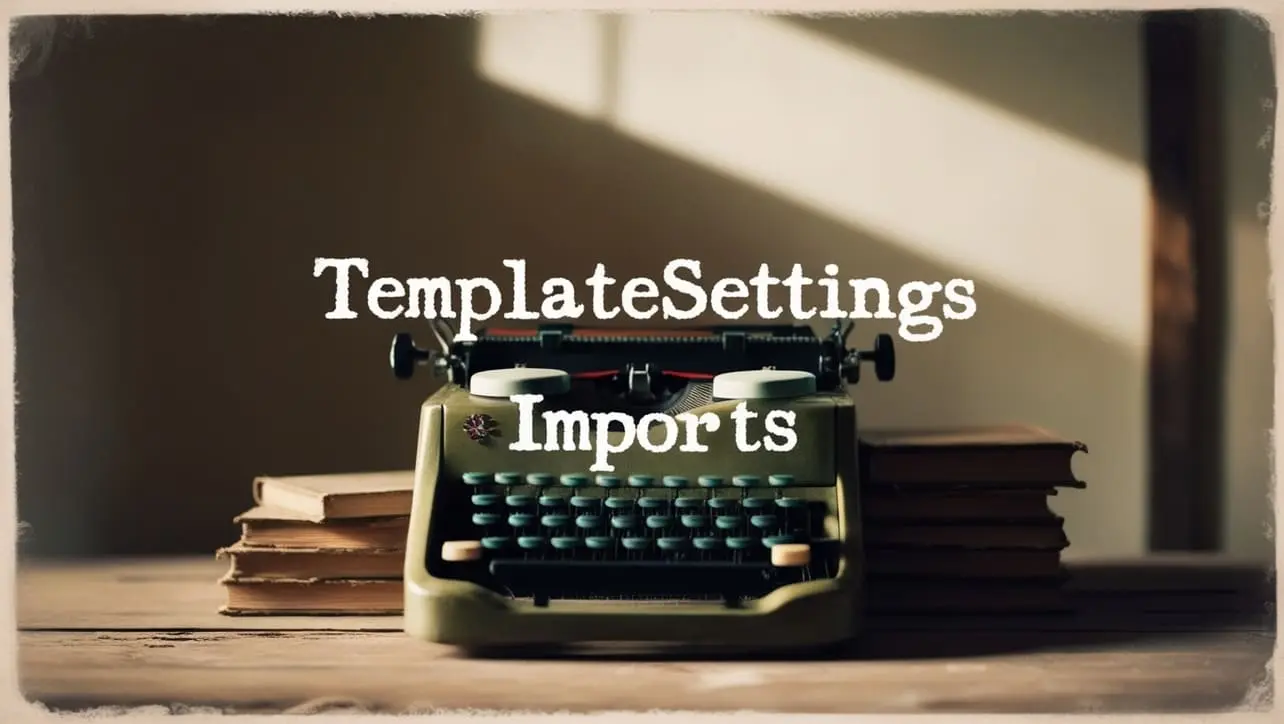
Lodash _.reject() Collection Method
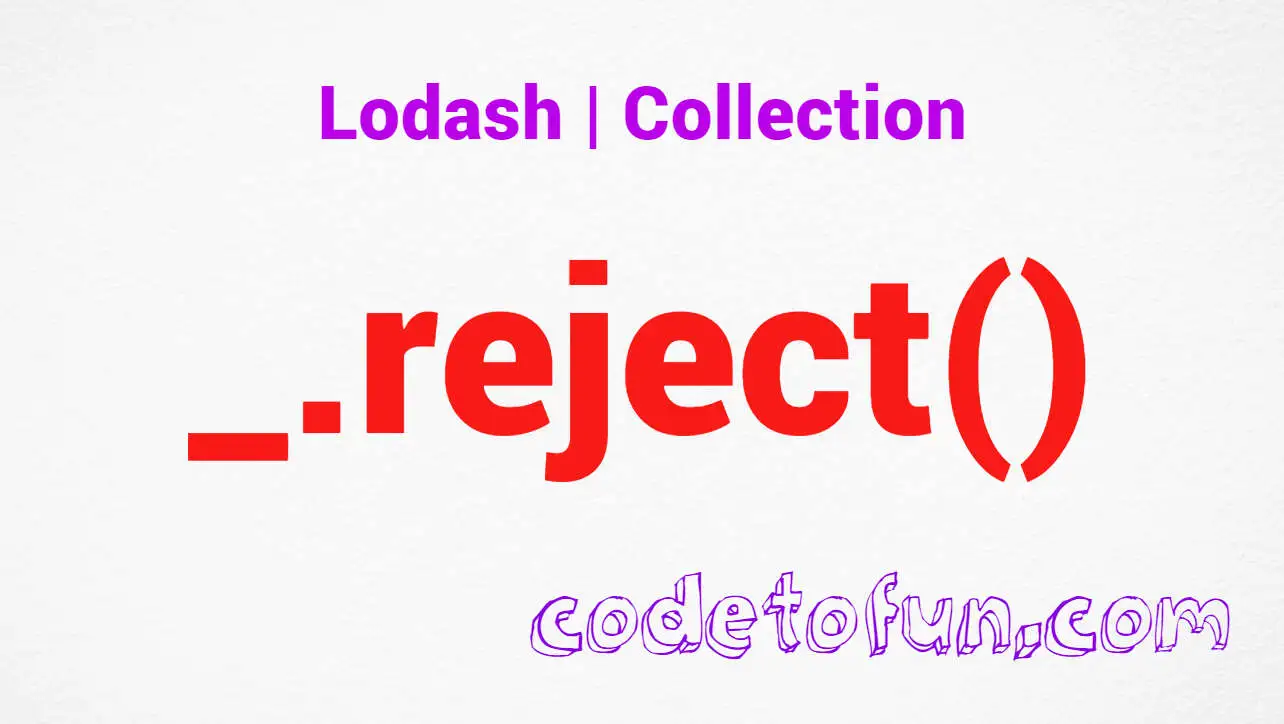
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript programming, effective handling of collections is essential. Lodash, a comprehensive utility library, offers a plethora of functions to streamline these operations. Among them, the _.reject()
method stands out as a powerful tool for selectively excluding elements from a collection based on a given predicate.
This method empowers developers to filter and manipulate data with ease, enhancing the flexibility and expressiveness of their code.
🧠 Understanding _.reject() Method
The _.reject()
method in Lodash provides a straightforward way to filter out elements from a collection that do not meet a specified condition. By utilizing a predicate function, developers can precisely define the criteria for exclusion, allowing for dynamic and customizable filtering.
💡 Syntax
The syntax for the _.reject()
method is straightforward:
_.reject(collection, predicate)
- collection: The collection to iterate over.
- predicate: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.reject()
method:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5, 6];
const rejectedNumbers = _.reject(numbers, n => n % 2 === 0);
console.log(rejectedNumbers);
// Output: [1, 3, 5]
In this example, the _.reject()
method filters out even numbers, creating a new array with only odd numbers.
