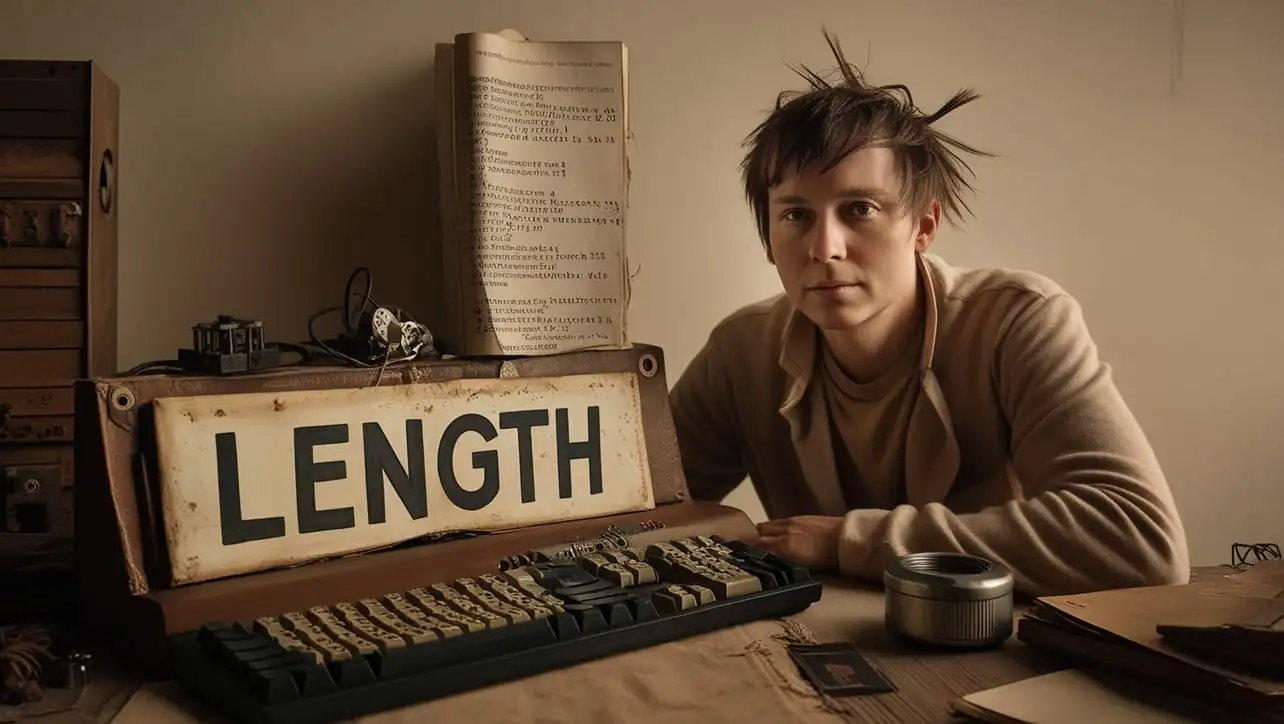
JS Date Methods
JavaScript Date setMinutes() Method

Photo Credit to CodeToFun
🙋 Introduction
Manipulating dates and times is a common requirement in programming, and JavaScript provides a rich set of methods to facilitate these operations. The setMinutes()
method is one such tool, allowing you to set the minutes of a Date object.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the setMinutes()
method.
🧠 Understanding setMinutes() Method
The setMinutes()
method is used to set the minutes for a specific Date object. This method is particularly useful when you need to adjust the time component of a date without altering other parts, such as the day or month.
💡 Syntax
The syntax for the setMinutes()
method is straightforward:
dateObj.setMinutes(minutesValue[, secondsValue[, msValue]]);
- dateObj: The Date object for which you want to set the minutes.
- minutesValue: An integer representing the minutes.
- secondsValue (optional): An integer representing the seconds (default is 0).
- msValue (optional): An integer representing the milliseconds (default is 0).
📝 Example
Let's dive into a simple example to illustrate the usage of the setMinutes()
method:
// Creating a Date object for the current date and time
const currentDate = new Date();
// Setting the minutes to 30
currentDate.setMinutes(30);
console.log(currentDate);
In this example, the setMinutes()
method is used to set the minutes of the currentDate object to 30.
🏆 Best Practices
When working with the setMinutes()
method, consider the following best practices:
Immutable Approach:
Remember that the
setMinutes()
method does not modify the original Date object but returns a new Date object with the updated value. Always capture the result to reflect changes.example.jsCopiedconst originalDate = new Date(); const updatedDate = new Date(originalDate.setMinutes(45));
Validating Input:
Ensure that the values provided for minutes, seconds, and milliseconds are within the valid ranges to avoid unexpected behavior.
example.jsCopiedconst minutesValue = 30; const secondsValue = 45; const msValue = 500; if ( minutesValue >= 0 && minutesValue < 60 && secondsValue >= 0 && secondsValue < 60 && msValue >= 0 && msValue < 1000 ) { const updatedDate = new Date().setMinutes(minutesValue, secondsValue, msValue); console.log(updatedDate); } else { console.error('Invalid input values.'); }
📚 Use Cases
Scheduling a Future Time:
The
setMinutes()
method can be used to schedule a future time by adjusting the minutes:example.jsCopiedconst currentDate = new Date(); const futureDate = new Date(currentDate.setMinutes(currentDate.getMinutes() + 15)); console.log(`Meeting scheduled for: ${futureDate}`);
Resetting Time to the Beginning of the Hour:
You can reset the minutes and seconds to the beginning of the hour:
example.jsCopiedconst currentDate = new Date(); const startOfHour = new Date(currentDate.setMinutes(0, 0)); console.log(`Time reset to the beginning of the hour: ${startOfHour}`);
🎉 Conclusion
The setMinutes()
method in JavaScript empowers you to manipulate dates with precision, specifically adjusting the minutes component of a Date object.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setMinutes()
method in your JavaScript projects.
👨💻 Join our Community:
Author
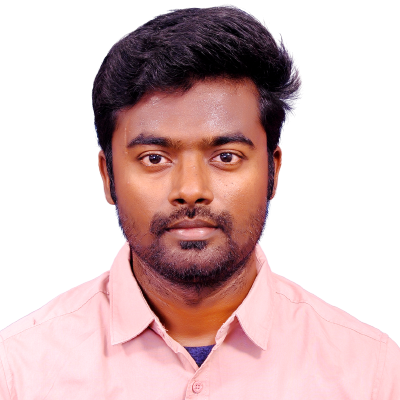
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setMinutes() Method), please comment here. I will help you immediately.