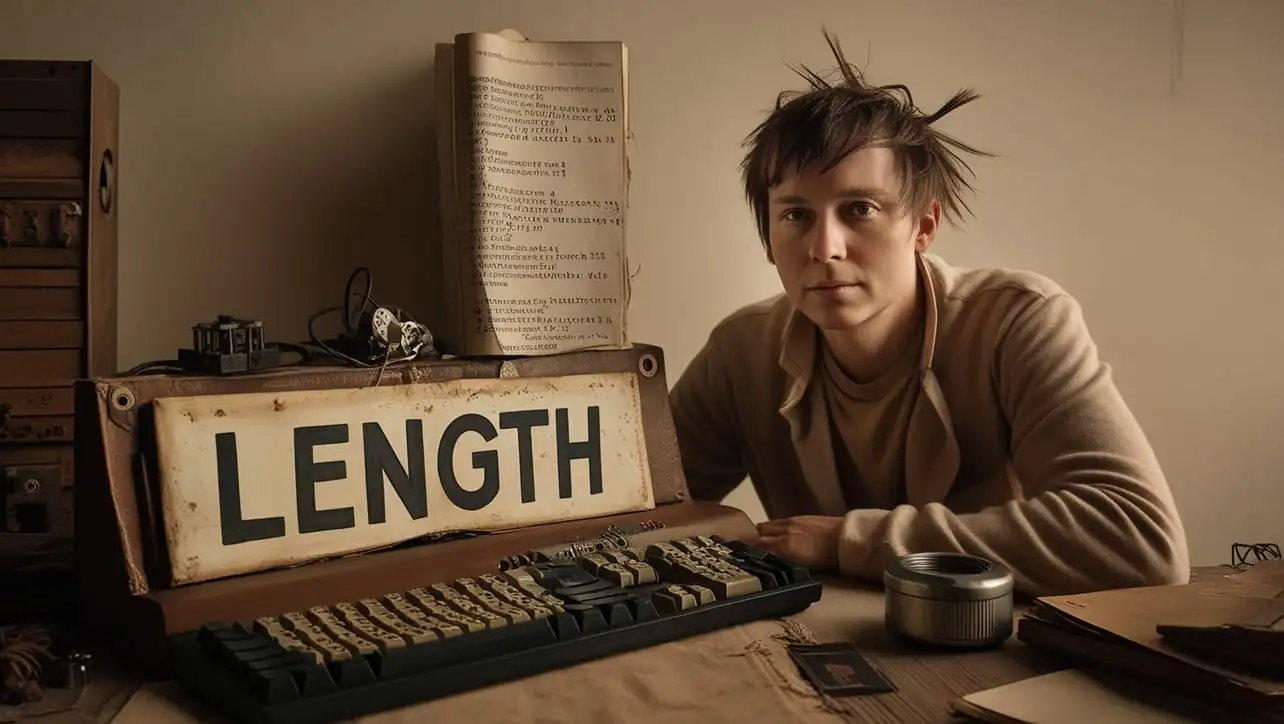
JS Date Methods
JavaScript Date getTime() Methods

Photo Credit to CodeToFun
🙋 Introduction
Working with dates and time in JavaScript is a common requirement in many applications. The getTime()
method is a powerful tool that simplifies the process of obtaining the timestamp of a Date object.
In this guide, we'll explore the getTime()
method, understand its syntax, examine its usage, and discuss best practices and practical examples.
🧠 Understanding getTime() Method
The getTime()
method is a part of the JavaScript Date object, and it returns the numeric value corresponding to the time for the specified date according to universal time. This numeric value represents the number of milliseconds since the epoch (January 1, 1970, 00:00:00 UTC).
💡 Syntax
The syntax for the getTime()
method is straightforward:
const timestamp = date.getTime();
- date: The Date object for which you want to retrieve the timestamp.
📝 Example
Let's explore a simple example to illustrate the usage of the getTime()
method:
// Create a Date object for the current date and time
const currentDate = new Date();
// Get the timestamp using getTime()
const timestamp = currentDate.getTime();
console.log(timestamp); // Output: Numeric value representing current time in milliseconds
In this example, we create a Date object for the current date and time, and then use getTime()
to obtain its timestamp.
🏆 Best Practices
When working with the getTime()
method, consider the following best practices:
Storing Timestamps:
If you need to perform multiple operations with the timestamp, store it in a variable to avoid repeatedly calling
getTime()
.example.jsCopiedconst currentDate = new Date(); const timestamp = currentDate.getTime(); // Perform operations using timestamp // ... // Later in the code, you can still use the stored timestamp console.log(timestamp);
Compatibility Checking:
The
getTime()
method is widely supported, but always check for compatibility in your target environments, especially if dealing with older browsers.example.jsCopied// Check if the getTime() method is supported if (Date.prototype.getTime) { // Use the method safely const currentDate = new Date(); const timestamp = currentDate.getTime(); console.log(timestamp); } else { console.error('getTime() method not supported in this environment.'); }
📚 Use Cases
Calculating Time Differences:
The
getTime()
method is useful when calculating the difference between two dates:example.jsCopiedconst startDate = new Date('2022-01-01'); const endDate = new Date('2022-02-01'); const timeDifference = endDate.getTime() - startDate.getTime(); console.log(`Time difference in milliseconds: ${timeDifference}`);
Converting Timestamps:
You can convert a timestamp to a Date object using the Date constructor:
example.jsCopiedconst timestamp = 1643520000000; // Assuming a specific timestamp const dateFromTimestamp = new Date(timestamp); console.log(dateFromTimestamp);
🎉 Conclusion
The getTime()
method in JavaScript provides a straightforward way to obtain the timestamp of a Date object.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getTime()
method in your JavaScript projects.
👨💻 Join our Community:
Author
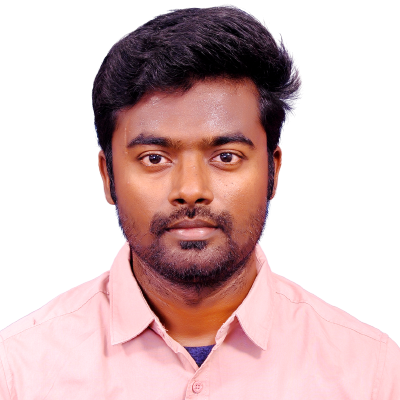
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getTime() Methods), please comment here. I will help you immediately.