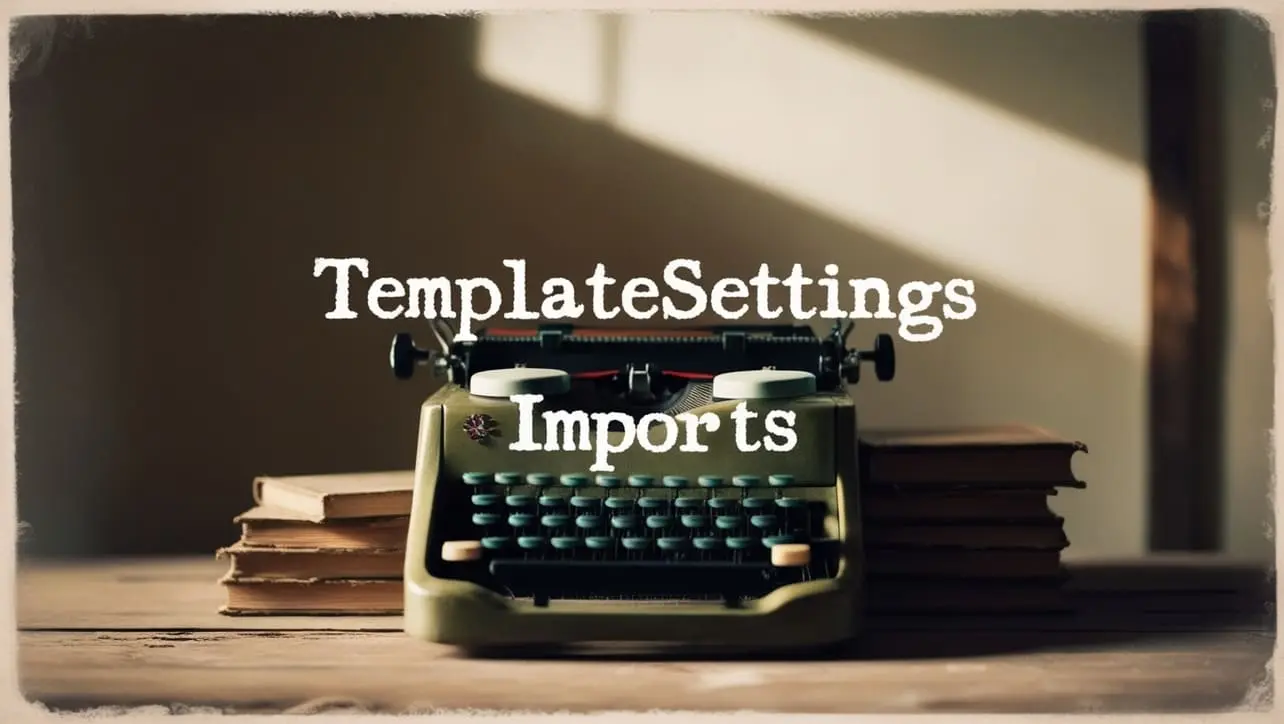
Lodash _.size() Collection Method
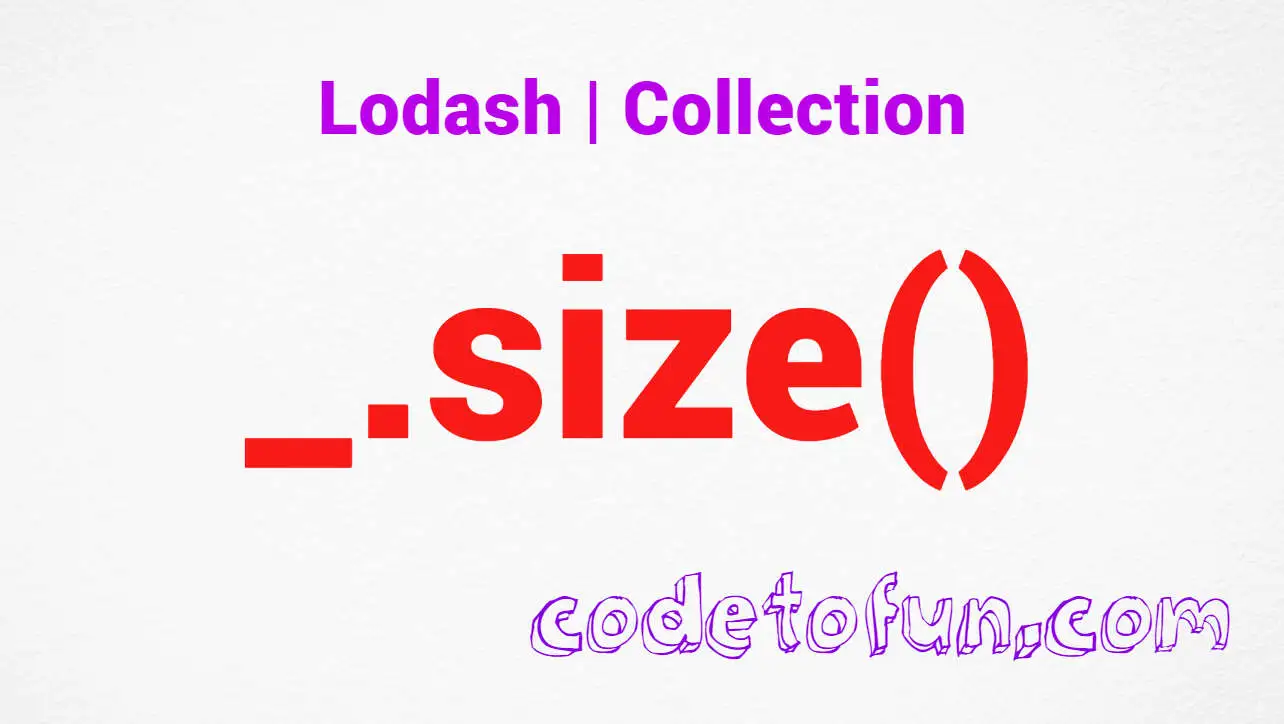
Photo Credit to CodeToFun
🙋 Introduction
fficiently managing the size of collections is a common task in JavaScript development. Lodash, a powerful utility library, provides the _.size()
method to simplify the process of determining the size of arrays, objects, and other iterable collections.
This method proves invaluable when working with dynamic data structures, offering a straightforward way to obtain the number of elements within a collection.
🧠 Understanding _.size() Method
The _.size()
method in Lodash is designed to calculate the number of elements in a collection, providing a universal solution for arrays, objects, and other iterable entities.
💡 Syntax
The syntax for the _.size()
method is straightforward:
_.size(collection)
- collection: The collection for which to determine the size.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.size()
method:
const _ = require('lodash');
const array = [1, 2, 3, 4, 5];
const object = { a: 1, b: 2, c: 3 };
const sizeOfArray = _.size(array);
const sizeOfObject = _.size(object);
console.log(sizeOfArray); // Output: 5
console.log(sizeOfObject); // Output: 3
In this example, _.size()
is used to determine the number of elements in both an array and an object.
