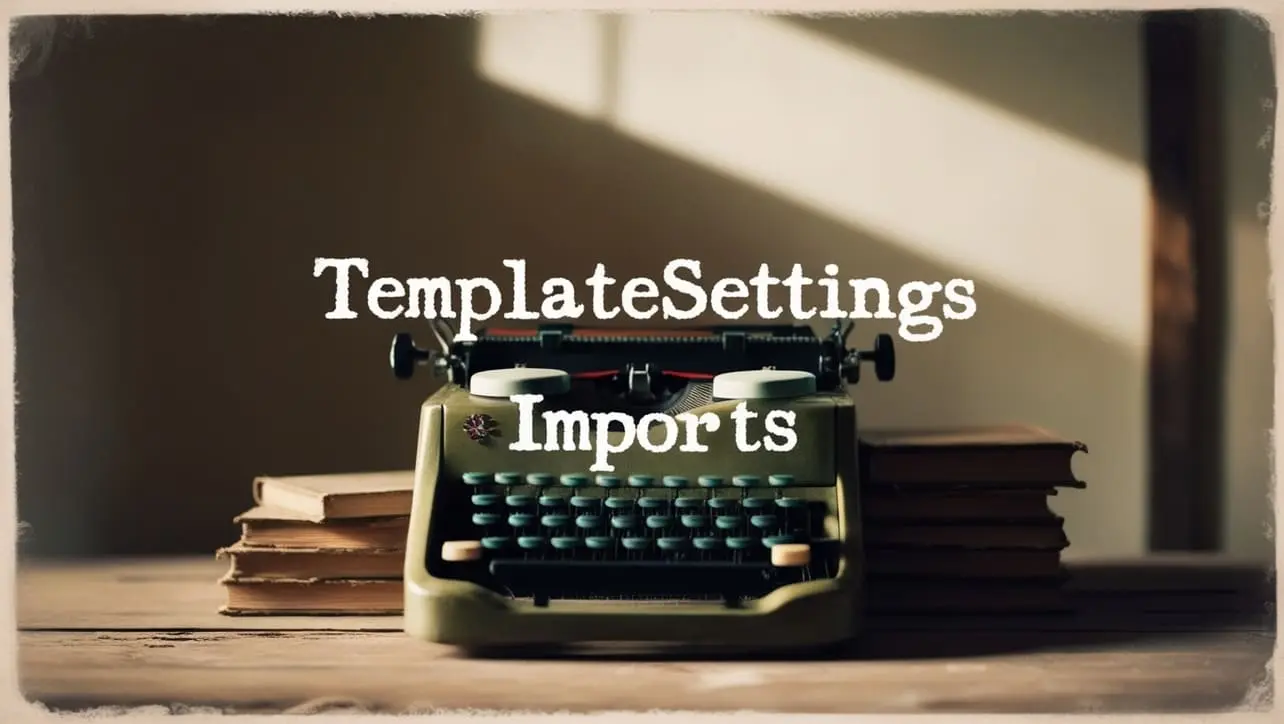
Lodash _.templateSettings.imports Property
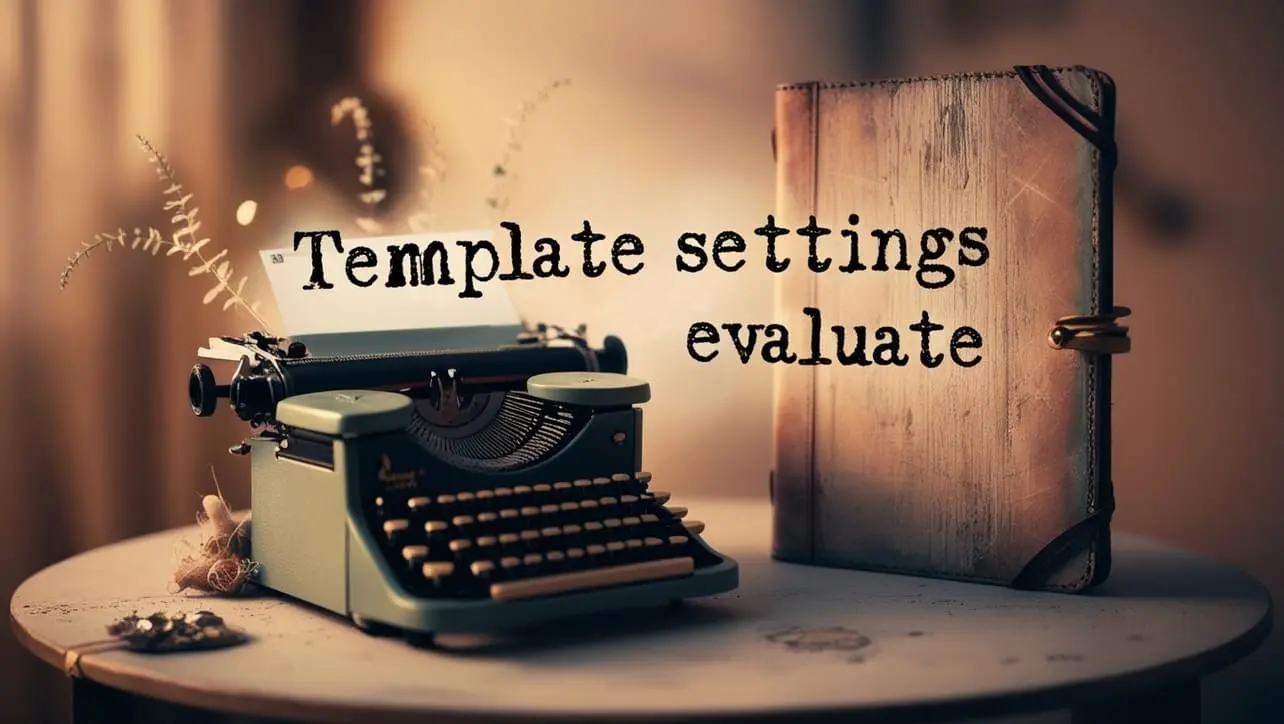
Lodash _.templateSettings.evaluate Property
