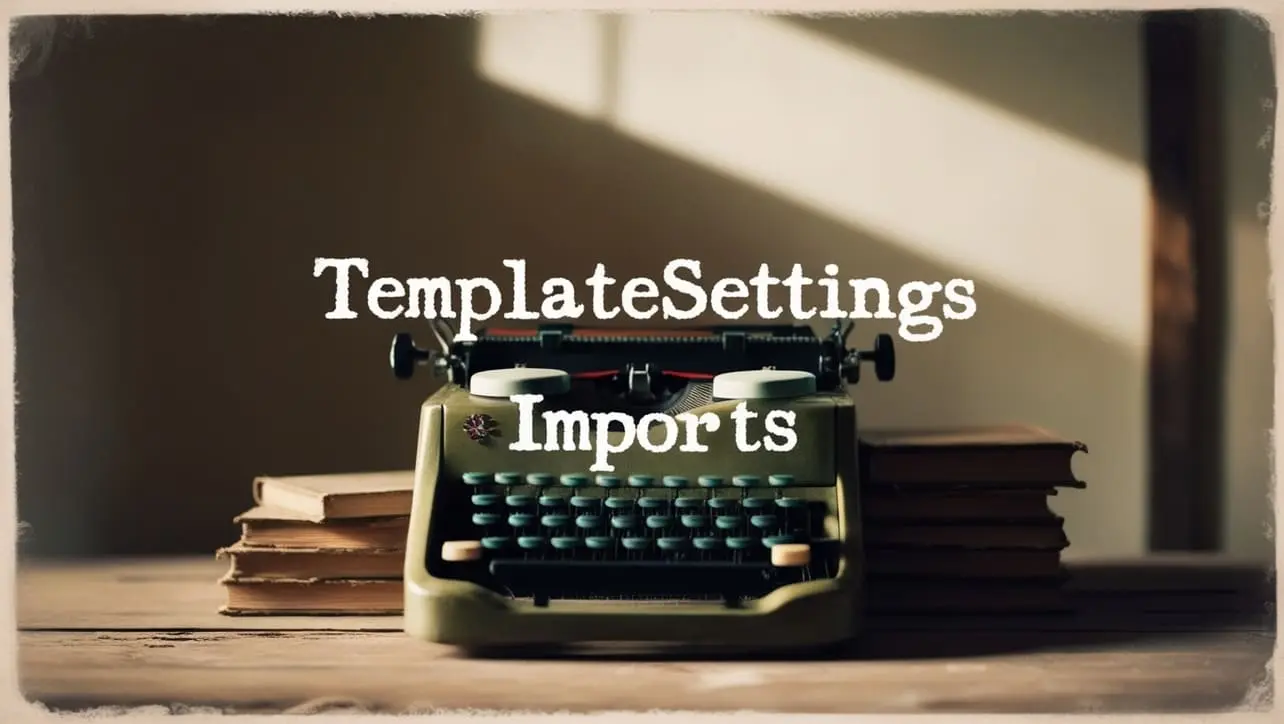
Lodash _.after() Function Method
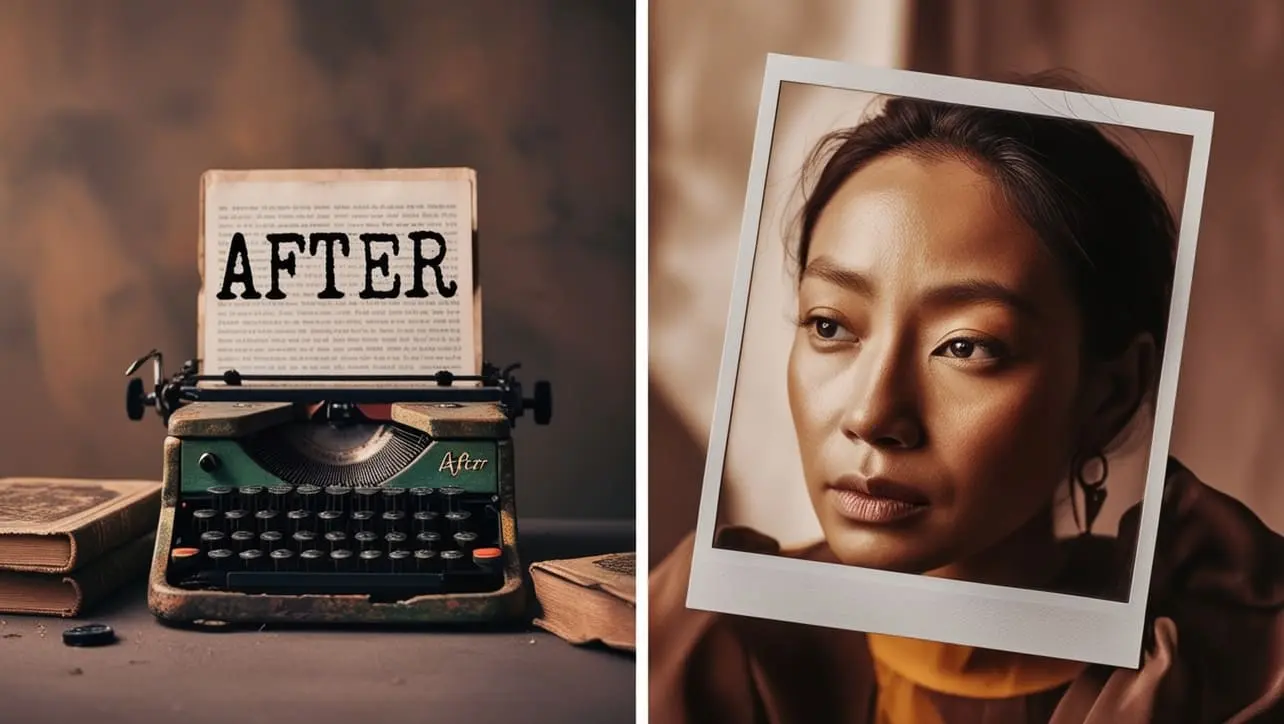
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, effective control over the execution flow is crucial. Lodash, a versatile utility library, provides the _.after()
function method to aid developers in managing the timing of function calls.
This method allows you to create a function that executes only after being called a specified number of times, providing control and precision in your code.
🧠 Understanding _.after() Method
The _.after()
method in Lodash is designed to create a function that will only execute after being invoked a specified number of times. This can be particularly useful when dealing with asynchronous operations or scenarios where a function should run only after a certain condition is met.
💡 Syntax
The syntax for the _.after()
method is straightforward:
_.after(n, func)
- n: The number of times the returned function must be called before the original function (func) is invoked.
- func: The function to be executed.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.after()
method:
const _ = require('lodash');
const afterTwoCalls = _.after(2, () => {
console.log('Function executed after two calls.');
});
afterTwoCalls();
afterTwoCalls();
// Output: Function executed after two calls.
In this example, the afterTwoCalls function is created using _.after(2), and it only executes the provided function after being called twice.
🏆 Best Practices
When working with the _.after()
method, consider the following best practices:
Asynchronous Operations:
Consider using
_.after()
in scenarios involving asynchronous operations where you need a function to execute only after a specific number of asynchronous tasks have completed.example.jsCopiedconst fetchData = async () => { // Simulate asynchronous data fetching return new Promise(resolve => setTimeout(() => resolve('Data loaded'), 1000)); }; const afterDataLoaded = _.after(3, () => { console.log('All data loaded successfully.'); }); fetchData().then(afterDataLoaded); fetchData().then(afterDataLoaded); fetchData().then(afterDataLoaded); // Output: All data loaded successfully.
