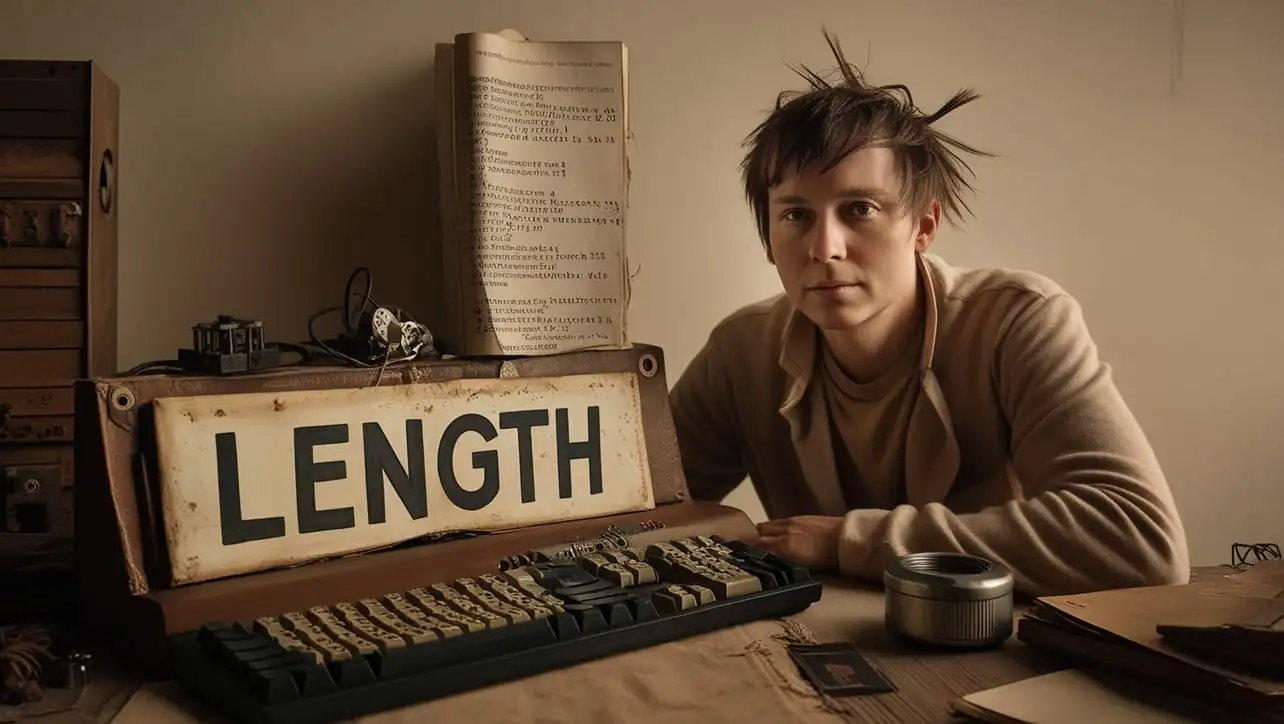
JS Date Methods
JavaScript Date toDateString() Method

Photo Credit to CodeToFun
🙋 Introduction
Working with dates in JavaScript becomes more seamless with the availability of various methods for formatting and extracting information. One such method is toDateString()
, which allows you to obtain a human-readable string representation of a date's portion excluding the time details.
In this guide, we'll explore the syntax, usage, best practices, and practical examples of the toDateString()
method.
🧠 Understanding toDateString() Method
The toDateString()
method is part of the JavaScript Date object, providing a simple way to obtain a string representation of the date portion of the object. This method returns a string representing the date portion in the format "ddd mmm dd yyyy," where:
- ddd: Day of the week (e.g., "Mon")
- mmm: Month (e.g., "Jan")
- dd: Day of the month (e.g., "01")
- yyyy: Year (e.g., "2022")
💡 Syntax
The syntax for the toDateString()
method is straightforward:
dateObj.toDateString();
- dateObj: The Date object for which you want to obtain the date string.
📝 Example
Let's explore a basic example to showcase the usage of the toDateString()
method:
// Creating a Date object
const today = new Date();
// Using toDateString() to obtain the date string
const dateString = today.toDateString();
console.log(dateString);
In this example, the toDateString()
method is called on a Date object (today), and the resulting date string is then logged to the console.
🏆 Best Practices
When working with the toDateString()
method, consider the following best practices:
Time Zone Awareness:
Be aware that the
toDateString()
method does not include time zone information. If time zone information is crucial, consider using other methods or libraries for handling time zones.example.jsCopiedconst timeZoneDateString = today.toLocaleDateString('en-US', { timeZone: 'UTC' }); console.log(timeZoneDateString);
Input Validation:
Ensure that the input to the
toDateString()
method is a valid Date object. Handling invalid dates can help prevent unexpected errors in your code.example.jsCopiedconst invalidDate = new Date('invalid'); if (!isNaN(invalidDate.getTime())) { const validDateString = invalidDate.toDateString(); console.log(validDateString); } else { console.error('Invalid date!'); }
📚 Use Cases
Displaying the Current Date:
A common use case for the
toDateString()
method is displaying the current date in a human-readable format:example.jsCopiedconst today = new Date(); const dateString = today.toDateString(); console.log(`Today's Date: ${dateString}`);
Creating a Custom Date Display:
You can leverage the
toDateString()
method to create a custom date display for your application:example.jsCopiedconst eventDate = new Date('2024-03-15'); const formattedDate = eventDate.toDateString(); console.log(`Event Date: ${formattedDate}`);
🎉 Conclusion
The toDateString()
method simplifies the process of obtaining a human-readable representation of the date portion of a Date object.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the toDateString()
method in your JavaScript projects.
👨💻 Join our Community:
Author
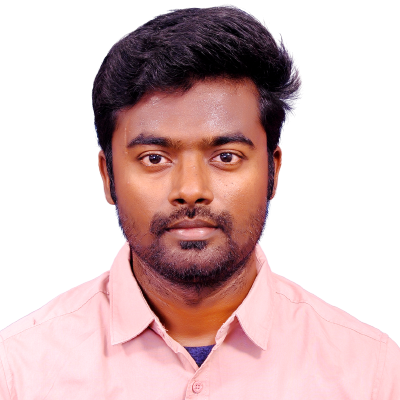
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date toDateString() Method), please comment here. I will help you immediately.