🏆 Best Practices
When working with the _.size()
method, consider the following best practices:
Consistent Handling of Different Collection Types:
Use
_.size()
consistently across different types of collections. Whether it's an array, object, or another iterable, this method provides a uniform way to obtain the size.example.jsCopiedconst mixedCollection = [1, { a: 2 }, [3, 4], 'five']; const sizeOfMixedCollection = _.size(mixedCollection); console.log(sizeOfMixedCollection); // Output: 4
Check for Undefined or Null Collections:
Before using
_.size()
, ensure that the collection is defined and not null. This helps prevent unexpected errors when determining the size.example.jsCopiedconst undefinedCollection = undefined; const nullCollection = null; const sizeOfUndefined = _.size(undefinedCollection); // Output: 0 const sizeOfNull = _.size(nullCollection); // Output: 0
Utilize with Conditional Logic:
Incorporate
_.size()
into conditional logic to handle cases where a collection might be empty or non-existent.example.jsCopiedconst dynamicCollection = /* ...some dynamically generated collection... */; if (_.size(dynamicCollection) > 0) { // Process the collection console.log('Collection has elements:', dynamicCollection); } else { console.log('Collection is empty or undefined.'); }
📚 Use Cases
Dynamic UI Rendering:
When dynamically rendering UI components based on data,
_.size()
can be used to check if there are elements in the dataset, influencing the display logic.example.jsCopiedconst dynamicData = /* ...fetch data from API or elsewhere... */; if (_.size(dynamicData) > 0) { // Render UI components console.log('Rendering UI with data:', dynamicData); } else { console.log('No data available for rendering.'); }
Validation and Error Handling:
In scenarios where input parameters are collections,
_.size()
