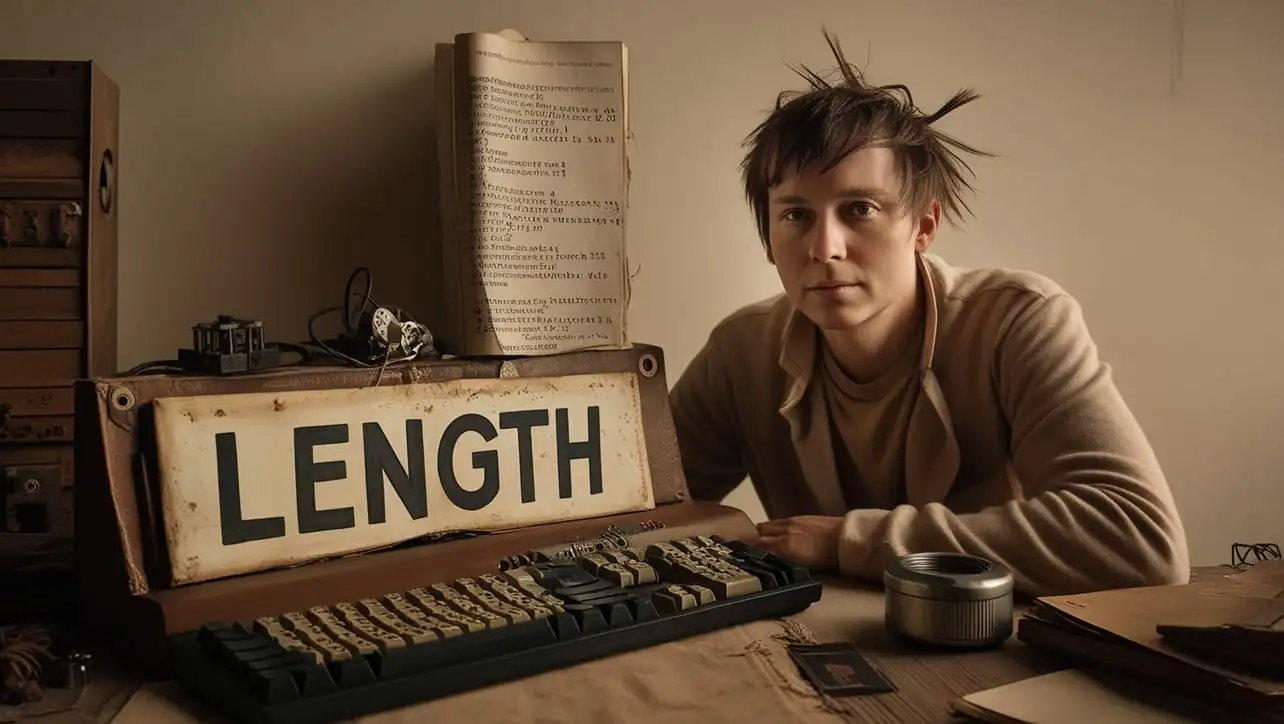
JS Date Methods
JavaScript Date setUTCMinutes() Method

Photo Credit to CodeToFun
🙋 Introduction
Working with dates in JavaScript is a common requirement, and the setUTCMinutes()
method provides a powerful tool for manipulating time components.
In this guide, we'll explore the setUTCMinutes()
method, unravel its syntax, usage, best practices, and delve into practical examples to showcase its capabilities.
🧠 Understanding setUTCMinutes() Method
The setUTCMinutes()
method is a part of the JavaScript Date object, allowing you to set the minutes of a date object based on Coordinated Universal Time (UTC). This method is particularly useful when you need to adjust the time portion of a date without affecting the date itself.
💡 Syntax
The syntax for the setUTCMinutes()
method is straightforward:
dateObject.setUTCMinutes(minutesValue[, secondsValue[, millisecondsValue]]);
- dateObject: The Date object you want to modify.
- minutesValue: The numeric value for the minutes (0 to 59).
- secondsValue: The numeric value for the seconds (0 to 59).
- millisecondsValue: The numeric value for the milliseconds (0 to 999).
📝 Example
Let's delve into a practical example to illustrate the usage of the setUTCMinutes()
method:
// Create a new Date object
let myDate = new Date('2024-02-26T12:30:45Z');
// Set the UTC minutes to 15
myDate.setUTCMinutes(15);
console.log(myDate.toISOString());
In this example, the setUTCMinutes()
method is used to set the minutes of the myDate object to 15.
🏆 Best Practices
When working with the setUTCMinutes()
method, consider the following best practices:
Input Validation:
Ensure that the input values for minutes, seconds, and milliseconds are within the valid range to avoid unexpected behavior.
example.jsCopiedconst newMinutes = 45; const newSeconds = 30; const newMilliseconds = 500; if ( newMinutes >= 0 && newMinutes <= 59 && newSeconds >= 0 && newSeconds <= 59 && newMilliseconds >= 0 && newMilliseconds <= 999 ) { myDate.setUTCMinutes(newMinutes, newSeconds, newMilliseconds); } else { console.error('Invalid input values.'); }
Chaining:
Take advantage of method chaining to perform multiple date modifications in a concise manner.
example.jsCopiedmyDate.setUTCMinutes(15).setUTCSeconds(30).setUTCMilliseconds(500);
📚 Use Cases
Adjusting Time Components:
The primary use case for
setUTCMinutes()
is adjusting the minutes component of a date object:example.jsCopied// Increment the minutes by 10 myDate.setUTCMinutes(myDate.getUTCMinutes() + 10);
Setting Precise Timestamps:
When you need to set a precise timestamp, including minutes, seconds, and milliseconds:
example.jsCopiedmyDate.setUTCMinutes(45, 30, 500);
🎉 Conclusion
The setUTCMinutes()
method empowers you to precisely manipulate the minutes component of a JavaScript Date object, facilitating robust date and time operations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setUTCMinutes()
method in your JavaScript projects.
👨💻 Join our Community:
Author
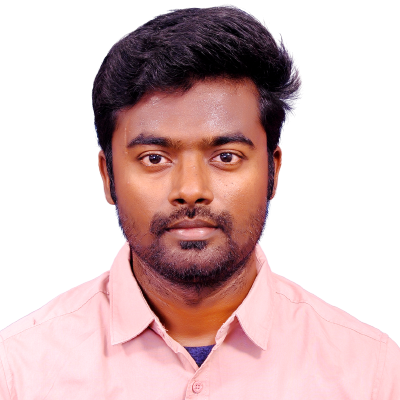
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setUTCMinutes() Method), please comment here. I will help you immediately.