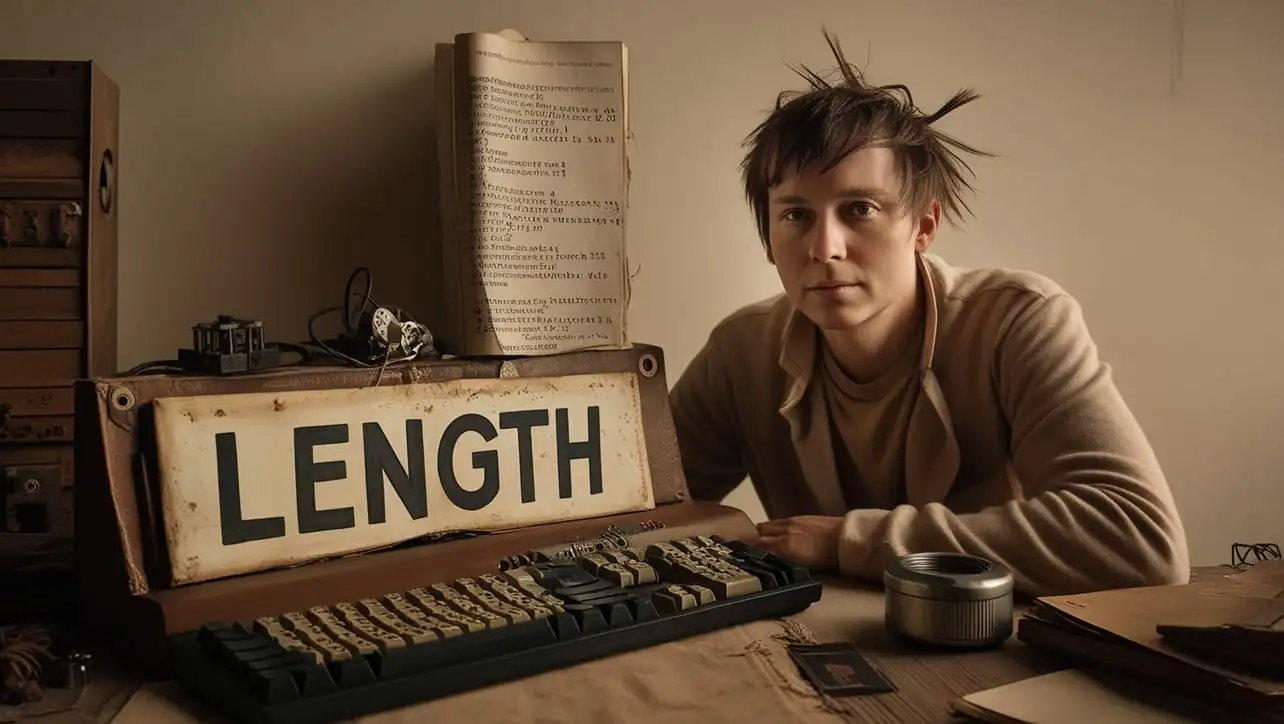
JS Date Methods
JavaScript Date getTimezoneOffset() Methods

Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript, handling dates and times across different time zones is a common challenge. The getTimezoneOffset()
method for Date objects comes to the rescue, providing a way to retrieve the time zone offset in minutes.
This guide will explore the syntax, usage, best practices, and practical applications of the getTimezoneOffset()
method.
🧠 Understanding getTimezoneOffset() Method
The getTimezoneOffset()
method is a valuable tool for obtaining the time zone offset, representing the difference between Coordinated Universal Time (UTC) and the local time in minutes. This information is crucial when working with dates and times in a global context.
💡 Syntax
The syntax for the getTimezoneOffset()
method is straightforward:
const offsetInMinutes = date.getTimezoneOffset();
- date: The Date object for which you want to retrieve the time zone offset.
📝 Example
Let's dive into an example to illustrate the usage of the getTimezoneOffset()
method:
// Create a Date object
const now = new Date();
// Get the time zone offset in minutes
const offsetInMinutes = now.getTimezoneOffset();
console.log(`Current time zone offset: ${offsetInMinutes} minutes`);
In this example, the getTimezoneOffset()
method is called on the current date, providing the time zone offset in minutes.
🏆 Best Practices
When working with the getTimezoneOffset()
method, consider the following best practices:
Use Negative Values:
The returned offset is positive if the local time zone is behind UTC and negative if it is ahead. Be mindful of this when interpreting the result.
example.jsCopiedconst offset = date.getTimezoneOffset(); const isAheadOfUTC = offset < 0; console.log(`Is ahead of UTC: ${isAheadOfUTC}`);
Convert to Hours:
For better readability, you can convert the offset from minutes to hours.
example.jsCopiedconst offsetInHours = date.getTimezoneOffset() / 60; console.log(`Time zone offset in hours: ${offsetInHours}`);
Consider Daylight Saving Time (DST):
Be aware that the offset may change during DST transitions, affecting the local time zone.
📚 Use Cases
International Date Formatting:
When displaying dates on a website or application, knowing the time zone offset is crucial for presenting accurate information to users around the world.
example.jsCopiedconst userDate = new Date(); // Assume this is obtained from user input const userOffset = userDate.getTimezoneOffset(); // Display the date in the user's local time const localDate = new Date(userDate.getTime() - userOffset * 60000); console.log(`Formatted Date: ${localDate.toLocaleString()}`);
Scheduling Events Across Time Zones:
When scheduling events or appointments, understanding the time zone offset helps in coordinating activities with participants in different regions.
example.jsCopiedconst eventDate = new Date('2024-03-01T12:00:00Z'); // Assuming a UTC time const participantOffset = -300; // Offset for a participant in UTC-5 // Adjust the event date for the participant's time zone const participantDate = new Date(eventDate.getTime() - participantOffset * 60000); console.log(`Participant's local time: ${participantDate.toLocaleString()}`);
🎉 Conclusion
The getTimezoneOffset()
method proves to be an indispensable asset when dealing with time zones in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getTimezoneOffset()
method in your JavaScript projects.
👨💻 Join our Community:
Author
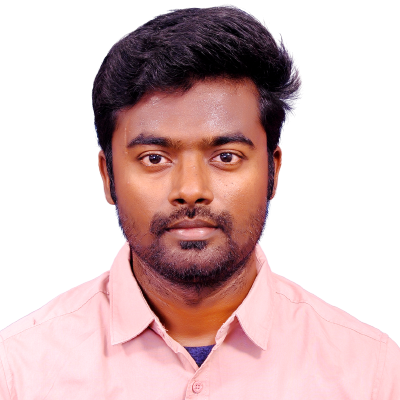
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getTimezoneOffset() Methods), please comment here. I will help you immediately.