can be used for validation and error handling to ensure that the expected data structure is provided.example.jsCopiedfunction processData(data) { if (_.size(data) === 0) { console.error('Error: Empty data provided.'); return; } // Continue processing data console.log('Processing data:', data); } processData(/* ...some data... */);
Iterating Over Dynamic Collections:
When iterating over dynamic collections with unknown structures,
_.size()
can help determine the size before performing iteration operations.example.jsCopiedconst dynamicCollection = /* ...fetch data from API or elsewhere... */; const collectionSize = _.size(dynamicCollection); for (let i = 0; i < collectionSize; i++) { // Iterate over the collection console.log('Element at index', i, ':', dynamicCollection[i]); }
🎉 Conclusion
The _.size()
method in Lodash is a versatile tool for determining the size of collections, be it arrays, objects, or other iterable entities. By embracing this method, developers can streamline their code when dealing with dynamic data structures, improving consistency and reducing the likelihood of errors.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.size()
method in your Lodash projects.
👨💻 Join our Community:
Author
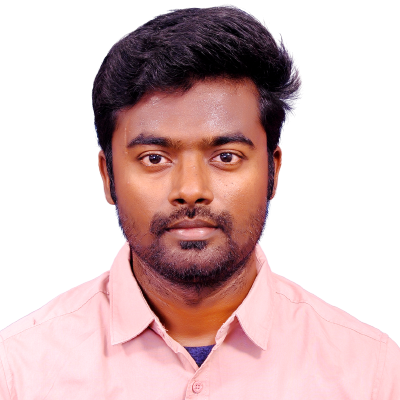
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
