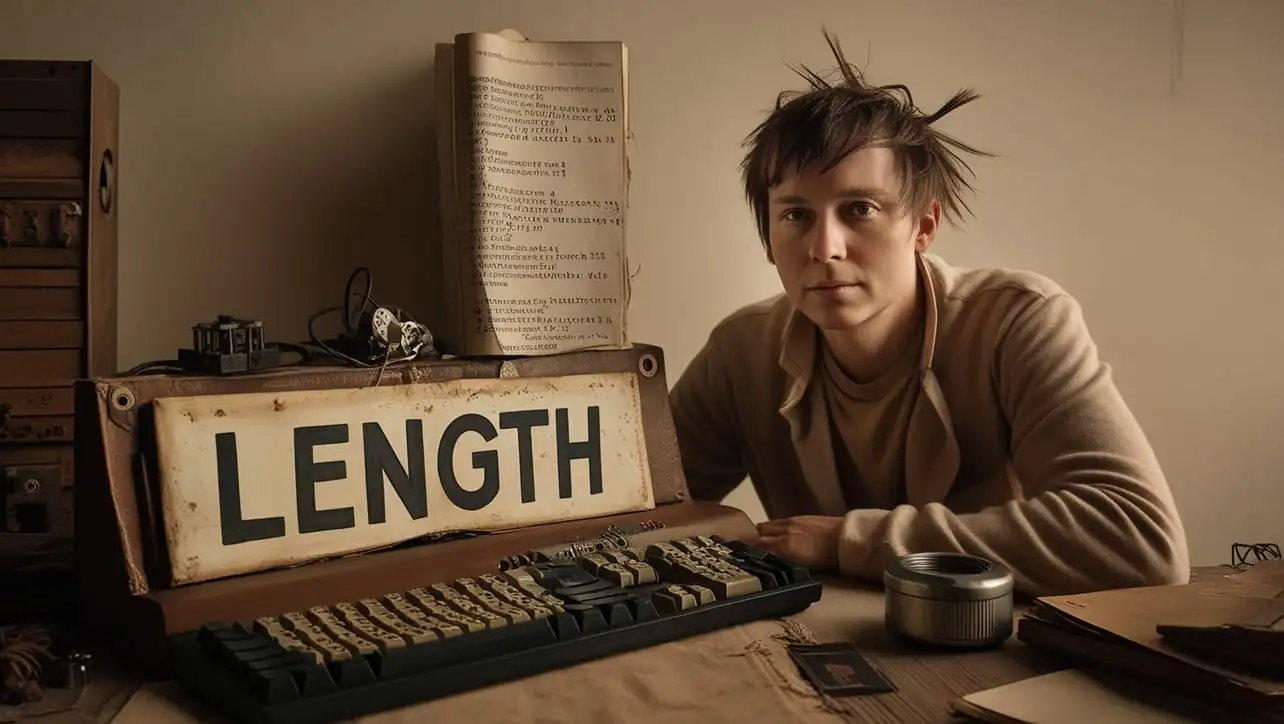
JS Date Methods
JavaScript Date getHours() Methods

Photo Credit to CodeToFun
🙋 Introduction
Working with dates and times is a common task in programming, and JavaScript provides a robust set of methods to handle these scenarios. One such method is getHours()
, which belongs to the Date object.
In this guide, we'll explore the getHours()
method, understand its syntax, and dive into practical examples and use cases.
🧠 Understanding getHours() Method
The getHours()
method is used to retrieve the hour of the day from a Date object. This method returns an integer between 0 and 23, representing the hours in the local time zone.
💡 Syntax
The syntax for the getHours()
method is straightforward:
date.getHours();
- date: The Date object from which you want to retrieve the hour.
📝 Example
Let's delve into a basic example to illustrate the usage of the getHours()
method:
// Create a new Date object for the current date and time
const currentDate = new Date();
// Get the current hour using getHours()
const currentHour = currentDate.getHours();
console.log(`The current hour is: ${currentHour}`);
In this example, we create a Date object representing the current date and time, and then use getHours()
to obtain the current hour.
🏆 Best Practices
When working with the getHours()
method, consider the following best practices:
Timezone Consideration:
Be aware that
getHours()
returns the hour in the local time zone. If working with different time zones, consider adjusting accordingly.example.jsCopied// Get the UTC hour using getUTCHours() const utcHour = currentDate.getUTCHours(); console.log(`The current UTC hour is: ${utcHour}`);
Error Handling:
Always check the validity of the Date object, especially when working with user inputs.
example.jsCopiedconst invalidDate = new Date('invalid'); if (!isNaN(invalidDate.getTime())) { const hour = invalidDate.getHours(); console.log(`The hour is: ${hour}`); } else { console.error('Invalid date provided.'); }
📚 Use Cases
Displaying a Greeting Message Based on Time:
The
getHours()
method is handy for implementing time-dependent features, such as displaying a different greeting message based on the time of day:example.jsCopiedconst hour = currentDate.getHours(); if (hour < 12) { console.log('Good morning!'); } else if (hour < 18) { console.log('Good afternoon!'); } else { console.log('Good evening!'); }
Scheduling Tasks:
When building scheduling functionalities, you can use
getHours()
to check if it's the right time to trigger a specific task:example.jsCopiedconst scheduledHour = 15; // 3:00 PM const currentHour = currentDate.getHours(); if (currentHour === scheduledHour) { console.log('Task scheduled for 3:00 PM is now running.'); } else { console.log('No task scheduled at the current hour.'); }
🎉 Conclusion
The getHours()
method is a valuable tool for working with dates and times in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getHours()
method in your JavaScript projects.
👨💻 Join our Community:
Author
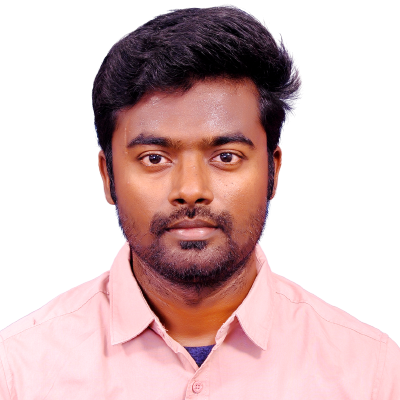
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getHours() Methods), please comment here. I will help you immediately.