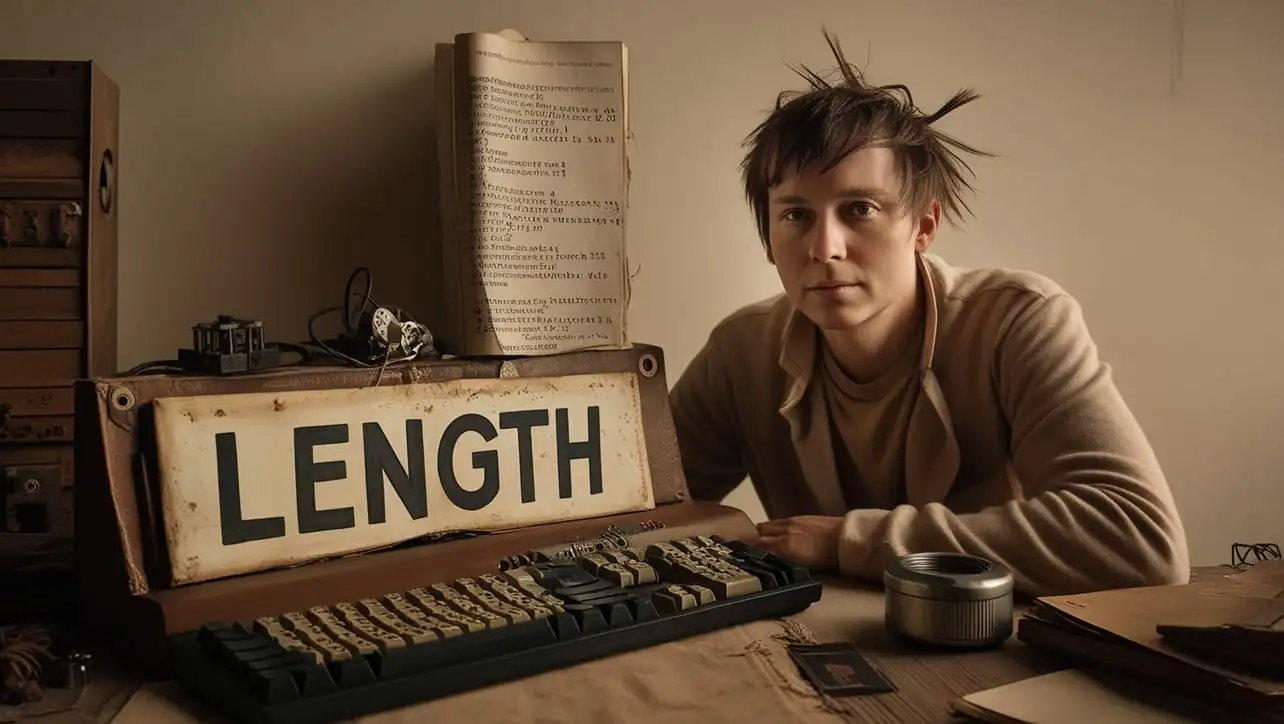
JS Date Methods
JavaScript Date getUTCDay() Methods

Photo Credit to CodeToFun
🙋 Introduction
Working with dates and times is a common requirement in programming, and JavaScript provides a variety of methods for handling them. The getUTCDay()
method is particularly useful when you need to extract the day of the week from a Date object in Coordinated Universal Time (UTC).
In this guide, we'll explore the getUTCDay()
method, its syntax, usage, best practices, and practical examples.
🧠 Understanding getUTCDay() Method
The getUTCDay()
method is used to retrieve the day of the week from a Date object in UTC. It returns a number representing the day of the week, where Sunday is 0, Monday is 1, and so on.
💡 Syntax
The syntax for the getUTCDay()
method is straightforward:
dateObj.getUTCDay();
- dateObj: The Date object from which you want to extract the day of the week.
📝 Example
Let's dive into a simple example to illustrate the usage of the getUTCDay()
method:
// Creating a Date object for February 26, 2024, UTC
const currentDate = new Date(Date.UTC(2024, 1, 26));
// Using getUTCDay() to retrieve the day of the week
const dayOfWeek = currentDate.getUTCDay();
console.log(`Day of the week (UTC): ${dayOfWeek}`);
In this example, the getUTCDay()
method is applied to a Date object representing February 26, 2024, in Coordinated Universal Time.
🏆 Best Practices
When working with the getUTCDay()
method, consider the following best practices:
Understanding Indexing:
Be aware that the days of the week are represented by numbers starting from 0 (Sunday) to 6 (Saturday).
example.jsCopiedconst dayIndex = currentDate.getUTCDay(); switch (dayIndex) { case 0: console.log('Sunday'); break; case 1: console.log('Monday'); break; // ... Continue for the remaining days }
Adjusting for Your Locale:
Keep in mind that the representation of the first day of the week might vary in different locales. Ensure you are considering the appropriate starting day for your application.
📚 Use Cases
Displaying Day of the Week:
The primary use case for
getUTCDay()
is to display or work with the day of the week in a human-readable format:example.jsCopiedconst daysOfWeek = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']; const dayIndex = currentDate.getUTCDay(); const dayName = daysOfWeek[dayIndex]; console.log(`Day of the week: ${dayName}`);
Conditional Operations Based on Day:
You can use the
getUTCDay()
method to perform conditional operations based on the day of the week:example.jsCopiedconst dayIndex = currentDate.getUTCDay(); if (dayIndex === 0 || dayIndex === 6) { console.log('It\'s the weekend!'); } else { console.log('It\'s a weekday.'); }
🎉 Conclusion
The getUTCDay()
method is a valuable tool for extracting the day of the week from Date objects in Coordinated Universal Time.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getUTCDay()
method in your JavaScript projects.
👨💻 Join our Community:
Author
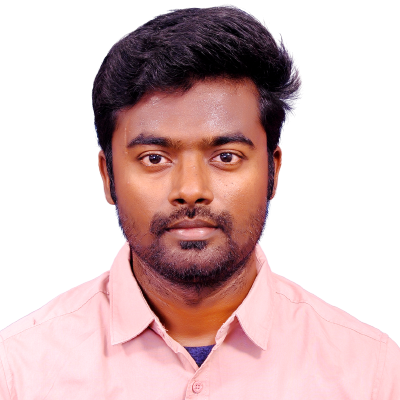
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getUTCDay() Methods), please comment here. I will help you immediately.