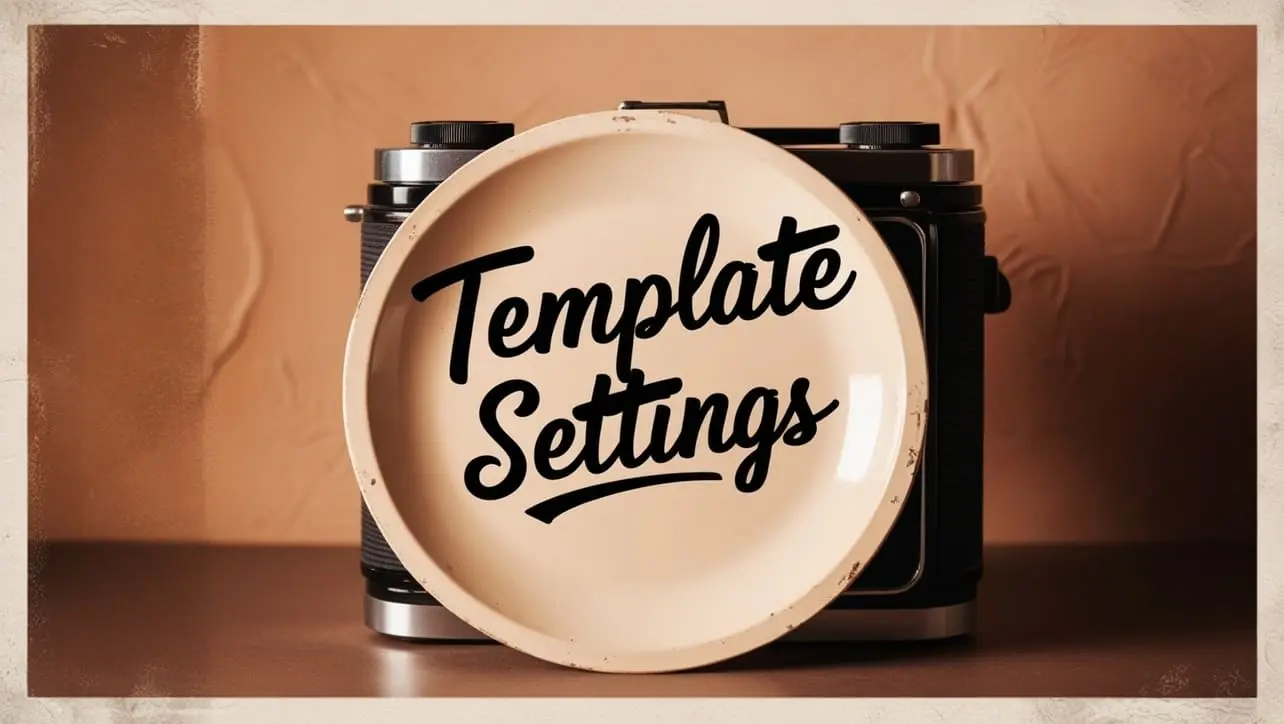
Lodash _.templateSettings Property
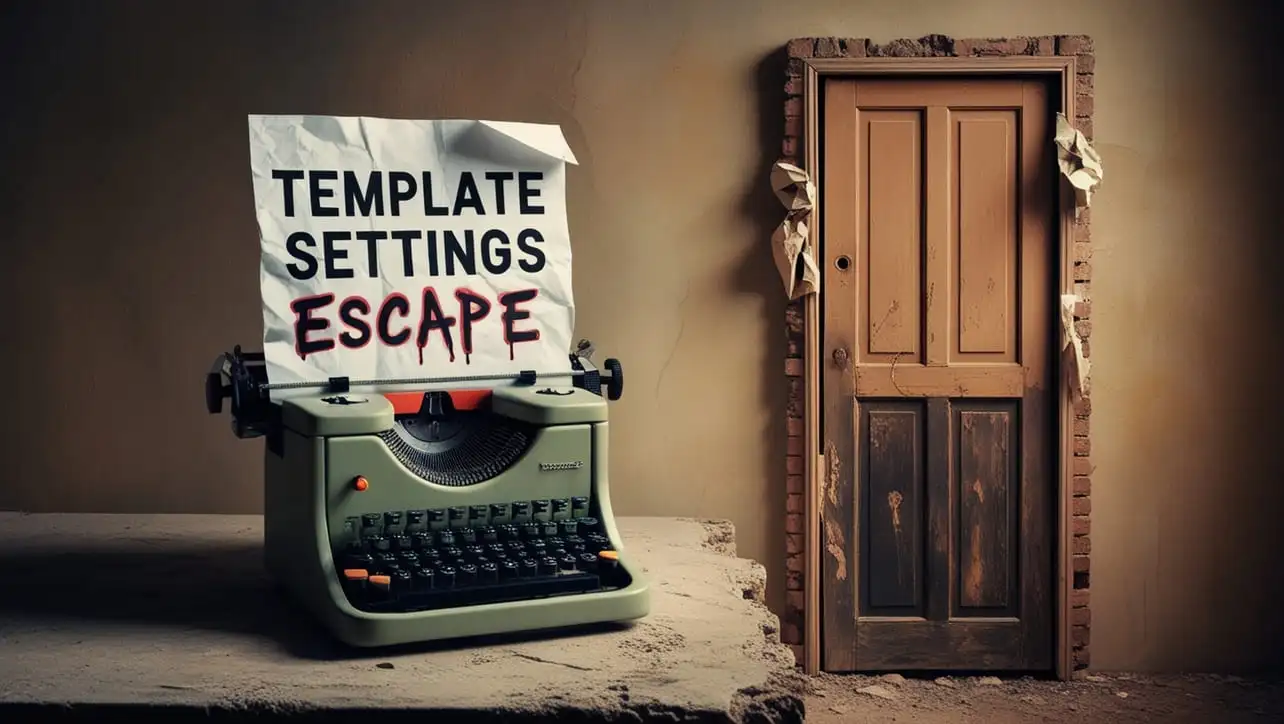