Event Handling:
Use
_.after()
for event handling scenarios where a function should be triggered only after a certain number of events have occurred.example.jsCopiedconst buttonClickHandler = _.after(2, () => { console.log('Button clicked twice. Performing action...'); // Perform the desired action after the button is clicked twice }); // Simulate button clicks buttonClickHandler(); buttonClickHandler();
Conditional Execution:
Employ
_.after()
for conditional execution, ensuring that a function runs only when a specific condition is met a certain number of times.example.jsCopiedconst checkCondition = _.after(3, () => { console.log('Condition met three times. Proceeding...'); // Proceed with further execution after the condition is met three times }); // Simulate checking a condition checkCondition(); checkCondition(); checkCondition(); // Output: Condition met three times. Proceeding...
📚 Use Cases
Throttling Function Execution:
Use
_.after()
to implement function throttling, allowing a function to execute only after a certain number of calls, preventing rapid-fire execution.example.jsCopiedconst throttledLog = _.after(3, message => { console.log(`Throttled log: ${message}`); }); throttledLog('First call'); throttledLog('Second call'); throttledLog('Third call'); // Output: Throttled log: Third call
Initialization After Setup:
Utilize
_.after()
for initialization tasks that should occur only after a series of setup functions have been called.example.jsCopiedconst initializeApp = _.after(2, () => { console.log('App initialized after setup.'); // Perform initialization tasks after two setup functions have completed }); // Simulate setup functions initializeApp(); initializeApp();