🏆 Best Practices
When working with the _.reject()
method, consider the following best practices:
Understand the Predicate:
Ensure a clear understanding of the predicate function. The
_.reject()
method excludes elements for which the predicate returns true. Make the predicate concise and expressive for better code readability.example.jsCopiedconst people = [ { name: 'Alice', age: 25 }, { name: 'Bob', age: 30 }, { name: 'Charlie', age: 22 }, ]; const adults = _.reject(people, person => person.age < 18); console.log(adults); // Output: [{ name: 'Alice', age: 25 }, { name: 'Bob', age: 30 }, { name: 'Charlie', age: 22 }]
Preserve the Original Collection:
If preserving the original collection is essential, consider creating a shallow copy before applying
_.reject()
to avoid unintended mutations.example.jsCopiedconst originalArray = [10, 20, 30, 40, 50]; const newArray = _.reject([...originalArray], num => num > 30); console.log(originalArray); // Output: [10, 20, 30, 40, 50] console.log(newArray); // Output: [10, 20, 30]
Utilize Other Lodash Functions:
Combine
_.reject()
with other Lodash functions for more complex filtering or transformations. This allows you to create powerful data pipelines.example.jsCopiedconst data = /* ...fetch data from API or elsewhere... */; const filteredData = _.reject(_.map(data, 'value'), value => value < 0); console.log(filteredData);
📚 Use Cases
Filtering by Condition:
_.reject()
is particularly useful when you need to filter elements based on a condition. This is beneficial for excluding items that don't meet specific criteria.example.jsCopiedconst scores = [90, 85, 78, 95, 60, 88]; const passingScores = _.reject(scores, score => score < 70); console.log(passingScores); // Output: [90, 85, 78, 95, 88]
Data Cleanup:
In scenarios where data cleanup is required,
_.reject()
