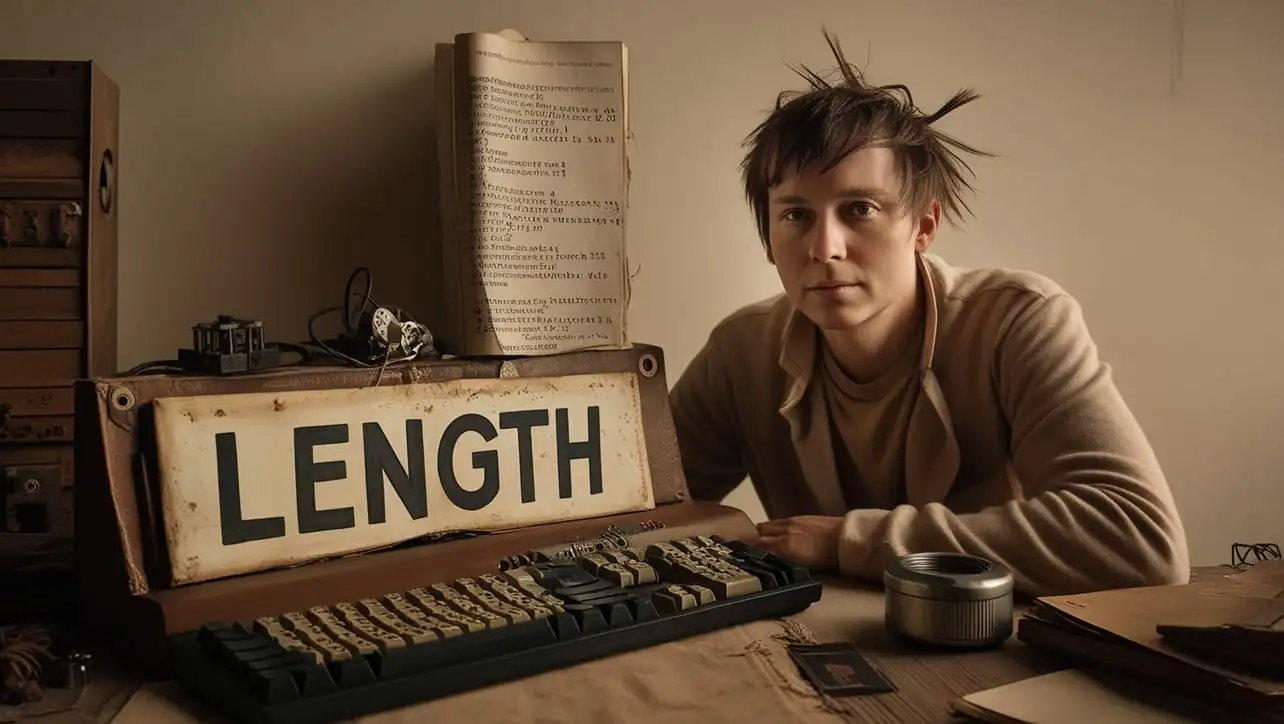
JS Window Methods
JavaScript Window resizeBy() Method

Photo Credit to CodeToFun
🙋 Introduction
The resizeBy()
method is a valuable feature in JavaScript, specifically designed for manipulating the dimensions of a browser window. This method provides a simple and effective way to resize a window by a specified amount.
In this guide, we'll explore the syntax, usage, best practices, and practical examples of the resizeBy()
method.
🧠 Understanding resizeBy() Method
The resizeBy()
method is used to change the size of the current window by a specified width and height. It's an essential tool for web developers who want to control the dimensions of a window dynamically.
💡 Syntax
The syntax for the resizeBy()
method is straightforward:
window.resizeBy(width, height);
- width: The amount by which the window's width should be resized.
- height: The amount by which the window's height should be resized.
📝 Example
Let's dive into a simple example to illustrate the usage of the resizeBy()
method:
// Resize the window by 200 pixels in width and 100 pixels in height
window.resizeBy(200, 100);
In this example, the window is resized by adding 200 pixels to its width and 100 pixels to its height.
🏆 Best Practices
When working with the resizeBy()
method, consider the following best practices:
Check Window Size:
Before using
resizeBy()
, check the current size of the window to ensure you are resizing it appropriately.example.jsCopied// Get the current size of the window const currentWidth = window.innerWidth; const currentHeight = window.innerHeight; // Resize the window based on the current size window.resizeBy(currentWidth + 100, currentHeight - 50);
Avoid Negative Values:
Be cautious when using negative values, as they can lead to unexpected results or errors.
example.jsCopied// Resize the window by a negative value (avoid doing this) window.resizeBy(-200, -100);
📚 Use Cases
Responsive Design Adjustment:
Use the
resizeBy()
method to create a responsive design that adjusts the window size based on certain conditions:example.jsCopied// Check if the window is smaller than a specific size if (window.innerWidth < 800) { // Resize the window to a mobile-friendly size window.resizeBy(-200, -100); }
Interactive UI Element:
Implement an interactive UI element that resizes the window when a button is clicked:
example.jsCopied// HTML: <button onclick="resizeWindow()">Resize Window</button> function resizeWindow() { // Resize the window by 300 pixels in width and 150 pixels in height window.resizeBy(300, 150); }
🎉 Conclusion
The resizeBy()
method empowers developers to dynamically adjust the dimensions of a browser window, offering flexibility in creating responsive and interactive web applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the resizeBy()
method in your JavaScript projects.
👨💻 Join our Community:
Author
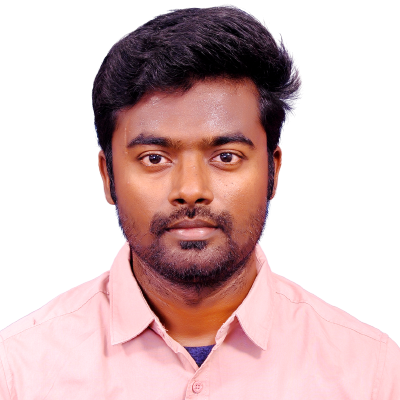
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window resizeBy() Method), please comment here. I will help you immediately.