Post-Validation Actions:
Apply
_.after()
for scenarios where certain actions should be taken only after a validation function has passed a specific number of times.example.jsCopiedconst validateUser = _.after(3, () => { console.log('User validated successfully. Granting access...'); // Grant access after user validation passes three times }); // Simulate user validation attempts validateUser(); validateUser(); validateUser(); // Output: User validated successfully. Granting access...
🎉 Conclusion
The _.after()
function method in Lodash empowers JavaScript developers with a powerful tool for controlling the timing of function execution. Whether you're dealing with asynchronous operations, event handling, or conditional execution, _.after()
provides a concise and effective way to manage the flow of your code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.after()
method in your Lodash projects.
👨💻 Join our Community:
Author
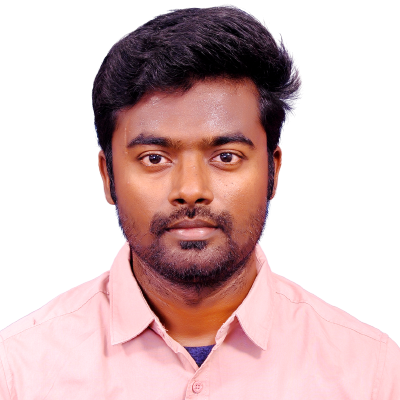
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
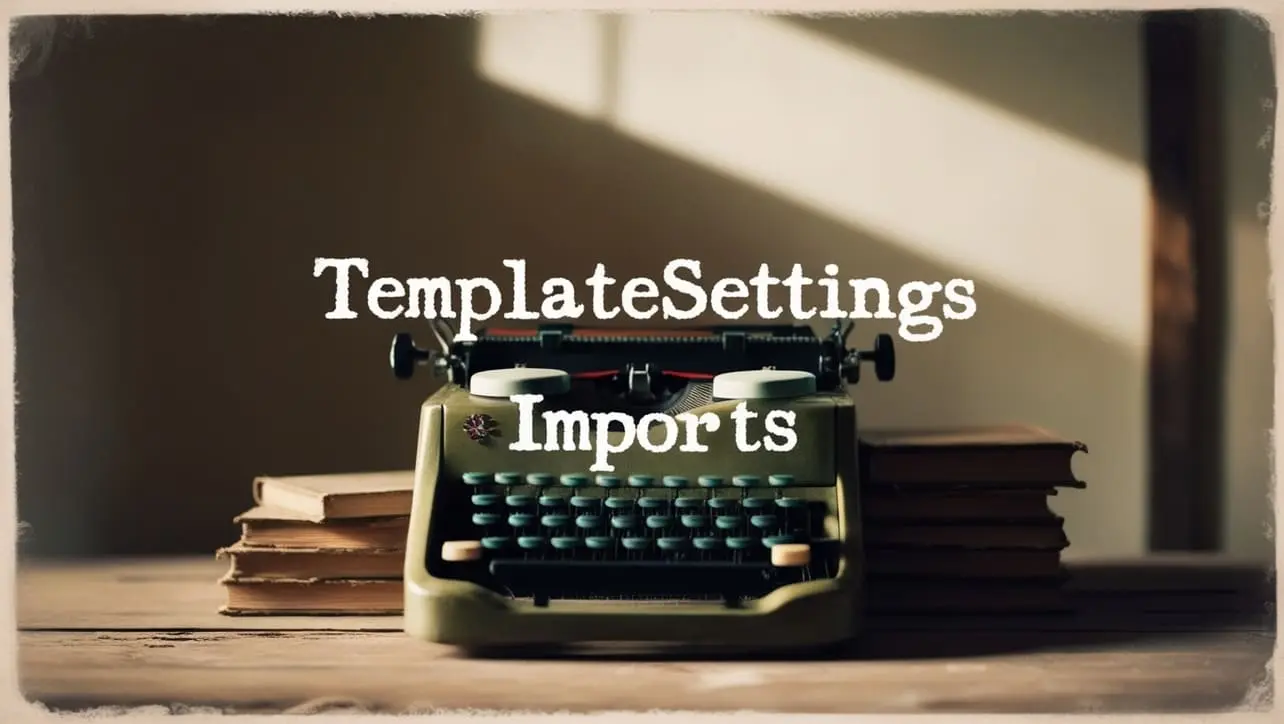
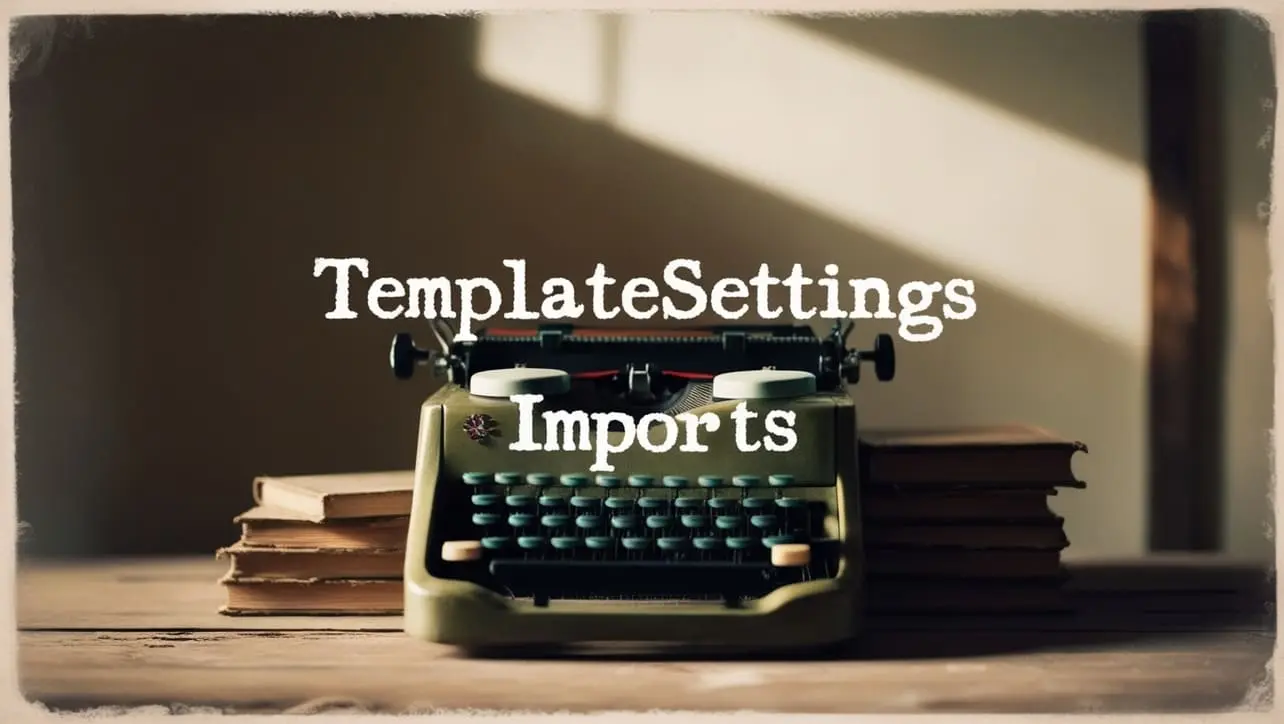