can be employed to remove unwanted or invalid entries from a collection.example.jsCopiedconst userProfiles = /* ...fetch user profiles... */; const validProfiles = _.reject(userProfiles, profile => !profile.isActive); console.log(validProfiles);
Dynamic Filtering:
Use
_.reject()
dynamically by accepting a predicate function as an argument. This provides a flexible solution for handling various filtering scenarios.example.jsCopiedconst books = /* ...fetch books data... */; function filterBooksByGenre(books, genre) { return _.reject(books, book => book.genre !== genre); } const fantasyBooks = filterBooksByGenre(books, 'Fantasy'); console.log(fantasyBooks);
🎉 Conclusion
The _.reject()
method in Lodash is a versatile tool for selectively filtering elements from a collection based on a given predicate. Whether you're cleaning up data, implementing dynamic filters, or excluding items by condition, this method provides an elegant and efficient solution for collection manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.reject()
method in your Lodash projects.
👨💻 Join our Community:
Author
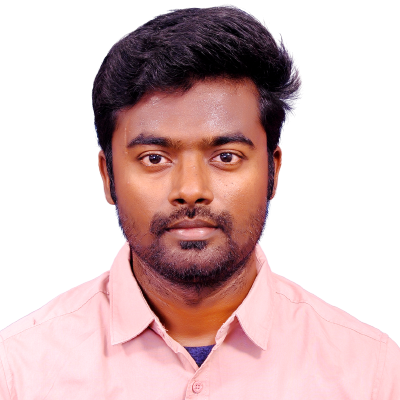
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
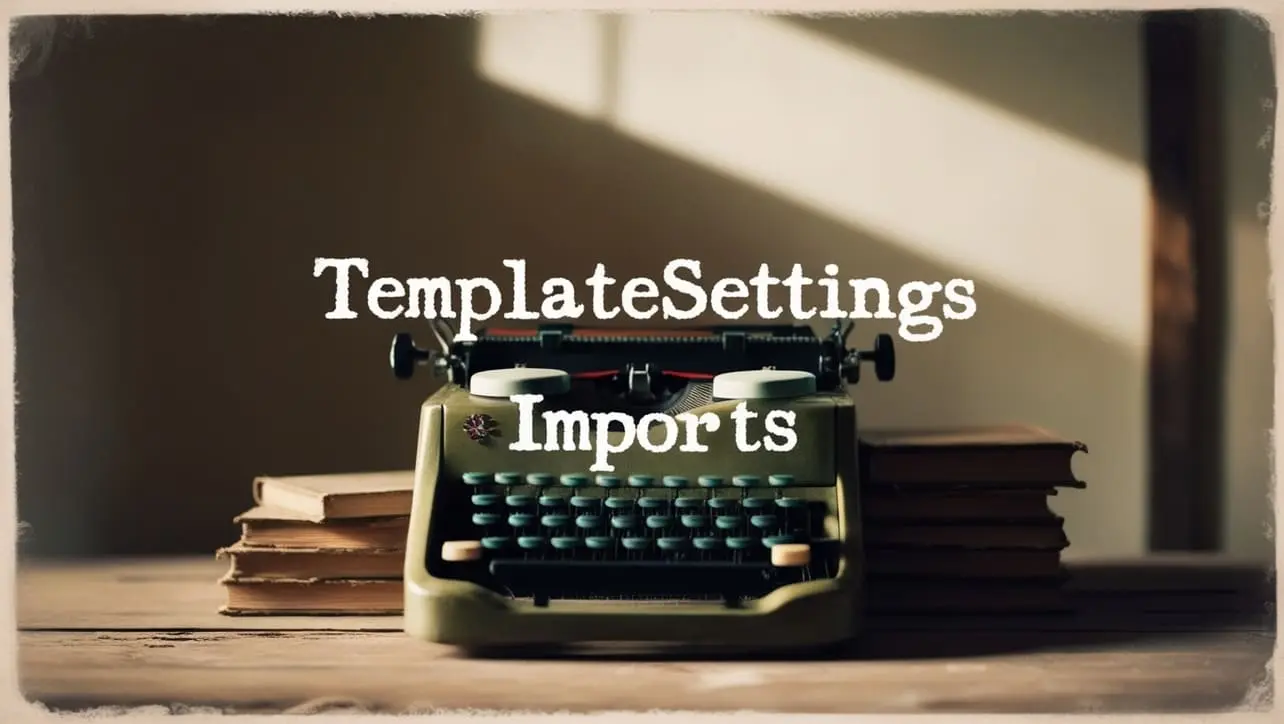
