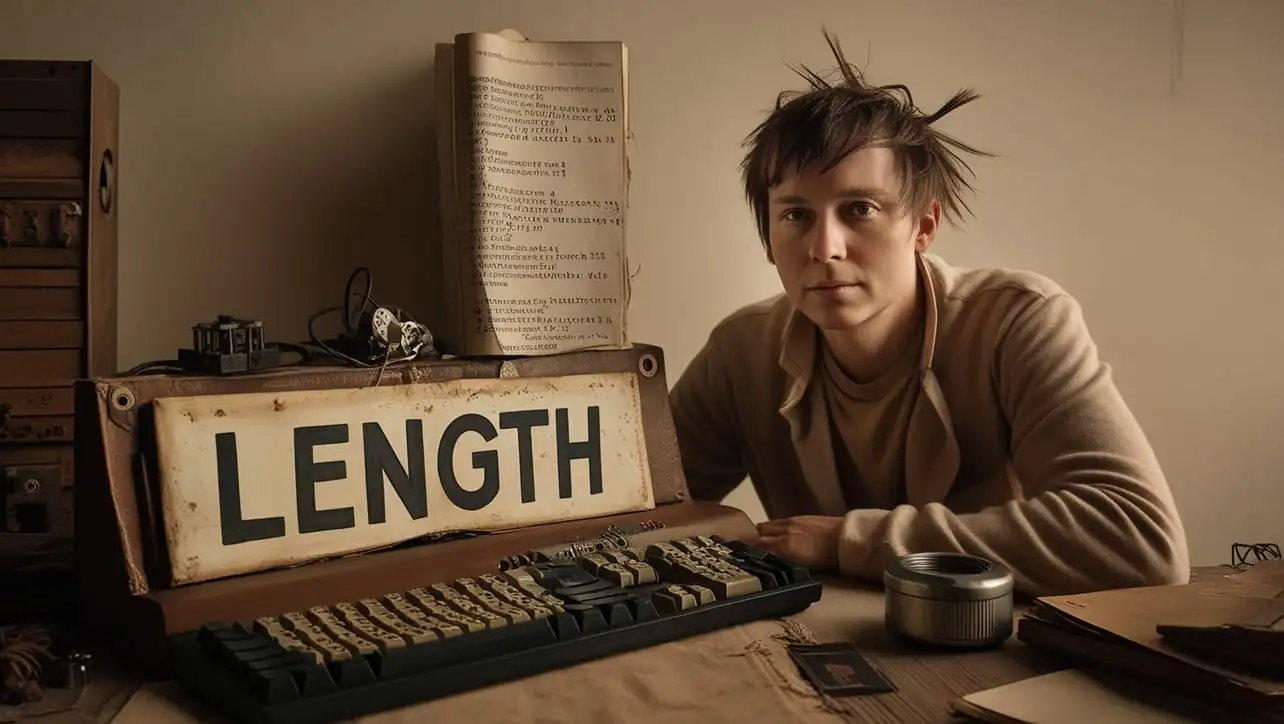
JS Window Methods
JavaScript Window scrollTo() Method

Photo Credit to CodeToFun
🙋 Introduction
In web development, controlling the scroll position of the window is a common requirement for creating smooth user experiences. The scrollTo()
method in JavaScript provides a straightforward way to achieve this.
In this guide, we'll explore the scrollTo()
method, its syntax, best practices, and practical use cases.
🧠 Understanding scrollTo() Method
The scrollTo()
method is part of the Window interface in JavaScript and is used to smoothly scroll to a specified set of coordinates within the document. It allows you to control both horizontal and vertical scrolling.
💡 Syntax
The syntax for the scrollTo()
method is straightforward:
window.scrollTo(x, y);
- x: The horizontal pixel value to scroll to.
- y: The vertical pixel value to scroll to.
📝 Example
Let's delve into a basic example to illustrate the usage of the scrollTo()
method:
// Scroll to coordinates (500, 0)
window.scrollTo(500, 0);
In this example, the scrollTo()
method is used to scroll to the horizontal coordinate 500 and the vertical coordinate 0.
🏆 Best Practices
When working with the scrollTo()
method, consider the following best practices:
Smooth Scrolling:
To achieve a smooth scrolling effect, use the behavior option with a value of "smooth".
example.jsCopied// Smoothly scroll to coordinates (500, 0) window.scrollTo({ top: 0, left: 500, behavior: 'smooth' });
Compatibility Checking:
Verify the compatibility of the
scrollTo()
method in your target environments, especially if supporting older browsers.example.jsCopied// Check if the scrollTo() method is supported if ('scrollTo' in window) { // Use the method safely window.scrollTo(500, 0); } else { console.error('scrollTo() method not supported in this environment.'); }
📚 Use Cases
Scroll to a Specific Element:
The
scrollTo()
method can be used to scroll to a specific element within the document:example.jsCopiedconst targetElement = document.getElementById('targetElement'); window.scrollTo({ top: targetElement.offsetTop, left: targetElement.offsetLeft, behavior: 'smooth' });
Scroll on Button Click:
Trigger a smooth scroll when a button is clicked:
example.jsCopiedconst scrollButton = document.getElementById('scrollButton'); scrollButton.addEventListener('click', () => { window.scrollTo({ top: 0, left: 0, behavior: 'smooth' }); });
🎉 Conclusion
The scrollTo()
method empowers developers to take control of the window's scroll position, providing a seamless way to enhance user navigation.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the scrollTo()
method in your JavaScript projects.
👨💻 Join our Community:
Author
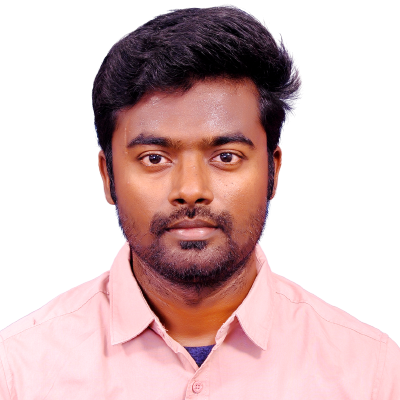
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window scrollTo() Method), please comment here. I will help you immediately.