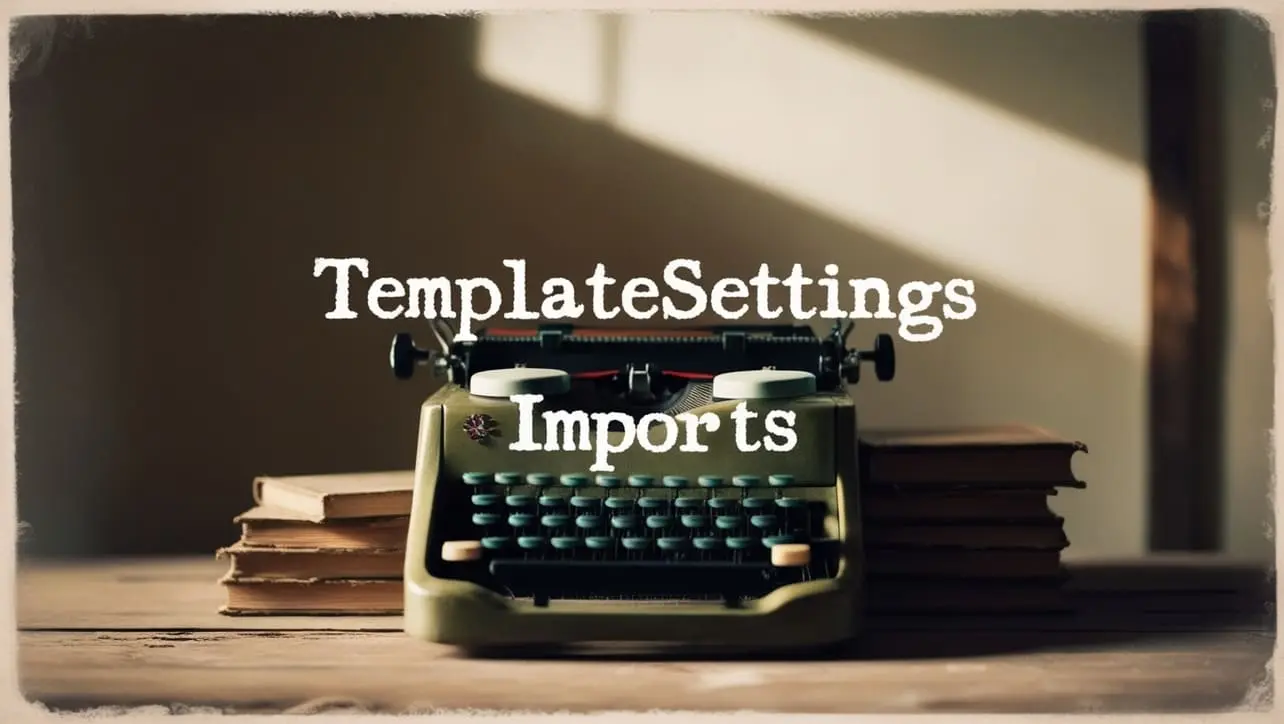
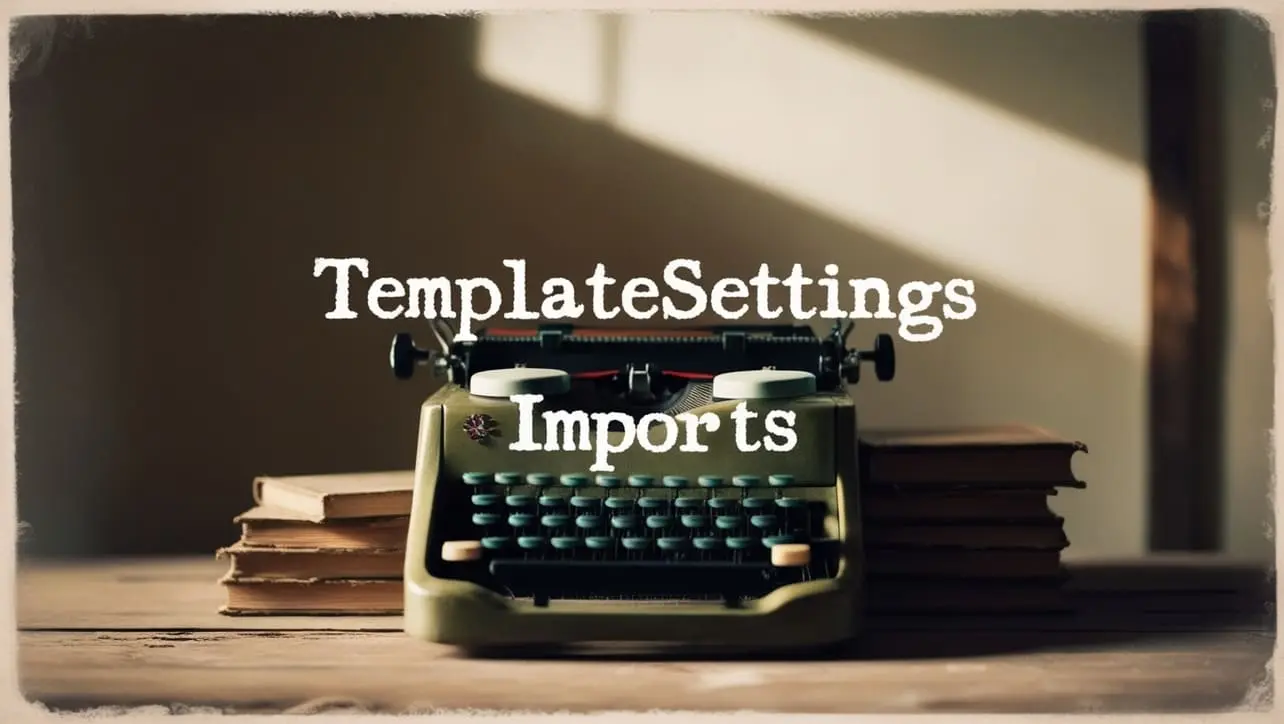
Lodash _.templateSettings.imports Property
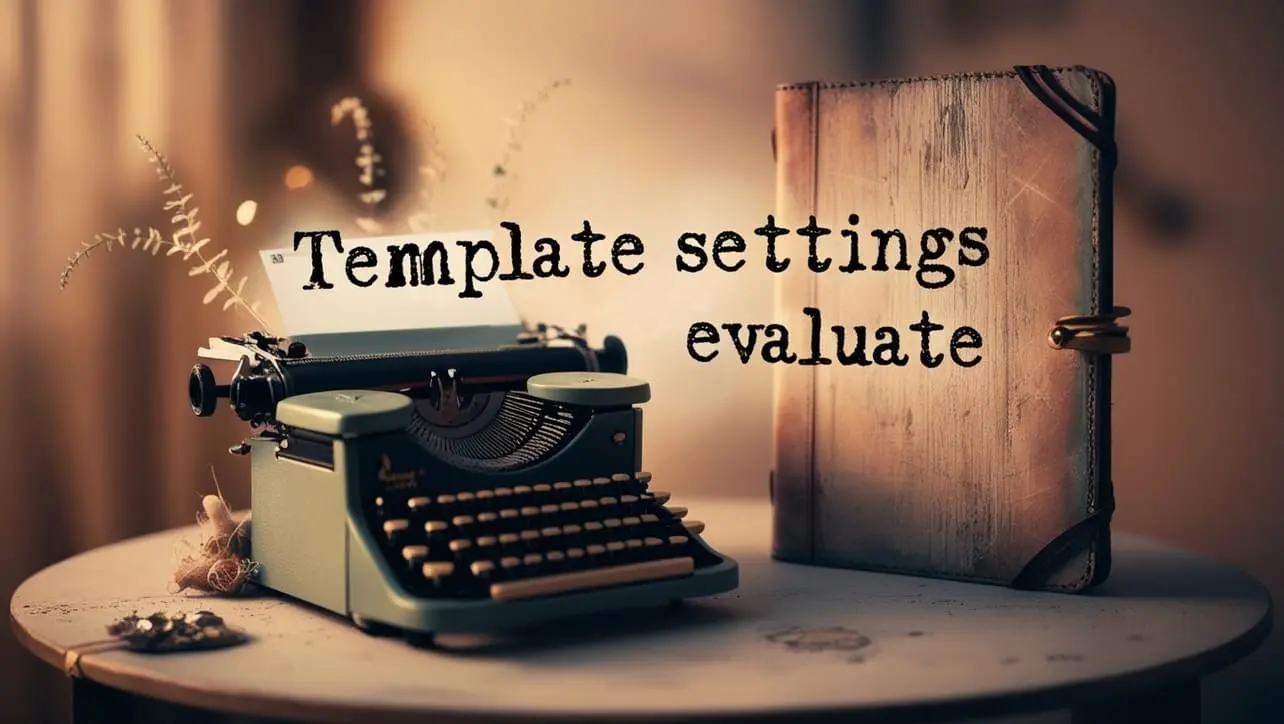
Lodash _.templateSettings.evaluate Property
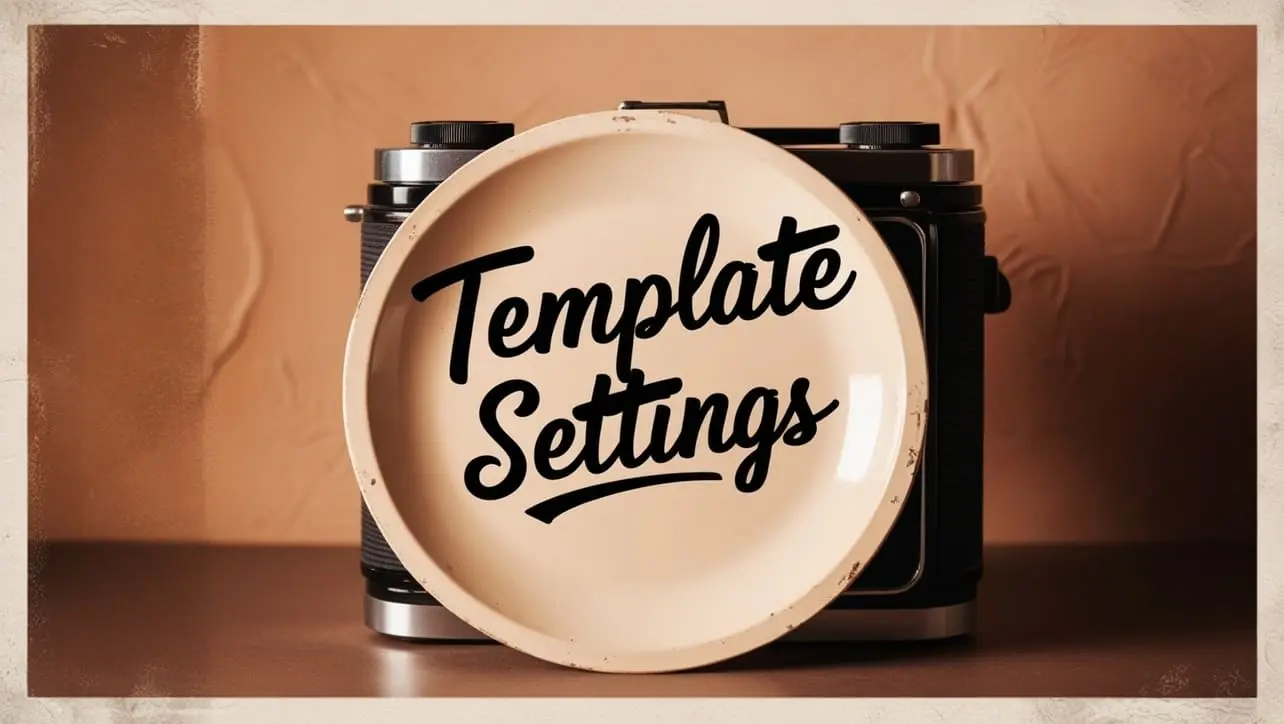
Lodash _.templateSettings Property
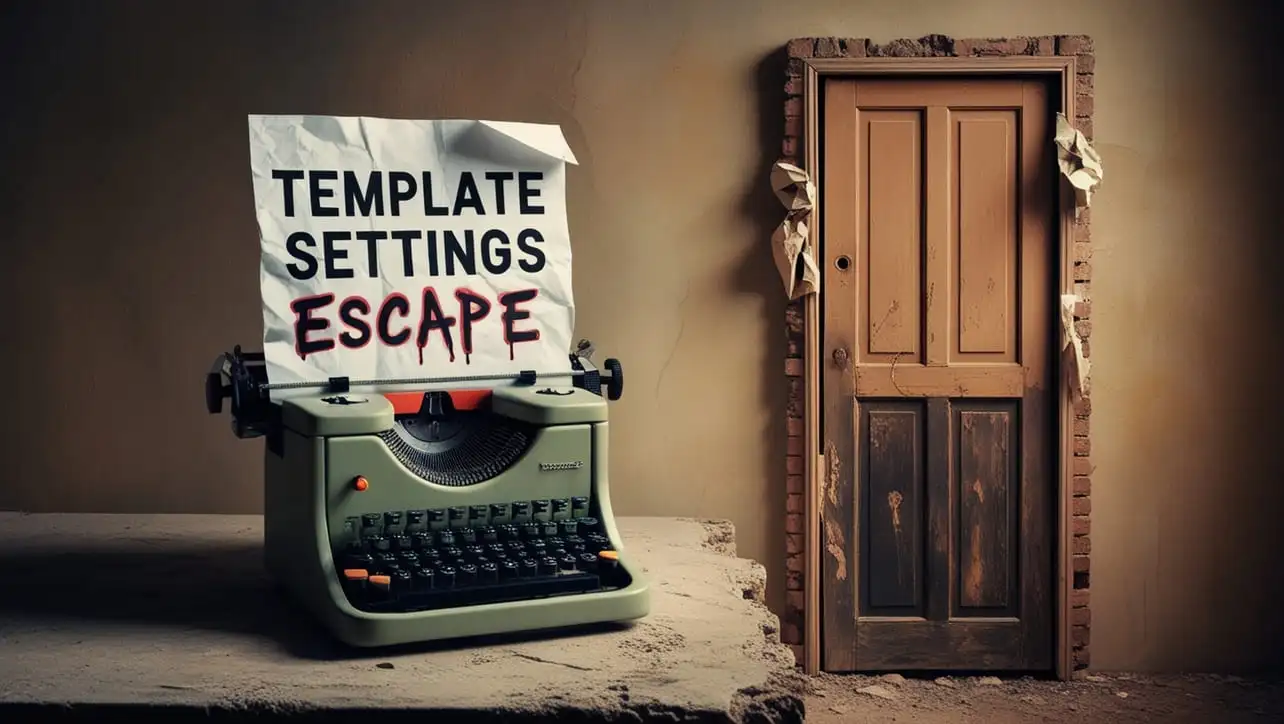
Lodash _.templateSettings.escape Property
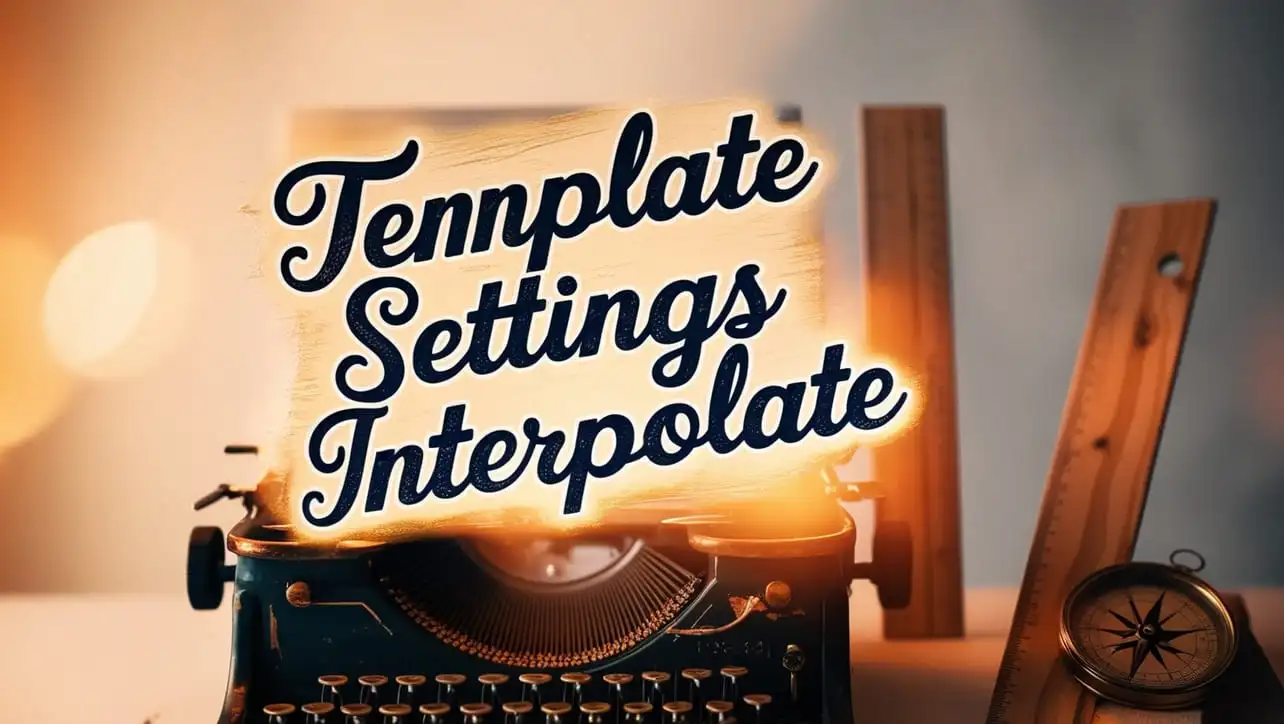
Lodash _.templateSettings.interpolate Property
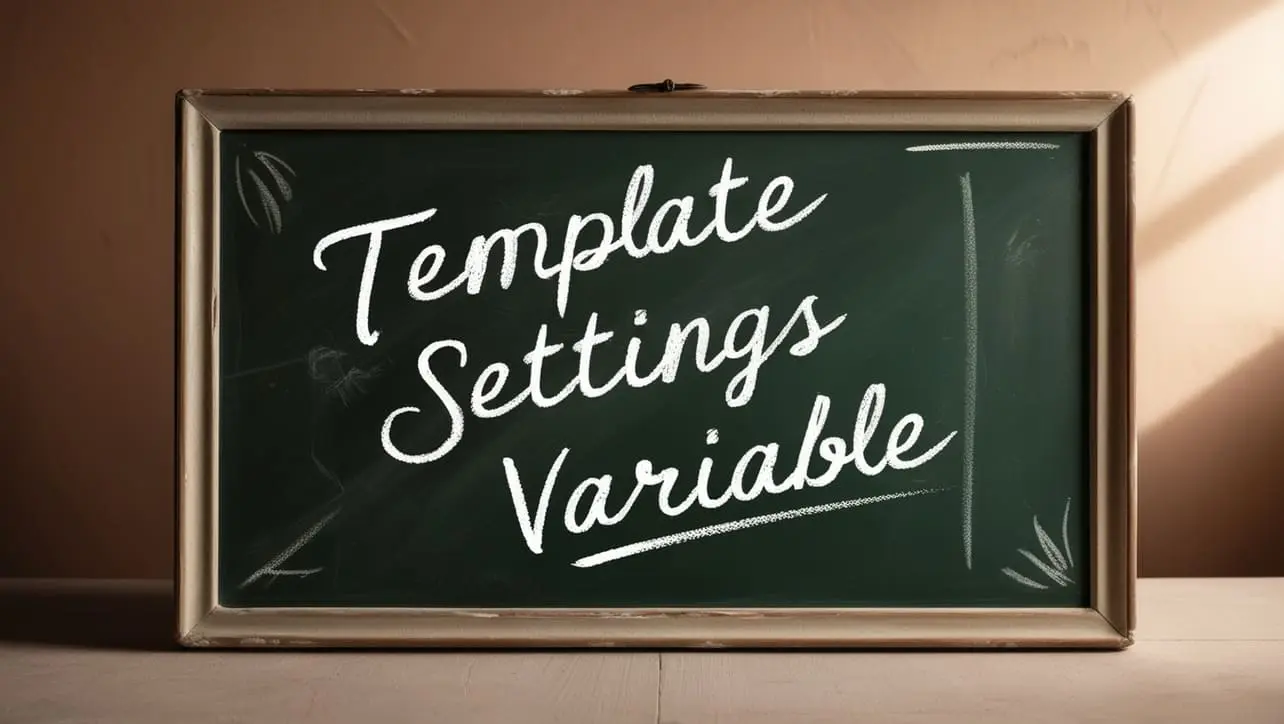
If you have any doubts regarding this article (Lodash _.size() Collection Method), please comment here. I will help you immediately.