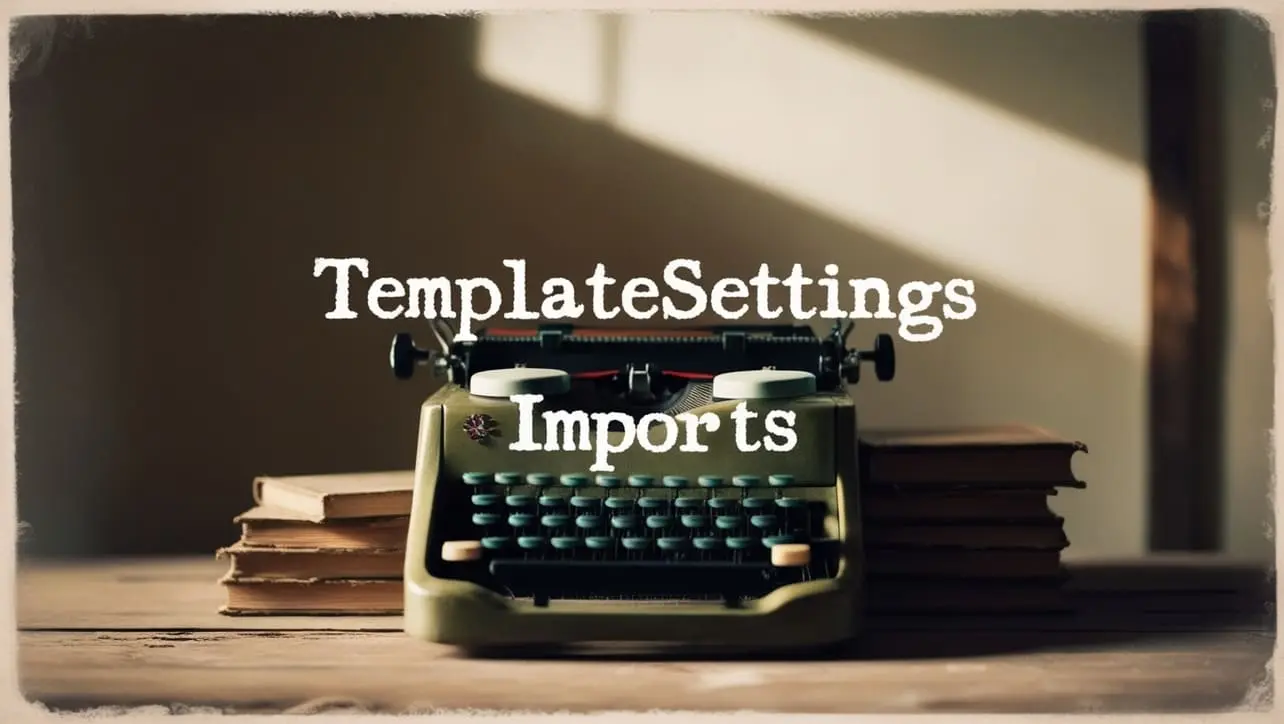
Lodash _.templateSettings.imports Property
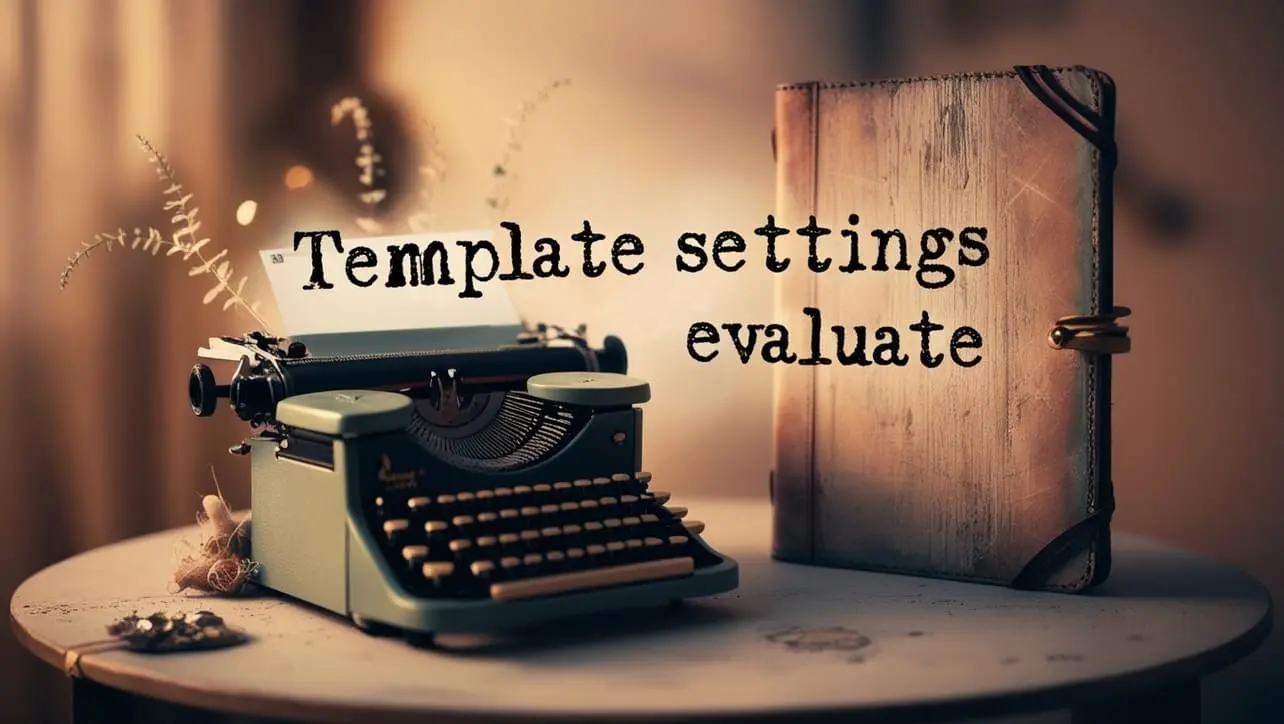
Lodash _.templateSettings.evaluate Property
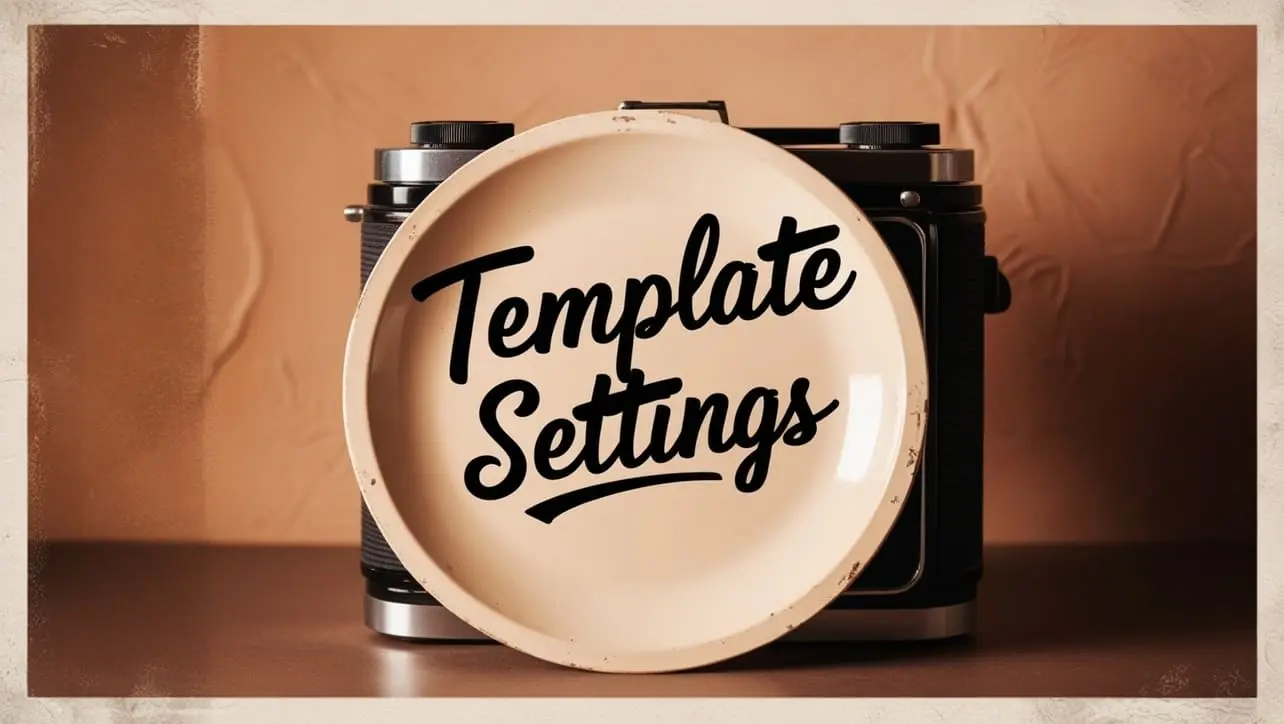
Lodash _.templateSettings Property
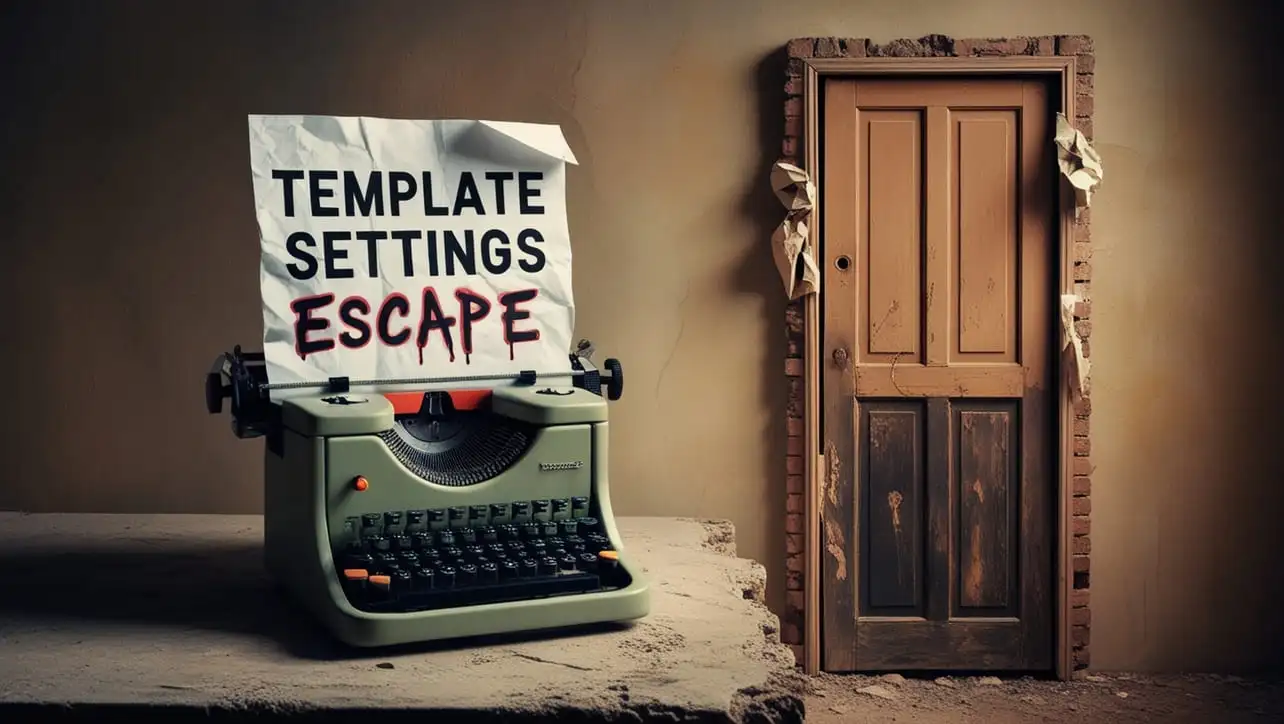
Lodash _.templateSettings.escape Property
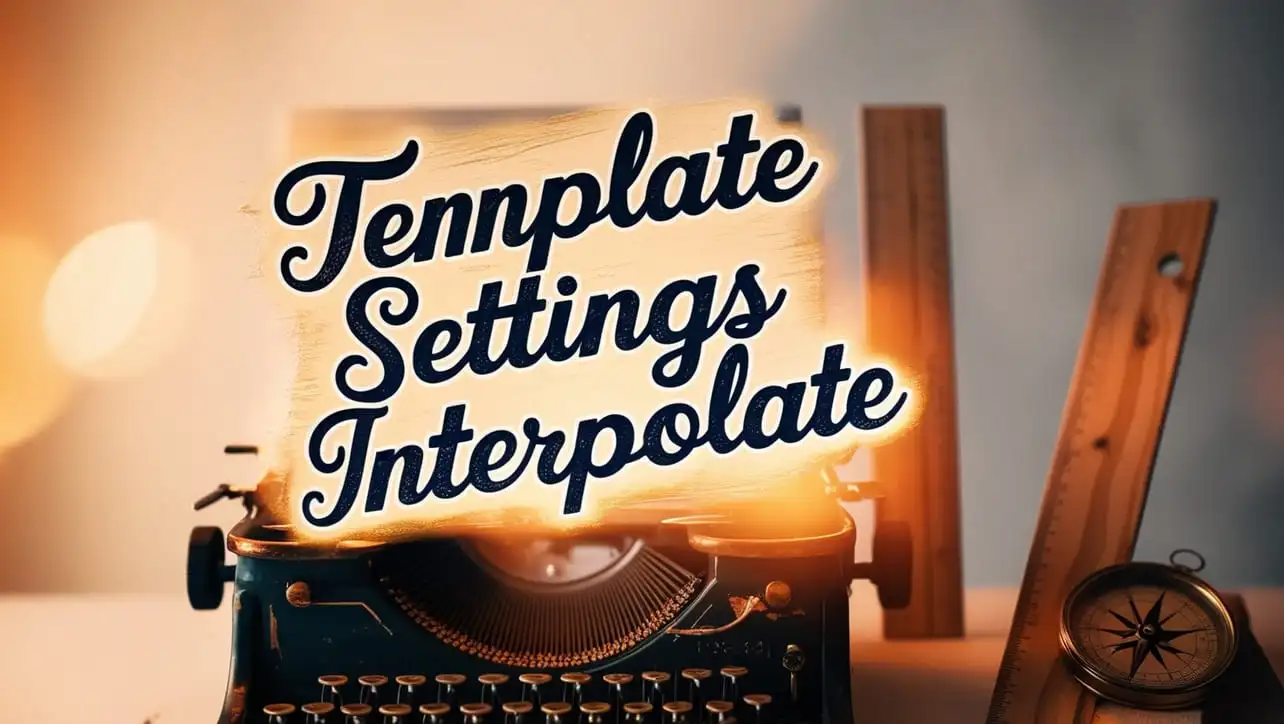
Lodash _.templateSettings.interpolate Property
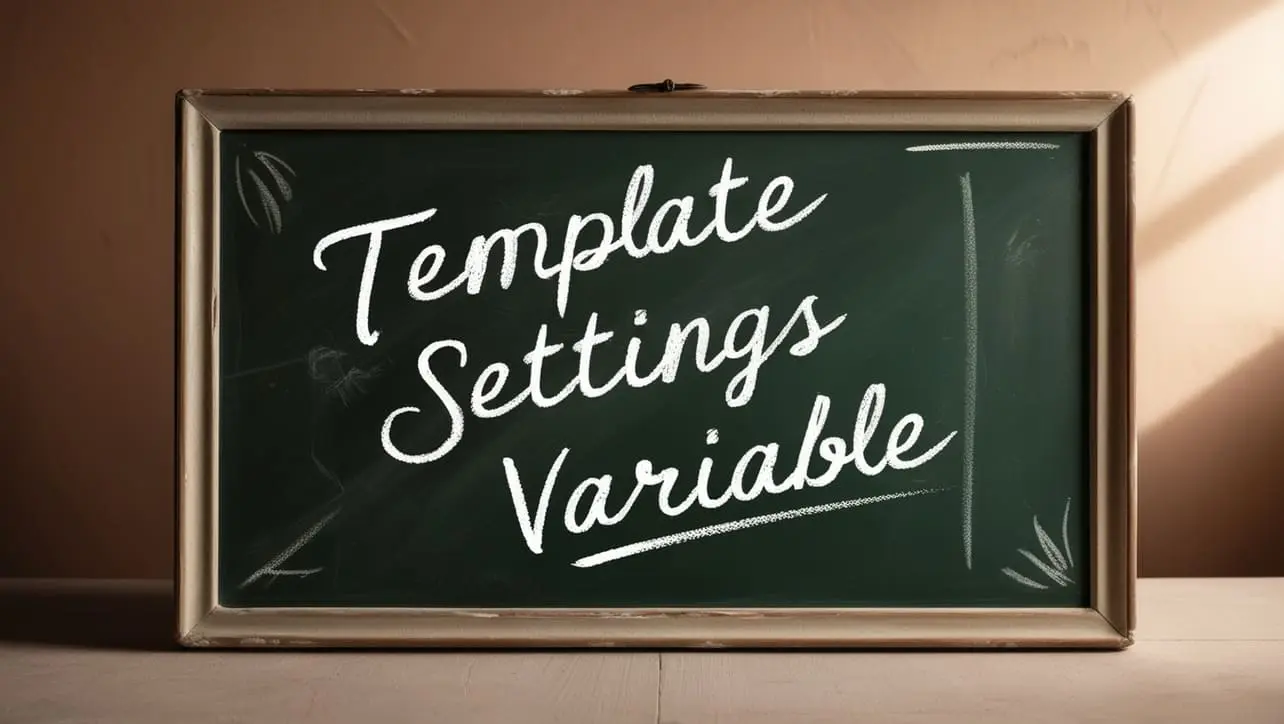
If you have any doubts regarding this article (Lodash _.reject() Collection Method), please comment here. I will help you immediately.