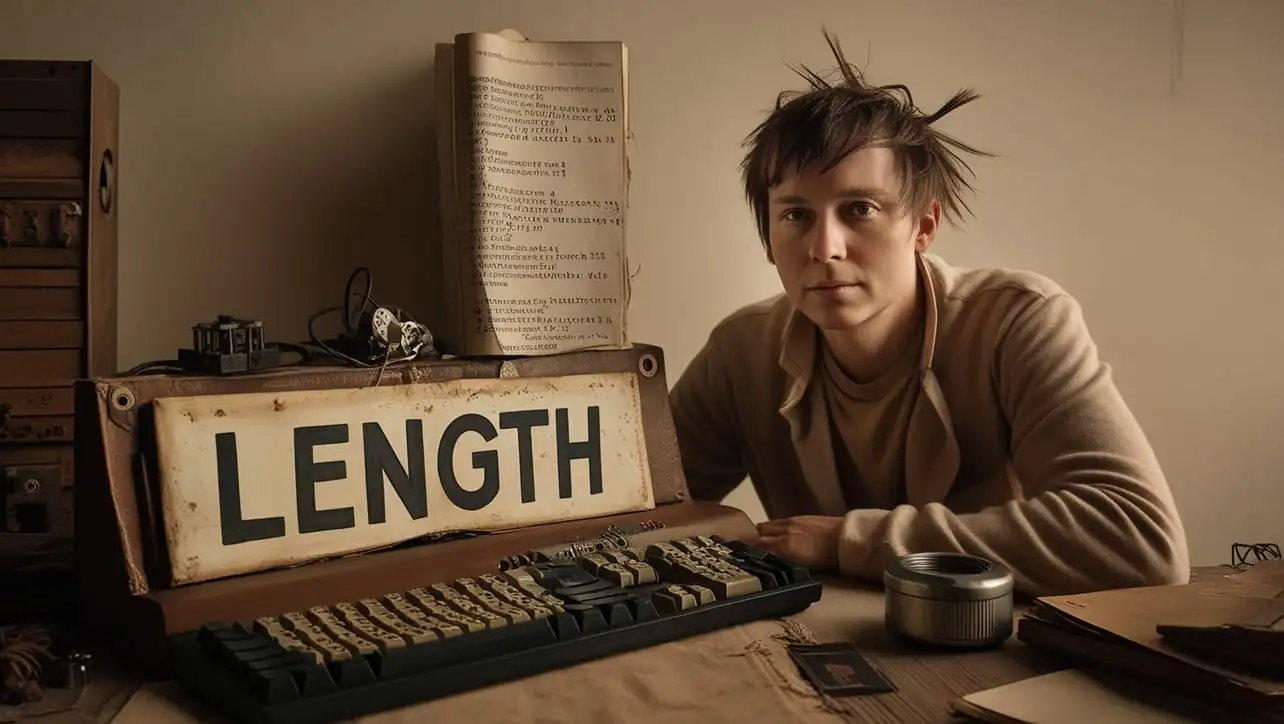
JS Window Methods
JavaScript Window clearTimeout() Method

Photo Credit to CodeToFun
🙋 Introduction
When it comes to managing asynchronous operations in JavaScript, the setTimeout() function is a common choice. However, there are situations where you might need to cancel a scheduled timeout before it executes. Enter the clearTimeout()
method, a powerful tool provided by the Window object.
In this guide, we'll explore the syntax, usage, best practices, and practical applications of the clearTimeout()
method.
🧠 Understanding clearTimeout() Method
The clearTimeout()
method is specifically designed to cancel a timeout set by the setTimeout() function. By providing the identifier returned by setTimeout(), you can prevent the associated function from executing after a certain delay.
💡 Syntax
The syntax for the clearTimeout()
method is straightforward:
clearTimeout(timeoutId);
- timeoutId: The identifier returned by the setTimeout() function that corresponds to the timeout you want to cancel.
📝 Example
Let's delve into a simple example to illustrate the usage of the clearTimeout()
method:
// Set a timeout
const delayedFunction = () => {
console.log('Delayed function executed!');
};
const timeoutId = setTimeout(delayedFunction, 2000);
// Cancel the timeout before it executes
clearTimeout(timeoutId);
In this example, we use setTimeout() to schedule the execution of delayedFunction after a delay of 2000 milliseconds. However, before it can run, we call clearTimeout()
with the timeoutId, preventing the function from being executed.
🏆 Best Practices
When working with the clearTimeout()
method, consider the following best practices:
Check for Valid Identifiers:
Before calling
clearTimeout()
, ensure that the provided timeout identifier is valid to avoid errors.example.jsCopiedif (typeof timeoutId === 'number') { clearTimeout(timeoutId); } else { console.error('Invalid timeout identifier.'); }
Clearing Only Necessary Timeouts:
Avoid unnecessary calls to
clearTimeout()
. Only use it when you genuinely need to cancel a scheduled timeout.
📚 Use Cases
User Interaction Delay:
The
clearTimeout()
method is often used to manage delays associated with user interactions. For example, you might delay the execution of a search function to allow users to finish typing, but if they continue typing, you can clear the timeout to avoid redundant searches.example.jsCopiedlet searchTimeout; const searchInput = document.getElementById('search-input'); searchInput.addEventListener('input', () => { clearTimeout(searchTimeout); searchTimeout = setTimeout(() => { // Perform search operation console.log('Searching...'); }, 500); });
Dynamic UI Updates:
When dynamically updating the UI based on user actions, you can use
clearTimeout()
to handle scenarios where multiple updates are triggered quickly.example.jsCopiedlet updateTimeout; function handleUserAction() { // Clear previous timeout clearTimeout(updateTimeout); // Set a new timeout for UI update updateTimeout = setTimeout(() => { // Update the UI based on user action console.log('UI updated!'); }, 1000); }
🎉 Conclusion
The clearTimeout()
method is an indispensable tool for managing timeouts and ensuring precise control over asynchronous operations in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the clearTimeout()
method in your JavaScript projects.
👨💻 Join our Community:
Author
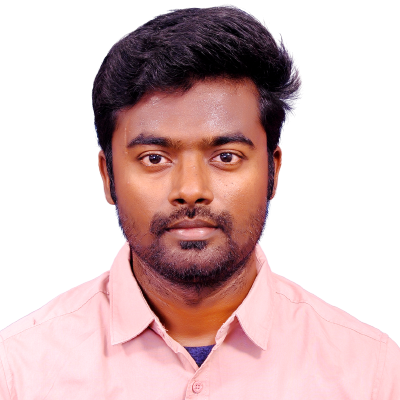
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window clearTimeout() Method), please comment here. I will help you immediately.