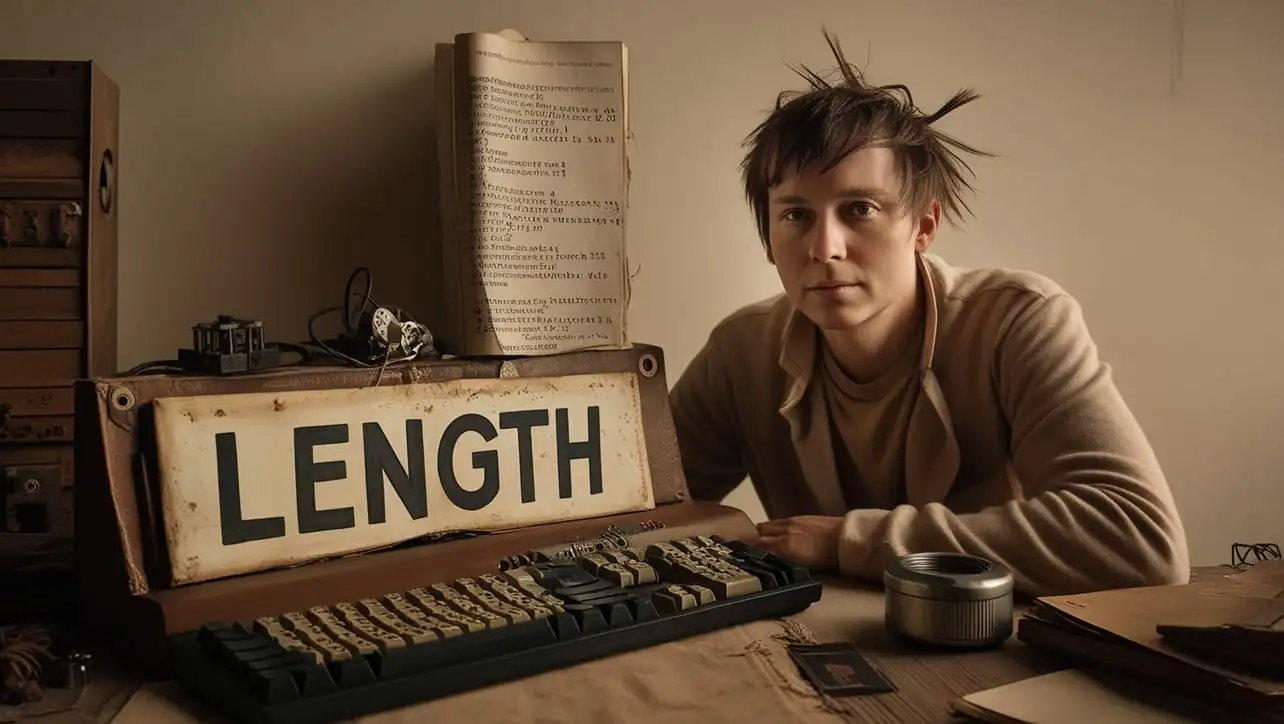
JS Date Methods
JavaScript Date getUTCMonth() Method

Photo Credit to CodeToFun
🙋 Introduction
Working with dates in JavaScript is a common task, and the getUTCMonth()
method provides a straightforward way to retrieve the month from a Date object in Coordinated Universal Time (UTC).
In this guide, we'll delve into the getUTCMonth()
method, exploring its syntax, usage, best practices, and practical examples.
🧠 Understanding getUTCMonth() Method
The getUTCMonth()
method is part of the Date object in JavaScript, and it returns the month of a date as an integer between 0 and 11, where 0 represents January and 11 represents December. Importantly, this method operates in UTC, ensuring consistency regardless of the user's local time zone.
💡 Syntax
The syntax for the getUTCMonth()
method is straightforward:
dateObject.getUTCMonth();
- dateObject: The Date object from which you want to retrieve the month.
📝 Example
Let's explore a basic example to illustrate the usage of the getUTCMonth()
method:
// Creating a Date object for March 15, 2022, in UTC
const utcDate = new Date(Date.UTC(2022, 2, 15));
// Using getUTCMonth() to retrieve the month
const month = utcDate.getUTCMonth();
console.log(`The month is: ${month}`); // Output: The month is: 2 (March is represented by 2)
In this example, the getUTCMonth()
method is used to extract the month from a Date object representing March 15, 2022, in UTC.
🏆 Best Practices
When working with the getUTCMonth()
method, consider the following best practices:
Handling Months Representation:
Remember that months are zero-indexed, so January is 0, February is 1, and so on. Adjust your logic accordingly when using the result of
getUTCMonth()
.example.jsCopiedconst month = utcDate.getUTCMonth() + 1; // Adding 1 to convert to standard month representation
Error Handling:
Ensure that the Date object is valid to avoid unexpected results. Invalid dates may lead to an incorrect month value.
example.jsCopiedif (!isNaN(utcDate)) { const month = utcDate.getUTCMonth(); console.log(`The month is: ${month}`); } else { console.error('Invalid Date object.'); }
📚 Use Cases
Displaying the Current Month:
A common use case is to display the current month in a user interface. The
getUTCMonth()
method, when combined with proper UI rendering, can achieve this easily.example.jsCopiedconst currentDate = new Date(); const currentMonth = currentDate.getUTCMonth(); console.log(`Current month: ${currentMonth}`);
Switching Between UTC and Local Time:
The
getUTCMonth()
method is particularly useful when you need to work with dates in a consistent, timezone-independent manner. Use it in conjunction with other Date methods for comprehensive date handling.example.jsCopiedconst localDate = new Date(); // Represents the current local time const utcMonth = localDate.getUTCMonth(); console.log(`UTC month: ${utcMonth}`);
🎉 Conclusion
The getUTCMonth()
method is a valuable tool for extracting month information from Date objects in a UTC context.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getUTCMonth()
method in your JavaScript projects.
👨💻 Join our Community:
Author
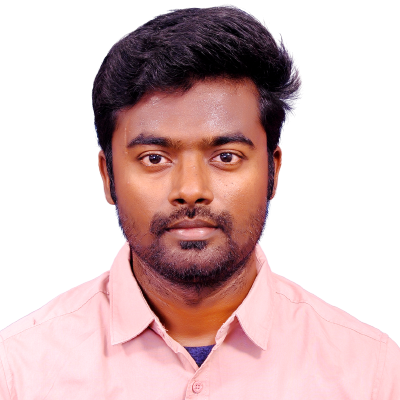
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getUTCMonth() Method), please comment here. I will help you immediately.