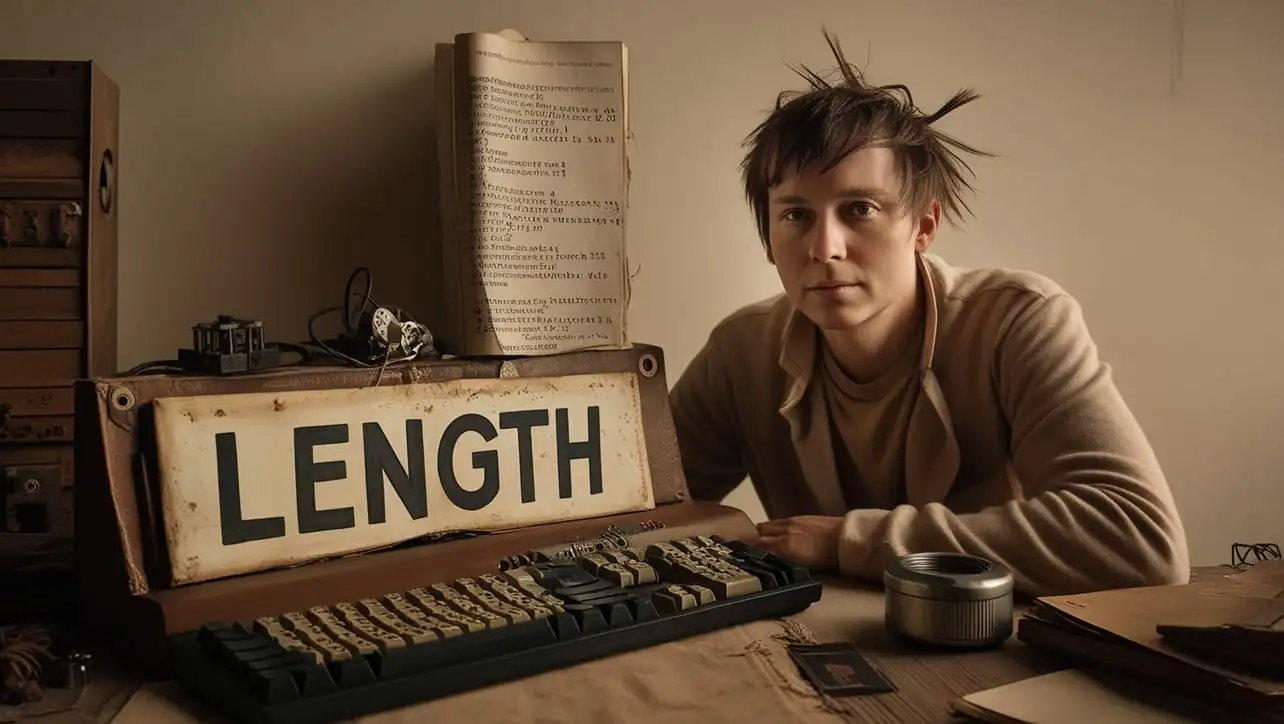
JS Date Methods
JavaScript Date getMilliseconds() Methods

Photo Credit to CodeToFun
🙋 Introduction
Working with dates is a crucial aspect of many JavaScript applications, and the getMilliseconds()
method provides a handy way to extract milliseconds from a Date object.
In this guide, we'll explore the getMilliseconds()
method, understand its syntax, and learn how to effectively use it in various scenarios.
🧠 Understanding getMilliseconds() Method
The getMilliseconds()
method is a part of the JavaScript Date object and is used to retrieve the milliseconds component of a date. It returns a value between 0 and 999, representing the milliseconds portion of the specified date.
💡 Syntax
The syntax for the getMilliseconds()
method is straightforward:
dateObj.getMilliseconds();
- dateObj: The Date object from which you want to extract the milliseconds.
📝 Example
Let's delve into a practical example to illustrate the usage of the getMilliseconds()
method:
// Creating a new Date object with the current date and time
const currentDate = new Date();
// Using getMilliseconds() to obtain the milliseconds
const milliseconds = currentDate.getMilliseconds();
console.log(`Milliseconds: ${milliseconds}`);
In this example, the getMilliseconds()
method is applied to a Date object to fetch the milliseconds portion.
🏆 Best Practices
When working with the getMilliseconds()
method, consider the following best practices:
Ensure Valid Date Object:
Verify that the object on which
getMilliseconds()
is called is a valid Date object to avoid unexpected results.example.jsCopiedconst potentialDate = new Date(); if (potentialDate instanceof Date && !isNaN(potentialDate)) { const milliseconds = potentialDate.getMilliseconds(); console.log(`Milliseconds: ${milliseconds}`); } else { console.error('Invalid Date object.'); }
UTC vs. Local Time:
Be aware of whether you want to retrieve milliseconds in UTC or local time, and adjust your Date object accordingly.
example.jsCopiedconst utcMilliseconds = currentDate.getUTCMilliseconds(); console.log(`UTC Milliseconds: ${utcMilliseconds}`);
📚 Use Cases
Precise Timing in Applications:
The
getMilliseconds()
method is particularly useful in scenarios where precise timing is crucial, such as measuring the execution time of a function or tracking intervals between events.example.jsCopied// Record the start time const startTime = new Date(); // Perform some time-consuming operation // ... // Record the end time const endTime = new Date(); // Calculate and log the elapsed time in milliseconds const elapsedMilliseconds = endTime - startTime; console.log(`Elapsed Time: ${elapsedMilliseconds} milliseconds`);
Animation and Multimedia Applications:
In applications involving animations or multimedia, the
getMilliseconds()
method can be employed to synchronize events with high precision.example.jsCopiedfunction animate() { const currentTime = new Date(); const milliseconds = currentTime.getMilliseconds(); // Perform animation logic based on milliseconds // ... requestAnimationFrame(animate); } animate();
🎉 Conclusion
The getMilliseconds()
method is a valuable tool when dealing with Date objects in JavaScript, allowing you to extract the milliseconds component with ease.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getMilliseconds()
method in your JavaScript projects.
👨💻 Join our Community:
Author
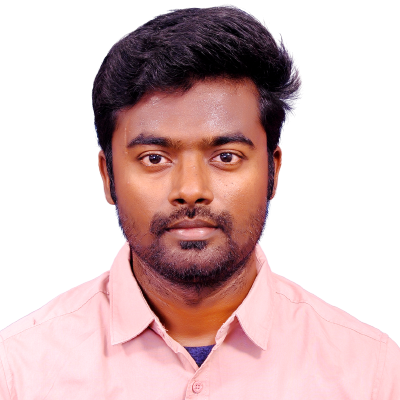
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getMilliseconds() Methods), please comment here. I will help you immediately.