Lodash _.templateSettings.imports Property
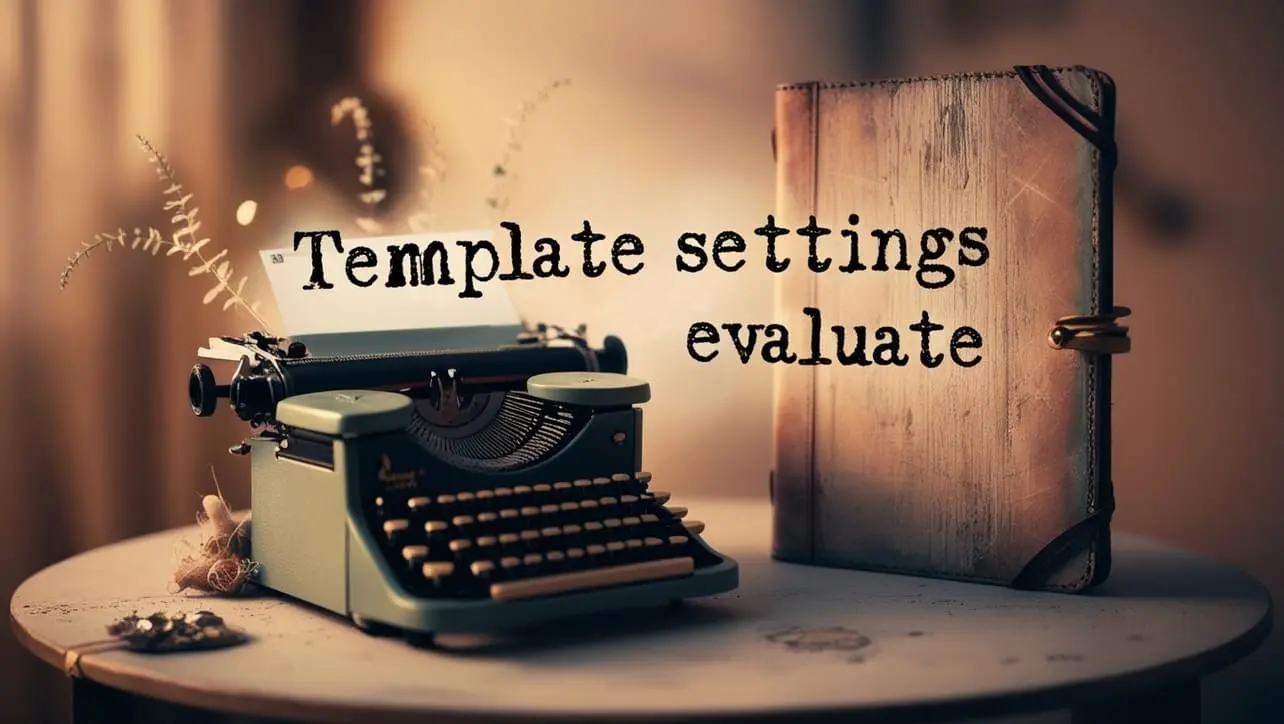
Lodash _.templateSettings.evaluate Property
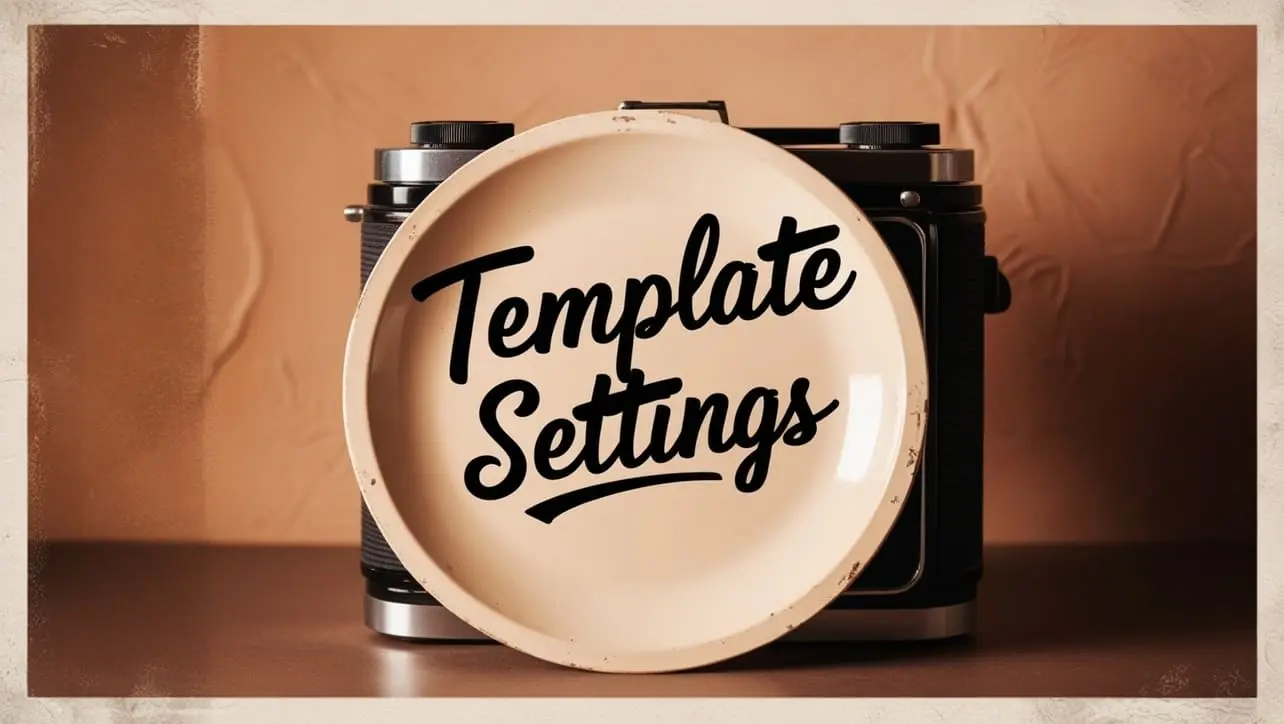
Lodash _.templateSettings Property
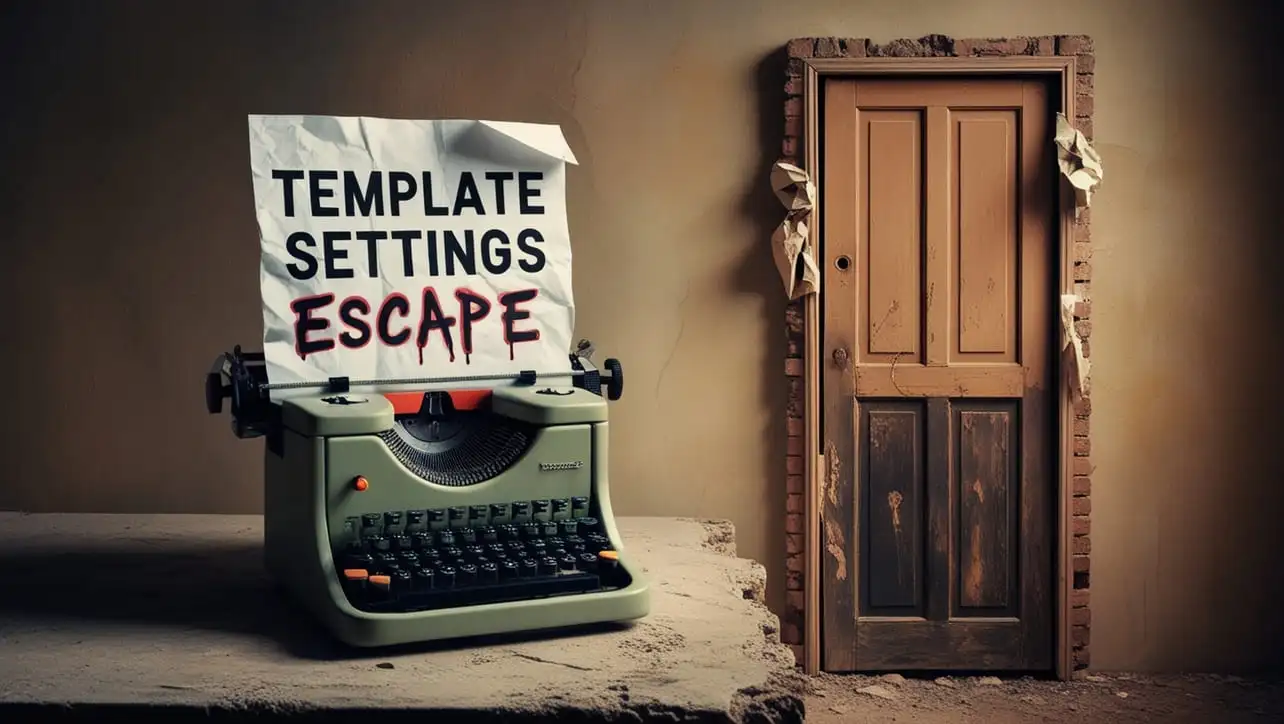
Lodash _.templateSettings.escape Property
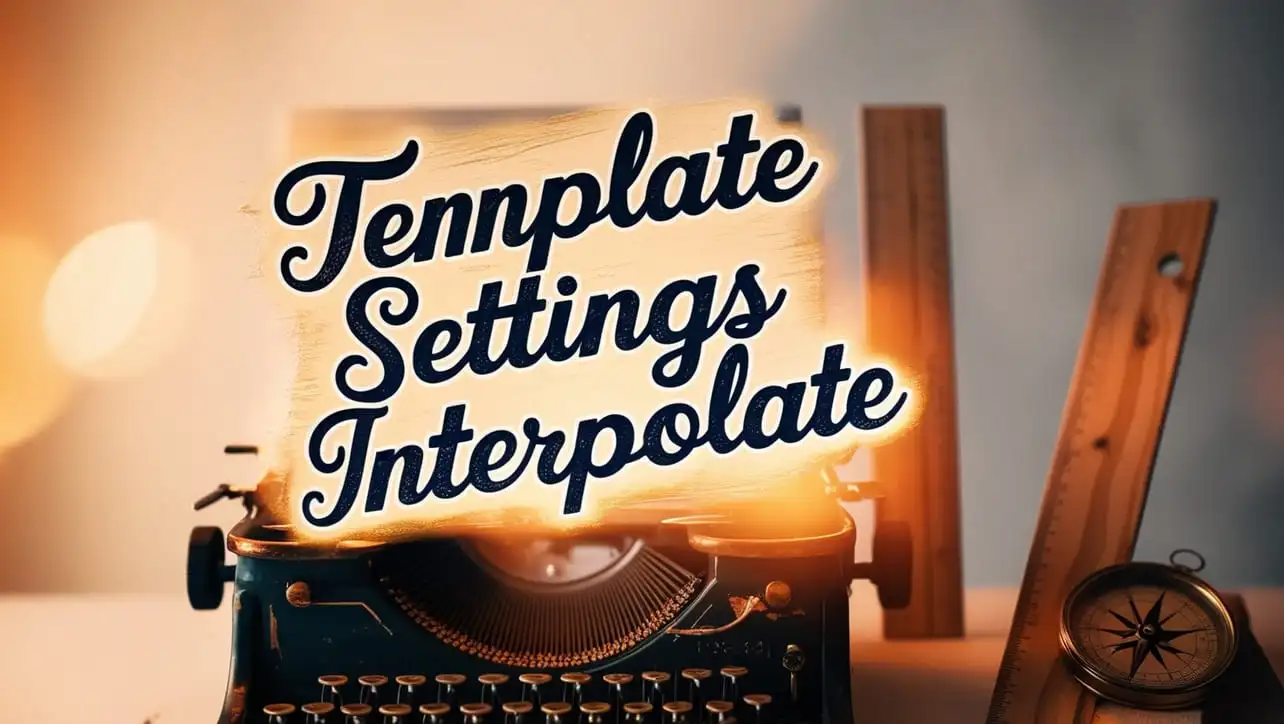
Lodash _.templateSettings.interpolate Property
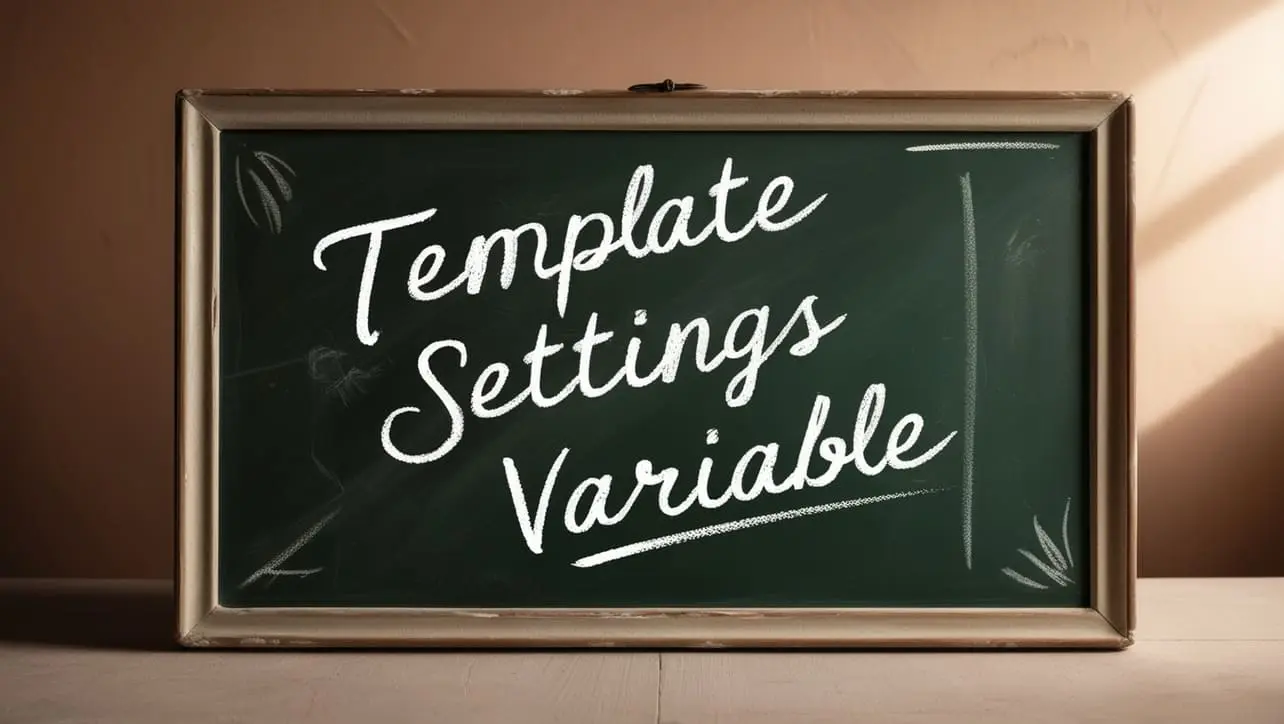
If you have any doubts regarding this article (Lodash _.after() Function Method), please comment here. I will help you immediately.