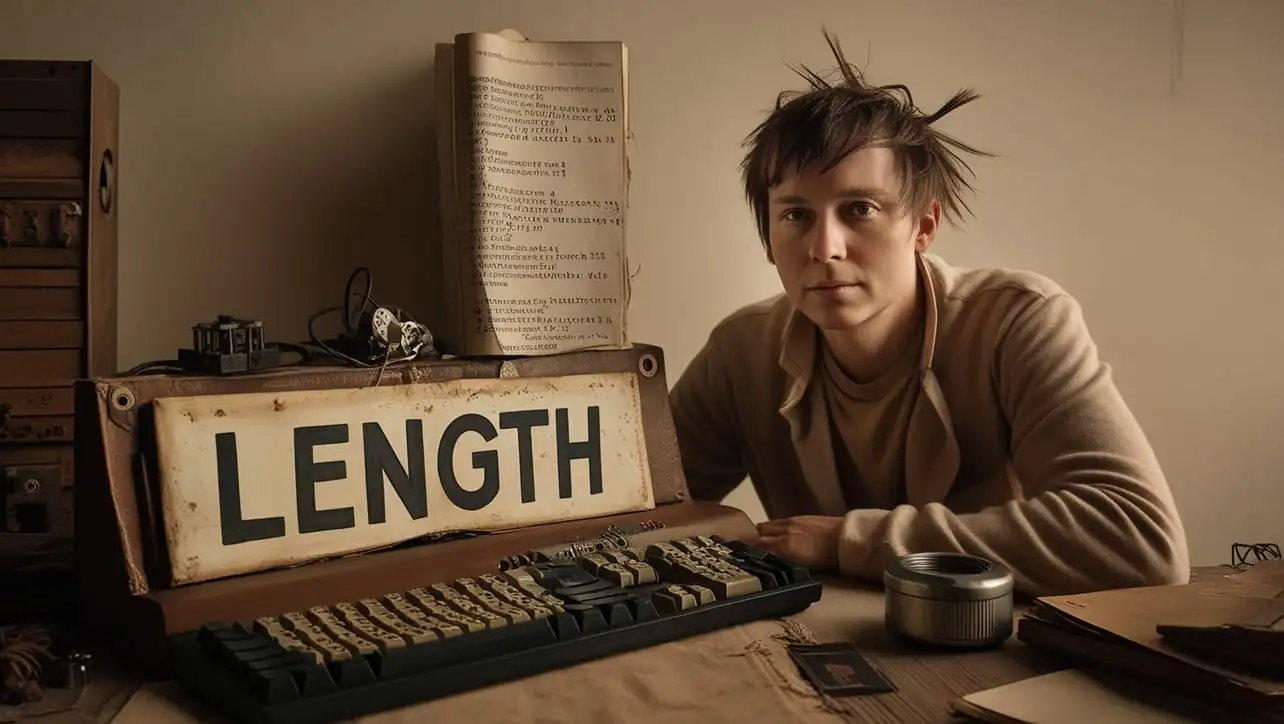
JS Date Methods
JavaScript Date getFullYear() Methods

Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, the Date object empowers developers to work with dates and times. One of the essential methods within this object is getFullYear()
, which provides an elegant way to retrieve the year from a date.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical applications of the getFullYear()
method.
🧠 Understanding getFullYear() Method
The getFullYear()
method is part of the Date object and is specifically designed to extract the four-digit year from a date instance.
💡 Syntax
The syntax for the getFullYear()
method is straightforward:
dateObject.getFullYear();
- dateObject: The Date object from which you want to obtain the year.
📝 Example
Let's dive into a practical example to illustrate the usage of the getFullYear()
method:
// Creating a new Date object
const currentDate = new Date();
// Using getFullYear() to retrieve the year
const currentYear = currentDate.getFullYear();
console.log(`Current year: ${currentYear}`);
In this example, we instantiate a new Date object representing the current date and then use getFullYear()
to obtain the current year.
🏆 Best Practices
When working with the getFullYear()
method, consider the following best practices:
Store the Result:
Since calling
getFullYear()
can be computationally expensive, especially when performed multiple times, consider storing the result in a variable if you need to reference it multiple times.example.jsCopiedconst currentYear = currentDate.getFullYear(); // Use currentYear multiple times instead of calling getFullYear() repeatedly
Error Handling:
When dealing with dynamic date values, ensure proper error handling to handle cases where the date might be invalid or undefined.
example.jsCopiedconst potentiallyInvalidDate = new Date('invalid date'); if (!isNaN(potentiallyInvalidDate)) { const year = potentiallyInvalidDate.getFullYear(); console.log(`Year: ${year}`); } else { console.error('Invalid date provided.'); }
📚 Use Cases
Displaying Copyright Year in a Footer:
One common use case for
getFullYear()
is in setting the copyright year dynamically in a website footer:example.jsCopiedconst copyrightYearElement = document.getElementById('copyrightYear'); const currentYear = new Date().getFullYear(); copyrightYearElement.textContent = currentYear;
Age Calculation:
Calculate a person's age based on their birthdate using the
getFullYear()
method:example.jsCopiedconst birthDate = new Date('1990-05-15'); const currentYear = new Date().getFullYear(); const age = currentYear - birthDate.getFullYear(); console.log(`Age: ${age} years`);
🎉 Conclusion
The getFullYear()
method is a valuable tool for extracting the year from a Date object in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getFullYear()
method in your JavaScript projects.
👨💻 Join our Community:
Author
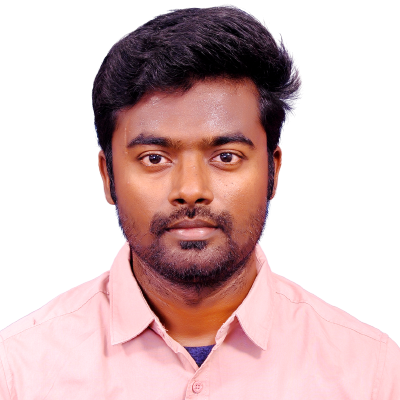
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getFullYear() Methods), please comment here. I will help you immediately.