Lodash _.templateSettings.escape Property
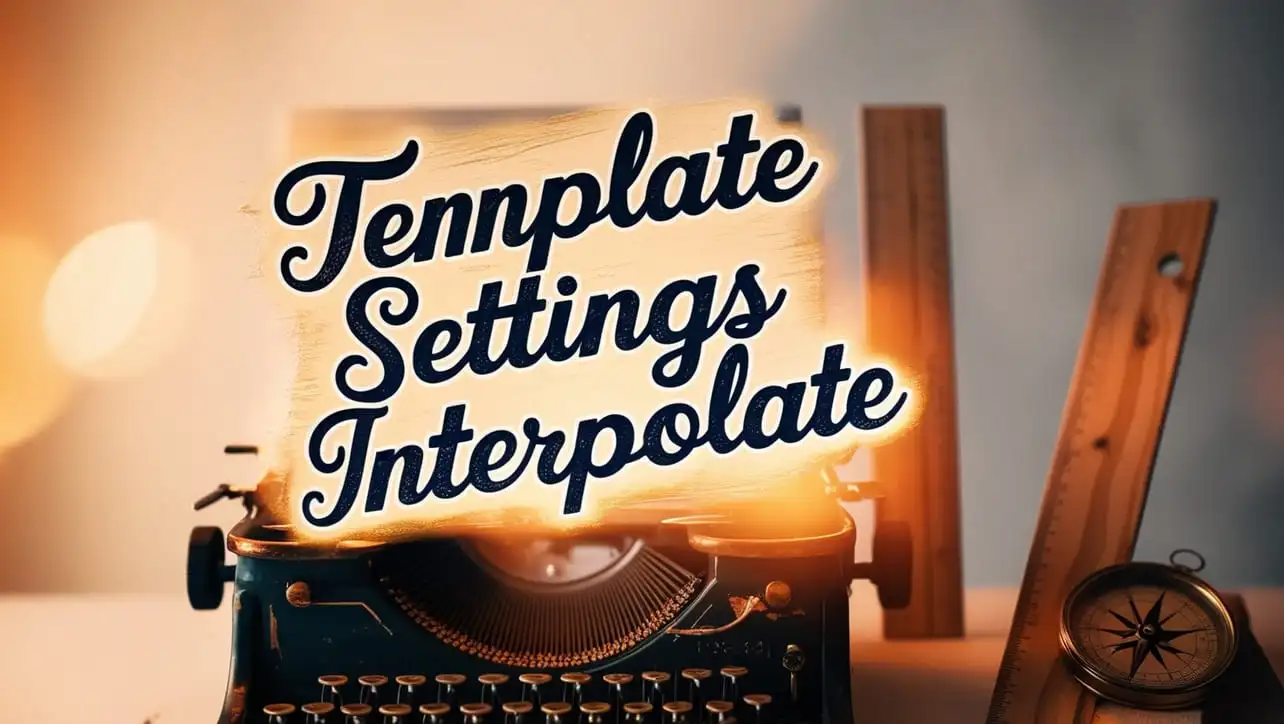
Lodash _.templateSettings.interpolate Property
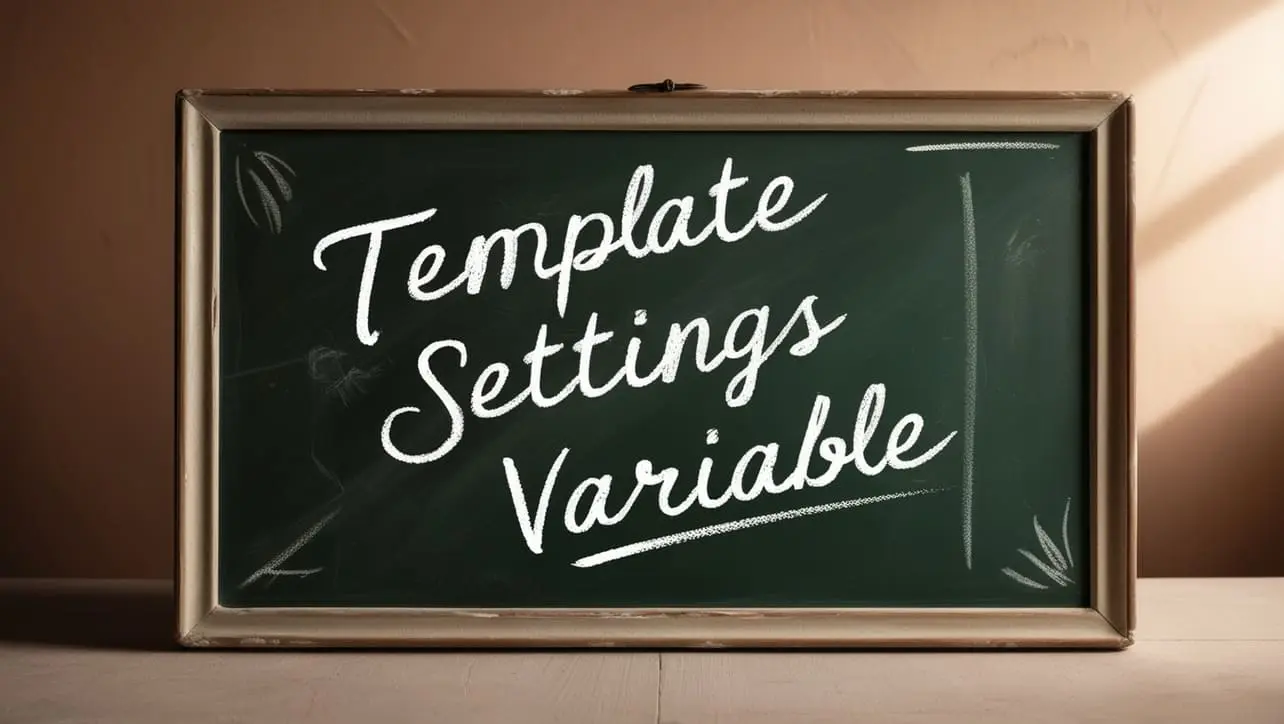
If you have any doubts regarding this article (Lodash _.before() Function Method), please comment here. I will help you immediately.